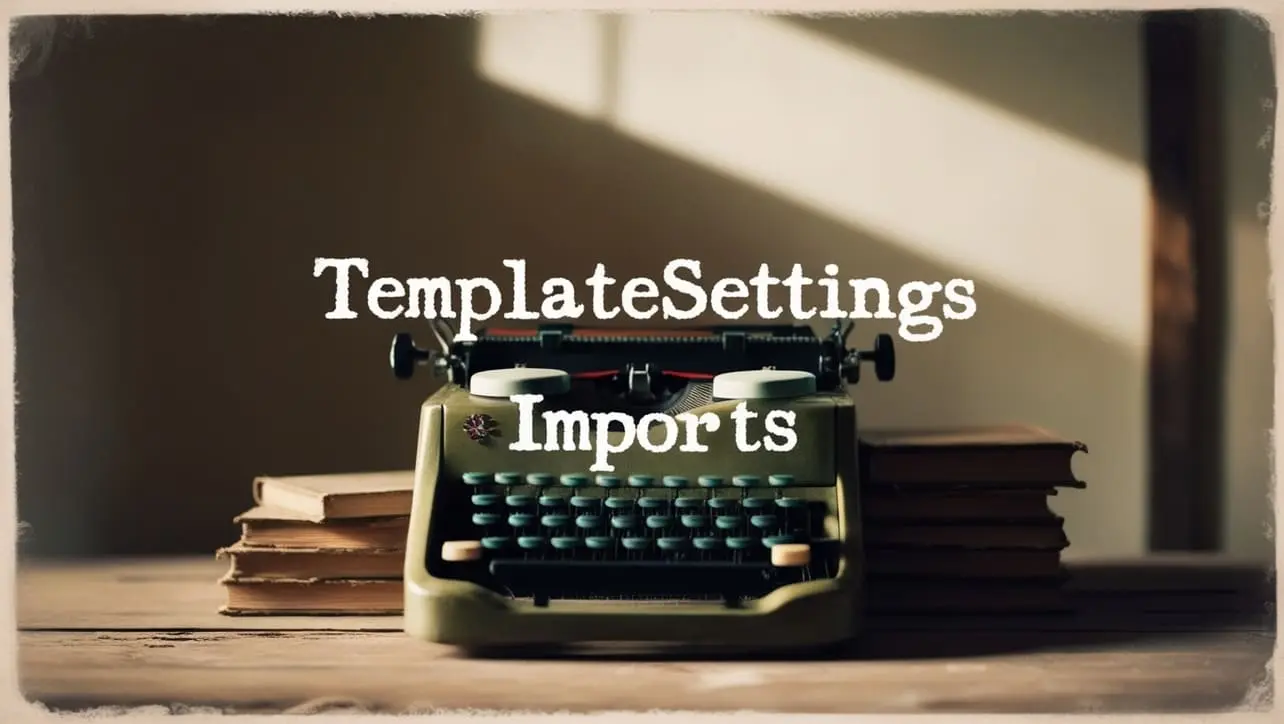
Lodash _.maxBy() Math Method
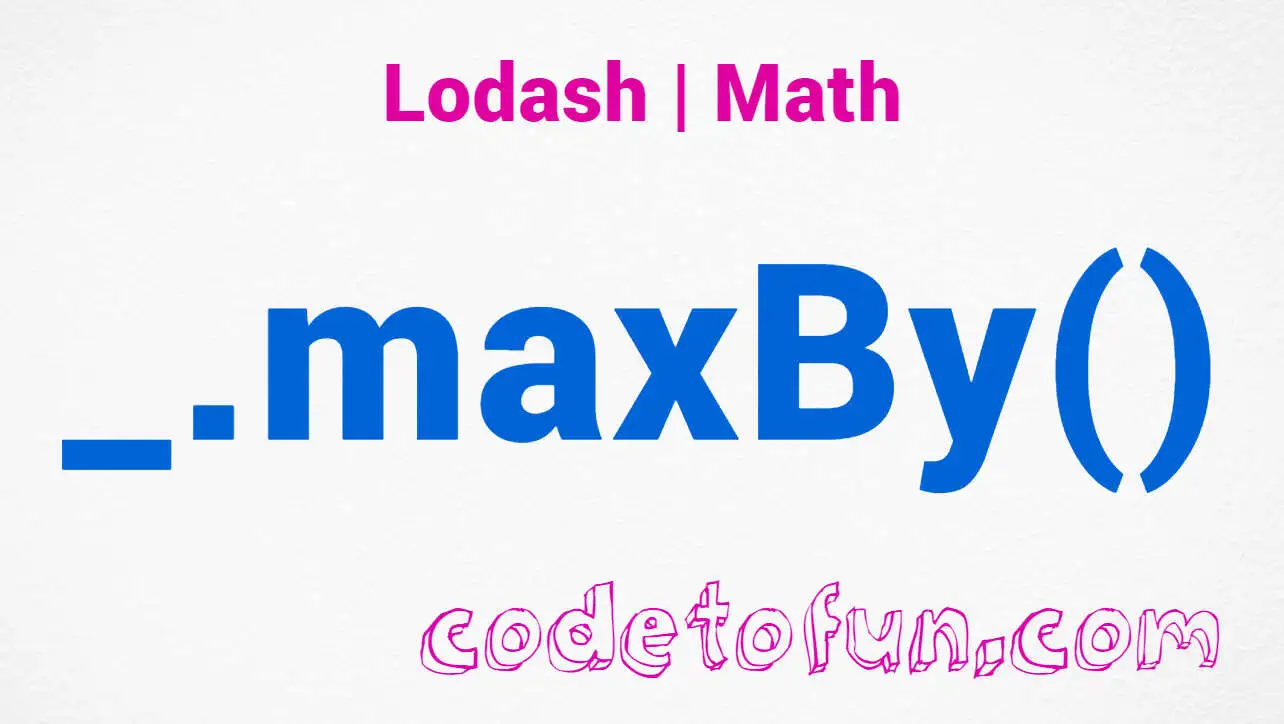
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficient data manipulation often involves finding the maximum value based on specific criteria. Lodash, a powerful utility library, provides the _.maxBy()
method, simplifying the process of identifying the maximum value within a collection of objects by leveraging a custom iterator function.
This method is particularly valuable when working with complex data structures, allowing developers to extract maximum values with ease.
🧠 Understanding _.maxBy() Method
The _.maxBy()
method in Lodash is designed to find the maximum value within a collection, such as an array of objects, based on a specified iteratee function. This iteratee function defines the criteria for determining the maximum value, offering flexibility and customization in the comparison process.
💡 Syntax
The syntax for the _.maxBy()
method is straightforward:
_.maxBy(collection, [iteratee])
- collection: The collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.maxBy()
method:
const _ = require('lodash');
const arrayOfObjects = [
{ id: 1, value: 15 },
{ id: 2, value: 30 },
{ id: 3, value: 20 },
];
const maxObject = _.maxBy(arrayOfObjects, obj => obj.value);
console.log(maxObject);
// Output: { id: 2, value: 30 }
In this example, the _.maxBy()
method is applied to the arrayOfObjects collection, finding the object with the maximum value property based on the iteratee function.
🏆 Best Practices
When working with the _.maxBy()
method, consider the following best practices:
Understanding Iteratee Function:
Comprehend the role of the iteratee function in determining the maximum value. Customize the iteratee function to focus on specific properties or calculations based on your requirements.
example.jsCopiedconst arrayOfObjects = [ { id: 1, score: { value: 80 } }, { id: 2, score: { value: 95 } }, { id: 3, score: { value: 90 } }, ]; const maxScoreObject = _.maxBy(arrayOfObjects, obj => obj.score.value); console.log(maxScoreObject); // Output: { id: 2, score: { value: 95 } }
Handling Empty Collections:
Account for scenarios where the collection might be empty. Implement proper error handling or default behaviors to manage such cases.
