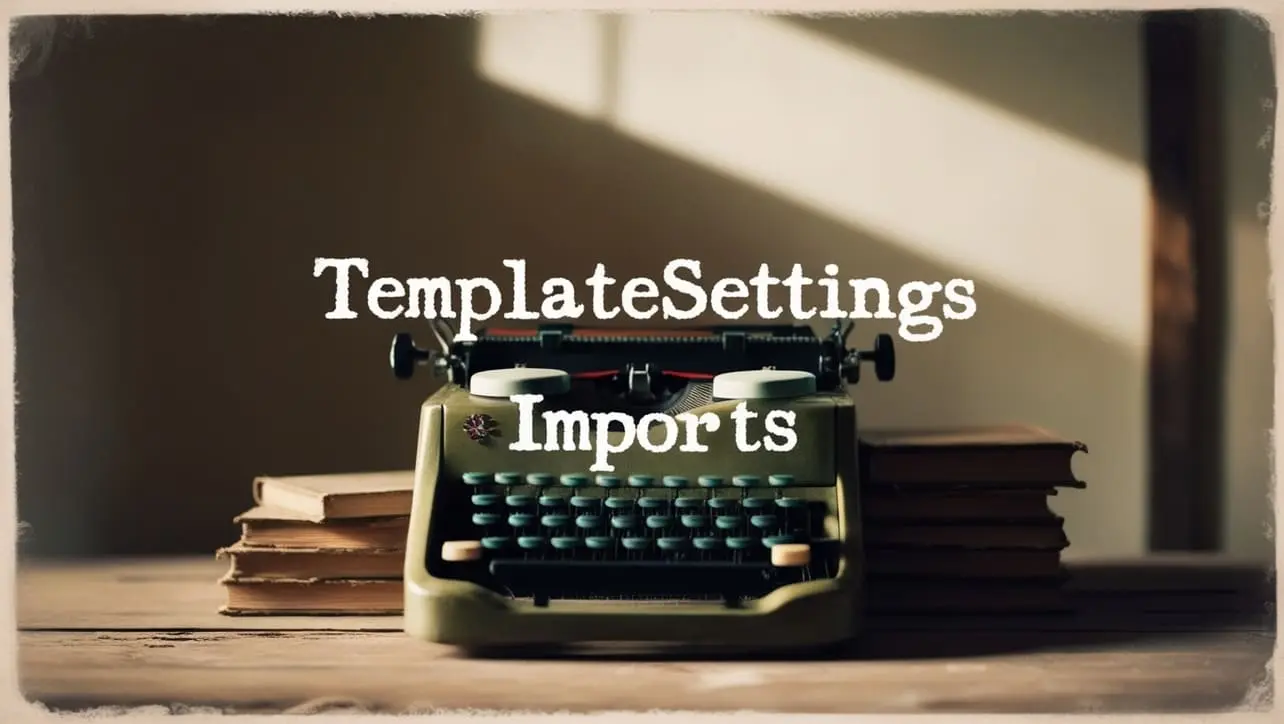
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.findLastKey() Object Method
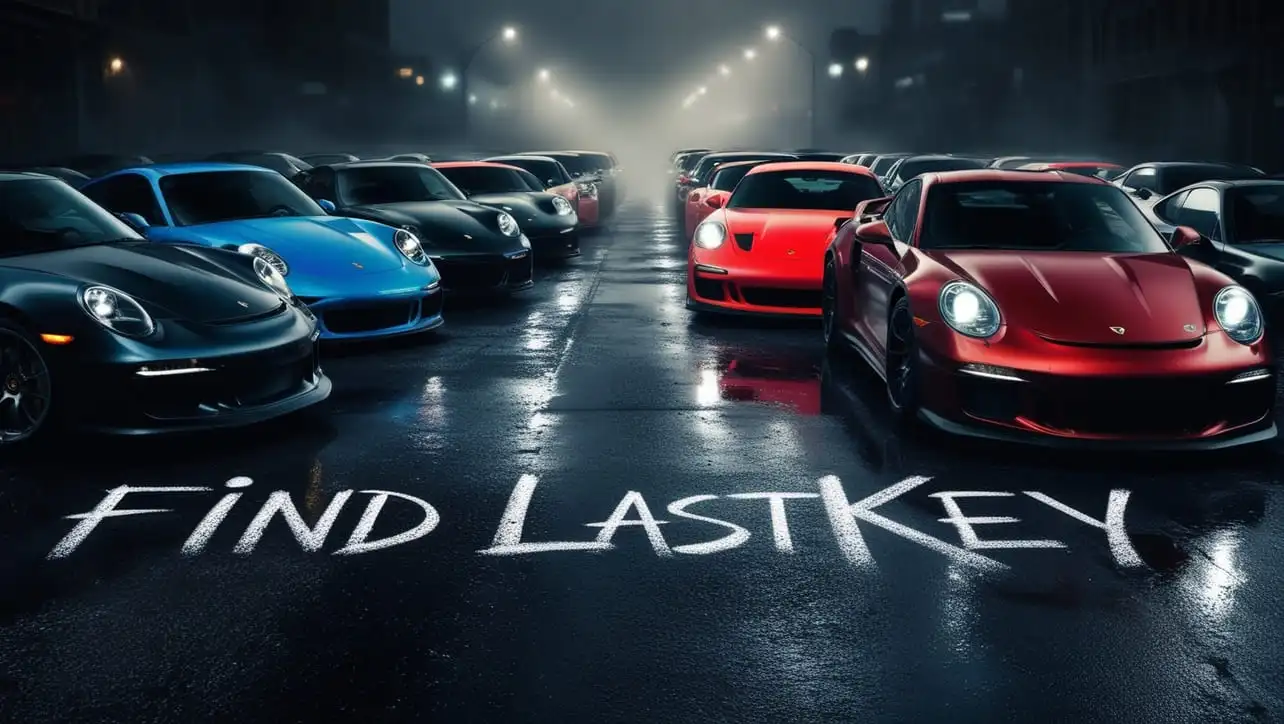
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, efficiently working with objects is a common necessity. Lodash, a powerful utility library, offers a plethora of functions to simplify object manipulation. Among these functions is the _.findLastKey()
method, a valuable tool for finding the last key in an object that satisfies a given condition.
This method provides flexibility and precision in object traversal, making it a must-have for developers dealing with complex data structures.
🧠 Understanding _.findLastKey() Method
The _.findLastKey()
method in Lodash is designed for object exploration. It iterates over the keys of an object, searching for the last key that matches a specified condition. This functionality is especially useful when you need to identify a key based on a custom criterion within an object.
💡 Syntax
The syntax for the _.findLastKey()
method is straightforward:
_.findLastKey(object, [predicate])
- object: The object to inspect.
- predicate (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.findLastKey()
method:
const _ = require('lodash');
const sampleObject = {
a: 10,
b: 20,
c: 30,
d: 40,
};
const lastKey = _.findLastKey(sampleObject, value => value > 25);
console.log(lastKey);
// Output: 'd'
In this example, the sampleObject is explored by _.findLastKey()
, searching for the last key where the corresponding value is greater than 25.
🏆 Best Practices
When working with the _.findLastKey()
method, consider the following best practices:
Handle Undefined Results:
Be prepared to handle cases where the result of
_.findLastKey()
is undefined. This can occur if no key in the object satisfies the provided condition.example.jsCopiedconst resultKey = _.findLastKey(sampleObject, value => value > 50); if(resultKey === undefined) { console.log('No key found matching the condition.'); } else { console.log(`Last key matching the condition: ${resultKey}`); }
Define Custom Criteria:
Utilize the predicate parameter to define custom criteria for key selection. This allows you to tailor the behavior of
_.findLastKey()
according to your specific requirements.example.jsCopiedconst customCondition = (value, key) => key.startsWith('c') && value < 35; const customKey = _.findLastKey(sampleObject, customCondition); console.log(`Last key matching custom condition: ${customKey}`);
Object Traversal Order:
Understand that the order of object traversal is not guaranteed in JavaScript, and
_.findLastKey()
will traverse the keys in their natural order. If order matters, consider using an array or another data structure.
