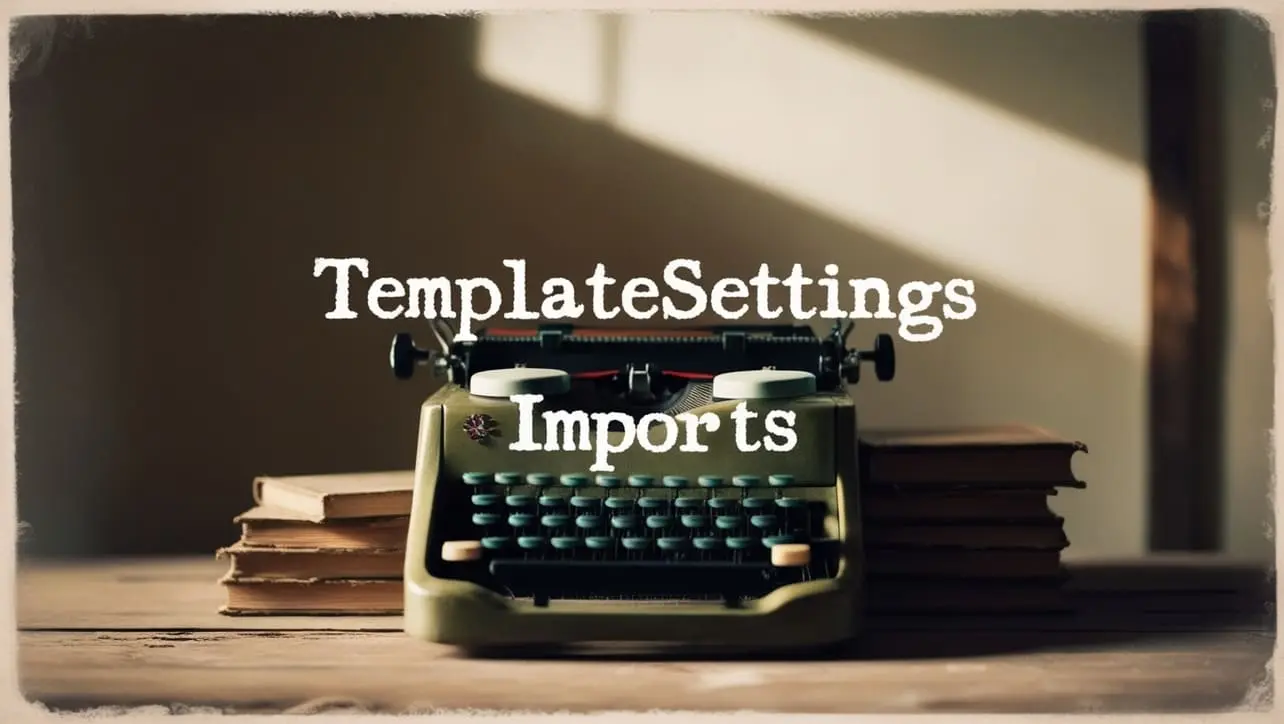
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.invoke() Object Method
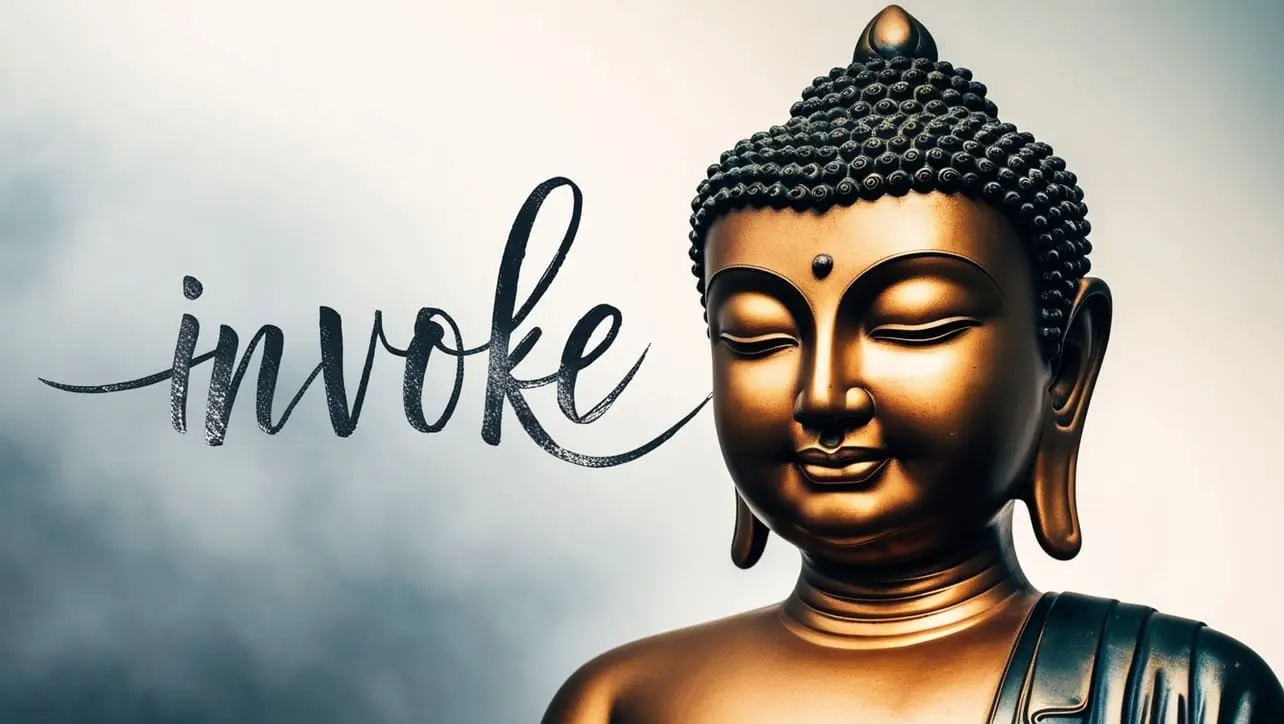
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, working with objects is commonplace. The Lodash library provides a plethora of utility functions to simplify object manipulation, and one such function is _.invoke()
.
This method allows developers to invoke a specified method on each element of an object, providing a concise and efficient way to perform actions across multiple objects.
🧠 Understanding _.invoke() Method
The _.invoke()
method in Lodash enables developers to invoke a specified method on each element of an object. This method accepts two parameters: the object to iterate over and the method to invoke on each element. By leveraging _.invoke()
, developers can streamline their code and perform consistent actions across diverse sets of objects.
💡 Syntax
The syntax for the _.invoke()
method is straightforward:
_.invoke(collection, methodName, ...args)
- collection: The object or array-like object to iterate over.
- methodName: The name of the method to invoke.
- ...args: Additional arguments to pass to the invoked method.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.invoke()
method:
const _ = require('lodash');
const myObjects = [
{
name: 'John',
greet: function() {
return `Hello, ${this.name}!`;
}
},
{
name: 'Alice',
greet: function() {
return `Hi, ${this.name}!`;
}
},
{
name: 'Bob',
greet: function() {
return `Hey, ${this.name}!`;
}
}];
const greetings = _.invoke(myObjects, 'greet');
console.log(greetings);
// Output: ['Hello, John!', 'Hi, Alice!', 'Hey, Bob!']
In this example, the greet() method is invoked on each object in the myObjects array using _.invoke()
, resulting in an array of greetings.
🏆 Best Practices
When working with the _.invoke()
method, consider the following best practices:
Ensure Method Existence:
Before using
_.invoke()
, ensure that the method exists on each object to prevent errors. Missing methods may result in unexpected behavior or runtime errors.example.jsCopiedconst myObjects = [ { name: 'John', greet: function() { return `Hello, ${this.name}!`; } }, { name: 'Alice' }, // Missing greet method { name: 'Bob', greet: function() { return `Hey, ${this.name}!`; } } ]; const greetings = _.invoke(myObjects, 'greet'); console.log(greetings); // Output: ['Hello, John!', undefined, 'Hey, Bob!']
