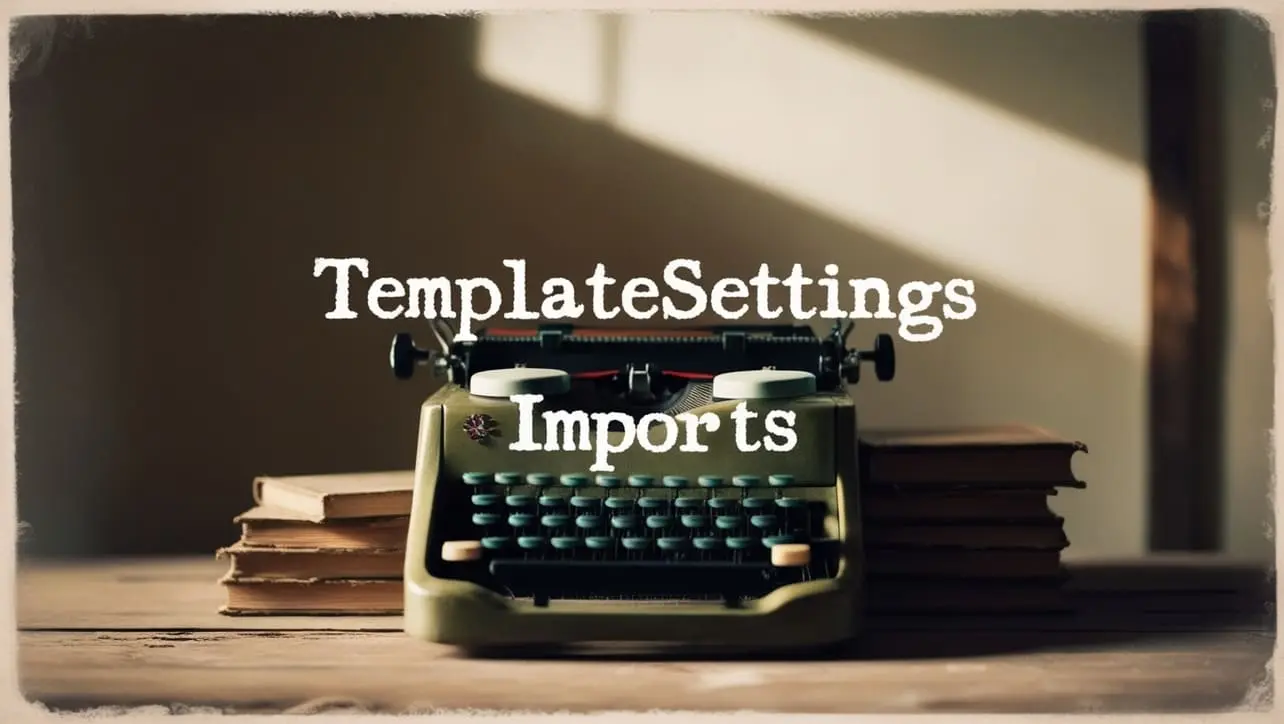
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.assignInWith() Object Method
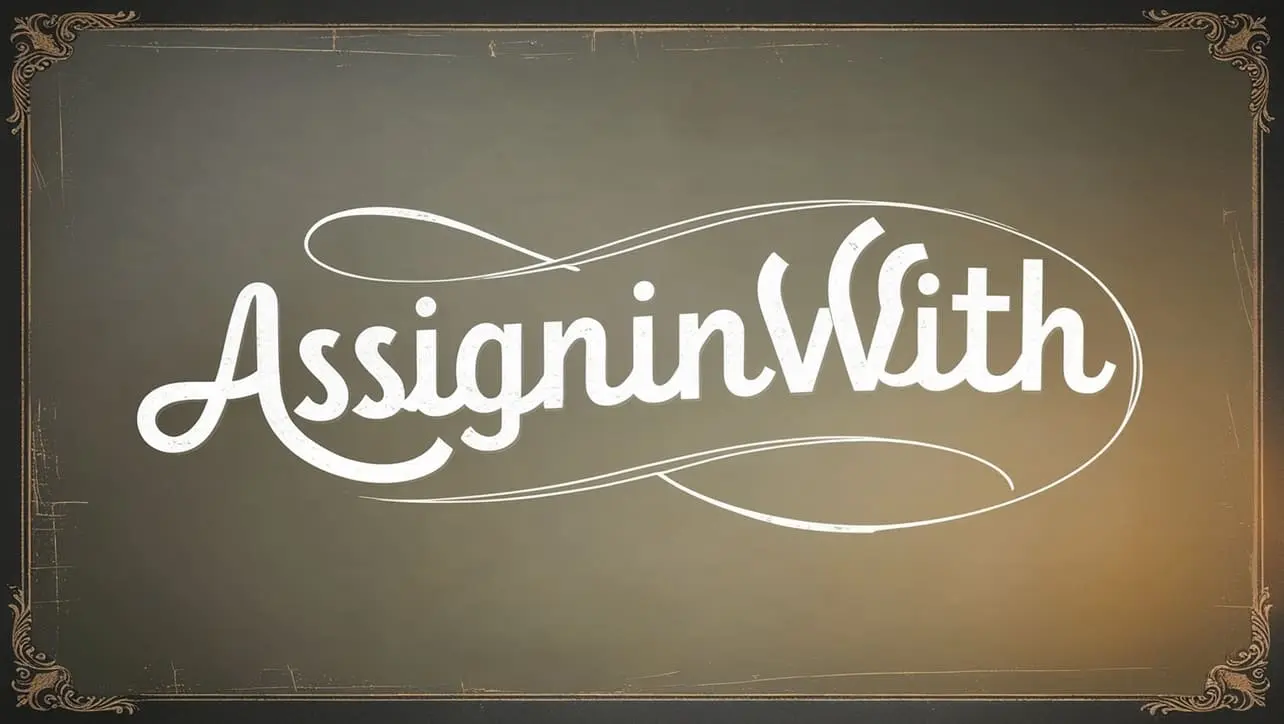
Photo Credit to CodeToFun
🙋 Introduction
Effective manipulation and merging of objects are essential tasks in JavaScript development. Lodash, a powerful utility library, provides a variety of functions to streamline these operations. Among them, the _.assignInWith()
method stands out as a versatile tool for merging multiple objects with customizable behavior.
This method enables developers to precisely control the merging process, making it a valuable asset in scenarios where fine-grained control over object assignment is crucial.
🧠 Understanding _.assignInWith() Method
The _.assignInWith()
method in Lodash is designed for merging multiple source objects into a target object. What sets it apart is the ability to customize the assignment logic using a customizer function. This allows developers to define how conflicts are resolved and how values are assigned during the merging process.
💡 Syntax
The syntax for the _.assignInWith()
method is straightforward:
_.assignInWith(object, ...sources, customizer)
- object: The target object.
- ...sources: The source objects to merge.
- customizer: The function to customize the assignment behavior.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.assignInWith()
method:
