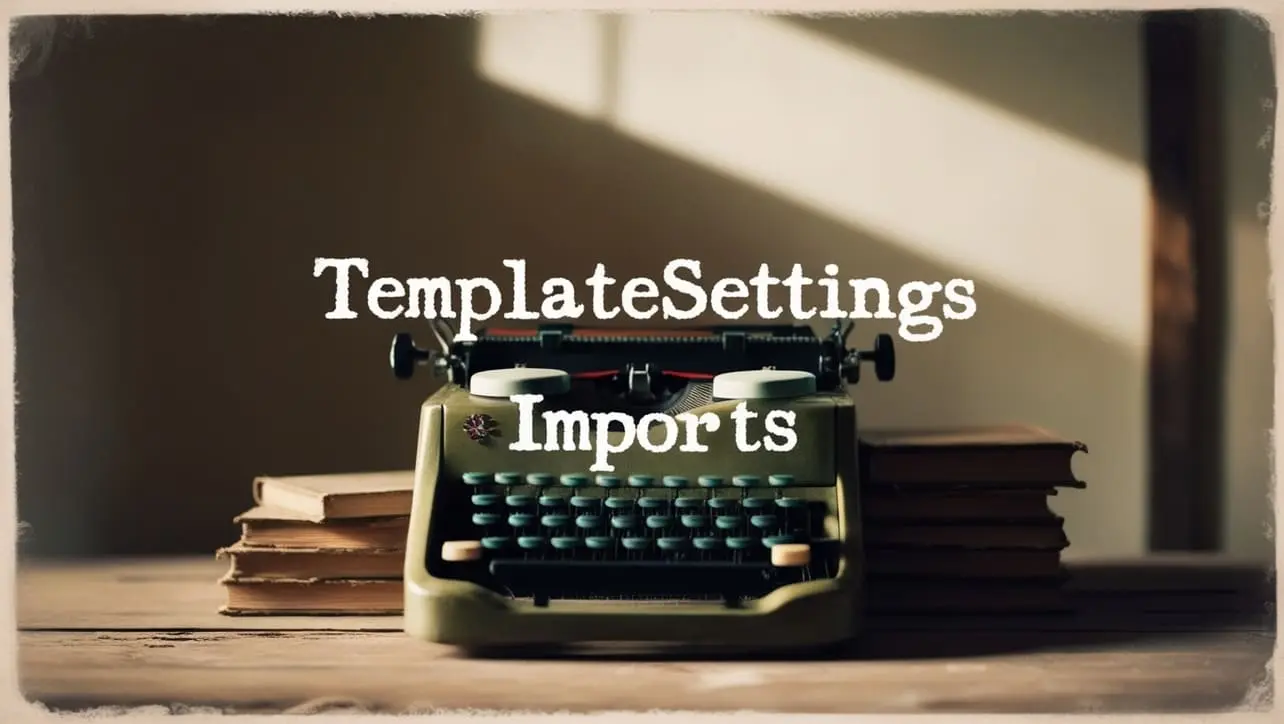
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.get() Object Method
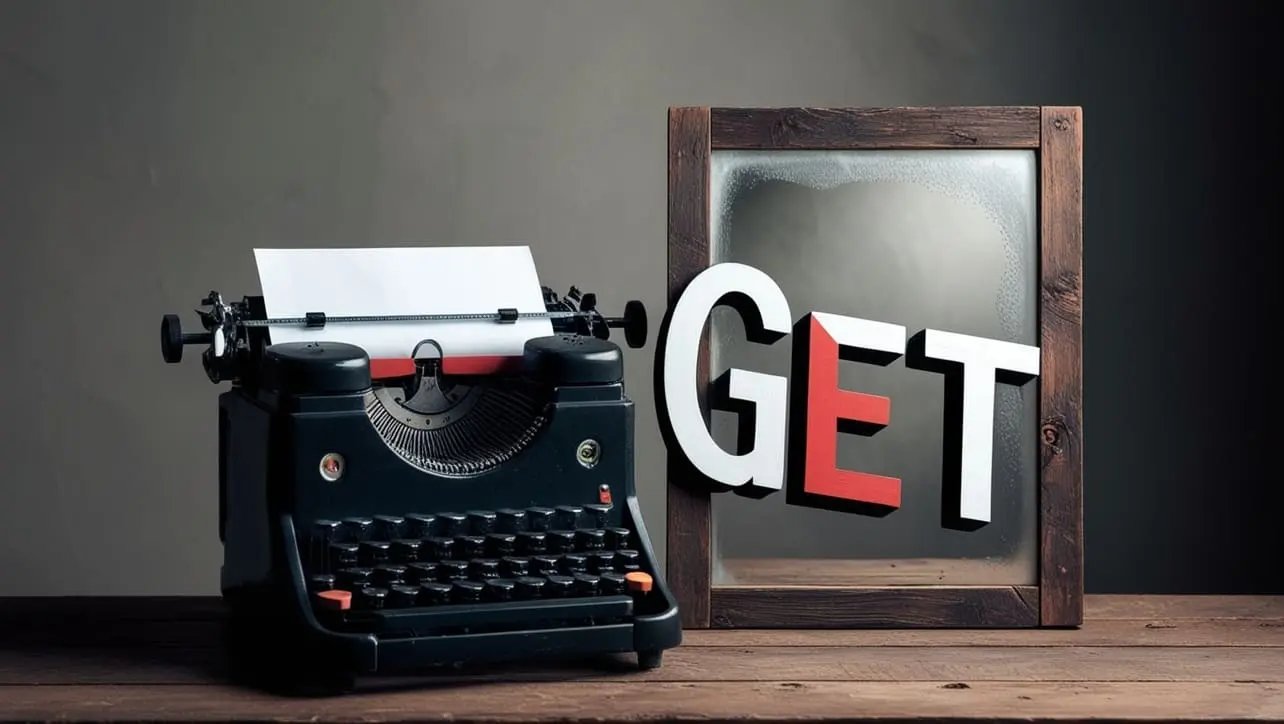
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, working with complex nested objects is a common challenge. Lodash, a versatile utility library, provides a solution to this problem with the _.get()
method.
This method simplifies the process of accessing nested properties within objects, offering a concise and reliable way to navigate through deeply nested structures.
🧠 Understanding _.get() Method
The _.get()
method in Lodash allows you to safely retrieve the value of a nested property within an object. It handles cases where intermediate properties may be undefined, preventing common pitfalls associated with direct property access.
💡 Syntax
The syntax for the _.get()
method is straightforward:
_.get(object, path, [defaultValue])
- object: The object to query.
- path: The path of the property to get.
- defaultValue (Optional): The value returned if the resolved value is undefined.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.get()
method:
const _ = require('lodash');
const nestedObject = {
user: {
details: {
name: 'John Doe',
age: 30,
},
preferences: {
theme: 'dark',
},
},
};
const userName = _.get(nestedObject, 'user.details.name', 'Unknown');
console.log(userName);
// Output: John Doe
In this example, the _.get()
method is used to safely retrieve the nested property user.details.name. If any part of the path is undefined, the method gracefully returns the specified default value, which is 'Unknown' in this case.
