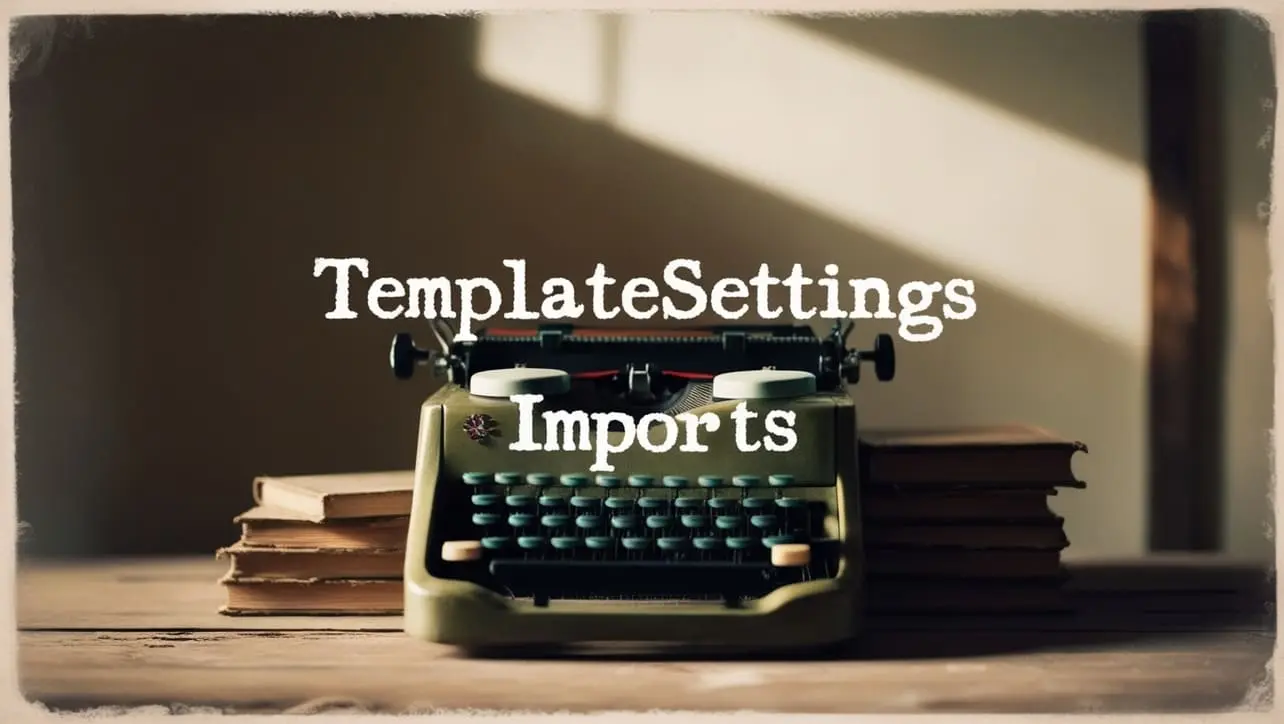
Lodash _.multiply() Math Method
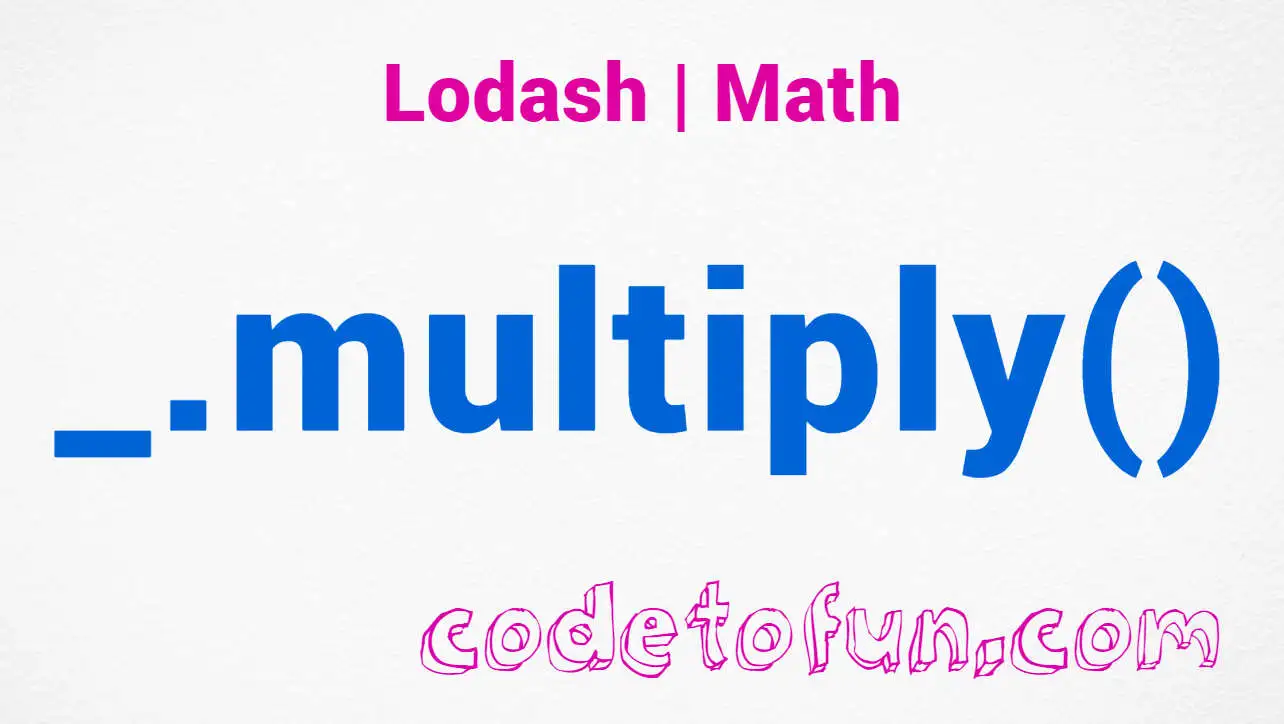
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, mathematical operations are fundamental, and the Lodash library provides a versatile set of tools to streamline these operations. Among these tools is the _.multiply()
method, a straightforward yet powerful function for multiplying numbers.
This method simplifies numeric calculations and enhances code readability, making it an invaluable asset for developers working with mathematical computations.
🧠 Understanding _.multiply() Method
The _.multiply()
method in Lodash is designed to multiply two numbers accurately. It accepts two parameters, multiplies them together, and returns the result. While the operation itself may seem basic, the utility of this method shines in scenarios where precision and consistency are paramount.
💡 Syntax
The syntax for the _.multiply()
method is straightforward:
_.multiply(multiplier, multiplicand)
- multiplier: The first number to be multiplied.
- multiplicand: The second number to be multiplied.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.multiply()
method:
const _ = require('lodash');
const result = _.multiply(5, 3);
console.log(result);
// Output: 15
In this example, _.multiply(5, 3) returns the product of 5 and 3, which is 15.
🏆 Best Practices
When working with the _.multiply()
method, consider the following best practices:
Input Validation:
Before using
_.multiply()
, ensure that the provided values are valid numbers to avoid unexpected results.example.jsCopiedconst num1 = 8; const num2 = 'invalid'; if (typeof num1 === 'number' && typeof num2 === 'number') { const result = _.multiply(num1, num2); console.log(result); } else { console.error('Invalid input. Both values must be numbers.'); }
Handling Decimals:
Consider the precision of decimal numbers when using
_.multiply()
. Due to the nature of floating-point arithmetic, be aware of potential rounding errors.example.jsCopiedconst decimalResult = _.multiply(0.1, 0.2); console.log(decimalResult); // Output: 0.020000000000000004
Scientific Notation:
Be cautious when dealing with very large or very small numbers, as the result may be displayed in scientific notation.
