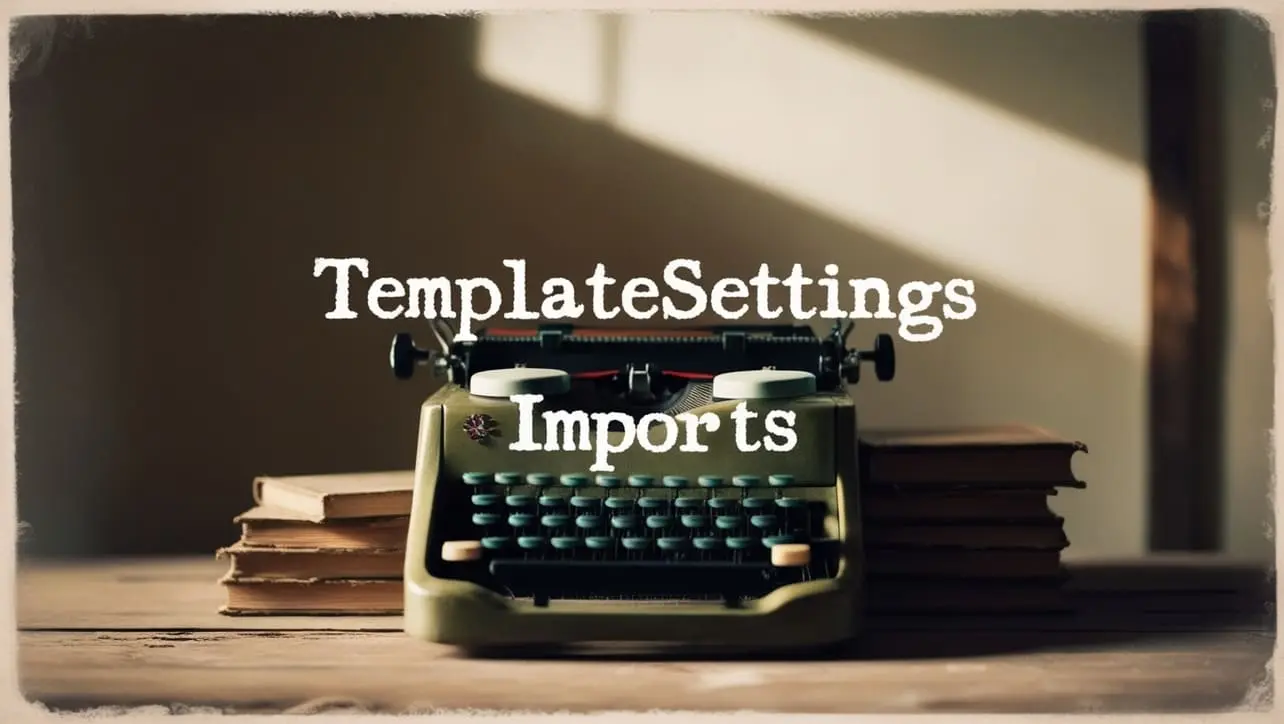
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toPlainObject() Lang Method
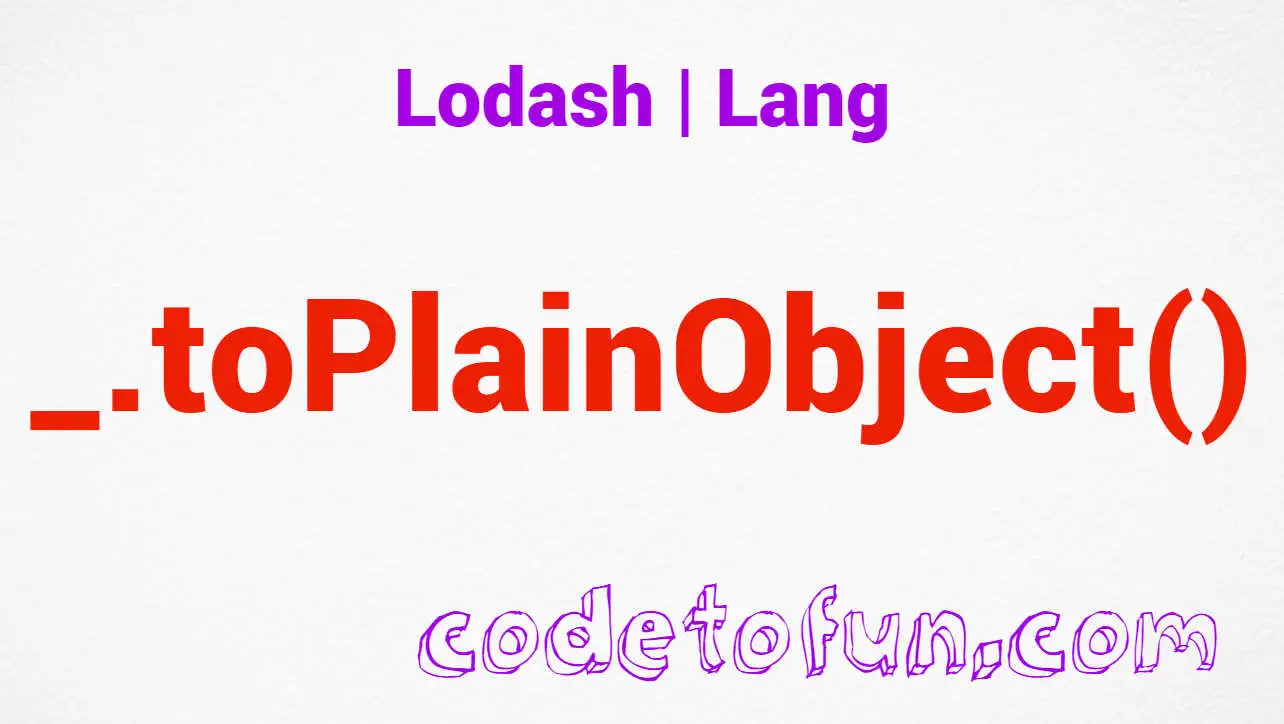
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, Lodash stands out as a comprehensive utility library, offering a myriad of functions to simplify and enhance everyday coding tasks. Among its versatile set of methods is _.toPlainObject()
, a utility in the "Lang" category.
This method serves to transform values into plain objects, providing a straightforward approach to object creation and manipulation.
🧠 Understanding _.toPlainObject() Method
The _.toPlainObject()
method in Lodash is designed to convert values into plain objects. Whether dealing with primitive values, arrays, or objects with prototype chains, this method ensures that the result is a plain, vanilla JavaScript object, free from any inherited properties.
💡 Syntax
The syntax for the _.toPlainObject()
method is straightforward:
_.toPlainObject(value)
- value: The value to convert to a plain object.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toPlainObject()
method:
const _ = require('lodash');
const complexValue = {
prop1: 'Hello',
prop2: ['world'],
prop3: { nested: 'objects' },
};
const plainObject = _.toPlainObject(complexValue);
console.log(plainObject);
// Output: { prop1: 'Hello', prop2: ['world'], prop3: { nested: 'objects' } }
In this example, the complexValue object, which includes an array and a nested object, is transformed into a plain object using _.toPlainObject()
.
