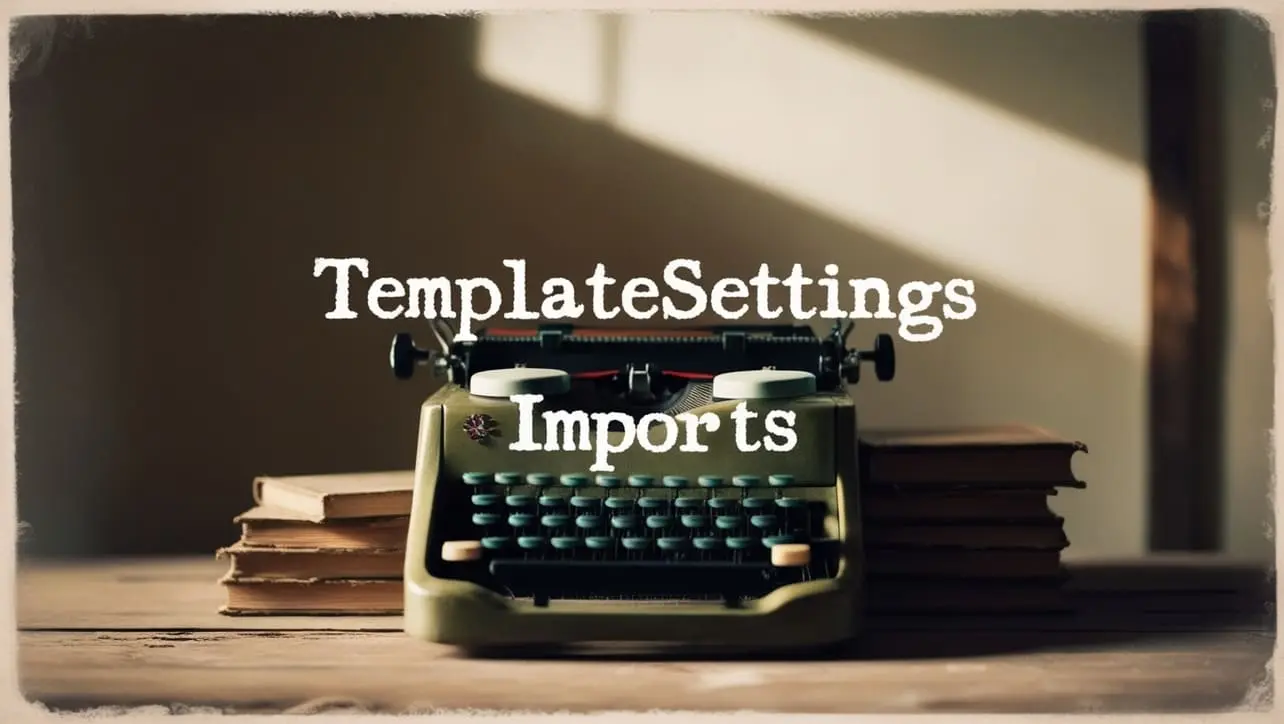
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.set() Object Method
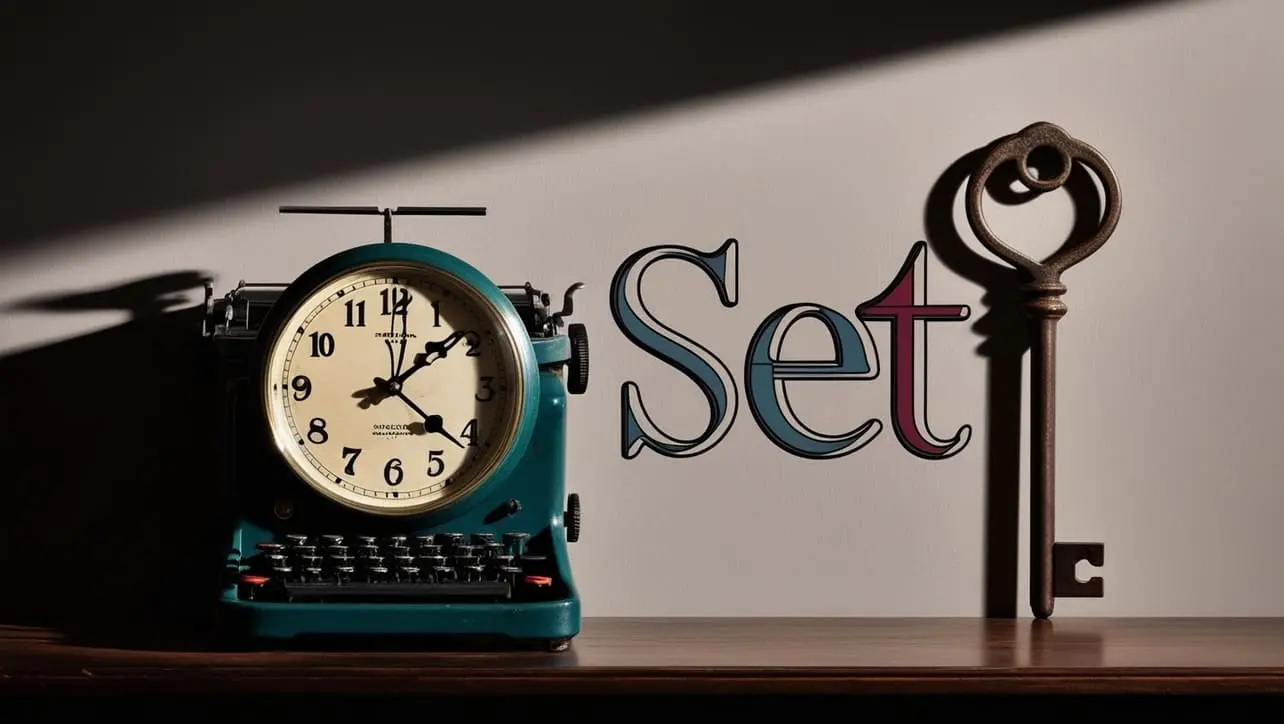
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, efficient manipulation of objects is crucial for building robust and maintainable applications. Enter Lodash, a feature-rich utility library that simplifies common programming tasks. Among its arsenal of functions lies the _.set()
method, a powerful tool for setting nested properties within JavaScript objects.
This method streamlines the process of updating complex data structures, enhancing code readability and maintainability.
🧠 Understanding _.set() Method
The _.set()
method in Lodash allows developers to set the value of a nested property within an object by providing a path and the desired value. This path can be a string or an array of keys, enabling flexible manipulation of object properties.
💡 Syntax
The syntax for the _.set()
method is straightforward:
_.set(object, path, value)
- object: The object to modify.
- path: The path of the property to set.
- value: The value to set.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.set()
method:
const _ = require('lodash');
const user = {};
_.set(user, 'profile.name', 'John Doe');
_.set(user, ['profile', 'age'], 30);
console.log(user);
// Output: { profile: { name: 'John Doe', age: 30 } }
In this example, the user object is modified using _.set()
to set nested properties for name and age within the profile object.
🏆 Best Practices
When working with the _.set()
method, consider the following best practices:
Understanding Nested Properties:
Before using
_.set()
, ensure a clear understanding of the object's structure and the path to the property you want to set. This helps prevent unintended modifications to unrelated properties.example.jsCopiedconst user = { profile: { address: { city: 'New York' } } }; _.set(user, 'profile.name', 'John Doe'); console.log(user); // Output: { profile: { address: { city: 'New York' }, name: 'John Doe' } }
Handling Non-Existent Paths:
When setting values for nested properties,
_.set()
automatically creates intermediate objects along the path if they do not exist. However, be cautious when setting values deep within nested structures to avoid unintended side effects.example.jsCopiedconst user = {}; _.set(user, 'profile.name.first', 'John'); _.set(user, 'profile.name.last', 'Doe'); console.log(user); // Output: { profile: { name: { first: 'John', last: 'Doe' } } }
Dealing with Arrays:
When dealing with array properties, use numeric indices in the path to set values within arrays.