Pass Additional Arguments:
Utilize the ...args parameter to pass additional arguments to the invoked method if required. This allows for greater flexibility and customization in method invocation.
example.jsCopiedconst myObjects = [ { name: 'John', greet: function(suffix) { return `Hello, ${this.name}${suffix}!`; } }, { name: 'Alice', greet: function(suffix) { return `Hi, ${this.name}${suffix}!`; } }, { name: 'Bob', greet: function(suffix) { return `Hey, ${this.name}${suffix}!`; } }]; const greetings = _.invoke(myObjects, 'greet', ' - How are you'); console.log(greetings); // Output: ['Hello, John - How are you!', 'Hi, Alice - How are you!', 'Hey, Bob - How are you!']
Consider Performance Implications:
Be mindful of the performance implications when using
_.invoke()
on large datasets. Iterating over a large number of objects or invoking complex methods may impact performance.example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; const results = _.invoke(largeDataset, 'processData'); console.log(results);
📚 Use Cases
Batch Processing:
_.invoke()
is useful for batch processing operations where the same method needs to be applied to multiple objects. This can streamline workflows and improve code maintainability.example.jsCopiedconst users = /* ...fetch user data from database or API... */; _.invoke(users, 'sendEmailNotification', 'New message!');
Dynamic Method Invocation:
In scenarios where the method to be invoked is determined dynamically,
_.invoke()
provides a convenient solution. This enables dynamic method invocation based on runtime conditions.example.jsCopiedconst shapes = /* ...fetch shape objects from data source... */; const methodToInvoke = userPreference === 'area' ? 