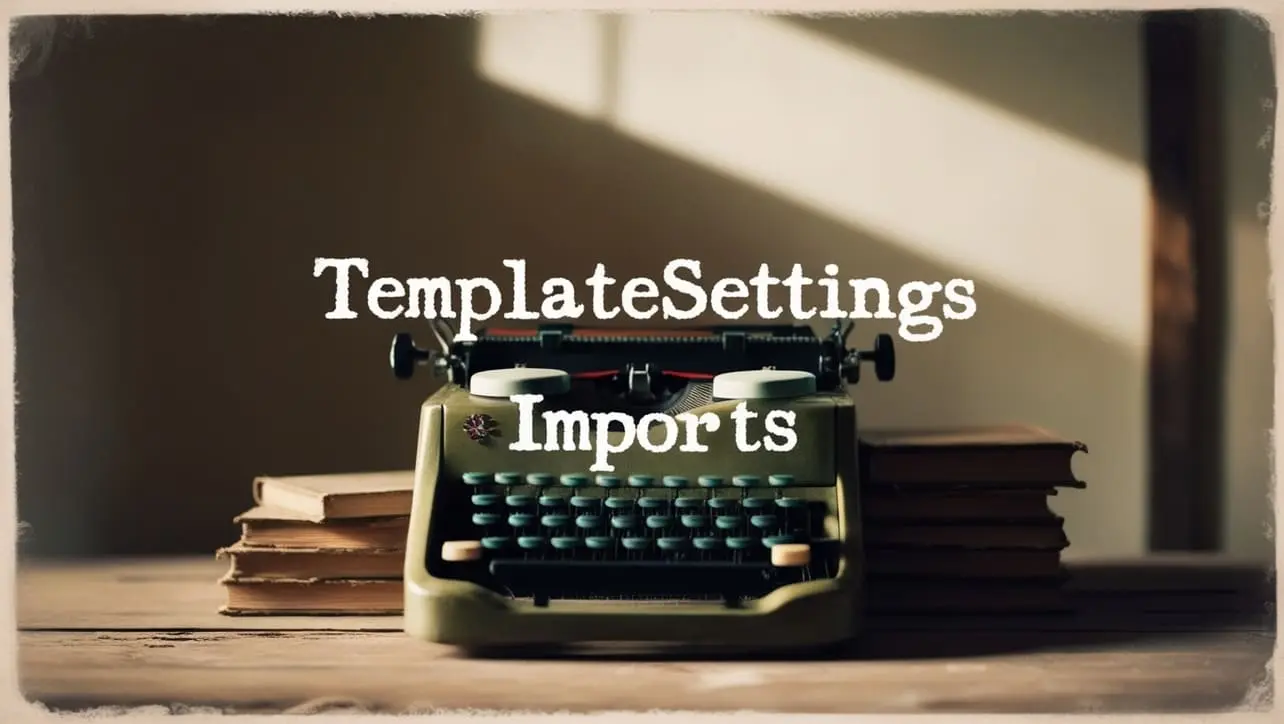
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.assignWith() Object Method
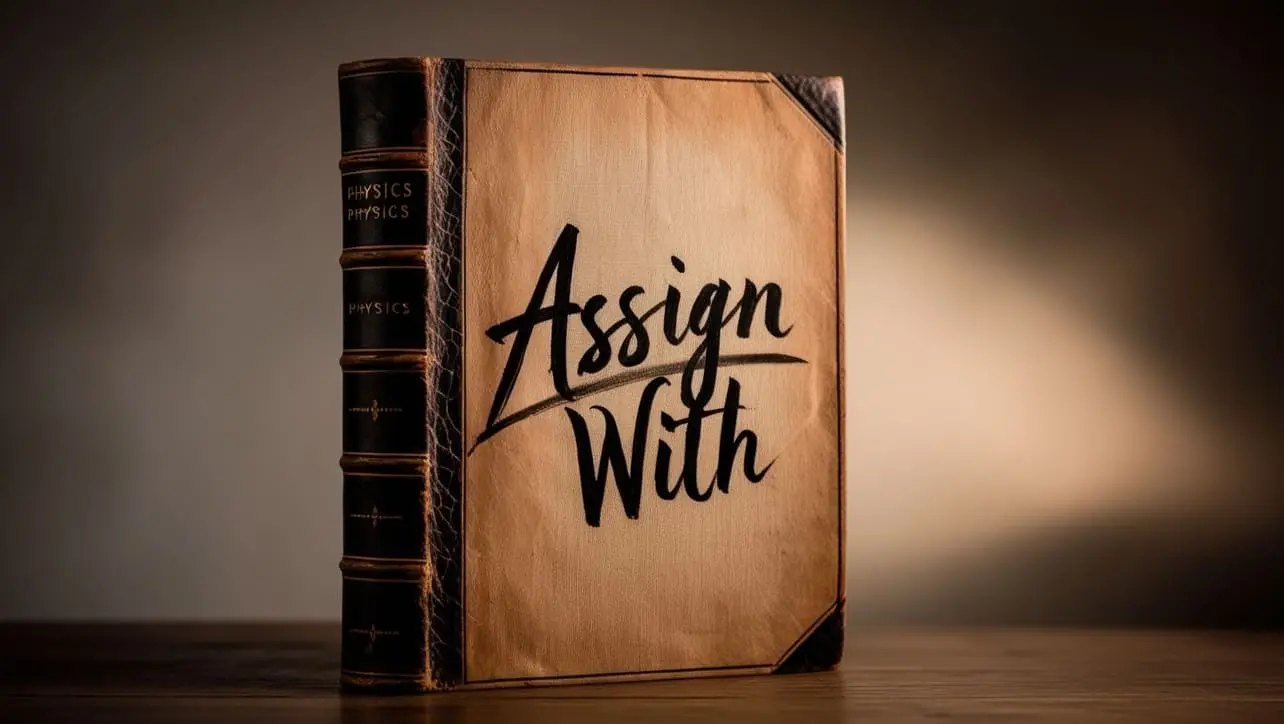
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript, efficient handling of objects is crucial for developers. Lodash, a feature-rich utility library, provides a plethora of functions to simplify object manipulation. One such indispensable tool is the _.assignWith()
method. This method allows developers to merge multiple source objects into a target object while providing a customizer function to control the merging process.
Let's delve into the details of _.assignWith()
and explore its applications in JavaScript development.
🧠 Understanding _.assignWith() Method
The _.assignWith()
method in Lodash is designed for merging multiple source objects into a target object. What sets it apart is the ability to customize the merging behavior using a customizer function. This function gives developers fine-grained control over how conflicts in key-value pairs are resolved during the merge.
💡 Syntax
The syntax for the _.assignWith()
method is straightforward:
_.assignWith(object, sources, customizer)
- object: The target object.
- sources: The source objects to merge.
- customizer: The function to customize assigned values.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.assignWith()
method:
const _ = require('lodash');
const targetObject = { a: 1, b: 2 };
const source1 = { b: 3, c: 4 };
const source2 = { d: 5 };
const mergedObject = _.assignWith(targetObject, source1, source2, (objValue, srcValue) => objValue + srcValue);
console.log(mergedObject);
// Output: { a: 1, b: 5, c: 4, d: 5 }
In this example, targetObject is merged with source1 and source2. The customizer function is used to sum the values for common keys, resulting in a merged object.
🏆 Best Practices
When working with the _.assignWith()
method, consider the following best practices:
Understand Customizer Function:
Comprehend the role of the customizer function in resolving conflicts during the merge. The customizer function takes four arguments: (objValue, srcValue, key, object).
example.jsCopiedconst customMerge = (objValue, srcValue, key) => { if(key === 'priority') { return Math.max(objValue, srcValue); } return undefined; // Default behavior for non-'priority' keys }; const mergedWithCustomizer = _.assignWith({ priority: 2 }, { priority: 5, status: 'active' }, customMerge); console.log(mergedWithCustomizer); // Output: { priority: 5, status: 'active' }
Handle Nested Objects:
When dealing with nested objects, ensure that the customizer function appropriately handles the merging process for nested structures.
