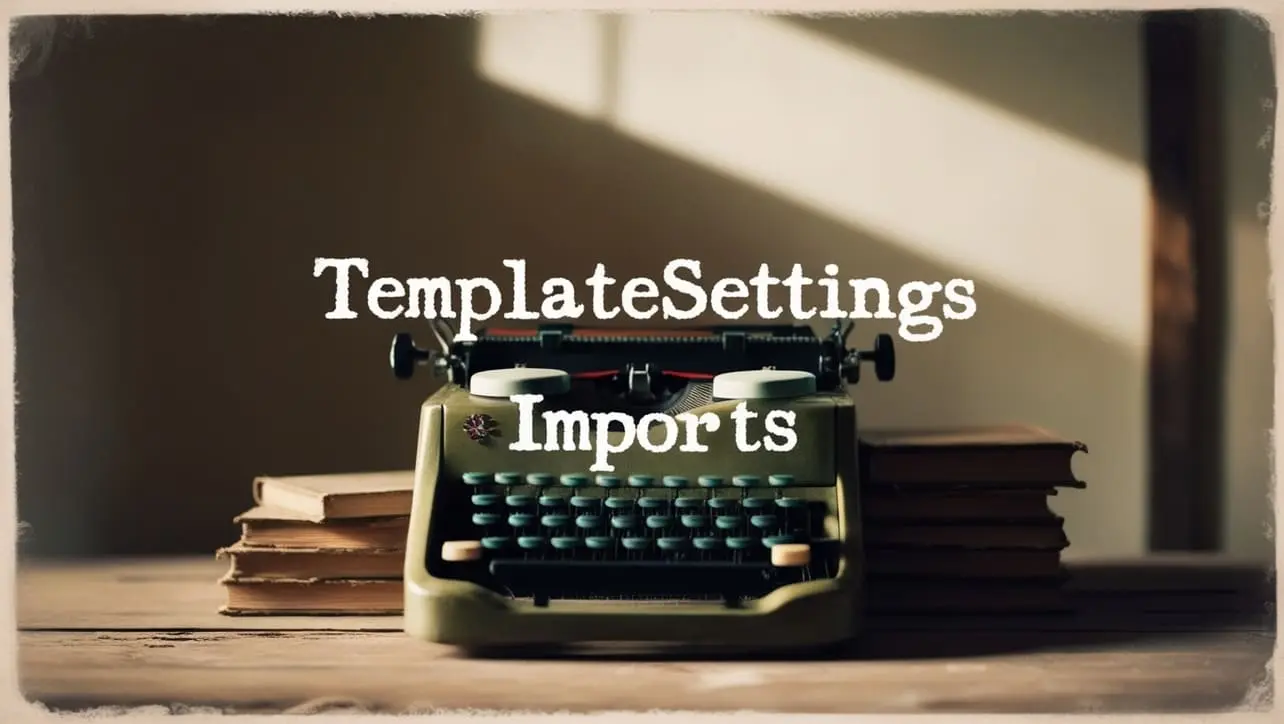
Lodash _.minBy() Math Method
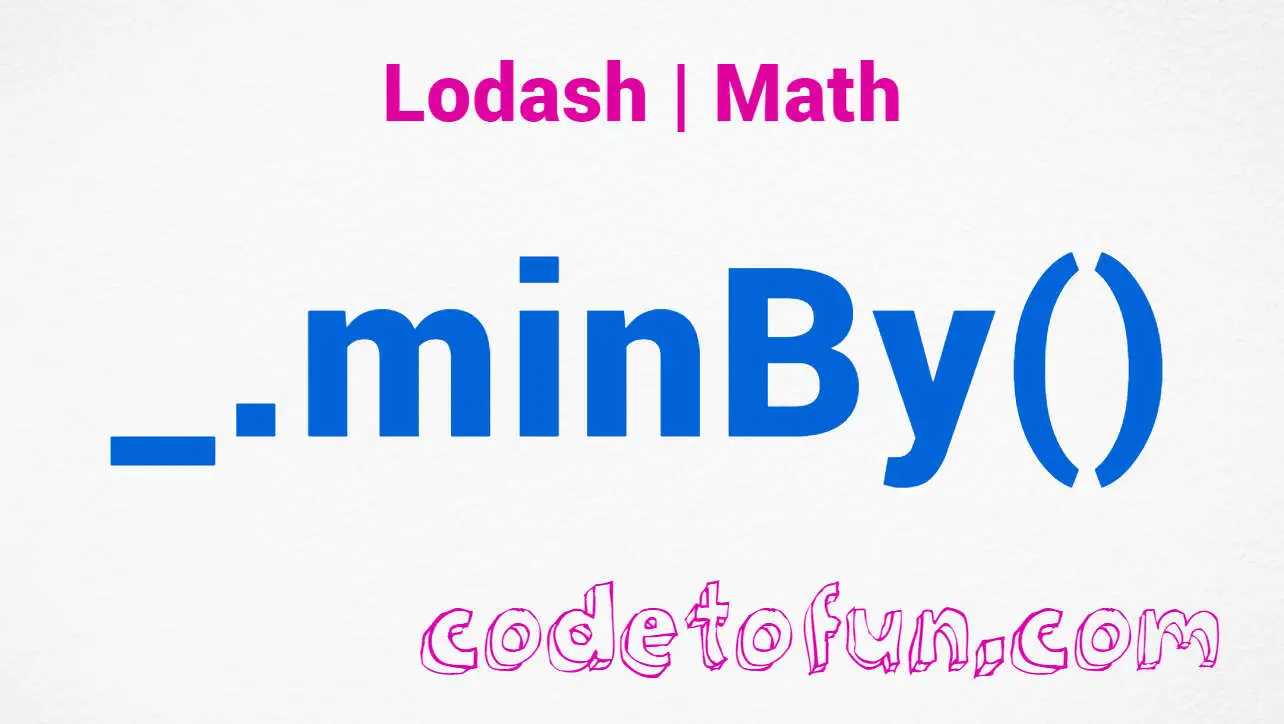
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, the need to find the minimum value within an array is a common task. Lodash, a versatile utility library, provides a solution with the _.minBy()
method. This function simplifies the process of locating the minimum value in an array, especially when dealing with complex data structures.
Join us as we explore the functionality and applications of the _.minBy()
method.
🧠 Understanding _.minBy() Method
The _.minBy()
method in Lodash is designed to find the minimum value in an array based on an iteratee function. This allows developers to specify the criterion by which the minimum value is determined, offering flexibility in scenarios involving complex data structures.
💡 Syntax
The syntax for the _.minBy()
method is straightforward:
_.minBy(array, iteratee)
- array: The array to iterate over.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.minBy()
method:
const _ = require('lodash');
const numbers = [{ 'n': 4 }, { 'n': 2 }, { 'n': 8 }, { 'n': 6 }];
const minNumber = _.minBy(numbers, 'n');
console.log(minNumber);
// Output: { 'n': 2 }
In this example, the _.minBy()
method is used to find the object with the minimum n value in the array.
🏆 Best Practices
When working with the _.minBy()
method, consider the following best practices:
Understand Data Structure:
Ensure a clear understanding of the data structure within the array. This is crucial for defining an appropriate iteratee function to extract the relevant values for comparison.
example.jsCopiedconst products = [ { name: 'Laptop', price: { base: 800, discount: 50 } }, { name: 'Smartphone', price: { base: 600, discount: 30 } }, { name: 'Tablet', price: { base: 400, discount: 20 } }, ]; const cheapestProduct = _.minBy(products, product => product.price.base - product.price.discount); console.log(cheapestProduct); // Output: { name: 'Tablet', price: { base: 400, discount: 20 } }
Handle Empty Arrays:
Consider scenarios where the input array might be empty. Implement appropriate checks to handle such cases and prevent unexpected behavior.
