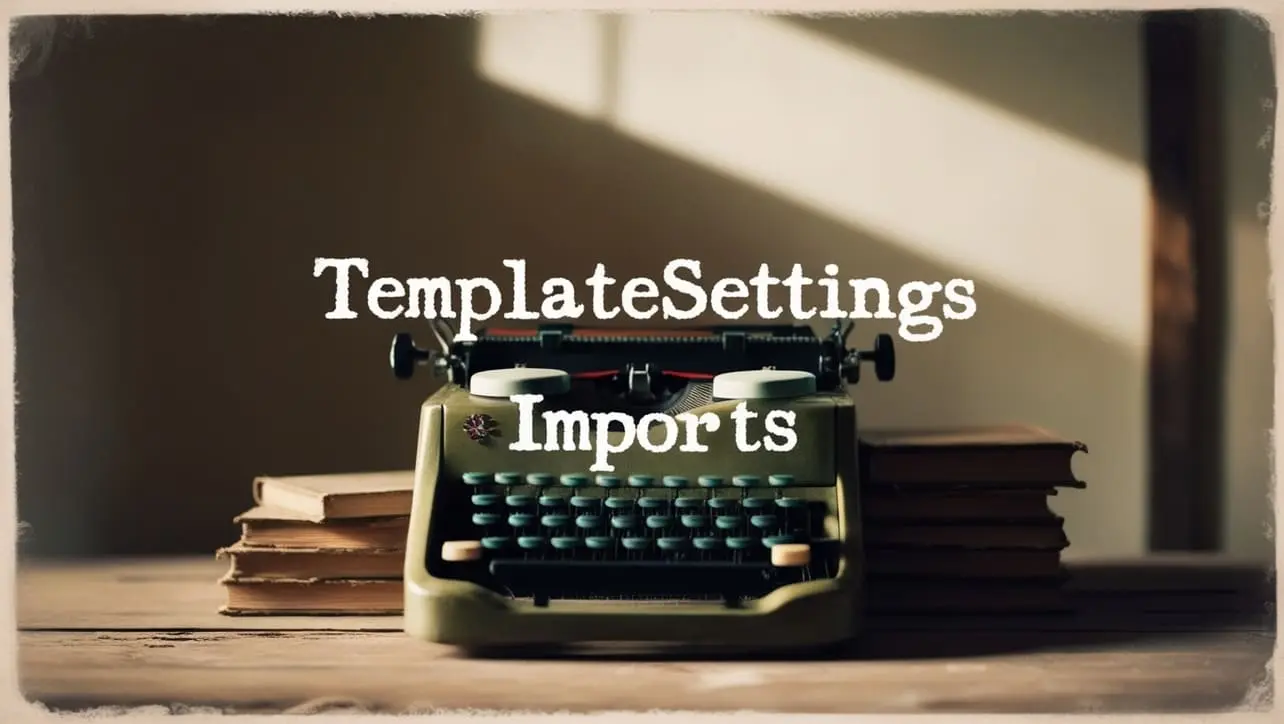
Lodash _.add() Math Method
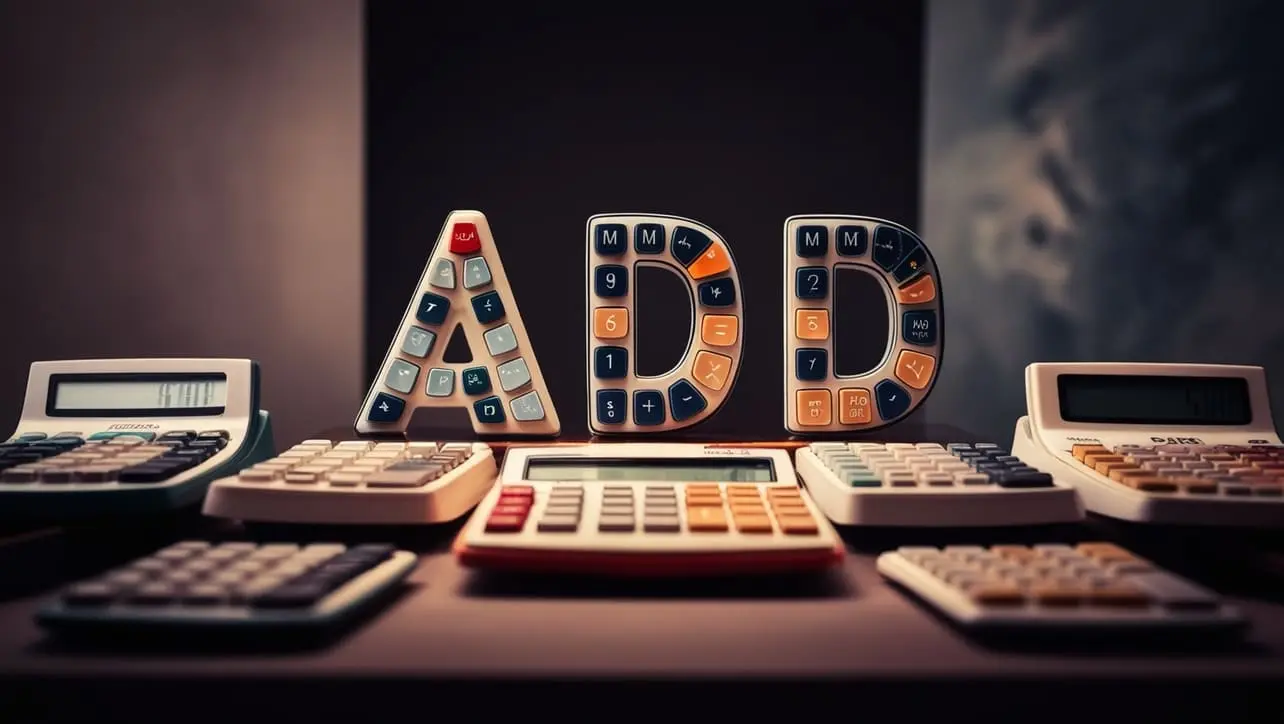
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript, performing mathematical operations is a fundamental aspect of programming. Lodash, a comprehensive utility library, provides a range of functions to simplify everyday coding tasks. Among these functions is the _.add()
method, a powerful tool for adding two numbers with precision and additional features.
This method enhances the clarity of mathematical operations in your JavaScript code.
🧠 Understanding _.add() Method
The _.add()
method in Lodash is designed to add two numbers accurately and handle potential floating-point precision issues. Additionally, it accommodates scenarios where one or both of the values might be undefined, providing a robust solution for arithmetic operations in a variety of situations.
💡 Syntax
The syntax for the _.add()
method is straightforward:
_.add(augend, addend)
- augend: The first number to be added.
- addend: The second number to be added.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.add()
method:
const _ = require('lodash');
const result = _.add(3, 4);
console.log(result);
// Output: 7
In this example, _.add()
takes two numbers, 3 and 4, and returns their sum.
