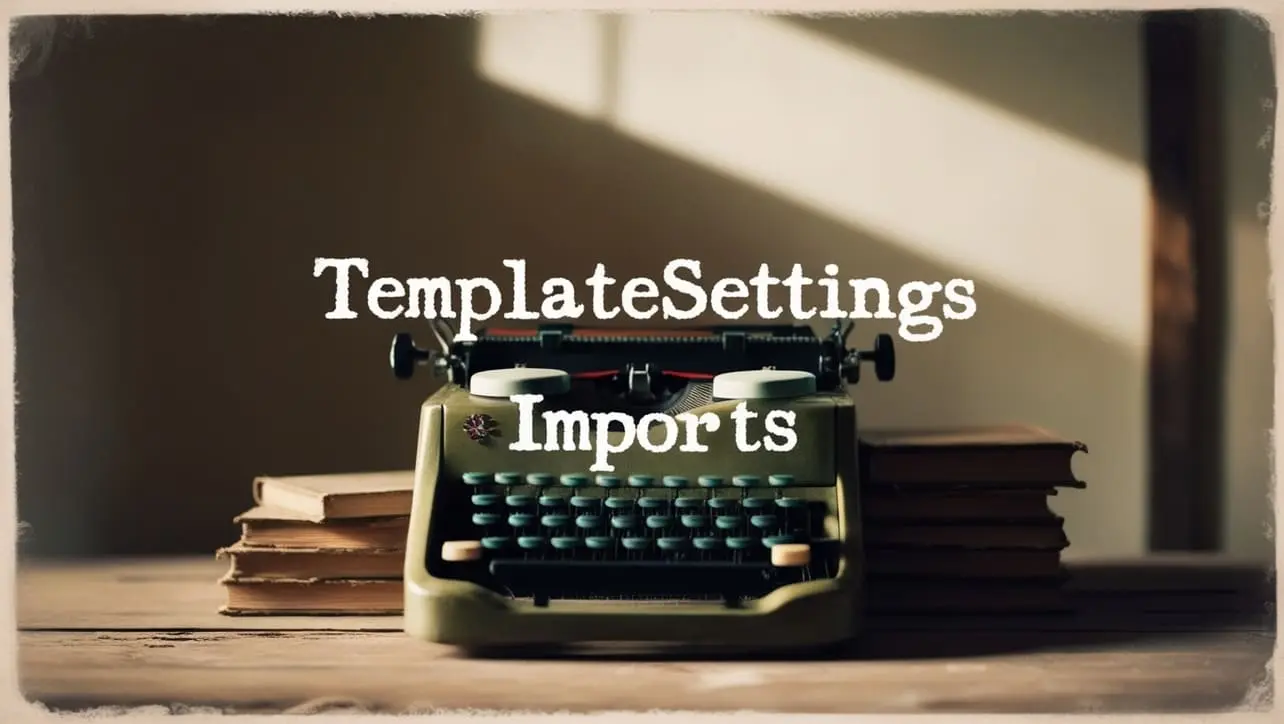
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.create() Object Method
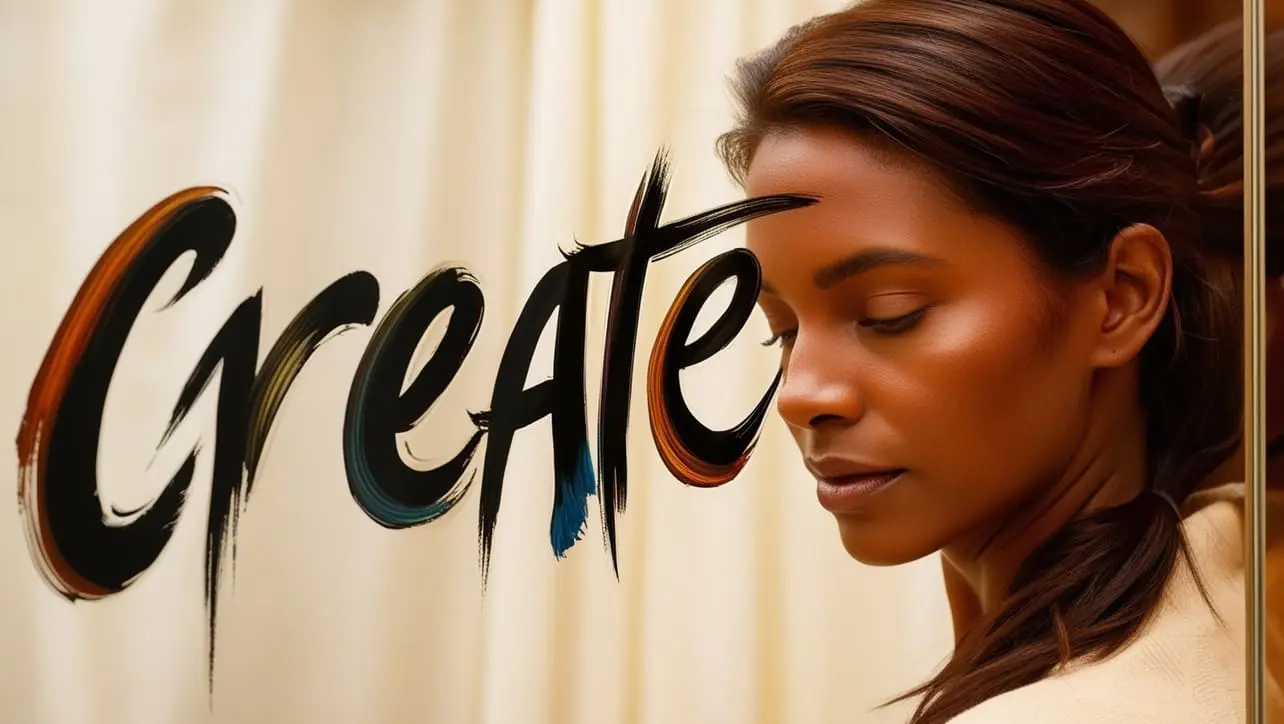
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, object creation and manipulation play a pivotal role. Lodash, a renowned utility library, offers a wealth of functions to streamline these tasks. Among them, the _.create()
method stands out, providing a versatile way to create objects with customizable prototypes.
This method empowers developers to construct objects efficiently while maintaining flexibility in their application structure.
🧠 Understanding _.create() Method
The _.create()
method in Lodash is designed to create a new object with the specified prototype. This prototype can be any object, allowing for the inheritance of properties and methods. This method is particularly useful for establishing a chain of prototypes or crafting objects with shared functionalities.
💡 Syntax
The syntax for the _.create()
method is straightforward:
_.create(prototype, [properties])
- prototype: The object to use as a prototype.
- properties (Optional): The properties to set on the newly created object.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.create()
method:
const _ = require('lodash');
// Create a prototype object
const animalPrototype = {
makeSound: function() {
console.log('Generic animal sound');
},
};
// Create a new object inheriting from the prototype
const cat = _.create(animalPrototype, {
species: 'Cat'
});
// Access properties and methods
console.log(cat.species); // Output: Cat
cat.makeSound(); // Output: Generic animal sound
In this example, cat is created with animalPrototype as its prototype, inheriting the makeSound method and having an additional property species.
🏆 Best Practices
When working with the _.create()
method, consider the following best practices:
Define Clear Prototypes:
When using
_.create()
, ensure that your prototypes are well-defined and encapsulate the shared properties and methods relevant to the objects you intend to create.example.jsCopiedconst vehiclePrototype = { startEngine: function() { console.log('Engine started'); }, stopEngine: function() { console.log('Engine stopped'); }, }; const car = _.create(vehiclePrototype, { type: 'Sedan' }); car.startEngine(); // Output: Engine started
Leverage Prototypal Inheritance:
Exploit the power of prototypal inheritance by creating chains of objects. This allows for the sharing of methods and properties across multiple levels of your application structure.