const _ = require('lodash');
const targetObject = {
a: 1,
b: 2
};
const source1 = {
b: 3,
c: 4
};
const source2 = {
d: 5
};
const customizer = (objValue, srcValue, key, object, source) => {
// Custom logic for assignment
return key === 'b' ? objValue * srcValue : undefined;
};
const mergedObject = _.assignInWith(targetObject, source1, source2, customizer);
console.log(mergedObject);
// Output: { a: 1, b: 6, c: 4, d: 5 }
In this example, the targetObject is merged with source1 and source2 using a customizer function that multiplies values when the key is 'b'.
🏆 Best Practices
When working with the _.assignInWith()
method, consider the following best practices:
Understand Customizer Function:
When using
_.assignInWith()
, thoroughly understand the customizer function as it determines how values are assigned during the merging process.example.jsCopiedconst targetObject = { a: 1, b: 2 }; const source = { b: 3, c: 4 }; const customizer = (objValue, srcValue, key, object, source) => { // Custom logic for assignment return key === 'b' ? objValue * srcValue : undefined; }; const mergedObject = _.assignInWith(targetObject, source, customizer); console.log(mergedObject); // Output: { a: 1, b: 6, c: 4 }
Handle Conflicts Carefully:
When merging objects with overlapping keys, be cautious about potential conflicts. The customizer function can help resolve conflicts based on your specific needs.