🏆 Best Practices
When working with the _.get()
method, consider the following best practices:
Safely Accessing Nested Properties:
Use
_.get()
to safely access nested properties within objects, especially when dealing with user inputs or dynamic data where properties may be missing.example.jsCopiedconst userProfile = { // Some dynamically generated data }; const userEmail = _.get(userProfile, 'contact.email', 'Not Available'); console.log(userEmail); // Output: Not Available
Provide Sensible Default Values:
When using
_.get()
, consider providing sensible default values. This ensures that your application gracefully handles undefined or missing properties.example.jsCopiedconst settings = { // Some user settings }; const themeColor = _.get(settings, 'appearance.themeColor', 'Default'); console.log(themeColor); // Output: Default
Dynamic Property Access:
Leverage the flexibility of
_.get()
for dynamic property access. This is particularly useful when property names are determined at runtime.example.jsCopiedconst propertyName = 'status'; const taskDetails = { status: 'In Progress', // ... other properties }; const dynamicPropertyValue = _.get(taskDetails, propertyName, 'Not Specified'); console.log(dynamicPropertyValue); // Output: In Progress
📚 Use Cases
Configurations and Settings:
Use
_.get()
to retrieve configuration values and settings from nested objects. This ensures a reliable way to access specific properties without encountering errors due to missing or undefined values.example.jsCopiedconst appConfig = { // ... various configuration settings }; const apiUrl = _.get(appConfig, 'api.url', 'https://default-api.com'); console.log(apiUrl); // Output: https://default-api.com
JSON Data Parsing:
When working with JSON data,
_.get()
