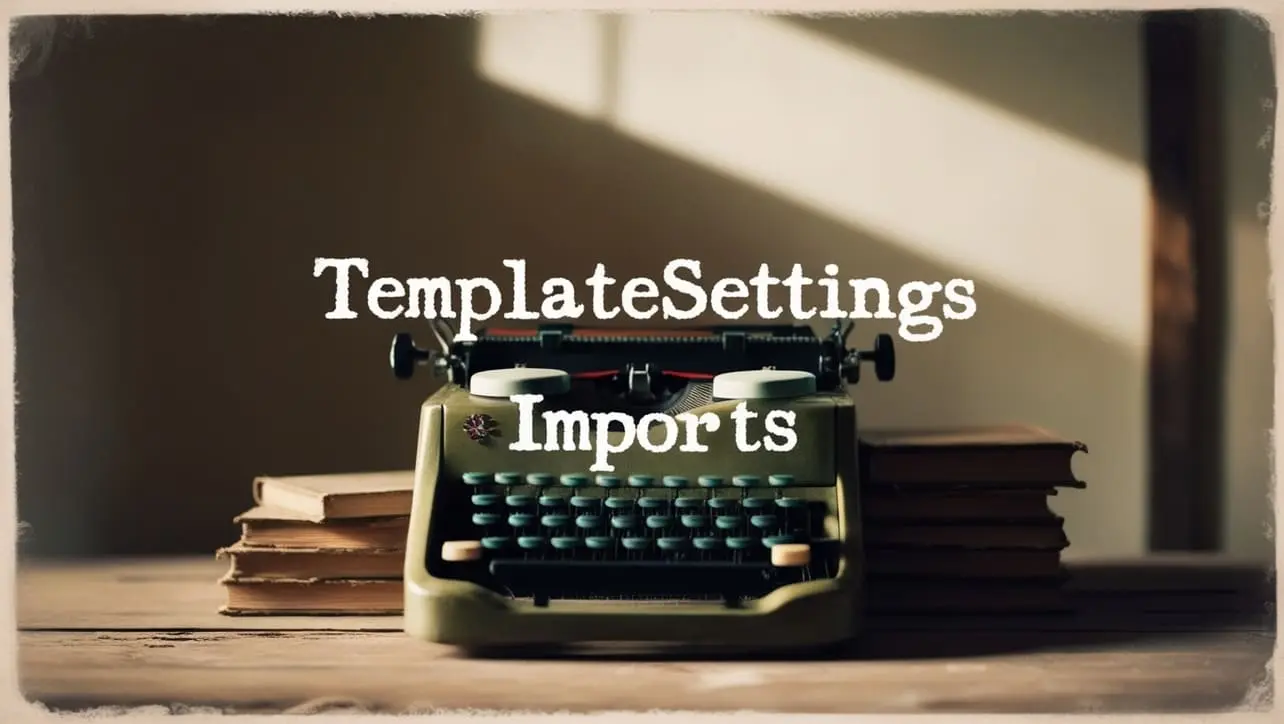
Lodash _.round() Math Method
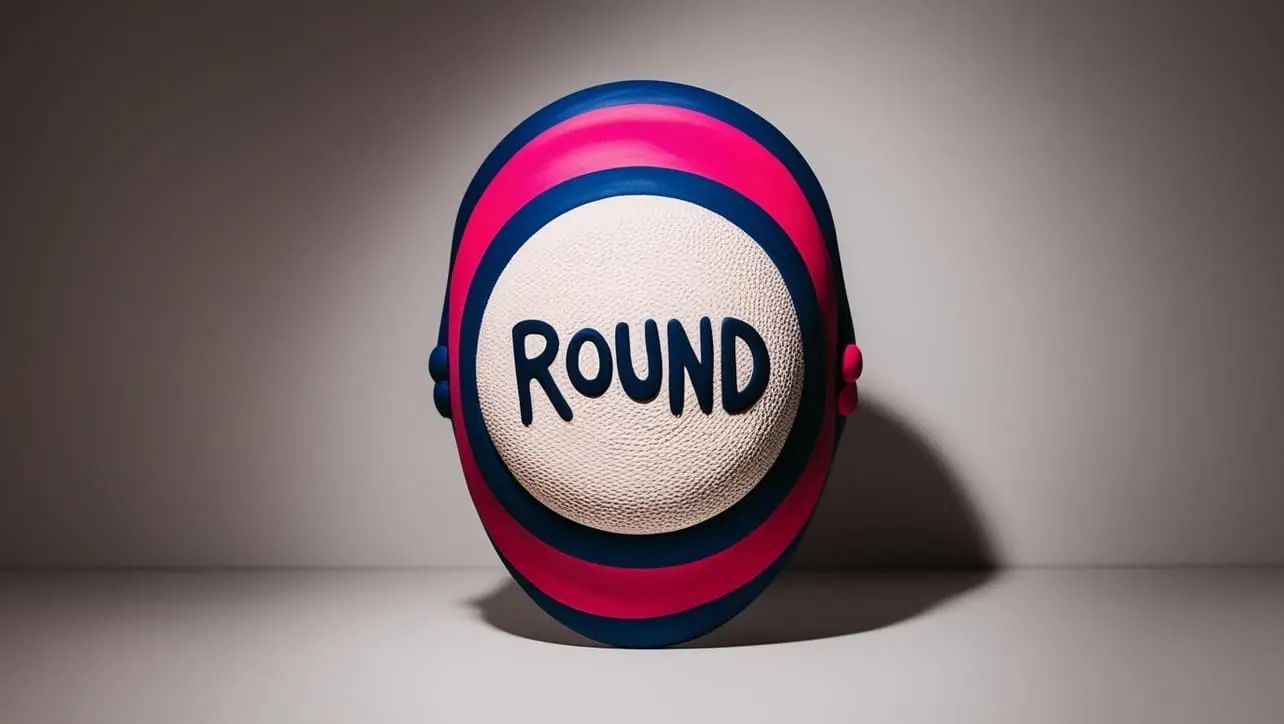
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, precise numeric calculations are often crucial. Lodash, a powerful utility library, provides a range of functions to simplify common tasks. Among these functions is the _.round()
method, a versatile tool for rounding numbers with customizable precision.
This method is invaluable for scenarios where accurate numeric representation is essential, making it a must-have for developers working with numeric data.
🧠 Understanding _.round() Method
The _.round()
method in Lodash is designed to round a number to a specified precision. This allows developers to control the number of decimal places in their numeric values, ensuring accuracy in calculations and representations.
💡 Syntax
The syntax for the _.round()
method is straightforward:
_.round(number, [precision=0])
- number: The number to round.
- precision (Optional): The precision to round to (default is 0).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.round()
method:
const _ = require('lodash');
const originalNumber = 5.678;
const roundedNumber = _.round(originalNumber, 1);
console.log(roundedNumber);
// Output: 5.7
In this example, the originalNumber is rounded to one decimal place using _.round()
.
🏆 Best Practices
When working with the _.round()
