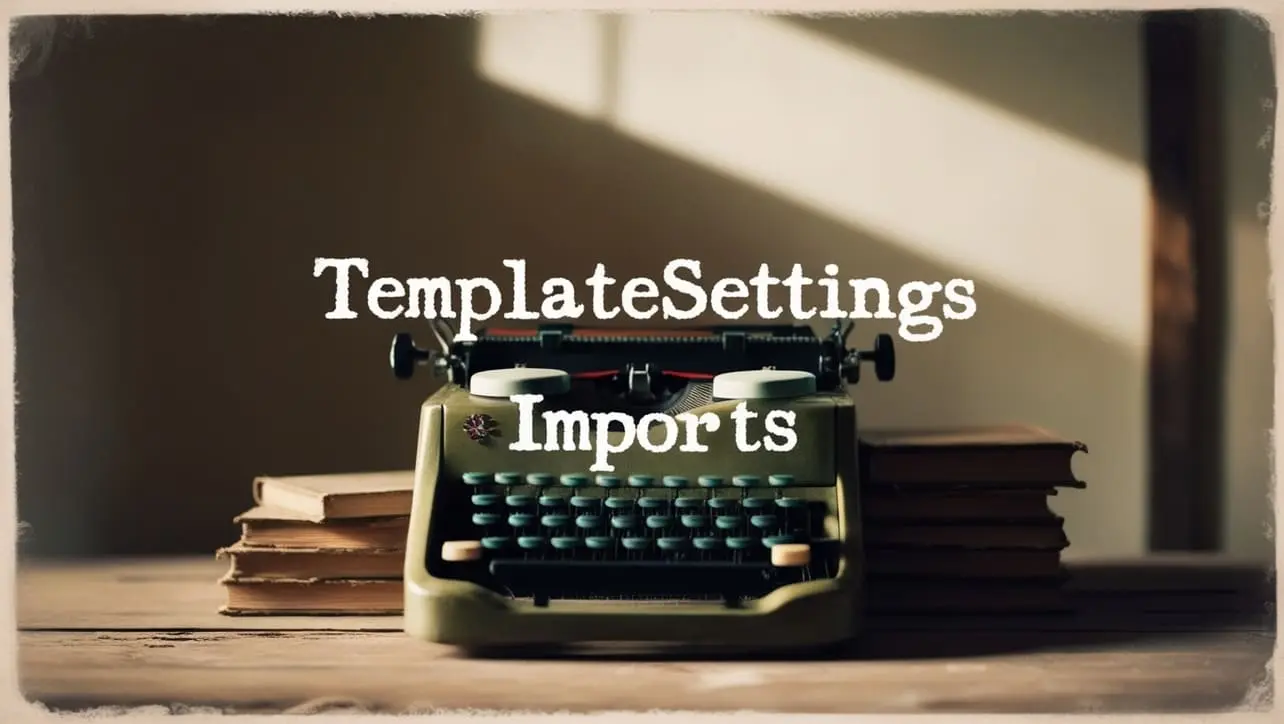
Lodash _.clamp() Number Method
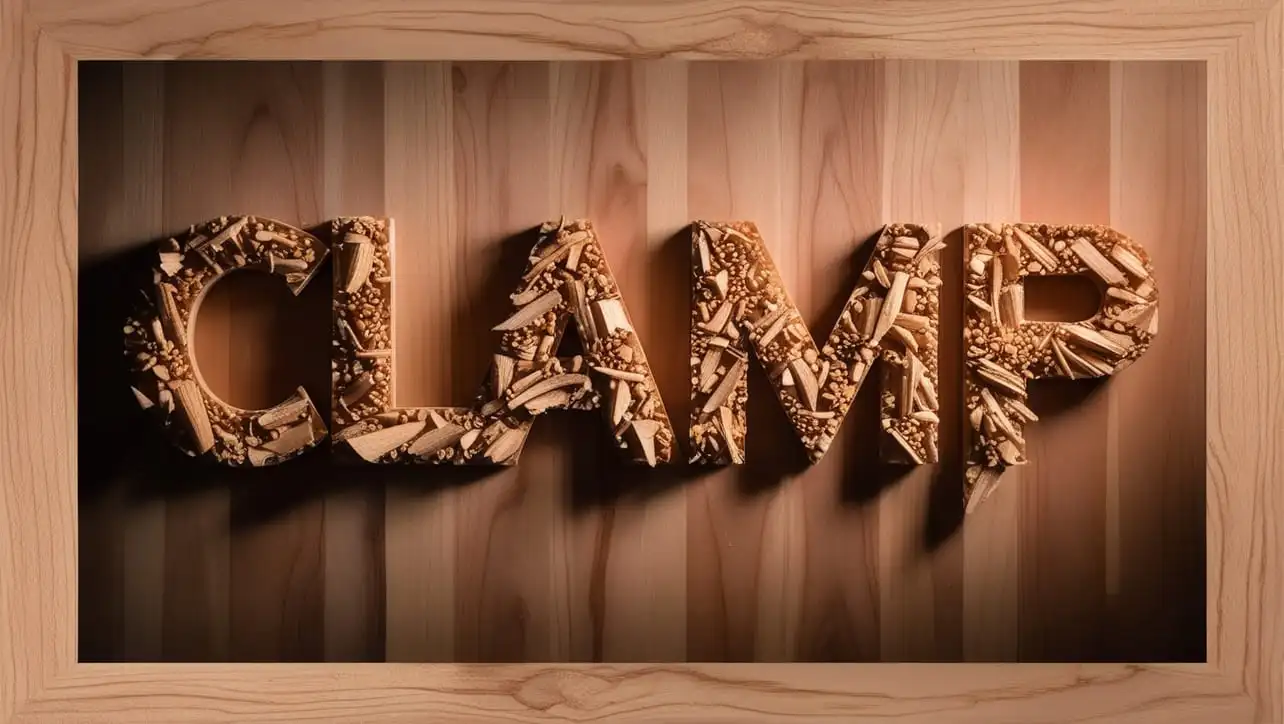
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, numeric range constraints are a common requirement. Lodash, a powerful utility library, provides the _.clamp()
method to simplify the process of constraining a number within a specified range.
This method is invaluable for developers seeking a clean and efficient solution to limit numeric values, ensuring they fall within a defined interval.
🧠 Understanding _.clamp() Method
The _.clamp()
method in Lodash allows you to restrict a numeric value to be within a specified range. This is achieved by setting upper and lower bounds for the given number, providing a convenient way to handle constraints without complex conditional statements.
💡 Syntax
The syntax for the _.clamp()
method is straightforward:
_.clamp(number, lower, upper)
- number: The number to be clamped.
- lower: The lower bound of the range.
- upper: The upper bound of the range.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.clamp()
method:
const _ = require('lodash');
const originalNumber = 15;
const clampedNumber = _.clamp(originalNumber, 5, 10);
console.log(clampedNumber);
// Output: 10
In this example, the originalNumber is clamped between 5 and 10 using _.clamp()
, ensuring that the resulting value does not exceed the specified range.
🏆 Best Practices
When working with the _.clamp()
method, consider the following best practices:
Clear Bound Definitions:
Define clear and meaningful lower and upper bounds for the range. This ensures that the purpose of clamping is evident and prevents unintended constraints.
example.jsCopiedconst temperature = 32; const clampedTemperature = _.clamp(temperature, 0, 100); console.log(clampedTemperature); // Output: 32
Handle Out-of-Range Values:
Consider the behavior of
_.clamp()
when the original number is outside the specified range. It automatically adjusts the value to meet the nearest bound.example.jsCopiedconst outOfRangeValue = -5; const clampedValue = _.clamp(outOfRangeValue, 0, 10); console.log(clampedValue); // Output: 0
Use Cases with Variables:
Integrate variables into the clamping process to dynamically adjust numeric values based on changing conditions or user input.