🏆 Best Practices
When working with the _.toPlainObject()
method, consider the following best practices:
Consistent Object Transformation:
Use
_.toPlainObject()
to ensure consistency when transforming values into objects. Whether the input is a primitive, array, or object, this method guarantees that the result is always a plain object.example.jsCopiedconst stringValue = 'Hello, Lodash!'; const arrayValue = [1, 2, 3]; const plainStringObject = _.toPlainObject(stringValue); const plainArrayObject = _.toPlainObject(arrayValue); console.log(plainStringObject); // Output: { '0': 'H', '1': 'e', '2': 'l', '3': 'l', '4': 'o', '5': ',', '6': ' ', '7': 'L', '8': 'o', '9': 'd', '10': 'a', '11': 's', '12': 'h', '13': '!', length: 14 } console.log(plainArrayObject); // Output: { '0': 1, '1': 2, '2': 3, length: 3 }
Handling Prototype Chains:
_.toPlainObject()
is particularly useful when dealing with objects that have prototype chains. It creates a clean, prototype-free object, avoiding any unintended inheritance.example.jsCopiedfunction CustomObject() { this.customProp = 'I am custom!'; } CustomObject.prototype.additionalProp = 'I should not be here!'; const customInstance = new CustomObject(); const plainCustomObject = _.toPlainObject(customInstance); console.log(plainCustomObject); // Output: { customProp: 'I am custom!' }
Transforming Values with Prototype Properties:
When working with values that have prototype properties, use
_.toPlainObject()