method, consider the following best practices:
Specify Precision:
When using
_.round()
, explicitly specify the precision to avoid unexpected rounding behavior. This is especially important when dealing with calculations that require a specific number of decimal places.example.jsCopiedconst preciseCalculation = 12.345 * 0.1; // Results in an imprecise floating-point number const roundedResult = _.round(preciseCalculation, 2); console.log(roundedResult); // Output: 1.23
Handle Edge Cases:
Be aware of potential edge cases, such as rounding very large or very small numbers. Adjust precision accordingly to avoid unintended outcomes.
example.jsCopiedconst largeNumber = 9876543210.123456789; const roundedLargeNumber = _.round(largeNumber, -5); console.log(roundedLargeNumber); // Output: 9876543210
Combine with Other Functions:
Integrate
_.round()
with other mathematical operations to create sophisticated numeric processing pipelines. This can be particularly useful in financial applications or scientific computations.example.jsCopiedconst calculateAverage = values => { const sum = _.sum(values); const average = sum / values.length; return _.round(average, 2); }; const sampleData = [23.456, 45.789, 12.345, 67.891]; const averageResult = calculateAverage(sampleData); console.log(averageResult); // Output: 37.12
📚 Use Cases
Financial Calculations:
In financial applications, precise rounding is often crucial.
