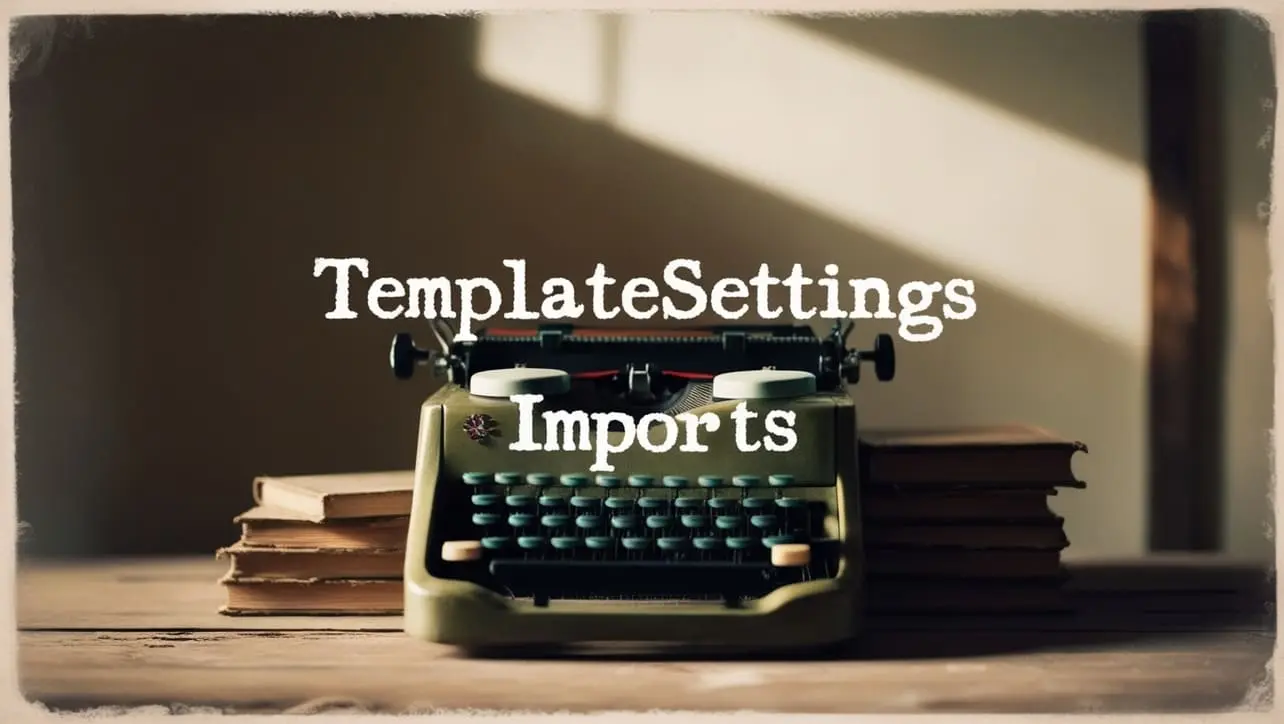
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.forOwn() Object Method
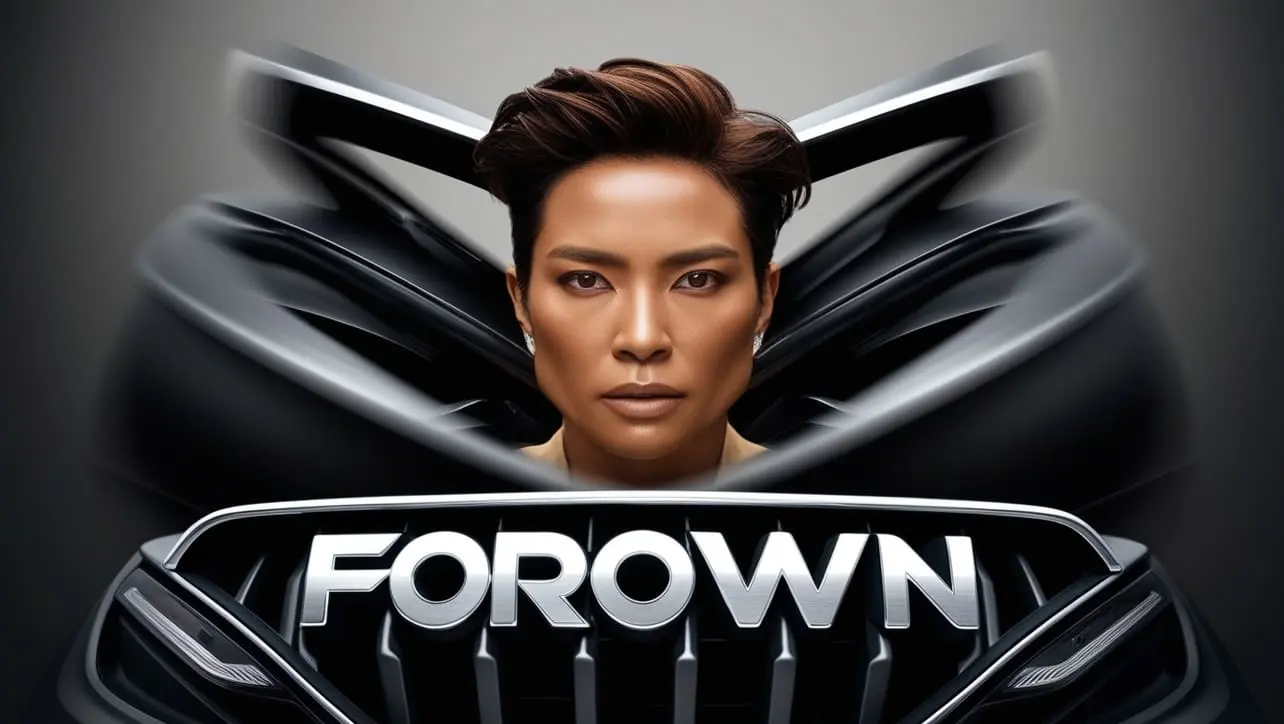
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, efficiently working with objects is fundamental. Lodash, a powerful utility library, offers a multitude of functions to simplify object manipulation, and one such gem is the _.forOwn()
method.
This method provides a convenient way to iterate over an object's own enumerable properties, making it an invaluable tool for developers dealing with complex data structures.
🧠 Understanding _.forOwn() Method
The _.forOwn()
method in Lodash allows you to iterate over an object's own properties, executing a callback function for each key-value pair. This method is particularly useful when you need to perform operations on the specific properties of an object, excluding inherited properties from the prototype chain.
💡 Syntax
The syntax for the _.forOwn()
method is straightforward:
_.forOwn(object, [iteratee])
- object: The object to iterate over.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.forOwn()
method:
const _ = require('lodash');
const sampleObject = {
name: 'John',
age: 30,
city: 'New York',
};
_.forOwn(sampleObject, (value, key) => {
console.log(`${key}: ${value}`);
});
In this example, _.forOwn()
iterates over the properties of sampleObject, logging each key-value pair to the console.
🏆 Best Practices
When working with the _.forOwn()
method, consider the following best practices:
Object Property Check:
Before using
_.forOwn()
, ensure that the target object is valid and not null or undefined. This helps prevent errors when iterating over properties.example.jsCopiedconst user = { name: 'Alice', age: 25, }; if (user) { _.forOwn(user, (value, key) => { console.log(`${key}: ${value}`); }); }
Handling Callback Logic:
Within the callback function, handle the logic for each property appropriately. This could involve data manipulation, validation, or any other specific operations.
example.jsCopiedconst person = { firstName: 'Bob', lastName: 'Johnson', age: 40, }; _.forOwn(person, (value, key) => { if(key === 'age' && value < 18) { console.log(`${person.firstName} is a minor.`); } else { console.log(`${key}: ${value}`); } });
Object Property Order:
Keep in mind that the order of properties is not guaranteed. If the order is crucial, consider using an array of keys for explicit ordering.