📚 Use Cases
Finding Latest Timestamp Key:
_.findLastKey()
is handy when dealing with objects representing time-series data, allowing you to find the latest timestamp key.example.jsCopiedconst timeSeriesData = { '2022-01-01': 100, '2022-01-02': 150, '2022-01-03': 200, }; const latestTimestamp = _.findLastKey(timeSeriesData, (value, key) => value > 120); console.log(`Latest timestamp with value greater than 120: ${latestTimestamp}`);
Filtering Configurable Properties:
When working with configuration objects, you can use
_.findLastKey()
to filter out properties based on configurable criteria.example.jsCopiedconst config = { theme: 'dark', fontSize: 16, language: 'en', loggingEnabled: true, }; const lastConfigurableProperty = _.findLastKey(config, (value, key) => typeof value === 'string'); console.log(`Last configurable property: ${lastConfigurableProperty}`);
Dynamic Object Exploration:
For scenarios where object keys and values change dynamically,
_.findLastKey()
provides a flexible solution for dynamic object exploration.example.jsCopiedconst dynamicObject = /* ...some dynamically generated object... */; const dynamicKey = _.findLastKey(dynamicObject, value => /* ...some custom condition... */); console.log(`Last key based on dynamic condition: ${dynamicKey}`);
🎉 Conclusion
The _.findLastKey()
method in Lodash serves as a valuable asset for navigating and extracting information from objects in JavaScript. Whether you're searching for the latest timestamp key in time-series data or filtering properties based on dynamic conditions, this method provides a versatile and efficient solution.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.findLastKey()