example.jsCopiedconst dynamicValue = /* ...obtain a value dynamically... */; const lowerBound = 10; const upperBound = 50; const clampedDynamicValue = _.clamp(dynamicValue, lowerBound, upperBound); console.log(clampedDynamicValue);
📚 Use Cases
Input Validation:
When accepting numeric input from users or external sources,
_.clamp()
can be employed to validate and ensure the input falls within acceptable limits.example.jsCopiedconst userInput = /* ...obtain numeric input from user... */; const validatedInput = _.clamp(userInput, 0, 100); console.log(validatedInput);
Animation and Transitions:
In scenarios involving animations or transitions, use
_.clamp()
to keep values within predefined ranges, preventing erratic behavior or unexpected visual elements.example.jsCopiedconst animationProgress = /* ...calculate animation progress... */; const clampedProgress = _.clamp(animationProgress, 0, 1); console.log(clampedProgress);
Gaming and Simulation:
For game development or simulations,
_.clamp()
is useful in scenarios where numeric values, such as player scores or simulation parameters, need to be constrained within specific limits.example.jsCopiedconst playerScore = /* ...calculate player score... */; const clampedScore = _.clamp(playerScore, 0, 100000); console.log(clampedScore);
🎉 Conclusion
The _.clamp()
method in Lodash provides an elegant solution for constraining numeric values within specified ranges. Whether you're validating user input, managing animation progress, or controlling game parameters, _.clamp()
