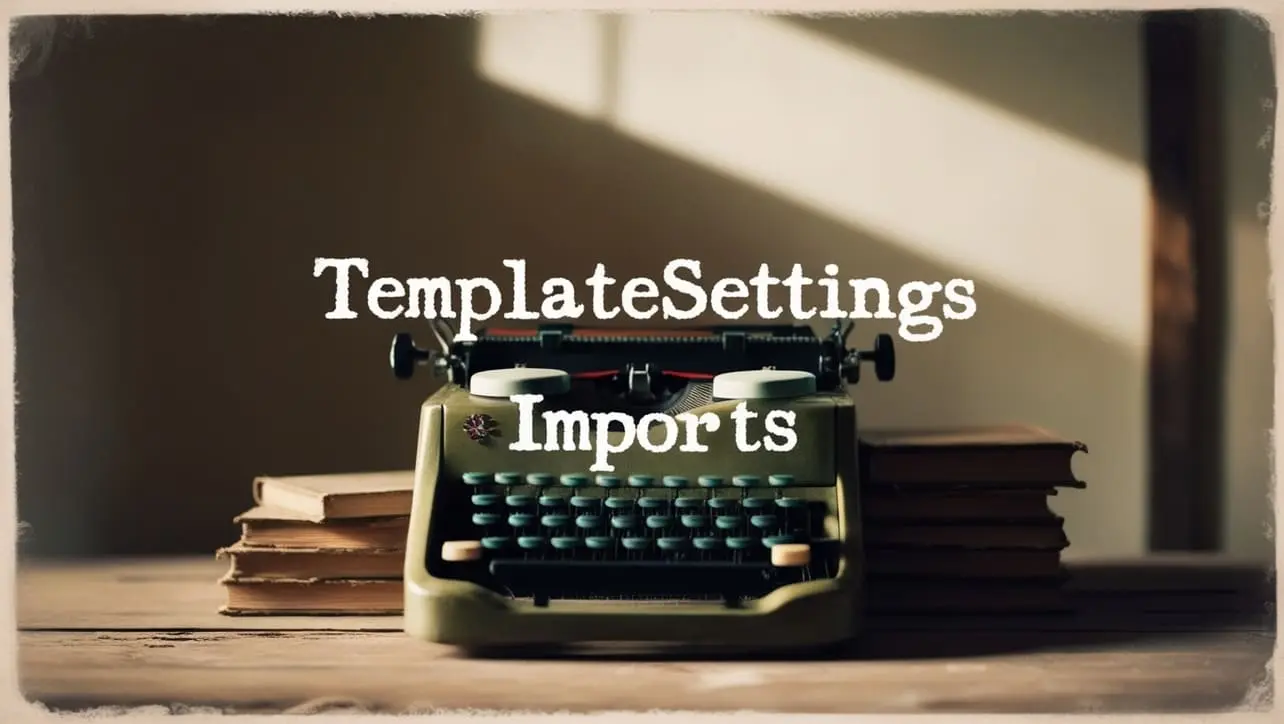
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.hasIn() Object Method
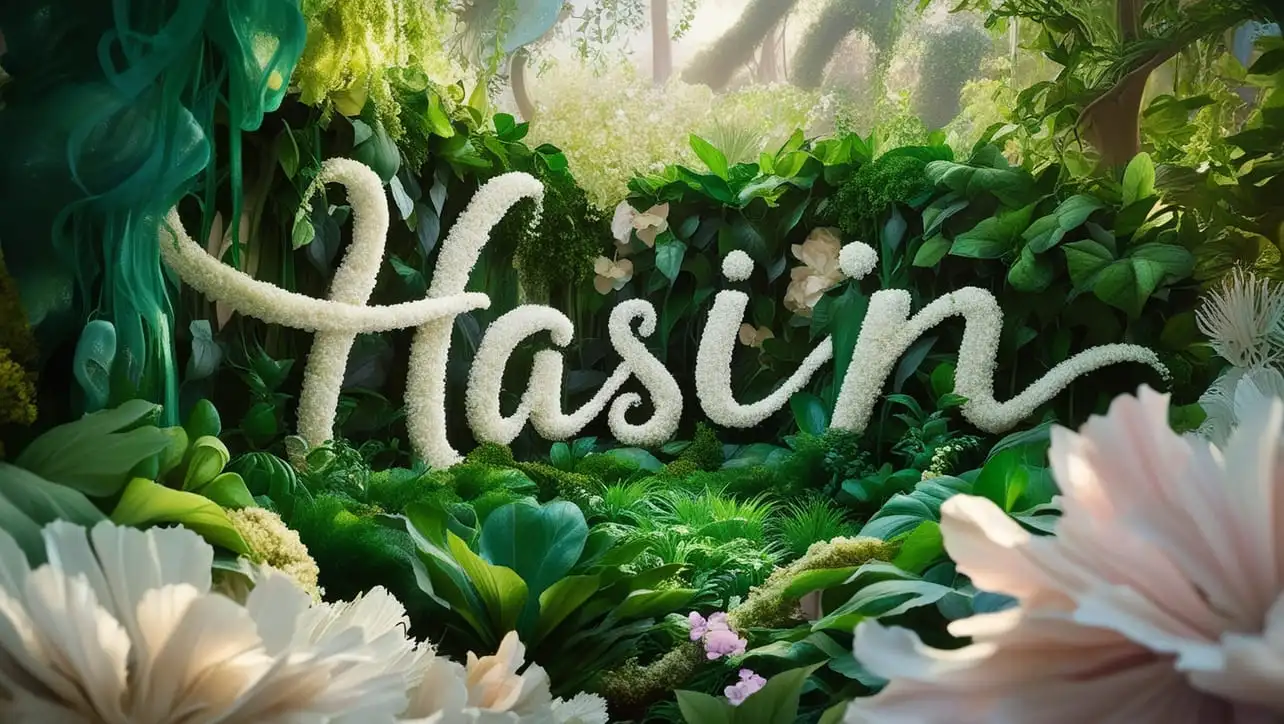
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, working with objects is a fundamental aspect of creating robust and dynamic applications. Lodash, a powerful utility library, provides a plethora of functions to simplify object manipulation. Among these functions is the _.hasIn()
method, a versatile tool for checking if a nested property exists within an object.
This method proves invaluable when dealing with complex data structures and dynamic object properties.
🧠 Understanding _.hasIn() Method
The _.hasIn()
method in Lodash is designed to determine whether a specified path exists in an object. Unlike _.has(), which checks for direct property existence, _.hasIn()
traverses nested properties, allowing you to verify the existence of deeply nested keys within an object.
💡 Syntax
The syntax for the _.hasIn()
method is straightforward:
_.hasIn(object, path)
- object: The object to query.
- path: The path to check for existence.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.hasIn()
method:
const _ = require('lodash');
const sampleObject = {
user: {
details: {
name: 'John Doe',
age: 30,
},
role: 'admin',
},
status: 'active',
};
const hasNestedProperty = _.hasIn(sampleObject, 'user.details.age');
console.log(hasNestedProperty);
// Output: true
In this example, _.hasIn()
checks if the nested property user.details.age exists within the sampleObject.
