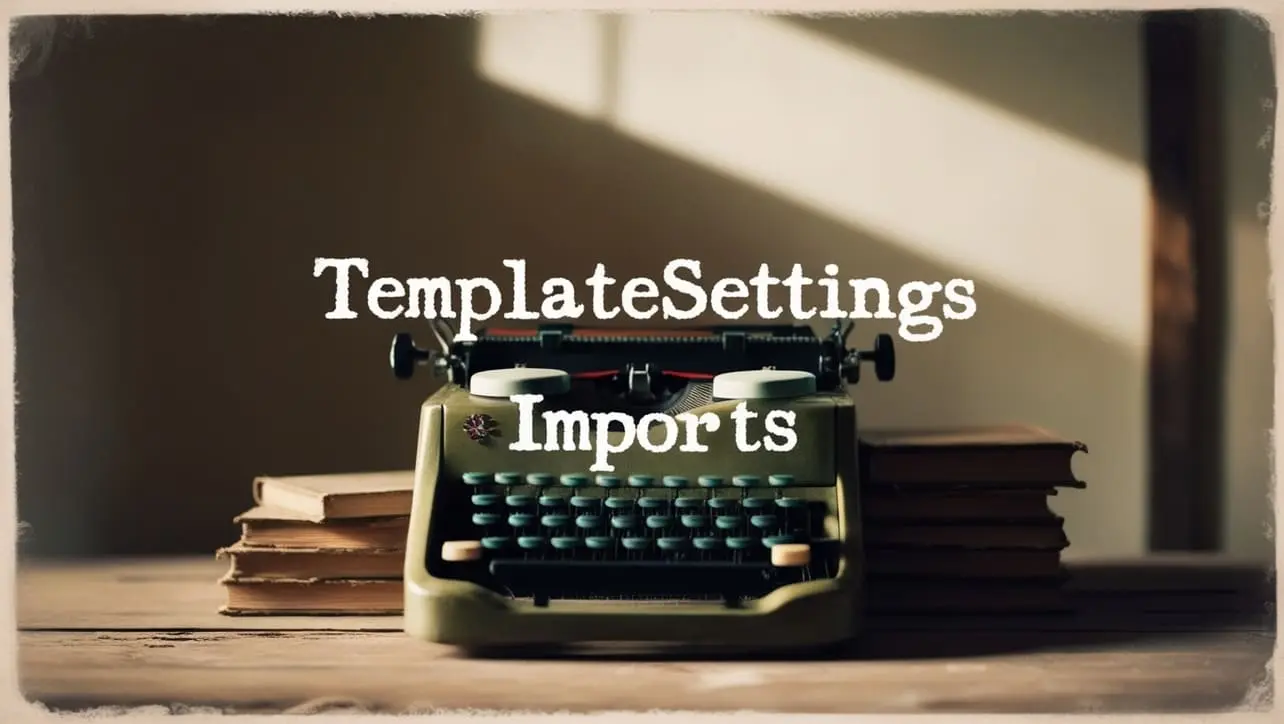
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.omit() Object Method
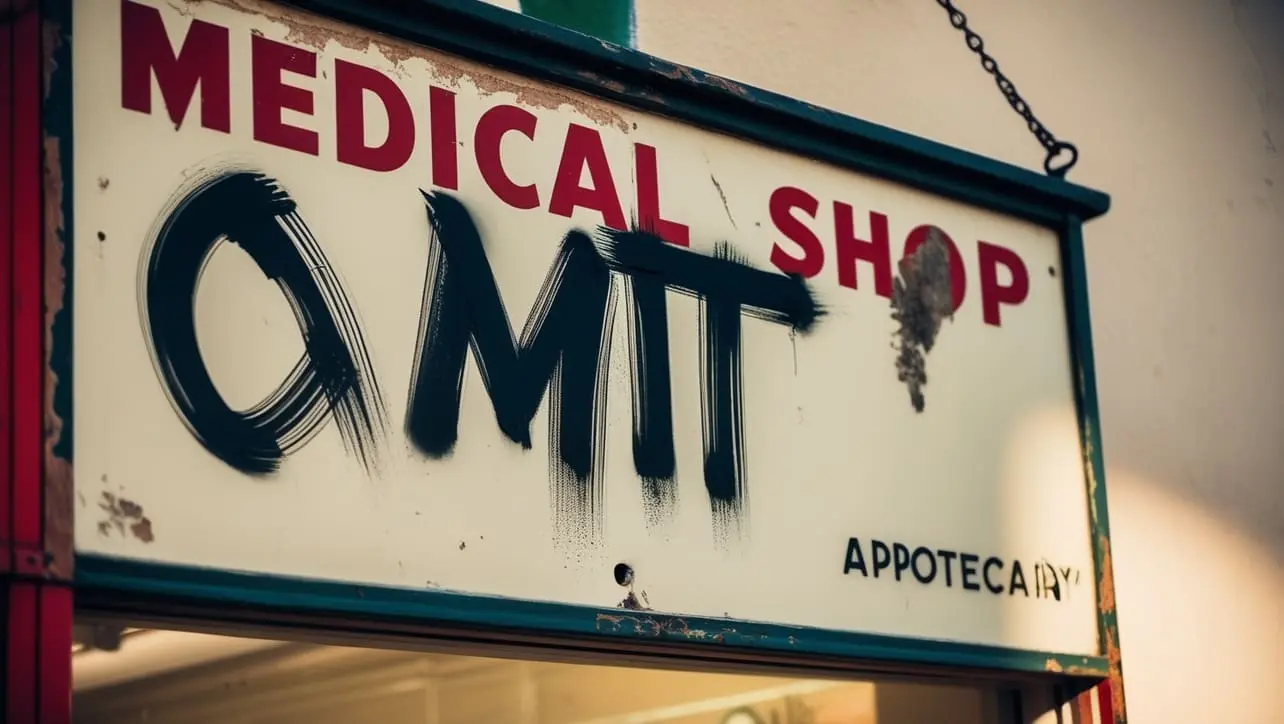
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, efficient management of objects is crucial for building robust applications. Lodash, a popular utility library, provides a wide range of functions to simplify object manipulation tasks. Among these functions, the _.omit()
method stands out as a powerful tool for excluding specified properties from an object.
This method enhances code readability and flexibility, making it invaluable for developers dealing with complex data structures.
🧠 Understanding _.omit() Method
This method enhances code readability and flexibility, making it invaluable for developers dealing with complex data structures.
💡 Syntax
The syntax for the _.omit()
method is straightforward:
_.omit(object, [props])
- object: The object from which properties will be omitted.
- props: The properties to omit (can be a single property or an array of properties).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.omit()
method:
const _ = require('lodash');
const originalObject = {
name: 'John',
age: 30,
email: 'john@example.com',
profession: 'Developer'
};
const modifiedObject = _.omit(originalObject, ['email', 'profession']);
console.log(modifiedObject);
// Output: { name: 'John', age: 30 }
In this example, the originalObject is modified using _.omit()
, resulting in a new object excluding the specified properties.
🏆 Best Practices
When working with the _.omit()
method, consider the following best practices:
Targeted Property Exclusion:
Be specific when specifying properties to omit using
_.omit()
. This ensures that only the intended properties are excluded, avoiding unintended modifications to the object structure.example.jsCopiedconst user = { name: 'Alice', age: 25, email: 'alice@example.com', isAdmin: true }; const modifiedUser = _.omit(user, ['email', 'isAdmin']); console.log(modifiedUser); // Output: { name: 'Alice', age: 25 }
Avoid Mutating Original Object:
To maintain data integrity, avoid mutating the original object when using
_.omit()
. Instead, create a new object with the desired properties excluded to preserve the original data.example.jsCopiedconst originalData = { a: 1, b: 2, c: 3 }; const excludedKeys = ['b', 'c']; const modifiedData = _.omit(originalData, excludedKeys); console.log(modifiedData); // Output: { a: 1 }
Consider Default Values:
When omitting properties, consider providing default values for omitted properties if necessary. This ensures consistent data structures and prevents unexpected behavior in downstream code.