example.jsCopiedconst targetObject = { a: 1, b: 2, c: { d: 3 } }; const source = { b: 3, c: { e: 4 } }; const customizer = (objValue, srcValue, key) => { if(key === 'c') { // Resolve conflicts within nested objects return _.mergeWith({}, objValue, srcValue, customizer); } }; const mergedObject = _.assignInWith(targetObject, source, customizer); console.log(mergedObject); // Output: { a: 1, b: 3, c: { d: 3, e: 4 } }
Use _.mergeWith() for Deep Merging:
For deep merging of nested objects, consider using _.mergeWith() within the customizer function.
example.jsCopiedconst targetObject = { a: 1, b: { c: 2 } }; const source = { b: { c: 3, d: 4 } }; const customizer = (objValue, srcValue) => { if(_.isObject(objValue)) { // Deep merge nested objects return _.mergeWith({}, objValue, srcValue, customizer); } }; const mergedObject = _.assignInWith(targetObject, source, customizer); console.log(mergedObject); // Output: { a: 1, b: { c: 3, d: 4 } }
📚 Use Cases
Configurable Object Merging:
_.assignInWith()
is excellent for scenarios where you need fine-grained control over how objects are merged. This is particularly useful when dealing with configuration objects.example.jsCopiedconst defaultConfig = { timeout: 1000, retries: 3 }; const userConfig = { timeout: 500, delay: 