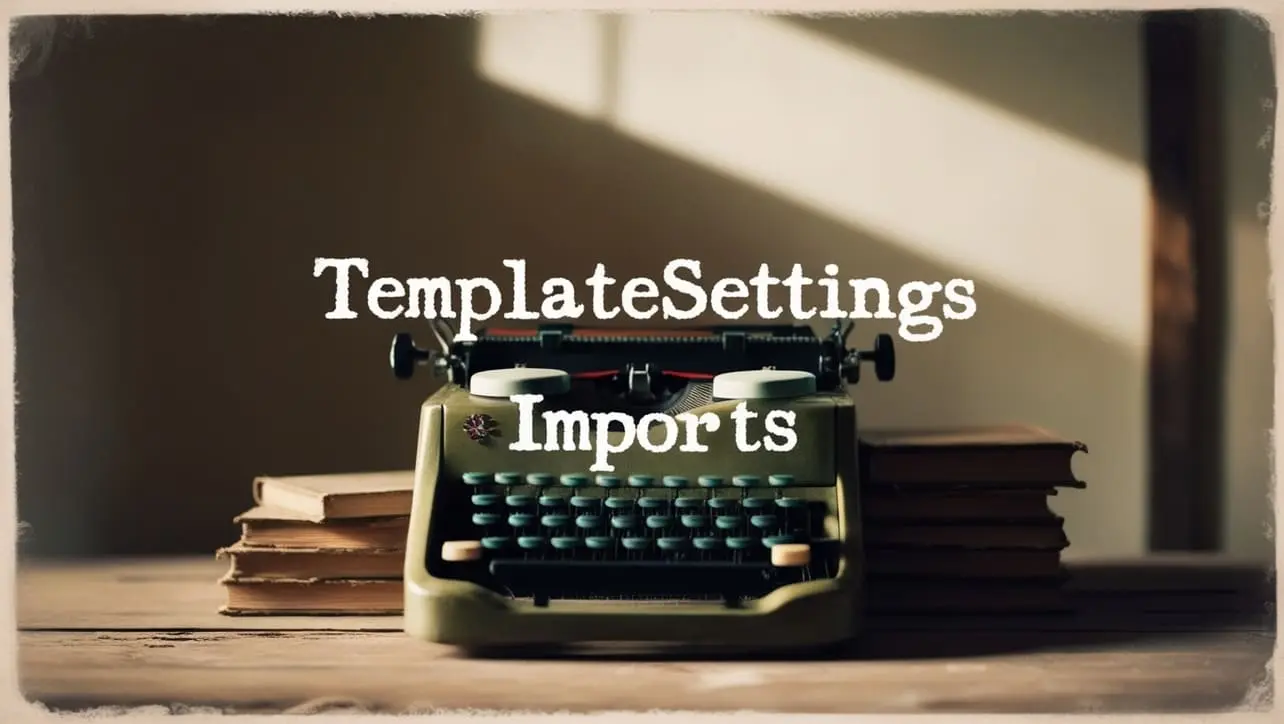
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.merge() Object Method
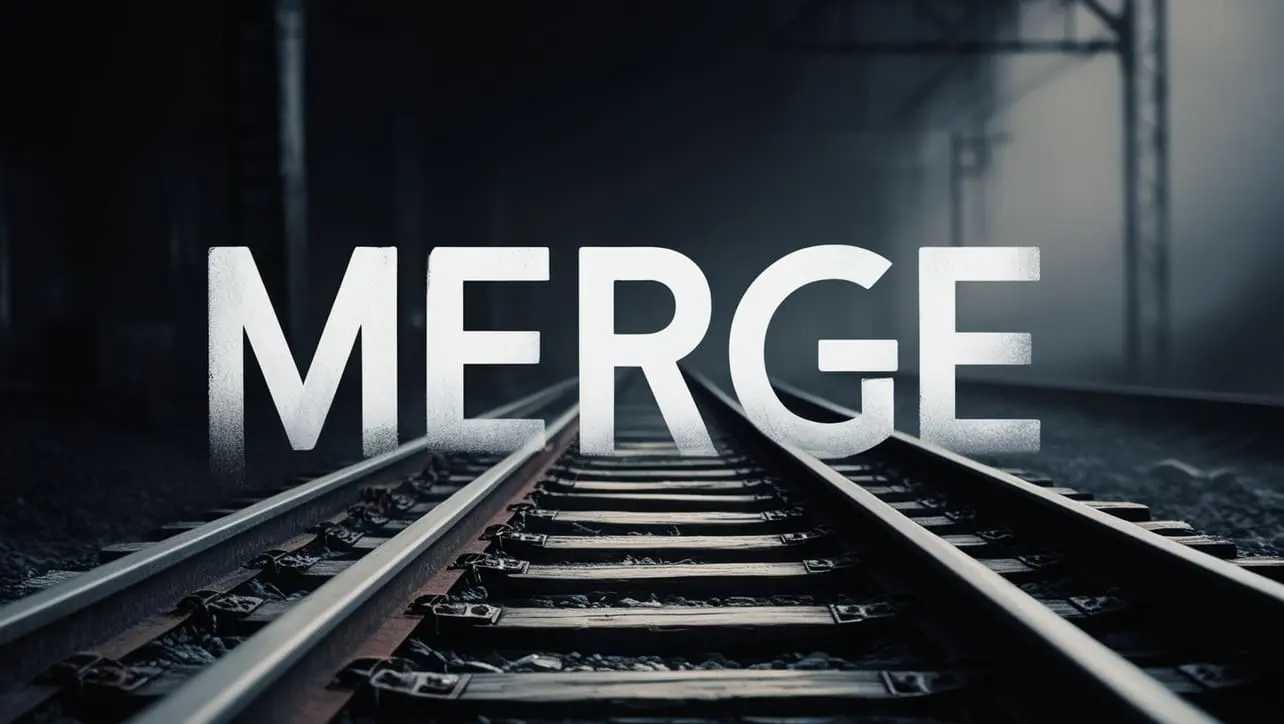
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, managing complex objects and their properties efficiently is crucial. Lodash, a widely-used utility library, offers a plethora of functions to simplify object manipulation. Among these functions lies the _.merge()
method, a powerful tool for merging two or more objects while handling nested properties with ease.
This method enhances code maintainability and readability, making it indispensable for developers dealing with complex data structures.
🧠 Understanding _.merge() Method
The _.merge()
method in Lodash is designed to merge multiple objects into a single object, with nested properties recursively merged. This enables developers to combine and consolidate data from disparate sources effortlessly, simplifying data management and manipulation.
💡 Syntax
The syntax for the _.merge()
method is straightforward:
_.merge(object, [sources])
- object: The destination object to merge into.
- sources: The source objects to merge from.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.merge()
method:
const _ = require('lodash');
const destinationObject = {
name: 'John',
age: 30,
address: {
city: 'New York',
country: 'USA'
}
};
const sourceObject = {
age: 35,
address: {
city: 'San Francisco'
}
};
const mergedObject = _.merge(destinationObject, sourceObject);
console.log(mergedObject);