example.jsCopiedconst emptyArray = []; const result = _.minBy(emptyArray, 'property'); console.log(result); // Output: undefined
Optimize Iteratee Function:
Optimize the iteratee function for better performance. Keep it simple and efficient, especially when working with large datasets.
example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; const minItem = _.minBy(largeDataset, item => item.property); console.log(minItem);
📚 Use Cases
Price Comparison:
_.minBy()
is handy when comparing prices in an array of products, enabling you to find the product with the lowest price.example.jsCopiedconst products = [ { name: 'TV', price: 1200 }, { name: 'Soundbar', price: 400 }, { name: 'Game Console', price: 300 }, ]; const cheapestProduct = _.minBy(products, 'price'); console.log(cheapestProduct); // Output: { name: 'Game Console', price: 300 }
Data Analytics:
In scenarios involving data analytics,
_.minBy()
can be applied to identify the minimum value within a dataset, aiding in statistical analysis.example.jsCopiedconst dataSet = /* ...fetch data from analytics platform... */; const minDataPoint = _.minBy(dataSet, 'value'); console.log(minDataPoint);
Temperature Monitoring:
For applications such as weather monitoring,
_.minBy()
can assist in finding the coldest temperature recorded over a period.example.jsCopiedconst temperatureData = [ { location: 'City