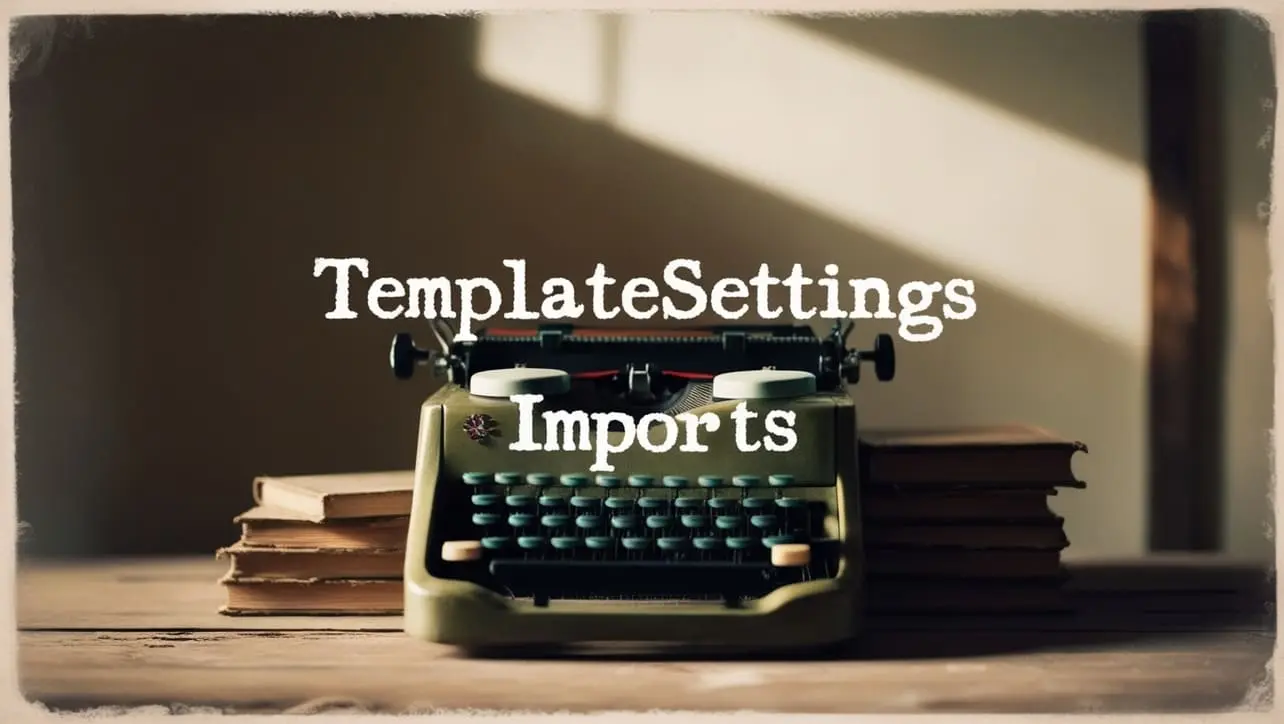
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.mapKeys() Object Method
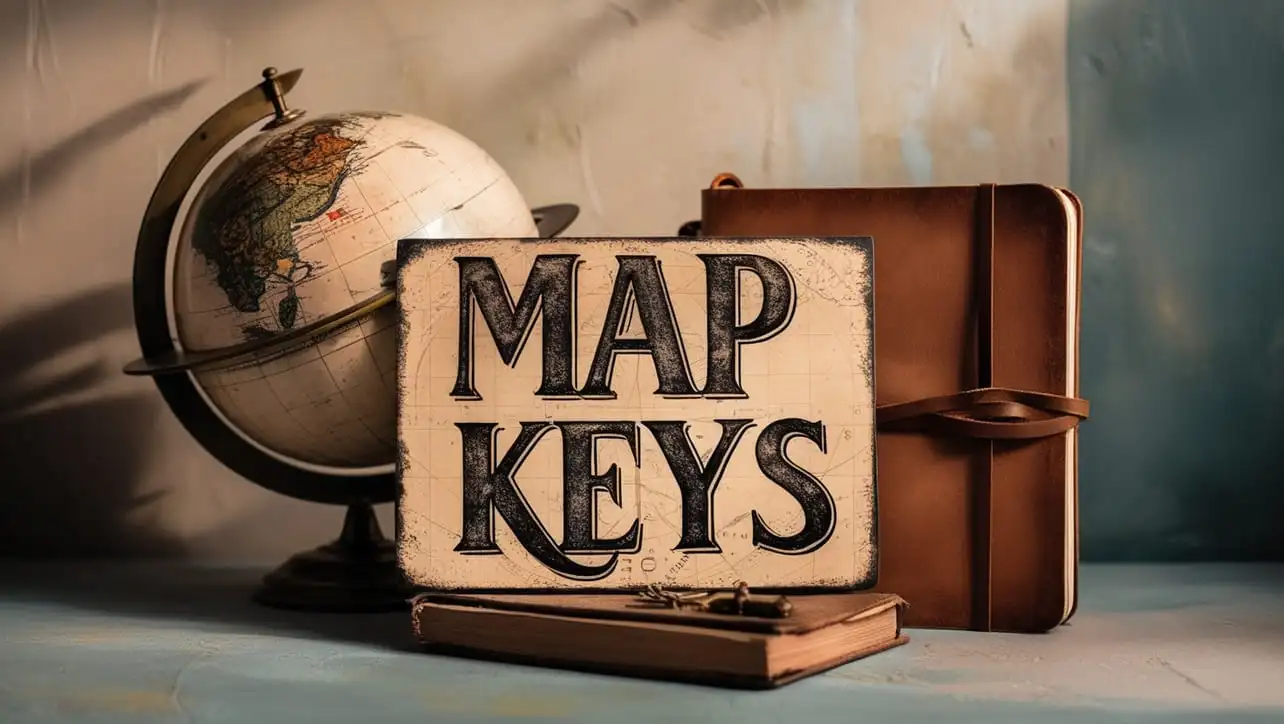
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, manipulating objects is a common task. Lodash, a popular utility library, offers a plethora of functions to streamline object manipulation. One such function is _.mapKeys()
, which allows developers to transform the keys of an object based on a provided mapping function.
This method enhances code readability and flexibility, making it a valuable tool in the developer's arsenal.
🧠 Understanding _.mapKeys() Method
The _.mapKeys()
method in Lodash is designed to create a new object by transforming the keys of an existing object. It iterates over the keys of the input object and applies a mapping function to each key, producing a new object with the transformed keys.
💡 Syntax
The syntax for the _.mapKeys()
method is straightforward:
_.mapKeys(object, [iteratee])
- object: The object to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.mapKeys()
method:
const _ = require('lodash');
const originalObject = { a: 1, b: 2, c: 3 };
const transformedObject = _.mapKeys(originalObject, (value, key) => key.toUpperCase());
console.log(transformedObject);
// Output: { A: 1, B: 2, C: 3 }
In this example, the keys of originalObject are transformed to uppercase using _.mapKeys()
, resulting in a new object transformedObject.
🏆 Best Practices
When working with the _.mapKeys()
method, consider the following best practices:
Understanding Mapping Functions:
Familiarize yourself with mapping functions and their capabilities to effectively utilize
_.mapKeys()
. Mapping functions allow you to specify the transformation logic for object keys.example.jsCopiedconst originalObject = { name: 'John', age: 30 }; const transformedObject = _.mapKeys(originalObject, (value, key) => `${key}_updated`); console.log(transformedObject); // Output: { name_updated: 'John', age_updated: 30 }
Handle Edge Cases:
Consider potential edge cases when using
_.mapKeys()
, such as empty objects or objects with non-string keys. Implement appropriate error handling or default behaviors to address these scenarios.example.jsCopiedconst emptyObject = {}; const transformedEmptyObject = _.mapKeys(emptyObject, (value, key) => key.toUpperCase()); console.log(transformedEmptyObject); // Output: {}
Maintain Object Integrity:
Ensure that the transformation process preserves the integrity of the original object's data. Validate the mapping function to avoid unintended side effects or data loss.
