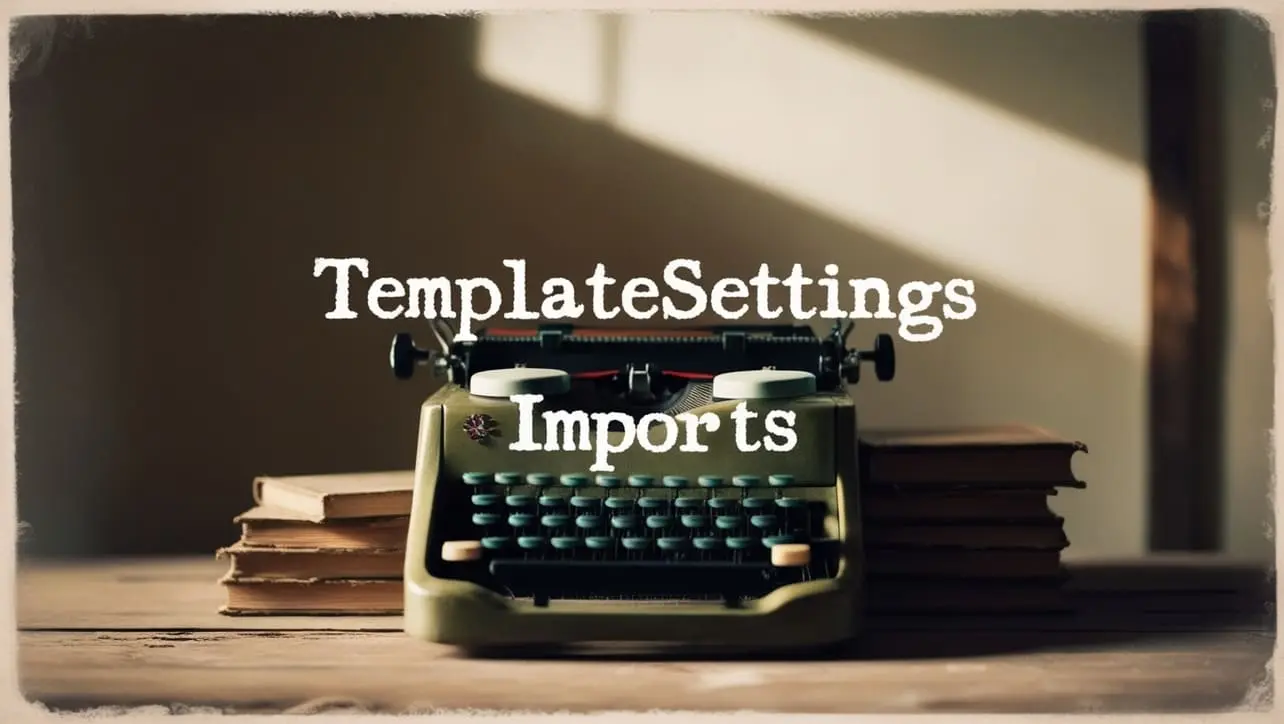
Lodash _.meanBy() Math Method
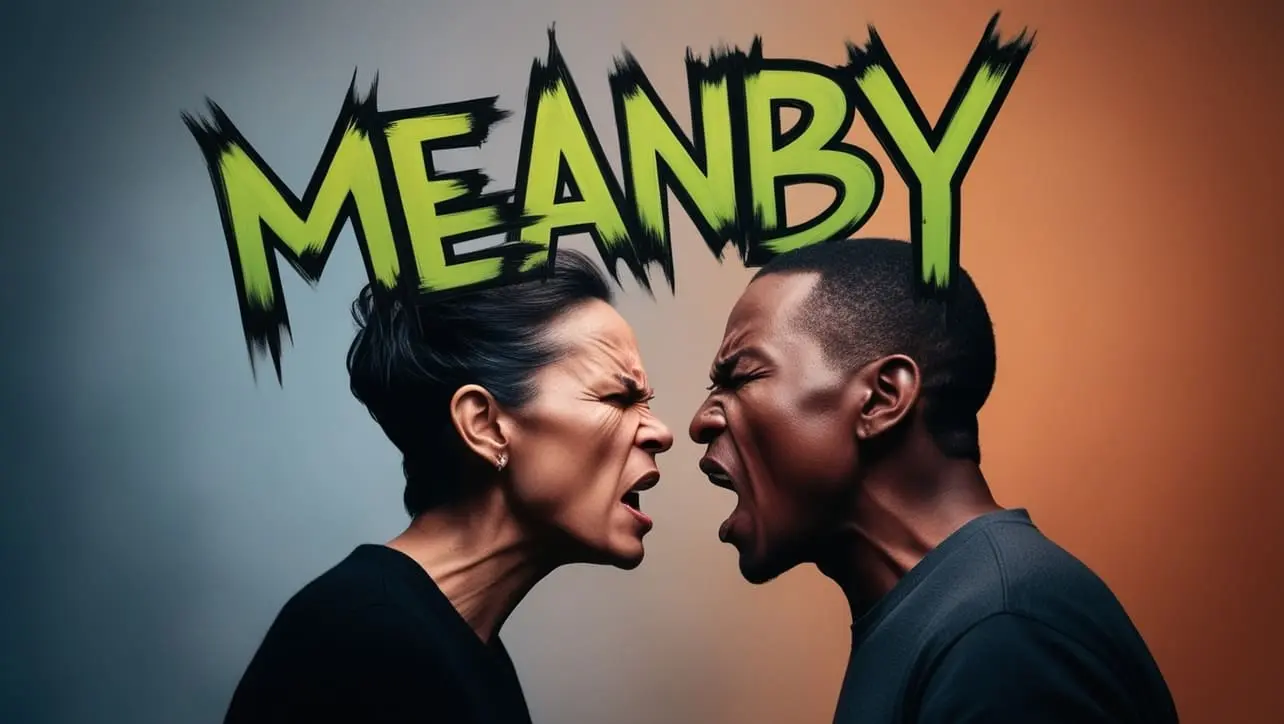
Photo Credit to CodeToFun
🙋 Introduction
When working with numeric data in JavaScript, having efficient tools for calculations is essential. Lodash, a comprehensive utility library, offers a variety of functions to simplify array manipulation. Among these functions is the _.meanBy()
method, a powerful tool for calculating the mean (average) of an array based on a specific criterion.
This method not only simplifies mathematical operations but also provides flexibility in determining the criteria for the mean calculation.
🧠 Understanding _.meanBy() Method
The _.meanBy()
method in Lodash allows you to calculate the mean of an array based on a specified iteratee function. This is particularly useful when working with arrays of objects and needing to calculate the mean based on a specific property or condition.
💡 Syntax
The syntax for the _.meanBy()
method is straightforward:
_.meanBy(array, iteratee)
- array: The array to process.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.meanBy()
method:
const _ = require('lodash');
const data = [
{ value: 10 },
{ value: 20 },
{ value: 30 },
];
const meanValue = _.meanBy(data, 'value');
console.log(meanValue);
// Output: 20
In this example, the mean value of the 'value' property in the array of objects is calculated using _.meanBy()
.
🏆 Best Practices
When working with the _.meanBy()
method, consider the following best practices:
Understanding Iteratee Function:
Comprehend the role of the iteratee function in calculating the mean. The iteratee function defines the criterion based on which the mean is calculated, providing flexibility in the process.
example.jsCopiedconst dataSet = [ { sales: 100 }, { sales: 150 }, { sales: 200 }, ]; const meanSales = _.meanBy(dataSet, 'sales'); console.log(meanSales); // Output: 150
Handling Empty Arrays:
Account for scenarios where the input array might be empty. Implement appropriate checks to handle such cases and avoid potential errors.
example.jsCopiedconst emptyArray = []; const meanValue = _.meanBy(emptyArray, 'value'); console.log(meanValue); // Output: NaN (Not a Number)
Utilizing Custom Iteratee:
Leverage the flexibility of the iteratee function by customizing it according to your specific requirements. This allows you to calculate the mean based on complex conditions or calculations.