example.jsCopiedconst data = {}; _.set(data, 'users[0].name', 'Alice'); _.set(data, 'users[1].name', 'Bob'); console.log(data); // Output: { users: [{ name: 'Alice' }, { name: 'Bob' }] }
📚 Use Cases
User Profiles:
_.set()
is particularly useful when managing user profiles with nested properties such as name, address, and contact information. This method simplifies the process of updating user details within a structured data model.example.jsCopiedconst userProfile = {}; _.set(userProfile, 'name', 'John Doe'); _.set(userProfile, 'address.city', 'New York'); _.set(userProfile, 'contact.email', 'john.doe@example.com'); console.log(userProfile);
Configuration Objects:
In applications with configuration objects containing nested properties,
_.set()
can be employed to dynamically update configuration settings based on user input or system requirements.example.jsCopiedconst config = {}; _.set(config, 'app.theme.primaryColor', '#3366FF'); _.set(config, 'app.debug', true); console.log(config);
Data Transformation:
When transforming data structures,
_.set()
facilitates the manipulation of nested properties, enabling seamless conversion between different formats or schemas.example.jsCopiedconst originalData = {}; _.set(originalData, 'user.name', 'Alice'); _.set(originalData, 'user.age', 25); const transformedData = _.set({}, 'profile', _.get(originalData, 'user')); console.log(transformedData);
🎉 Conclusion
The _.set()
method in Lodash provides a convenient and efficient solution for setting nested properties within JavaScript