In this example, destinationObject and sourceObject are merged using _.merge()
, resulting in a new object containing the combined properties:
{
"name": "John",
"age": 35,
"address": {
"city": "San Francisco",
"country": "USA"
}
}
🏆 Best Practices
When working with the _.merge()
method, consider the following best practices:
Preserve Destination Object:
To prevent unintentional modification of the destination object, ensure that the destination object is a separate instance or clone.
example.jsCopiedconst destinationObject = { name: 'John', address: { city: 'New York' } }; const sourceObject = { age: 30 }; const clonedDestination = _.cloneDeep(destinationObject); const mergedObject = _.merge(clonedDestination, sourceObject); console.log(mergedObject);
Handle Array Merging:
Be aware that
_.merge()
does not handle array merging by default. If you need to merge arrays within objects, consider using custom merging logic or Lodash's _.mergeWith() method.example.jsCopiedconst destinationObject = { colors: ['red', 'green'], }; const sourceObject = { colors: ['blue'], }; const mergedObject = _.mergeWith(destinationObject, sourceObject, (objValue, srcValue) => { if(_.isArray(objValue)) { return objValue.concat(srcValue); } }); console.log(mergedObject); // Output: { colors: ['red', 'green', 'blue'] }
Avoid Circular References:
Exercise caution when dealing with objects containing circular references, as
_.merge()
does not handle circular references and may result in stack overflow errors.
