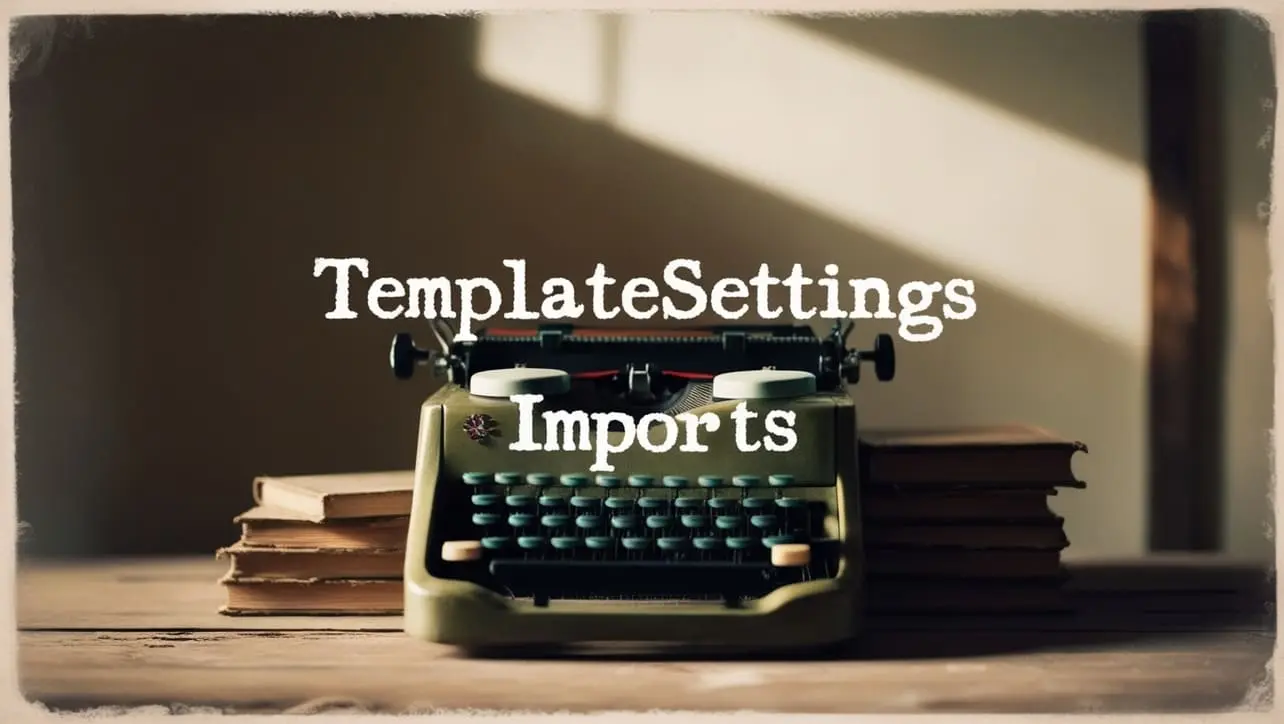
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toPairs() Object Method
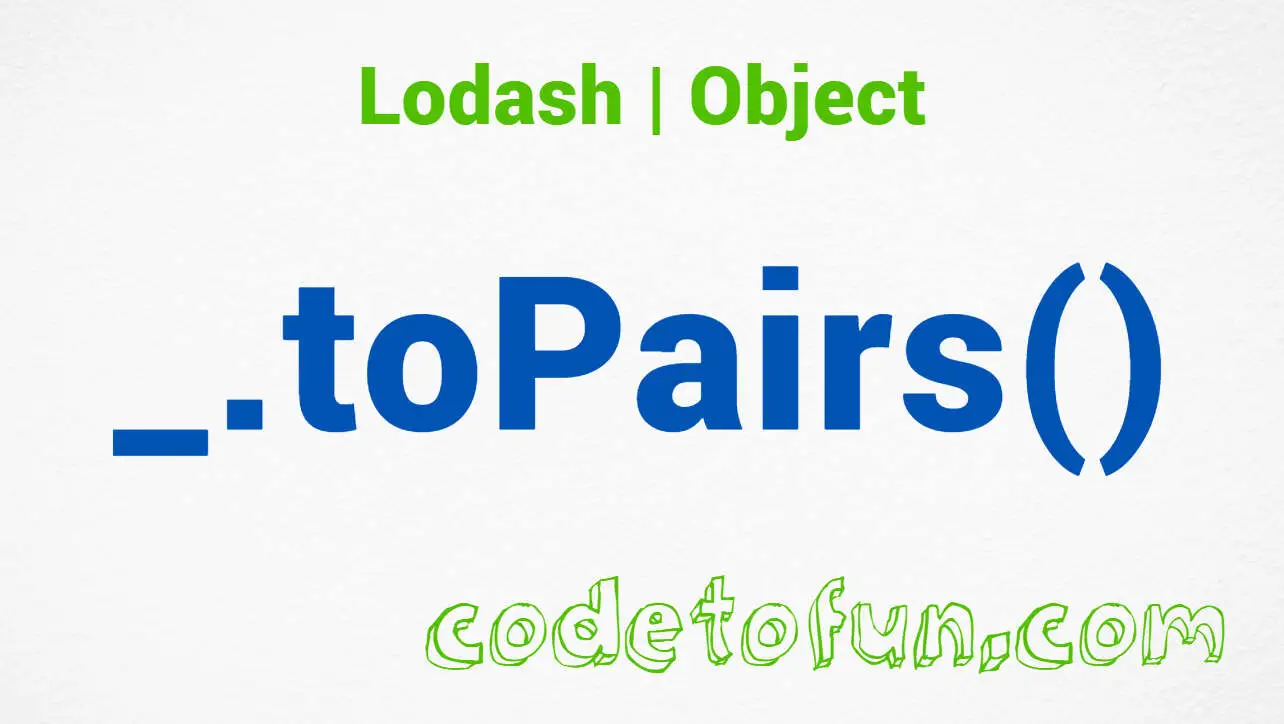
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, managing objects and their properties is a common task. Lodash, a popular utility library, provides a variety of methods to simplify object manipulation. Among these methods is _.toPairs()
, which transforms an object into an array of key-value pairs.
This method offers flexibility and convenience, making it a valuable asset for developers dealing with complex data structures.
🧠 Understanding _.toPairs() Method
The _.toPairs()
method in Lodash converts an object into an array of key-value pairs. Each pair consists of the object's property name (key) and its corresponding value. This transformation facilitates easier iteration and manipulation of object data, enabling developers to perform various operations with simplicity and efficiency.
💡 Syntax
The syntax for the _.toPairs()
method is straightforward:
_.toPairs(object)
- object: The object to convert into key-value pairs.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toPairs()
method:
const _ = require('lodash');
const myObject = { a: 1, b: 2, c: 3 };
const pairsArray = _.toPairs(myObject);
console.log(pairsArray);
// Output: [['a', 1], ['b', 2], ['c', 3]]
In this example, the myObject is transformed into an array of key-value pairs using _.toPairs()
.
🏆 Best Practices
When working with the _.toPairs()
method, consider the following best practices:
Object Property Order:
Keep in mind that the order of key-value pairs in the resulting array may not be guaranteed to match the order of properties in the original object. JavaScript objects do not guarantee property order, and
_.toPairs()
may reflect this behavior.example.jsCopiedconst myObject = { b: 2, a: 1, c: 3 }; const pairsArray = _.toPairs(myObject); console.log(pairsArray); // Output: [['b', 2], ['a', 1], ['c', 3]]
Nested Objects:
When dealing with nested objects,
_.toPairs()
operates recursively, converting nested objects into arrays of key-value pairs as well. This behavior allows for convenient handling of complex data structures.example.jsCopiedconst nestedObject = { a: { nestedProp: 1 }, b: 2 }; const nestedPairsArray = _.toPairs(nestedObject); console.log(nestedPairsArray); // Output: [['a', { nestedProp: 1 }], ['b', 2]]
Iteration and Transformation:
Once converted into an array of key-value pairs, you can easily iterate over the pairs using array methods like forEach() or map(). This enables seamless transformation and manipulation of object data.