example.jsCopiedconst shapePrototype = { calculateArea: function() { console.log('Calculating area'); }, }; const circle = _.create(shapePrototype, { type: 'Circle' }); const square = _.create(shapePrototype, { type: 'Square' }); circle.calculateArea(); // Output: Calculating area square.calculateArea(); // Output: Calculating area
Customize Object Properties:
Take advantage of the optional properties parameter to customize the properties of the newly created object. This provides flexibility in tailoring objects to specific requirements.
example.jsCopiedconst userPrototype = { greet: function() { console.log(`Hello, ${this.name}!`); }, }; const newUser = _.create(userPrototype, { name: 'John Doe', age: 30 }); newUser.greet(); // Output: Hello, John Doe! console.log(newUser.age); // Output: 30
📚 Use Cases
Object Factories:
Use
_.create()
to create object factories, enabling the efficient generation of objects with shared behaviors and structures.example.jsCopiedfunction createPerson(name, age) { return _.create(userPrototype, { name, age }); } const person1 = createPerson('Alice', 25); const person2 = createPerson('Bob', 30); person1.greet(); // Output: Hello, Alice! person2.greet(); // Output: Hello, Bob!
Prototype Chains:
Build prototype chains for complex applications, organizing shared functionalities at various levels of the chain.
example.jsCopiedconst mammalPrototype = { giveBirth: function() { console.log('Live birth'); }, }; const dogPrototype = _.create(mammalPrototype, { breed: 'Labrador', bark: function() { console.log('Woof, woof!'); }, }); const labrador = _.create(dogPrototype, { color: 'Golden' }); labrador.giveBirth(); // Output: Live birth labrador.bark(); // Output: Woof, woof!
Dynamic Object Configuration:
Dynamically configure objects by providing different prototypes and properties based on runtime conditions or user input.