🏆 Best Practices
When working with the _.add()
method, consider the following best practices:
Handling Undefined Values:
Use
_.add()
when dealing with situations where one or both of the values might be undefined. This method gracefully handles such cases and provides a meaningful result.example.jsCopiedconst undefinedValue = undefined; const fixedValue = 5; const sum = _.add(undefinedValue, fixedValue); console.log(sum); // Output: 5
Floating-Point Precision:
Leverage
_.add()
to mitigate floating-point precision issues that can arise during standard addition operations in JavaScript.example.jsCopiedconst standardSum = 0.1 + 0.2; // Result: 0.30000000000000004 const lodashSum = _.add(0.1, 0.2); // Result: 0.3 console.log(standardSum); console.log(lodashSum);
Ensuring Numerical Values:
Before using
_.add()
, ensure that both values are valid numbers to avoid unexpected behavior.example.jsCopiedconst validNumber = 10; const nonNumber = 'abc'; const sum = _.add(validNumber, nonNumber); console.log(sum); // Output: NaN
📚 Use Cases
Arithmetic Operations:
_.add()
is a reliable choice for basic arithmetic operations, providing accurate results and handling edge cases.example.jsCopiedconst result = _.add(8, 12); console.log(result); // Output: 20
Conditional Addition:
When dealing with values that might be undefined or need to be conditionally added,
_.add()
simplifies the logic.example.jsCopiedconst value = /* ...some condition... ? 5 : undefined ... */; const total = _.add(value, 10); console.log(total);