example.jsCopiedconst originalObject = { a: 1, b: 2, c: 3 }; const transformedObject = _.mapKeys(originalObject, (value, key) => key.slice(0, 1)); console.log(transformedObject); // Output: { a: 1, b: 2, c: 3 }
📚 Use Cases
Normalizing Data:
_.mapKeys()
can be utilized to normalize data by standardizing object keys. This is particularly useful when integrating data from multiple sources with different key conventions.example.jsCopiedconst rawData = { first_name: 'John', last_name: 'Doe', age: 30 }; const normalizedData = _.mapKeys(rawData, (value, key) => key.replace('_', ' ')); console.log(normalizedData); // Output: { 'first name': 'John', 'last name': 'Doe', age: 30 }
Renaming Object Keys:
When you need to rename keys within an object,
_.mapKeys()
provides a convenient solution. By specifying a mapping function, you can easily rename keys based on a predefined convention.example.jsCopiedconst user = { username: 'john_doe', email: 'john@example.com' }; const updatedUser = _.mapKeys(user, (value, key) => { if (key === 'username') return 'username_new'; return key; }); console.log(updatedUser); // Output: { username_new: 'john_doe', email: 'john@example.com' }
Custom Key Transformation:
For scenarios requiring custom key transformation logic,
_.mapKeys()
empowers developers to implement tailored solutions. Whether it involves prefixing, suffixing, or entirely changing key formats, the flexibility of_.mapKeys()