to create a new object without inherited properties.example.jsCopiedconst objectWithPrototype = Object.create({ prototypeProp: 'Inherited property' }); objectWithPrototype.ownProp = 'Own property'; const plainObjectWithoutPrototype = _.toPlainObject(objectWithPrototype); console.log(plainObjectWithoutPrototype); // Output: { ownProp: 'Own property' }
📚 Use Cases
Object Transformation:
_.toPlainObject()
is invaluable for consistent object transformation, ensuring that any input value is converted into a plain object.example.jsCopiedconst someValue = /* ...retrieve a value dynamically... */; const plainObjectValue = _.toPlainObject(someValue); console.log(plainObjectValue);
Simplifying Object Copying:
When copying objects and desiring a clean, prototype-free result,
_.toPlainObject()
can be employed to simplify the copying process.example.jsCopiedconst originalObject = { key: 'value', nested: { innerKey: 'innerValue' } }; const copiedObject = _.toPlainObject(originalObject); console.log(copiedObject); // Output: { key: 'value', nested: { innerKey: 'innerValue' } }
Clean Object Creation:
For scenarios where a new, clean object is needed,
_.toPlainObject()
ensures that the result is void of any prototype chain or inherited properties.example.jsCopiedconst emptyObject = _.toPlainObject(null); console.log(emptyObject); // Output: {}
🎉 Conclusion
The _.toPlainObject()
method in Lodash is a versatile utility in the "Lang" category, providing a reliable means to transform values into plain, prototype-free objects. Whether dealing with inconsistent input