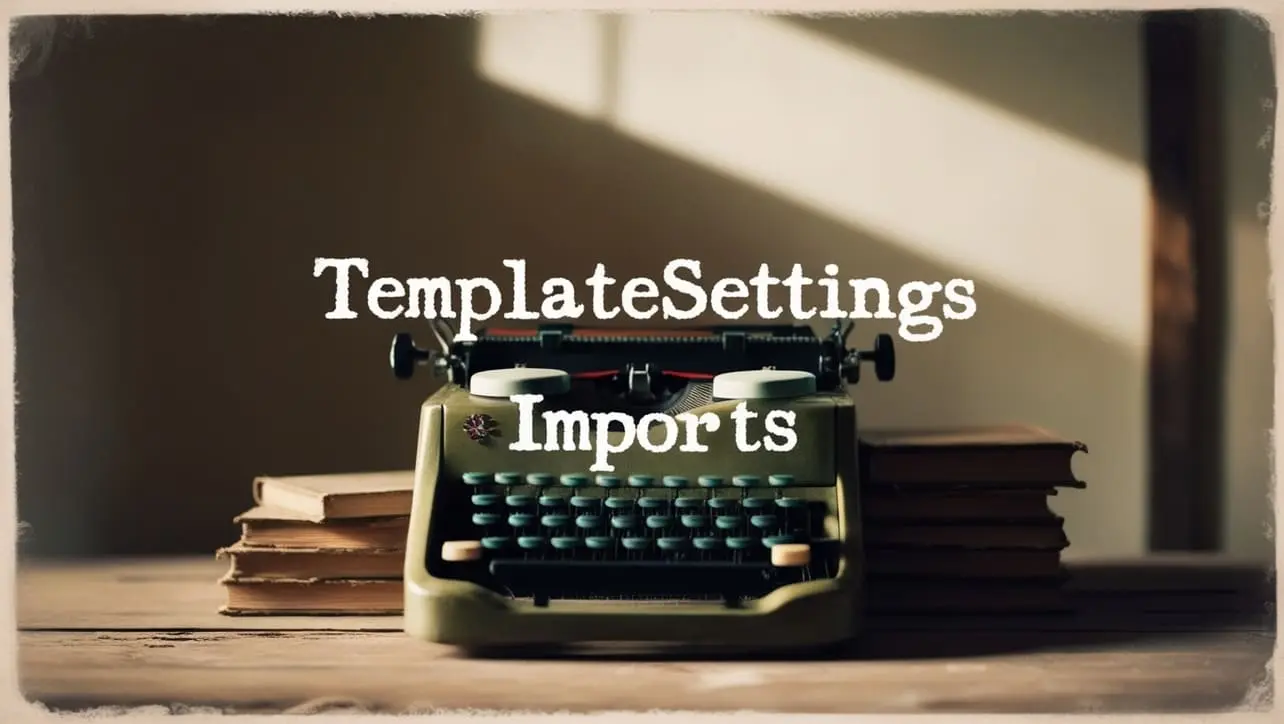
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.forIn() Object Method
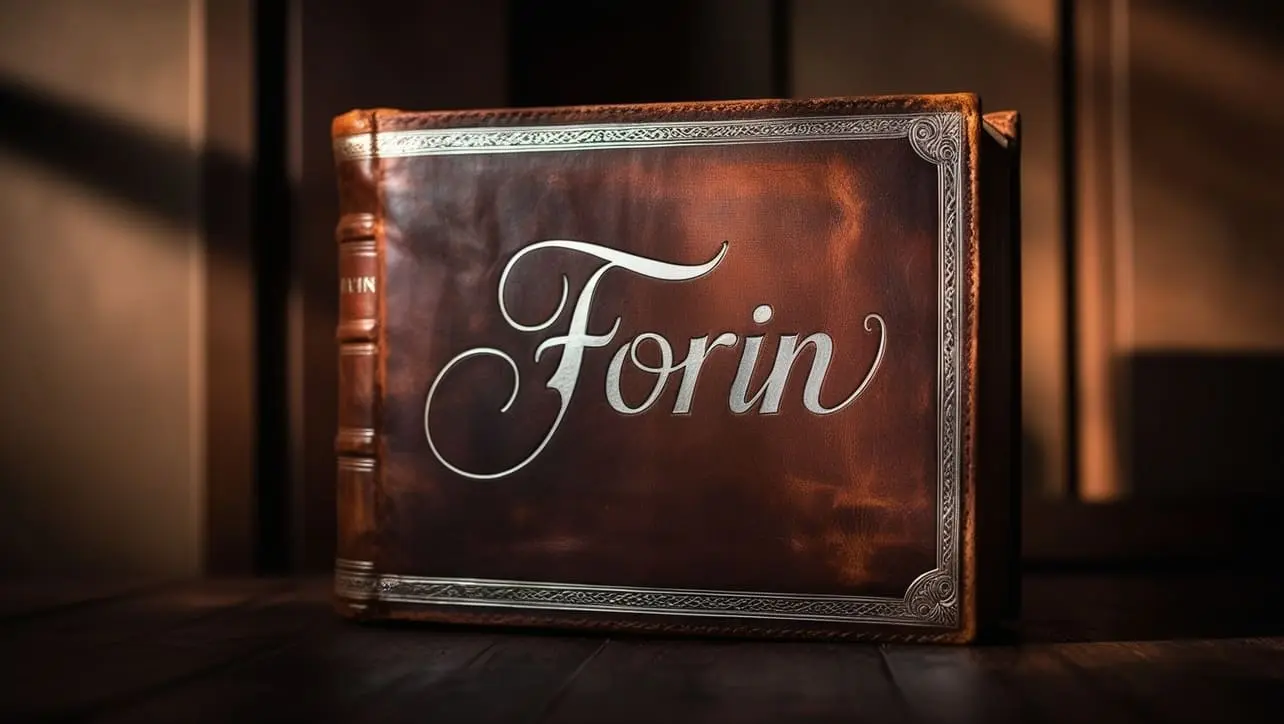
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, effective iteration through object properties is a common requirement. Lodash, a versatile utility library, offers a multitude of functions to simplify JavaScript coding. Among these functions is the _.forIn()
method, a powerful tool for iterating over the enumerable properties of an object.
This method enhances the developer's ability to navigate and manipulate object properties with ease.
🧠 Understanding _.forIn() Method
The _.forIn()
method in Lodash is designed for iterating over an object's own and inherited enumerable properties. It provides a straightforward way to access and perform operations on each key-value pair within an object.
💡 Syntax
The syntax for the _.forIn()
method is straightforward:
_.forIn(object, iteratee)
- object: The object to iterate over.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.forIn()
method:
const _ = require('lodash');
const sampleObject = {
name: 'John',
age: 30,
city: 'New York',
};
_.forIn(sampleObject, (value, key) => {
console.log(`${key}: ${value}`);
});
In this example, _.forIn()
is used to iterate over the sampleObject, logging each key-value pair to the console.