A', temperature: -5 }, { location: 'City B', temperature: 2 }, { location: 'City C', temperature: -10 }, ]; const coldestCity = _.minBy(temperatureData, 'temperature'); console.log(coldestCity); // Output: { location: 'City C', temperature: -10 }
🎉 Conclusion
The _.minBy()
method in Lodash provides a concise and efficient solution for finding the minimum value within an array based on a specified criterion. Whether you're comparing prices, analyzing data, or monitoring temperatures, this method offers flexibility and ease of use.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.minBy()
method in your Lodash projects.
👨💻 Join our Community:
Author
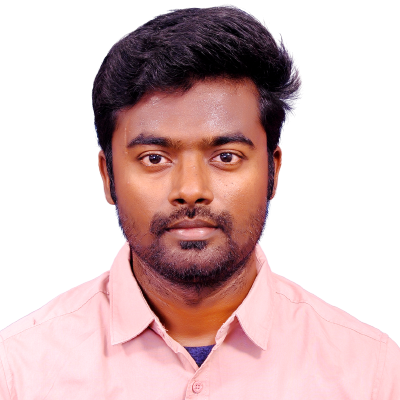
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
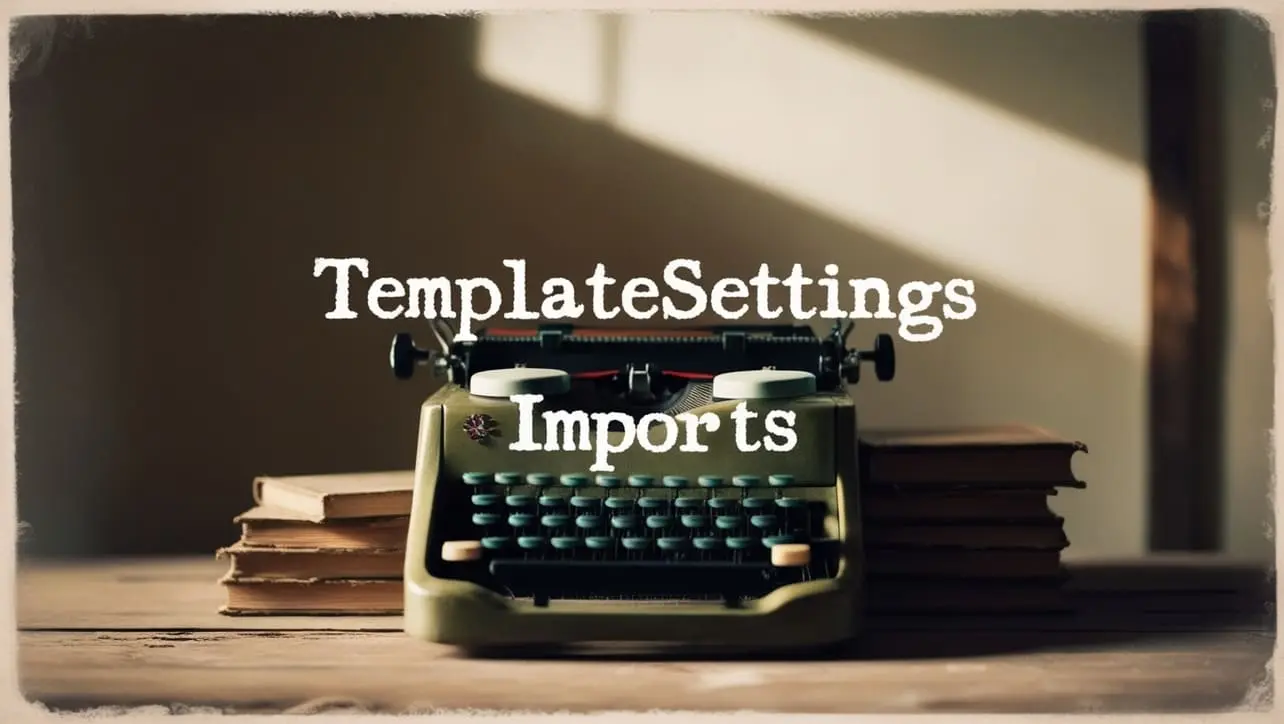
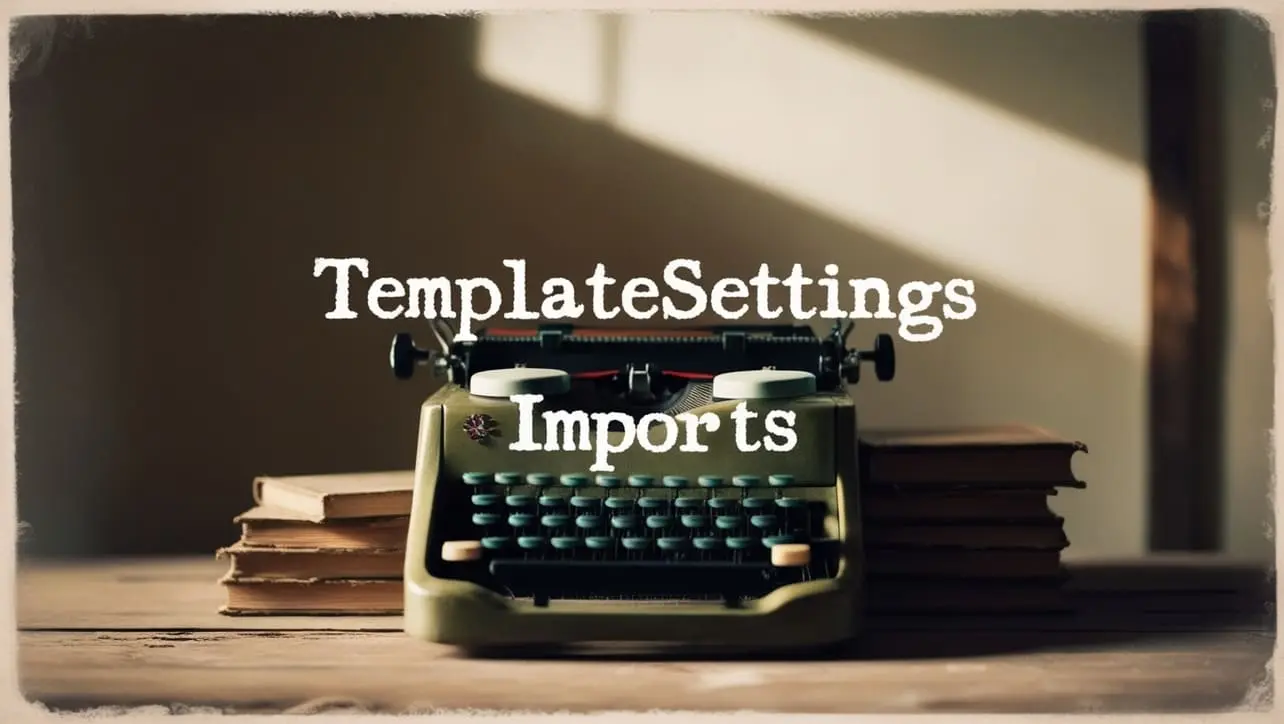
Lodash _.templateSettings.imports Property
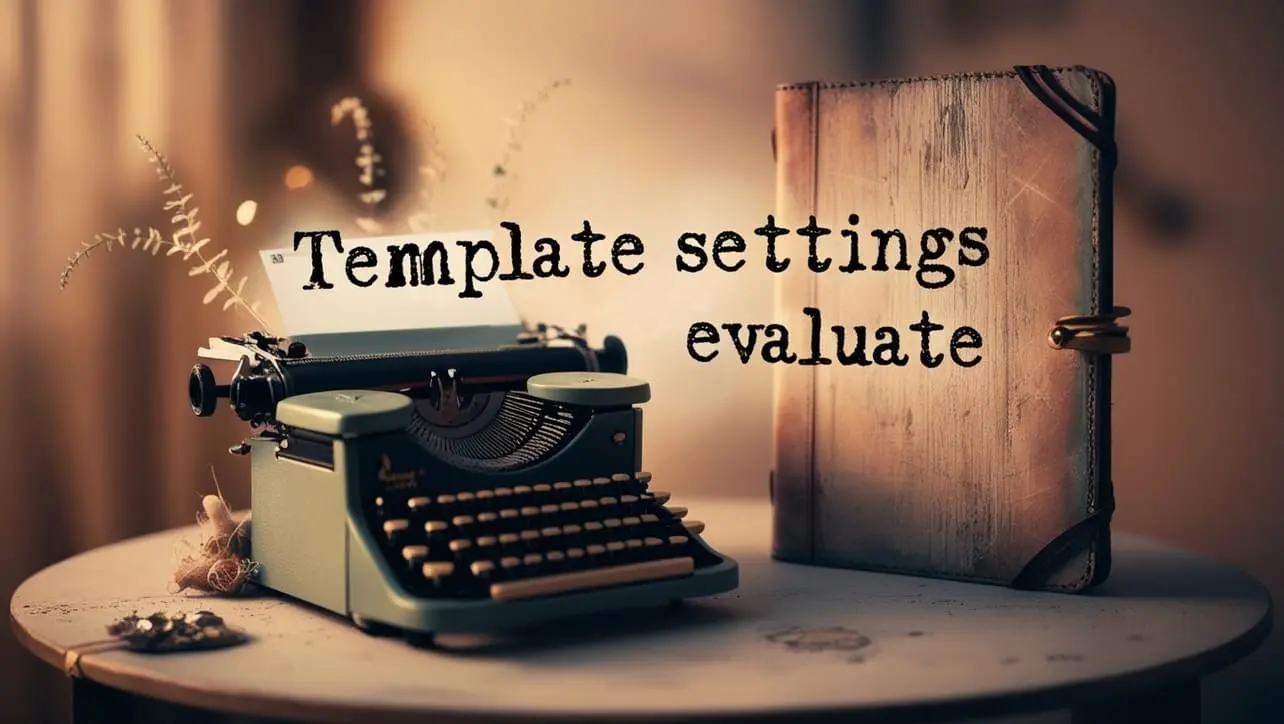