objects. Whether you're managing user profiles, configuring application settings, or transforming data structures, _.set()
offers a versatile tool for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.set()
method in your Lodash projects.
👨💻 Join our Community:
Author
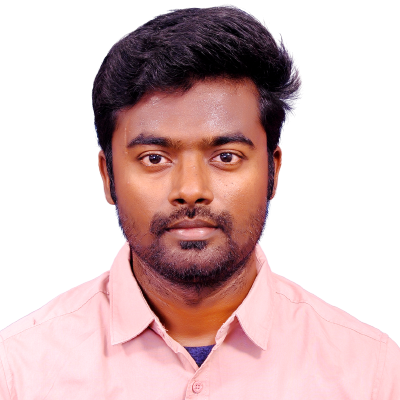
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
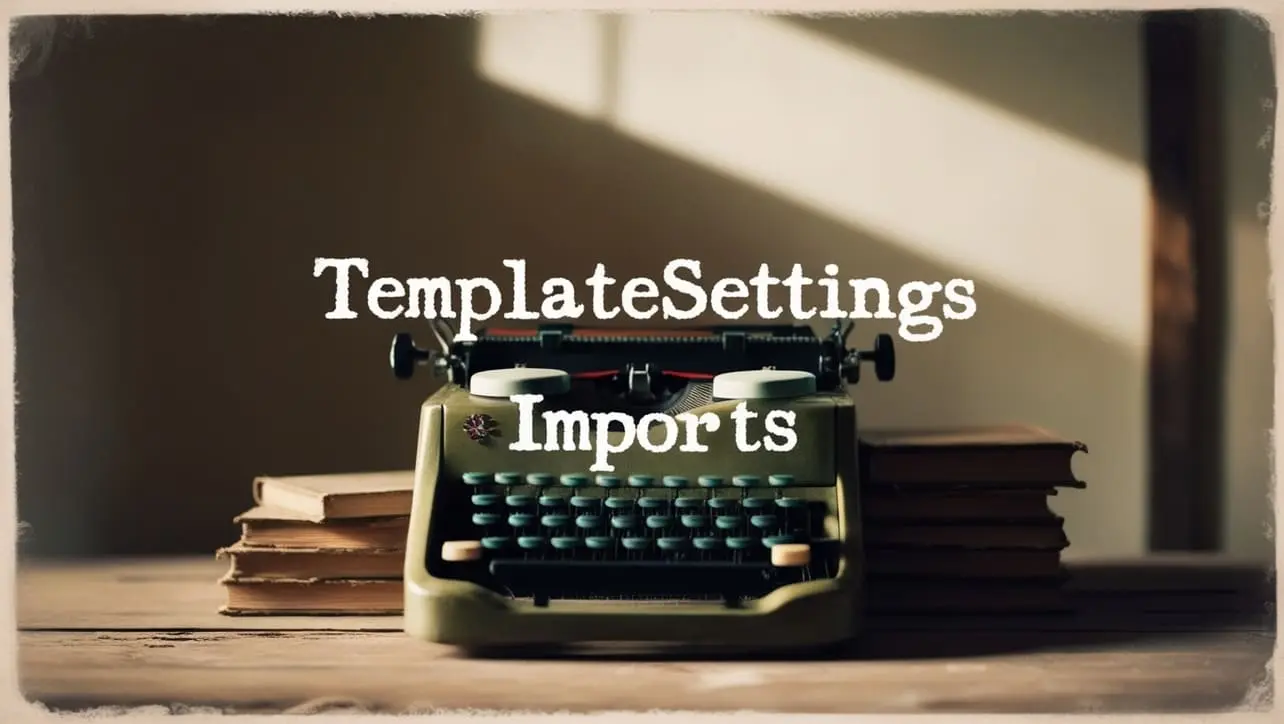