example.jsCopiedconst emptyCollection = []; const defaultMax = _.maxBy(emptyCollection, obj => obj.value) || { value: 0 }; console.log(defaultMax); // Output: { value: 0 }
Performance Considerations:
Be mindful of the performance implications when dealing with large collections. While
_.maxBy()
is efficient, consider the size of your dataset and optimize accordingly.example.jsCopiedconst largeCollection = /* ...generate a large collection... */; console.time('maxBy'); const maxObjectInLargeCollection = _.maxBy(largeCollection, obj => obj.value); console.timeEnd('maxBy'); console.log(maxObjectInLargeCollection);
📚 Use Cases
Extracting Maximum Values:
Use
_.maxBy()
to easily extract objects with the maximum values from a collection, streamlining the process of identifying top-performing elements.example.jsCopiedconst studentScores = [ { student: 'Alice', score: 95 }, { student: 'Bob', score: 88 }, { student: 'Charlie', score: 92 }, ]; const topScorer = _.maxBy(studentScores, obj => obj.score); console.log(topScorer); // Output: { student: 'Alice', score: 95 }
Ranking and Sorting:
Leverage
_.maxBy()
in conjunction with sorting to create a ranking system based on specific criteria within your collection.example.jsCopiedconst players = [ { name: 'John', points: 120 }, { name: 'Emma', points: 95 }, { name: 'Mike', points: 110 }, ]; const topPlayer = _.maxBy(players, obj => obj.points); console.log(topPlayer); // Output: { name: 'John', points: 120 }
Dynamic Property Comparison:
Employ
_.maxBy()
