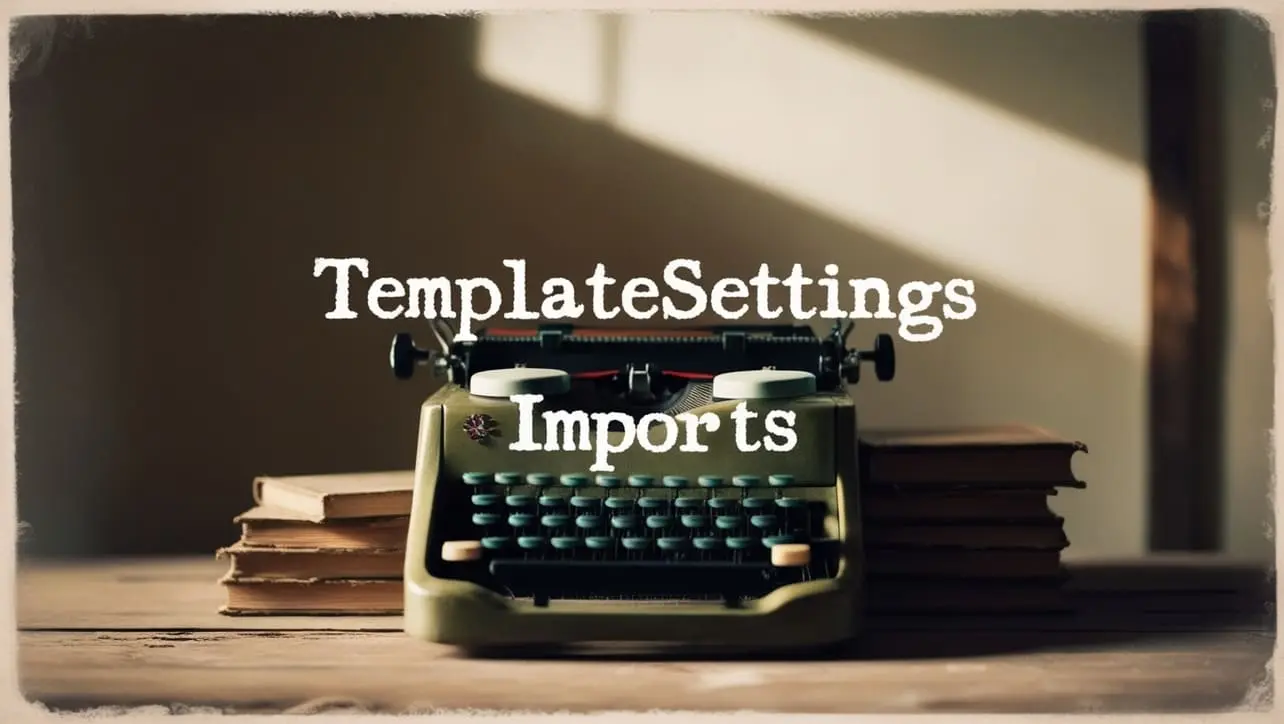
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.keysIn() Object Method
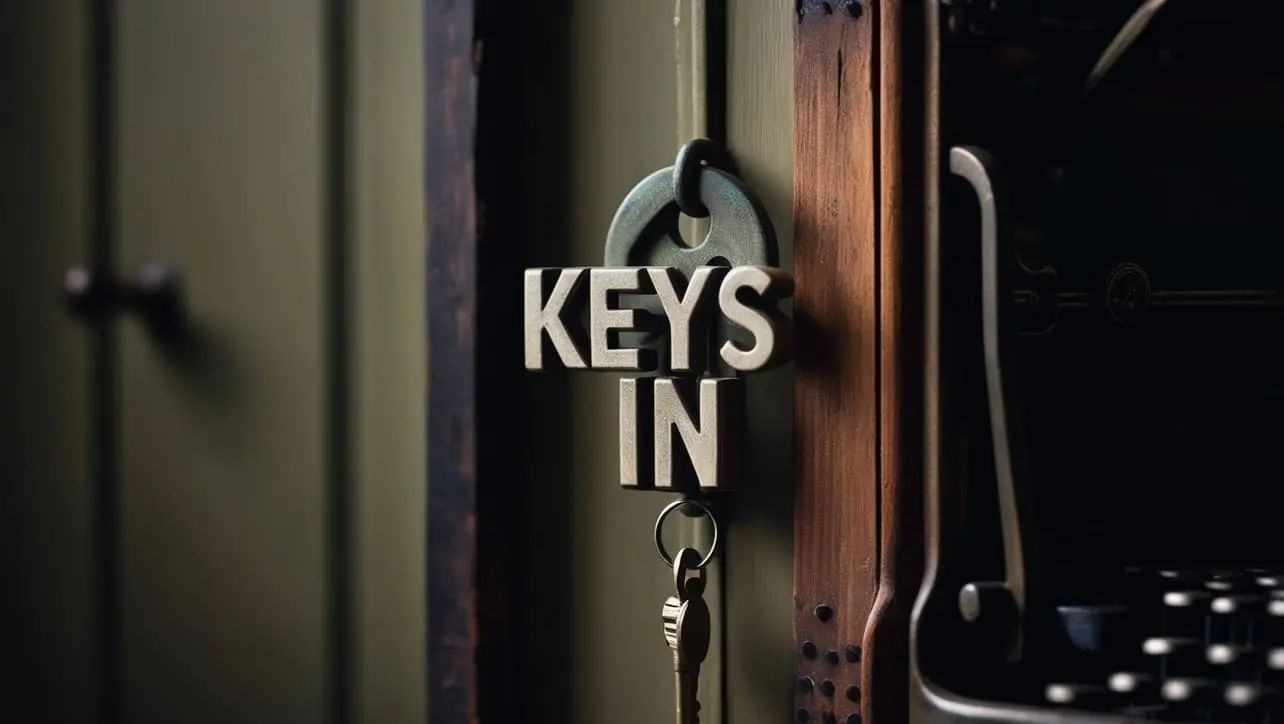
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, navigating and manipulating objects is a common task for developers. Lodash, a popular utility library, provides a plethora of functions to streamline object manipulation. Among these functions is _.keysIn()
, a versatile method that retrieves all enumerable property names of an object, including properties from its prototype chain.
Understanding and utilizing _.keysIn()
can greatly enhance your ability to work with objects in JavaScript.
🧠 Understanding _.keysIn() Method
The _.keysIn()
method in Lodash allows you to obtain an array of all enumerable property names of an object, including properties inherited from its prototype chain. This provides a comprehensive view of an object's structure, enabling developers to iterate through its properties or perform various operations.
💡 Syntax
The syntax for the _.keysIn()
method is straightforward:
_.keysIn(object)
- object: The object whose enumerable property names will be retrieved.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.keysIn()
method:
const _ = require('lodash');
function Vehicle(make, model) {
this.make = make;
this.model = model;
}
Vehicle.prototype.start = function() {
console.log('Starting the vehicle...');
};
const car = new Vehicle('Toyota', 'Camry');
const keys = _.keysIn(car);
console.log(keys);
// Output: ['make', 'model', 'start']
In this example, _.keysIn()
is used to retrieve all enumerable property names of the car object, including properties inherited from its prototype chain.