example.jsCopiedconst complexDataSet = [ { duration: { minutes: 30 } }, { duration: { minutes: 45 } }, { duration: { minutes: 60 } }, ]; const meanDuration = _.meanBy(complexDataSet, data => data.duration.minutes); console.log(meanDuration); // Output: 45
📚 Use Cases
Statistical Analysis:
Perform statistical analysis on datasets by using
_.meanBy()
to calculate the mean based on relevant properties. This is valuable for gaining insights into the central tendencies of your data.example.jsCopiedconst statisticalData = /* ...fetch data from a statistical study... */; const meanResults = _.meanBy(statisticalData, 'result'); console.log(meanResults);
Dynamic Data Filtering:
When working with dynamic datasets, use
_.meanBy()
to dynamically calculate the mean based on different criteria or properties, providing adaptability to changing requirements.example.jsCopiedconst dynamicData = /* ...fetch data with dynamic properties... */; const selectedProperty = /* ...user-selected property... */; const dynamicMean = _.meanBy(dynamicData, selectedProperty); console.log(dynamicMean);
Complex Calculations:
For scenarios requiring more complex calculations, utilize the custom iteratee function to calculate the mean based on intricate conditions or nested properties.
example.jsCopiedconst complexData = /* ...fetch data with complex structure... */; const meanComplexValue = _.meanBy(complexData, data => { // Perform complex calculation or condition return /* ...result of the complex calculation... */; }); console.log(meanComplexValue);
🎉 Conclusion
The _.meanBy()
method in Lodash serves as a versatile tool for calculating the mean of an array based on specified criteria. Whether you're dealing with simple numeric arrays or complex datasets, this method offers flexibility