Precision-Critical Calculations:
In scenarios where precision is crucial, such as financial calculations,
_.add()
helps maintain accuracy.example.jsCopiedconst amount1 = 0.1; const amount2 = 0.2; const totalAmount = _.add(amount1, amount2); console.log(totalAmount); // Output: 0.3
🎉 Conclusion
The _.add()
method in Lodash is a valuable tool for performing addition operations in JavaScript with precision and versatility. Whether you're adding numbers, handling undefined values, or ensuring floating-point accuracy, this method provides a reliable solution for various mathematical scenarios.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.add()
method in your Lodash projects.
👨💻 Join our Community:
Author
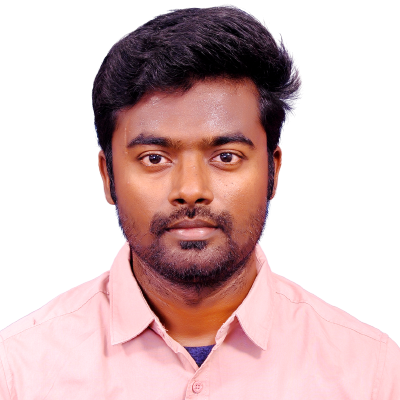
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
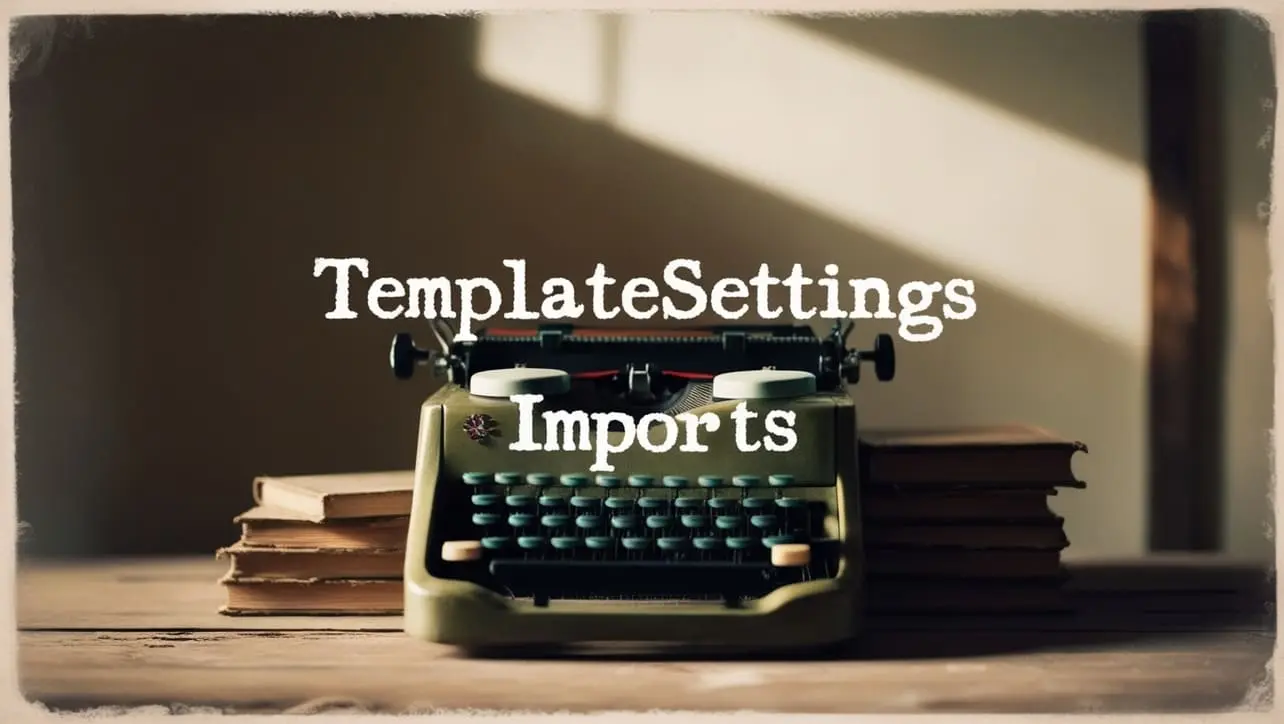
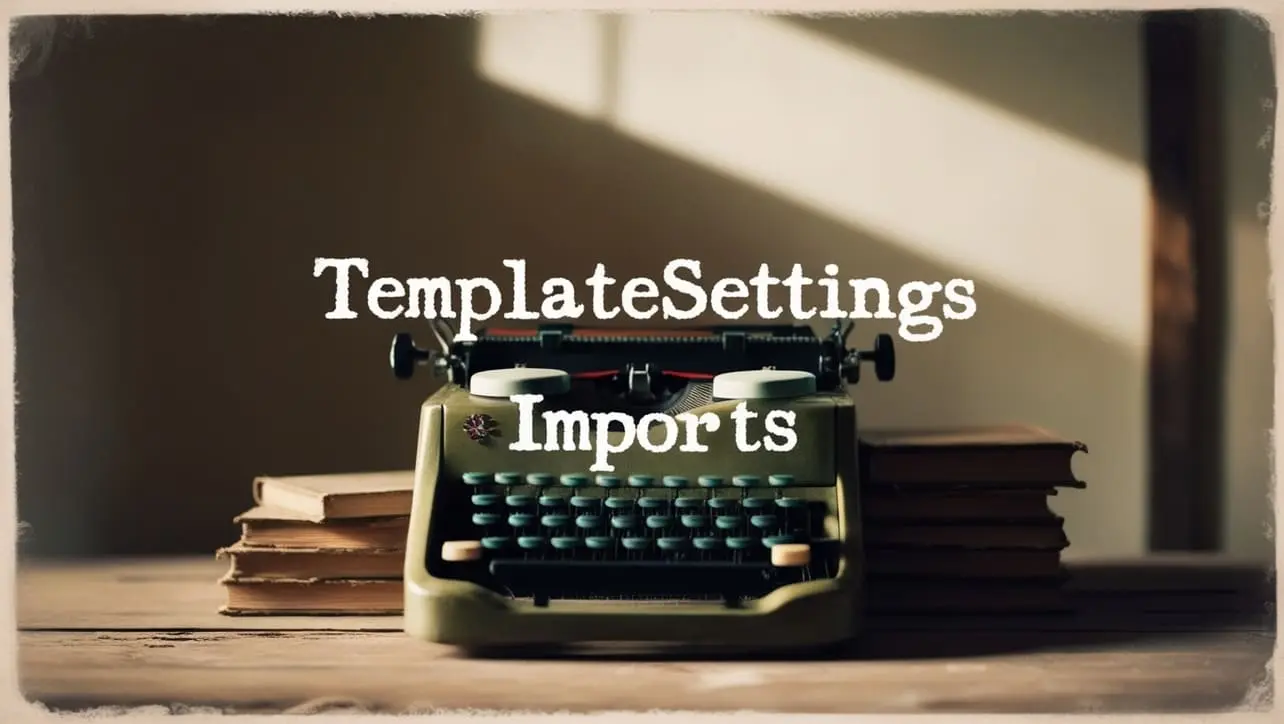
Lodash _.templateSettings.imports Property