for dynamic property comparison, allowing users or configurations to determine which property defines the maximum value.example.jsCopiedconst data = [ { id: 1, sales: 150 }, { id: 2, revenue: 200 }, { id: 3, profit: 180 }, ]; const maxSales = _.maxBy(data, obj => obj.sales) || { sales: 0 }; console.log(maxSales); // Output: { id: 2, sales: 200 }
🎉 Conclusion
The _.maxBy()
method in Lodash provides a valuable solution for efficiently finding the maximum value within a collection based on a custom iteratee function. Whether you're extracting top performers, implementing ranking systems, or dynamically comparing properties, this method empowers developers to handle complex data structures with ease.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.maxBy()
method in your Lodash projects.
👨💻 Join our Community:
Author
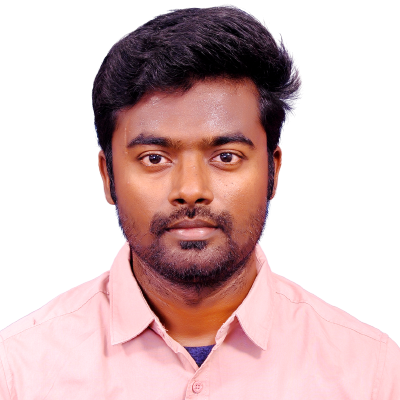
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
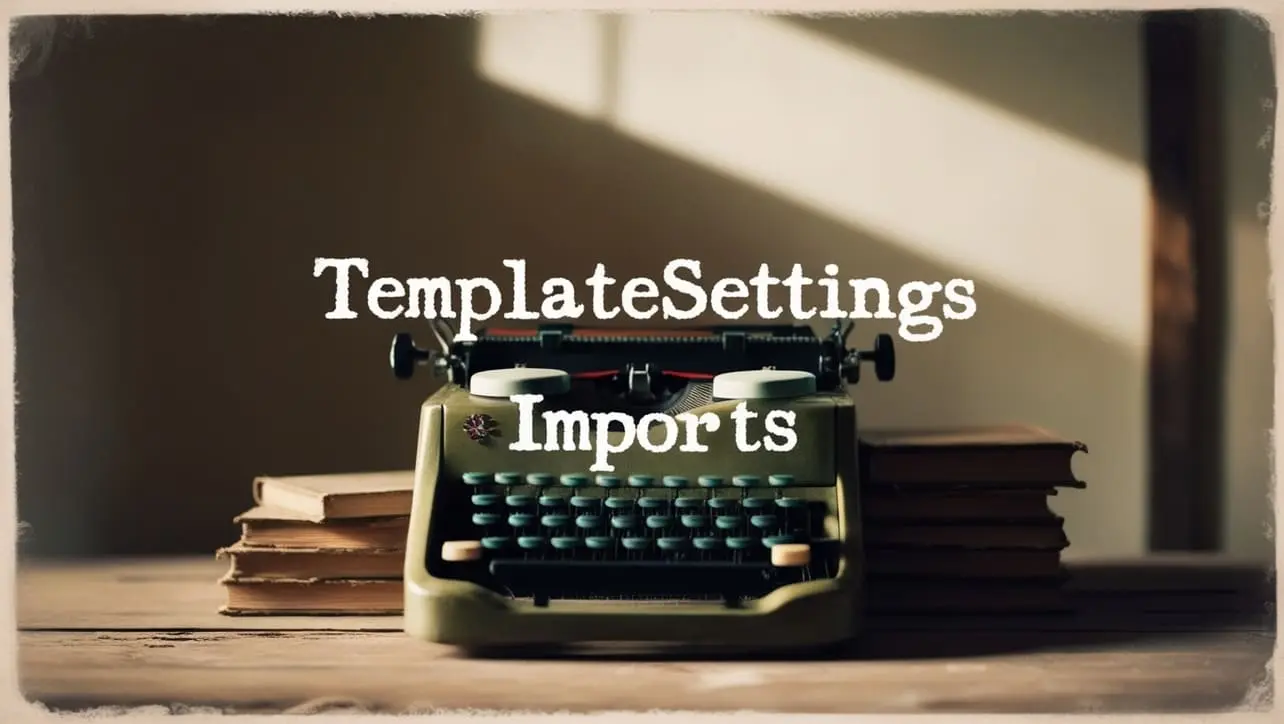
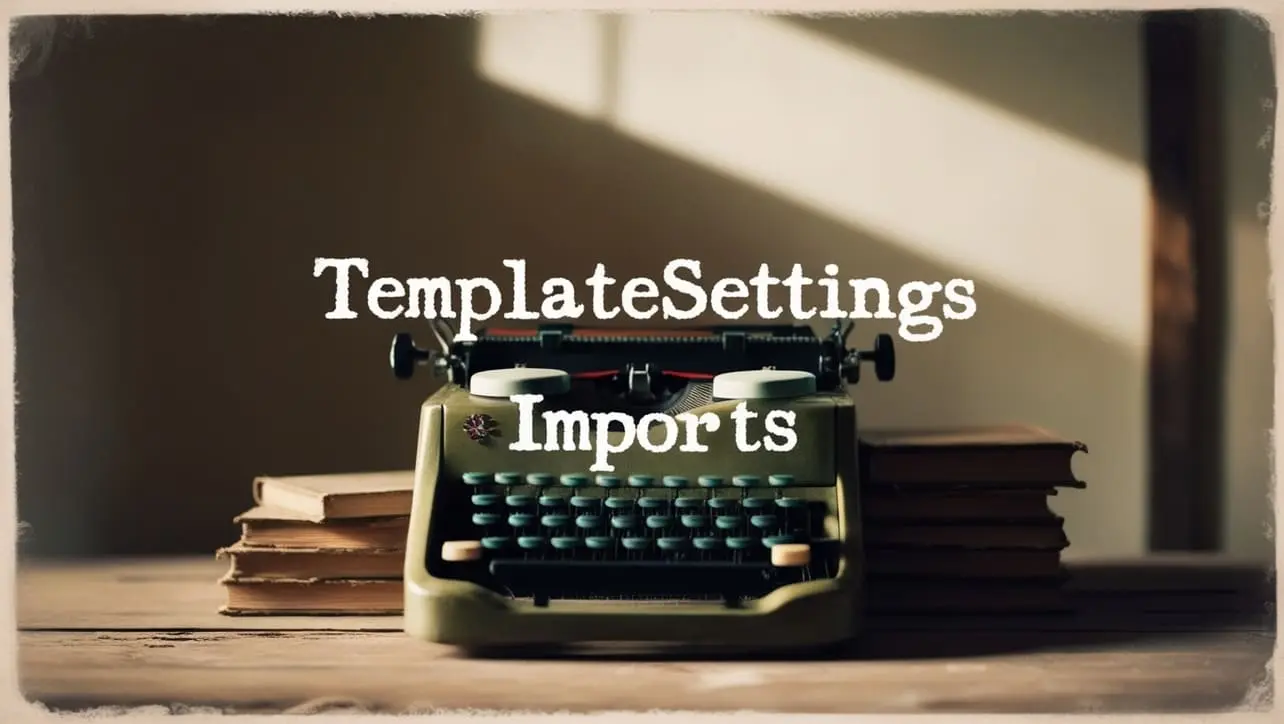
Lodash _.templateSettings.imports Property