_.round()
can be employed to ensure accurate representation of monetary values.example.jsCopiedconst financialValue = 1234.56789; const roundedFinancialValue = _.round(financialValue, 2); console.log(roundedFinancialValue); // Output: 1234.57
User Interface Display:
When displaying numeric values in a user interface, using
_.round()
can provide a clean and visually appealing presentation.example.jsCopiedconst rawInput = 36.789654; const formattedDisplay = _.round(rawInput, 1); console.log(formattedDisplay); // Output: 36.8
Scientific Calculations:
For scientific or engineering applications where precision is critical,
_.round()
can be part of a toolkit for accurate numeric manipulations.example.jsCopiedconst scientificValue = 3.14159265358979323846; const roundedScientificValue = _.round(scientificValue, 5); console.log(roundedScientificValue); // Output: 3.14159
🎉 Conclusion
The _.round()
method in Lodash provides a reliable and customizable solution for rounding numbers in JavaScript. Whether you're working on financial applications, user interfaces, or scientific computations, _.round()
empowers you to achieve precision and accuracy in your numeric manipulations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.round()
method in your Lodash projects.
👨💻 Join our Community:
Author
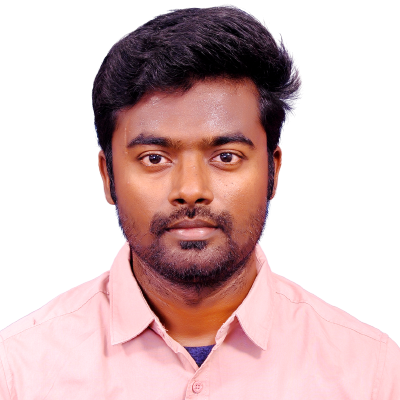