example.jsCopiedconst userData = { name: 'Bob', age: 30, email: 'bob@example.com' }; const modifiedUserData = _.defaults(_.omit(userData, ['email']), { profession: 'Unknown' }); console.log(modifiedUserData); // Output: { name: 'Bob', age: 30, profession: 'Unknown' }
📚 Use Cases
Data Transformation:
_.omit()
is particularly useful when transforming data structures, such as excluding sensitive information before sending objects to clients or APIs.example.jsCopiedconst sensitiveUserData = { username: 'john_doe', password: 'securepassword123', email: 'john@example.com' }; const sanitizedUserData = _.omit(sensitiveUserData, ['password']); console.log(sanitizedUserData); // Output: { username: 'john_doe', email: 'john@example.com' }
Simplifying Objects:
When working with complex objects,
_.omit()
can simplify object structures by removing unnecessary properties, improving code readability and maintainability.example.jsCopiedconst complexObject = { id: 1, name: 'Product', description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.', category: 'Electronics', price: 999.99, createdAt: '2024-02-24T12:00:00Z' }; const simplifiedObject = _.omit(complexObject, ['description', 'createdAt']); console.log(simplifiedObject); // Output: { id: 1, name: 'Product', category: 'Electronics', price: 999.99 }
Object Filtering:
In scenarios where selective data presentation is required,
_.omit()