offers a versatile tool for ensuring numeric values stay within desired limits.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.clamp()
method in your Lodash projects.
👨💻 Join our Community:
Author
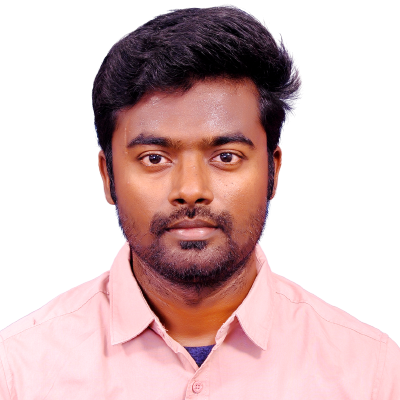
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
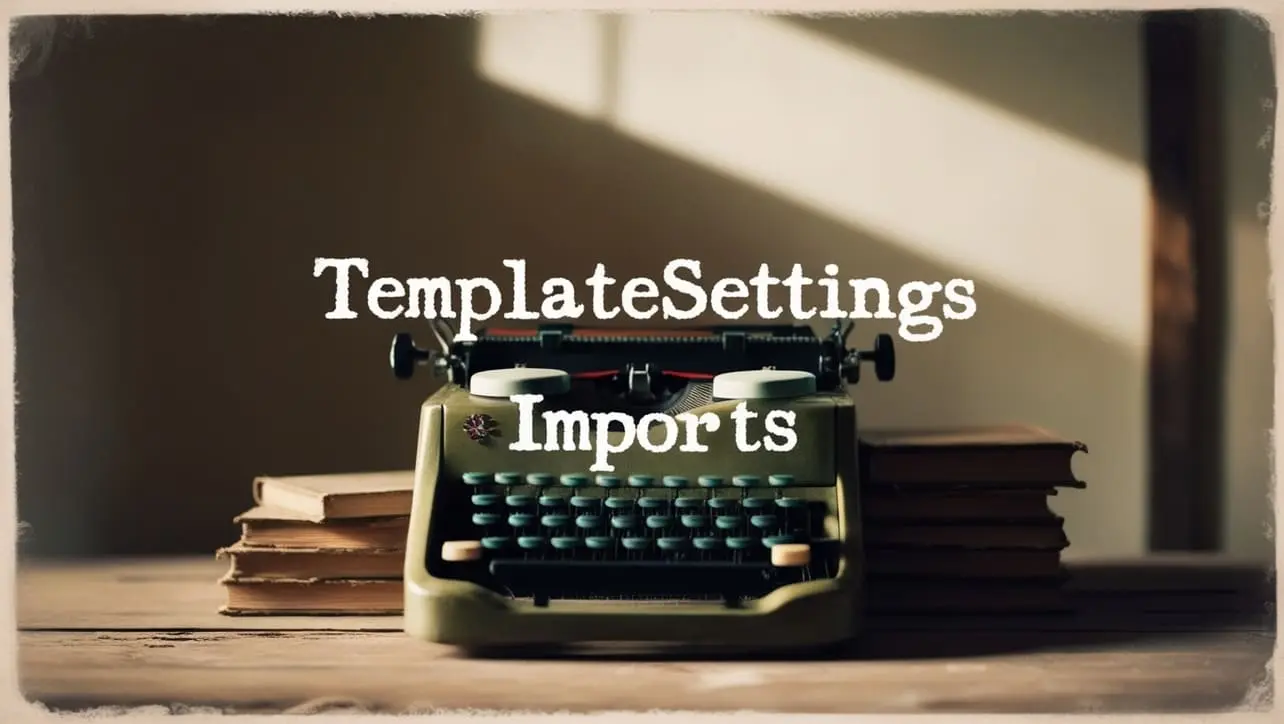