example.jsCopiedconst pairsArray = [['a', 1], ['b', 2], ['c', 3]]; pairsArray.forEach(([key, value]) => { console.log(`Key: ${key}, Value: ${value}`); });
📚 Use Cases
Iterating Over Object Properties:
_.toPairs()
simplifies the process of iterating over object properties by converting them into a format conducive to array iteration. This is particularly useful when you need to perform operations on each property or extract specific information from the object.example.jsCopiedconst myObject = { name: 'John', age: 30, city: 'New York' }; _.toPairs(myObject).forEach(([key, value]) => { console.log(`${key}: ${value}`); });
Object Data Transformation:
When transforming object data for further processing or presentation,
_.toPairs()
provides a structured format that facilitates manipulation and conversion. This enables seamless integration with other data structures or serialization formats.example.jsCopiedconst userObject = { name: 'Alice', age: 25, isAdmin: true }; const transformedData = /* ...perform data transformation using _.toPairs()... */; console.log(transformedData);
Object Serialization:
For scenarios where you need to serialize object data for storage or transmission,
_.toPairs()
offers a convenient way to convert objects into a format that can be easily serialized, such as JSON.example.jsCopiedconst userData = { username: 'user123', email: 'user@example.com', password: 'securepassword' }; const serializedData = JSON.stringify(_.toPairs(userData)); console.log(serializedData);
🎉 Conclusion
The _.toPairs()
method in Lodash provides a versatile solution for converting objects into arrays of key-value pairs, facilitating easier iteration, transformation, and serialization of object data. Whether you're iterating over properties, transforming data, or serializing objects, _.toPairs()