makes it suitable for diverse use cases.example.jsCopiedconst data = { name: 'John', age: 30 }; const transformedData = _.mapKeys(data, (value, key) => `new_${key}`); console.log(transformedData); // Output: { new_name: 'John', new_age: 30 }
🎉 Conclusion
The _.mapKeys()
method in Lodash offers a versatile solution for transforming object keys based on a mapping function. Whether you need to standardize data, rename keys, or implement custom key transformations, _.mapKeys()
provides a convenient and efficient approach to object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.mapKeys()
method in your Lodash projects.
👨💻 Join our Community:
Author
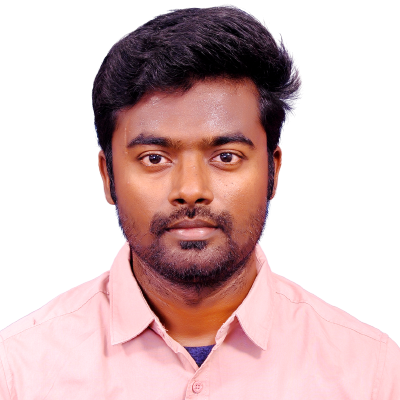
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
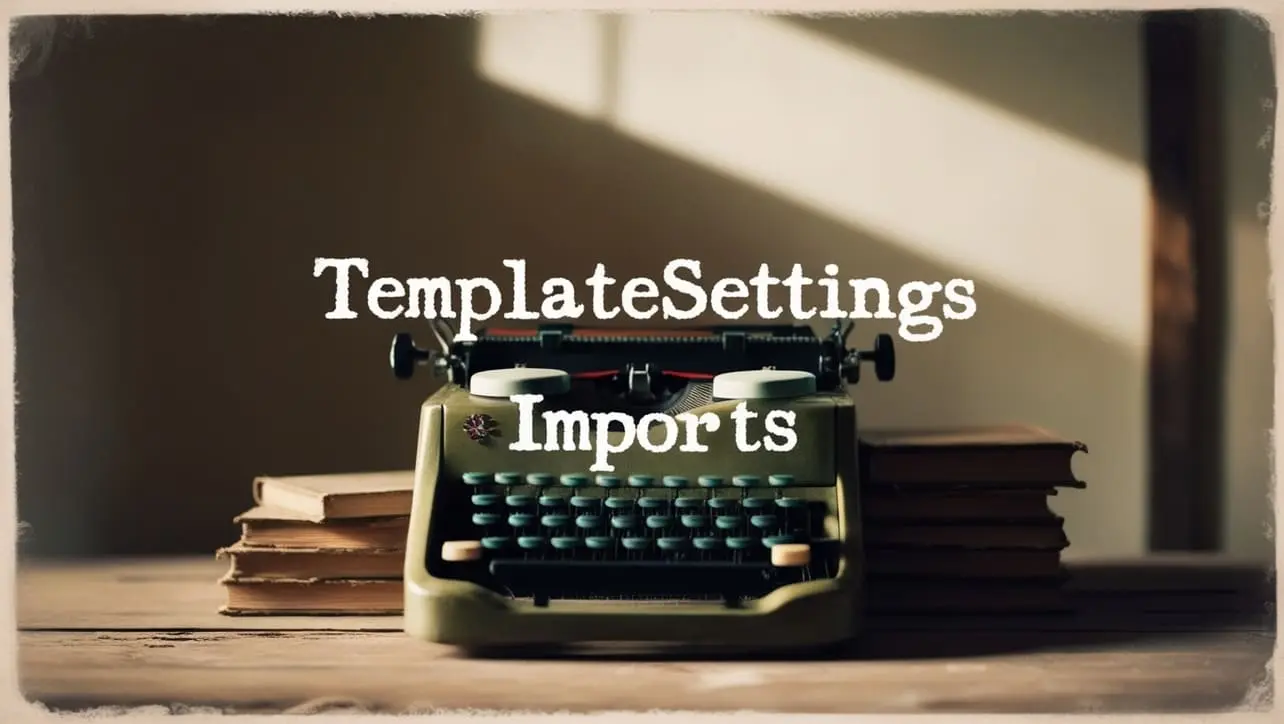
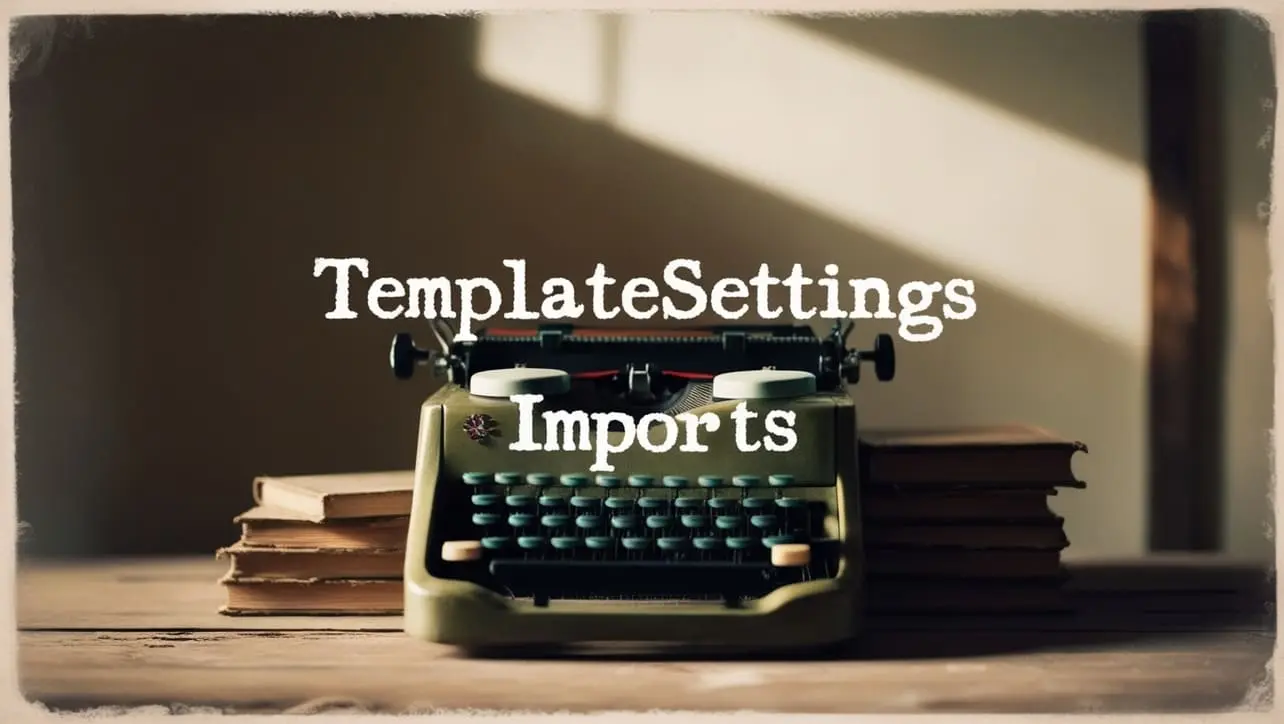
Lodash _.templateSettings.imports Property
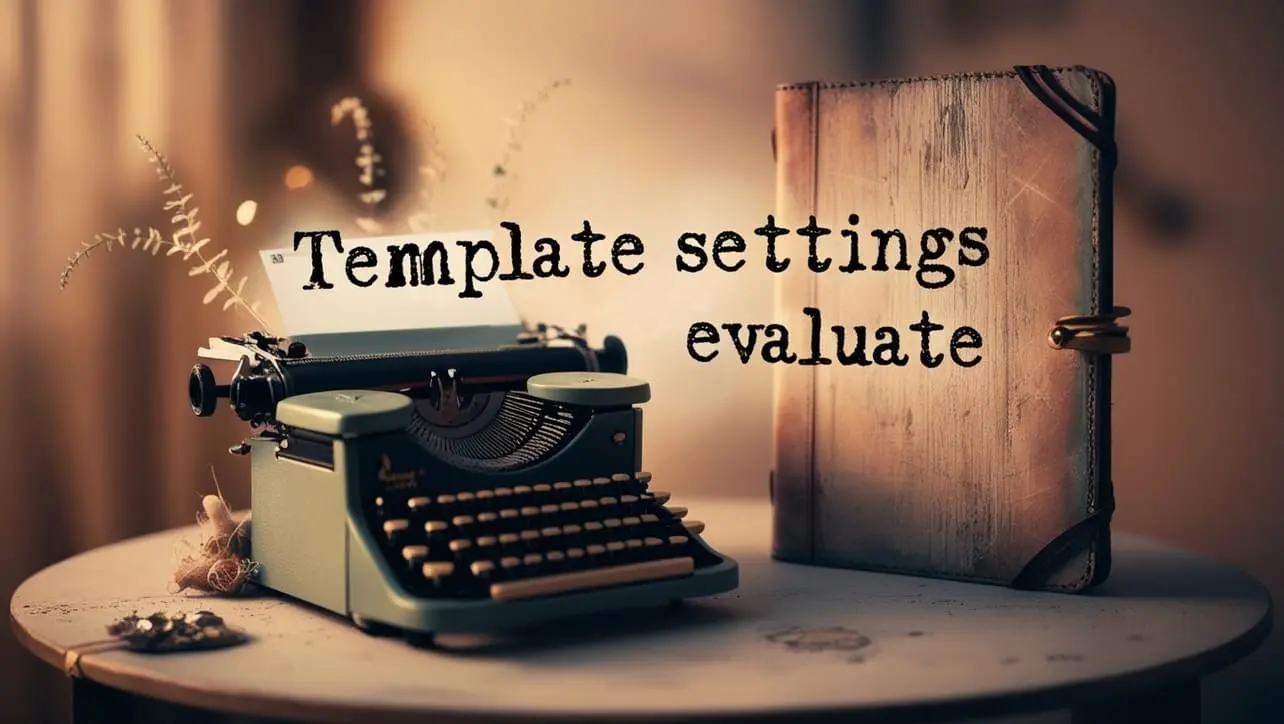