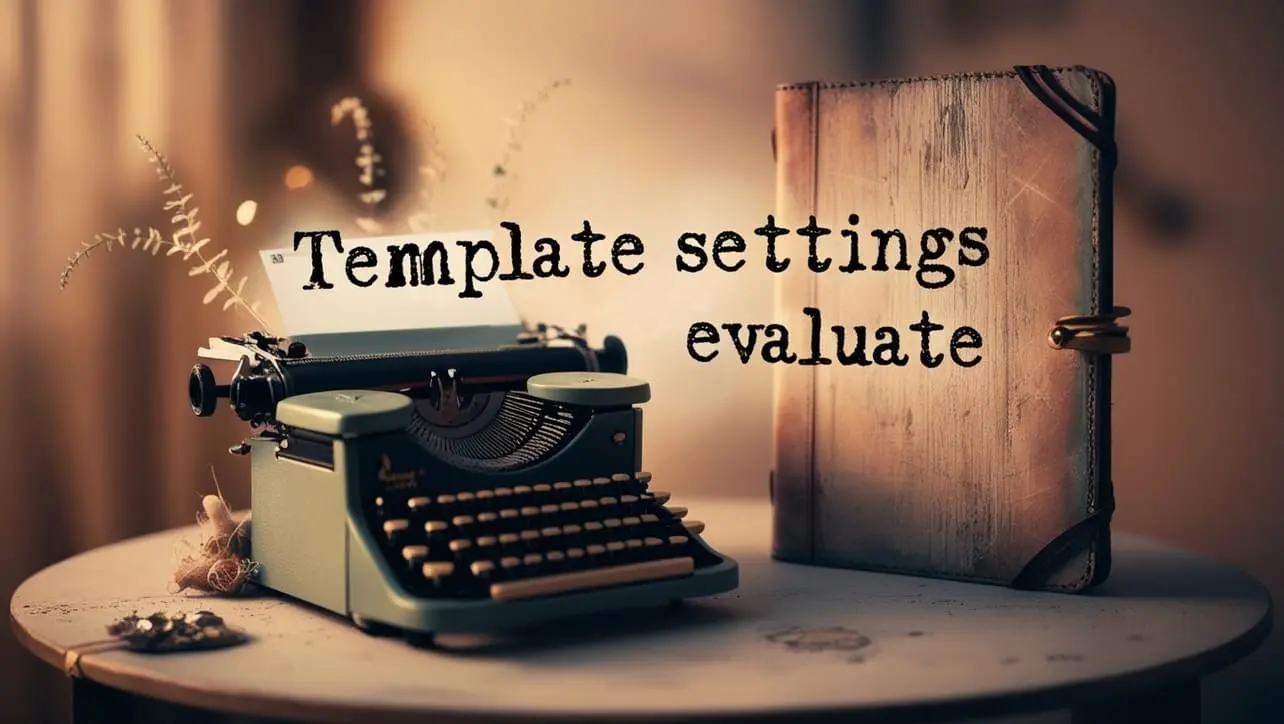
Lodash _.templateSettings.evaluate Property
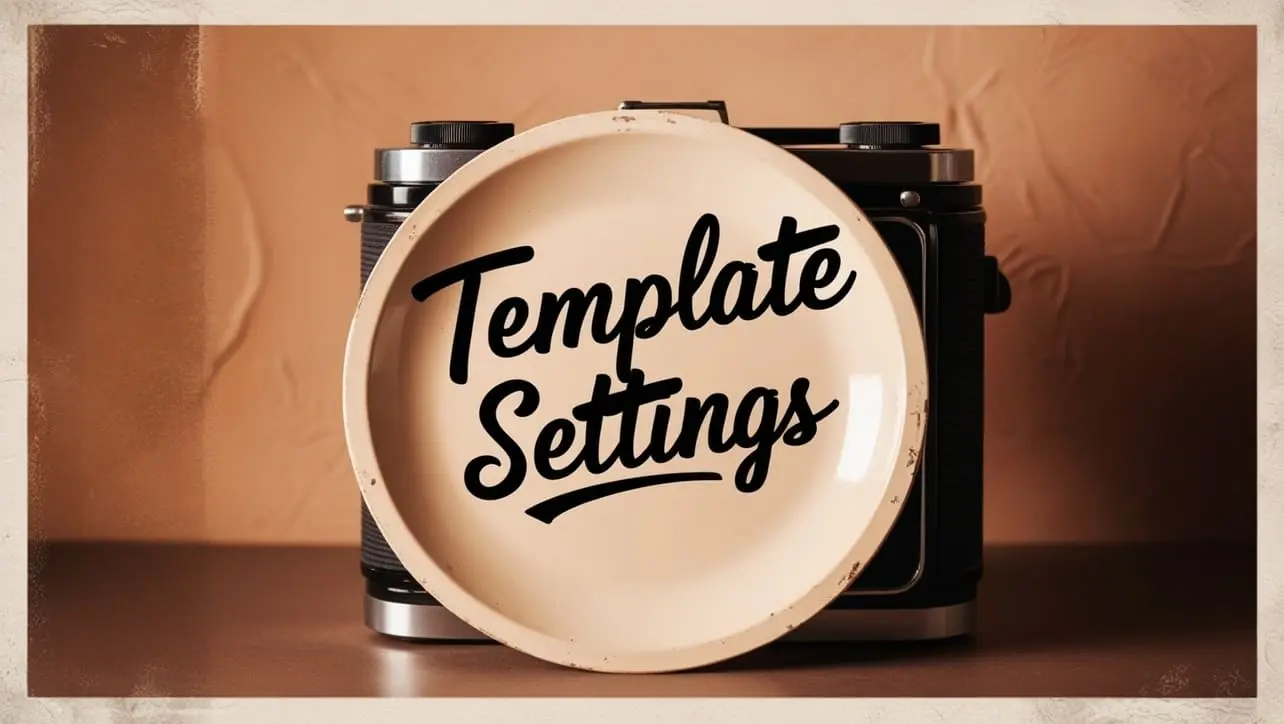
Lodash _.templateSettings Property
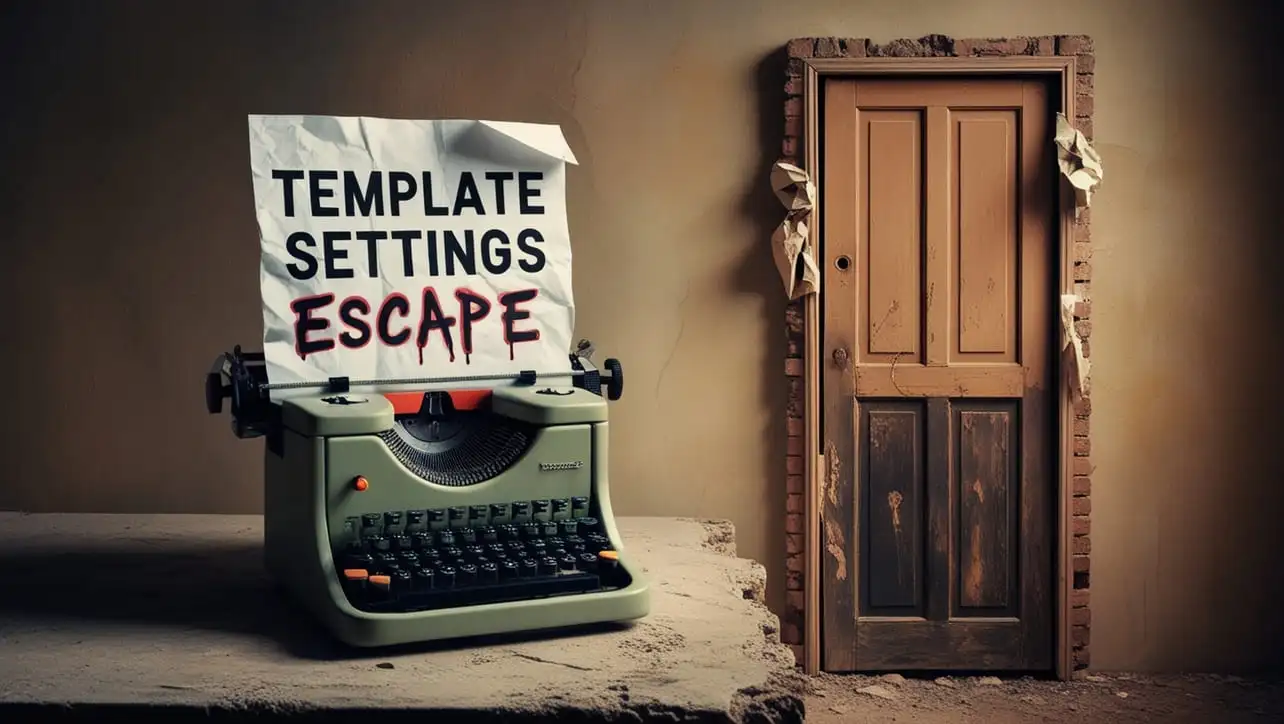
Lodash _.templateSettings.escape Property
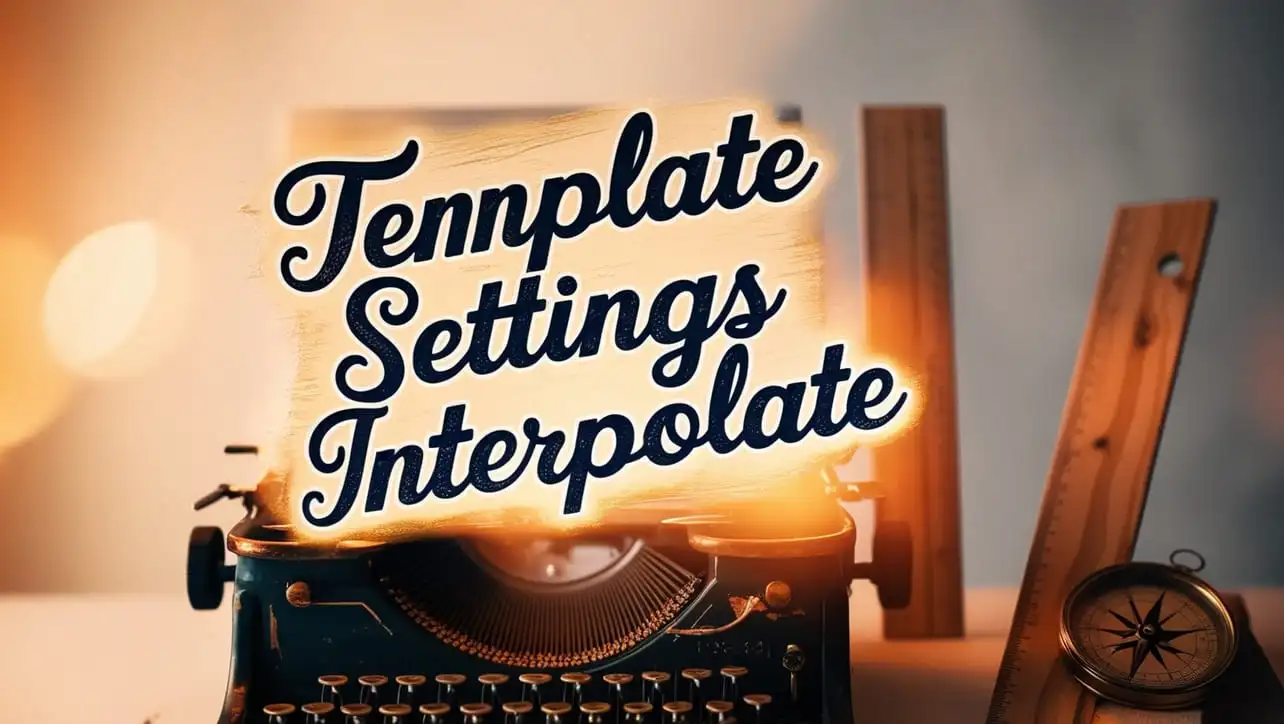
Lodash _.templateSettings.interpolate Property
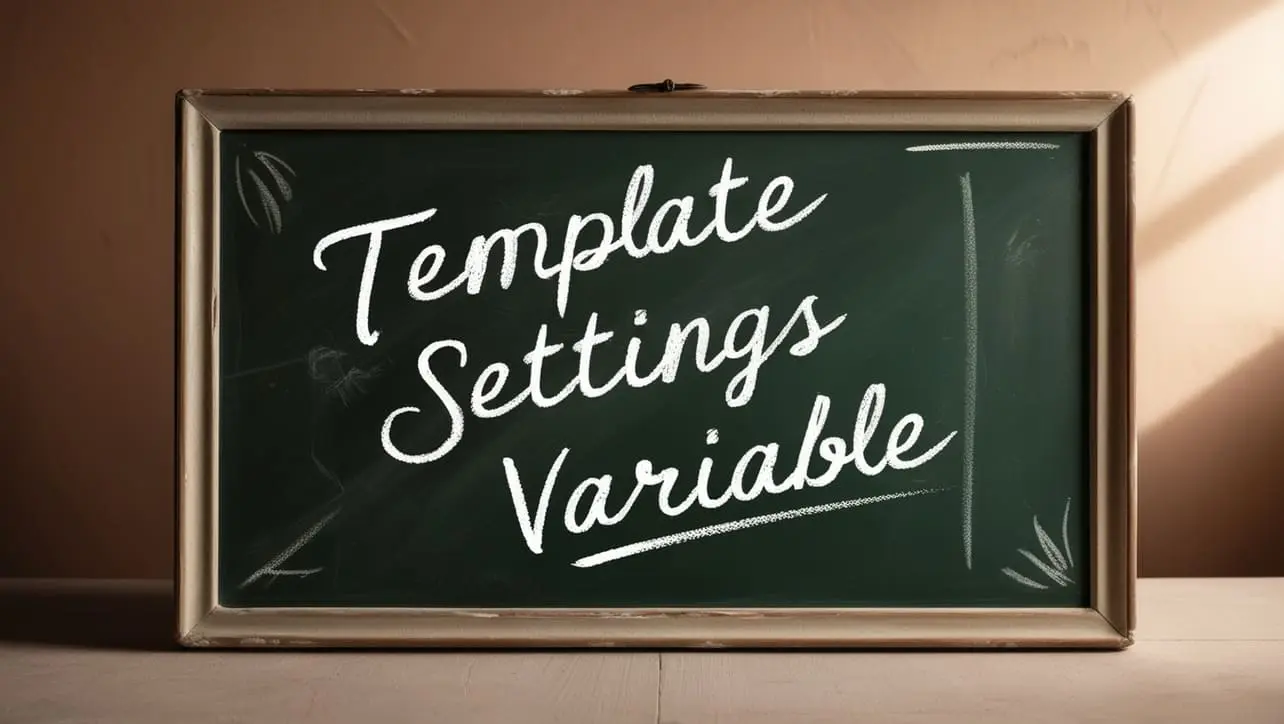
If you have any doubts regarding this article (Lodash _.add() Math Method), please comment here. I will help you immediately.