🏆 Best Practices
When working with the _.keysIn()
method, consider the following best practices:
Iterate Through Object Properties:
Utilize
_.keysIn()
to iterate through the properties of an object, enabling dynamic property access and manipulation.example.jsCopiedconst person = { name: 'John', age: 30, city: 'New York' }; _.keysIn(person).forEach(key => { console.log(`${key}: ${person[key]}`); });
Property Existence Checking:
Check for the existence of specific properties within an object using
_.keysIn()
, facilitating conditional logic and error handling.example.jsCopiedconst user = { username: 'johndoe123', email: 'johndoe@example.com' }; if(_.keysIn(user).includes('email')) { console.log('Email property exists'); } else { console.log('Email property does not exist'); }
Object Property Enumeration:
Employ
_.keysIn()
to enumerate over an object's properties and perform various operations, such as filtering or transformation.example.jsCopiedconst data = { name: 'John', age: 30, city: 'New York' }; const filteredKeys = _.keysIn(data).filter(key => key !== 'city'); console.log(filteredKeys); // Output: ['name', 'age']
📚 Use Cases
Object Property Inspection:
_.keysIn()
is invaluable for inspecting the properties of an object, providing insight into its structure and facilitating further analysis or debugging.example.jsCopiedconst student = { name: 'Alice', grade: 'A', subjects: ['Math', 'Science'] }; const properties = _.keysIn(student); console.log(properties);
Dynamic Property Access:
Dynamic property access and manipulation can be achieved using
_.keysIn()
, allowing for flexible and dynamic object handling in JavaScript applications.example.jsCopiedconst customer = { firstName: 