offers a streamlined approach to object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toPairs()
method in your Lodash projects.
👨💻 Join our Community:
Author
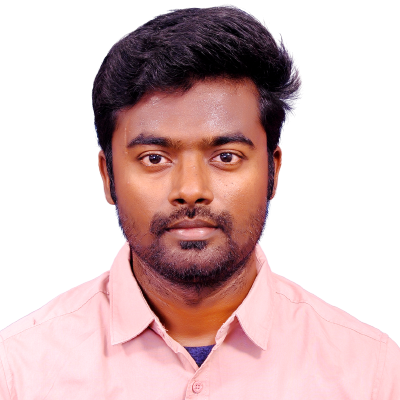
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
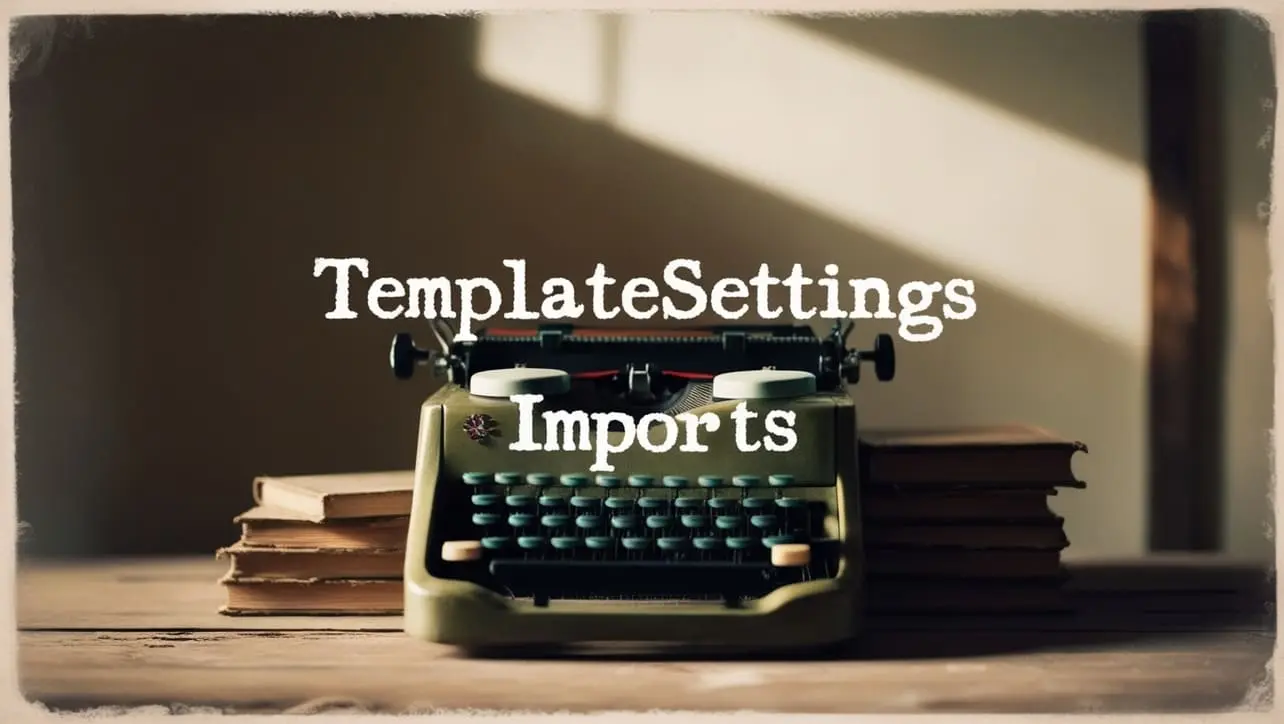
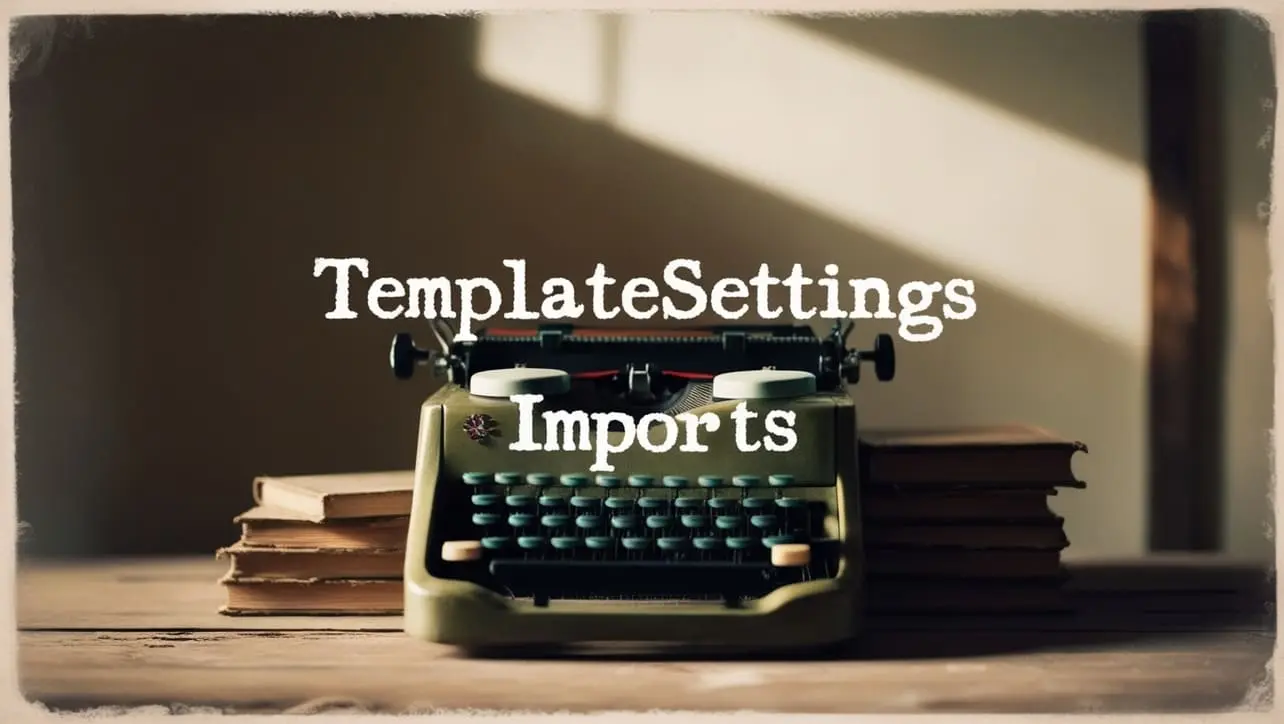
Lodash _.templateSettings.imports Property
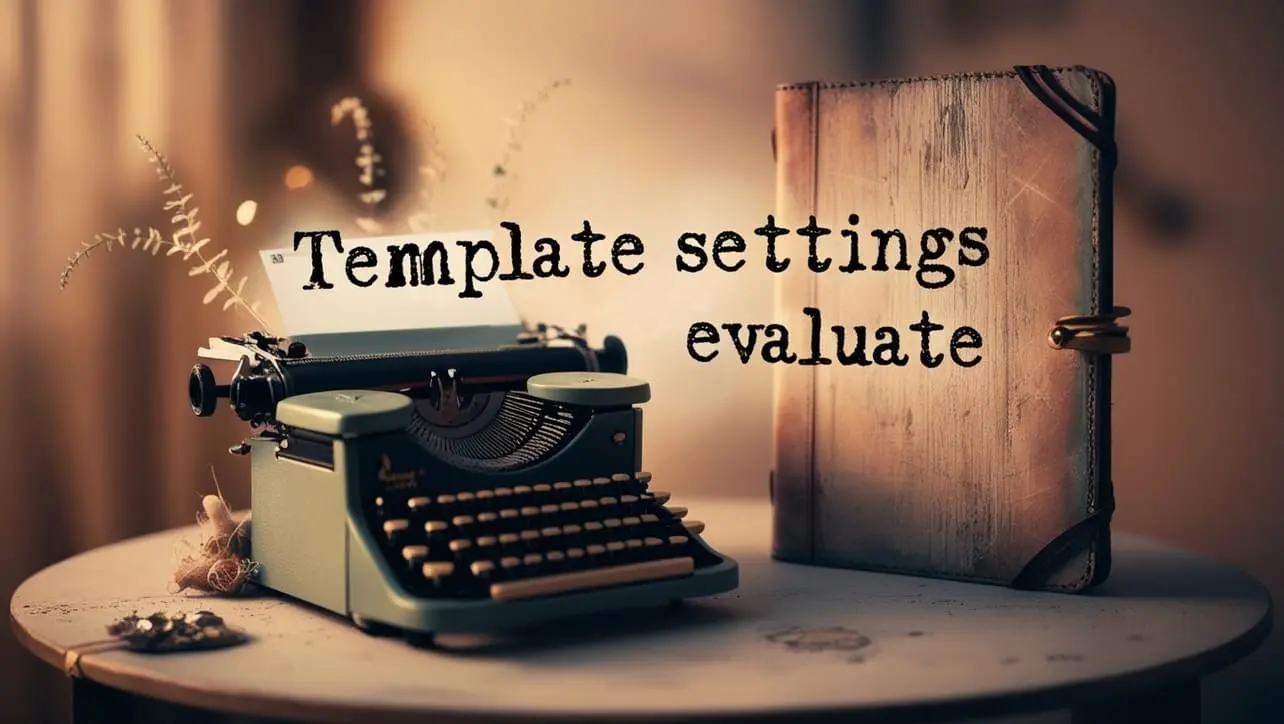