🏆 Best Practices
When working with the _.forIn()
method, consider the following best practices:
Iterating Over Object Properties:
Use
_.forIn()
when you need to iterate over object properties, including inherited properties. This method ensures a comprehensive exploration of an object's structure.example.jsCopiedfunction CustomObject() { this.propertyA = 'Value A'; } CustomObject.prototype.propertyB = 'Value B'; const customInstance = new CustomObject(); _.forIn(customInstance, (value, key) => { console.log(`${key}: ${value}`); });
Check Object Properties:
Leverage
_.forIn()
to perform checks on object properties dynamically. You can use this method to inspect and validate the properties of an object based on your specific requirements.example.jsCopiedconst user = { username: 'john_doe', email: 'john@example.com', age: 25, }; _.forIn(user, (value, key) => { if (key === 'email' && !value.includes('@')) { console.error('Invalid email format'); } });
Property Modification:
Utilize
_.forIn()
to modify object properties dynamically. This can be valuable when you need to update or transform certain properties of an object.example.jsCopiedconst mutableObject = { count: 5, price: 10, }; _.forIn(mutableObject, (value, key, obj) => { obj[key] = value * 2; // Double the value of each property }); console.log(mutableObject); // Output: { count: 10, price: 20 }
📚 Use Cases
Object Property Validation:
_.forIn()
is particularly useful when you need to validate or perform specific actions based on the properties of an object.example.jsCopiedconst userData = { username: 'john_doe', password: 'securepassword123', isAdmin: true, }; let isValid = true; _.forIn(userData, (value, key) => { if (key === 'password' && value.length < 8) { console.error('Password must be at least 8 characters long'); isValid = false; } }); if (isValid) { console.log('User data is valid.'); }
Dynamic Object Property Generation:
When dealing with dynamic data,
_.forIn()