'John', lastName: 'Doe', address: { street: '123 Main St', city: 'Anytown' } }; _.keysIn(customer.address).forEach(key => { console.log(`${key}: ${customer.address[key]}`); });
Prototype Chain Exploration:
Explore an object's prototype chain by utilizing
_.keysIn()
, gaining insights into inherited properties and their values.example.jsCopiedconst animal = { legs: 4, tail: true }; function Dog(name) { this.name = name; } Dog.prototype = animal; const myDog = new Dog('Buddy'); const dogProperties = _.keysIn(myDog); console.log(dogProperties);
🎉 Conclusion
The _.keysIn()
method in Lodash offers a convenient and powerful solution for working with object properties in JavaScript. By providing access to all enumerable property names, including those inherited from the prototype chain, _.keysIn()
empowers developers to navigate and manipulate objects with ease.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.keysIn()
method in your Lodash projects.
👨💻 Join our Community:
Author
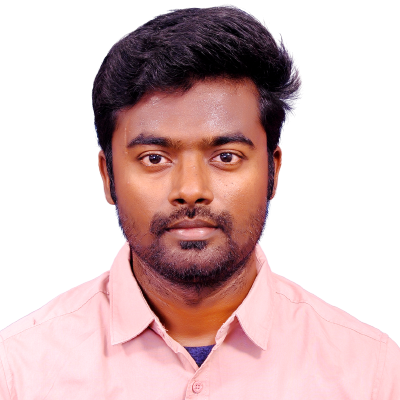
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
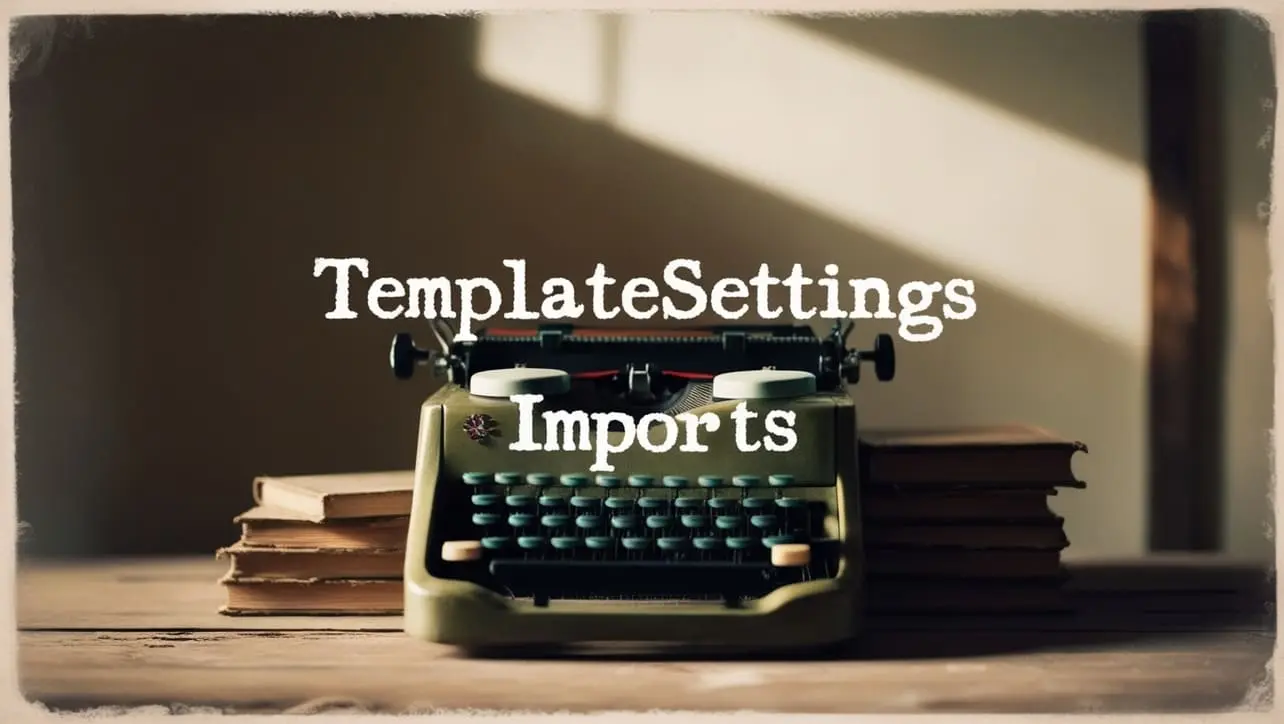