Lodash _.templateSettings.evaluate Property
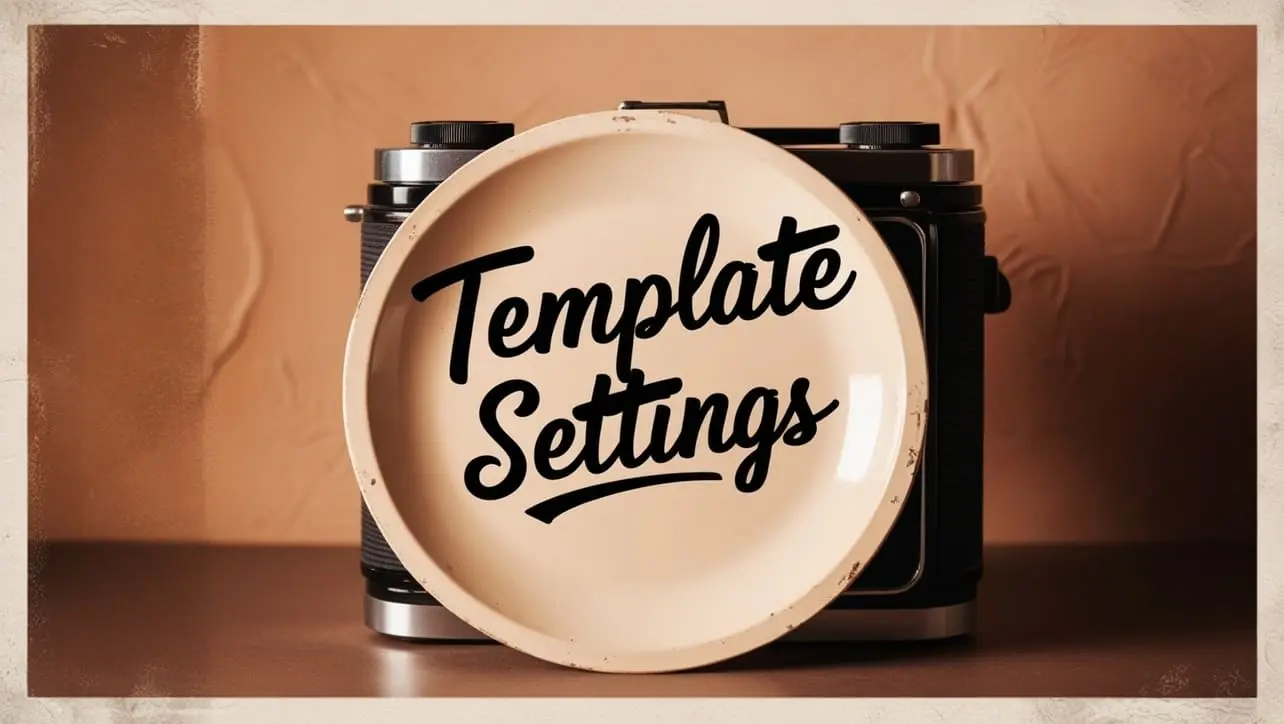
Lodash _.templateSettings Property
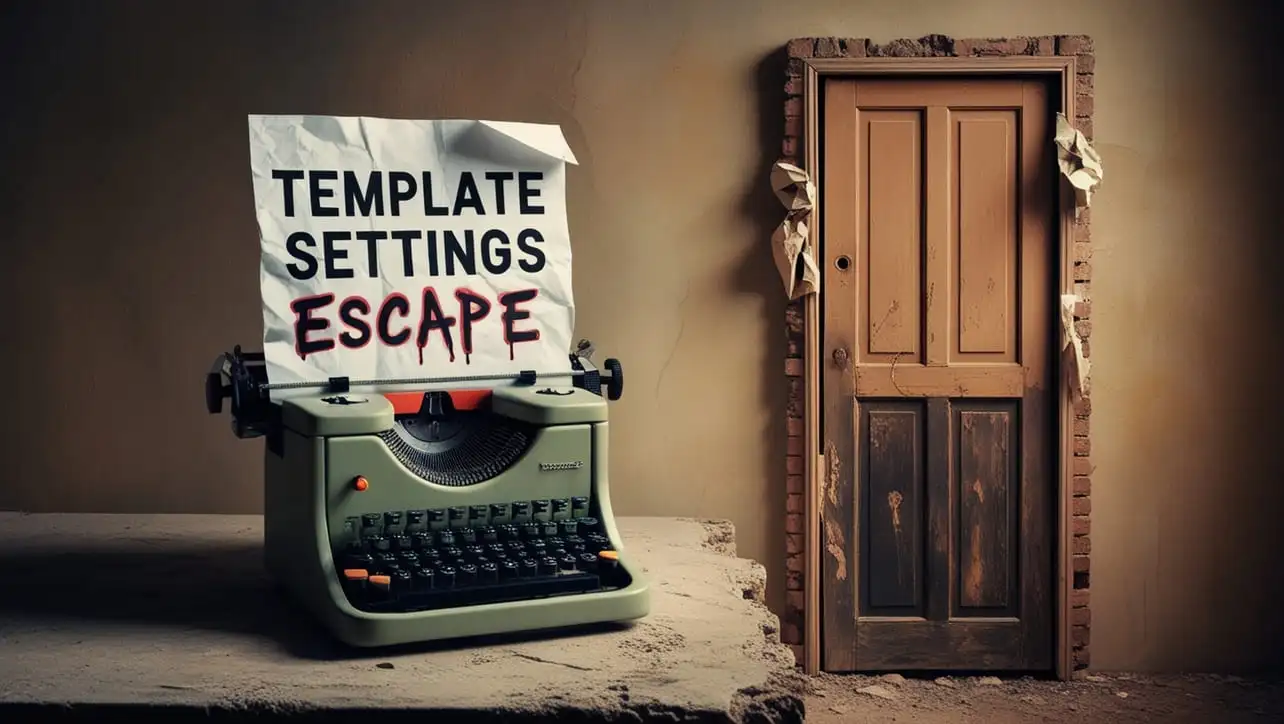
Lodash _.templateSettings.escape Property
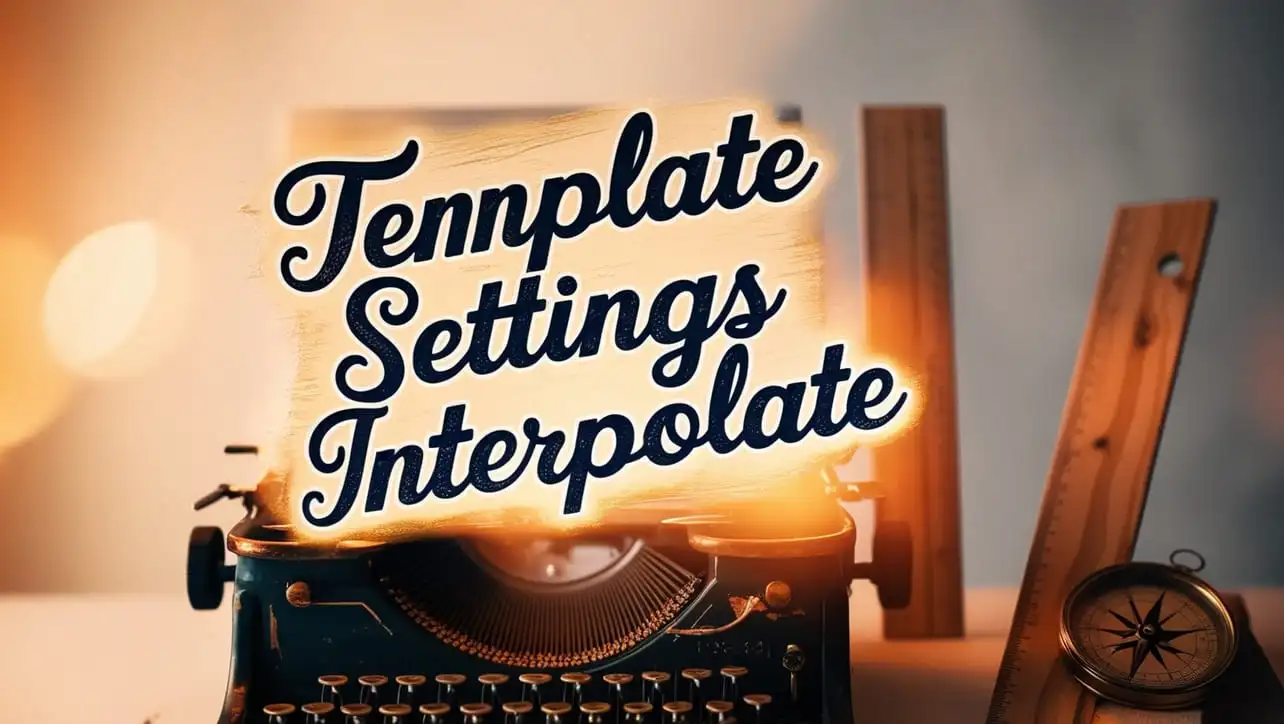
Lodash _.templateSettings.interpolate Property
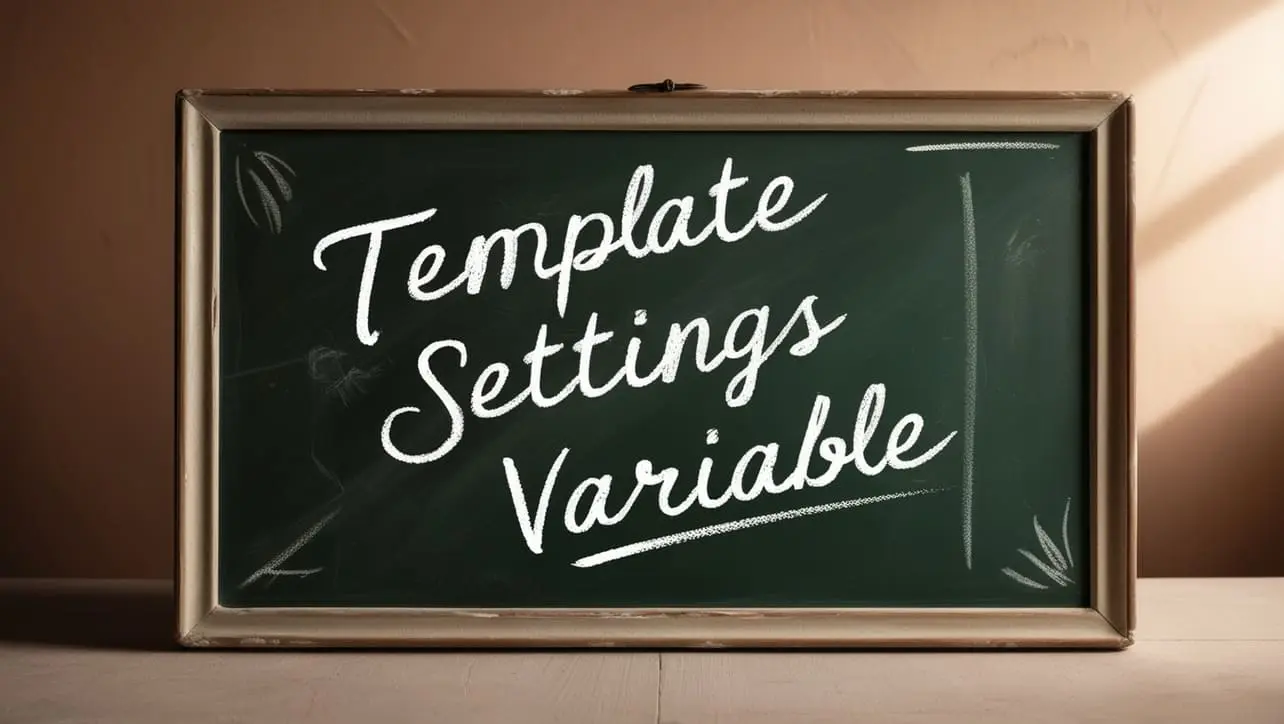
If you have any doubts regarding this article (Lodash _.toPairs() Object Method), please comment here. I will help you immediately.