and efficiency in your mathematical operations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.meanBy()
method in your Lodash projects.
👨💻 Join our Community:
Author
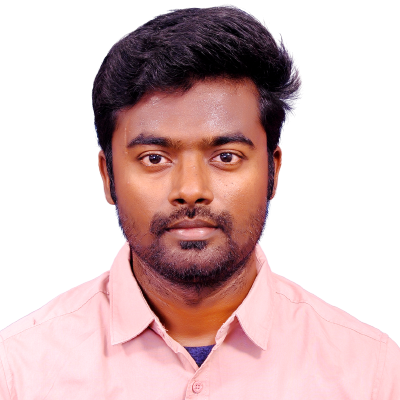
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
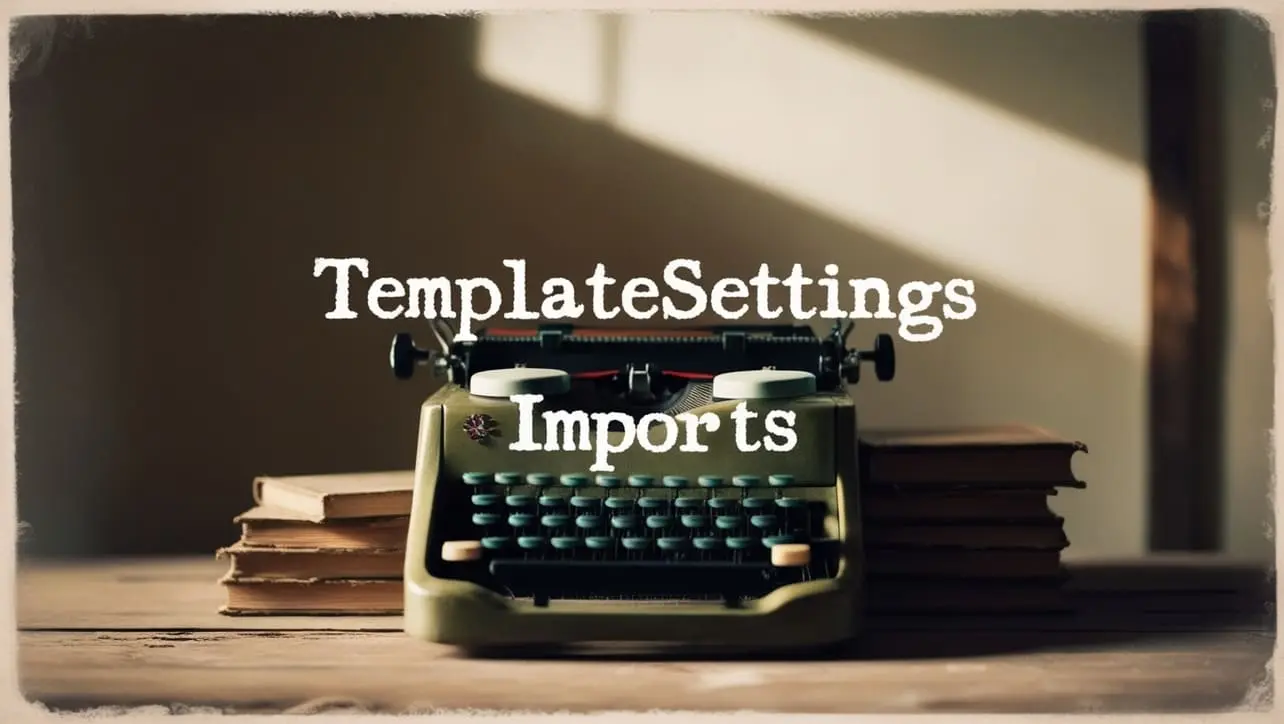
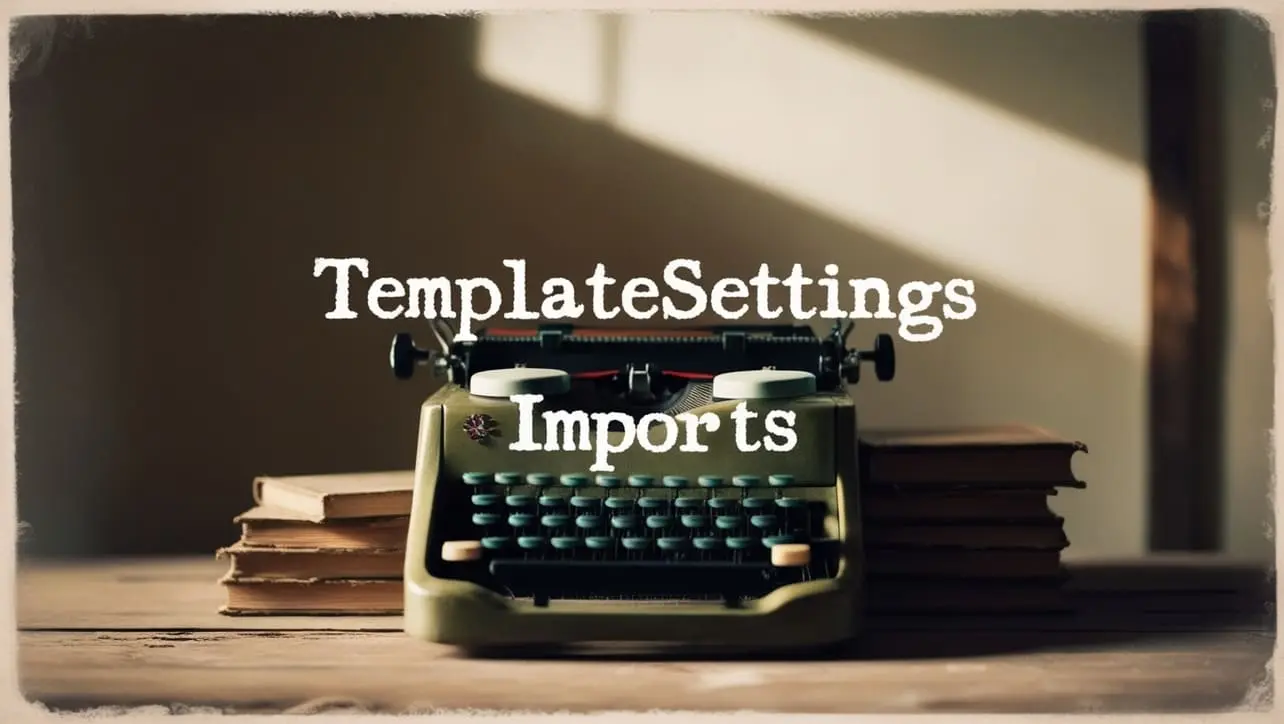
Lodash _.templateSettings.imports Property
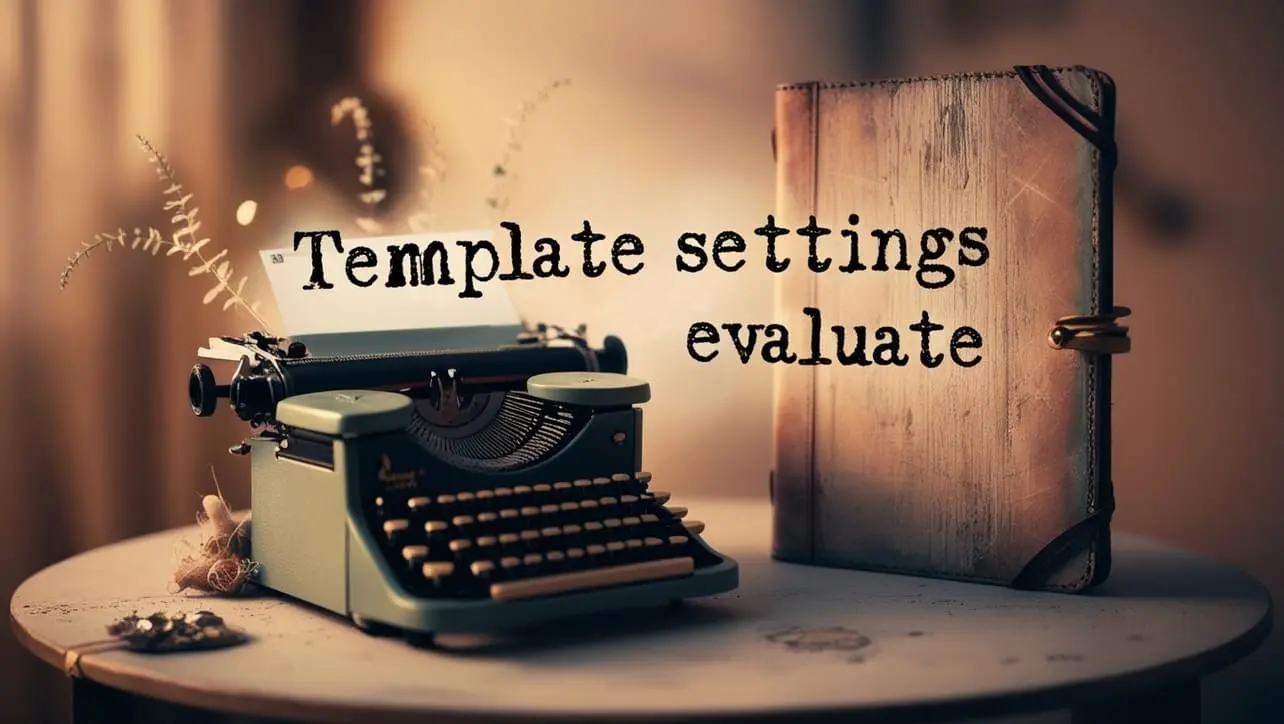