🏆 Best Practices
When working with the _.hasIn()
method, consider the following best practices:
Path Existence Check:
Use
_.hasIn()
to perform path existence checks on nested properties. This method is particularly beneficial when dealing with dynamic data structures where the existence of a property might vary.example.jsCopiedconst dynamicObject = /* ...generate object dynamically... */; const hasDynamicProperty = _.hasIn(dynamicObject, 'nestedProperty.subProperty'); console.log(hasDynamicProperty);
Safeguarding Property Access:
Before accessing a nested property, employ
_.hasIn()
to ensure that the path exists. This helps prevent runtime errors when trying to access properties that may not be present.example.jsCopiedconst userPreferences = { settings: { theme: 'dark', language: 'en', }, }; const requestedProperty = 'settings.timezone'; if(_.hasIn(userPreferences, requestedProperty)) { const timezone = _.get(userPreferences, requestedProperty); console.log('Timezone:', timezone); } else { console.log('Requested property does not exist.'); }
Default Values:
Combine
_.hasIn()
with _.get() to provide default values for nested properties that may not exist. This ensures graceful fallbacks when accessing potentially absent properties.example.jsCopiedconst websiteConfig = { appearance: { colors: { primary: 'blue', }, }, }; const requestedColor = 'appearance.colors.secondary'; const color = _.get(websiteConfig, requestedColor, 'defaultColor'); console.log('Selected Color:', color);
📚 Use Cases
Dynamic Data Structures:
When working with dynamic data structures or data fetched from external sources,
_.hasIn()
is invaluable for checking the existence of specific paths within an object.example.jsCopiedconst fetchedData = /* ...fetch data from API or elsewhere... */; const hasRequiredPath = _.hasIn(fetchedData, 'user.preferences.notifications'); console.log(hasRequiredPath);
Configurations and Settings:
In scenarios where applications have configuration objects or settings,
_.hasIn()