example.jsCopiedconst targetNested = { a: { b: 2 } }; const sourceNested = { a: { c: 3 } }; const mergedNested = _.assignWith(targetNested, sourceNested, (objValue, srcValue) => { if(_.isObject(objValue)) { return _.assignWith(objValue, srcValue, (nestedObj, nestedSrc) => nestedObj + nestedSrc); } return undefined; }); console.log(mergedNested); // Output: { a: { b: 2, c: 3 } }
Maintain Immutability:
If maintaining immutability is a concern, consider using the spread operator or other techniques to create a new object instead of modifying the target object in-place.
example.jsCopiedconst targetImmutable = { a: 1 }; const sourceImmutable = { b: 2 }; const mergedImmutable = { ...targetImmutable, ...sourceImmutable }; console.log(mergedImmutable); // Output: { a: 1, b: 2 }
📚 Use Cases
Conflict Resolution:
_.assignWith()
is particularly useful when merging objects with conflicting keys. The customizer function allows you to define rules for resolving conflicts.example.jsCopiedconst targetConflicts = { a: 1, b: 2, priority: 3 }; const sourceConflicts = { b: 4, priority: 5, status: 'active' }; const resolvedConflicts = _.assignWith(targetConflicts, sourceConflicts, (objValue, srcValue, key) => { if(key === 