'calculateArea' : 'calculatePerimeter'; const results = _.invoke(shapes, methodToInvoke);
Object Transformation:
When transforming objects by applying a common operation, such as formatting or validation,
_.invoke()
can simplify the transformation process and improve code readability.example.jsCopiedconst data = /* ...fetch raw data from external source... */; const transformedData = _.invoke(data, 'transform');
🎉 Conclusion
The _.invoke()
method in Lodash offers a powerful mechanism for invoking a specified method on each element of an object. Whether you're performing batch processing, dynamic method invocation, or object transformation, _.invoke()
provides a versatile and efficient solution for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.invoke()
method in your Lodash projects.
👨💻 Join our Community:
Author
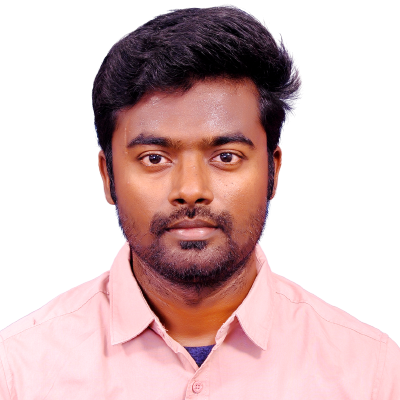
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
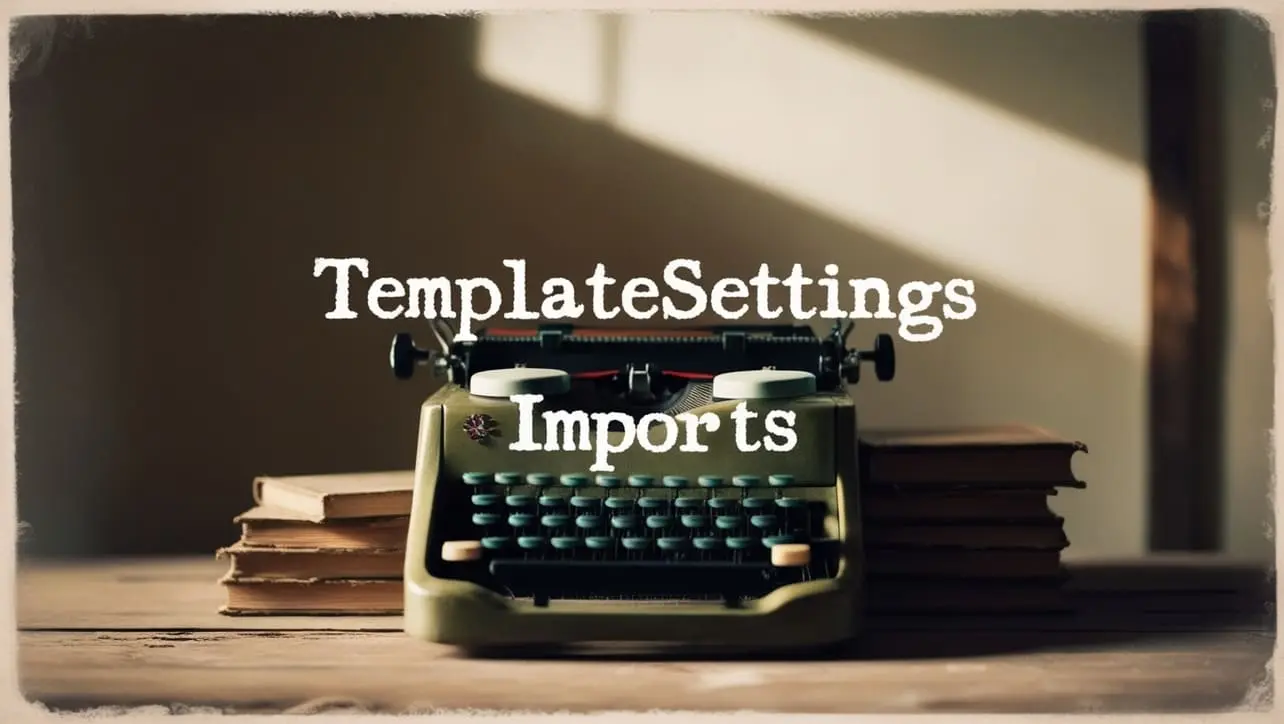
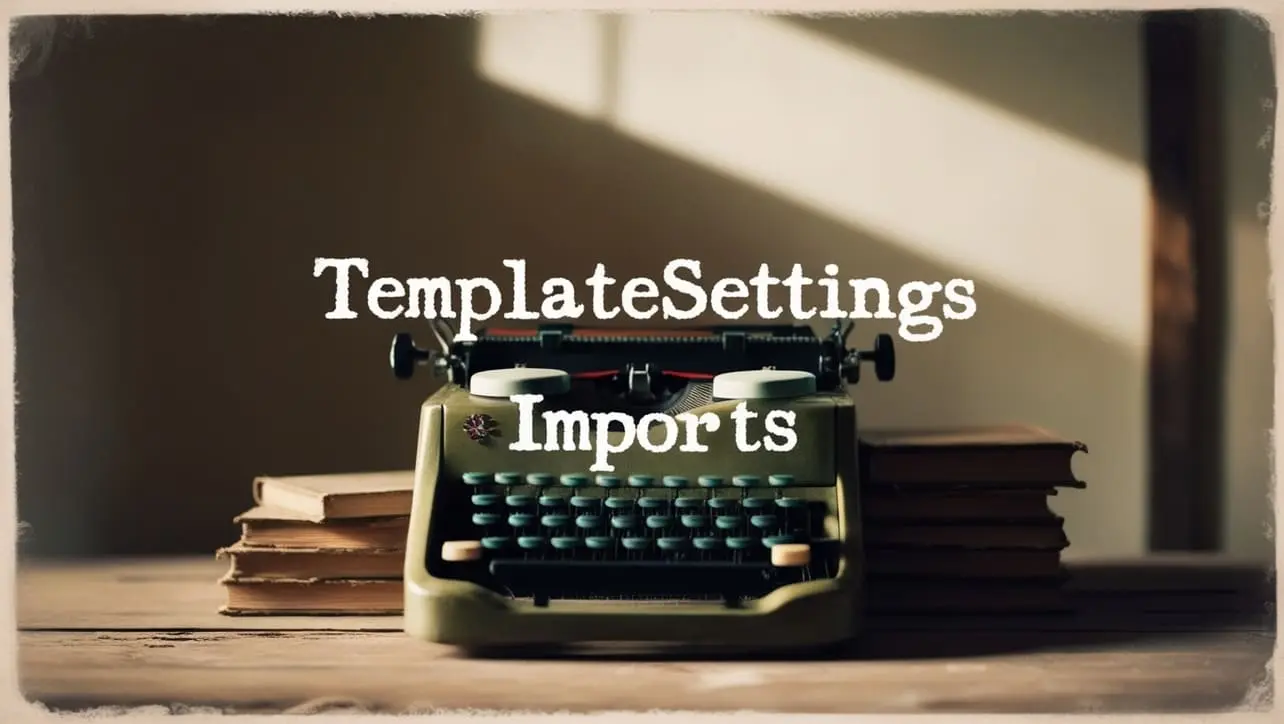