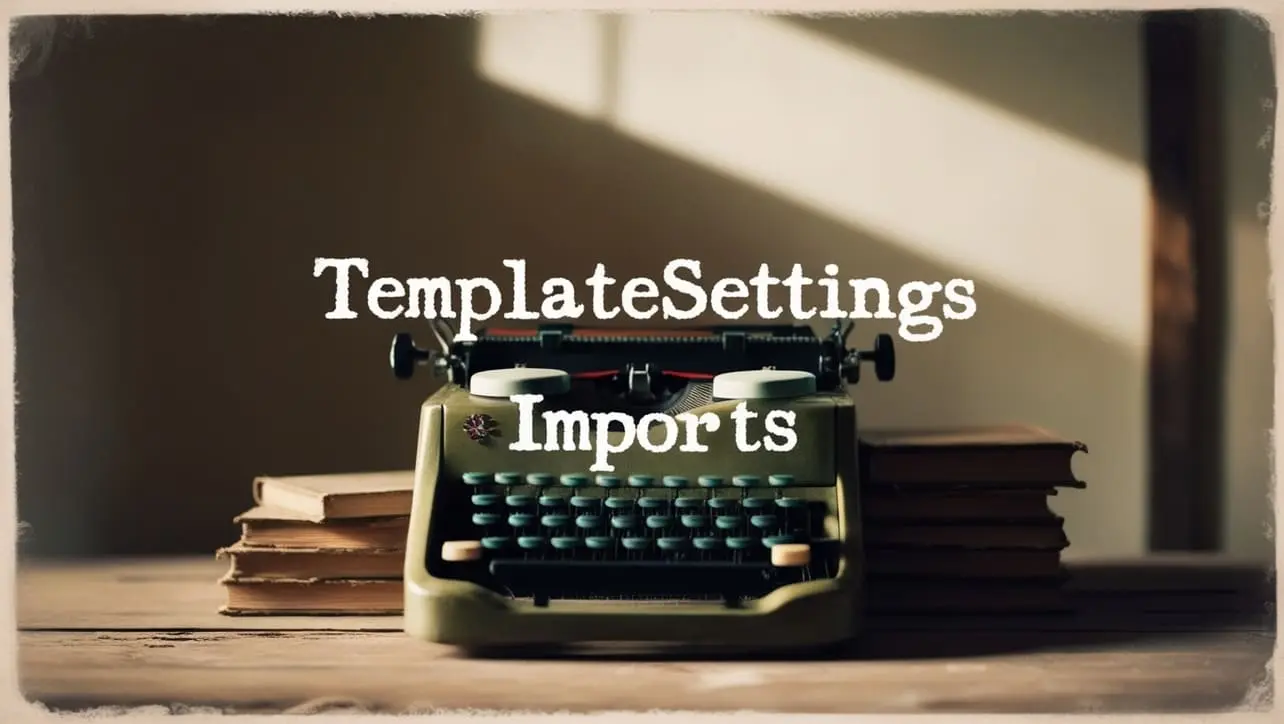
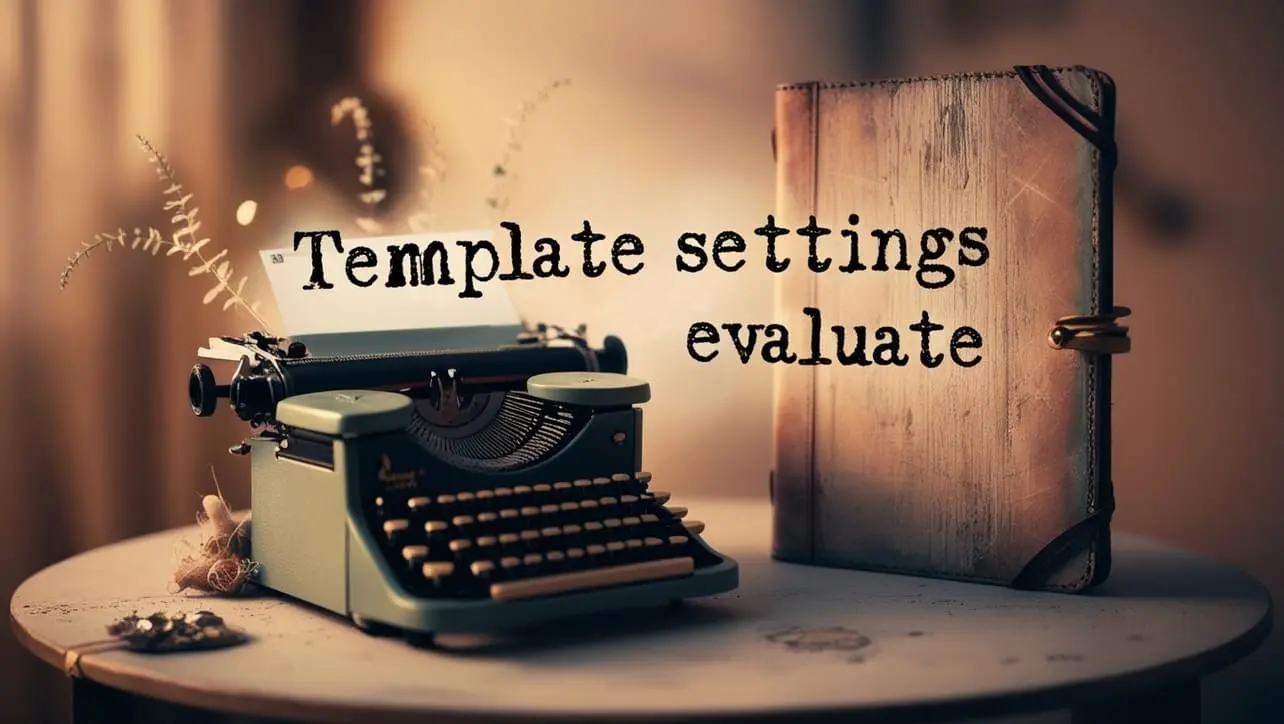
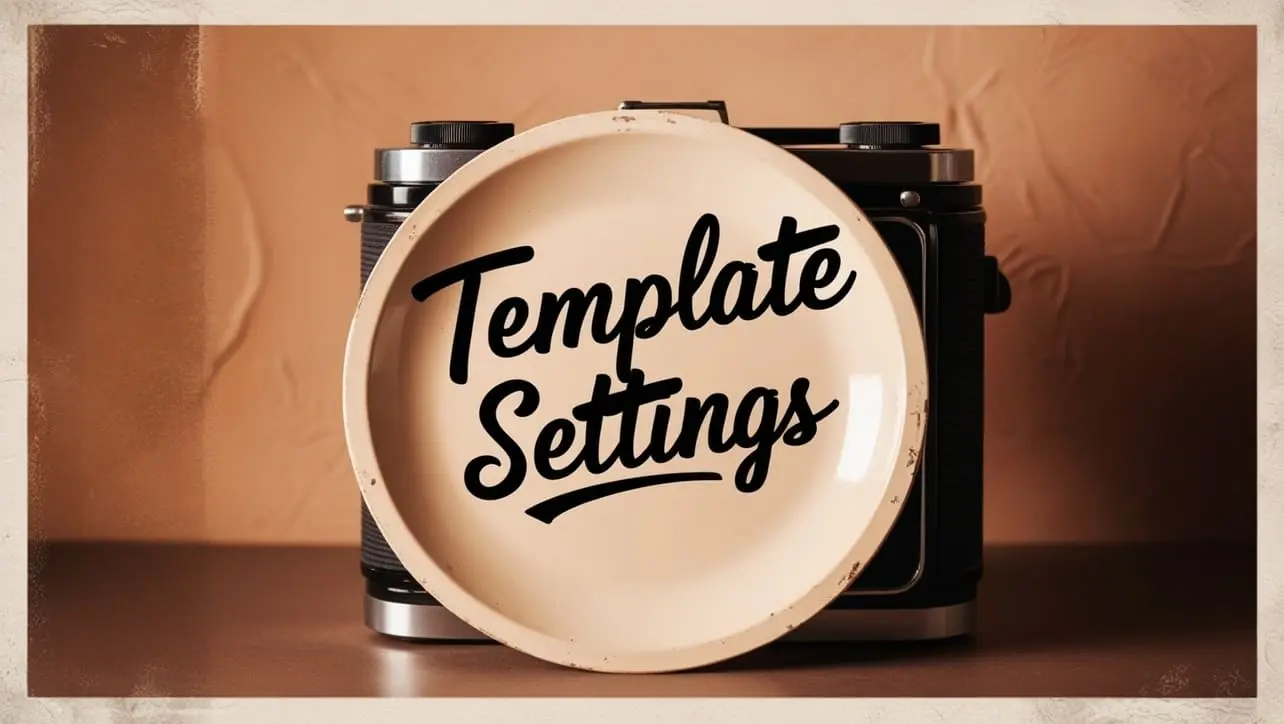
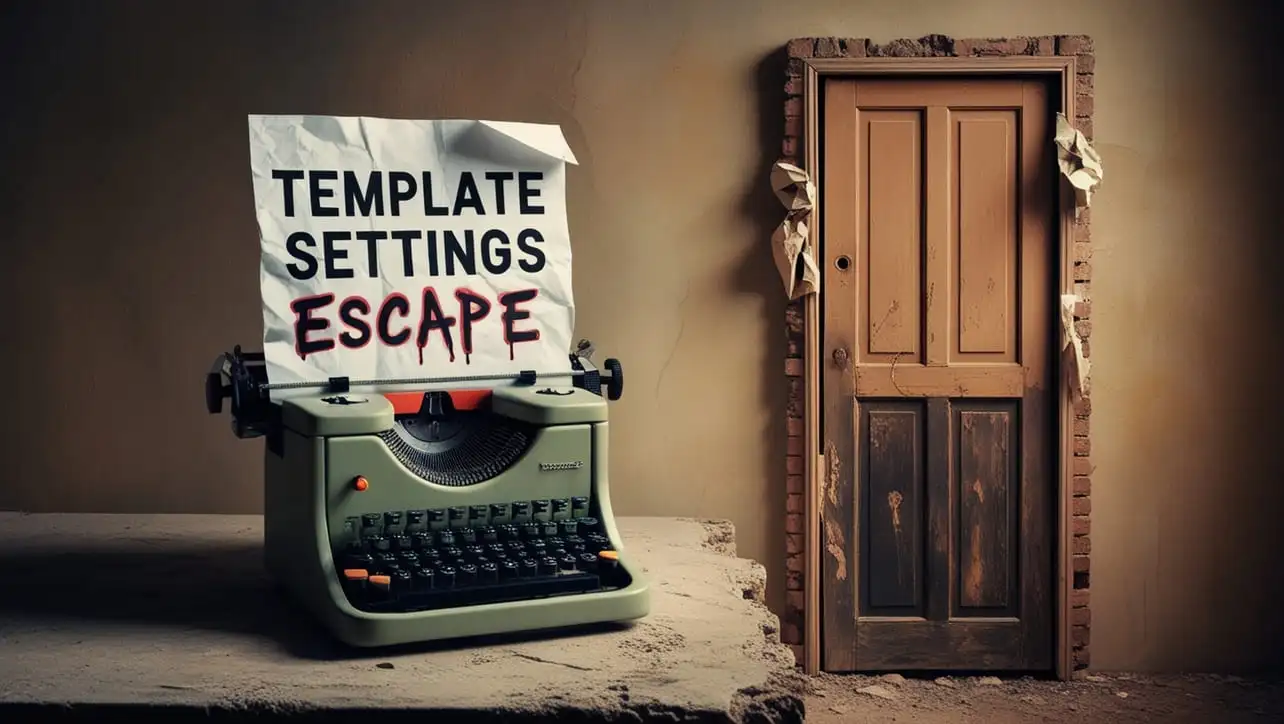
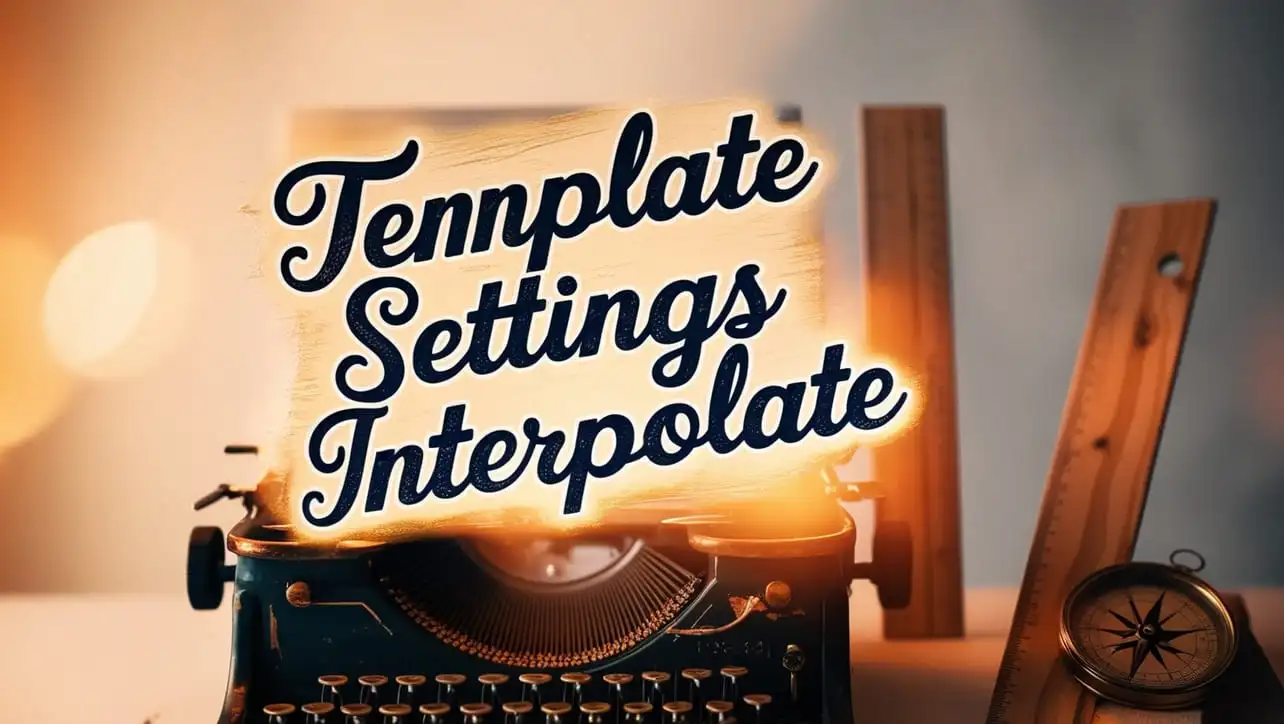
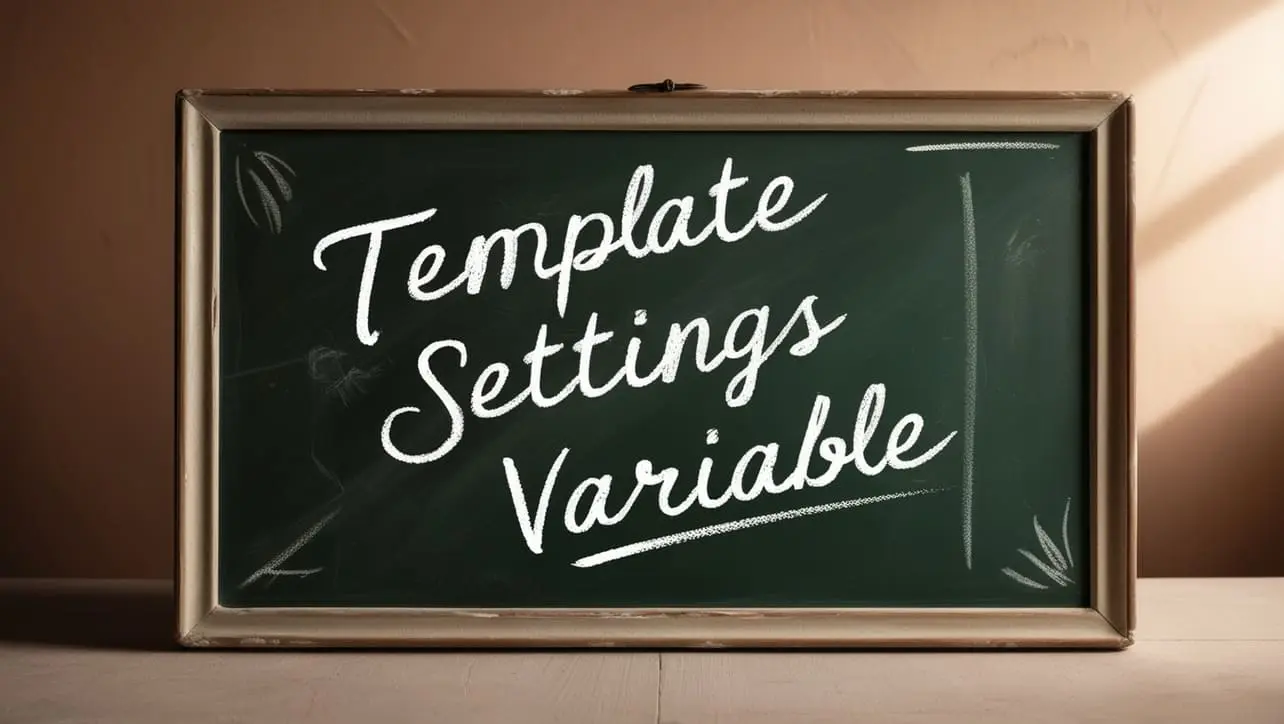
If you have any doubts regarding this article (Lodash _.clamp() Number Method), please comment here. I will help you immediately.