can be used to filter out specific properties, ensuring that only relevant information is included in the output.example.jsCopiedconst userPreferences = { darkMode: true, fontSize: 'medium', language: 'en', showNotifications: true }; const filteredPreferences = _.omit(userPreferences, ['fontSize', 'showNotifications']); console.log(filteredPreferences); // Output: { darkMode: true, language: 'en' }
🎉 Conclusion
The _.omit()
method in Lodash offers a versatile solution for excluding specified properties from objects, enhancing code flexibility and maintainability. Whether you're transforming data structures, simplifying objects, or filtering properties, _.omit()
provides a powerful tool for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.omit()
method in your Lodash projects.
👨💻 Join our Community:
Author
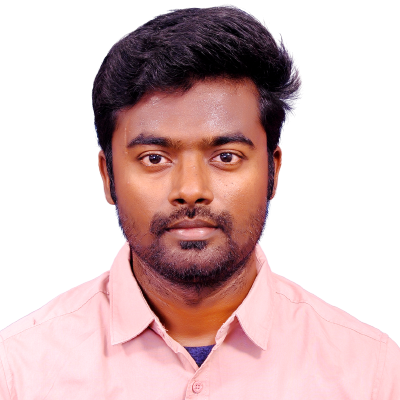
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
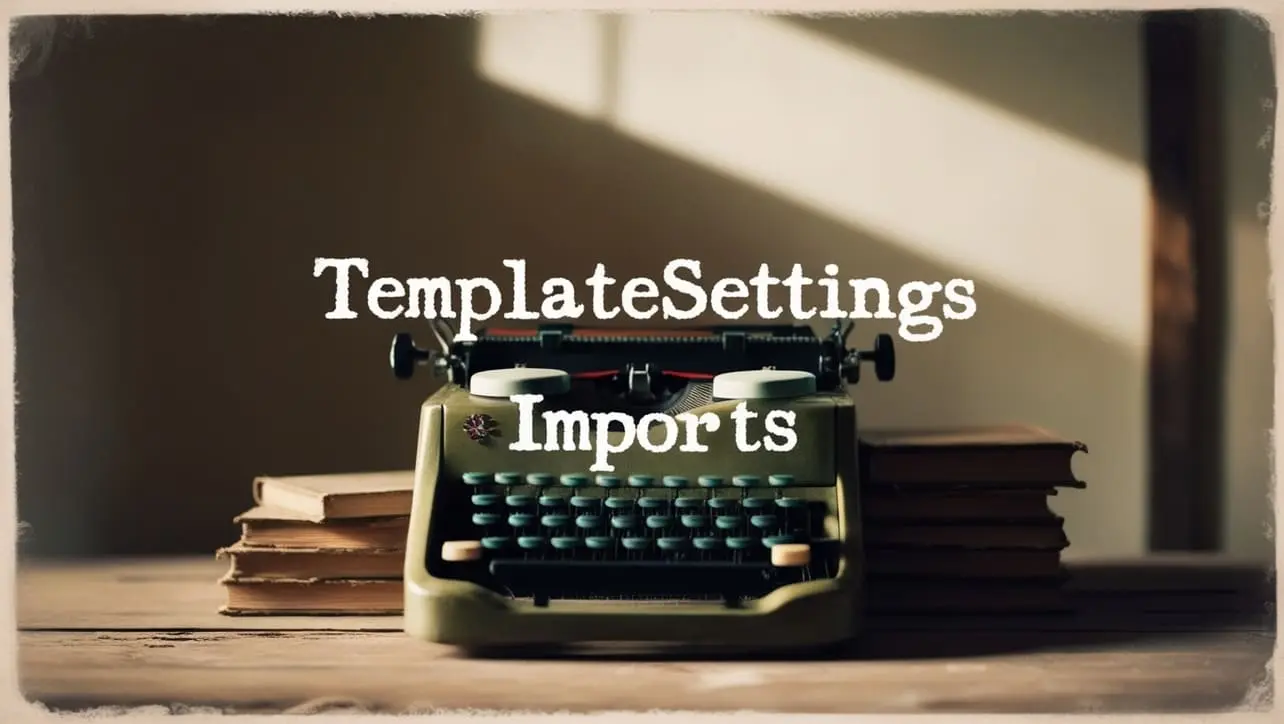
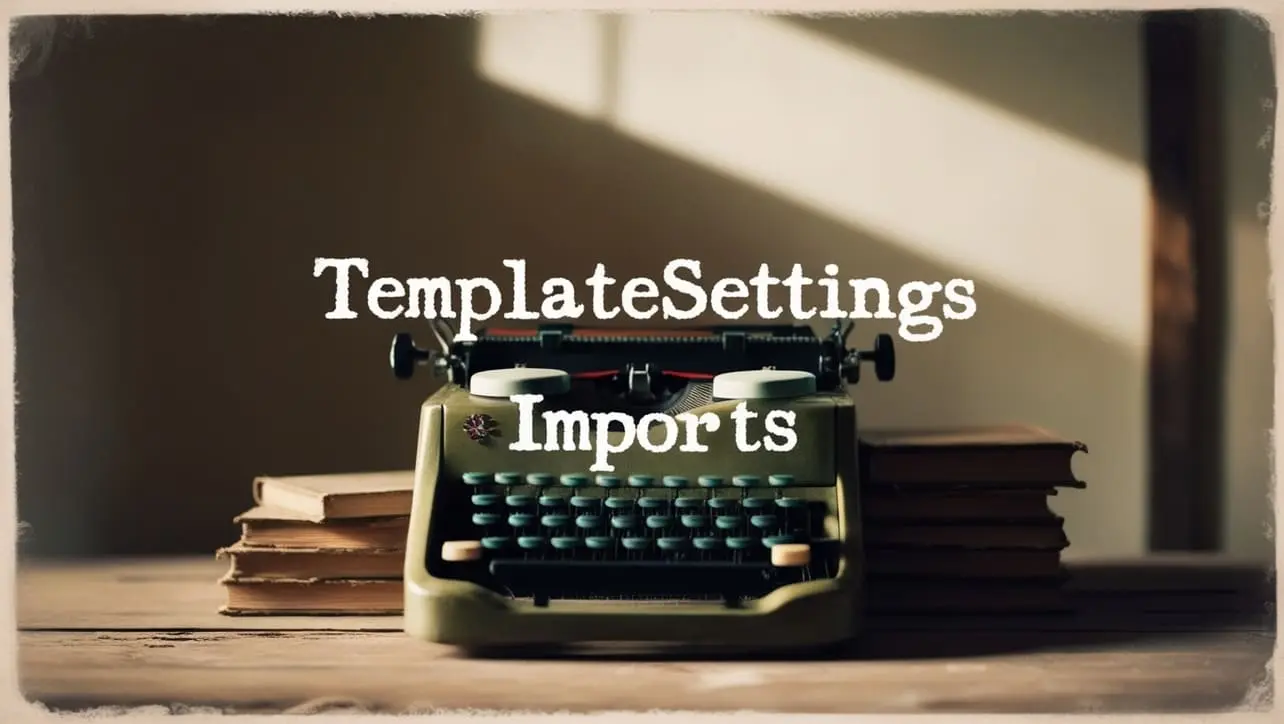
Lodash _.templateSettings.imports Property