Lodash _.templateSettings.evaluate Property
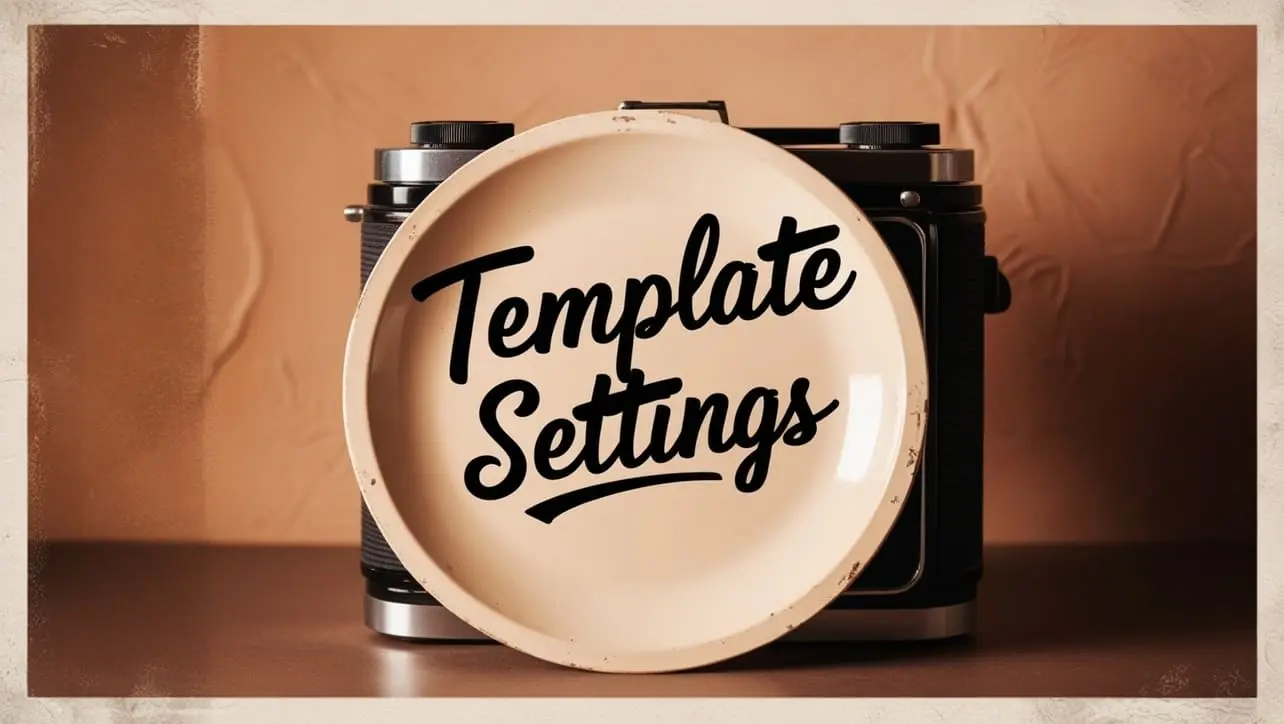
Lodash _.templateSettings Property
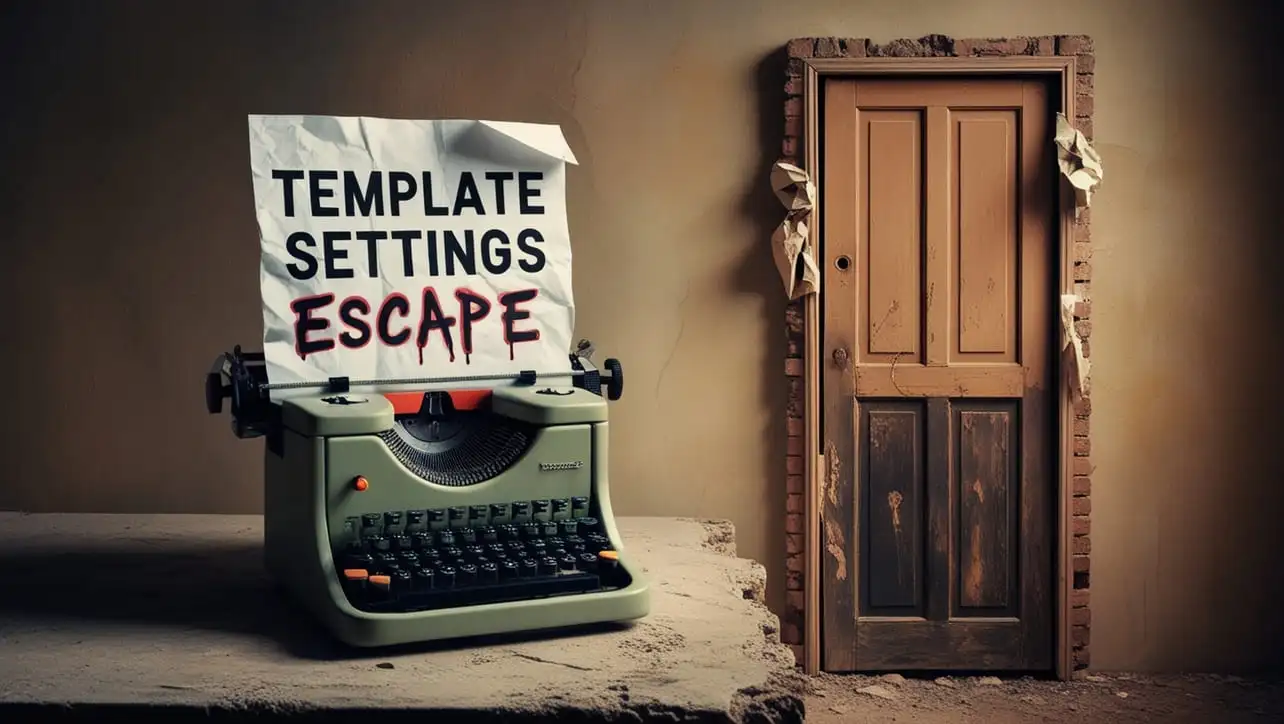
Lodash _.templateSettings.escape Property
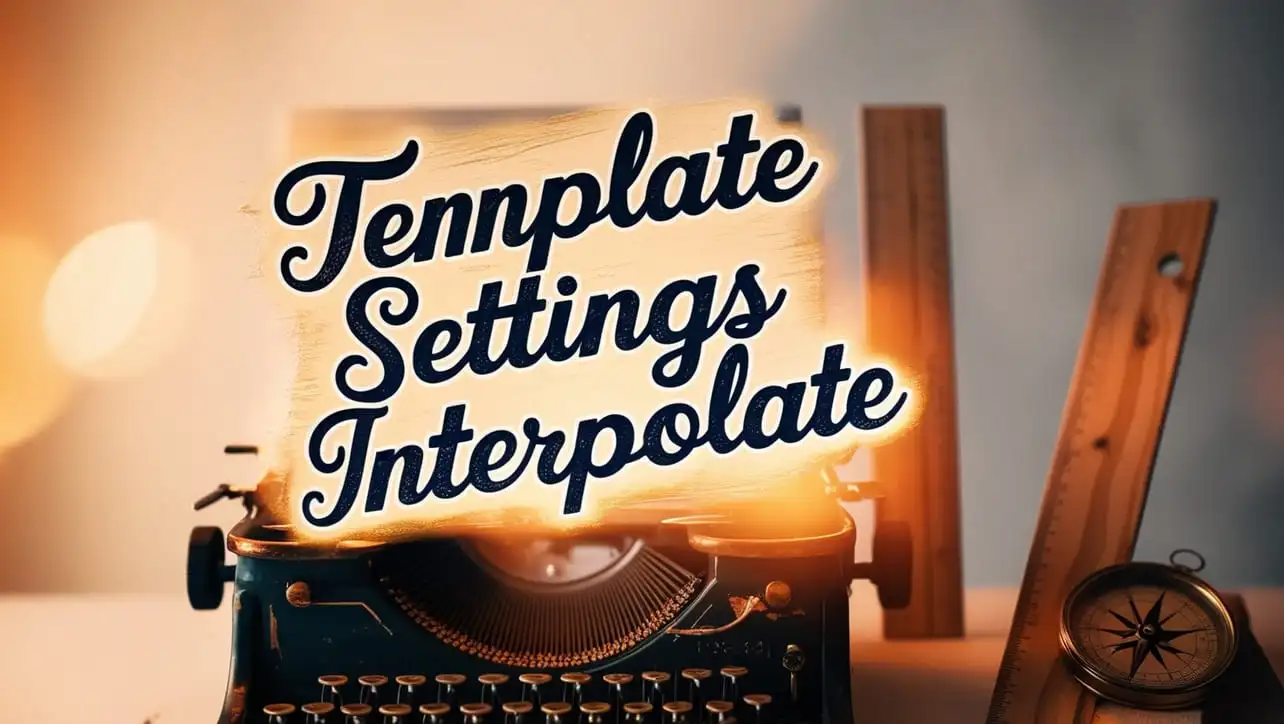
Lodash _.templateSettings.interpolate Property
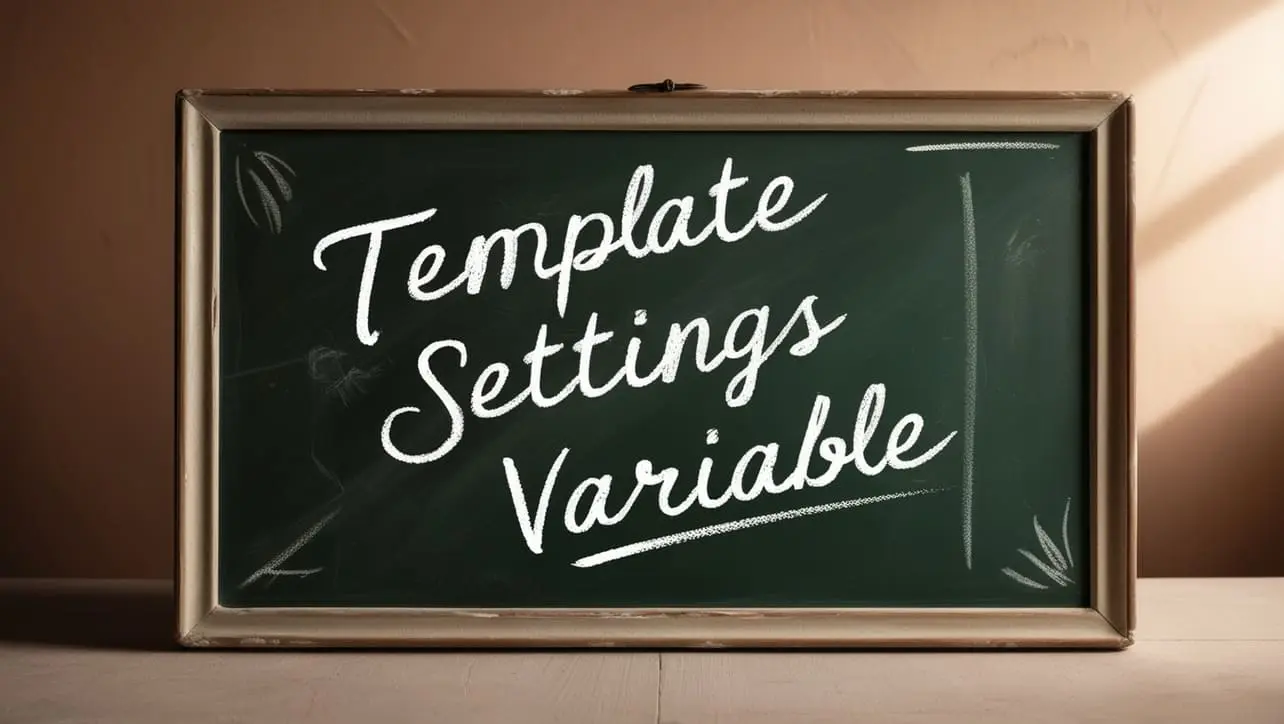
If you have any doubts regarding this article (Lodash _.mapKeys() Object Method), please comment here. I will help you immediately.