method in your Lodash projects.
👨💻 Join our Community:
Author
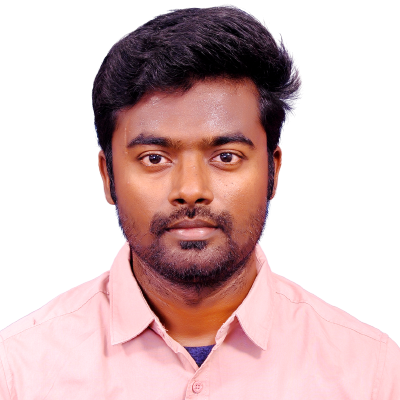
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
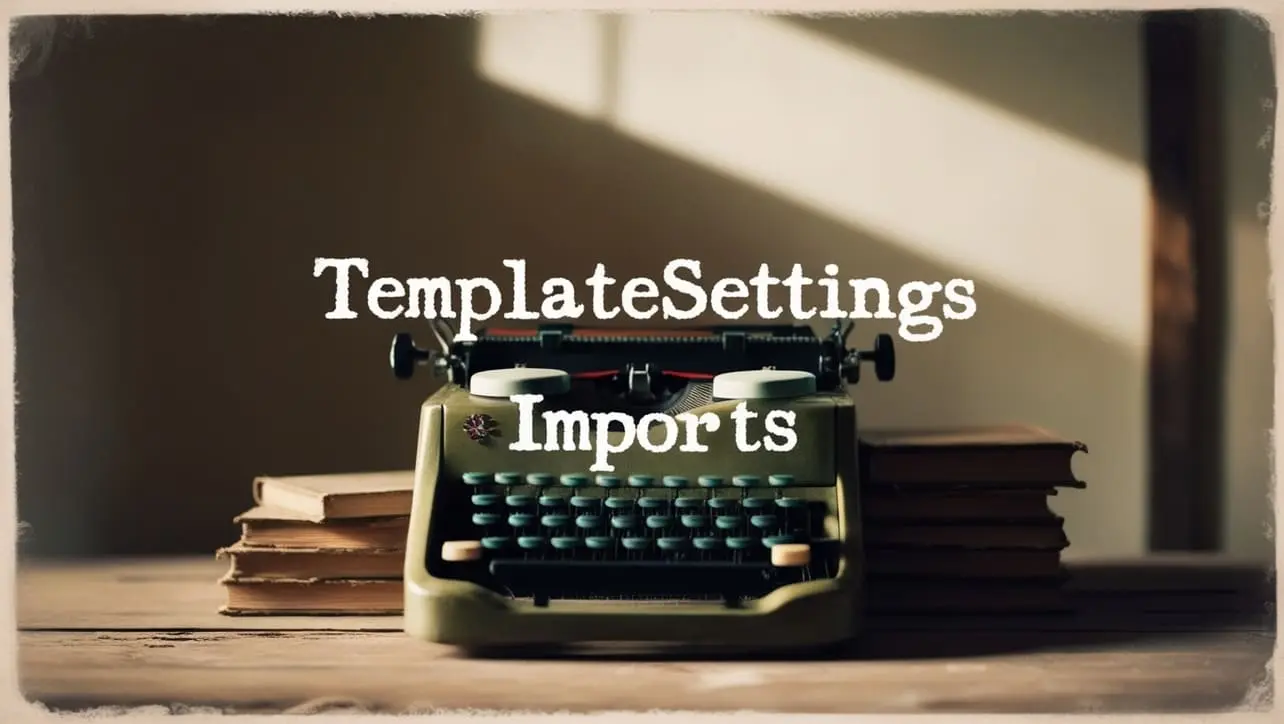
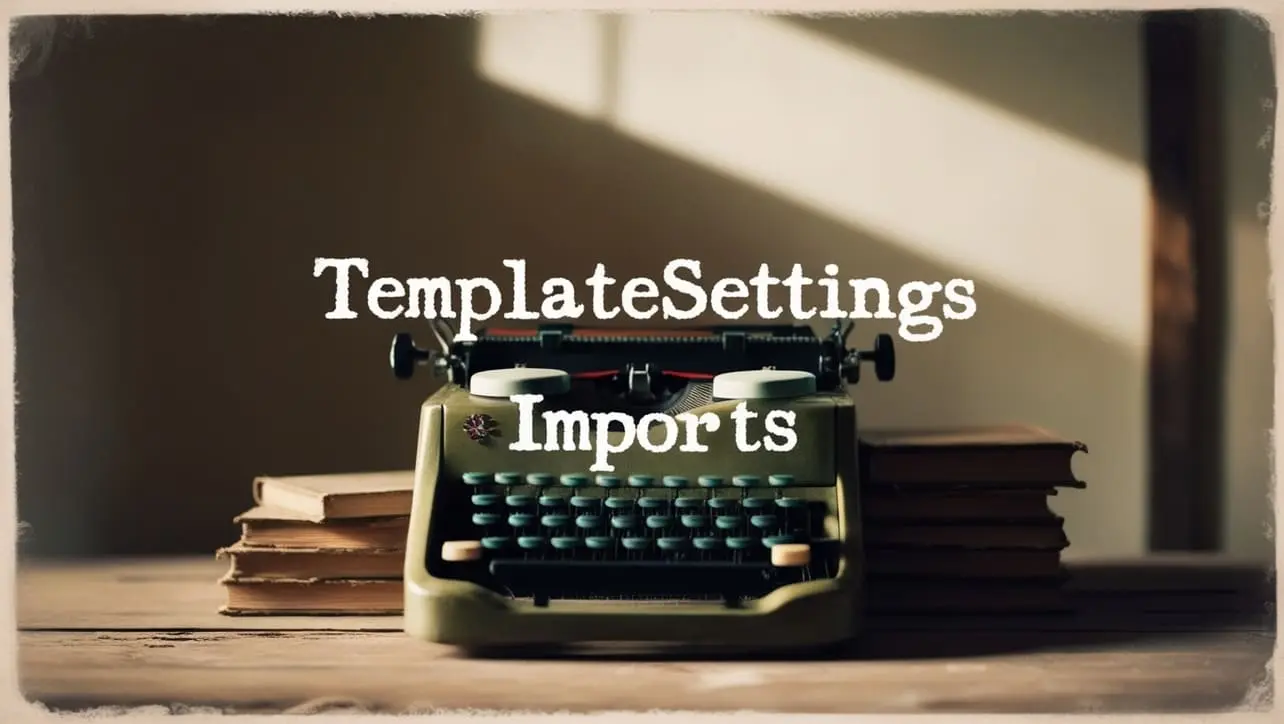
Lodash _.templateSettings.imports Property
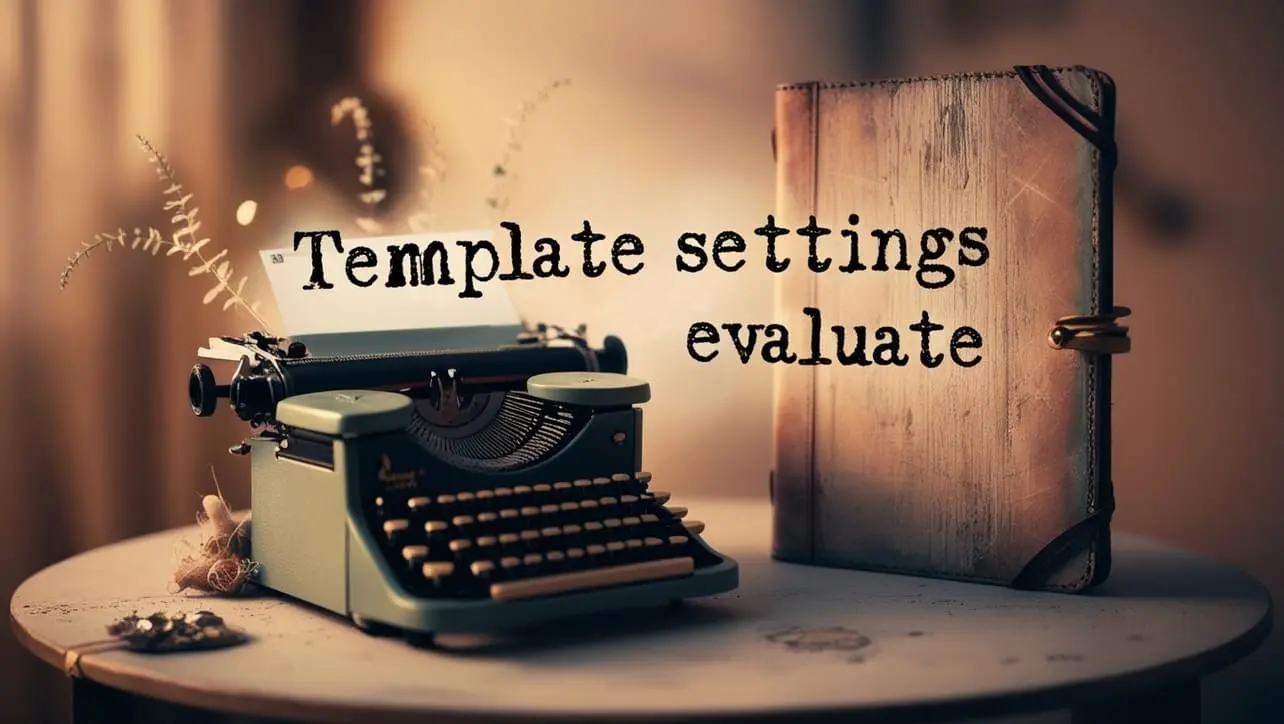
Lodash _.templateSettings.evaluate Property