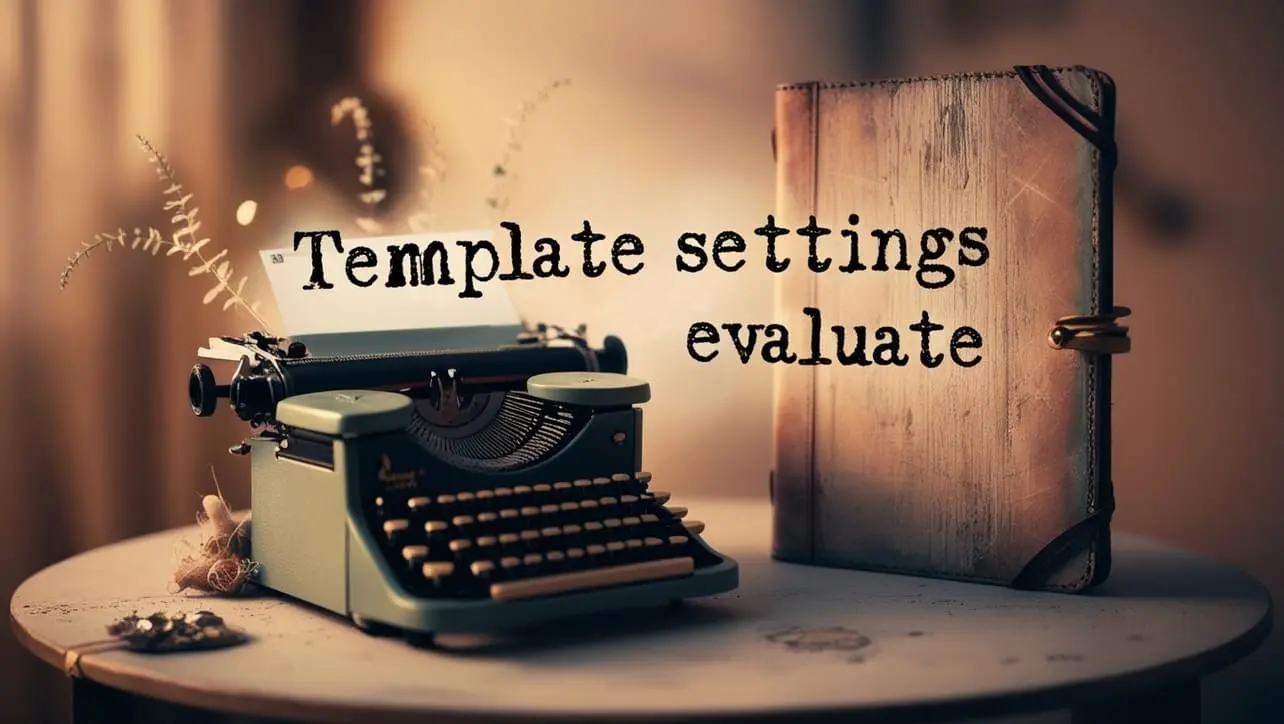
Lodash _.templateSettings.evaluate Property
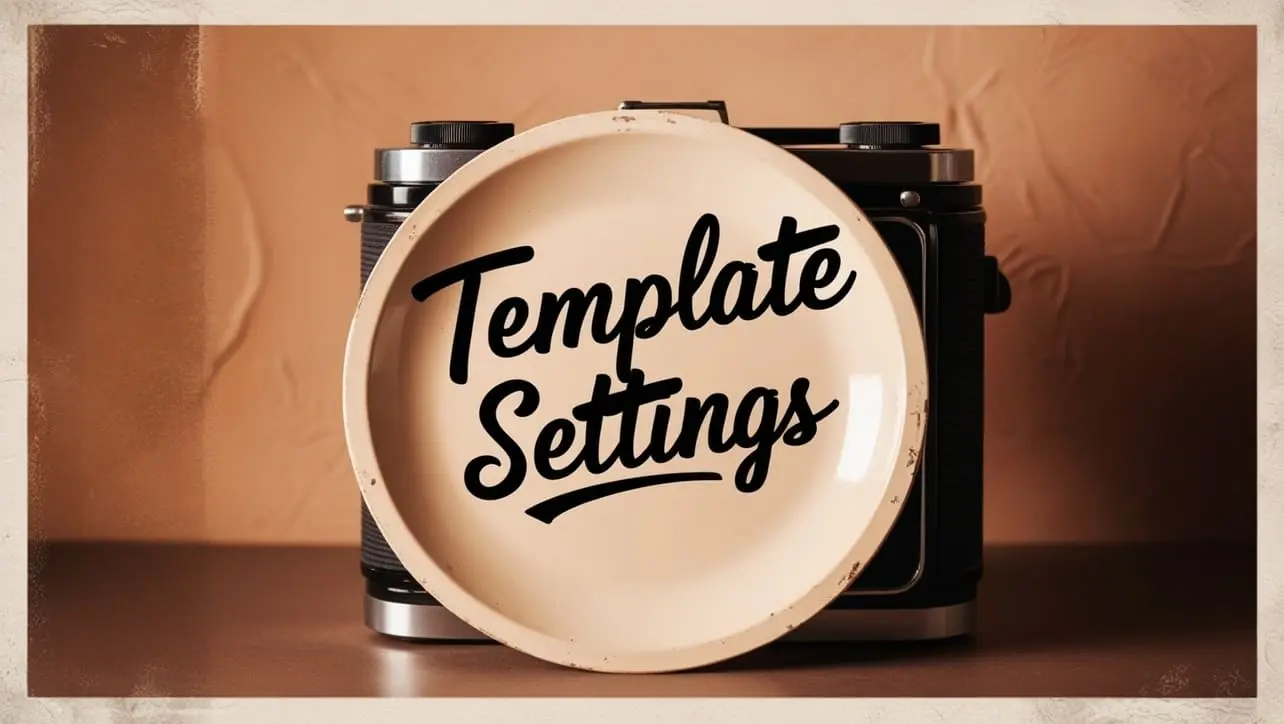
Lodash _.templateSettings Property
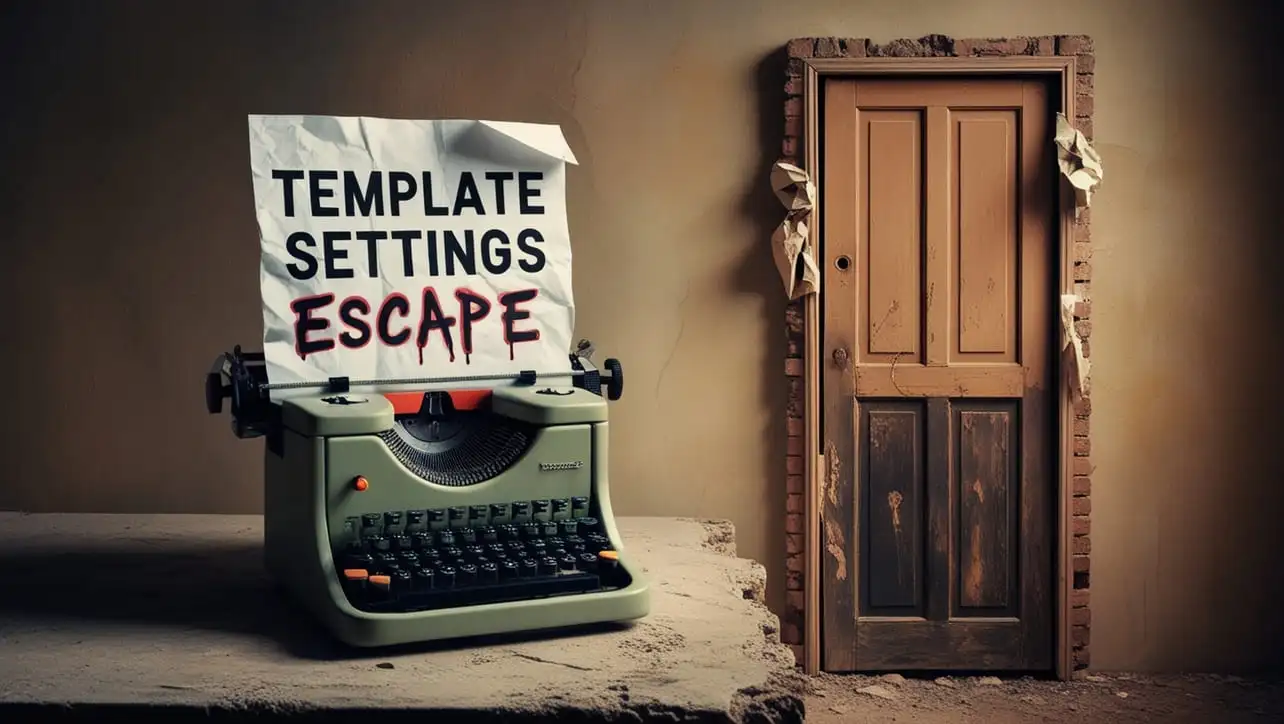
Lodash _.templateSettings.escape Property
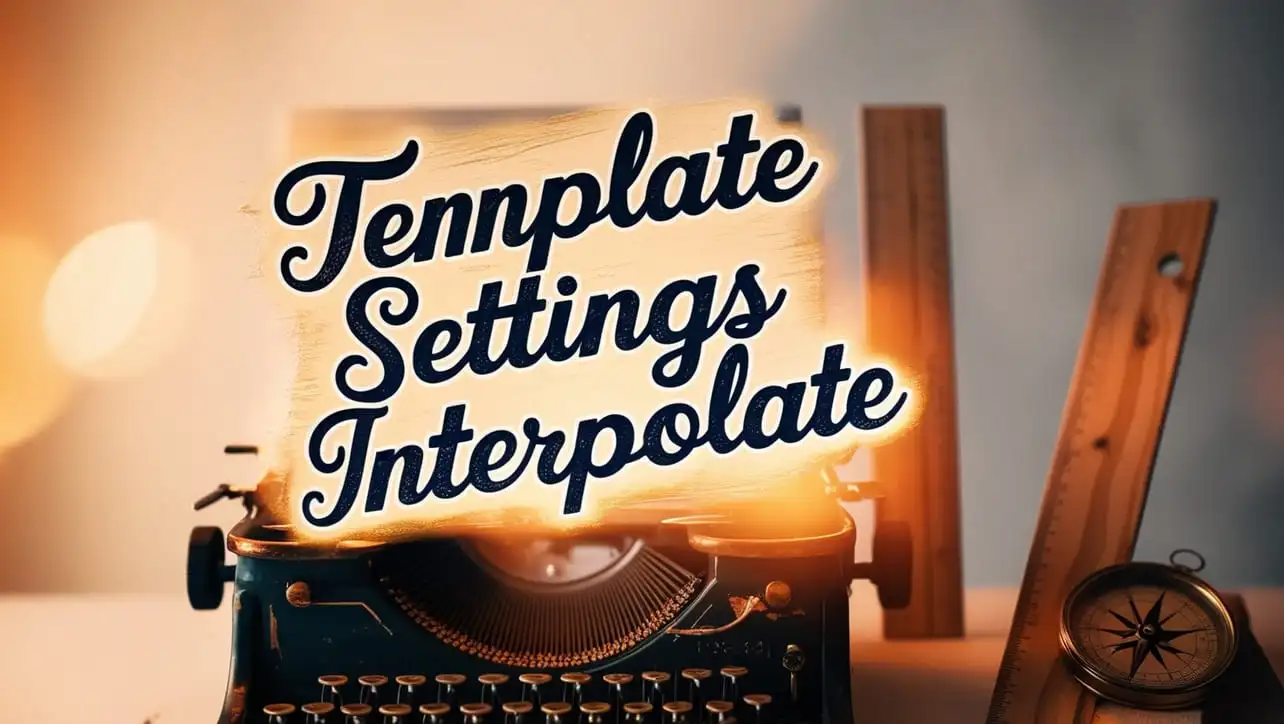
Lodash _.templateSettings.interpolate Property
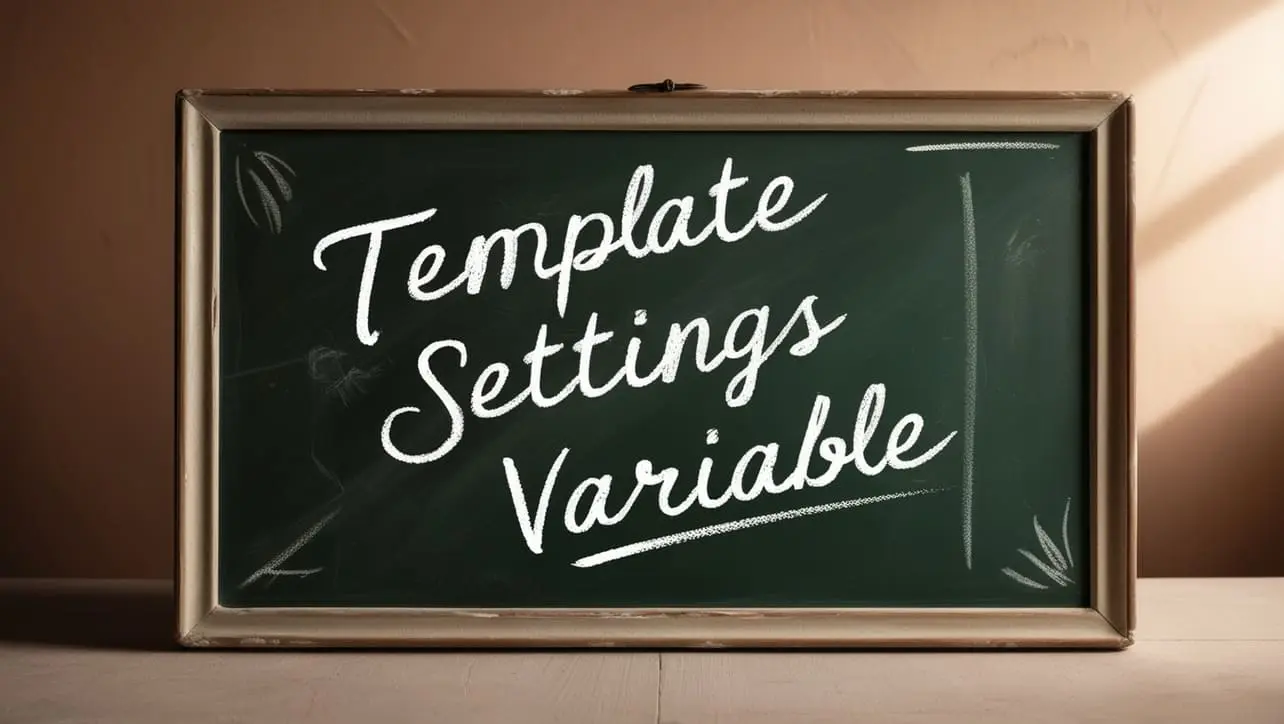
If you have any doubts regarding this article (Lodash _.omit() Object Method), please comment here. I will help you immediately.