example.jsCopiedfunction createDynamicObject(type) { const basePrototype = { displayType: function() { console.log(`Object type: ${this.type}`); }, }; const specificProperties = type === 'A' ? { propertyA: true } : { propertyB: true }; return _.create(basePrototype, { type, ...specificProperties }); } const dynamicObjectA = createDynamicObject('A'); const dynamicObjectB = createDynamicObject('B'); dynamicObjectA.displayType(); // Output: Object type: A console.log(dynamicObjectA.propertyA); // Output: true dynamicObjectB.displayType(); // Output: Object type: B console.log(dynamicObjectB.propertyB); // Output: true
🎉 Conclusion
The _.create()
method in Lodash offers a versatile approach to object creation and prototypal inheritance in JavaScript. Whether you're building prototype chains, creating object factories, or dynamically configuring objects, _.create()
provides a powerful tool for crafting flexible and efficient object structures.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.create()
method in your Lodash projects.
👨💻 Join our Community:
Author
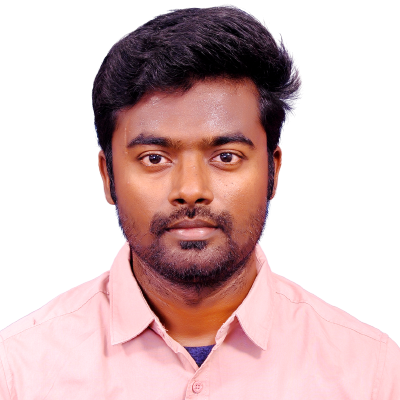
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