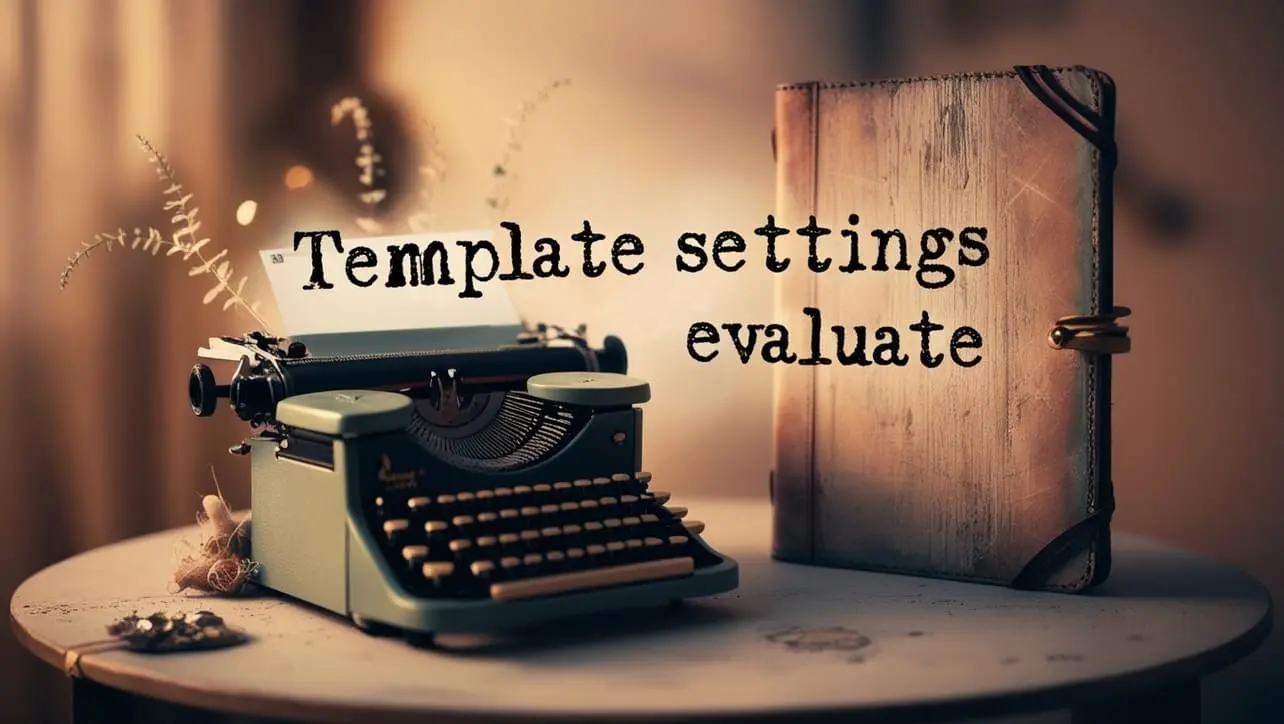
Lodash _.templateSettings.evaluate Property
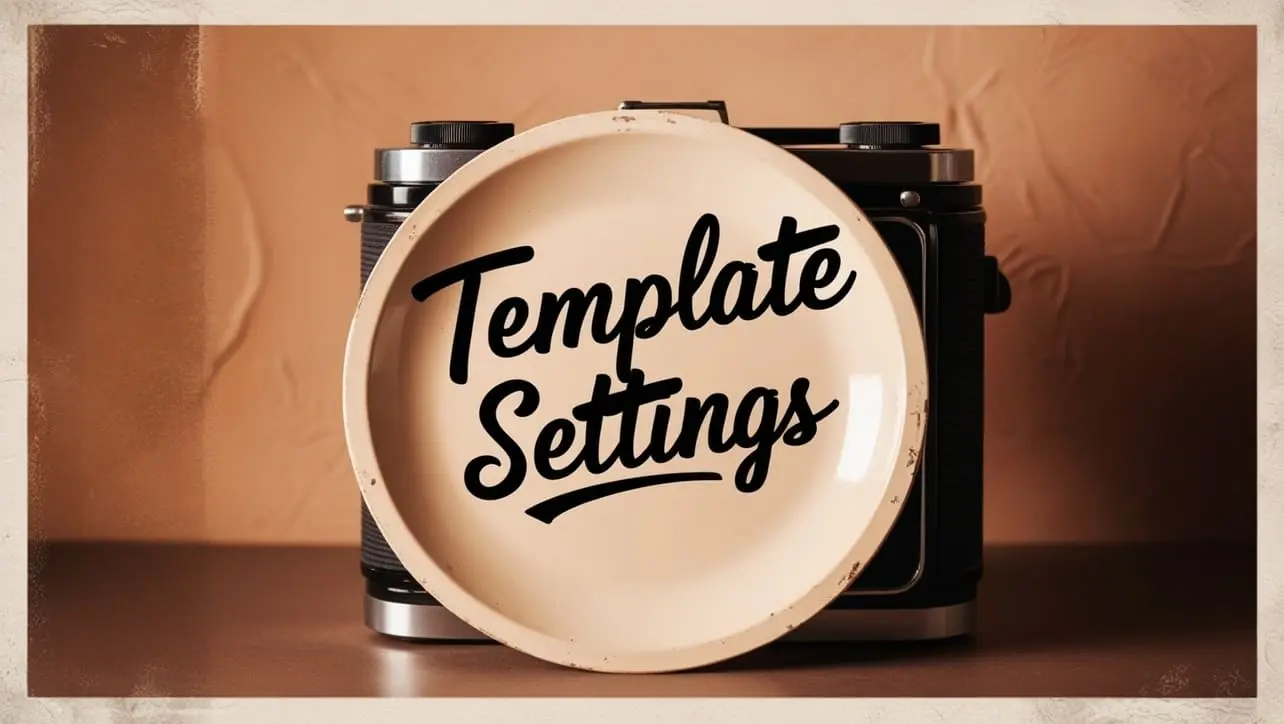
Lodash _.templateSettings Property
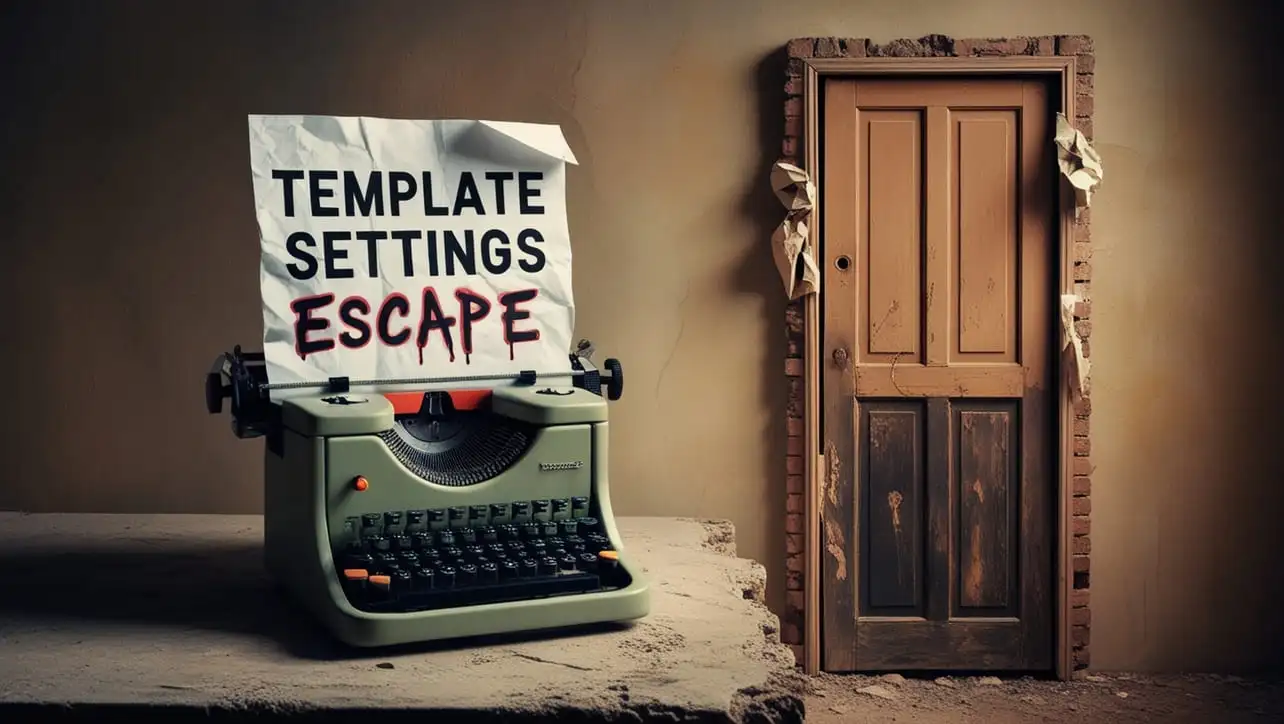
Lodash _.templateSettings.escape Property
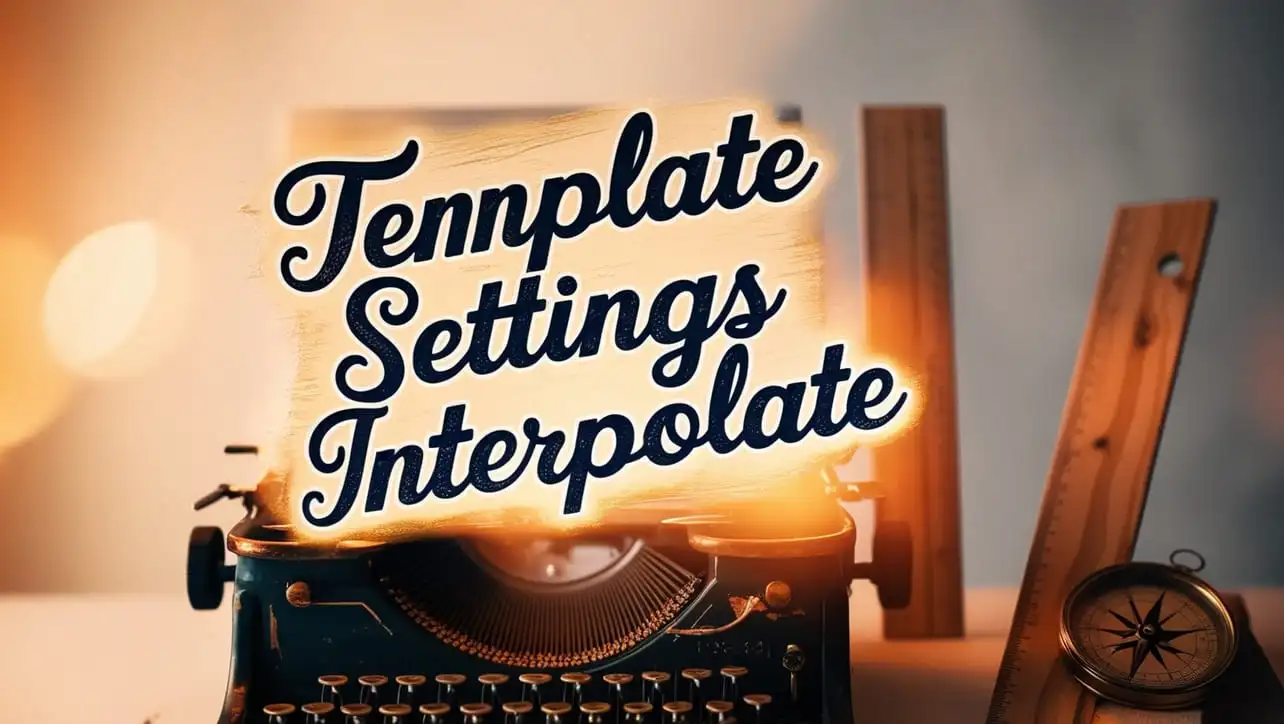
Lodash _.templateSettings.interpolate Property
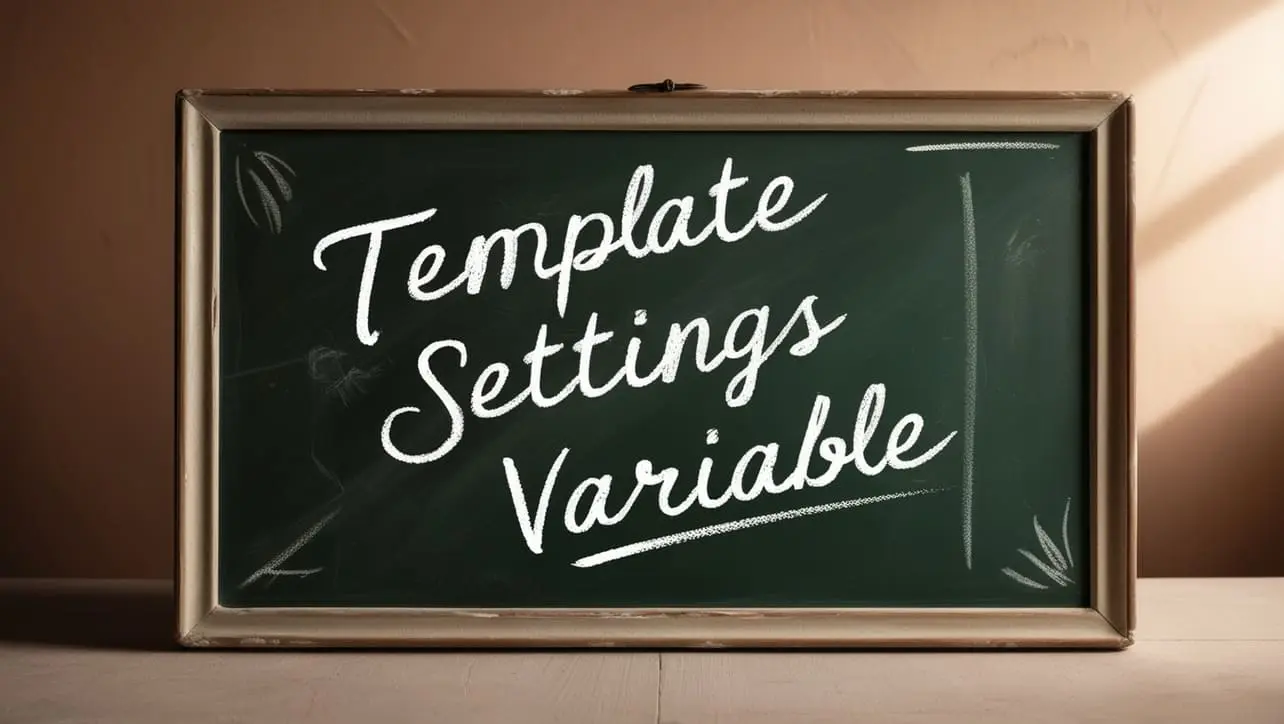
If you have any doubts regarding this article (Lodash _.maxBy() Math Method), please comment here. I will help you immediately.