📚 Use Cases
Configuration Merging:
_.merge()
is particularly useful when merging configurations from multiple sources, such as default settings and user preferences, into a single configuration object.example.jsCopiedconst defaultConfig = { theme: 'light', fontSize: 16 }; const userConfig = { theme: 'dark', showSidebar: true }; const mergedConfig = _.merge(defaultConfig, userConfig); console.log(mergedConfig);
Data Aggregation:
In data-intensive applications,
_.merge()
can be employed to aggregate data from various sources into a unified dataset, facilitating analysis and processing.example.jsCopiedconst dataFromAPI = /* ...fetch data from API... */; const localData = /* ...load data from local storage... */; const aggregatedData = _.merge(dataFromAPI, localData); console.log(aggregatedData);
Object Patching:
When updating an object with new information,
_.merge()
can be used to patch the existing object with the changes without losing existing data.example.jsCopiedconst existingObject = /* ...retrieve existing object... */; const changes = /* ...retrieve changes... */; const patchedObject = _.merge(existingObject, changes); console.log(patchedObject);
🎉 Conclusion
The _.merge()
method in Lodash offers a versatile solution for merging objects and consolidating data from multiple sources effortlessly. Whether you're dealing with configuration settings, aggregating data, or updating objects dynamically, _.merge()
provides a powerful tool for managing complex data structures in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.merge()