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
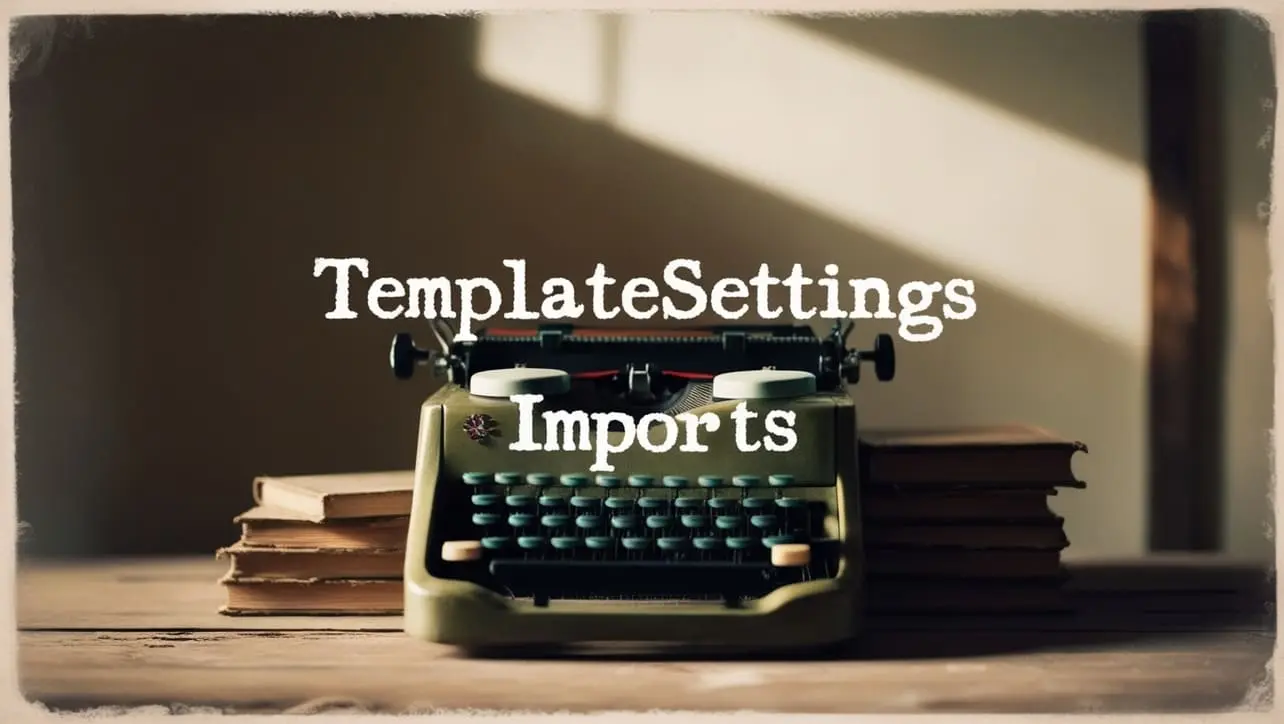
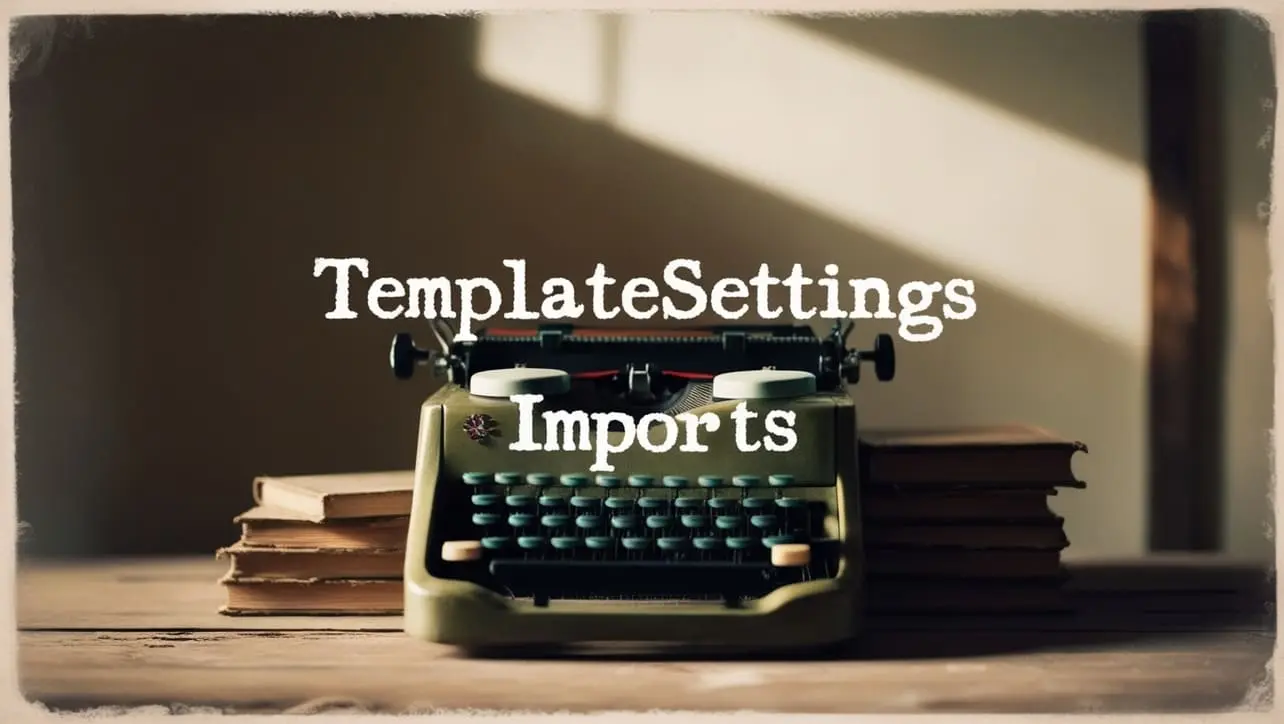
Lodash _.templateSettings.imports Property
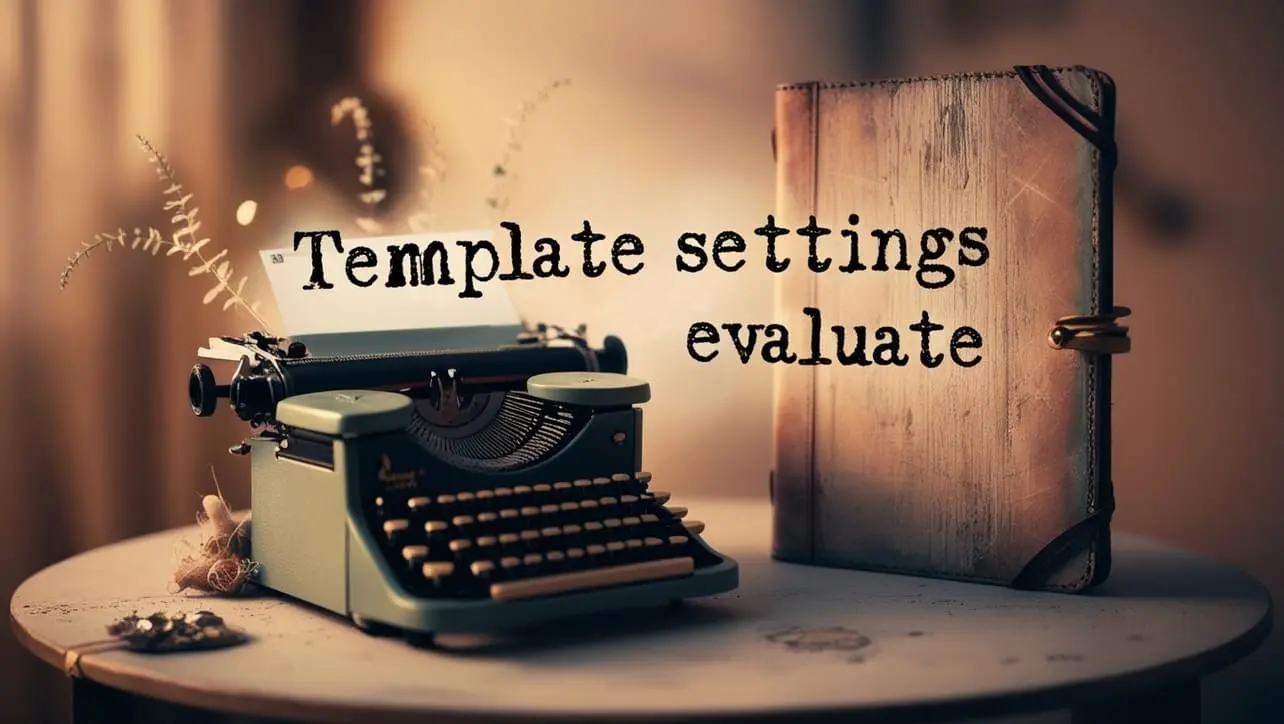
Lodash _.templateSettings.evaluate Property
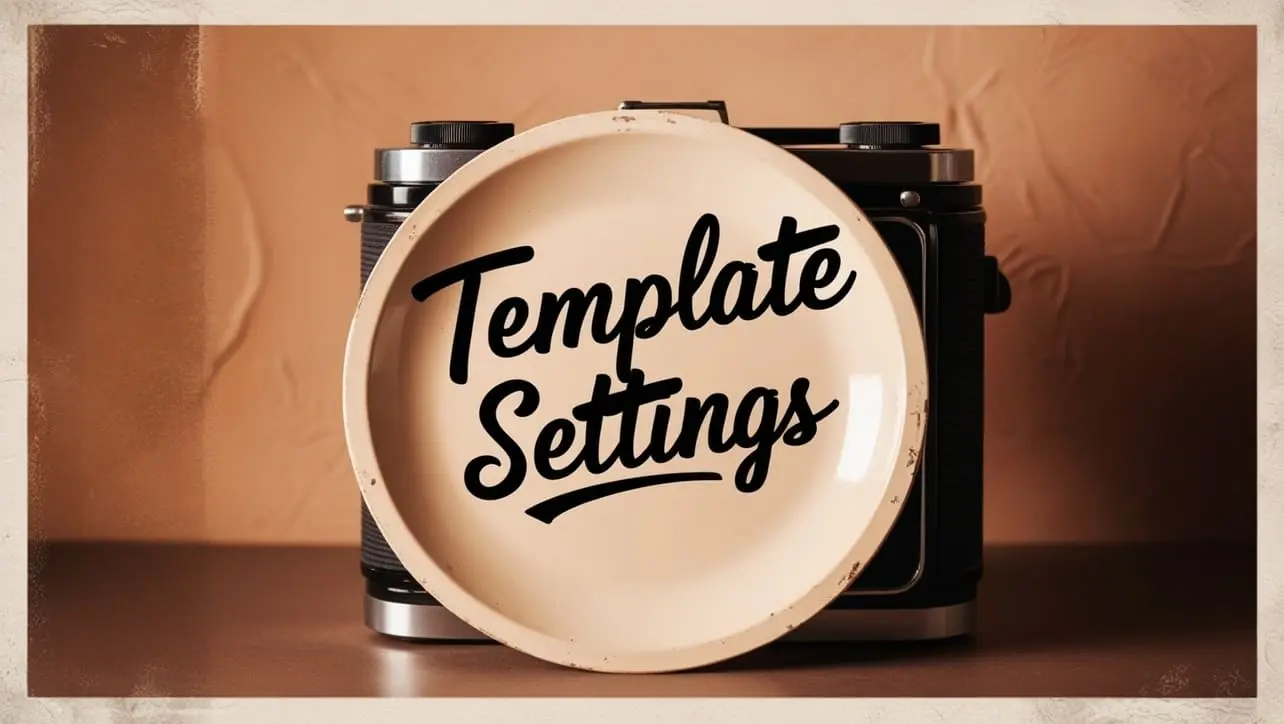
Lodash _.templateSettings Property