example.jsCopiedconst scientificResult = _.multiply(1e22, 1e22); console.log(scientificResult); // Output: 1e+44
📚 Use Cases
Basic Multiplication:
_.multiply()
excels in simple multiplication operations, offering a clean and readable alternative to the native JavaScript * operator.example.jsCopiedconst product = _.multiply(7, 9); console.log(product); // Output: 63
Calculations within Loops:
When performing multiplication within loops, using
_.multiply()
can enhance code readability.example.jsCopiedconst factors = [2, 3, 4]; let totalProduct = 1; factors.forEach(factor => { totalProduct = _.multiply(totalProduct, factor); }); console.log(totalProduct); // Output: 24
Precision-Dependent Operations:
For operations where precision is crucial, such as financial calculations,
_.multiply()
ensures accurate results.example.jsCopiedconst price = 19.99; const quantity = 3; const totalCost = _.multiply(price, quantity); console.log(totalCost); // Output: 59.97
🎉 Conclusion
The _.multiply()
method in Lodash provides a reliable and readable way to perform multiplication operations in JavaScript. Whether you're working on basic calculations or precision-dependent operations, this method offers simplicity and consistency.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.multiply()
method in your Lodash projects.
👨💻 Join our Community:
Author
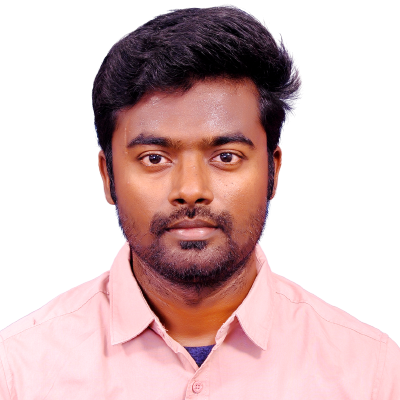