Lodash _.templateSettings.imports Property
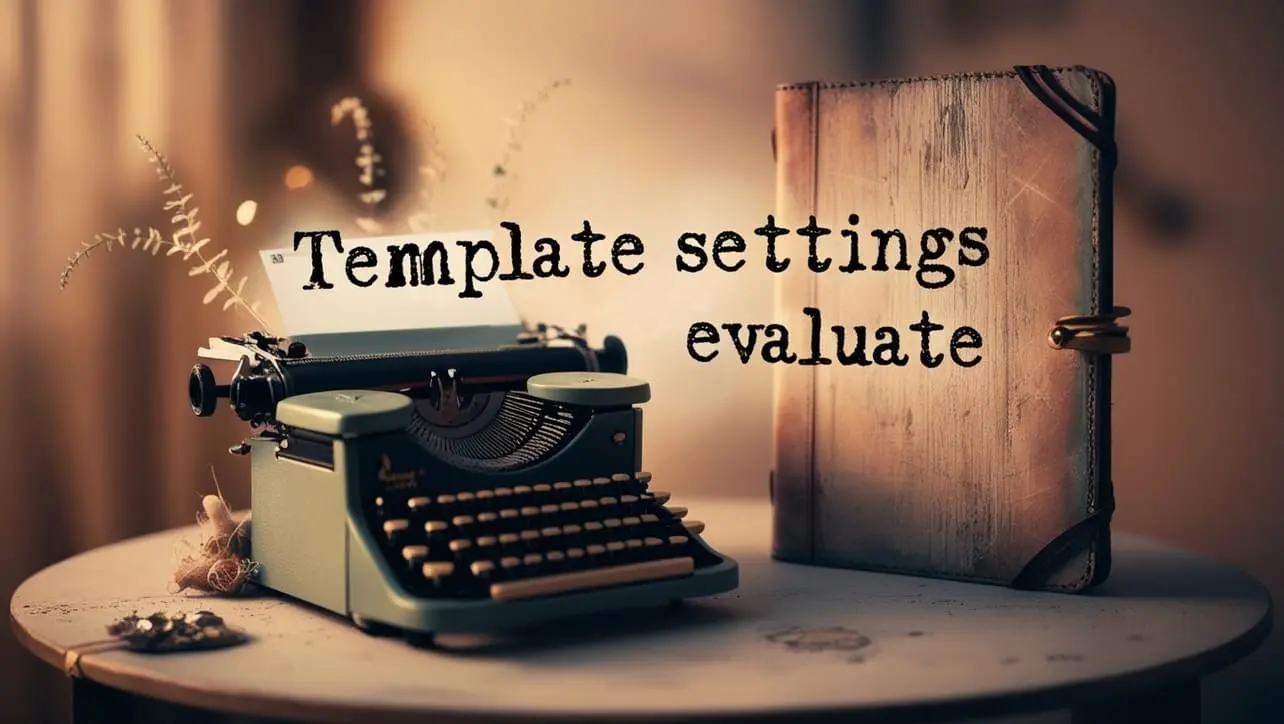
Lodash _.templateSettings.evaluate Property
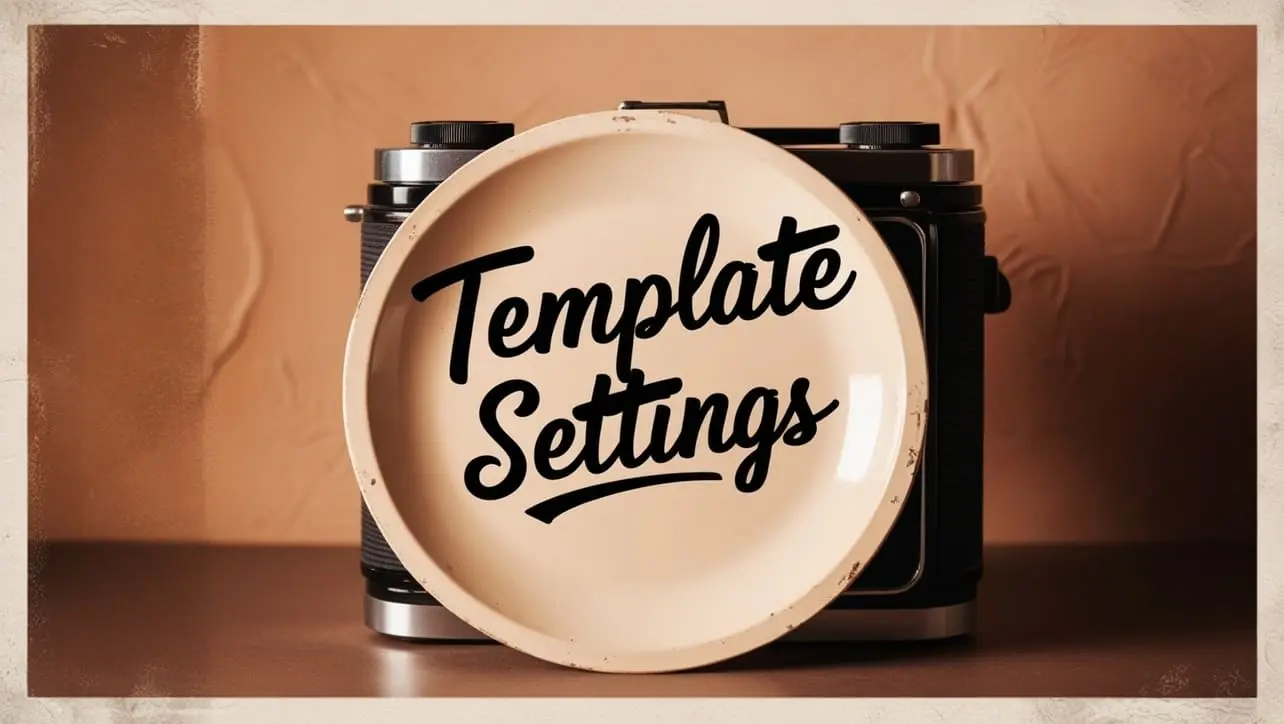
Lodash _.templateSettings Property
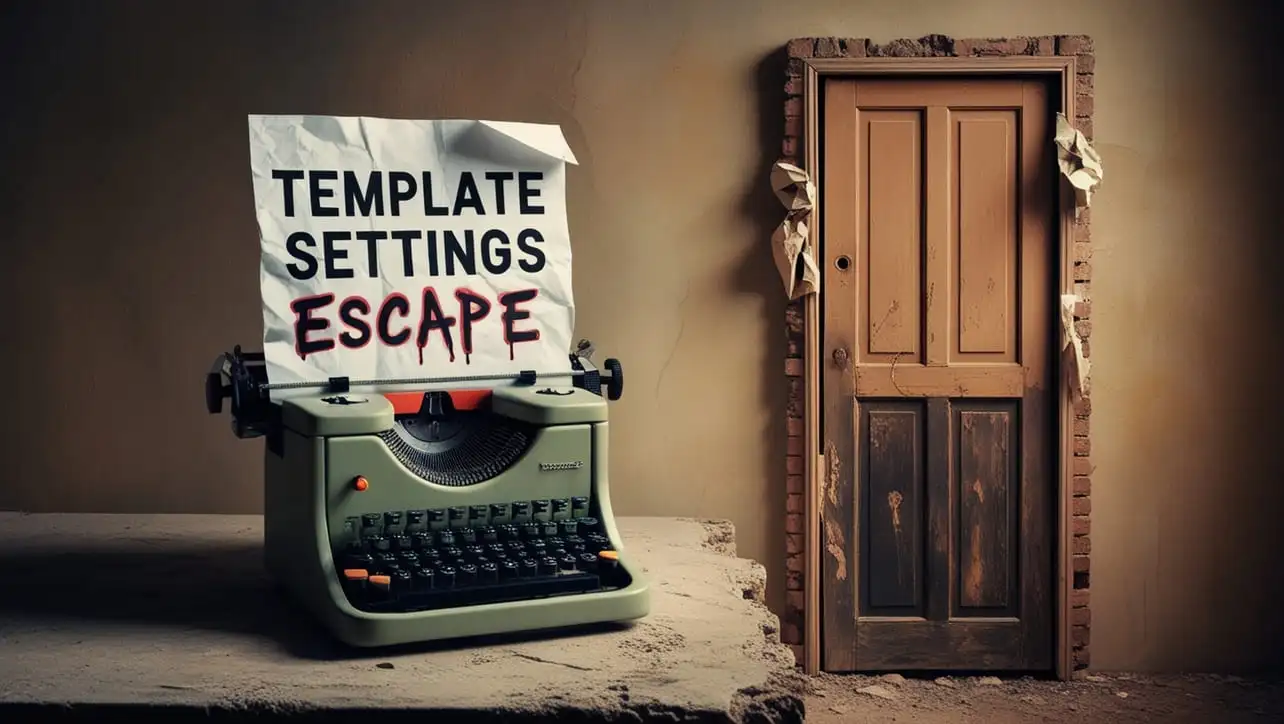
Lodash _.templateSettings.escape Property
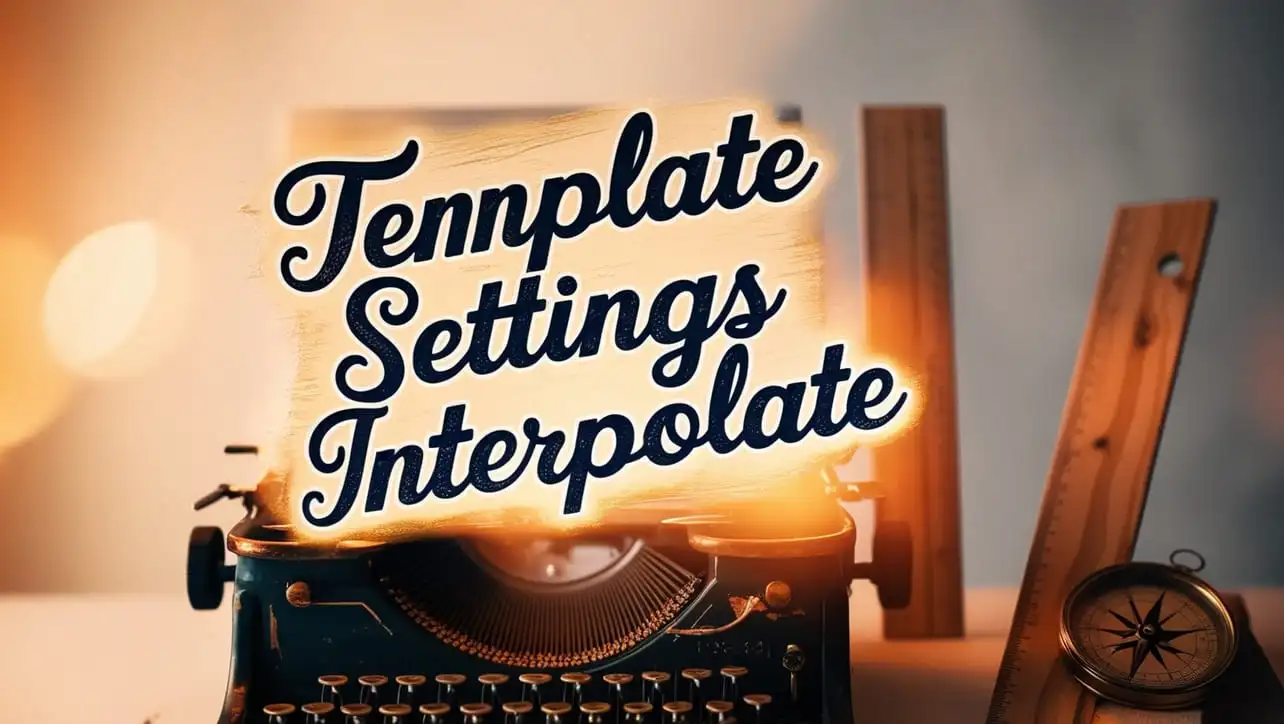
Lodash _.templateSettings.interpolate Property
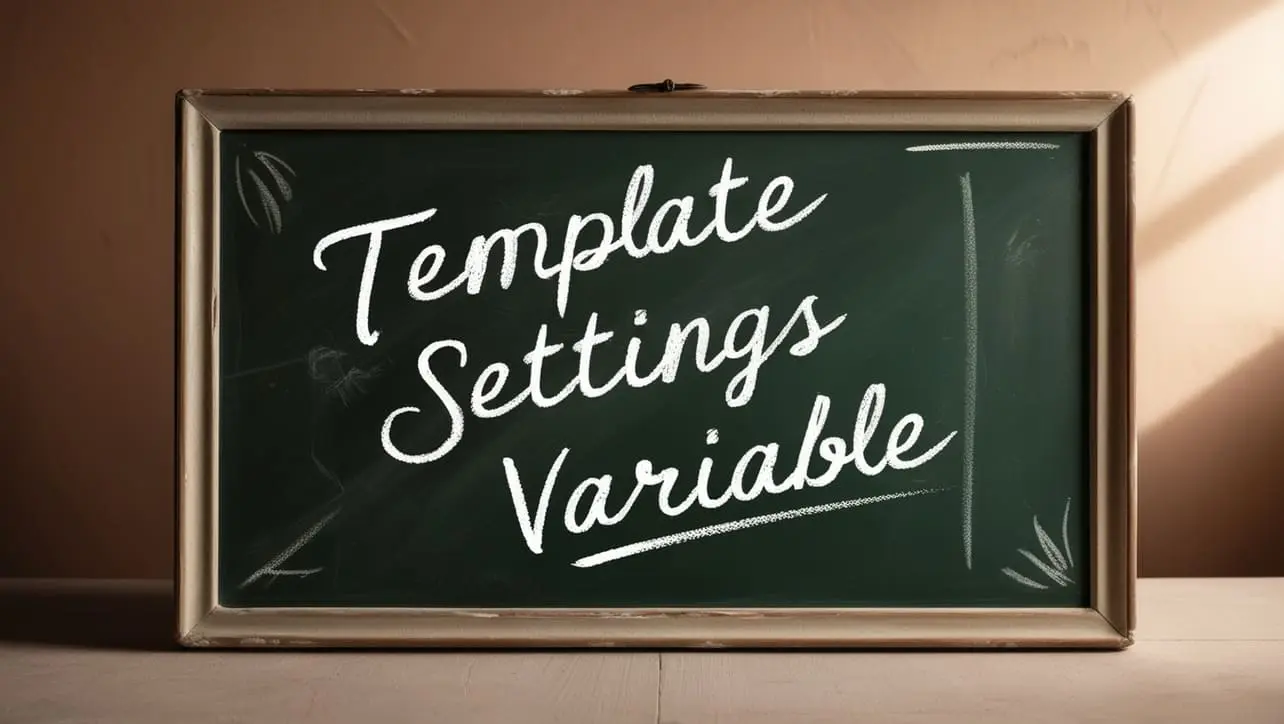
If you have any doubts regarding this article (Lodash _.invoke() Object Method), please comment here. I will help you immediately.