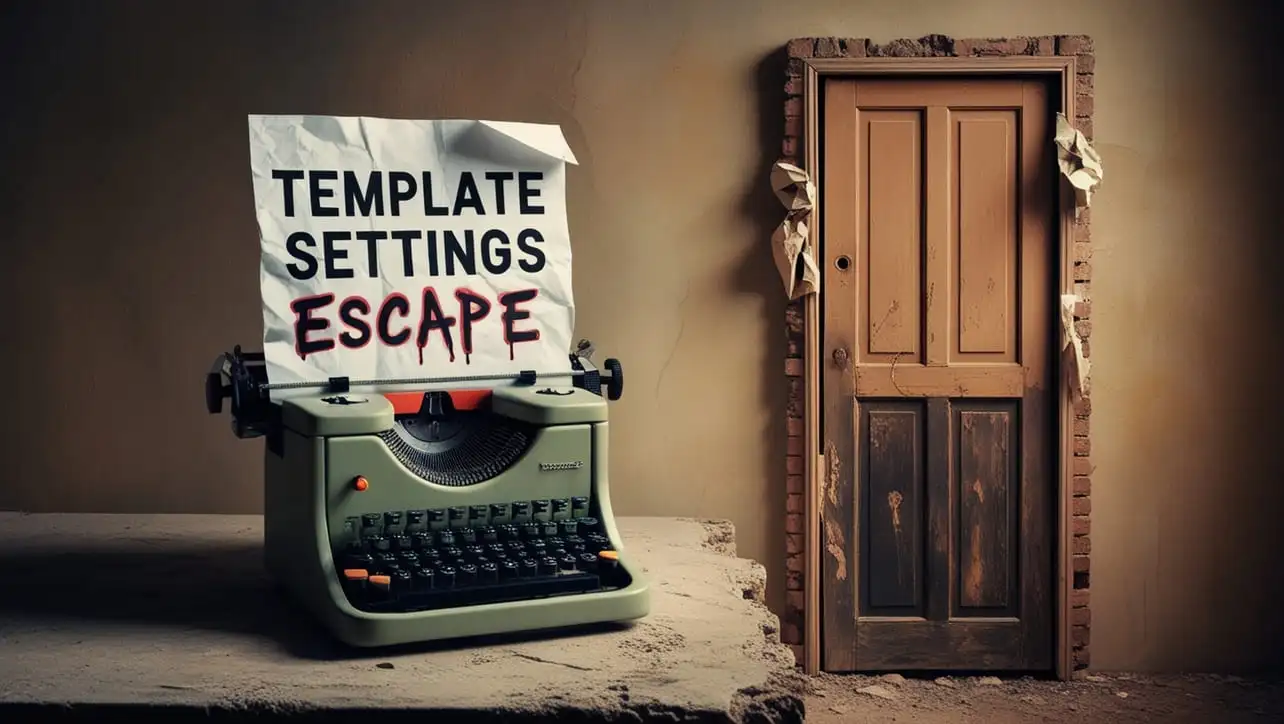
Lodash _.templateSettings.escape Property
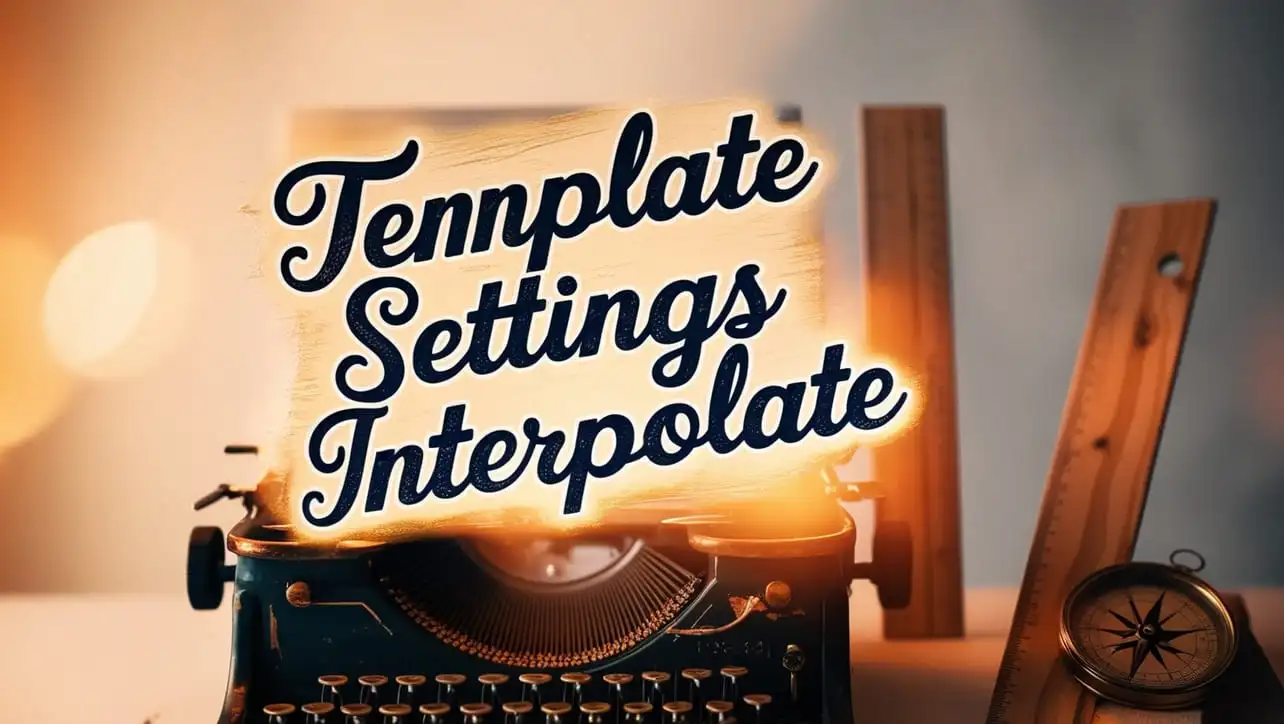
Lodash _.templateSettings.interpolate Property
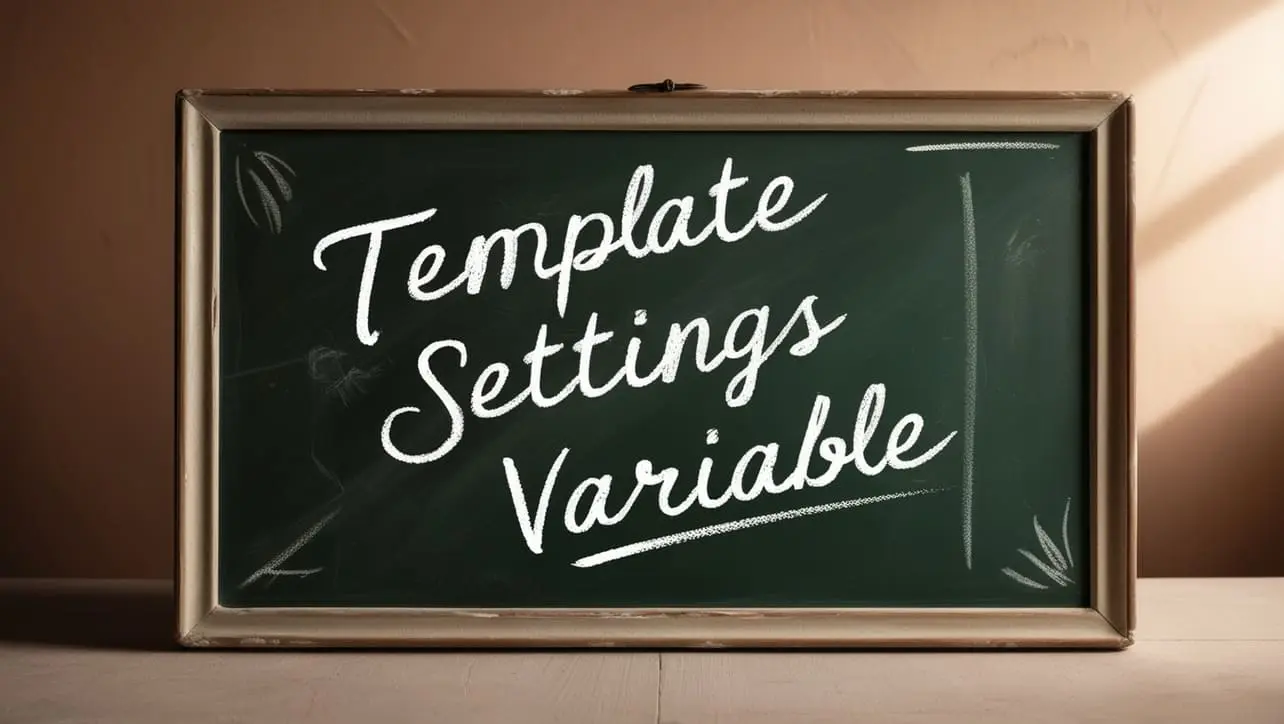
If you have any doubts regarding this article (Lodash _.round() Math Method), please comment here. I will help you immediately.