Lodash _.templateSettings.evaluate Property
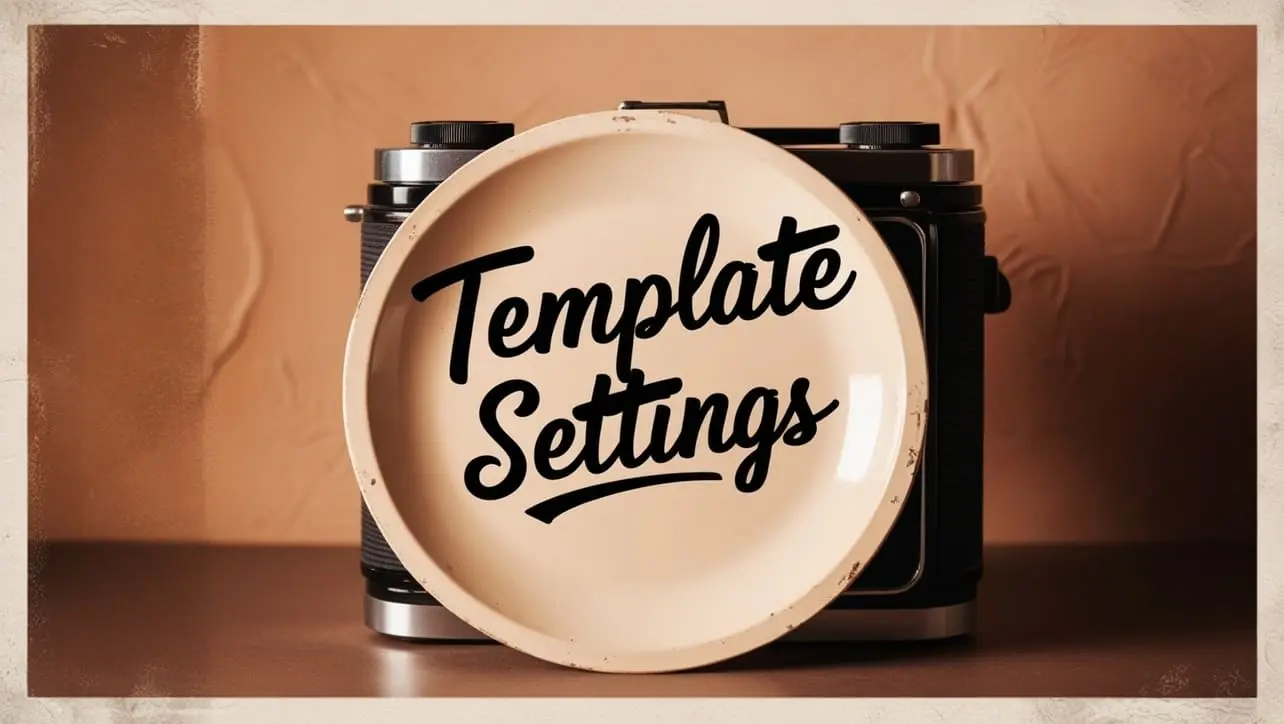
Lodash _.templateSettings Property
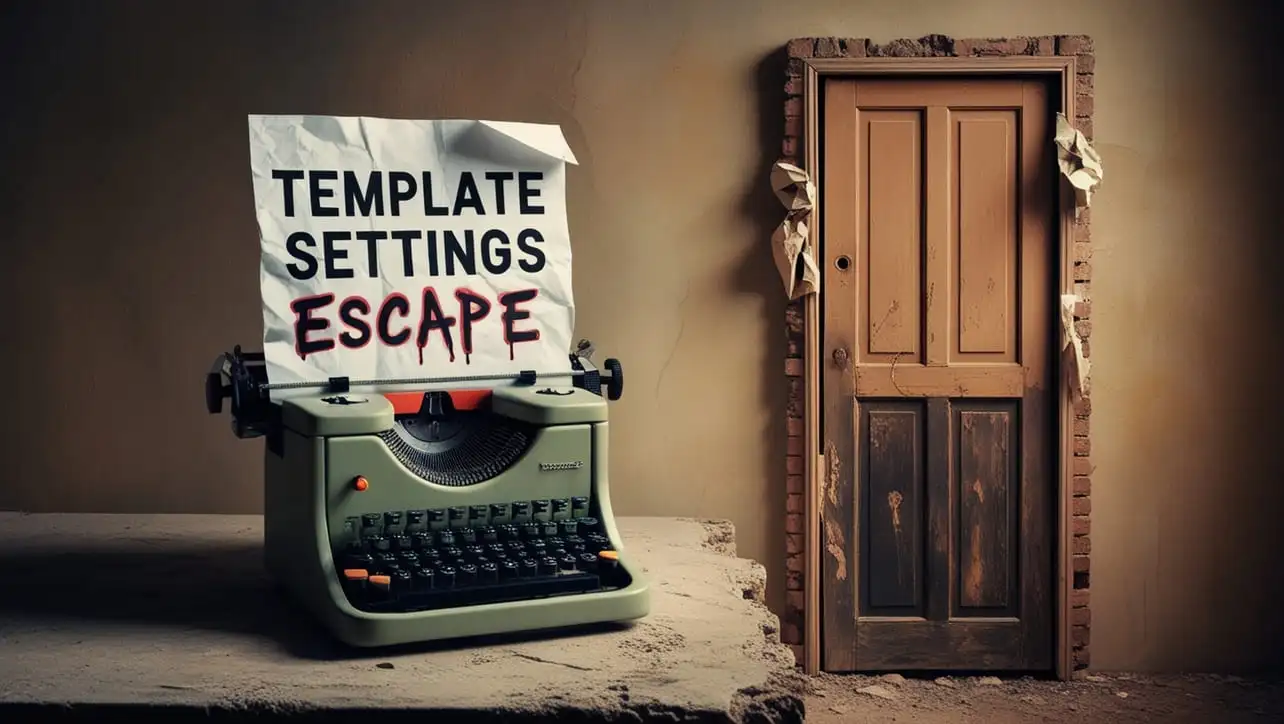
Lodash _.templateSettings.escape Property
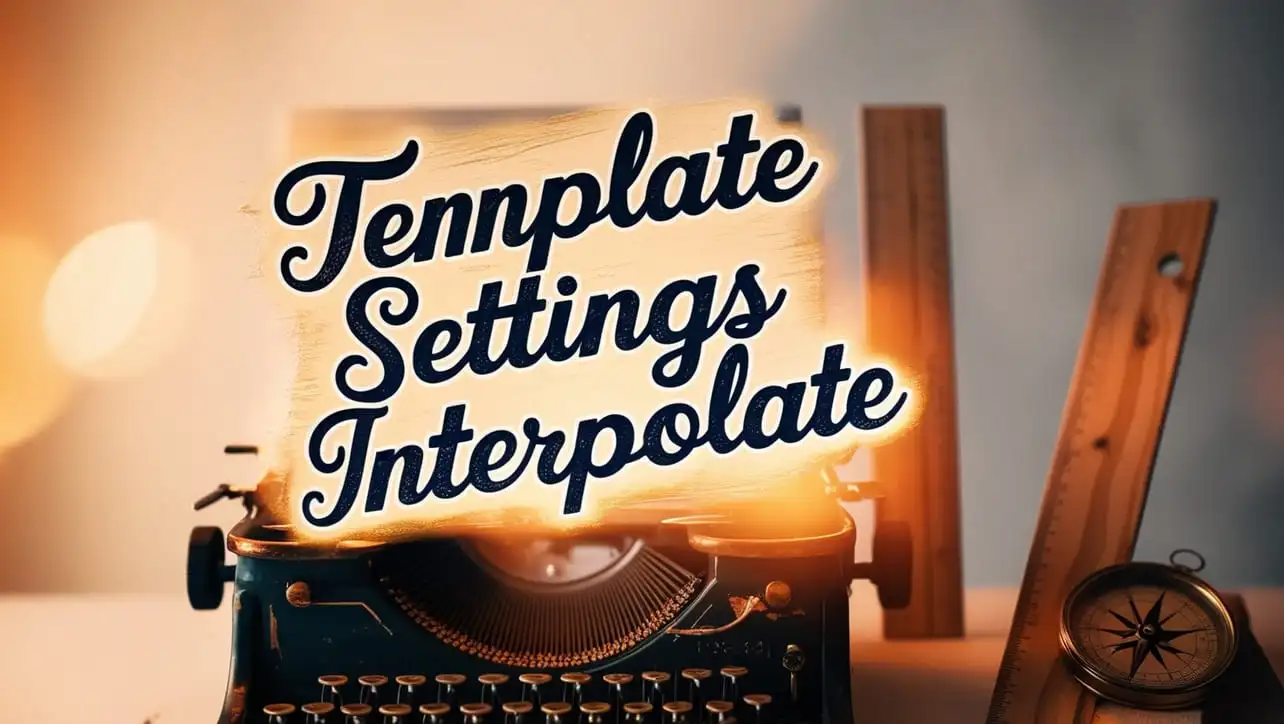
Lodash _.templateSettings.interpolate Property
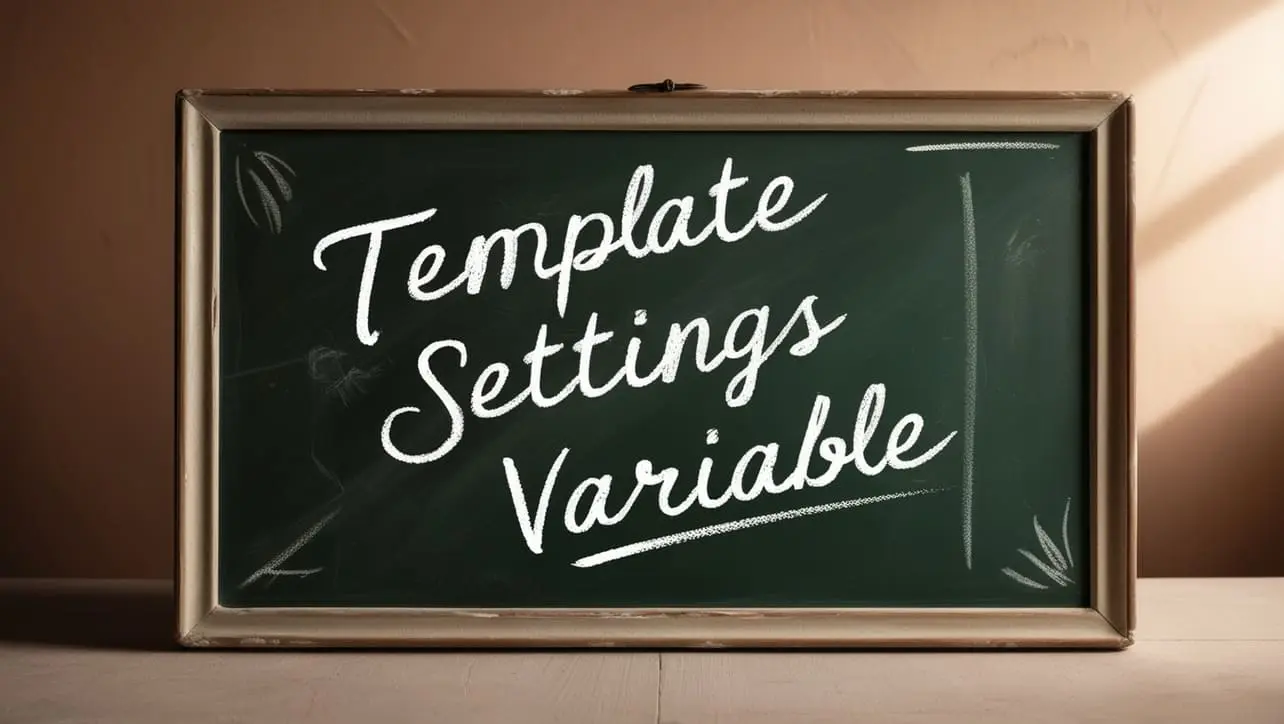
If you have any doubts regarding this article (Lodash _.minBy() Math Method), please comment here. I will help you immediately.