method in your Lodash projects.
👨💻 Join our Community:
Author
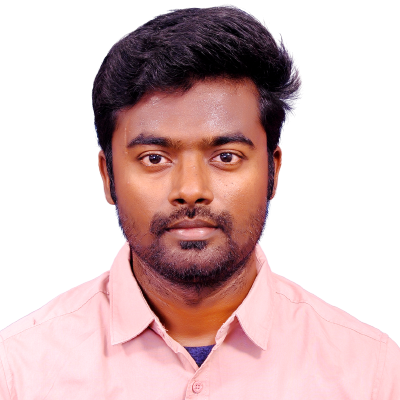
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
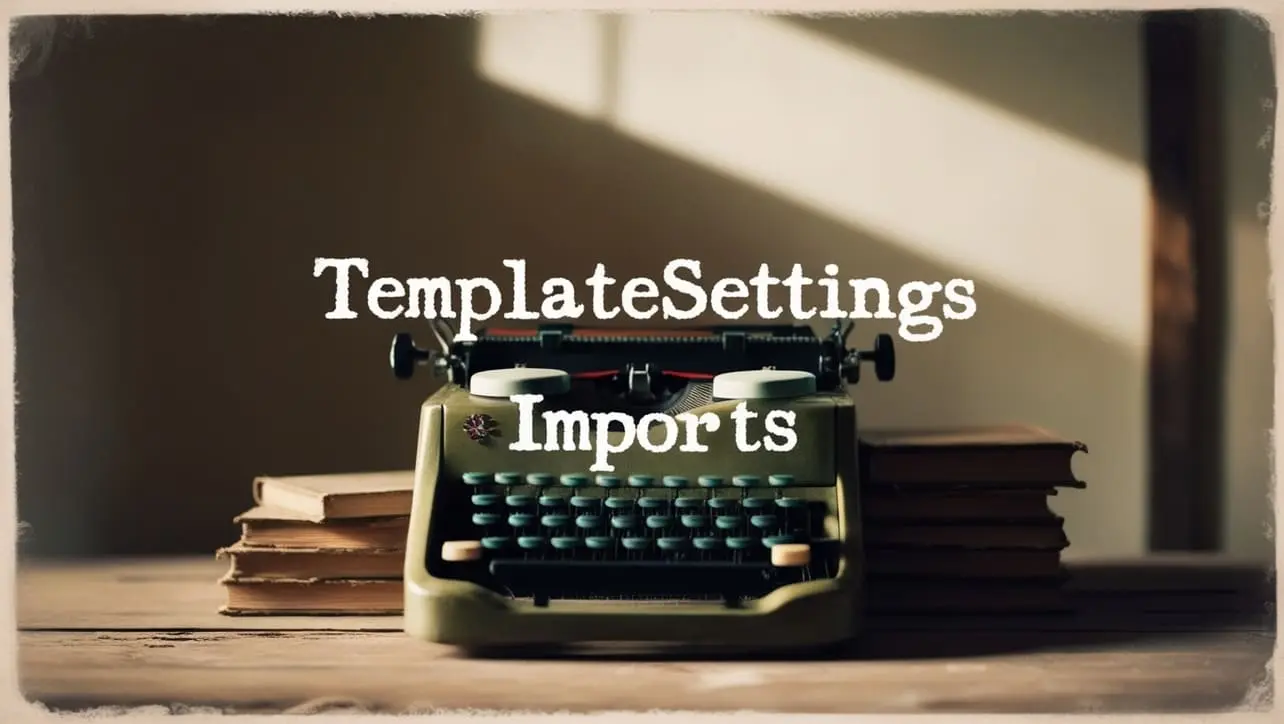
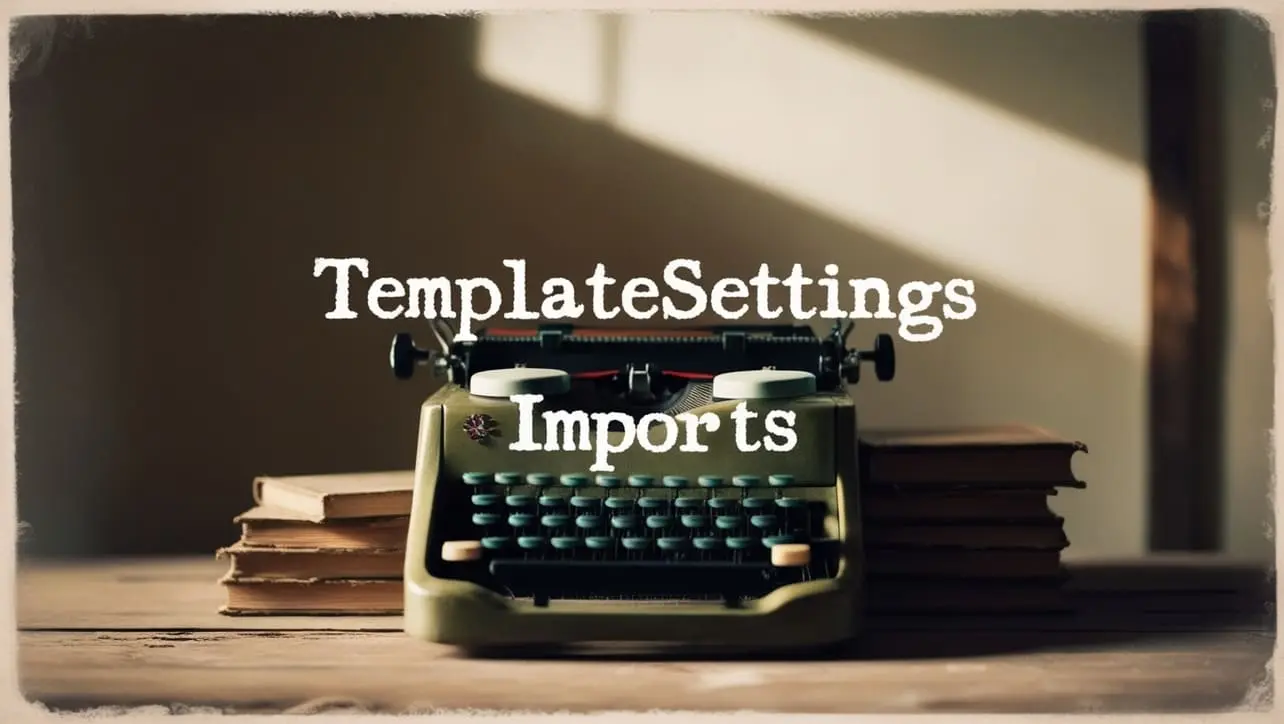
Lodash _.templateSettings.imports Property
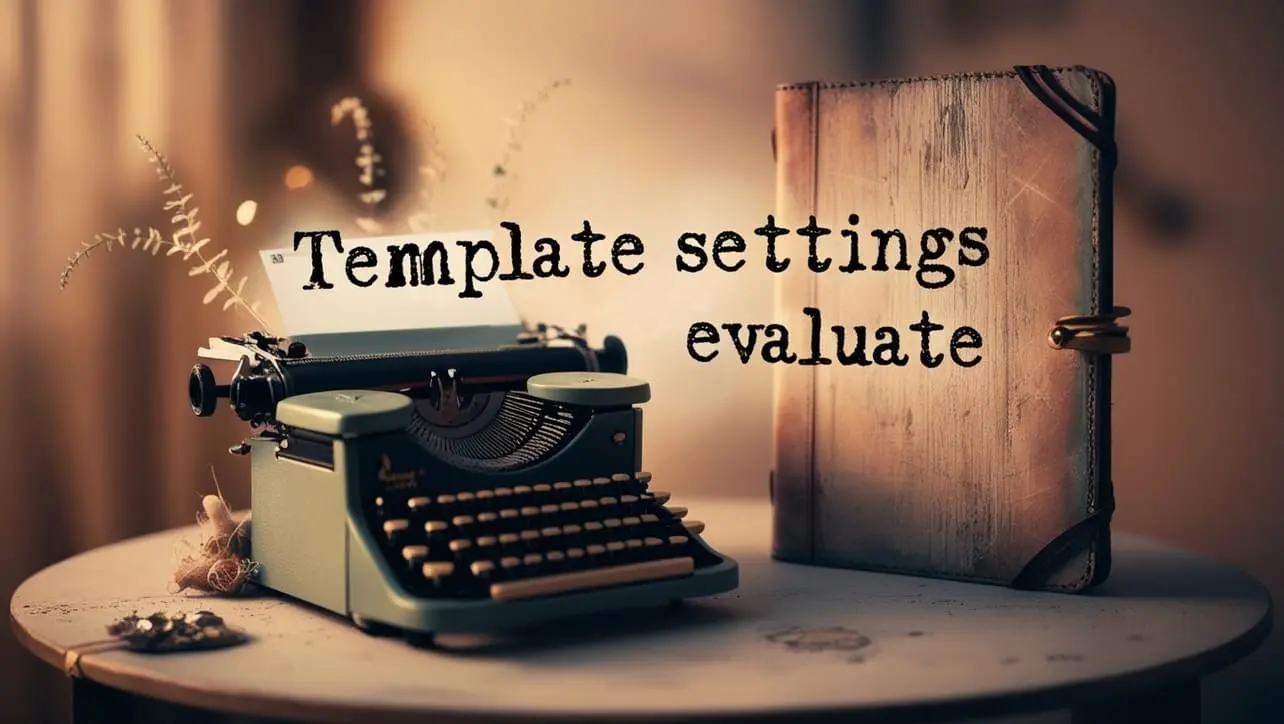
Lodash _.templateSettings.evaluate Property
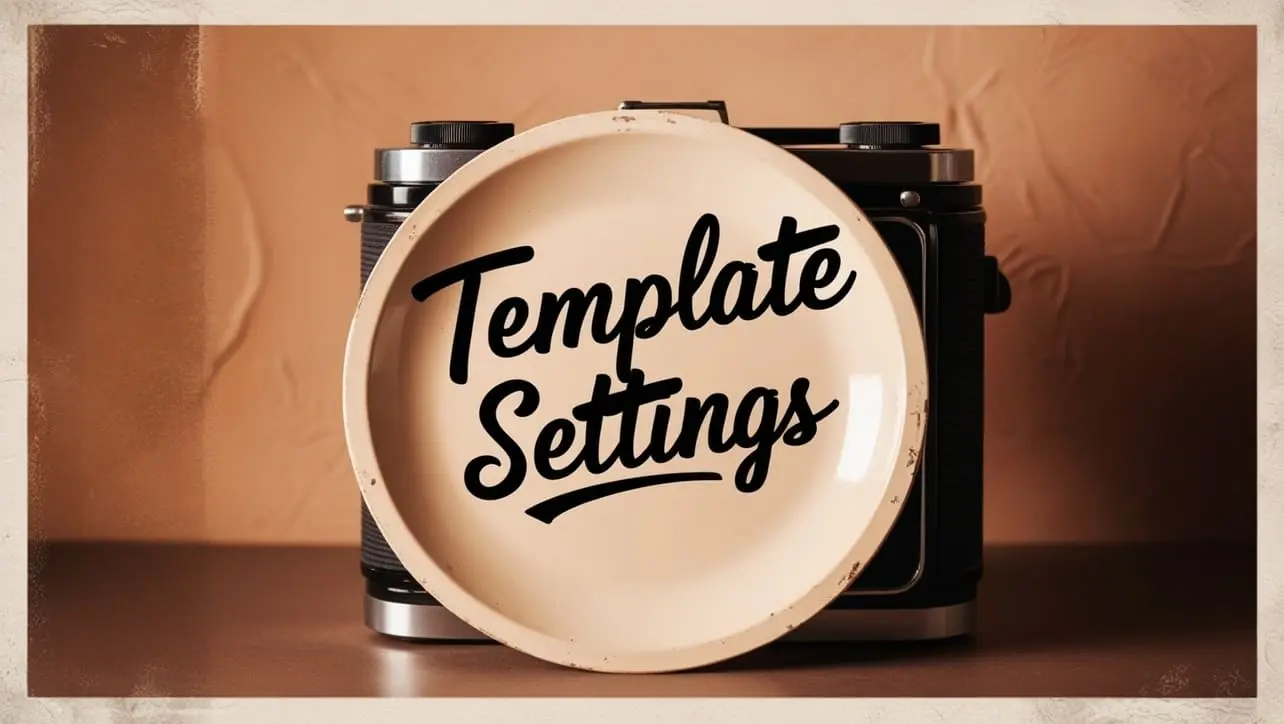