Lodash _.templateSettings.evaluate Property
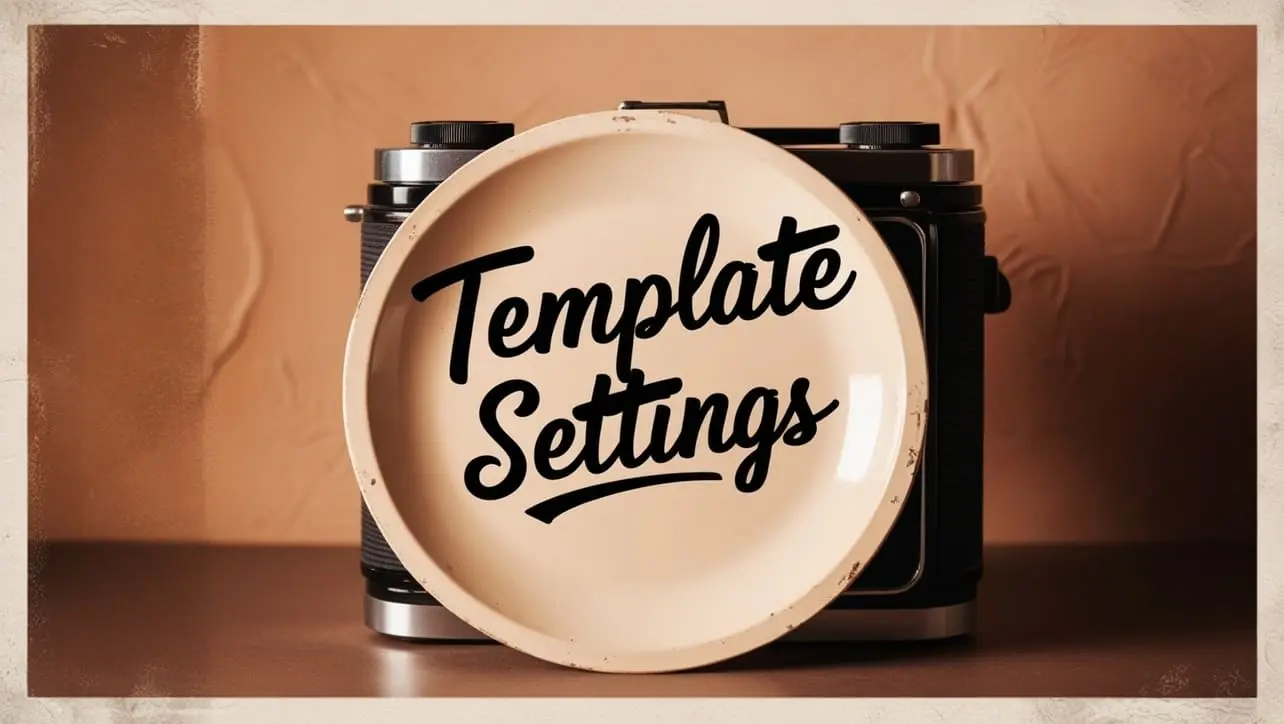
Lodash _.templateSettings Property
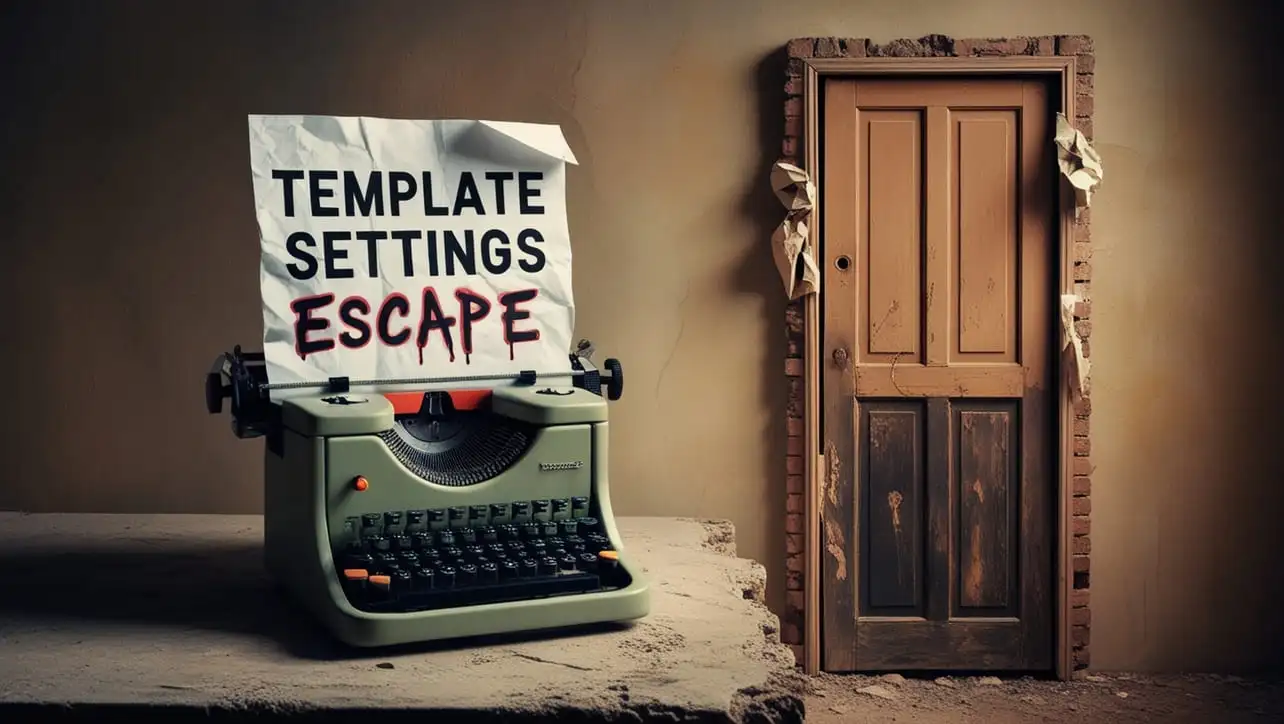
Lodash _.templateSettings.escape Property
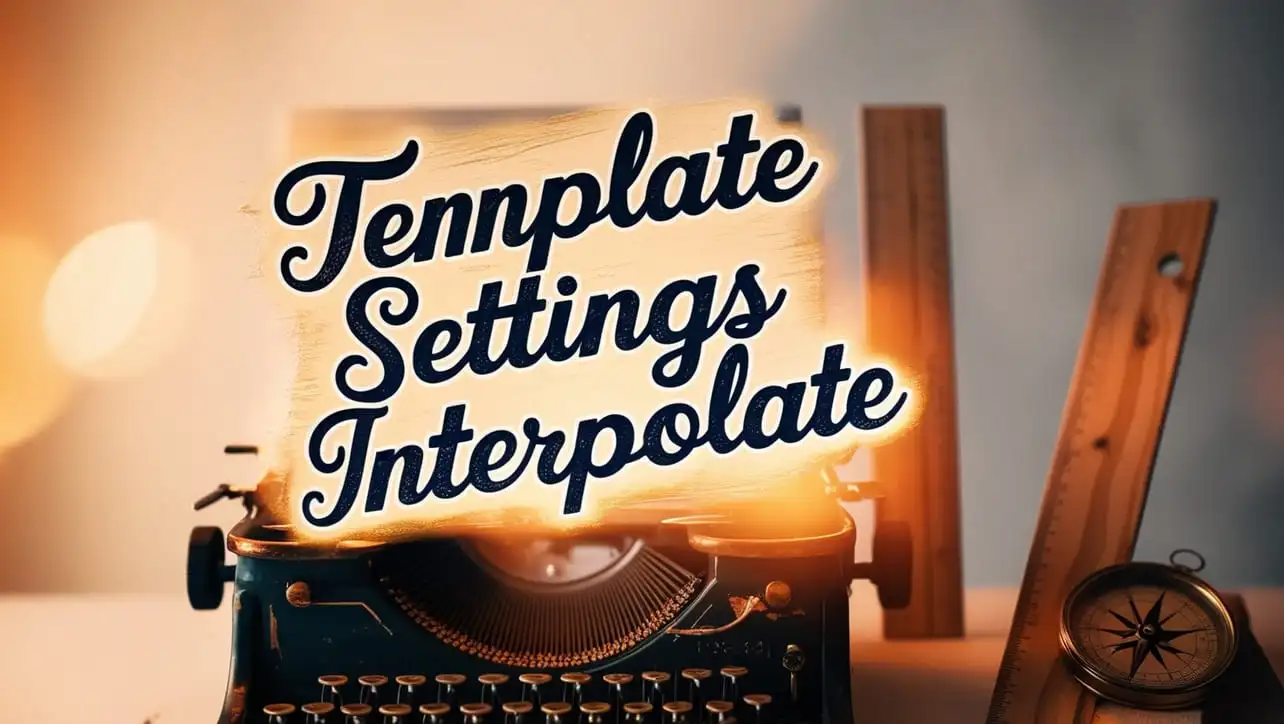
Lodash _.templateSettings.interpolate Property
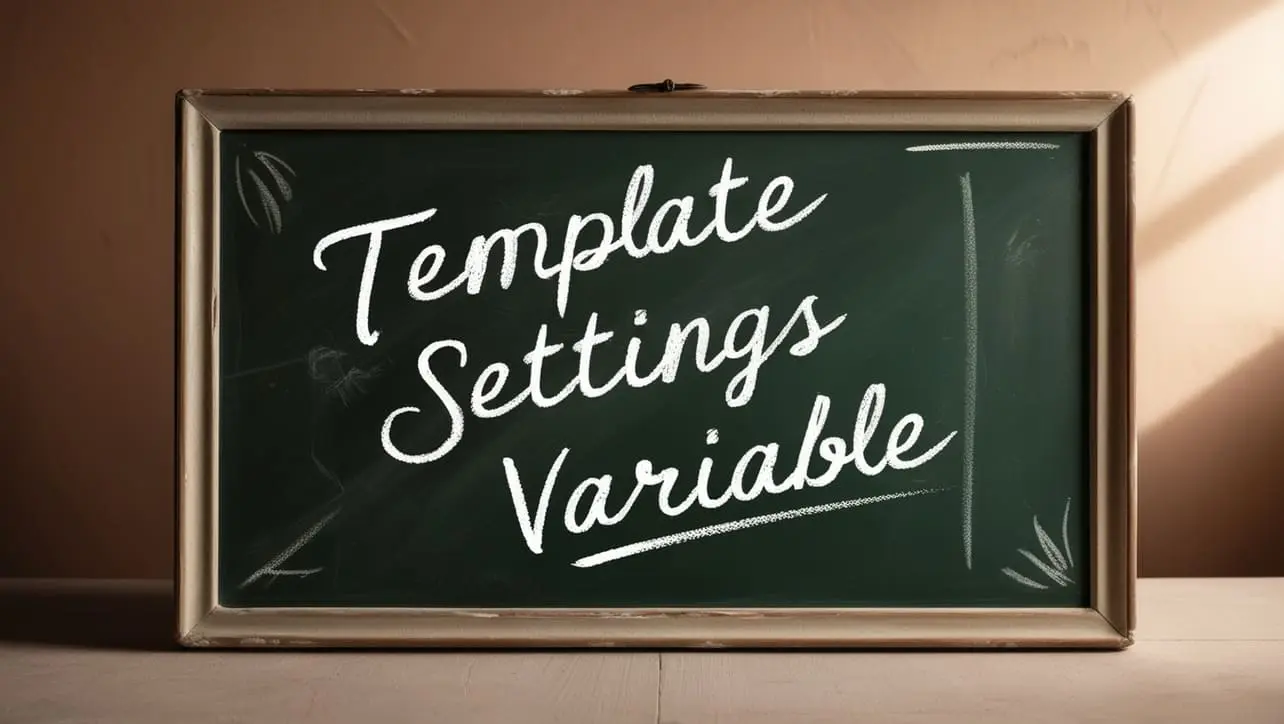
If you have any doubts regarding this article (Lodash _.meanBy() Math Method), please comment here. I will help you immediately.