can be employed to generate object properties dynamically, providing flexibility in your code.example.jsCopiedconst dynamicData = /* ...fetch dynamic data from an external source... */ ; const dynamicObject = {}; _.forIn(dynamicData, (value, key) => { dynamicObject[key] = value; }); console.log(dynamicObject);
Inherited Properties Handling:
If your application involves objects with inherited properties,
_.forIn()
ensures that both the object's own properties and inherited properties are considered during iteration.example.jsCopiedclass ParentClass { constructor() { this.parentProperty = 'Parent Property'; } } class ChildClass extends ParentClass { constructor() { super(); this.childProperty = 'Child Property'; } } const childInstance = new ChildClass(); _.forIn(childInstance, (value, key) => { console.log(`${key}: ${value}`); });
🎉 Conclusion
The _.forIn()
method in Lodash offers a flexible and efficient solution for iterating over object properties, including inherited ones. Whether you're validating object properties, dynamically generating properties, or handling inherited properties, _.forIn()
provides a reliable and versatile tool for traversing JavaScript objects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.forIn()
method in your Lodash projects.
👨💻 Join our Community:
Author
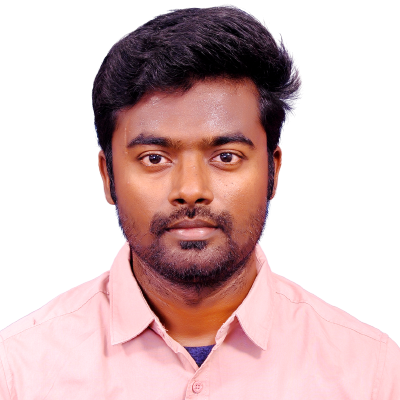