200 }; const customizer = (objValue, srcValue, key) => { if(key === 'timeout') { // Use user-configured timeout if available return srcValue; } }; const mergedConfig = _.assignInWith(defaultConfig, userConfig, customizer); console.log(mergedConfig); // Output: { timeout: 500, retries: 3, delay: 200 }
Dynamic Object Composition:
When dynamically composing objects based on various sources,
_.assignInWith()
allows you to control the composition logic.example.jsCopiedconst baseObject = { a: 1, b: 2 }; const dynamicProps = { b: 3, c: 4 }; const computedProps = { d: 5 }; const customizer = (objValue, srcValue, key) => { // Combine dynamic and computed properties return key === 'b' ? objValue + srcValue : undefined; }; const composedObject = _.assignInWith(baseObject, dynamicProps, computedProps, customizer); console.log(composedObject); // Output: { a: 1, b: 5, c: 4, d: 5 }
Merging Objects with Special Rules:
In scenarios where standard merging is insufficient and special rules apply,
_.assignInWith()
empowers you to define custom logic.example.jsCopiedconst baseObject = { a: 1, b: 2 }; const source = { b: 3, c: 4 }; const customizer = (objValue, srcValue, key) => { // Special rule: If the key is 'b', concatenate values as strings return key === 'b' ? `${objValue}${srcValue}` : undefined; }; const mergedObject = _.assignInWith(baseObject, source, customizer); console.log(mergedObject); // Output: { a: 1, b: '23', c: 4 }
🎉 Conclusion
The _.assignInWith()
method in Lodash provides a powerful and flexible solution for merging objects in JavaScript. With its customizable assignment logic, it caters to a wide range of scenarios where precise control over object composition is essential.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.assignInWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
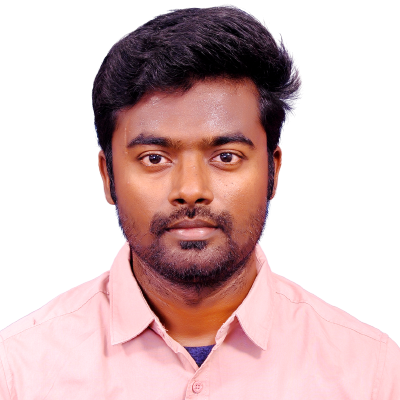
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