Lodash _.templateSettings Property
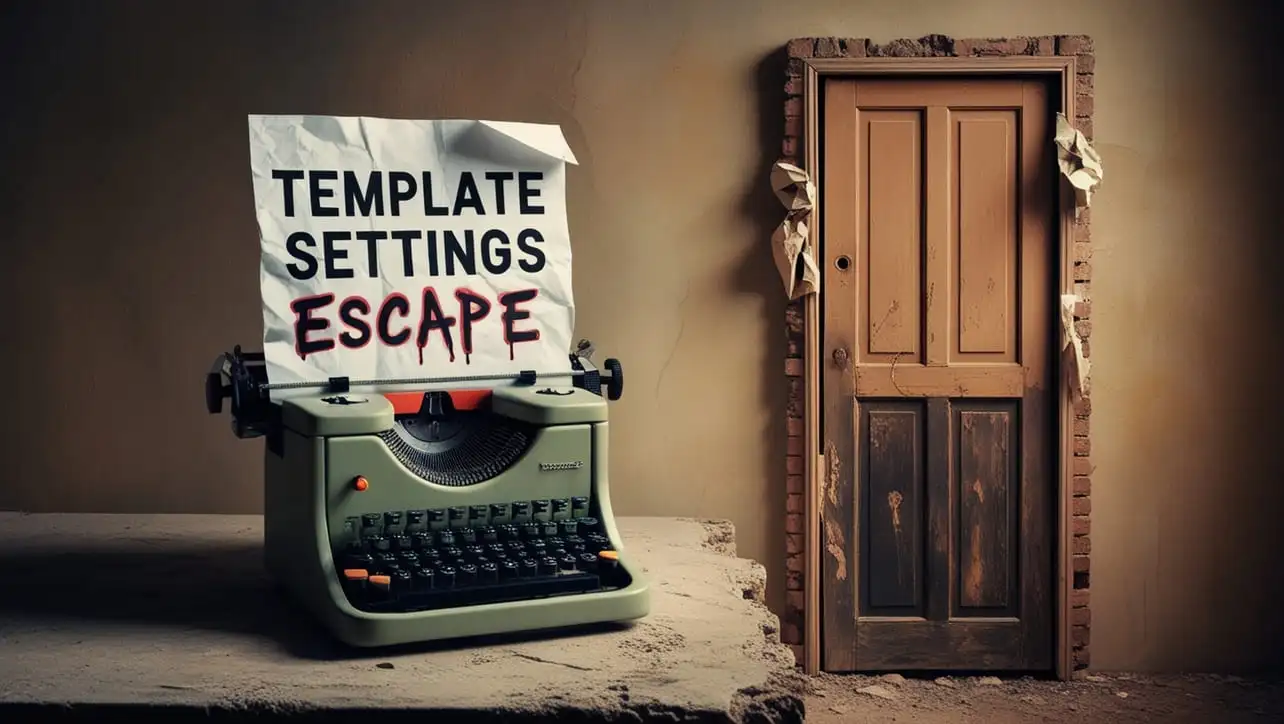
Lodash _.templateSettings.escape Property
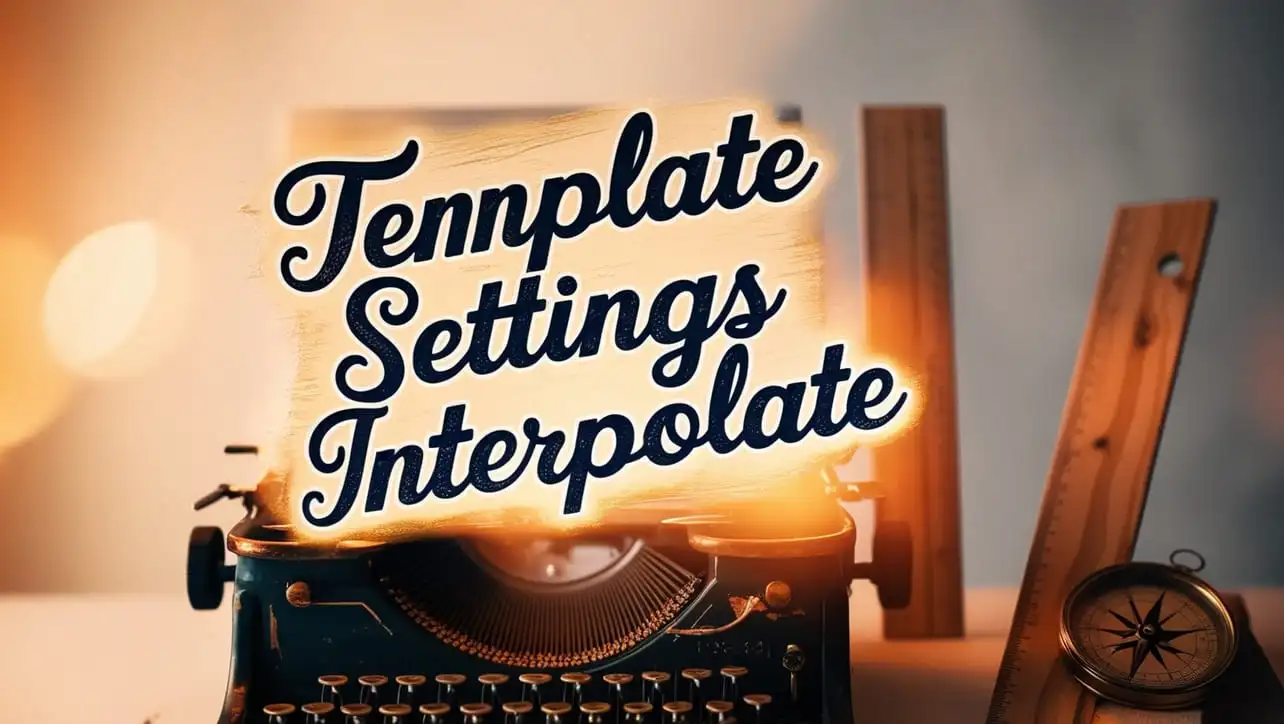
Lodash _.templateSettings.interpolate Property
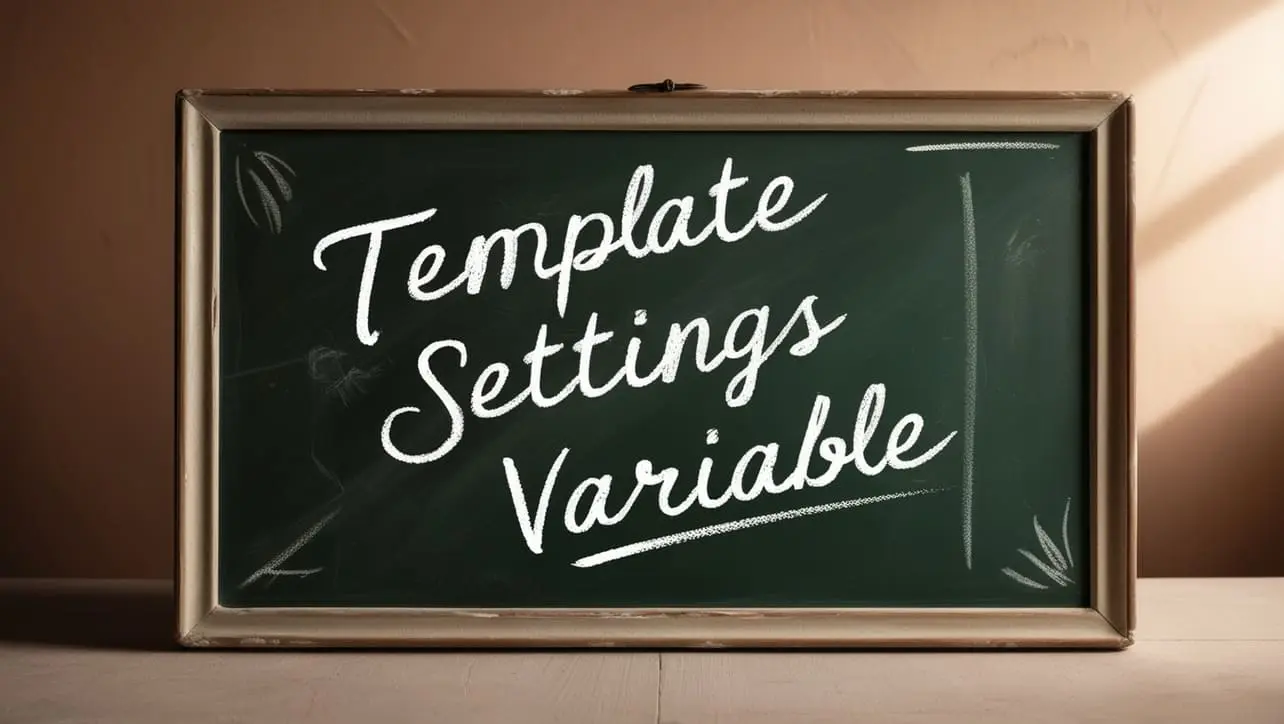
If you have any doubts regarding this article (Lodash _.merge() Object Method), please comment here. I will help you immediately.