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
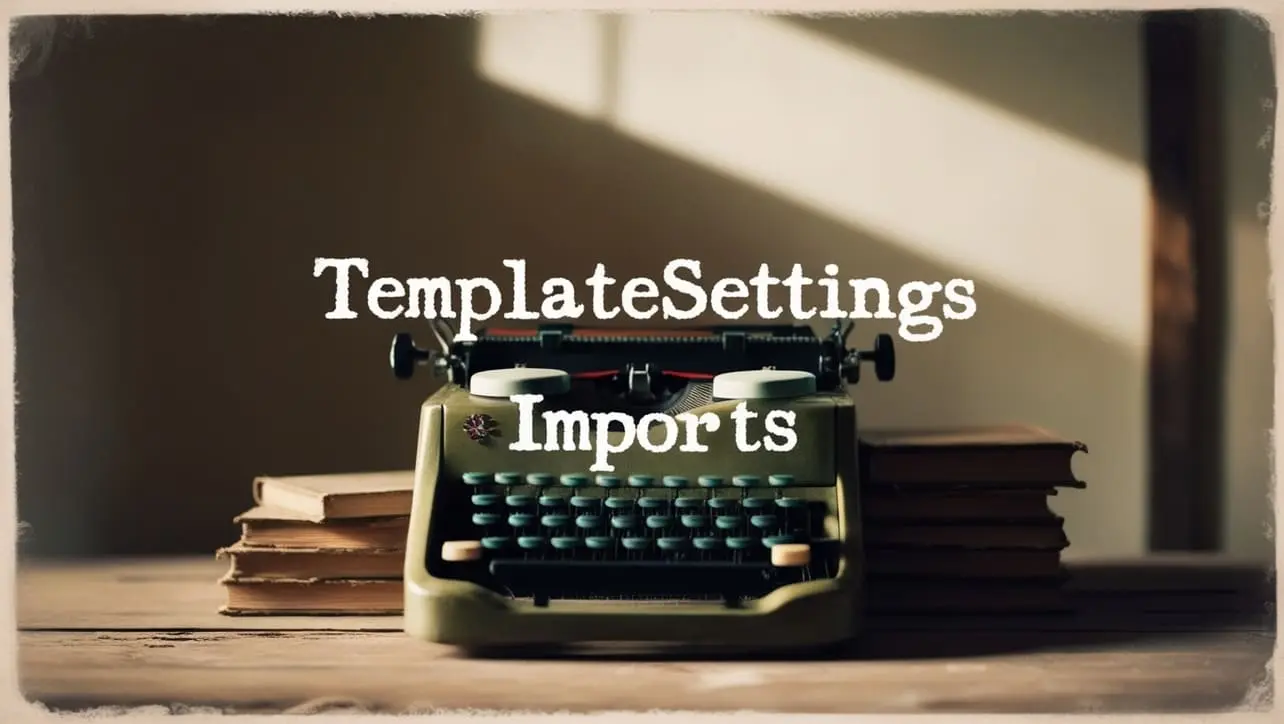
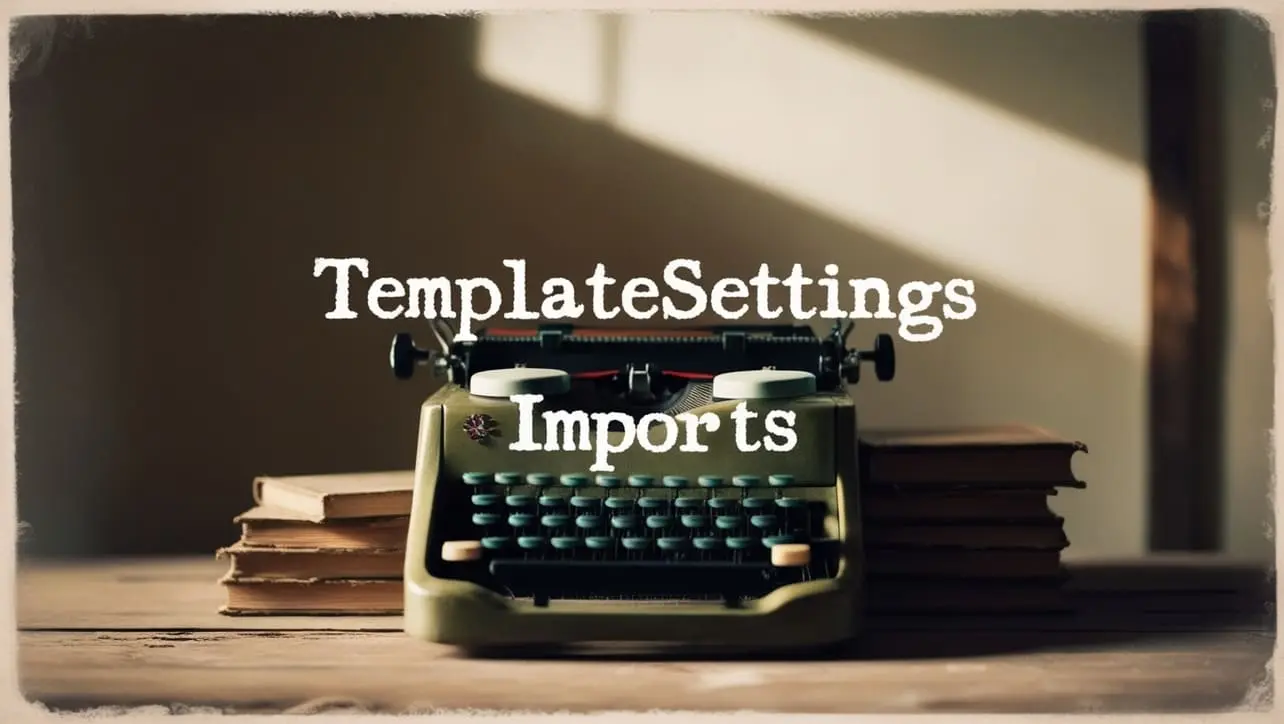
Lodash _.templateSettings.imports Property
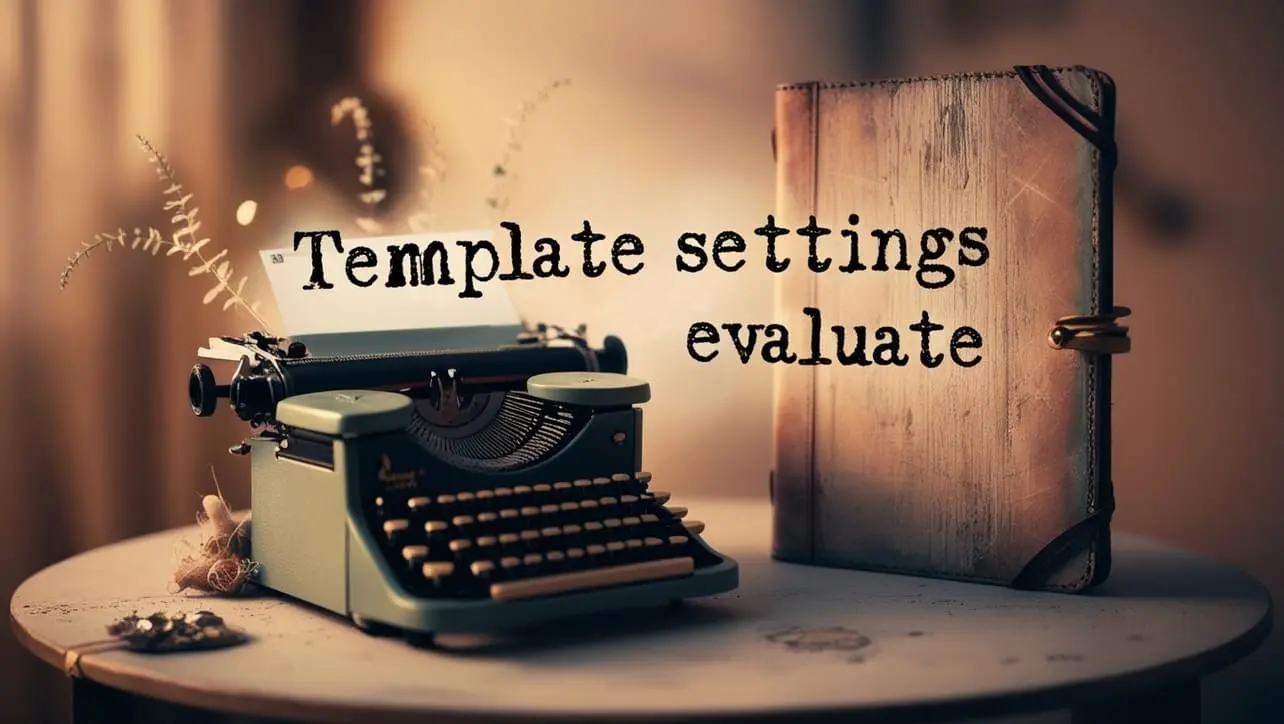
Lodash _.templateSettings.evaluate Property
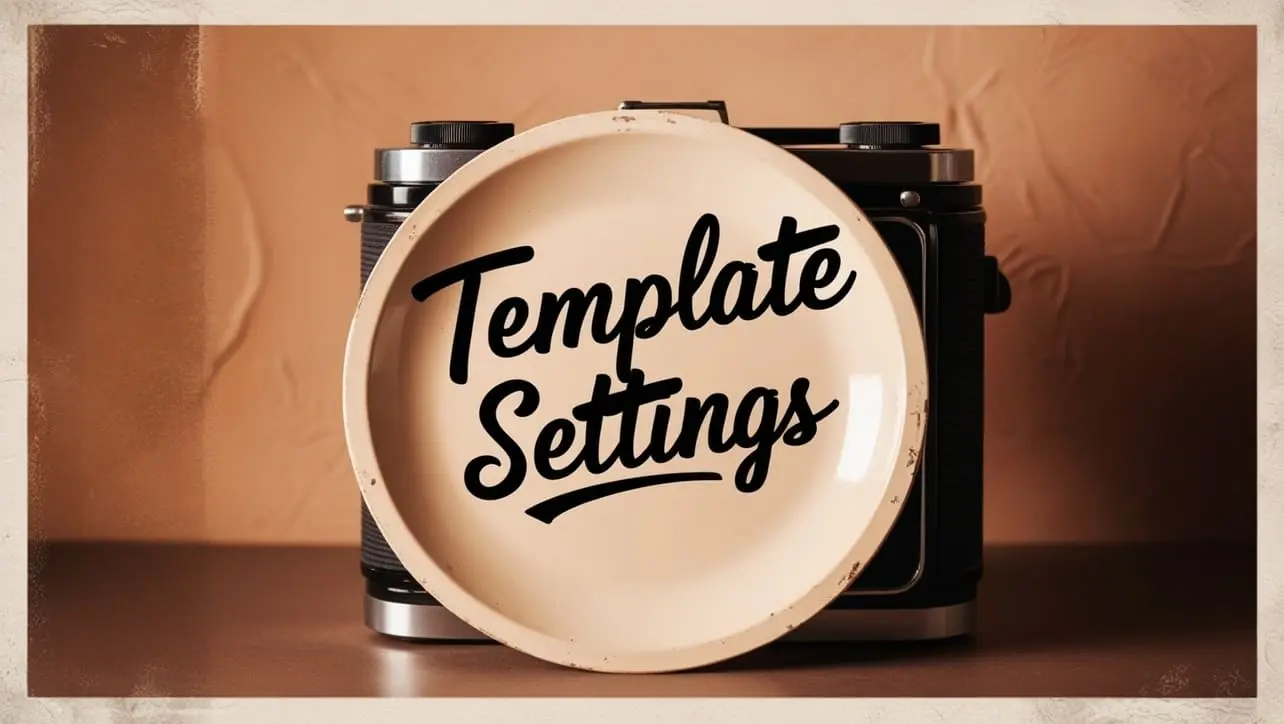
Lodash _.templateSettings Property