can be used to validate the existence of specific configuration parameters.example.jsCopiedconst appConfig = { features: { analytics: { enabled: true, trackingId: 'UA-123456789', }, }, }; const hasAnalyticsEnabled = _.hasIn(appConfig, 'features.analytics.enabled'); console.log('Analytics Enabled:', hasAnalyticsEnabled);
Form Validation:
For applications with dynamic forms,
_.hasIn()
can assist in form validation by checking if the expected form fields are present in the form data.example.jsCopiedconst formData = /* ...user-submitted form data... */; const requiredFieldPaths = ['user.details.name', 'user.details.email', 'user.preferences']; const allFieldsPresent = requiredFieldPaths.every(path => _.hasIn(formData, path)); console.log('All required fields present:', allFieldsPresent);
🎉 Conclusion
The _.hasIn()
method in Lodash is a valuable asset for JavaScript developers dealing with objects, especially in scenarios involving nested or dynamic data structures. Whether you're performing path existence checks, safeguarding property access, or handling dynamic configurations, _.hasIn()
provides a reliable solution for navigating and validating object properties.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.hasIn()
method in your Lodash projects.
👨💻 Join our Community:
Author
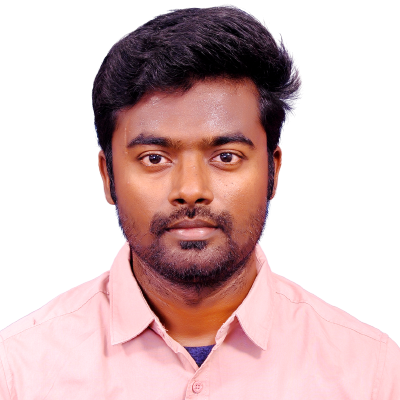