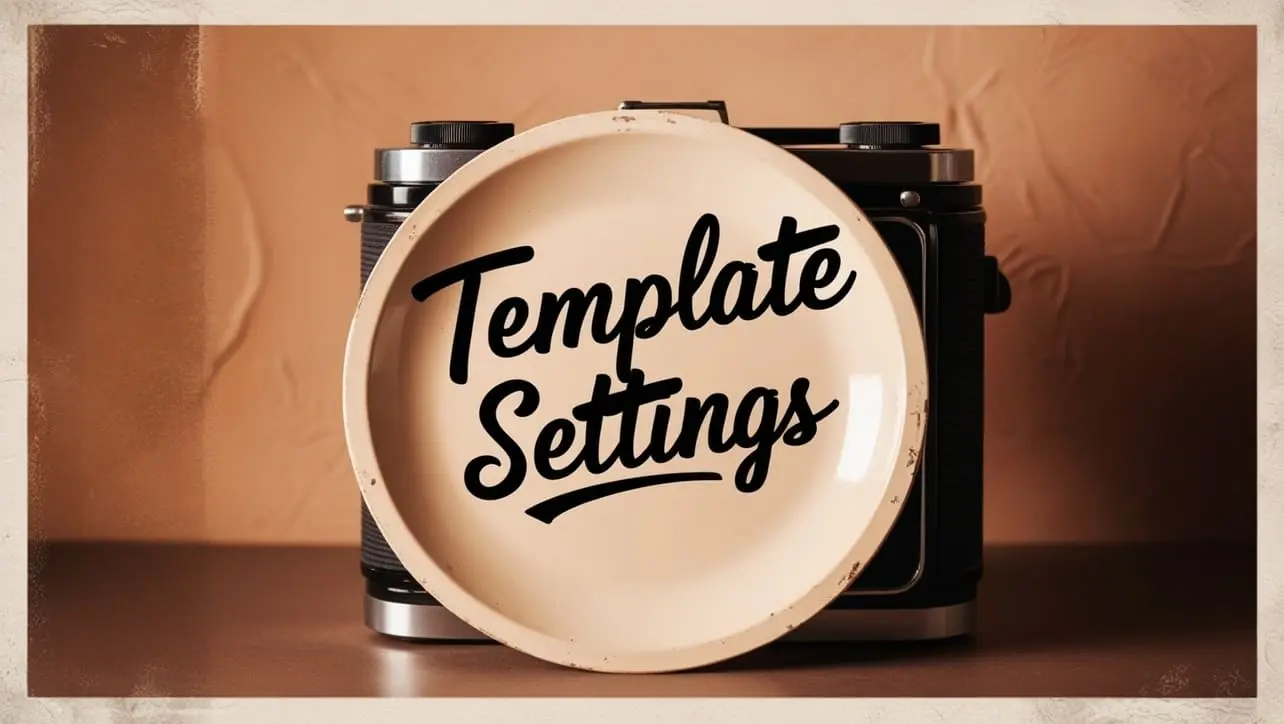
Lodash _.templateSettings Property
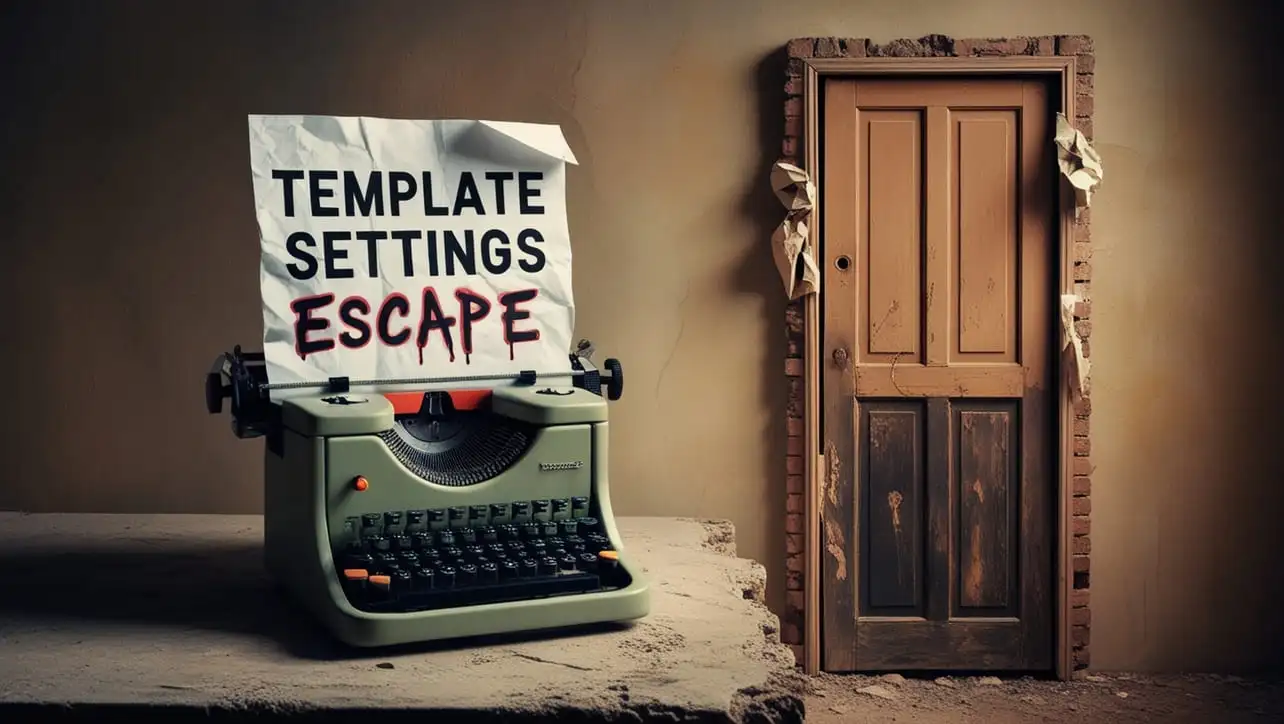
Lodash _.templateSettings.escape Property
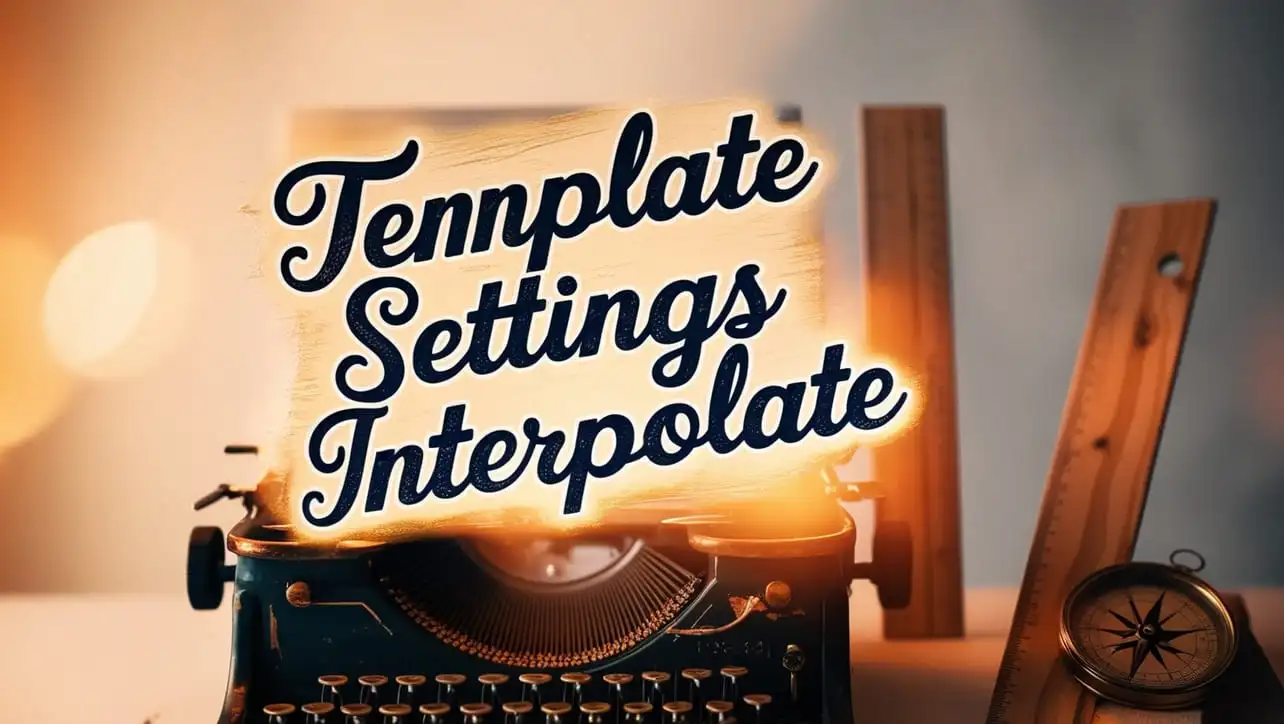
Lodash _.templateSettings.interpolate Property
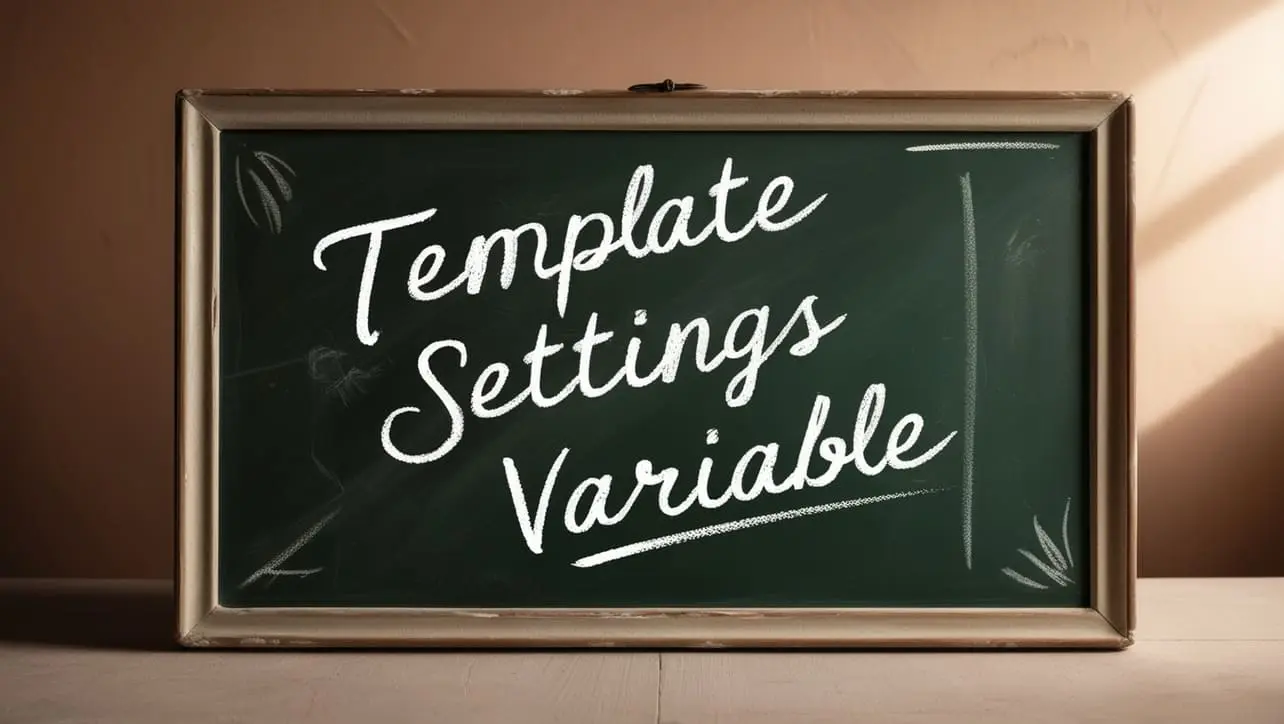
If you have any doubts regarding this article (Lodash _.findLastKey() Object Method), please comment here. I will help you immediately.