example.jsCopiedconst orderedObject = { name: 'Eva', age: 28, city: 'Paris', }; const orderedKeys = ['name', 'age', 'city']; orderedKeys.forEach(key => { console.log(`${key}: ${orderedObject[key]}`); });
📚 Use Cases
Object Transformation:
_.forOwn()
is handy when you need to transform the properties of an object based on specific criteria, providing a flexible approach to modify the object.example.jsCopiedconst studentGrades = { math: 90, science: 85, history: 95, }; _.forOwn(studentGrades, (score, subject) => { // Add bonus points to each subject studentGrades[subject] = score + 5; }); console.log(studentGrades);
Property Validation:
When you need to validate or filter object properties based on certain conditions,
_.forOwn()
facilitates the process.example.jsCopiedconst personInfo = { name: 'Sam', age: 22, email: 'sam@example.com', address: '', }; // Remove properties with empty values _.forOwn(personInfo, (value, key, obj) => { if(value === '') { delete obj[key]; } }); console.log(personInfo);
Dynamic Object Operations:
For scenarios where object properties or their values are determined dynamically,
_.forOwn()
provides a dynamic and adaptable solution.example.jsCopiedconst dynamicObject = /* ... fetch or generate object dynamically ... */; _.forOwn(dynamicObject, (value, key) => { // Perform dynamic operations based on key-value pairs console.log(`${key}: ${value}`); });
🎉 Conclusion
The _.forOwn()
method in Lodash empowers JavaScript developers by providing a clean and efficient way to iterate over an object's own enumerable properties. Whether you're transforming object properties, validating data, or performing dynamic operations, _.forOwn()