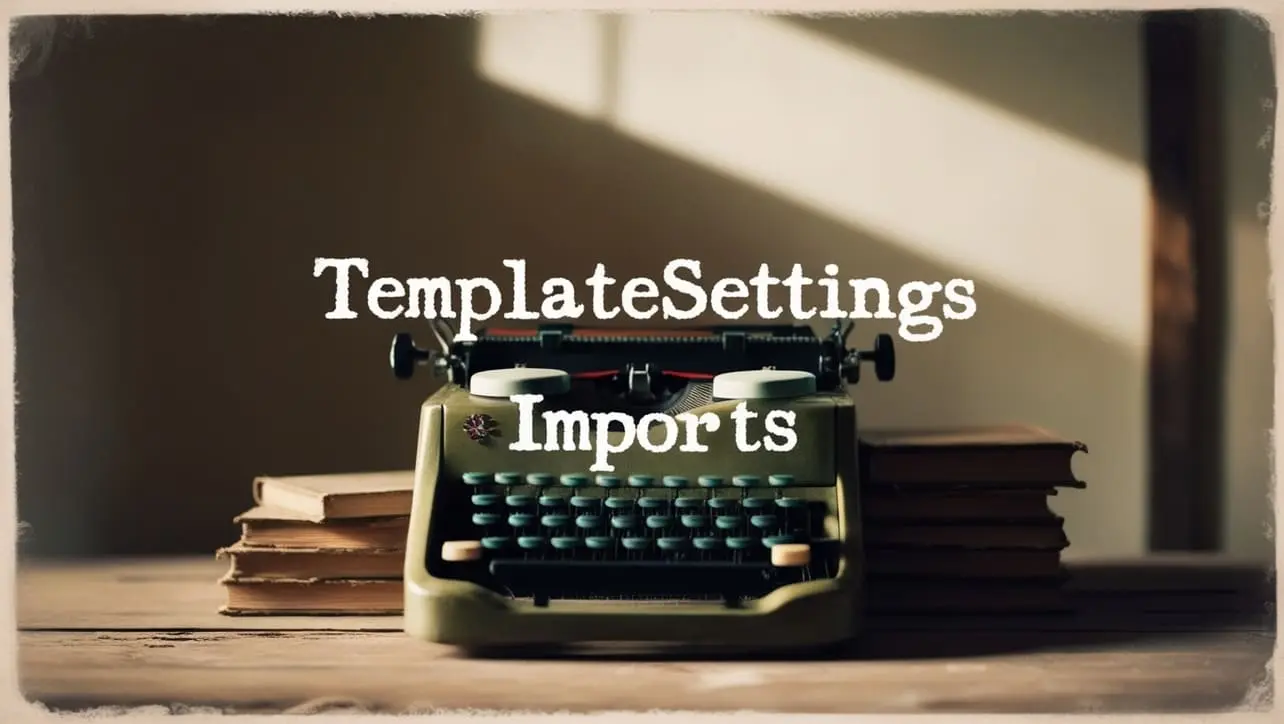
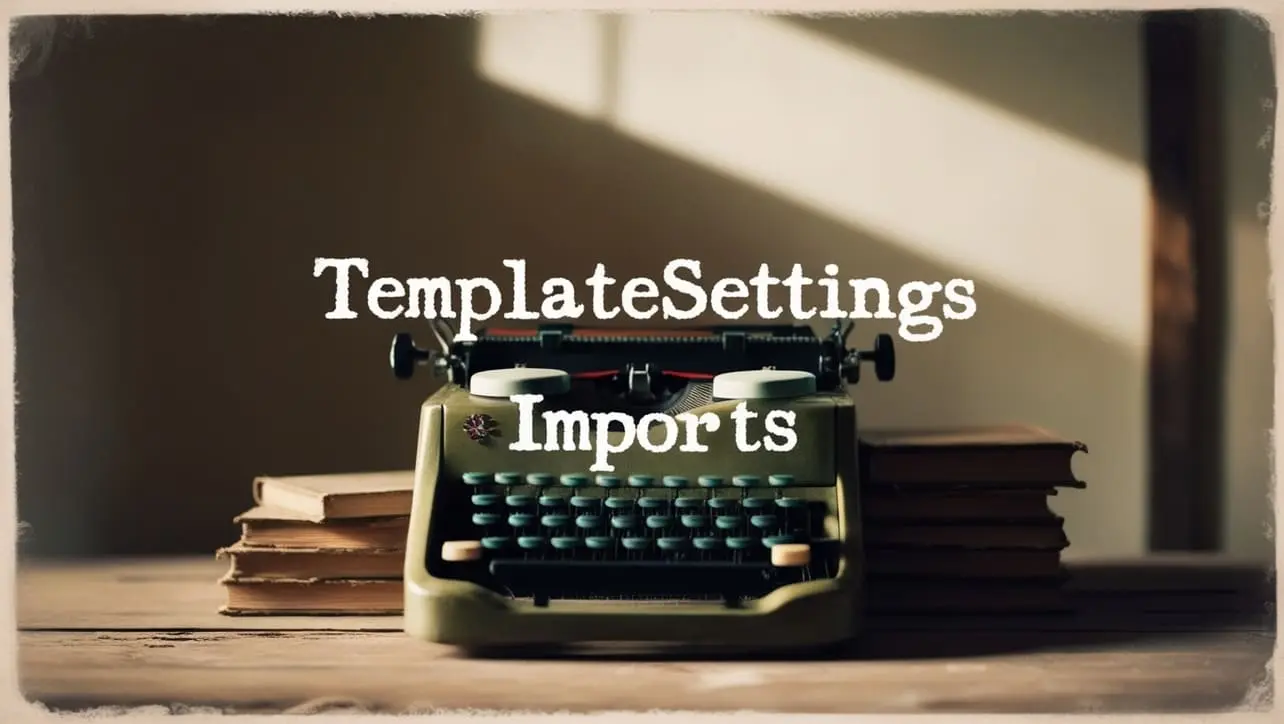
Lodash _.templateSettings.imports Property
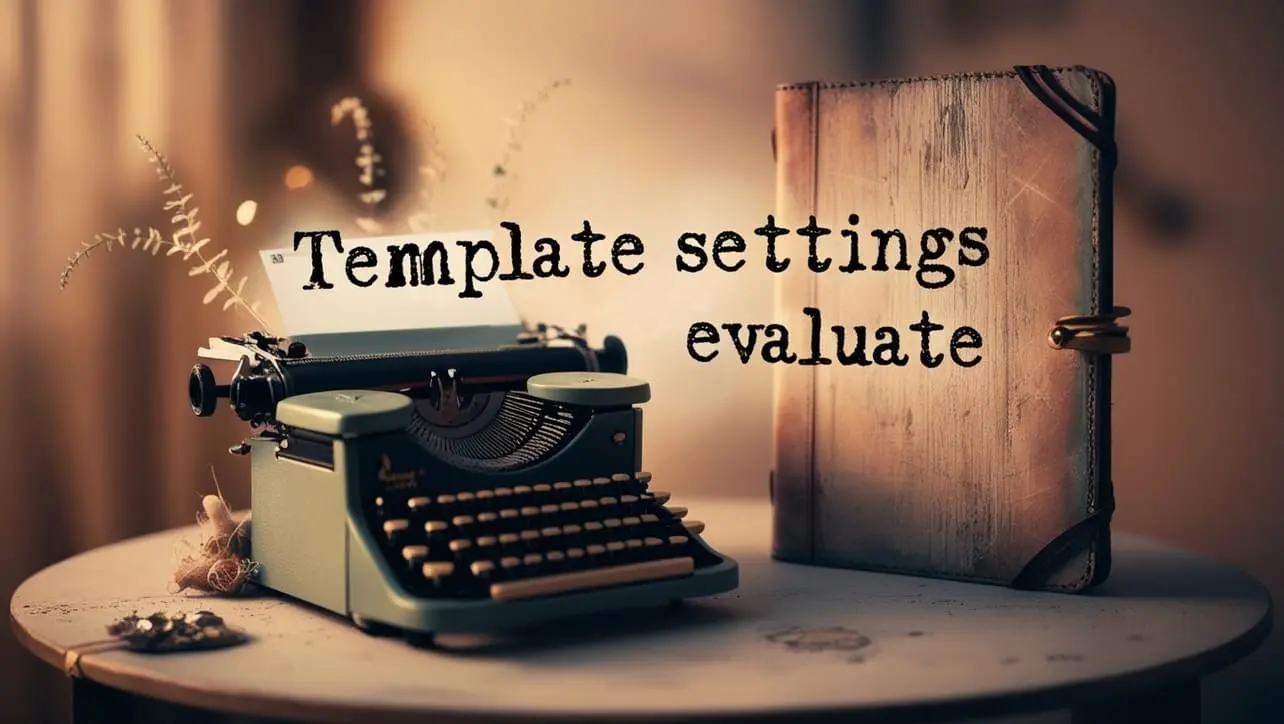
Lodash _.templateSettings.evaluate Property
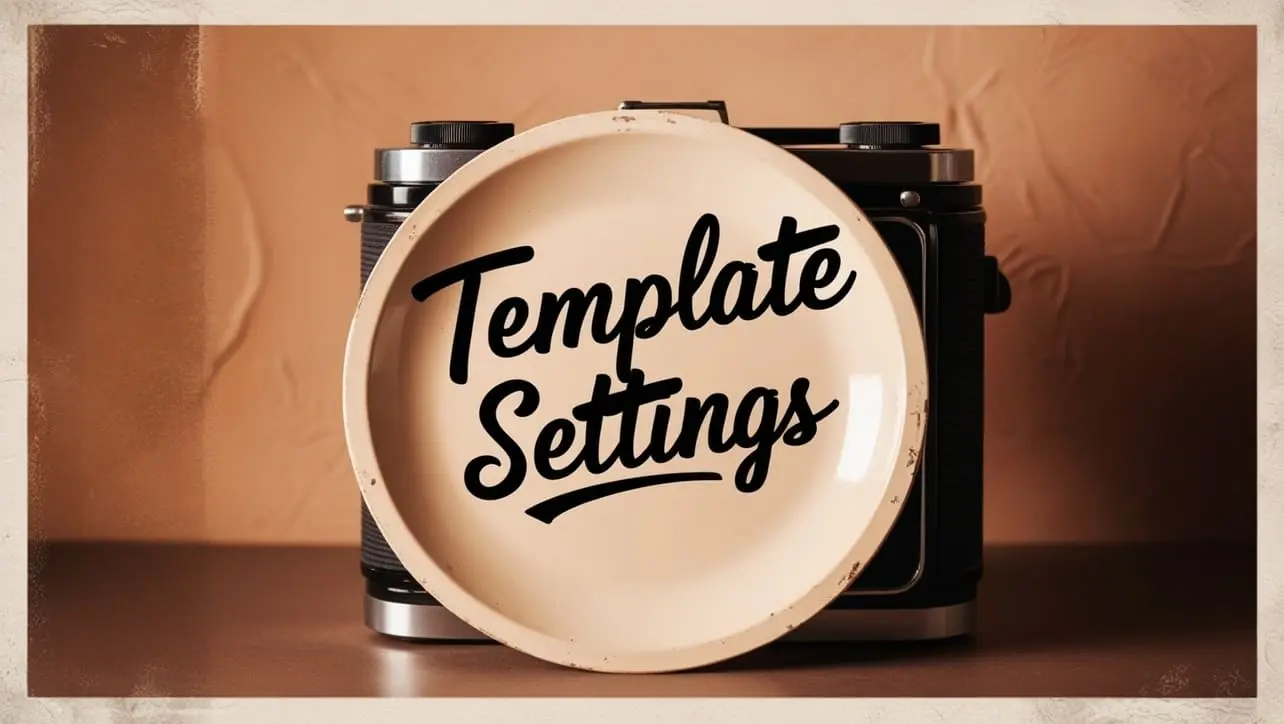
Lodash _.templateSettings Property
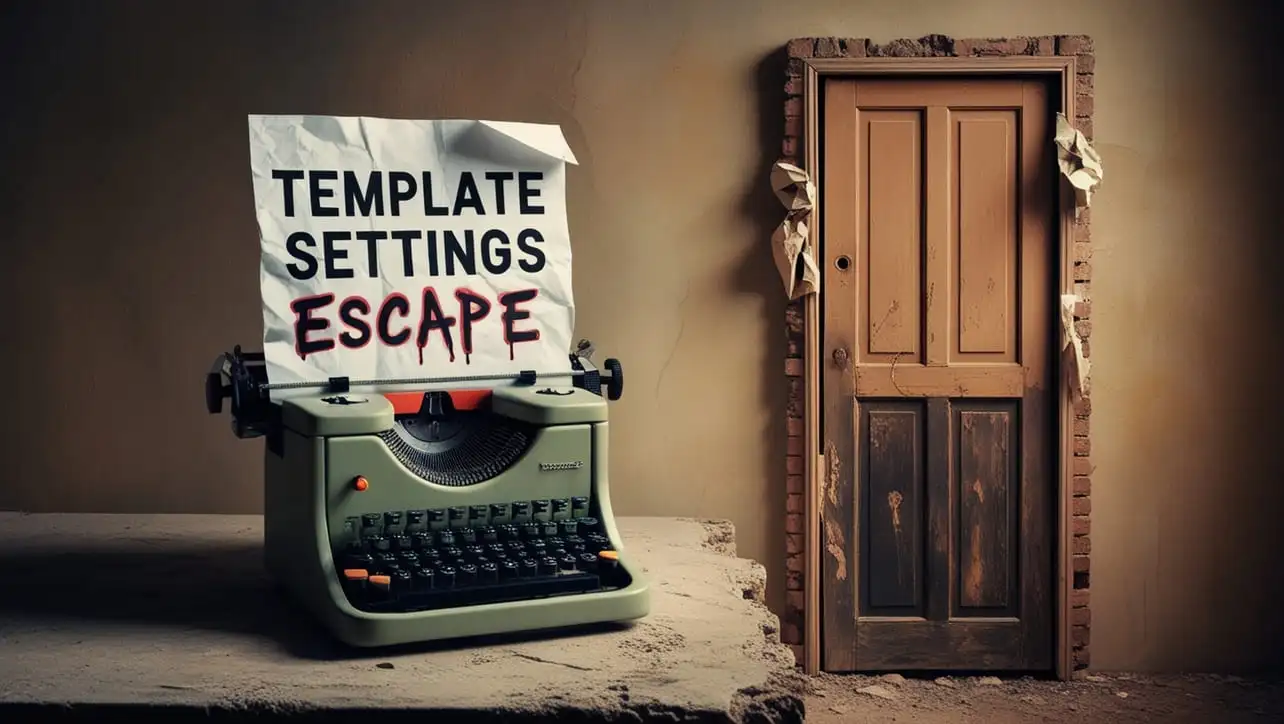
Lodash _.templateSettings.escape Property
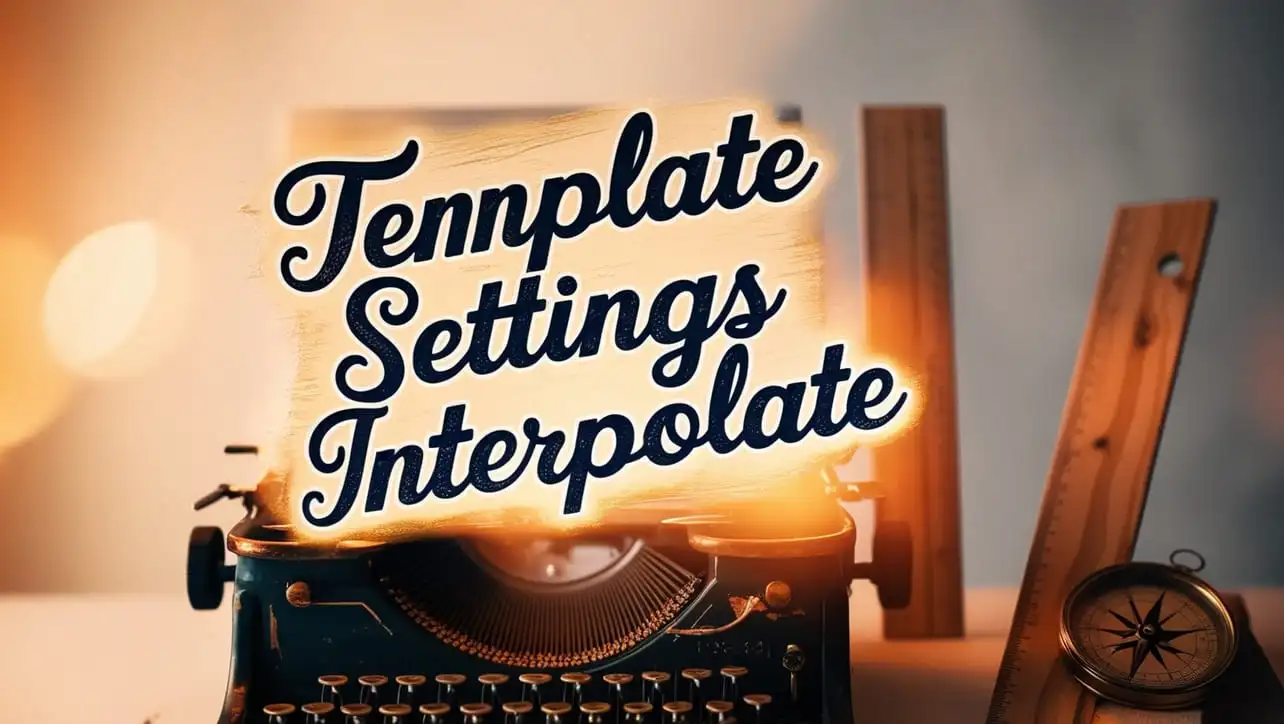
Lodash _.templateSettings.interpolate Property
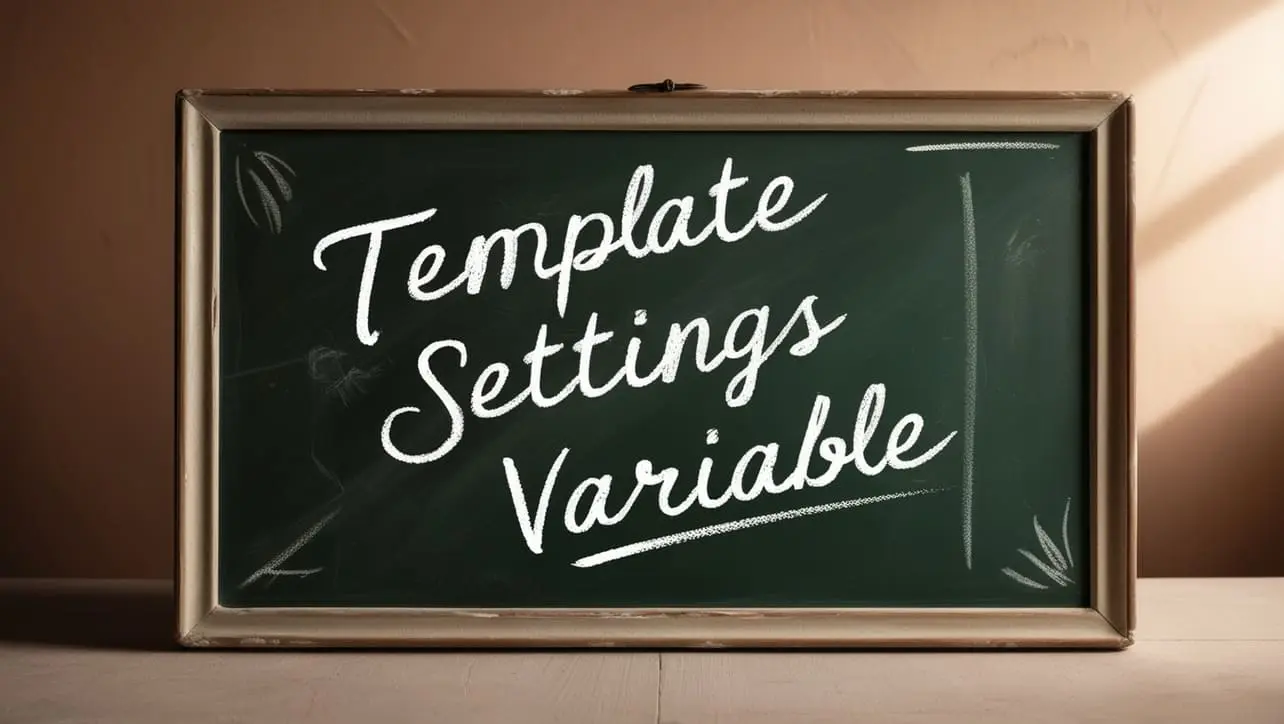
If you have any doubts regarding this article (Lodash _.assignInWith() Object Method), please comment here. I will help you immediately.