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
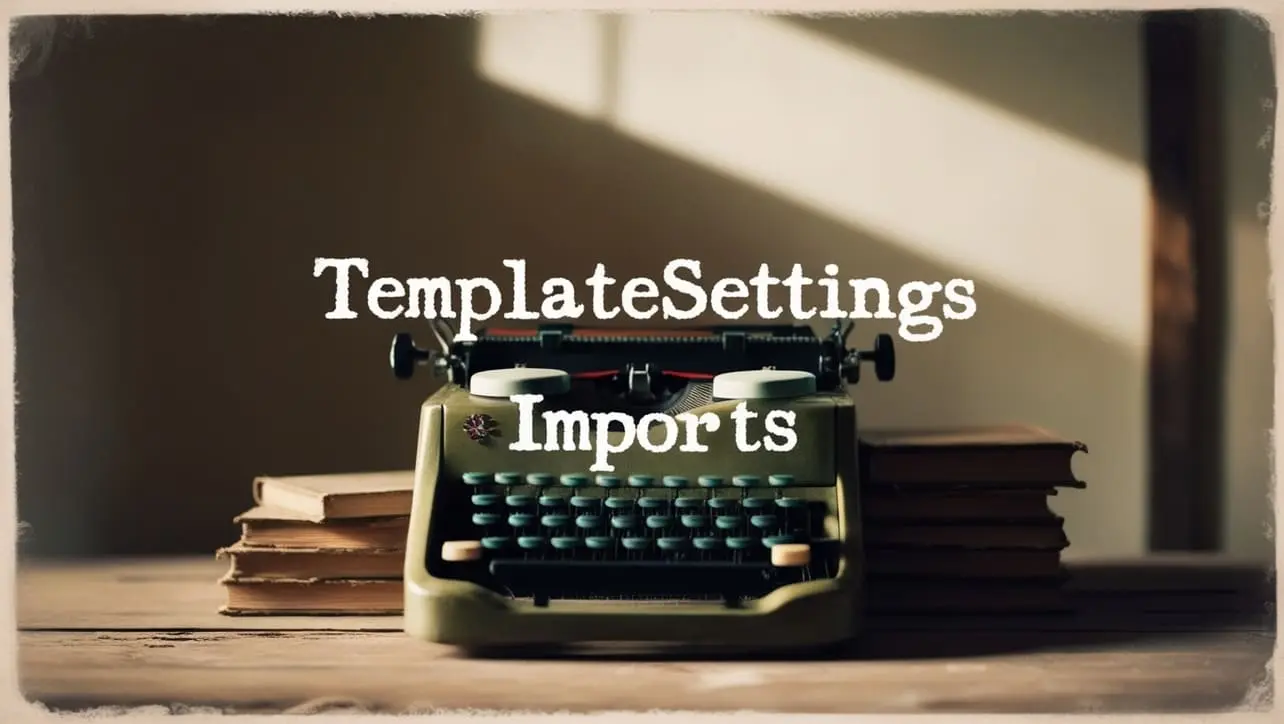
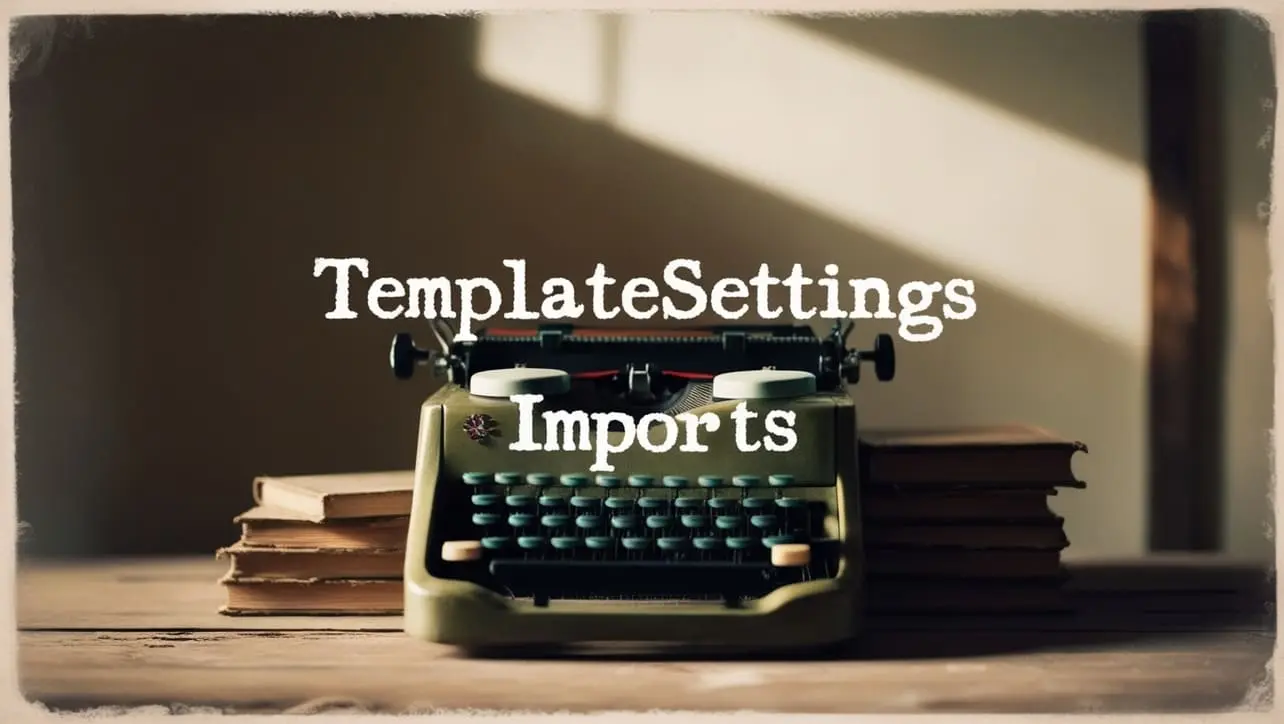
Lodash _.templateSettings.imports Property
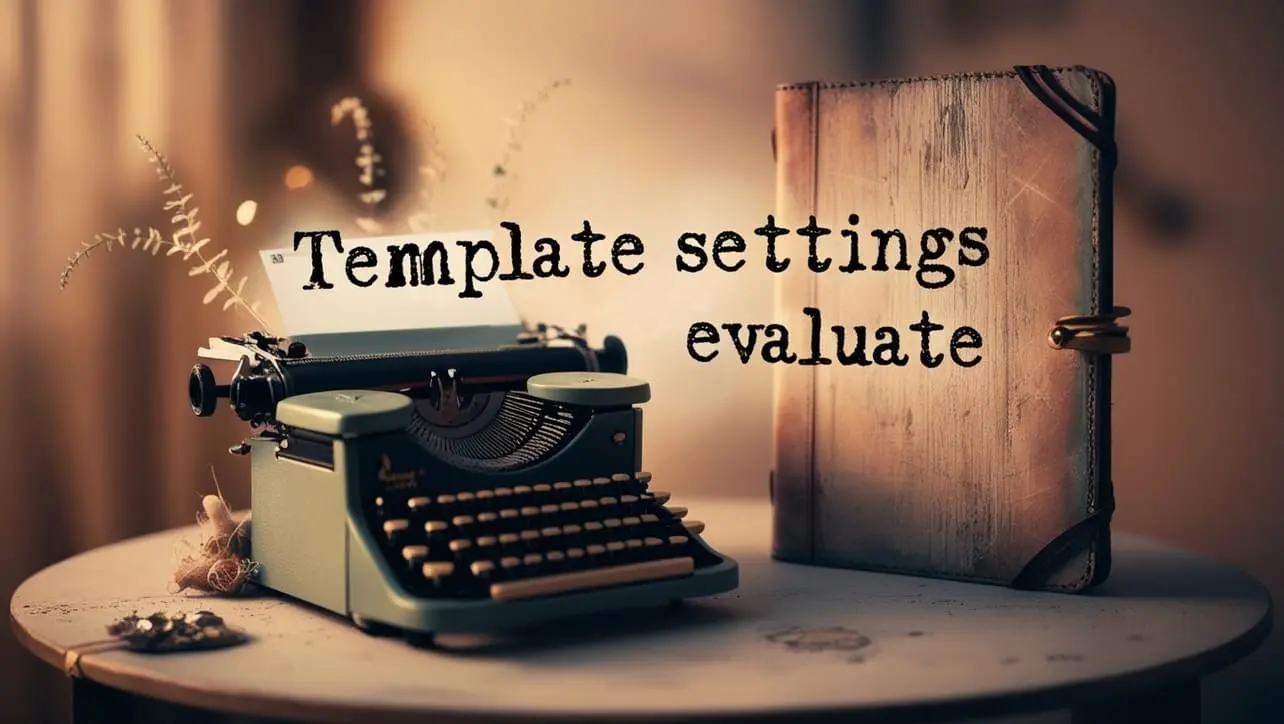
Lodash _.templateSettings.evaluate Property
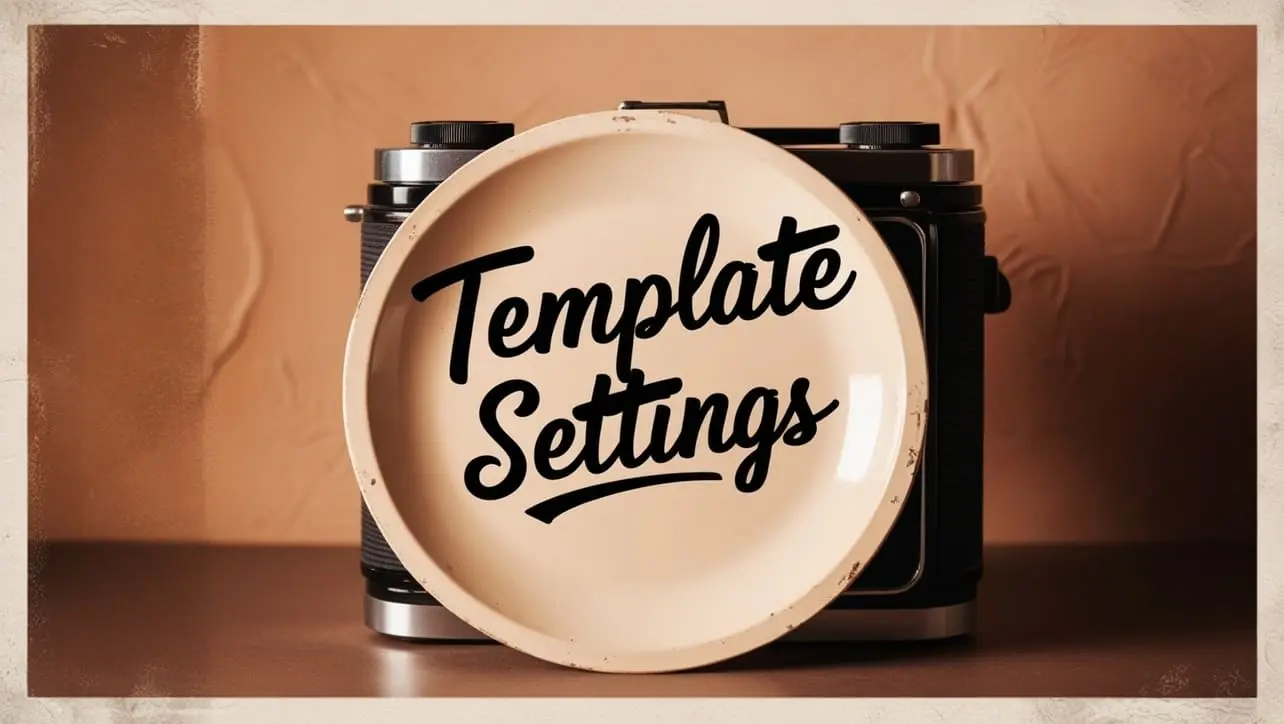
Lodash _.templateSettings Property
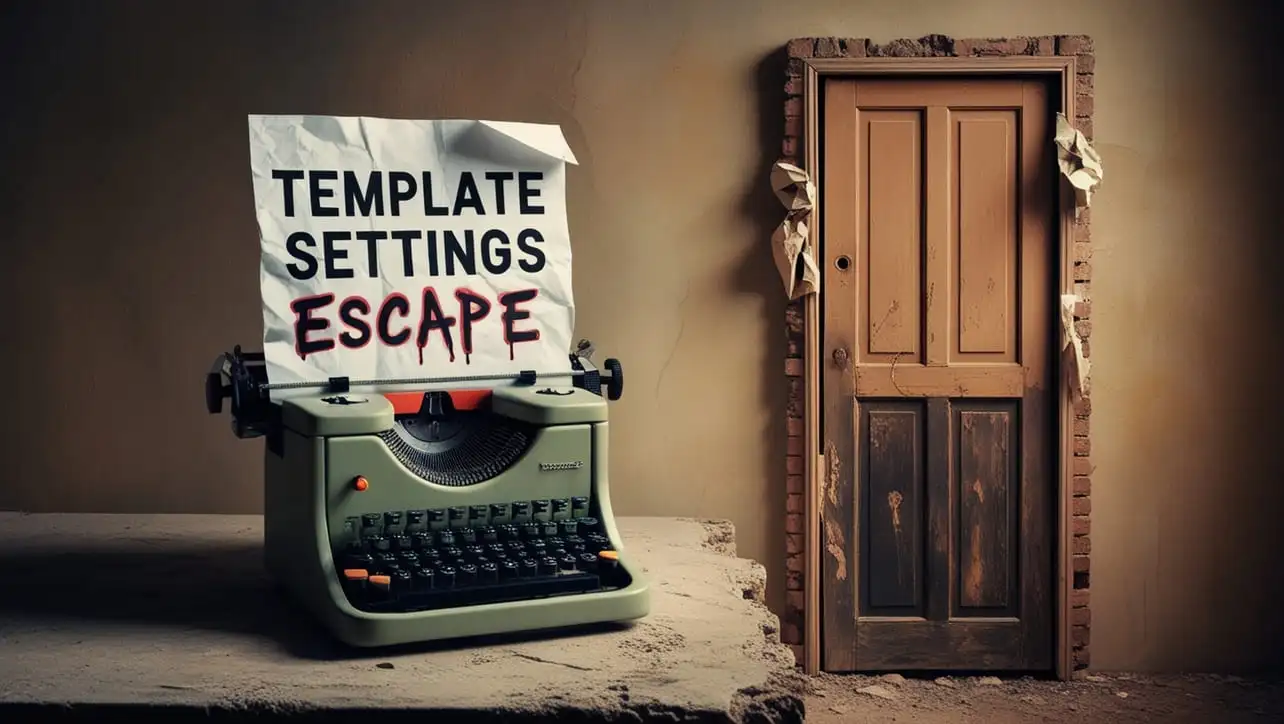
Lodash _.templateSettings.escape Property
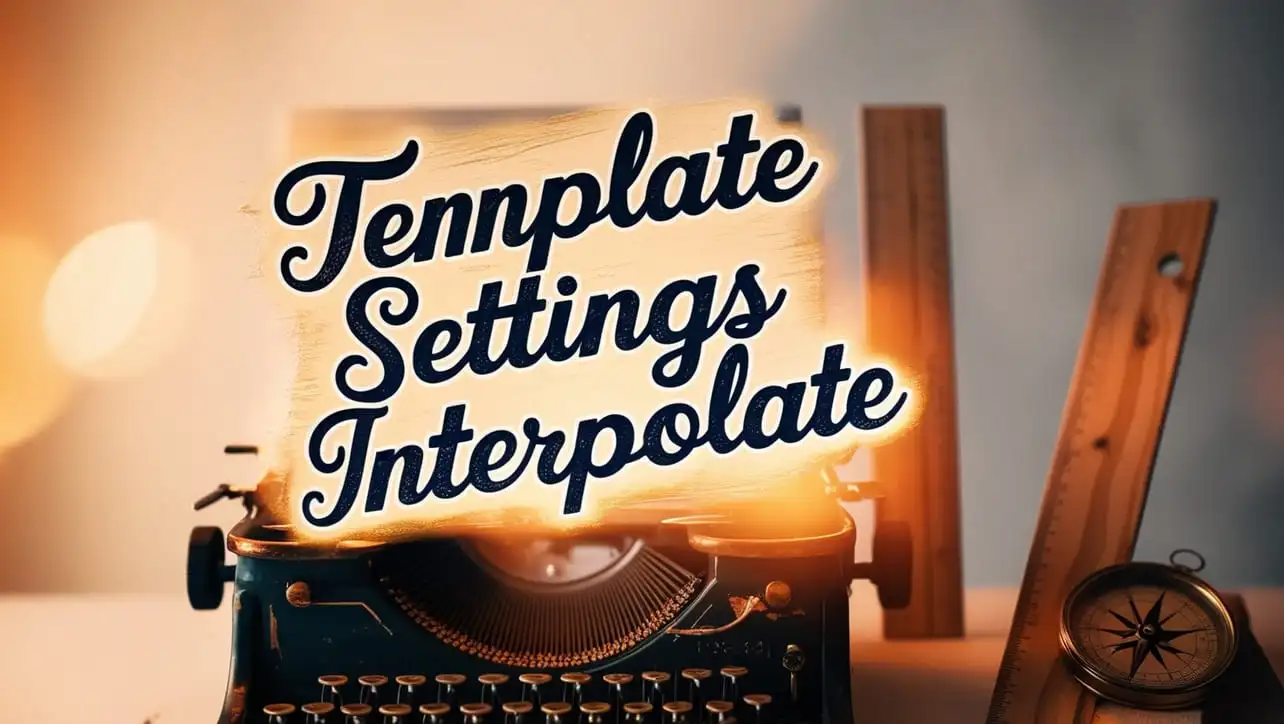
Lodash _.templateSettings.interpolate Property
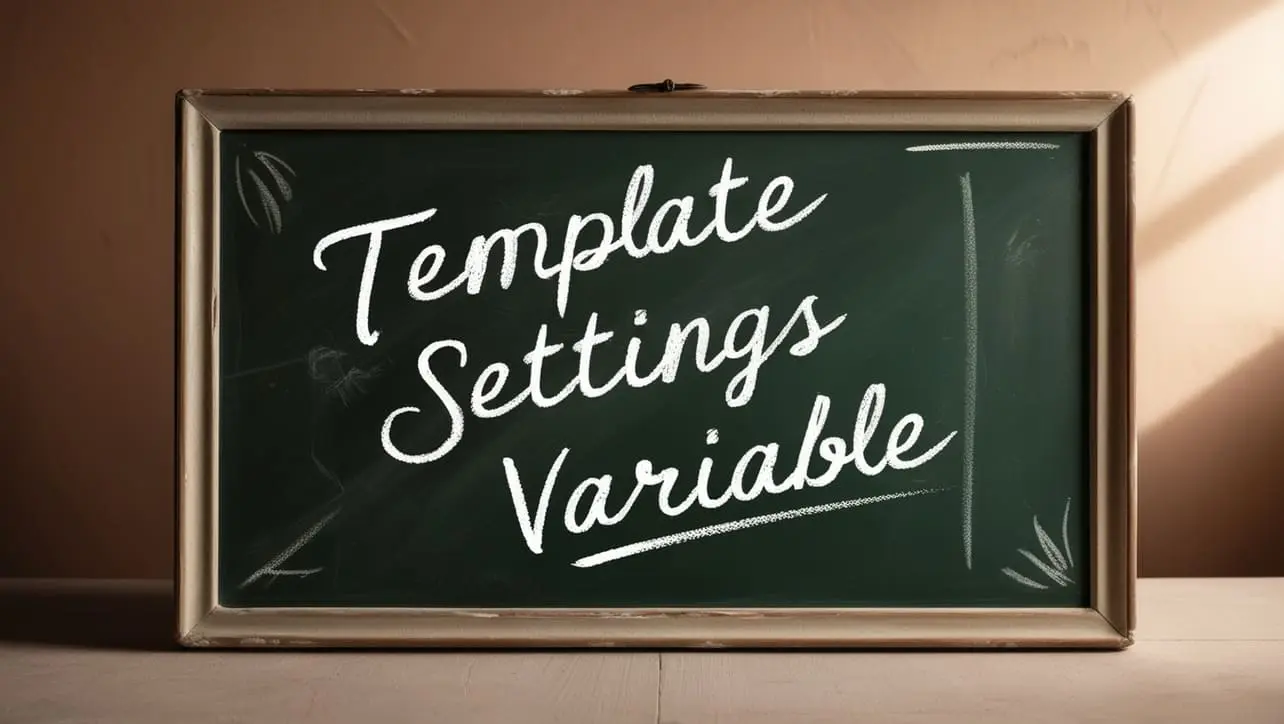
If you have any doubts regarding this article (Lodash _.forIn() Object Method), please comment here. I will help you immediately.