proves to be a versatile tool in your toolkit.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.forOwn()
method in your Lodash projects.
👨💻 Join our Community:
Author
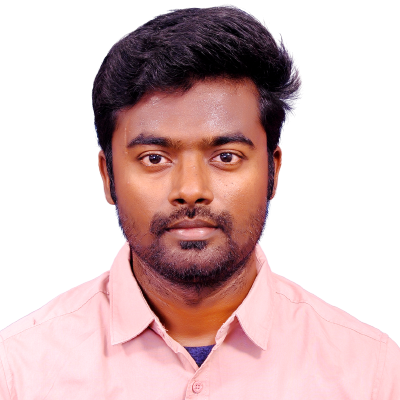
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
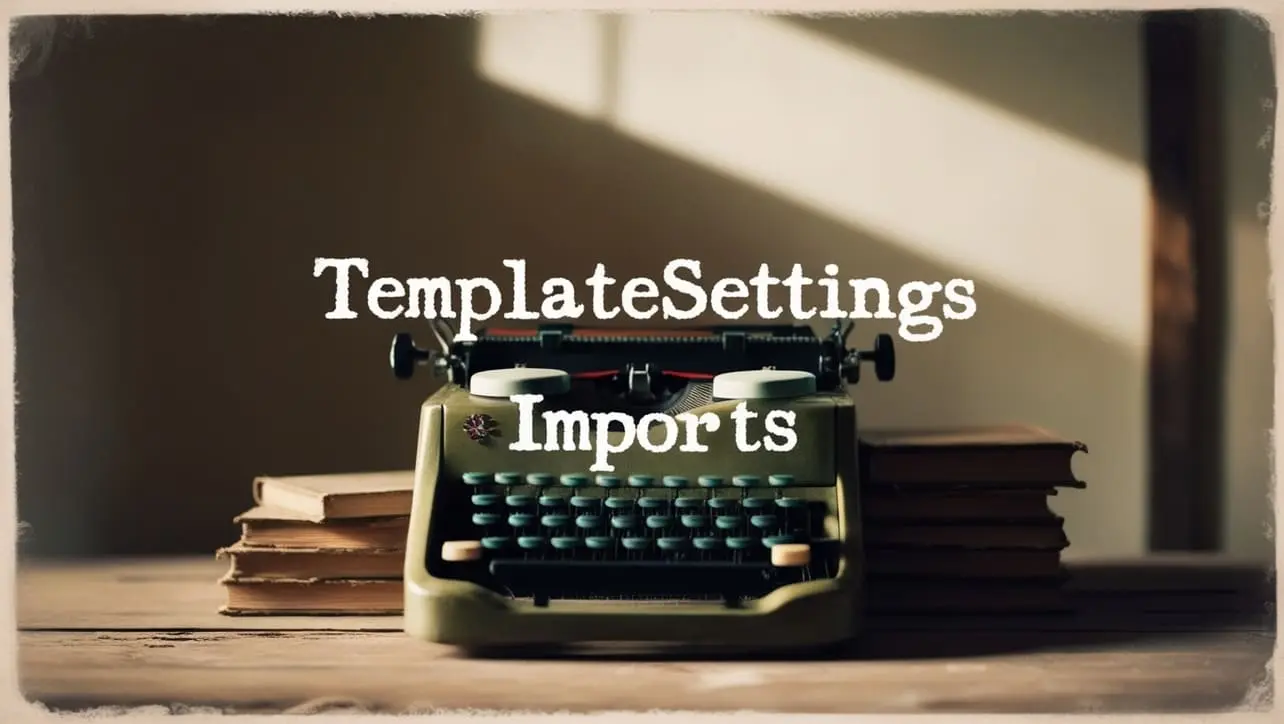
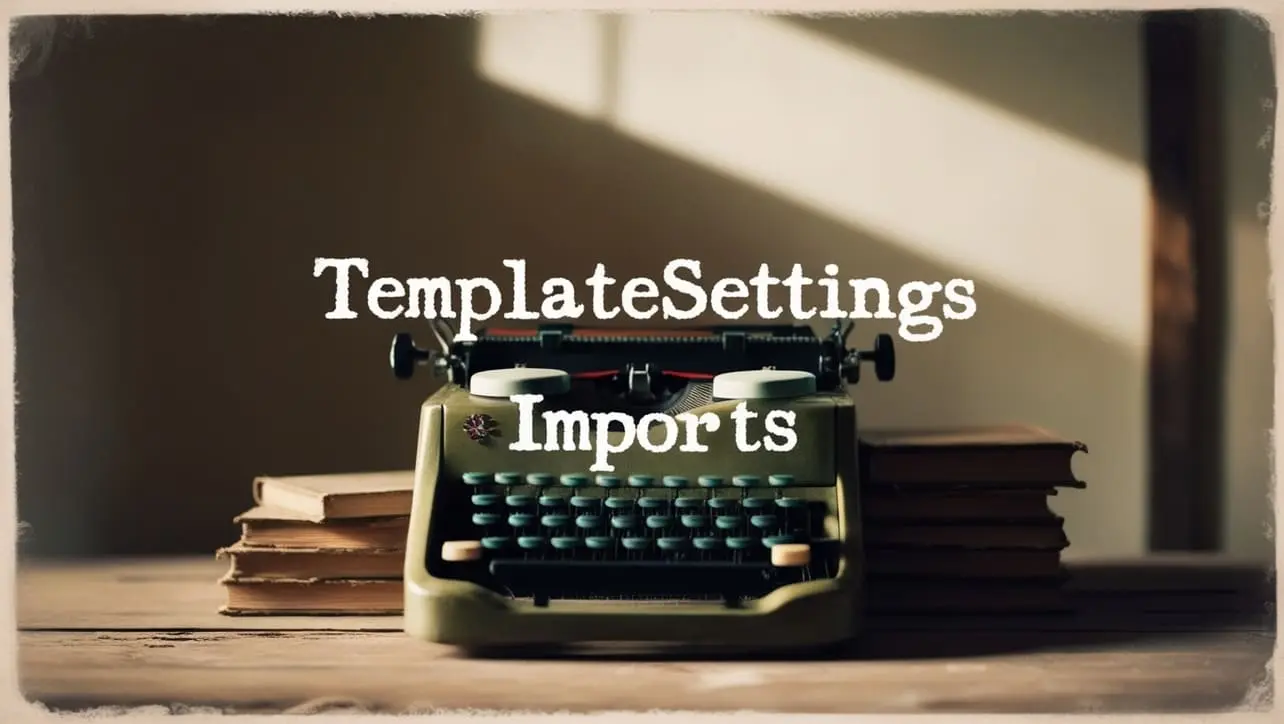
Lodash _.templateSettings.imports Property
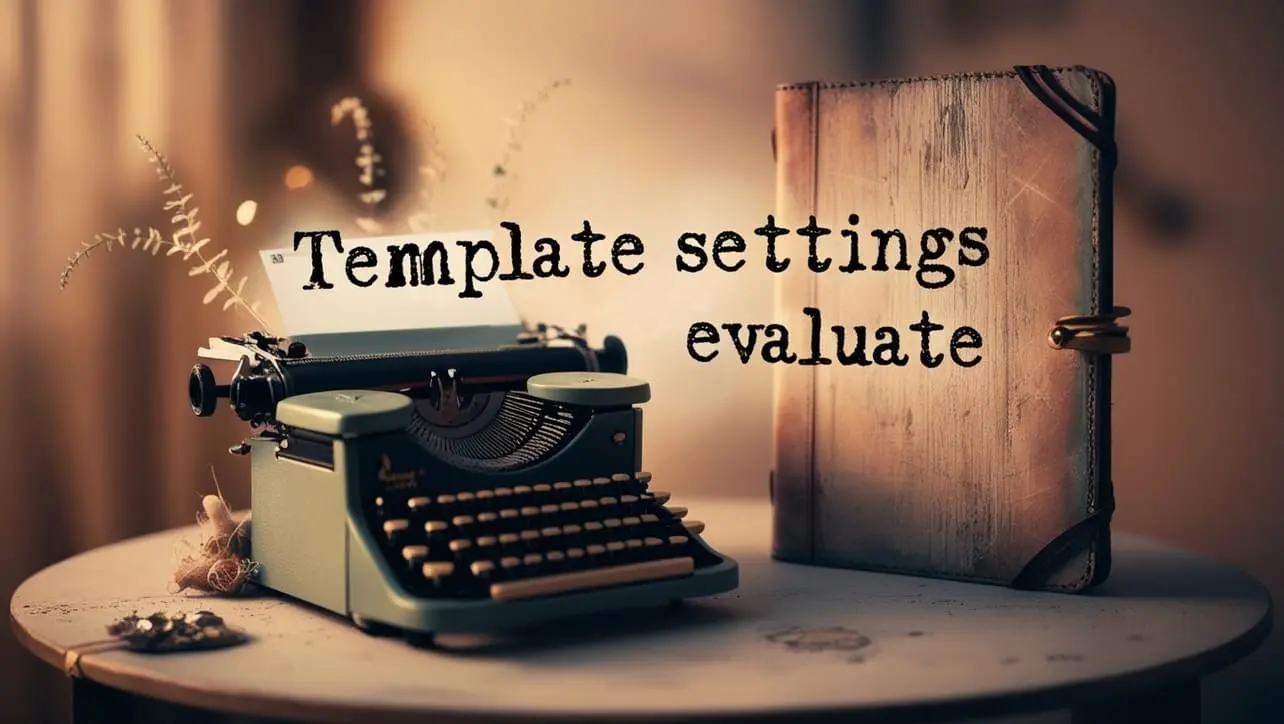
Lodash _.templateSettings.evaluate Property