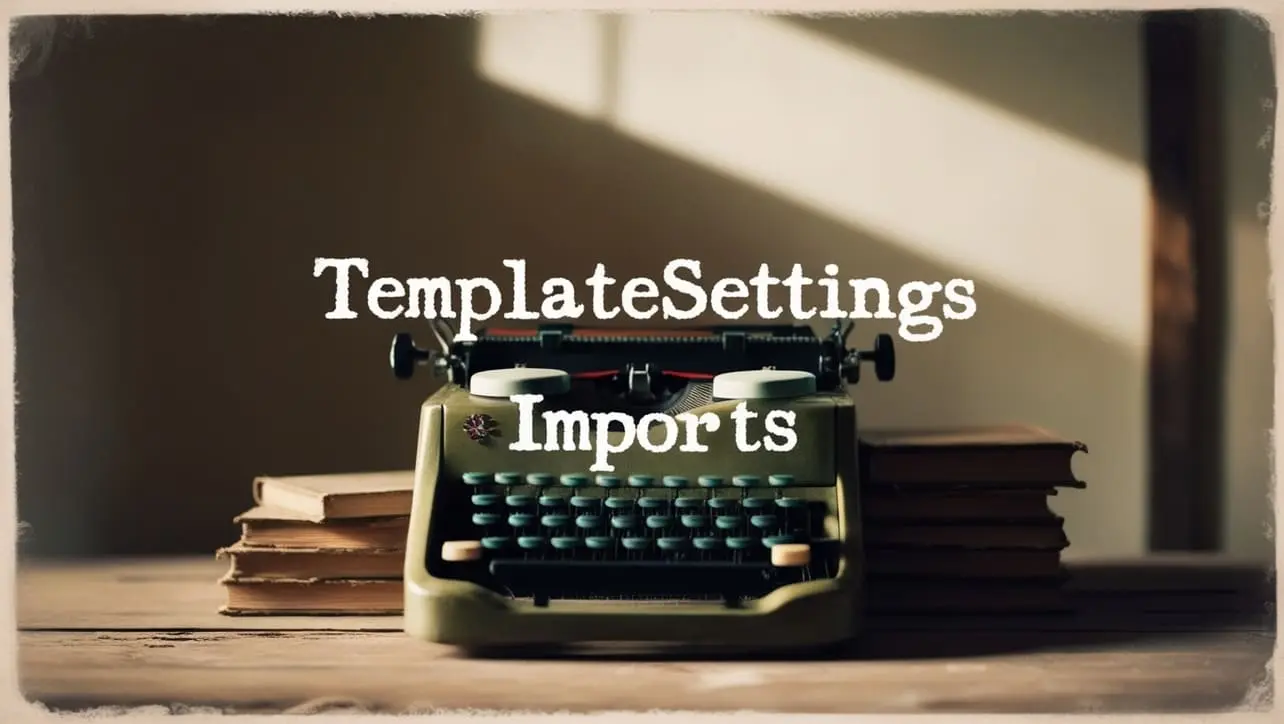
Lodash _.templateSettings.imports Property
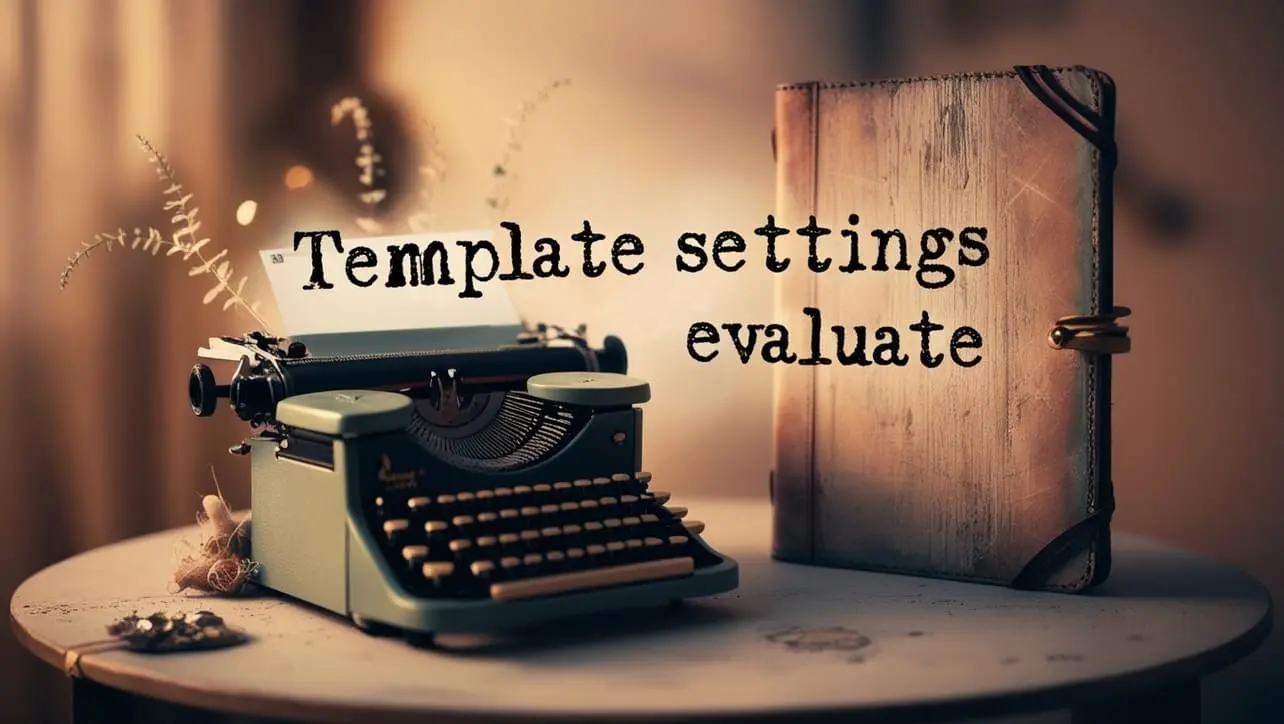
Lodash _.templateSettings.evaluate Property
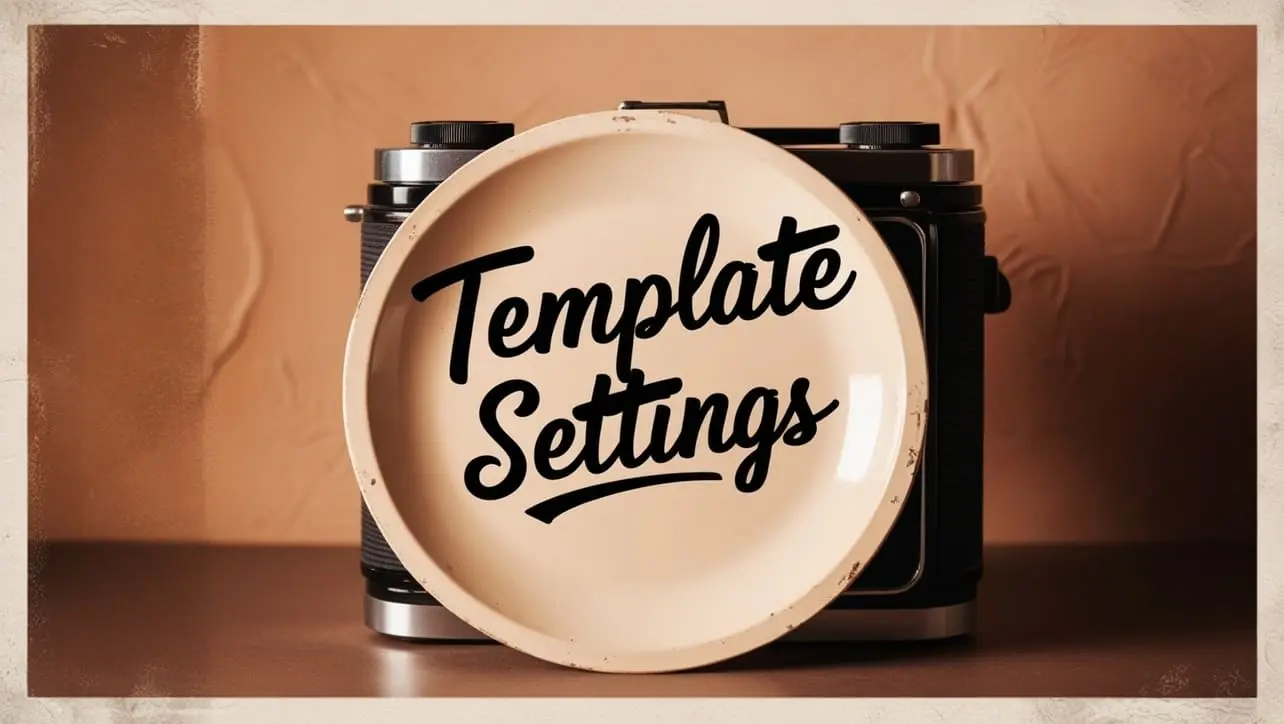
Lodash _.templateSettings Property
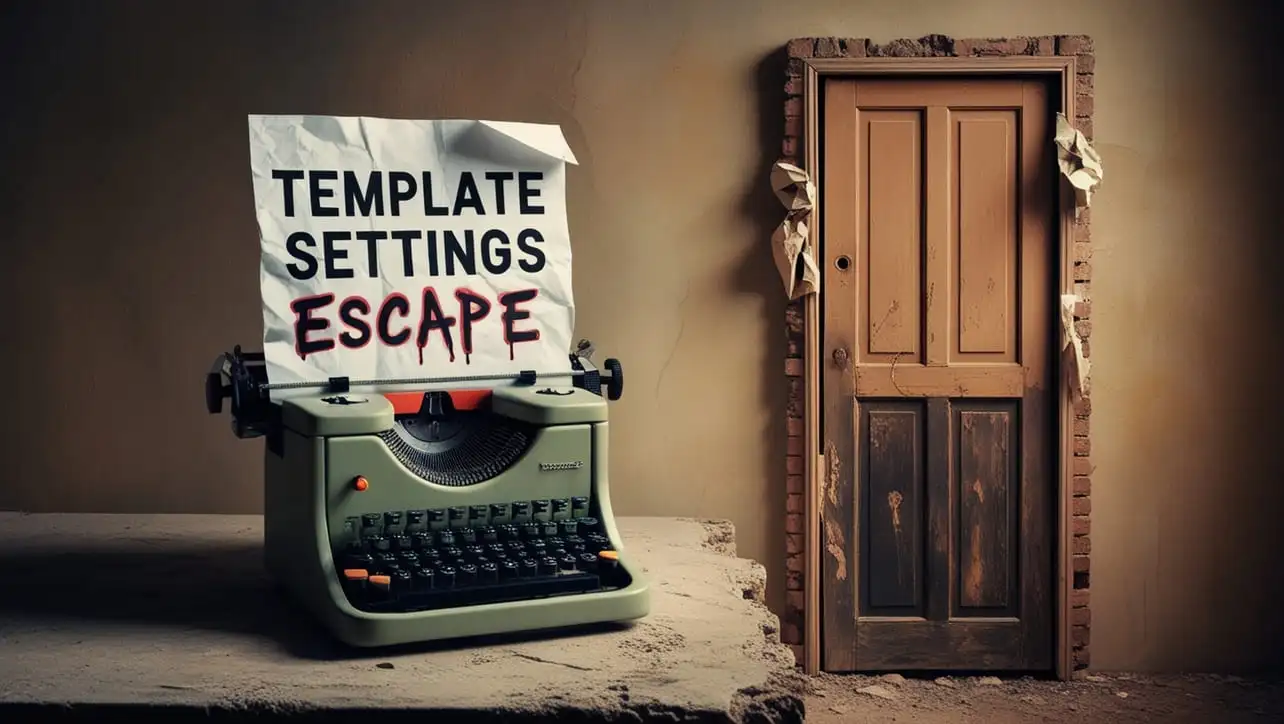
Lodash _.templateSettings.escape Property
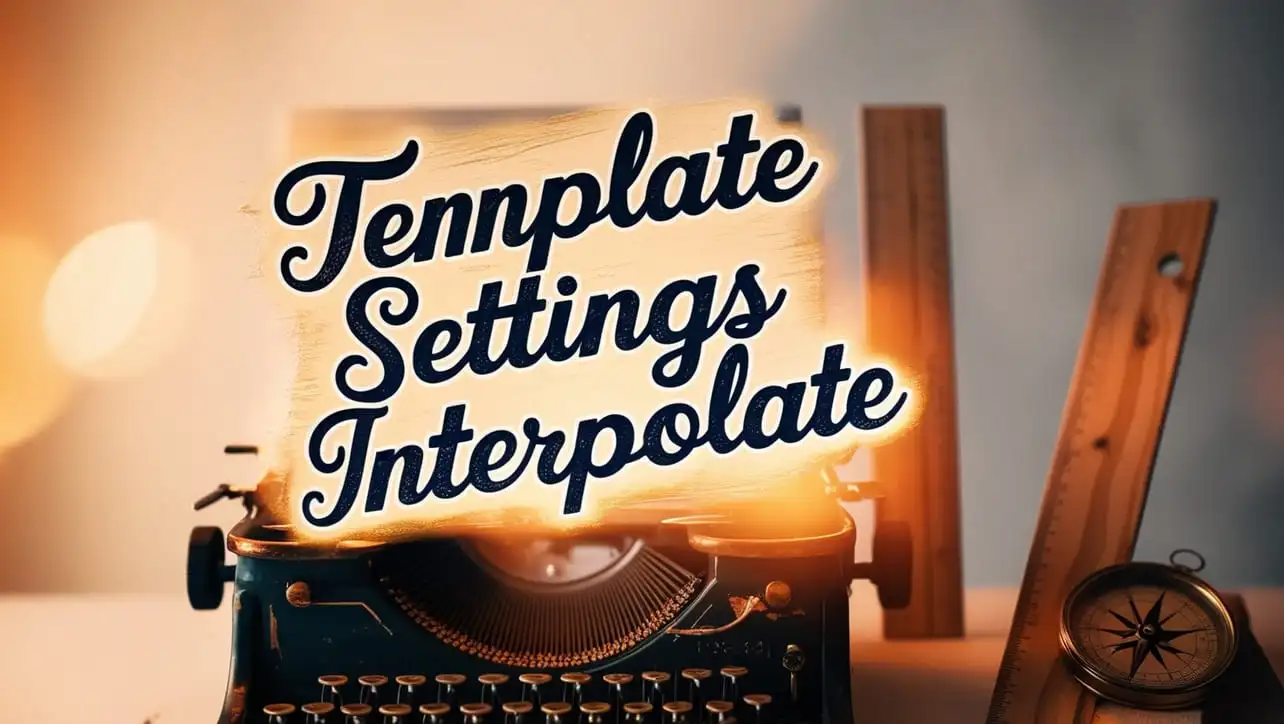
Lodash _.templateSettings.interpolate Property
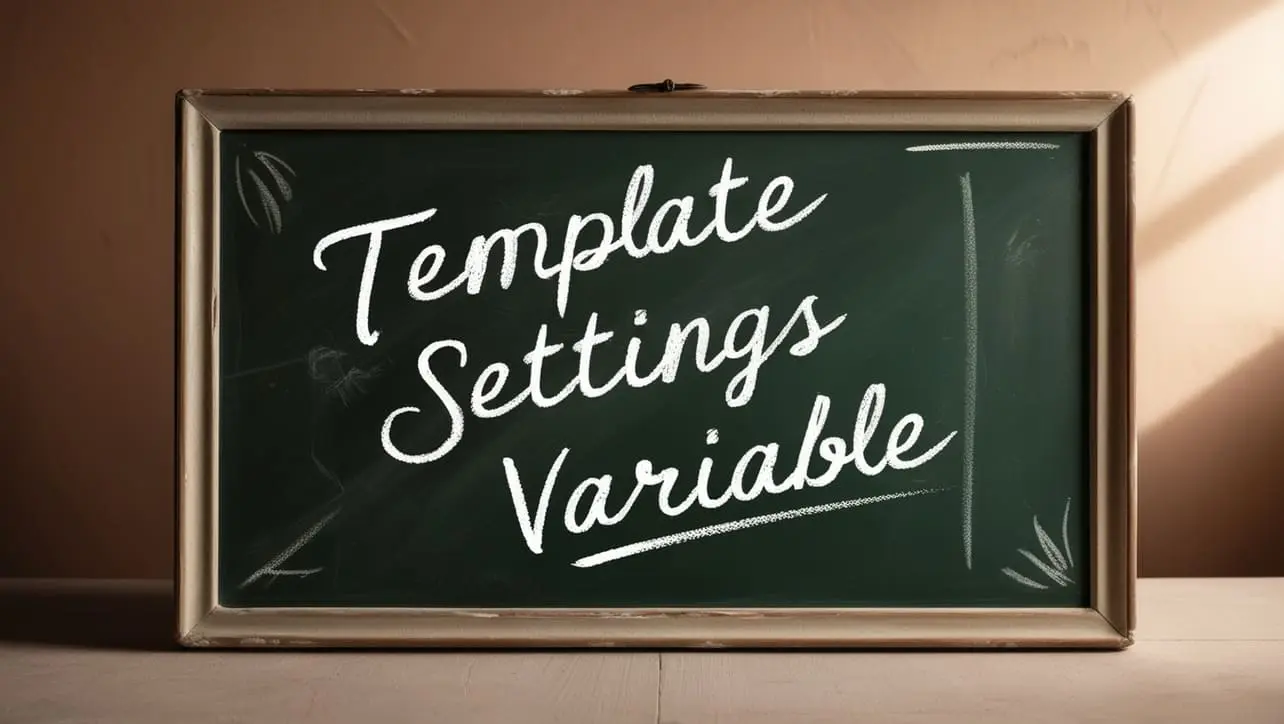
If you have any doubts regarding this article (Lodash _.keysIn() Object Method), please comment here. I will help you immediately.