'priority') { return Math.max(objValue, srcValue); } return undefined; // Default behavior for non-'priority' keys }); console.log(resolvedConflicts); // Output: { a: 1, b: 4, priority: 5, status: 'active' }
Custom Merging Logic:
When standard merging logic is insufficient, the customizer function empowers you to implement complex merging strategies tailored to your specific use case.
example.jsCopiedconst targetCustom = { a: 1, data: { x: 2 } }; const sourceCustom = { b: 3, data: { y: 4 } }; const customMergeLogic = (objValue, srcValue, key) => { if(key === 'data') { return { ...objValue, ...srcValue }; // Merge nested objects with spread operator } return undefined; // Default behavior for other keys }; const mergedWithCustomLogic = _.assignWith(targetCustom, sourceCustom, customMergeLogic); console.log(mergedWithCustomLogic); // Output: { a: 1, b: 3, data: { x: 2, y: 4 } }
Configuring Default Values:
_.assignWith()
can be used to merge objects while providing default values for missing keys.example.jsCopiedconst targetDefaults = { a: 1, b: 2 }; const sourceDefaults = { b: 3, c: 4 }; const mergedWithDefaults = _.assignWith(targetDefaults, sourceDefaults, (objValue, srcValue) => { if(_.isUndefined(objValue)) { return