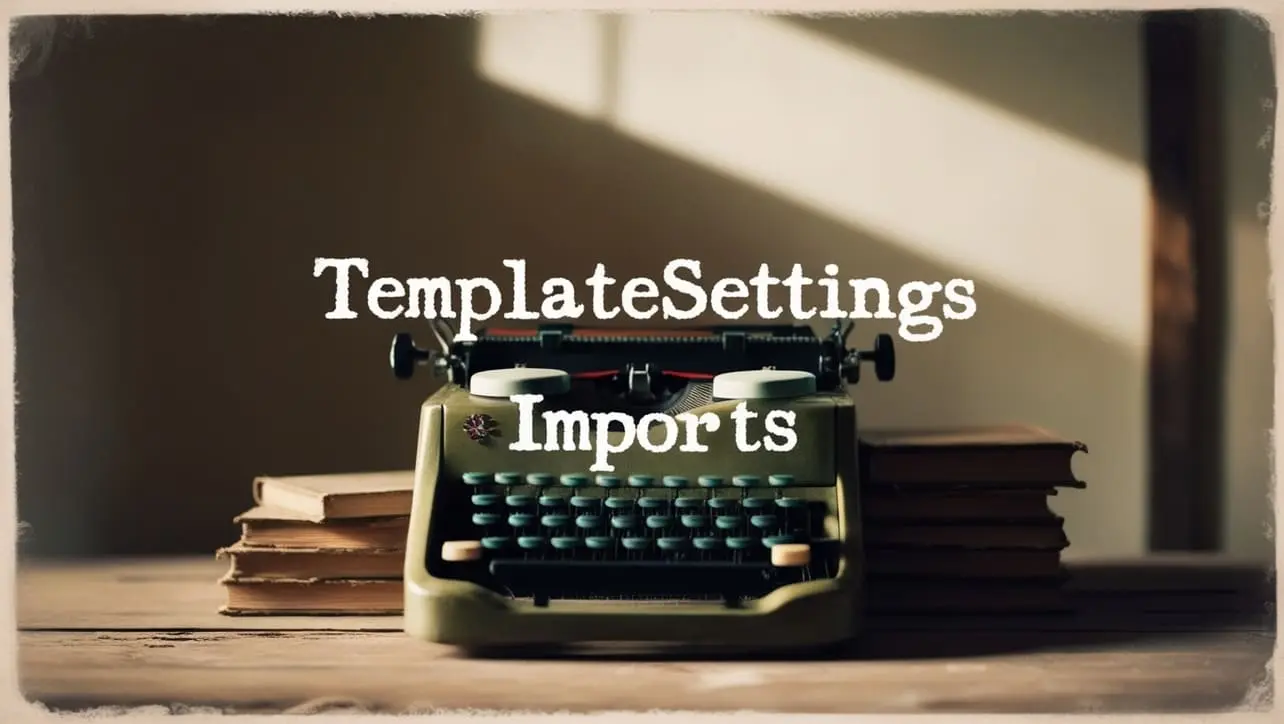
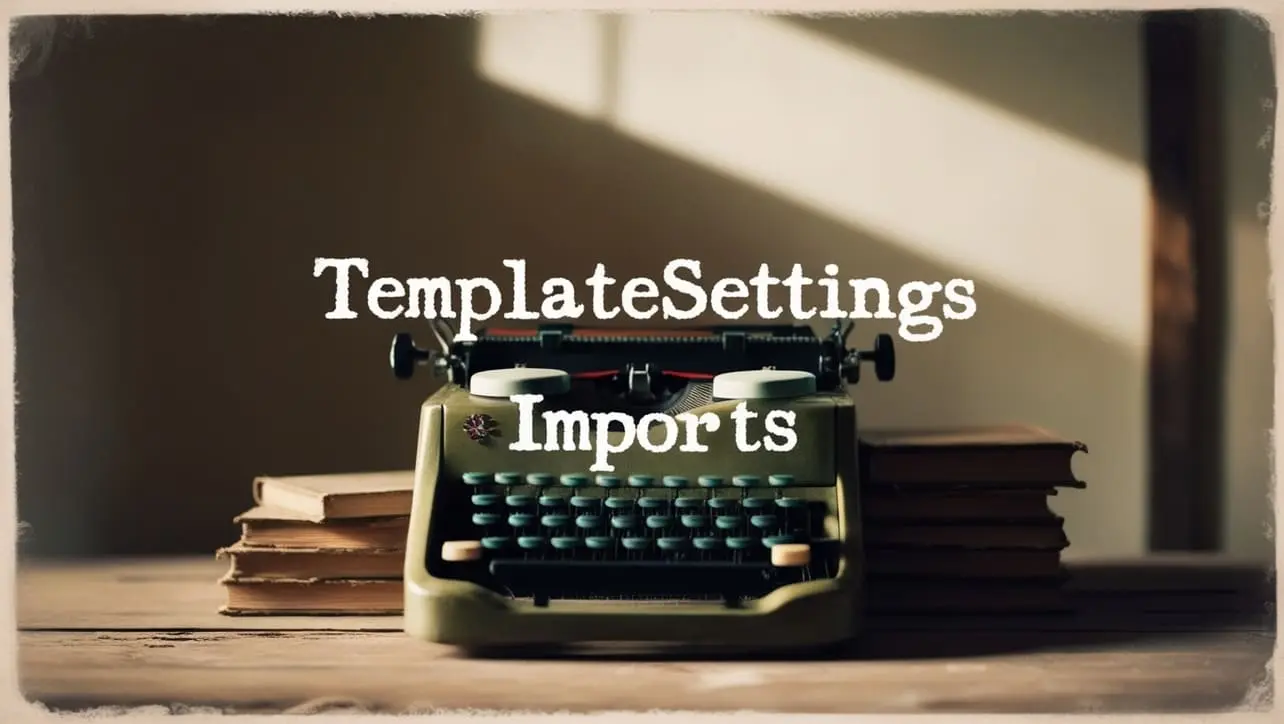
Lodash _.templateSettings.imports Property
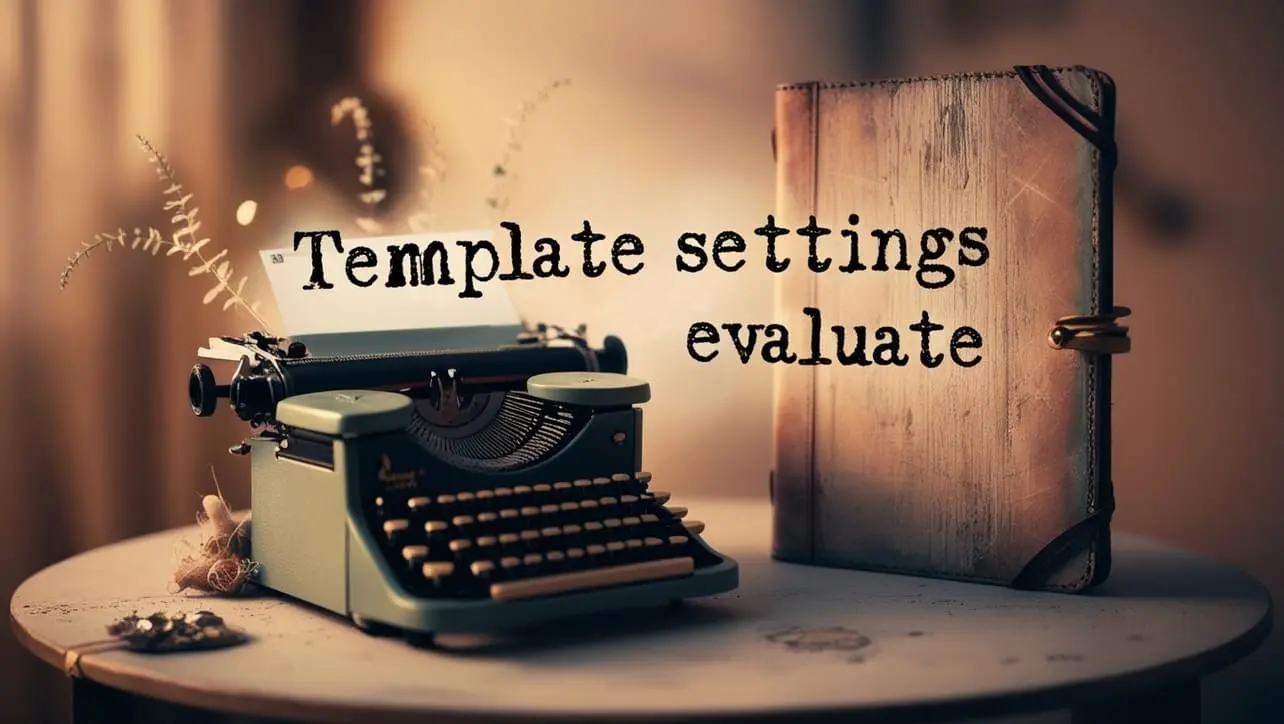
Lodash _.templateSettings.evaluate Property
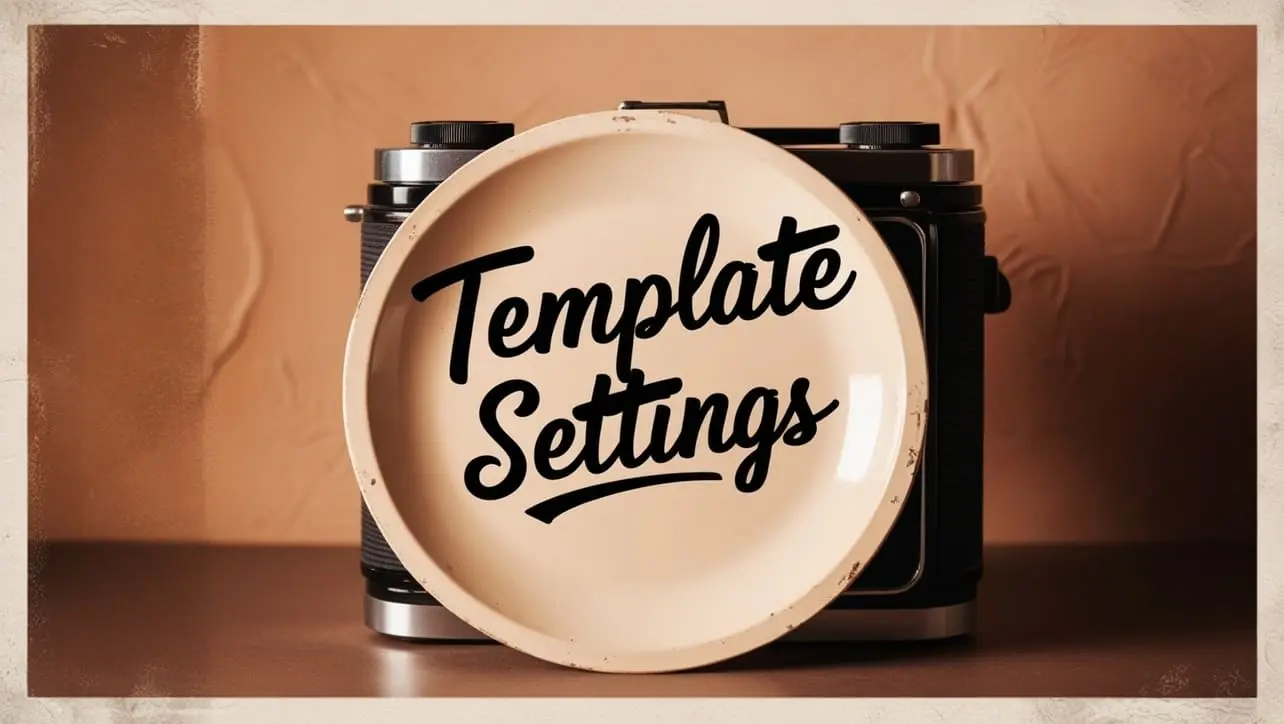
Lodash _.templateSettings Property
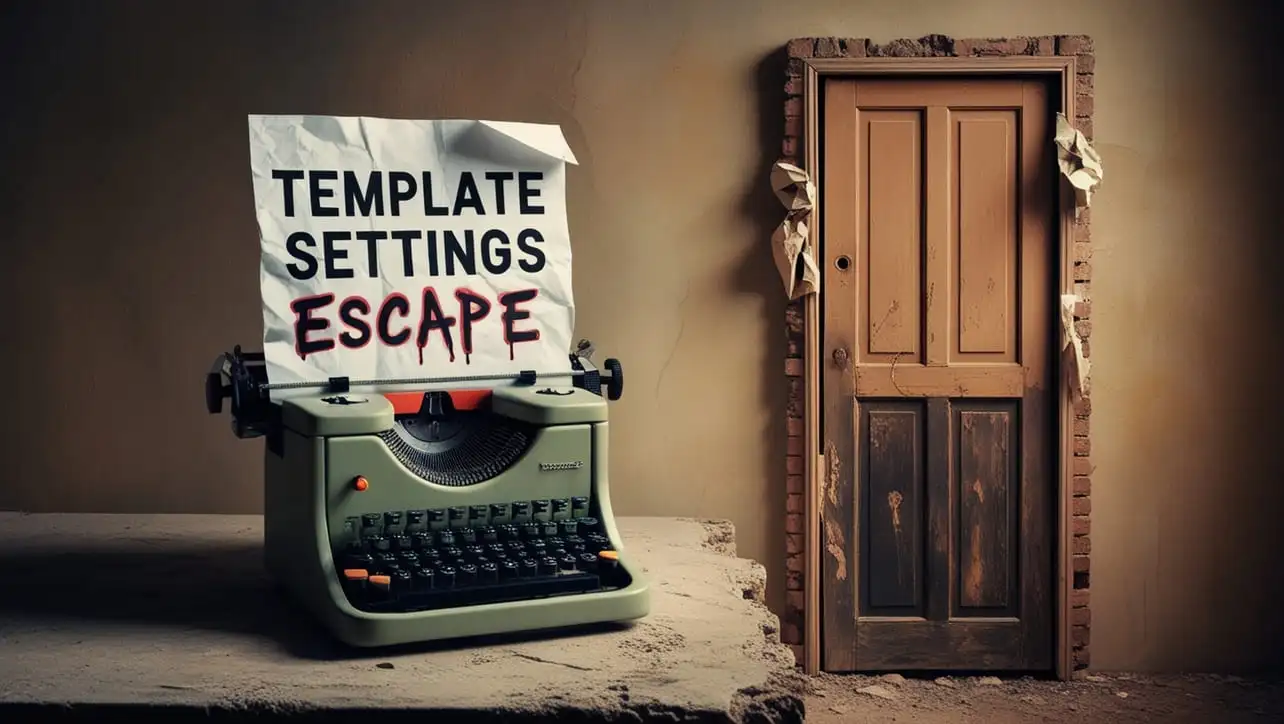
Lodash _.templateSettings.escape Property
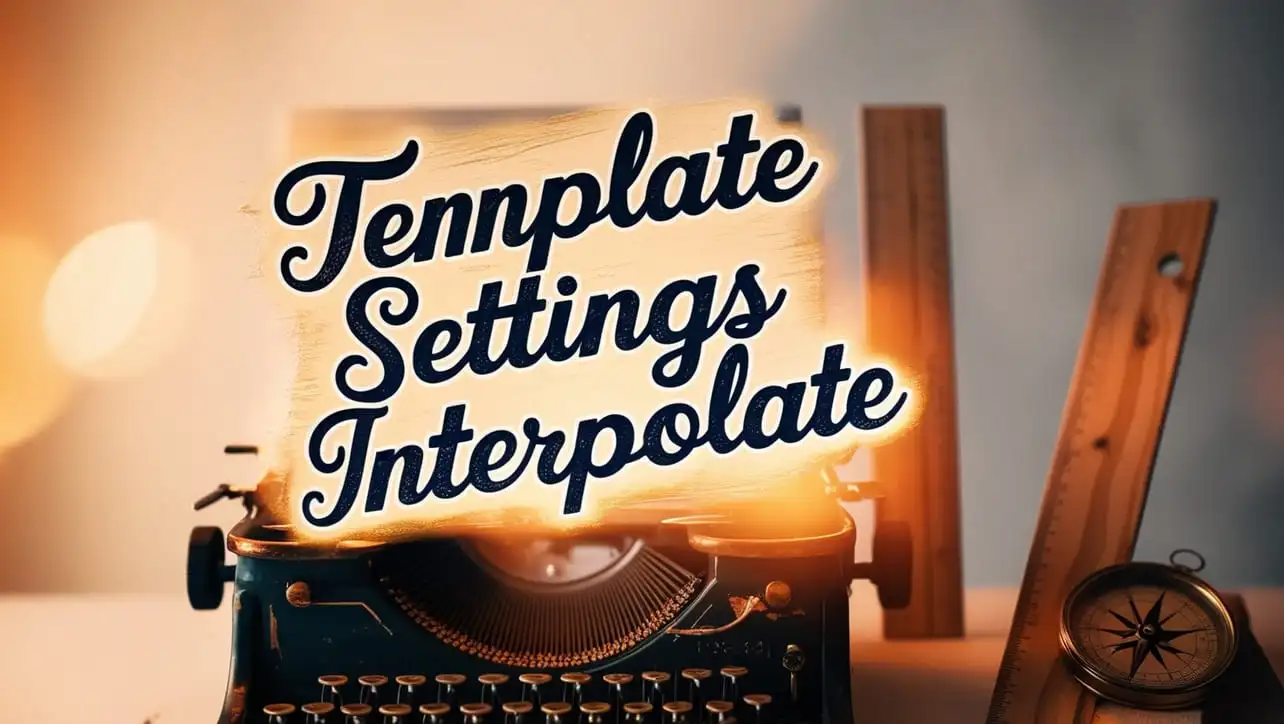
Lodash _.templateSettings.interpolate Property
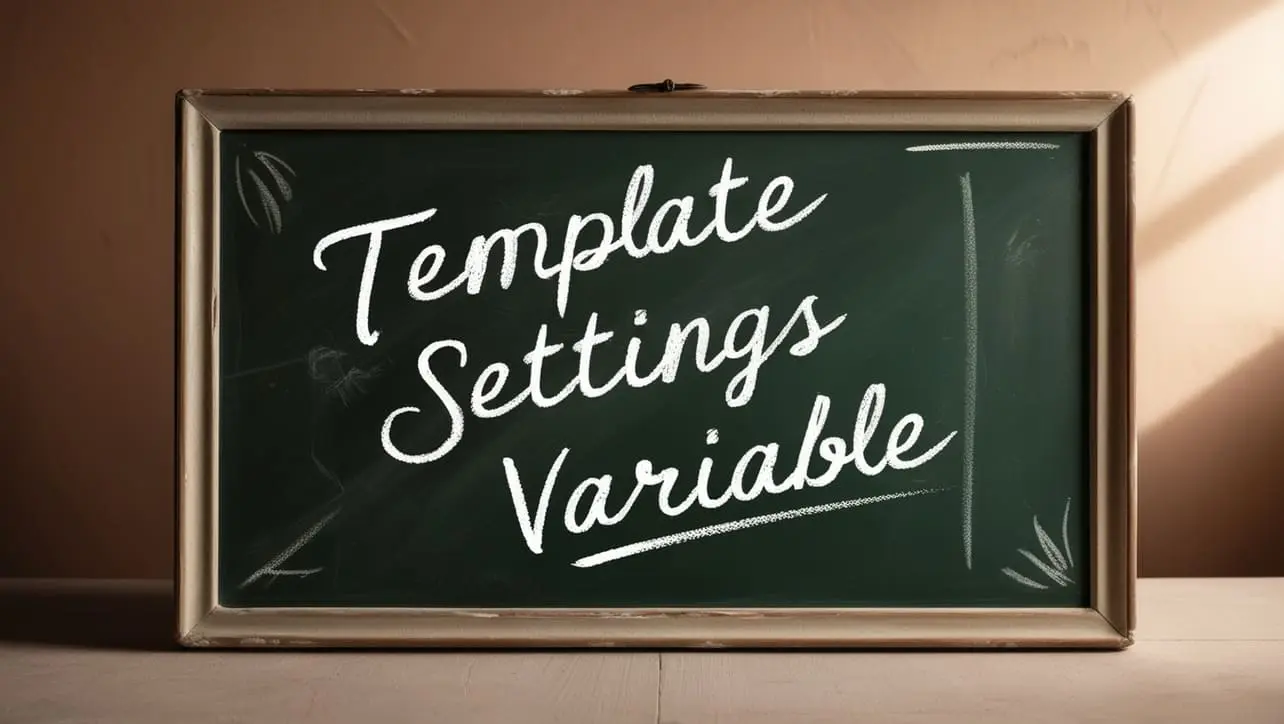
If you have any doubts regarding this article (Lodash _.create() Object Method), please comment here. I will help you immediately.