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
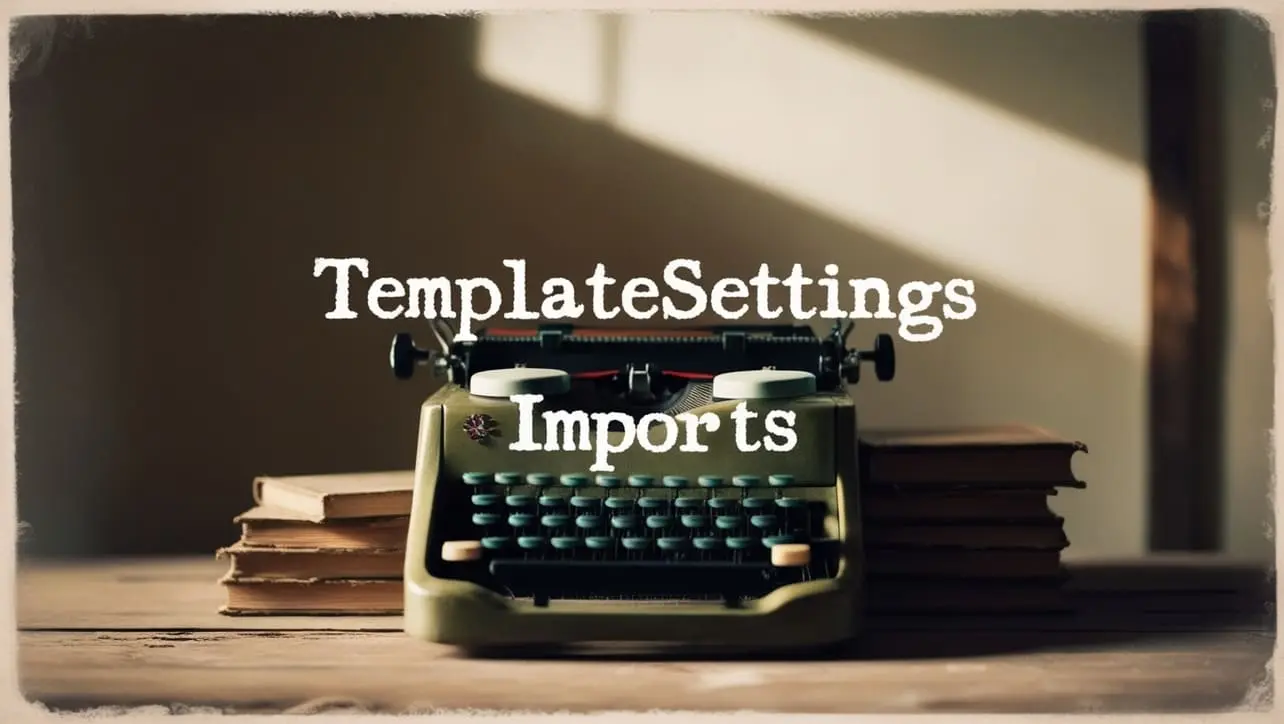
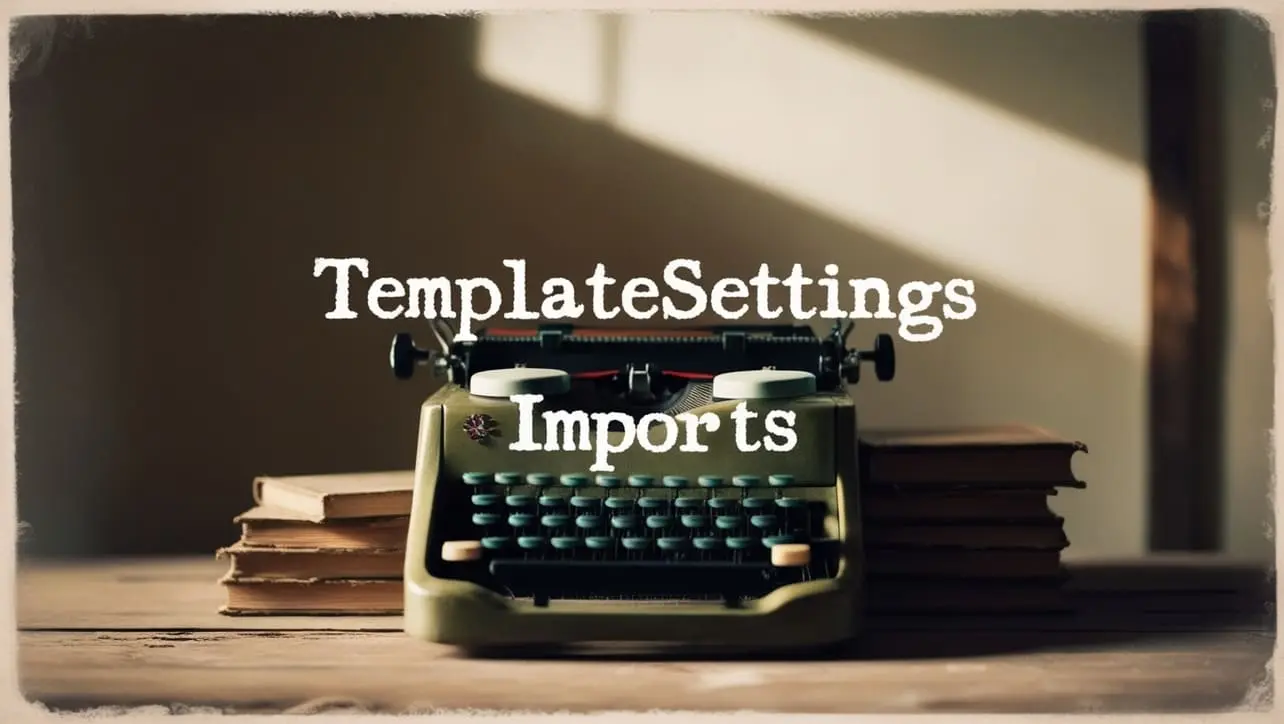
Lodash _.templateSettings.imports Property
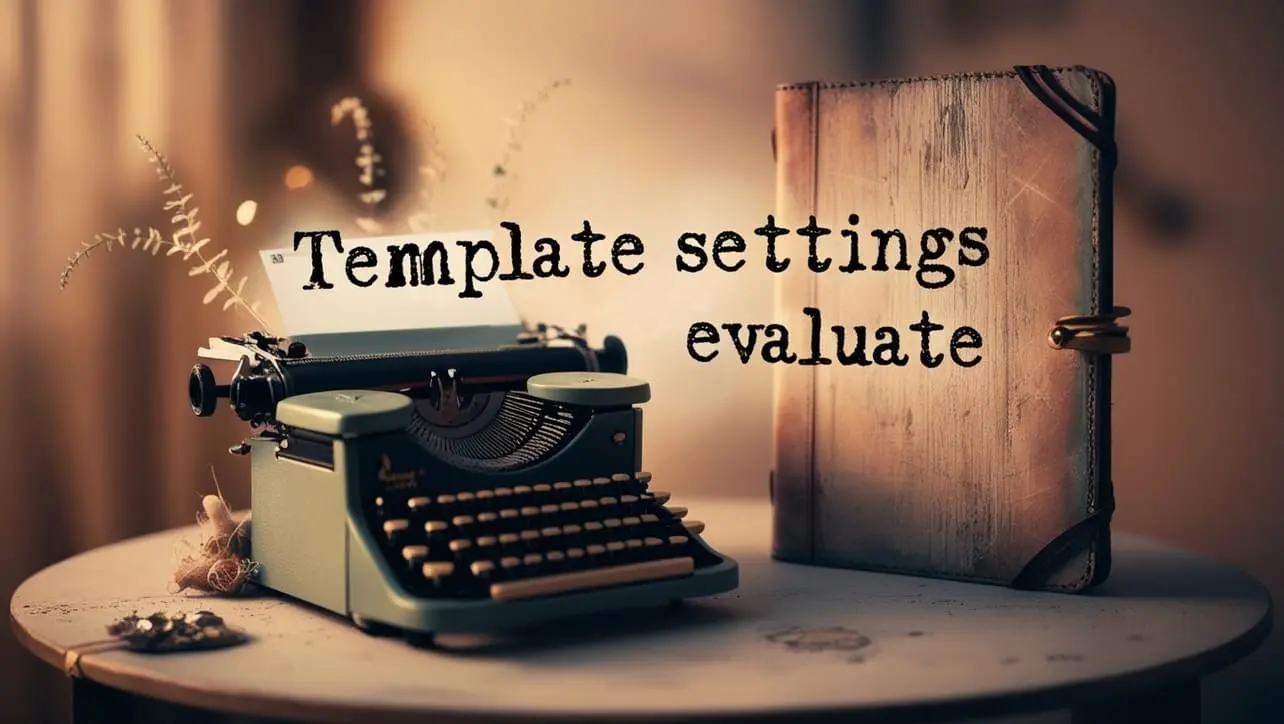
Lodash _.templateSettings.evaluate Property
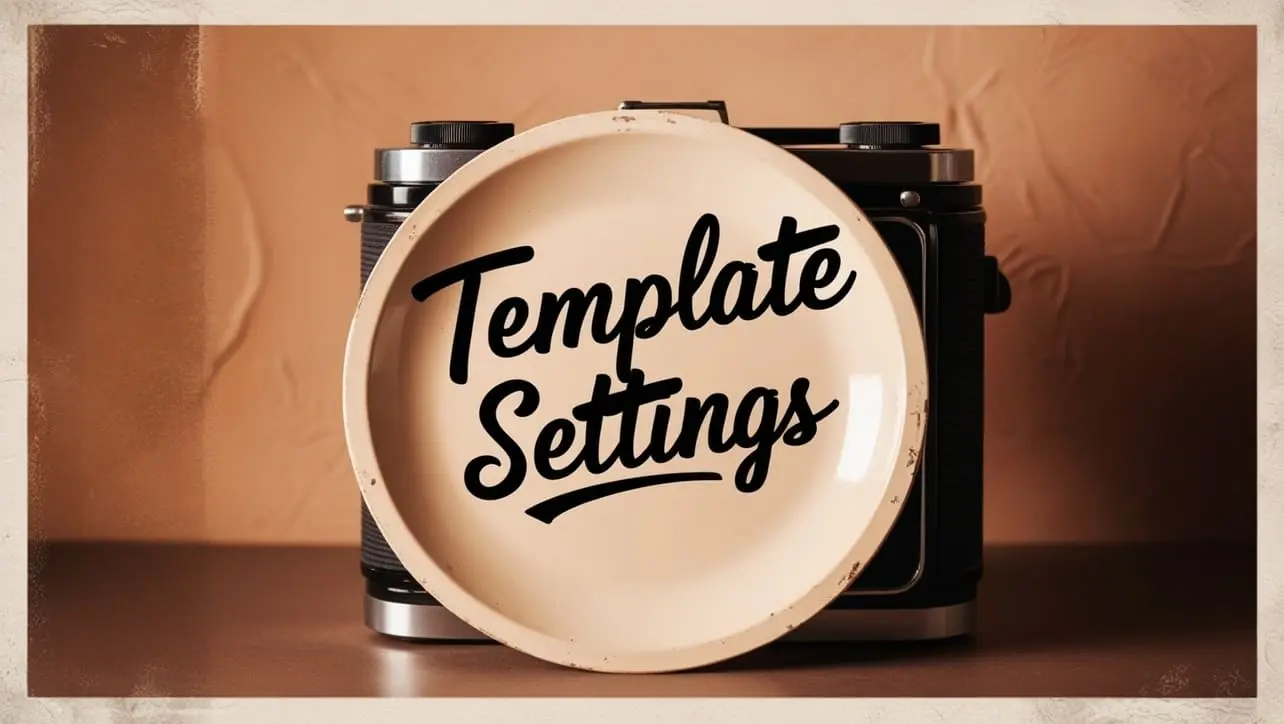
Lodash _.templateSettings Property
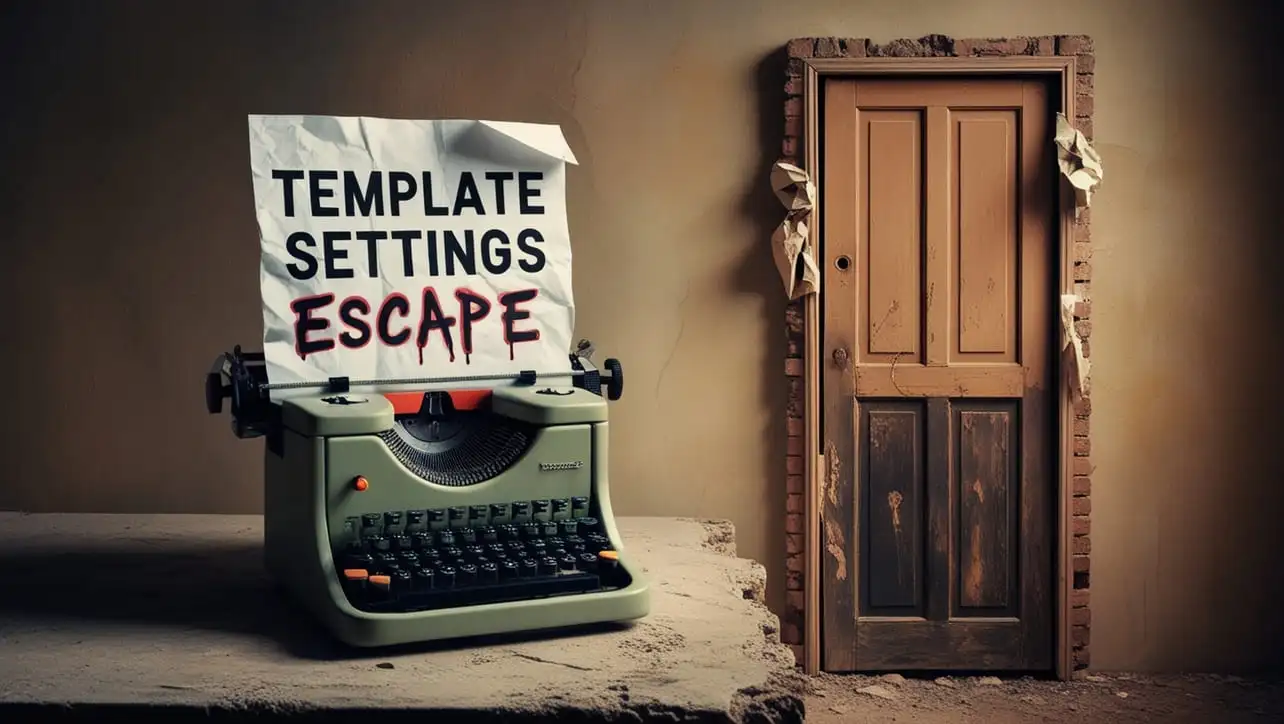
Lodash _.templateSettings.escape Property
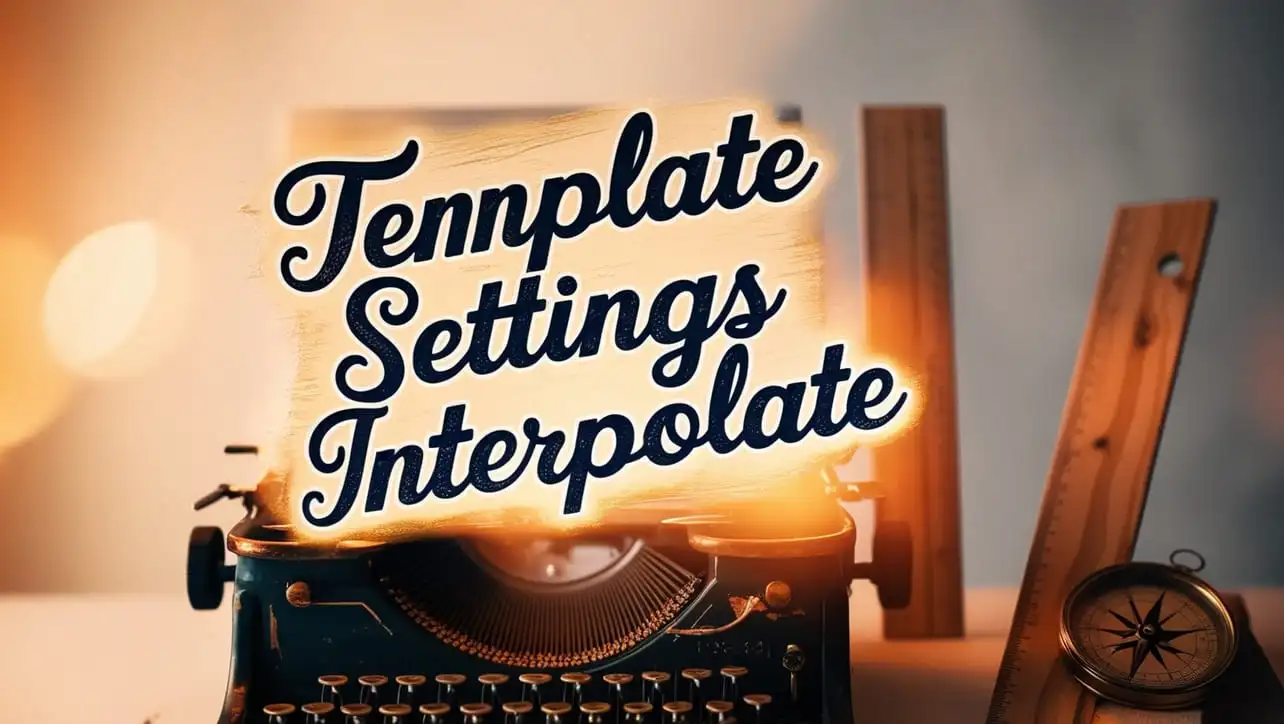
Lodash _.templateSettings.interpolate Property
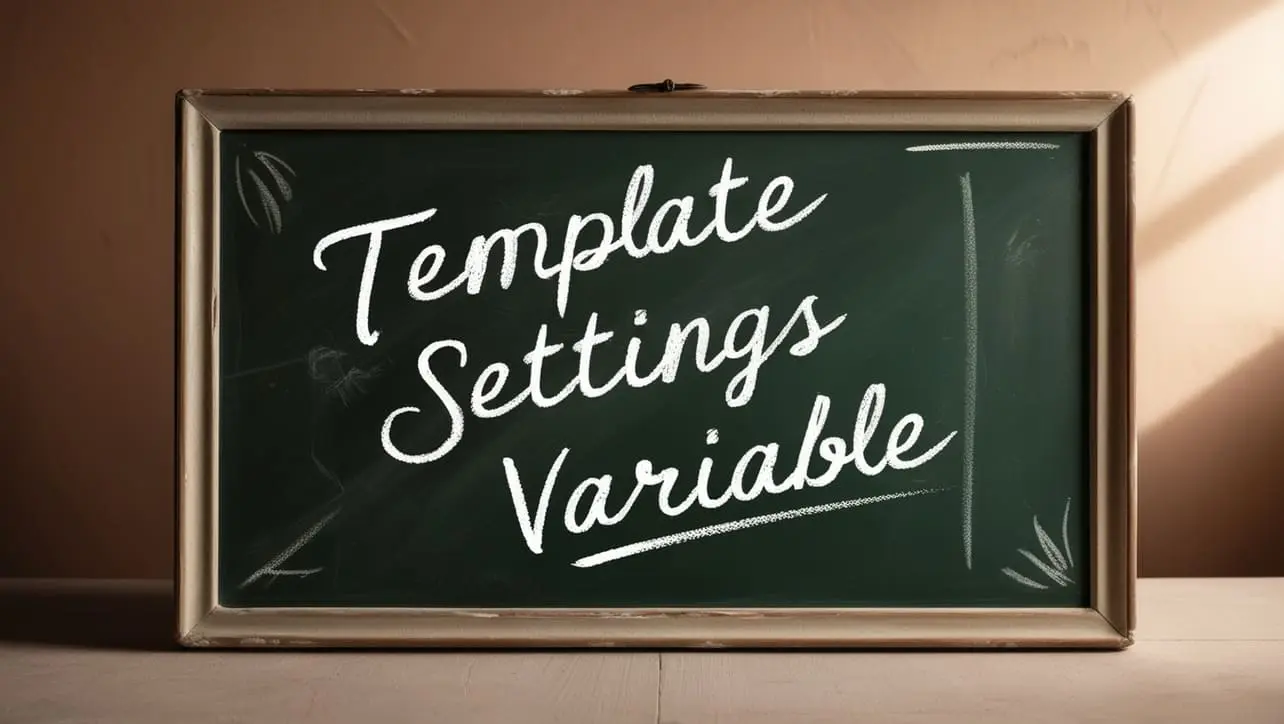
If you have any doubts regarding this article (Lodash _.hasIn() Object Method), please comment here. I will help you immediately.