srcValue; } return objValue; }); console.log(mergedWithDefaults); // Output: { a: 1, b: 2, c: 4 }
🎉 Conclusion
The _.assignWith()
method in Lodash is a versatile tool for merging objects with the added flexibility of a customizer function. Whether you're resolving conflicts, implementing custom merging logic, or configuring default values, this method provides a powerful solution for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.assignWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
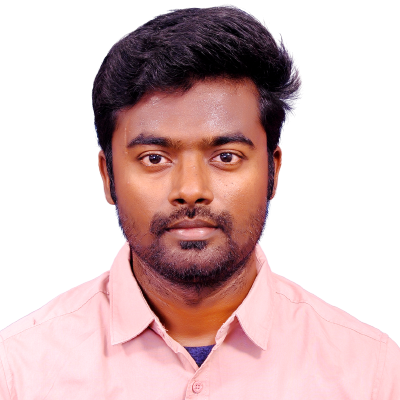
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
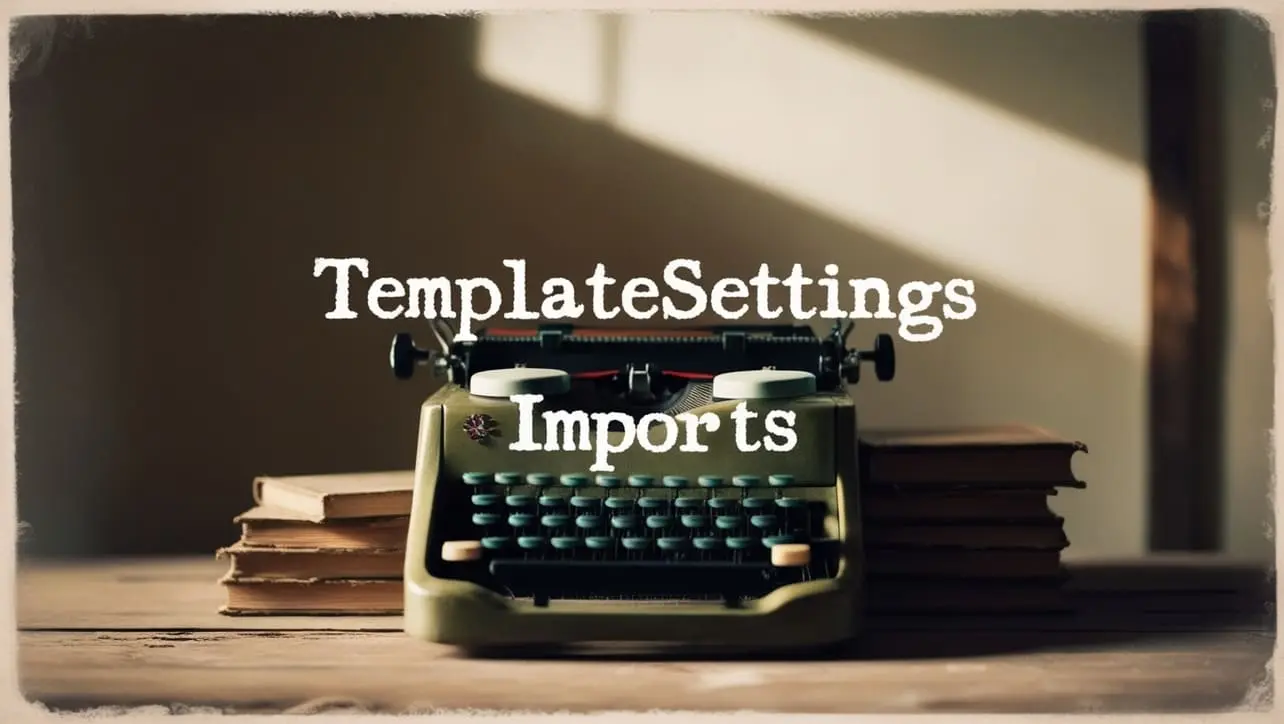
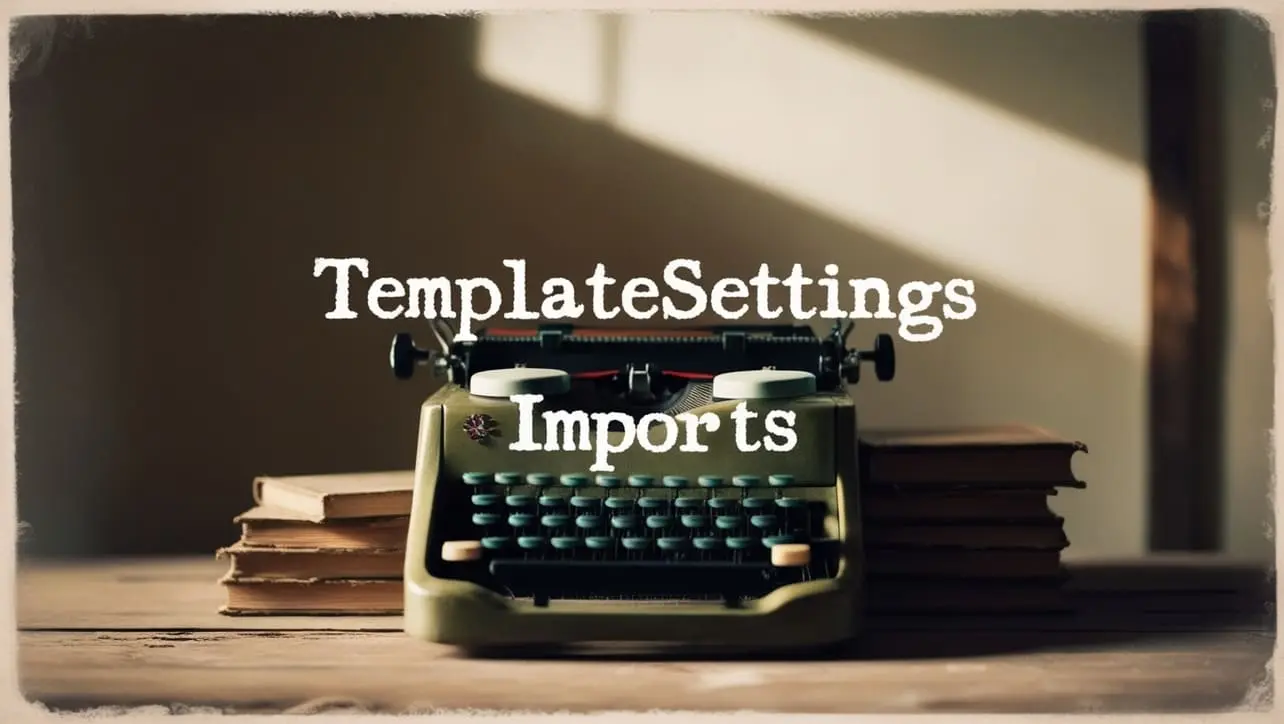
Lodash _.templateSettings.imports Property
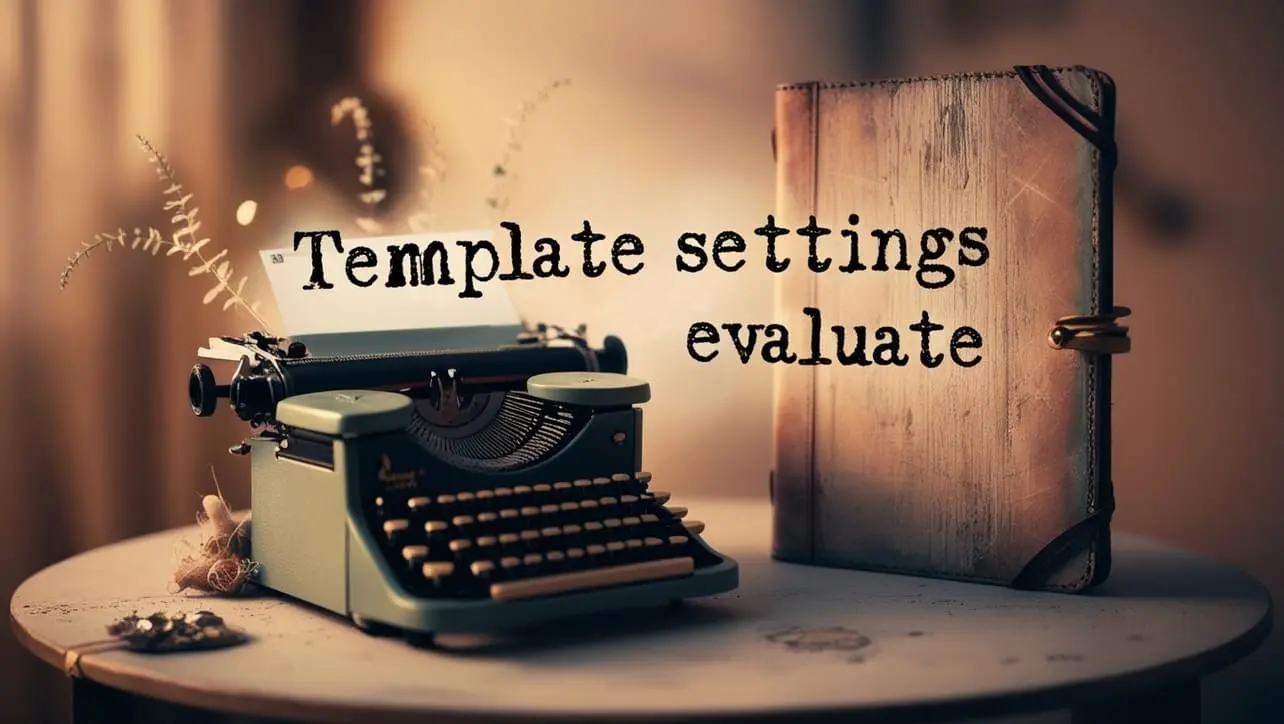
Lodash _.templateSettings.evaluate Property
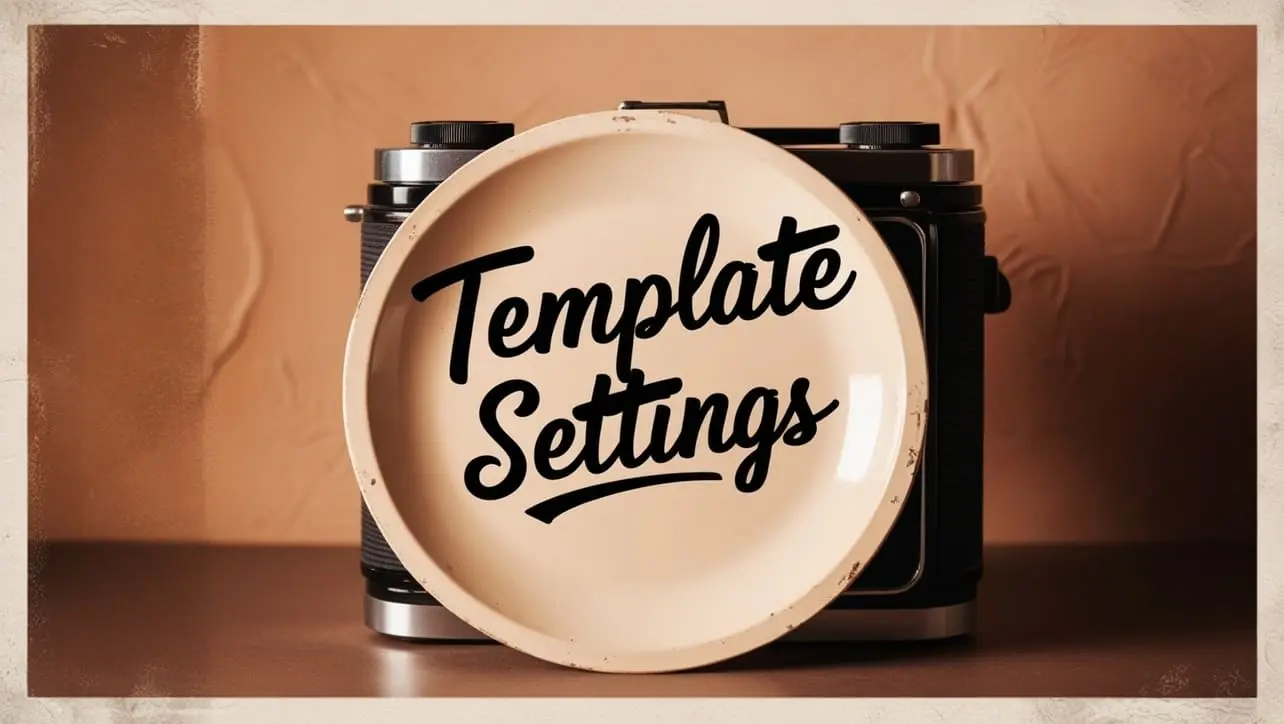
Lodash _.templateSettings Property
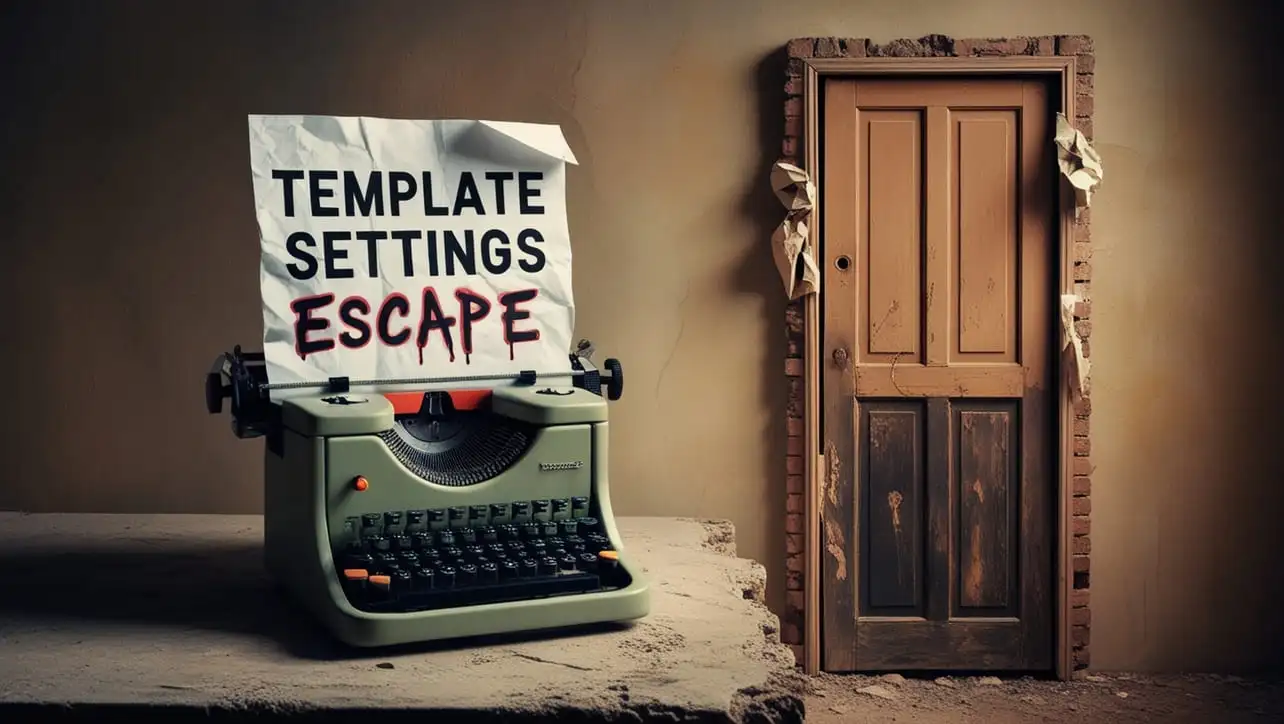
Lodash _.templateSettings.escape Property
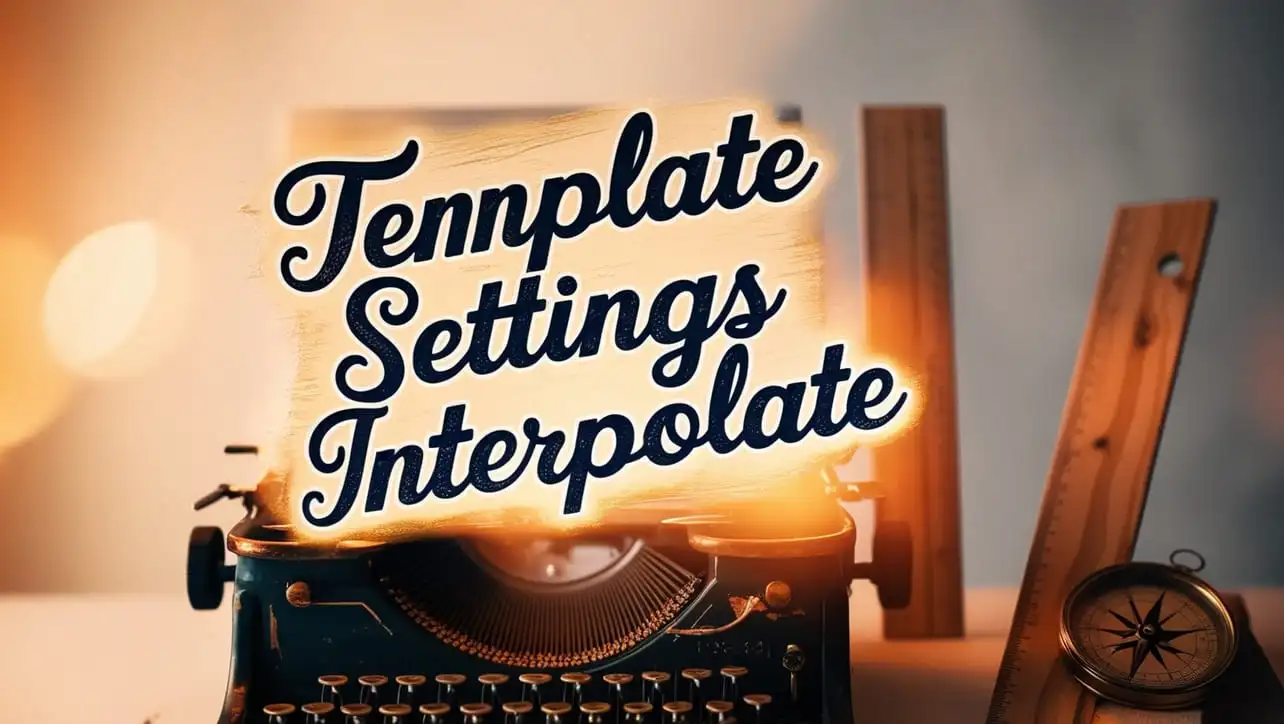
Lodash _.templateSettings.interpolate Property
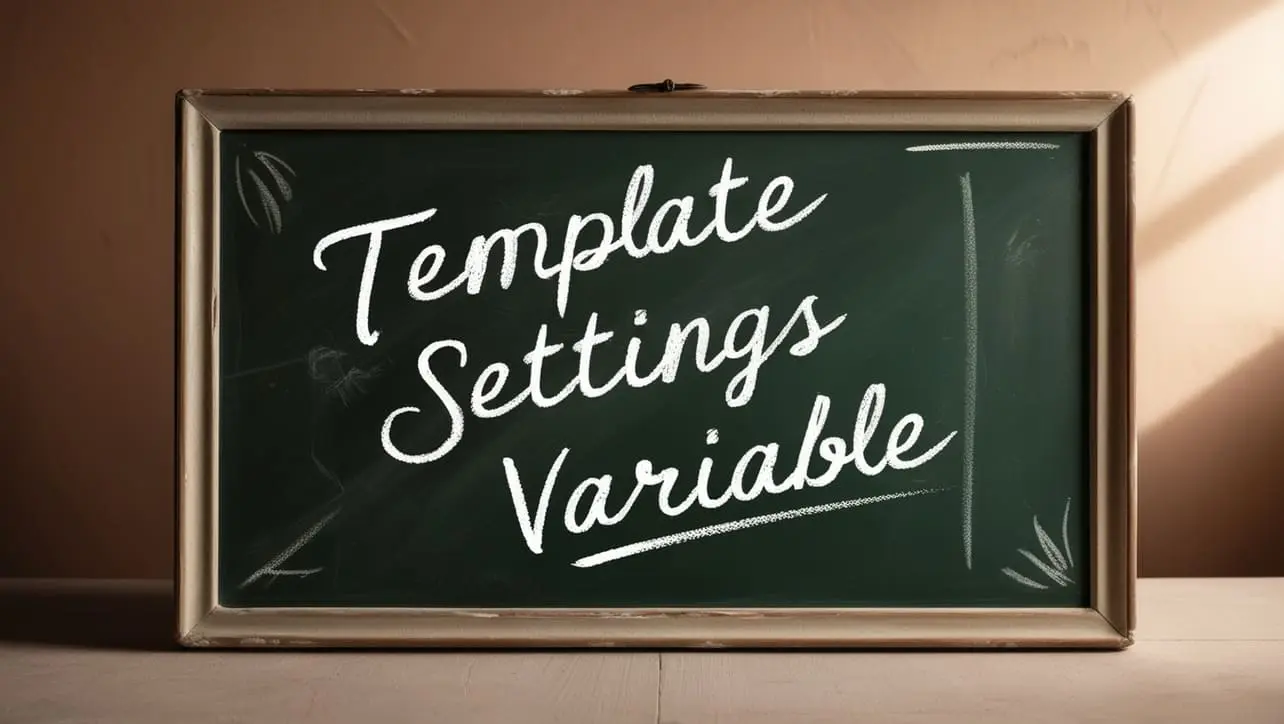
If you have any doubts regarding this article (Lodash _.assignWith() Object Method), please comment here. I will help you immediately.