can simplify the extraction of specific information, especially in scenarios where the structure may vary.example.jsCopiedconst jsonData = { // ... JSON data from an API response }; const userId = _.get(jsonData, 'user.id', 'Unknown'); console.log(userId); // Output: Unknown
Handling User Input:
In scenarios where user input or external data is involved,
_.get()
helps in safely extracting the required information without risking errors due to missing properties.example.jsCopiedconst userInput = { // ... user-provided data }; const userCity = _.get(userInput, 'address.city', 'City Not Provided'); console.log(userCity); // Output: City Not Provided
🎉 Conclusion
The _.get()
method in Lodash provides a reliable and efficient way to access nested properties within objects. Whether you're navigating through complex data structures or handling user input, this method simplifies the process and ensures your code remains robust and error-resistant.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.get()
method in your Lodash projects.
👨💻 Join our Community:
Author
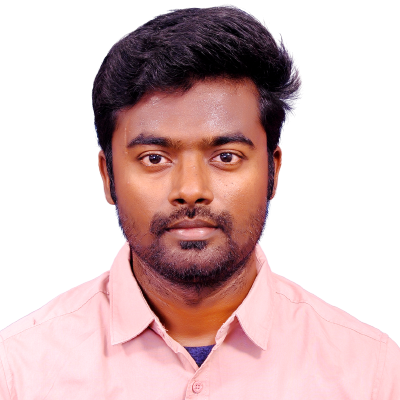
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
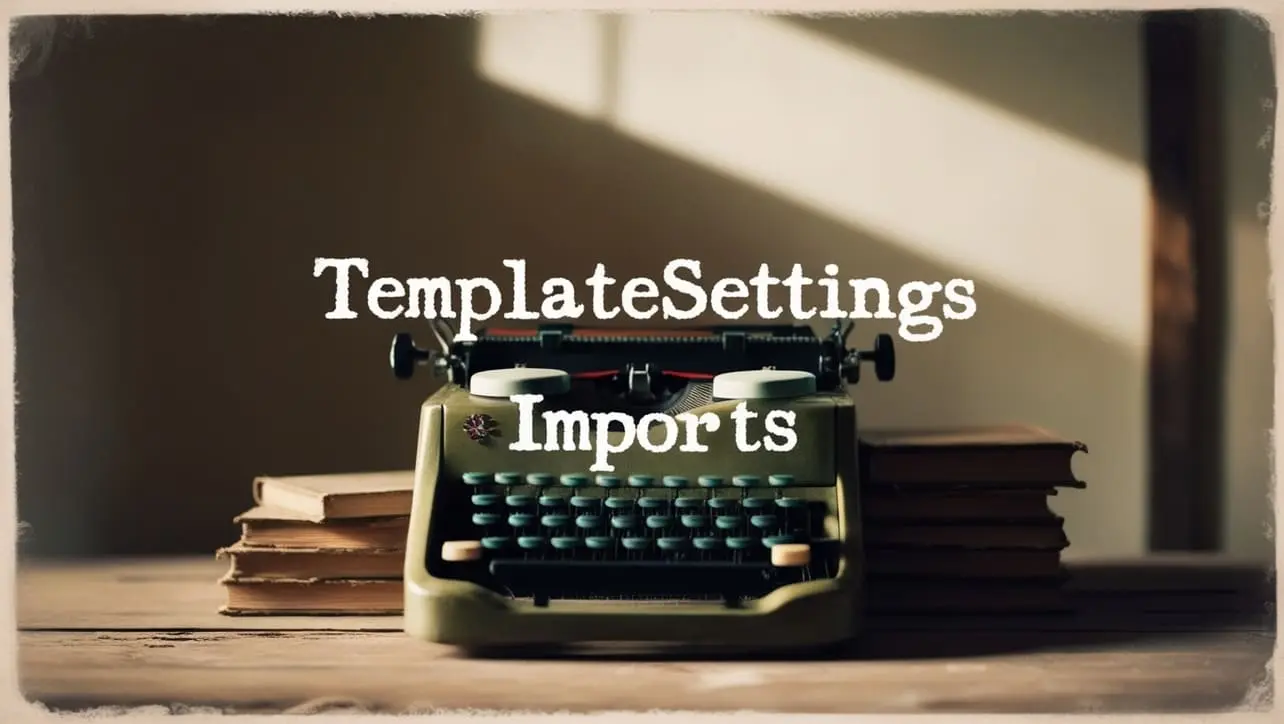