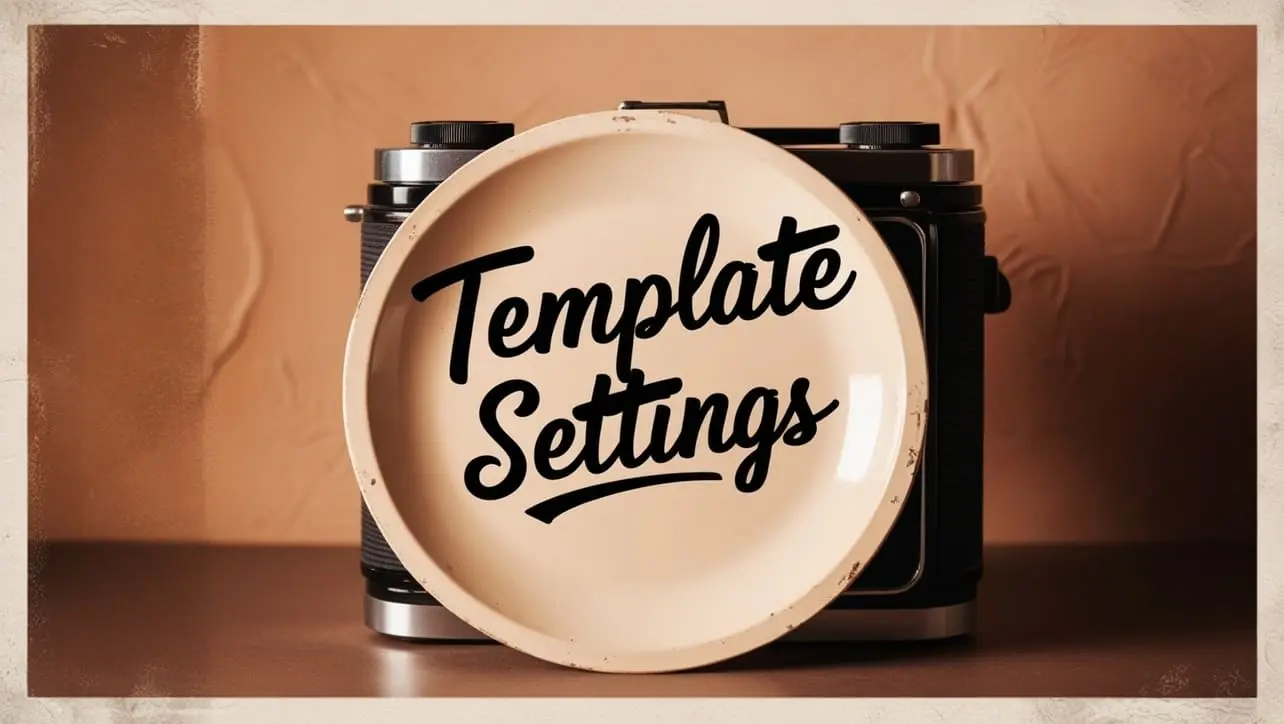
Lodash _.templateSettings Property
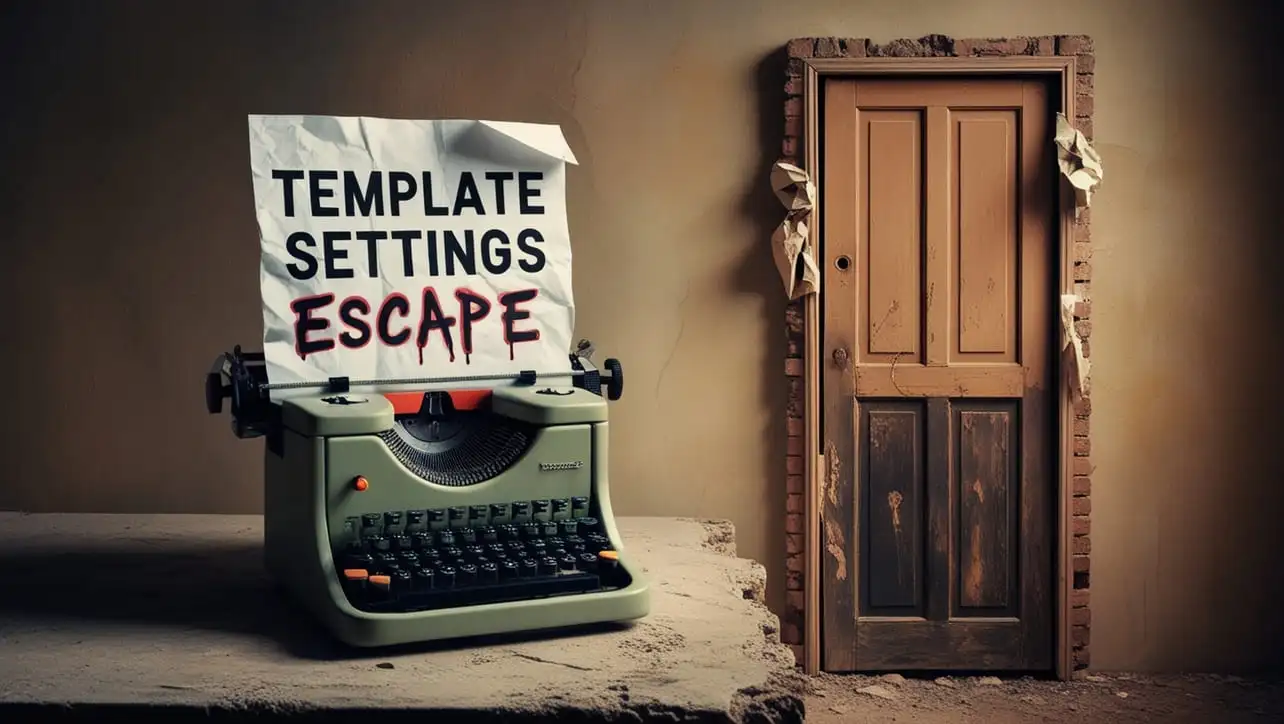
Lodash _.templateSettings.escape Property
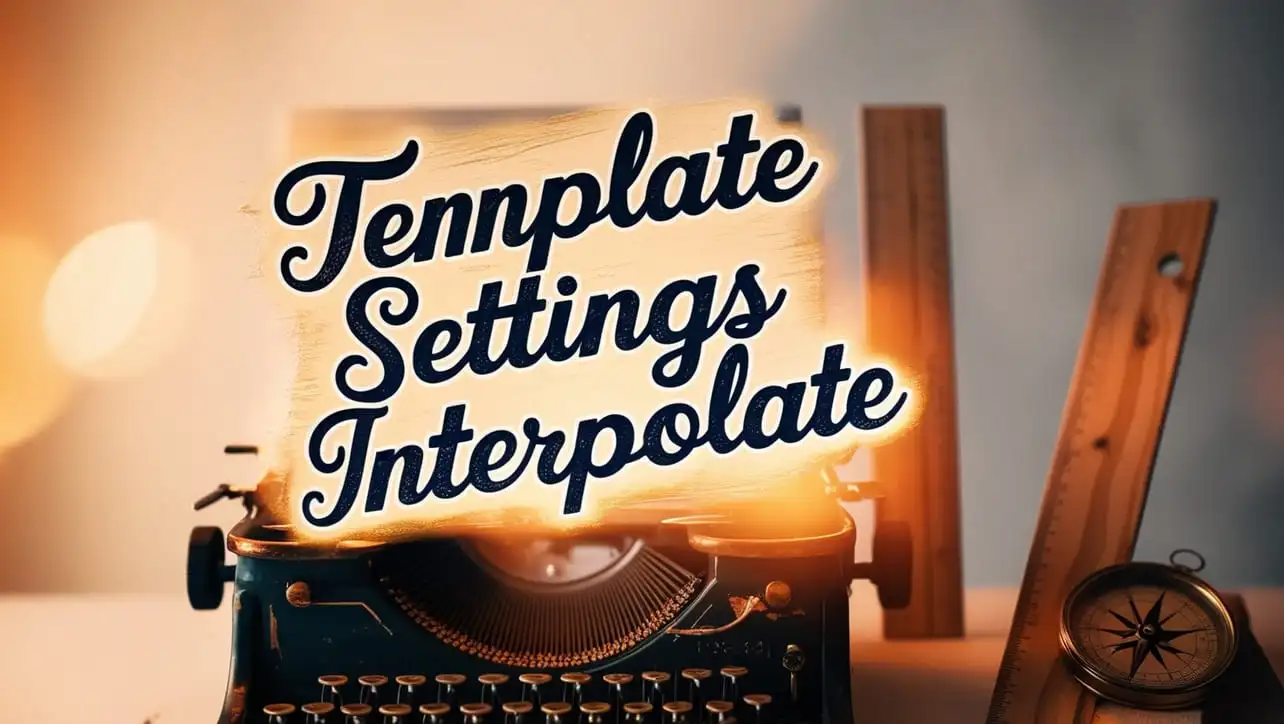
Lodash _.templateSettings.interpolate Property
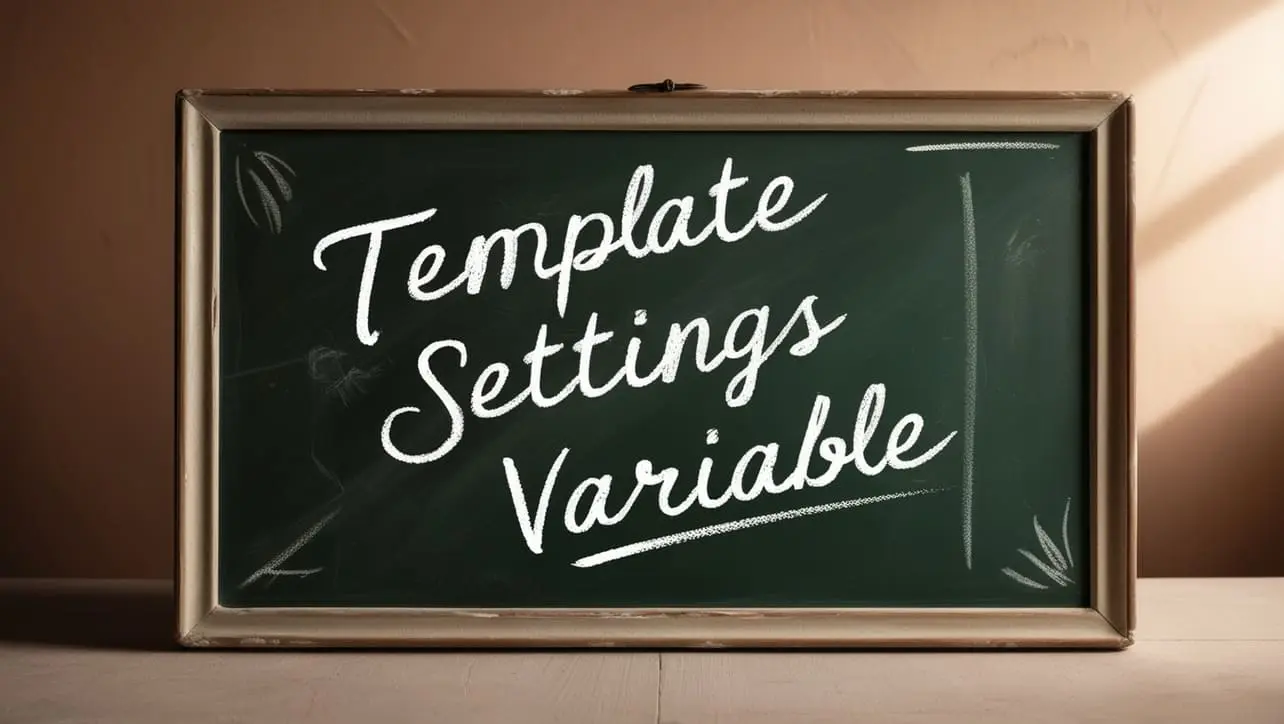
If you have any doubts regarding this article (Lodash _.forOwn() Object Method), please comment here. I will help you immediately.