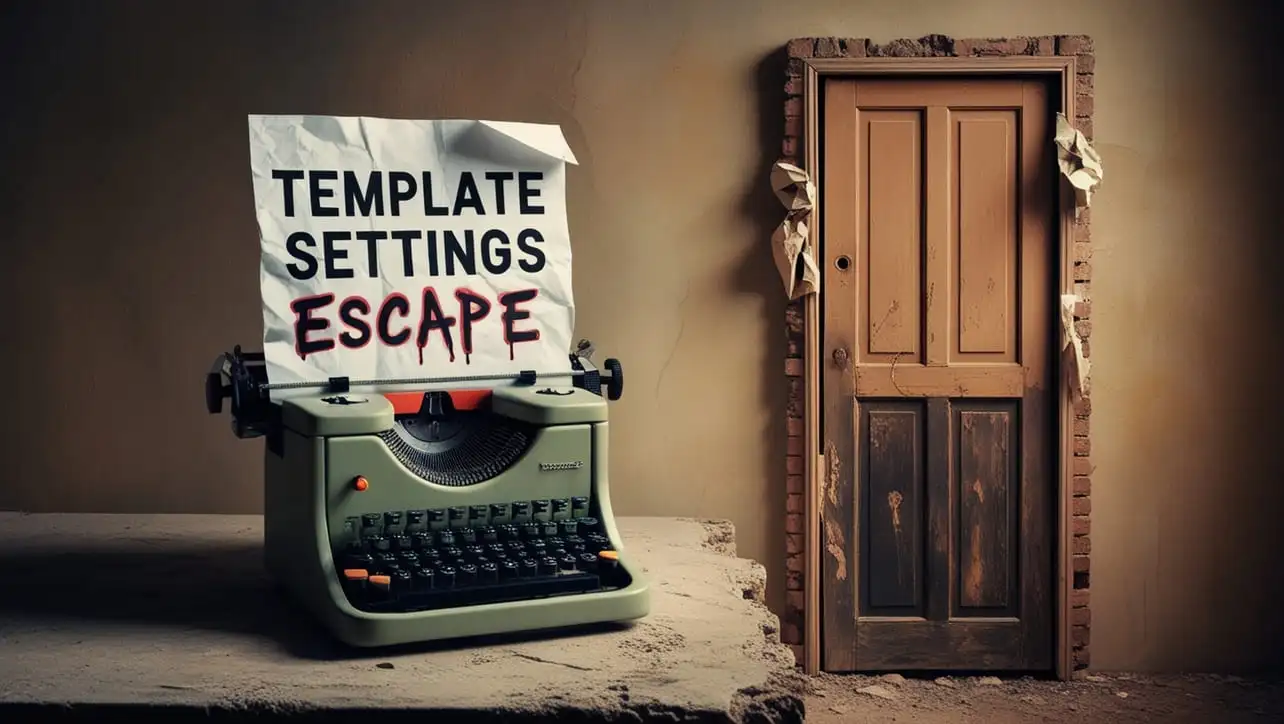
Lodash _.templateSettings.escape Property
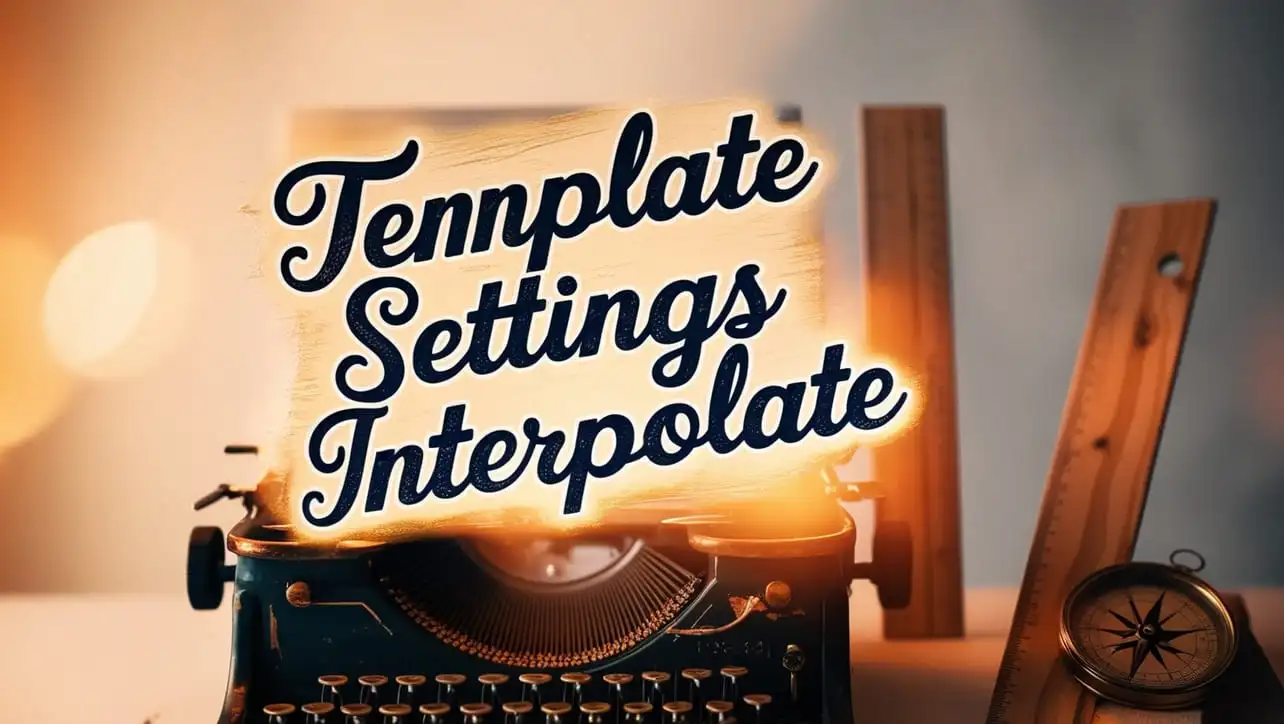
Lodash _.templateSettings.interpolate Property
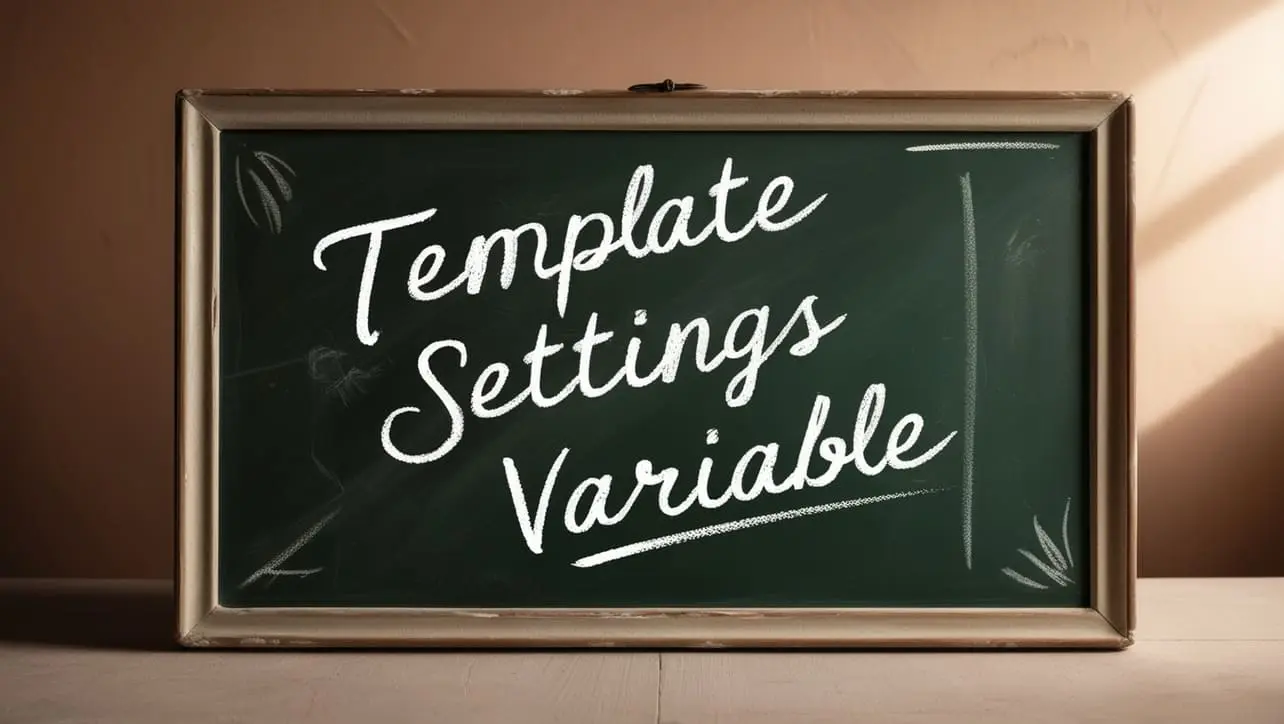
If you have any doubts regarding this article (Lodash _.multiply() Math Method), please comment here. I will help you immediately.