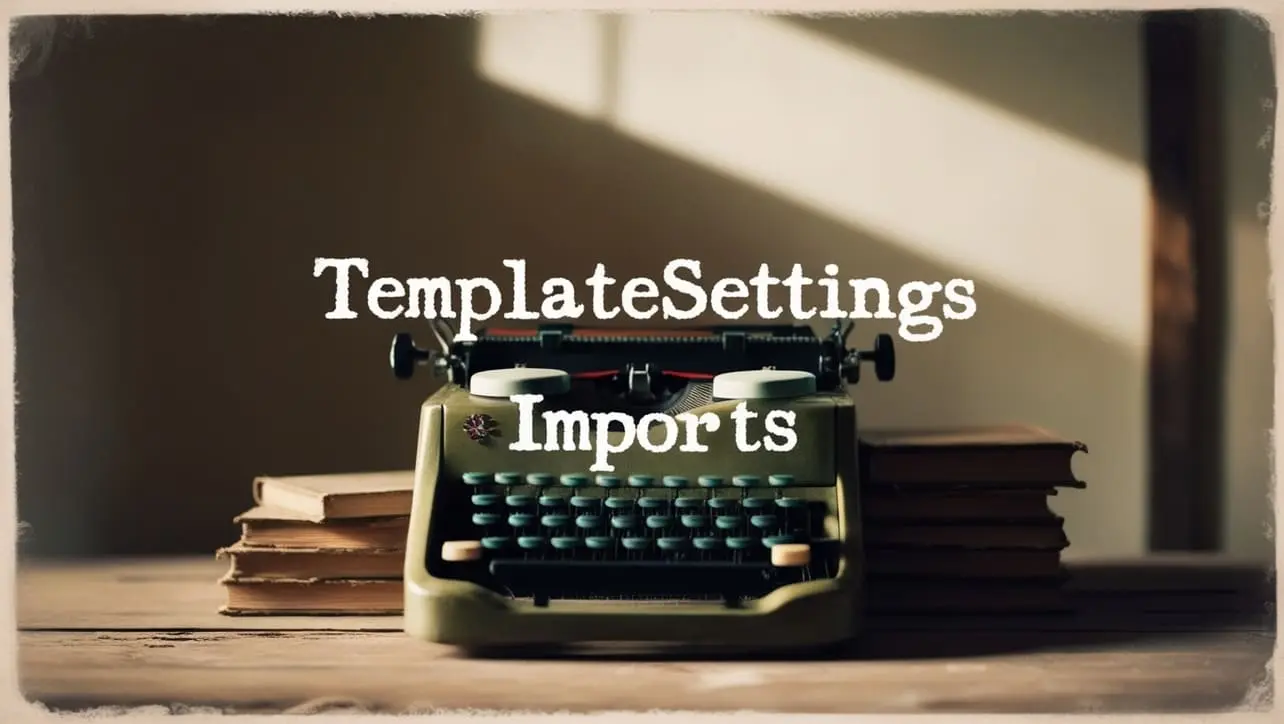
Lodash _.templateSettings.imports Property
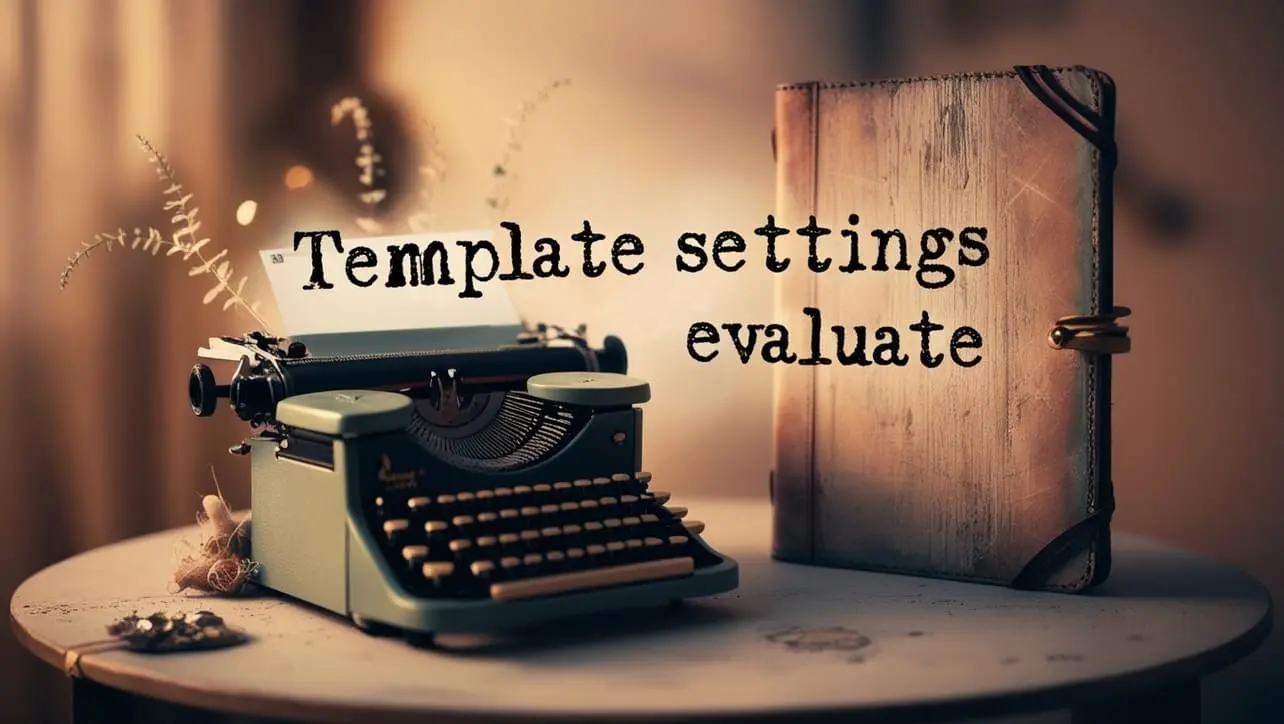
Lodash _.templateSettings.evaluate Property
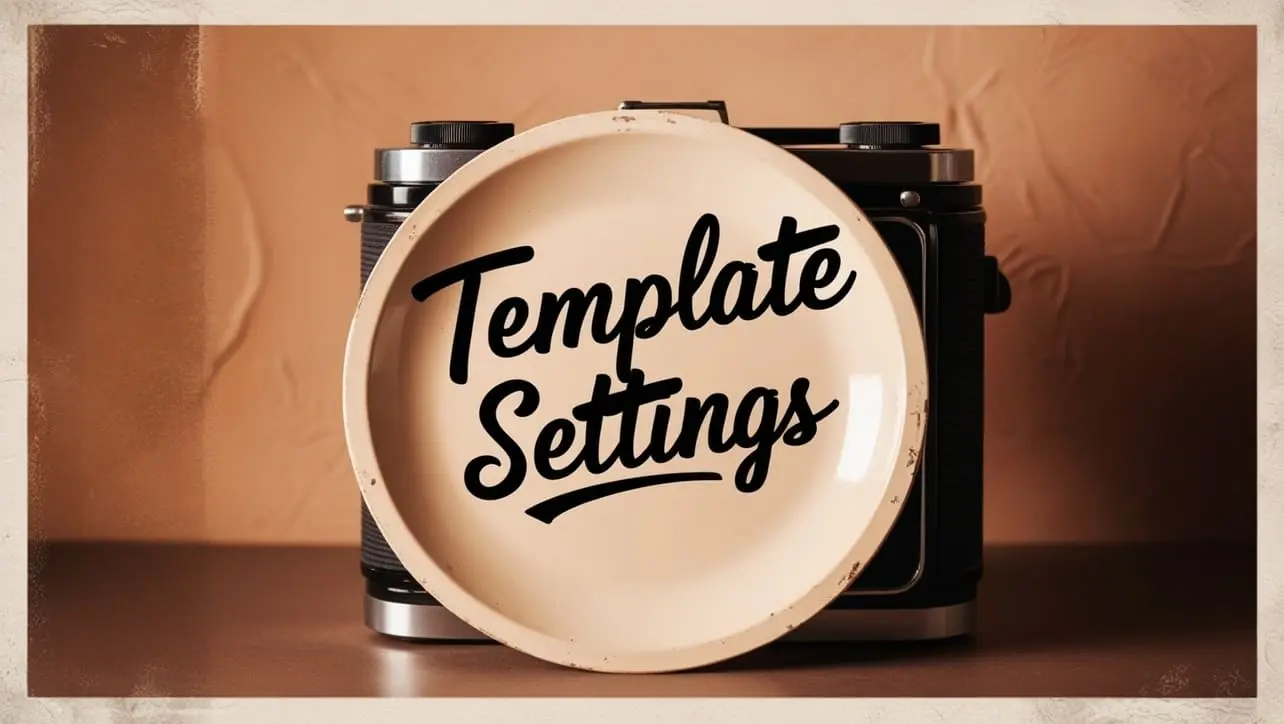
Lodash _.templateSettings Property
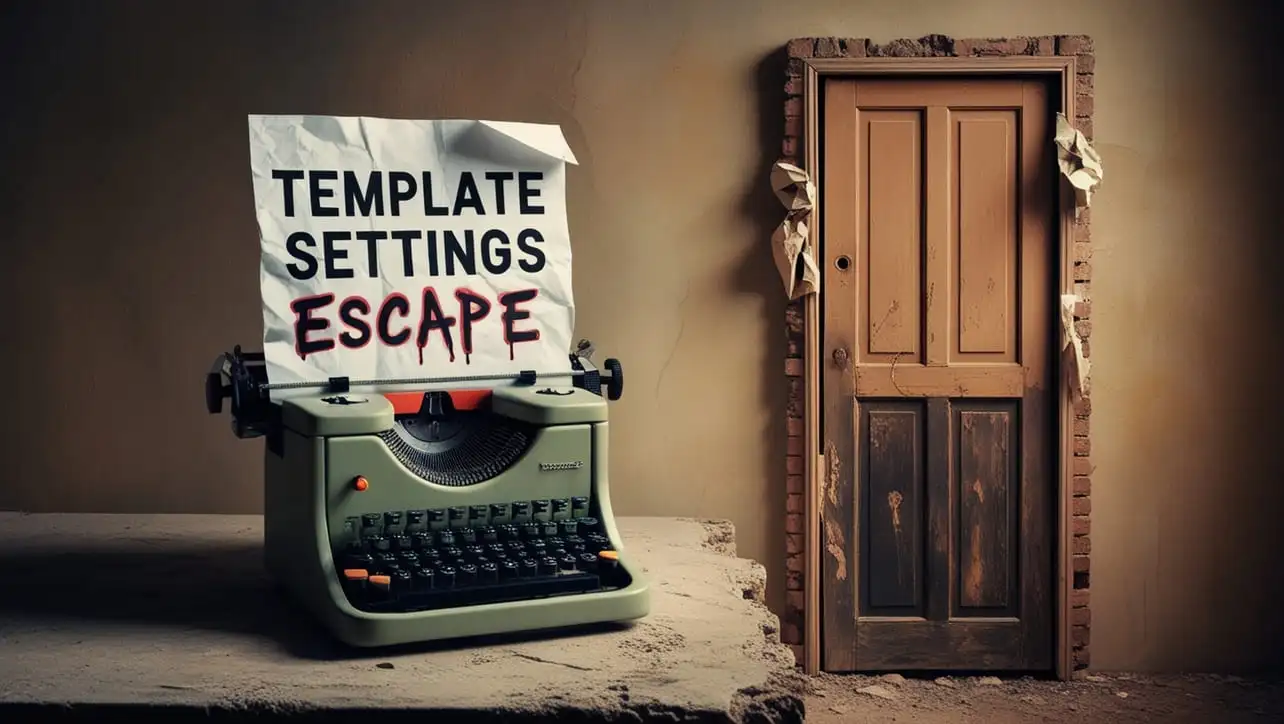
Lodash _.templateSettings.escape Property
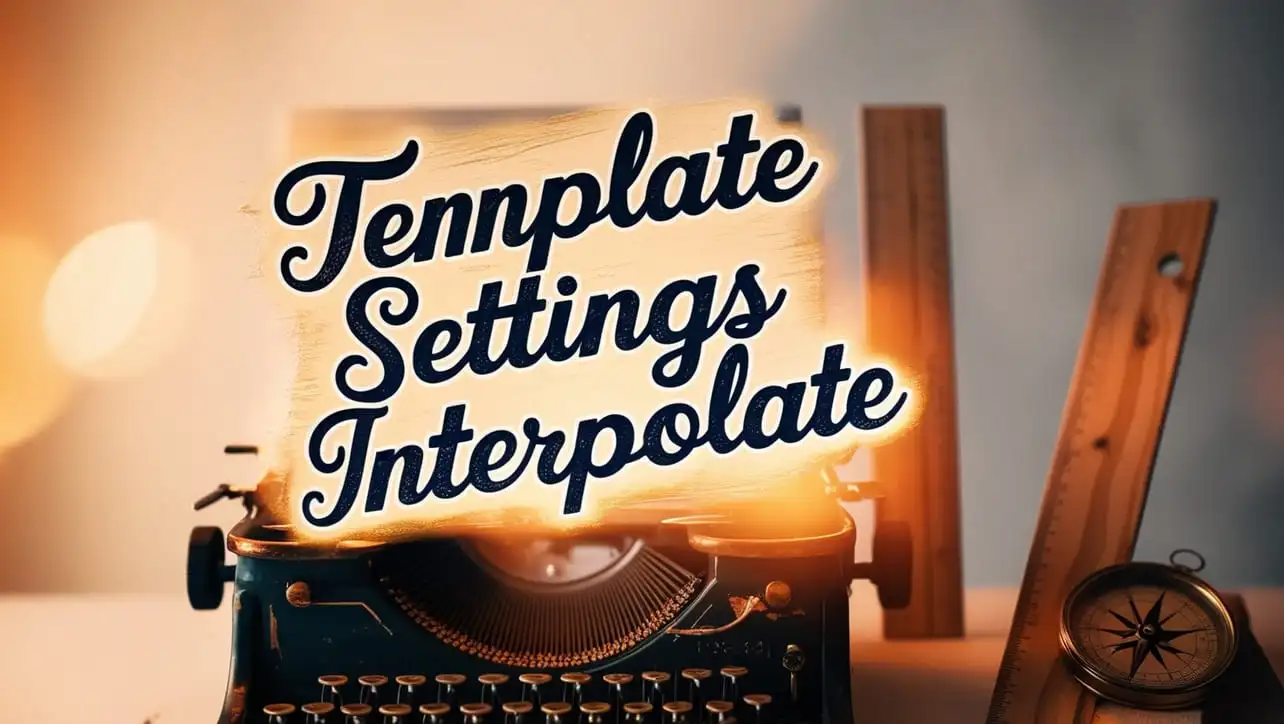
Lodash _.templateSettings.interpolate Property
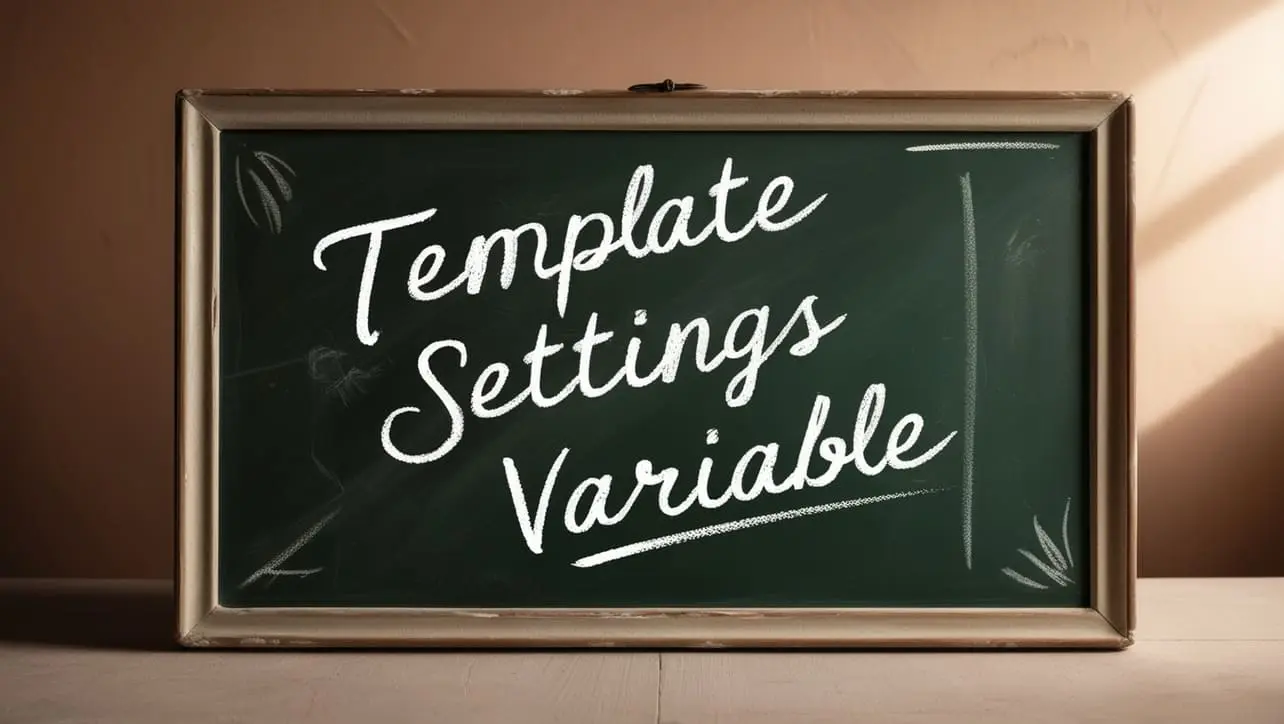
If you have any doubts regarding this article (Lodash _.get() Object Method), please comment here. I will help you immediately.