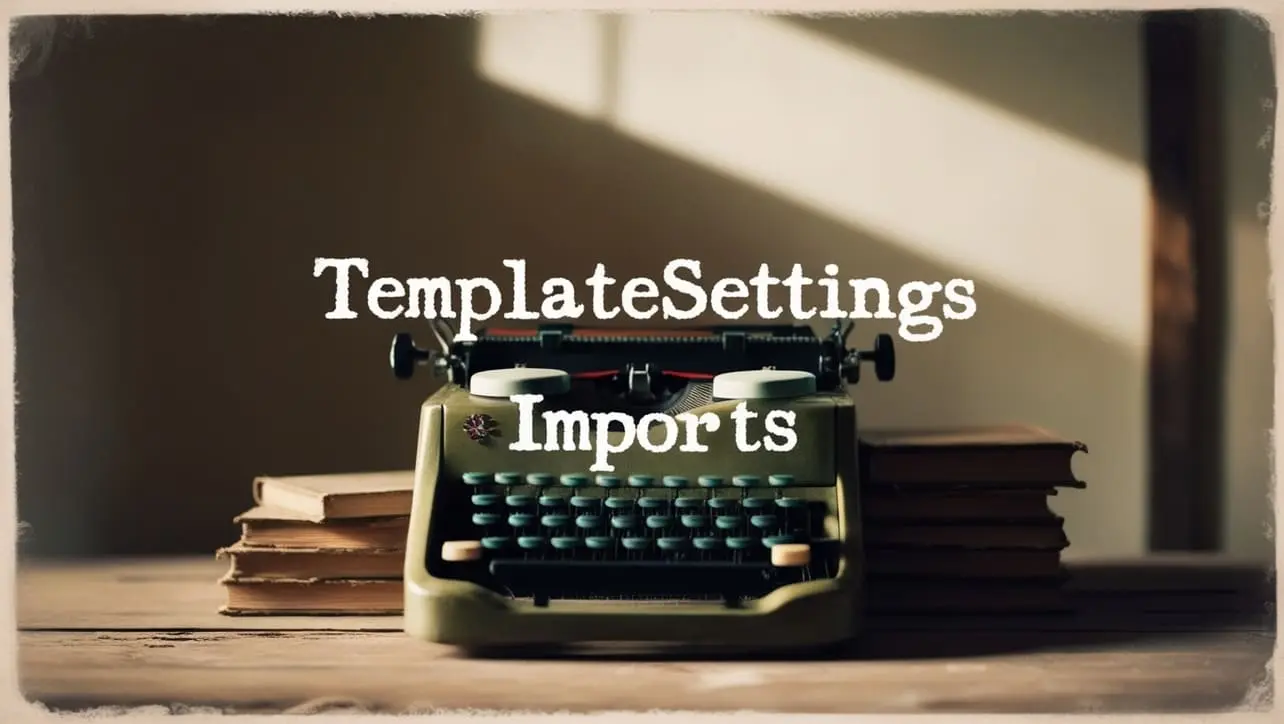
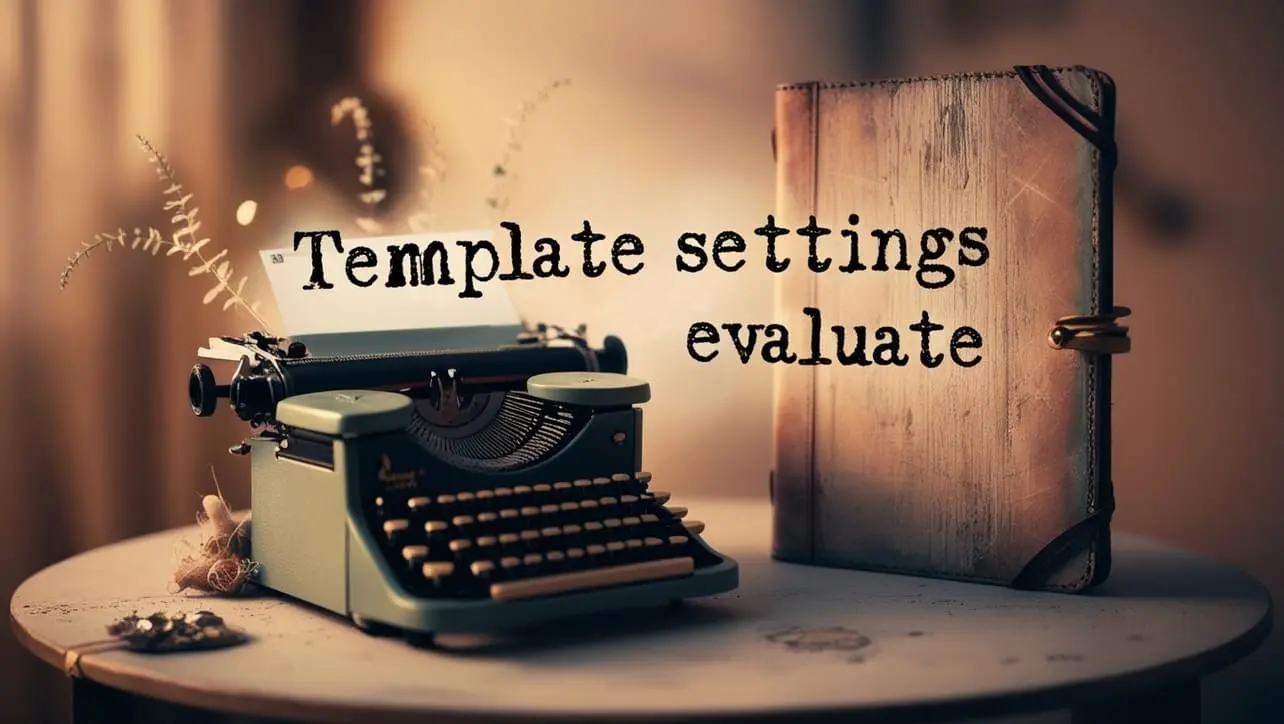
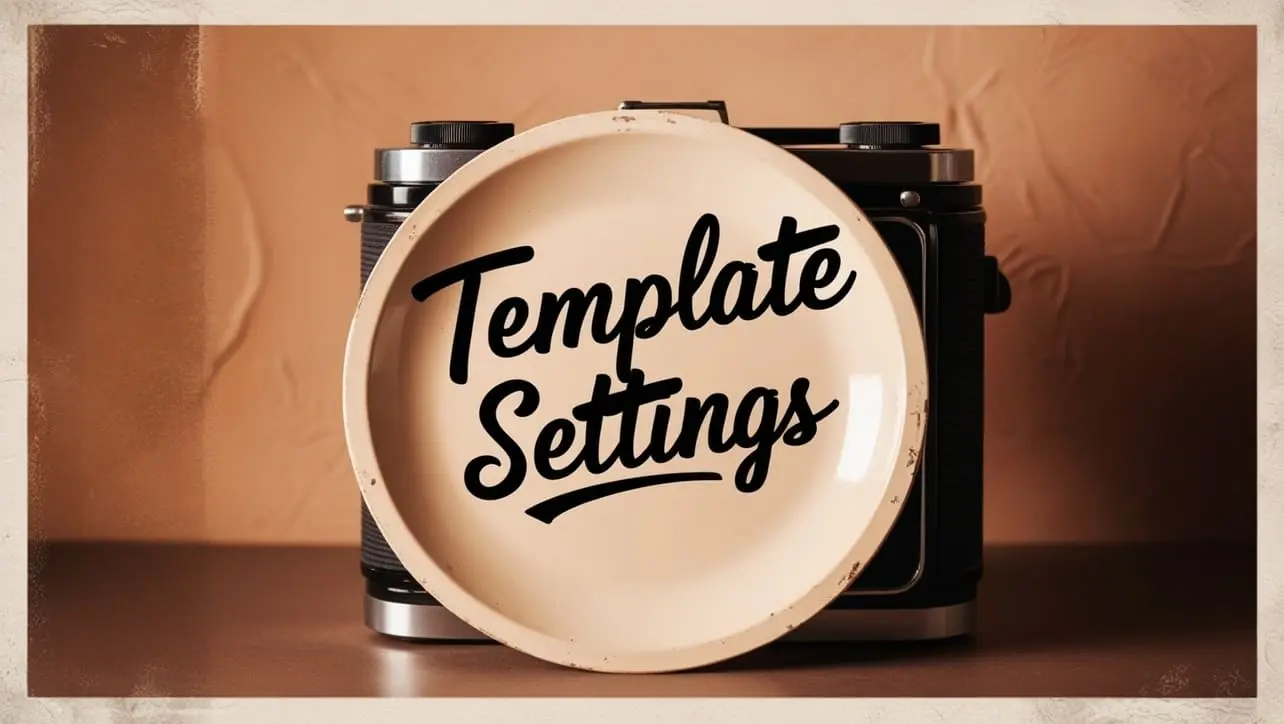
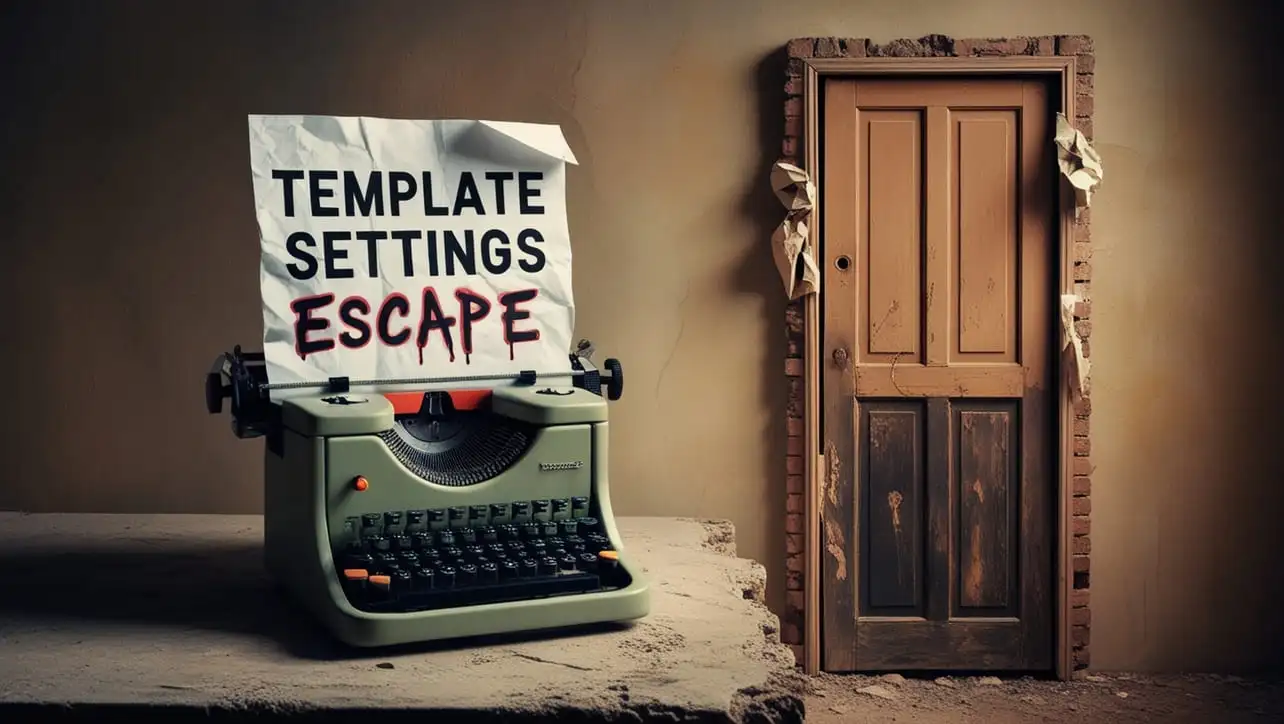
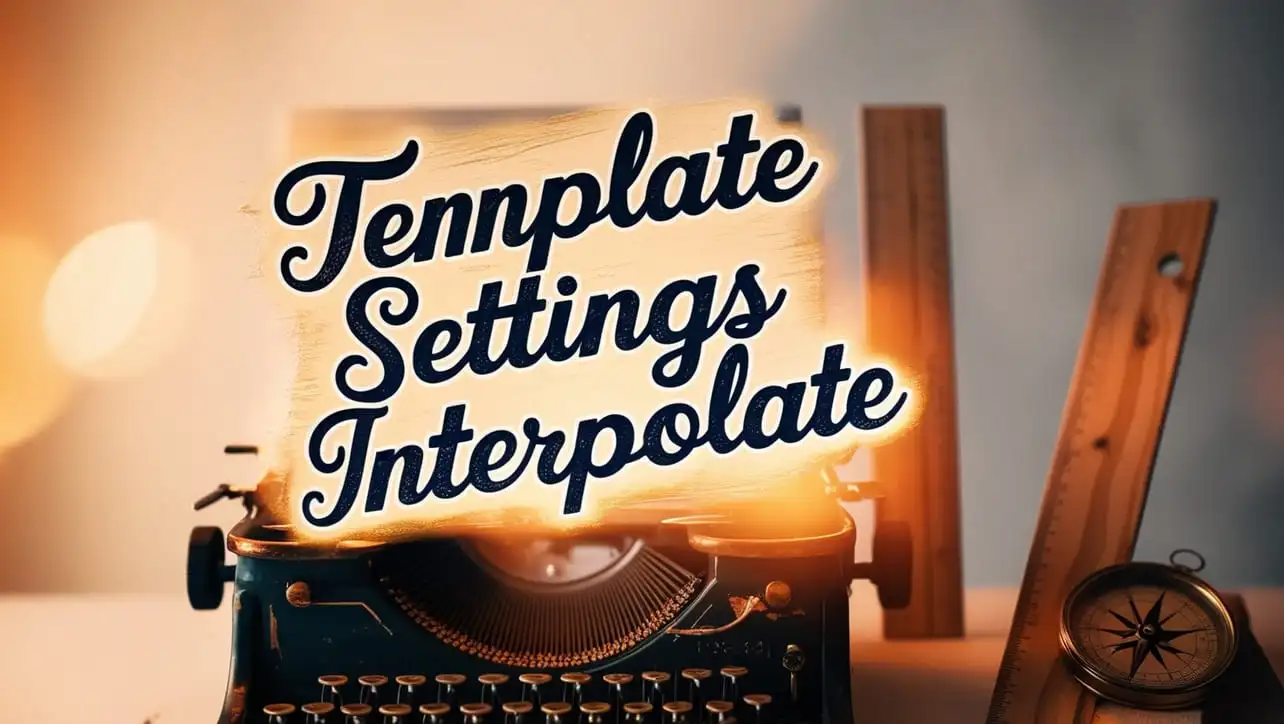
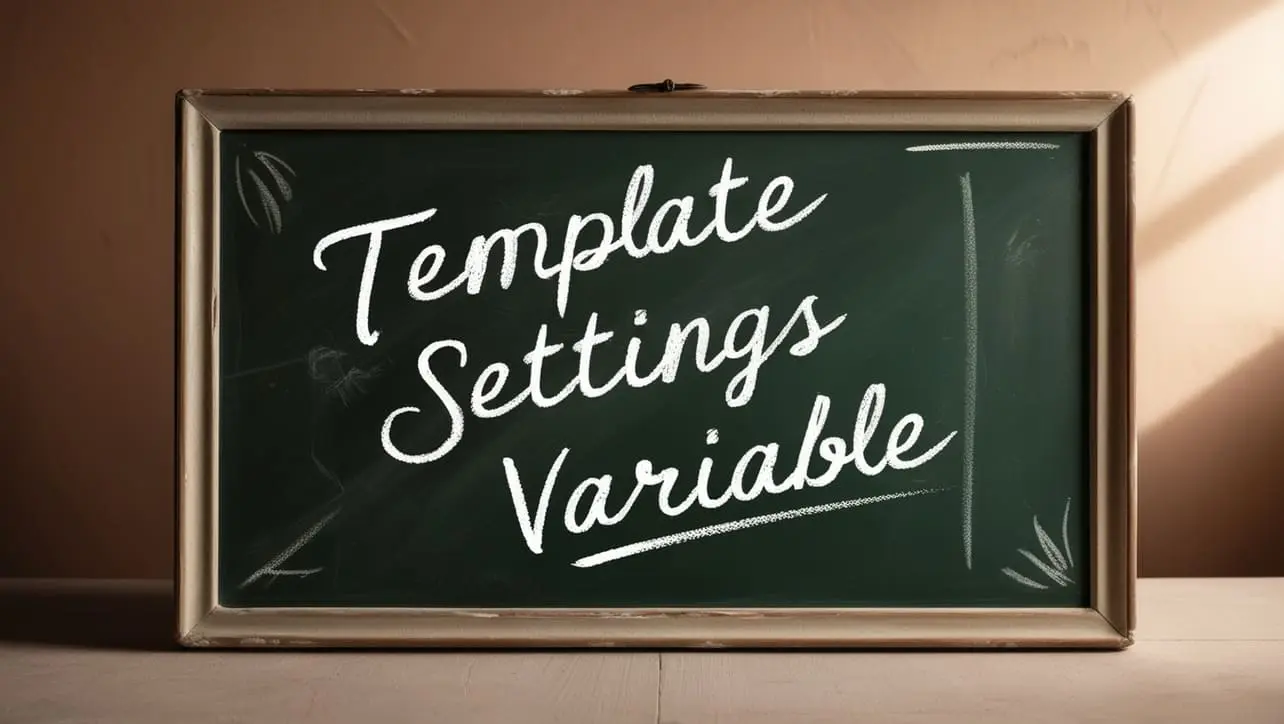
If you have any doubts regarding this article (Lodash _.set() Object Method), please comment here. I will help you immediately.