types or aiming for clean object creation, this method simplifies object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toPlainObject()
method in your Lodash projects.
👨💻 Join our Community:
Author
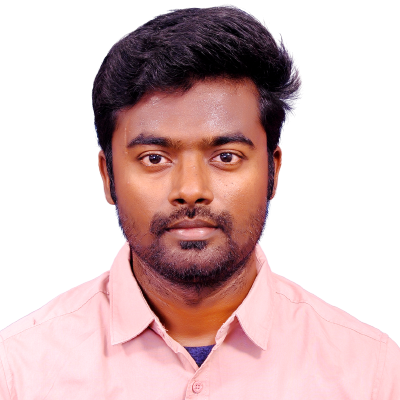
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
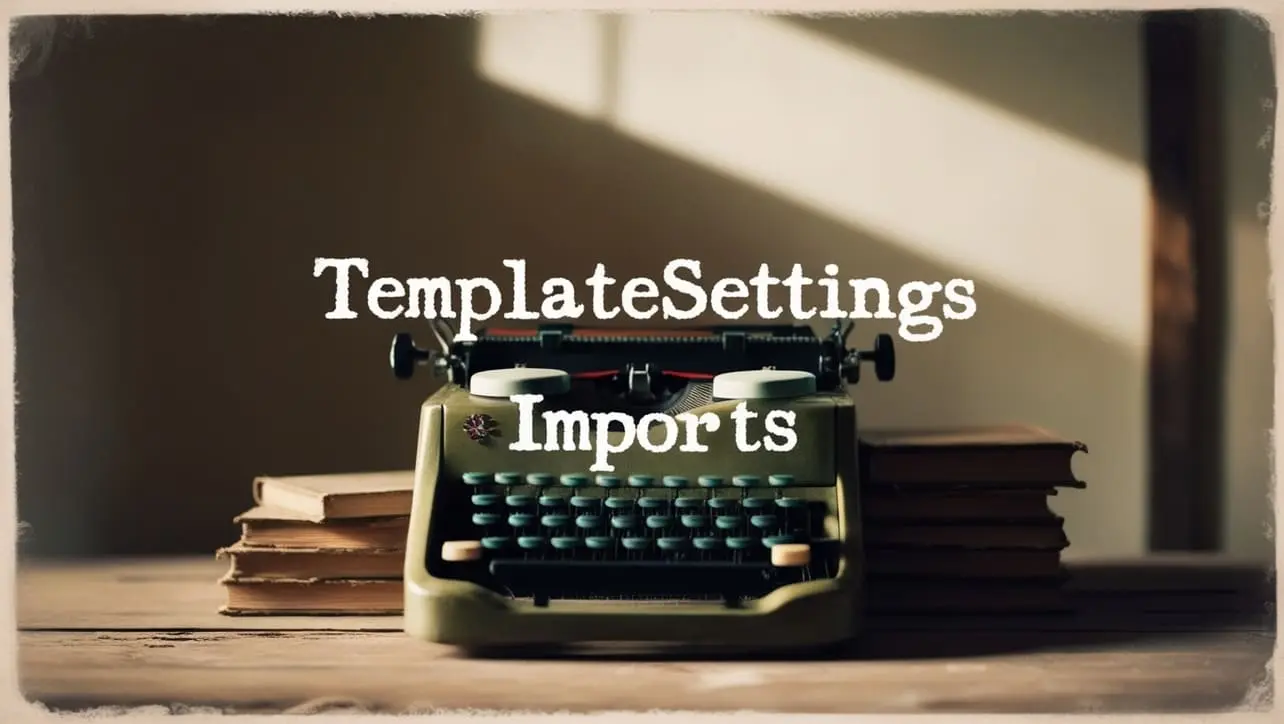
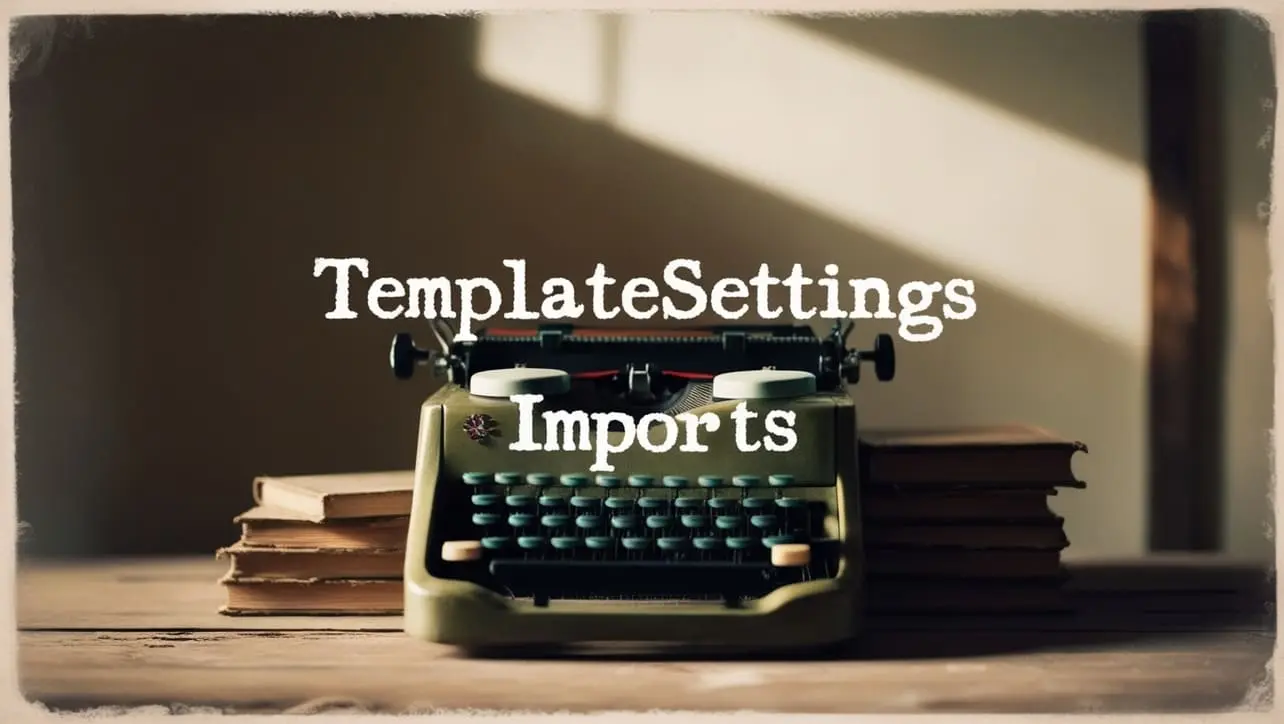
Lodash _.templateSettings.imports Property
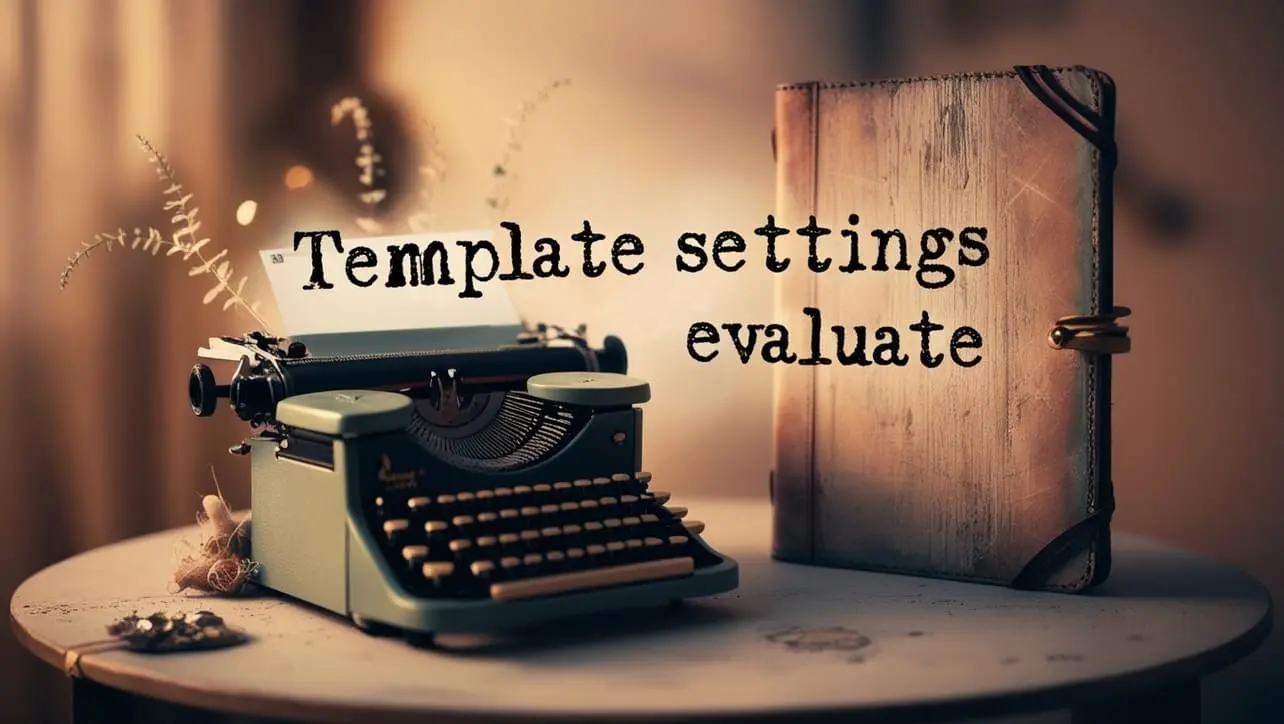
Lodash _.templateSettings.evaluate Property
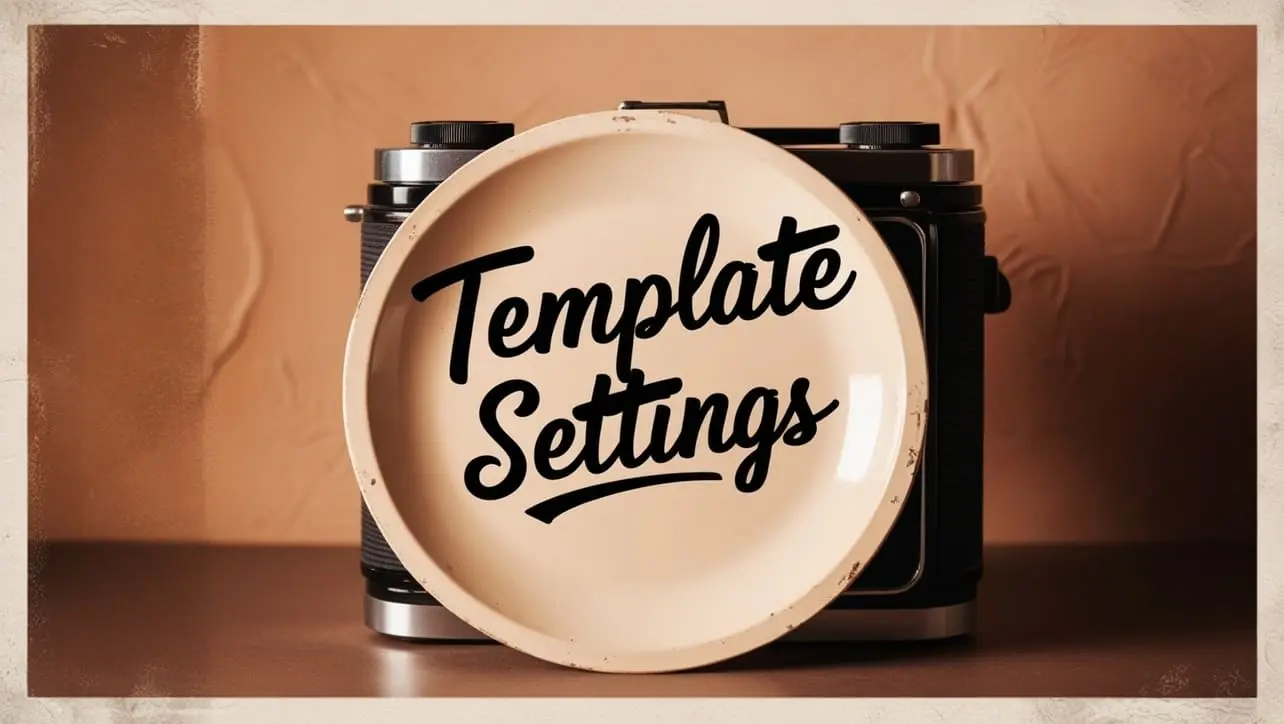
Lodash _.templateSettings Property
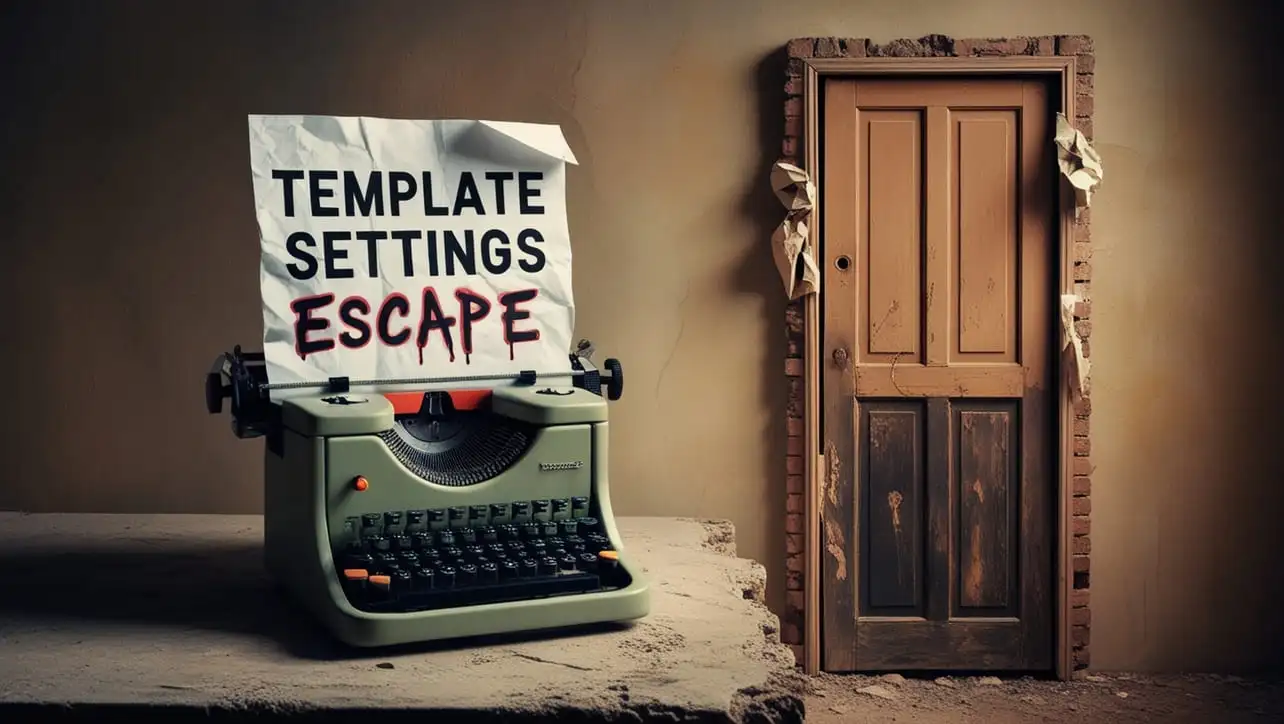
Lodash _.templateSettings.escape Property
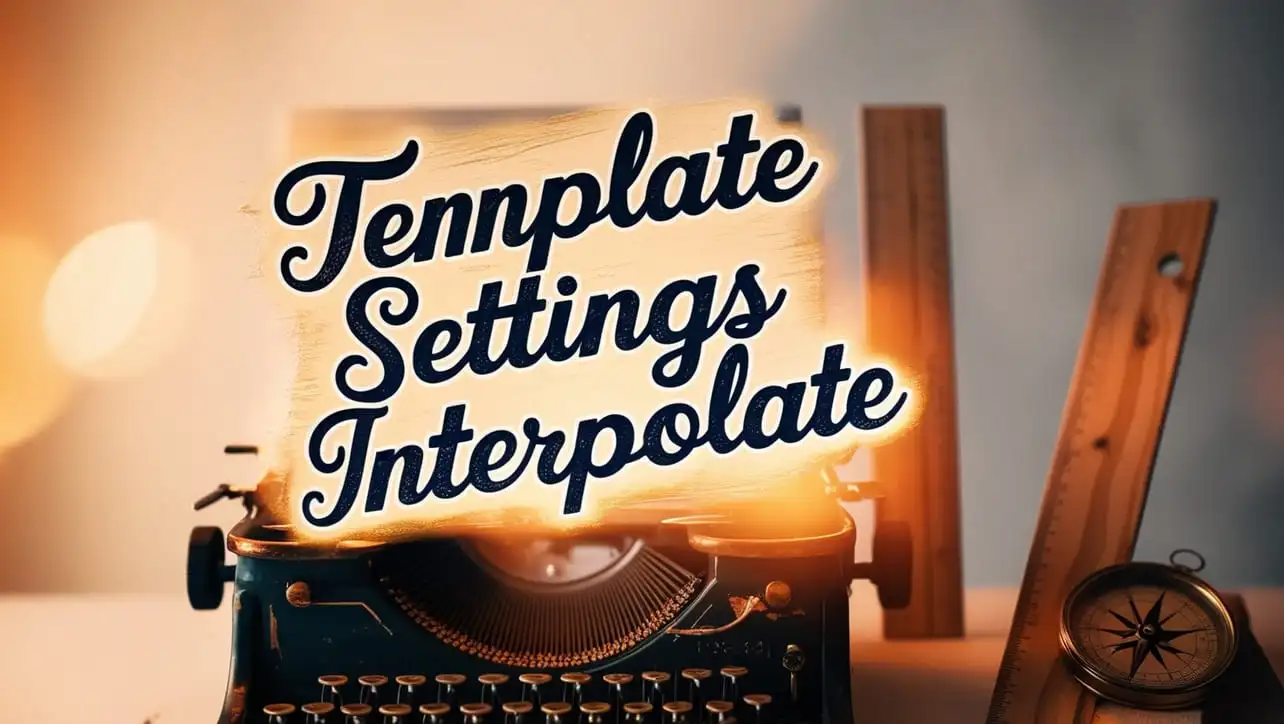
Lodash _.templateSettings.interpolate Property
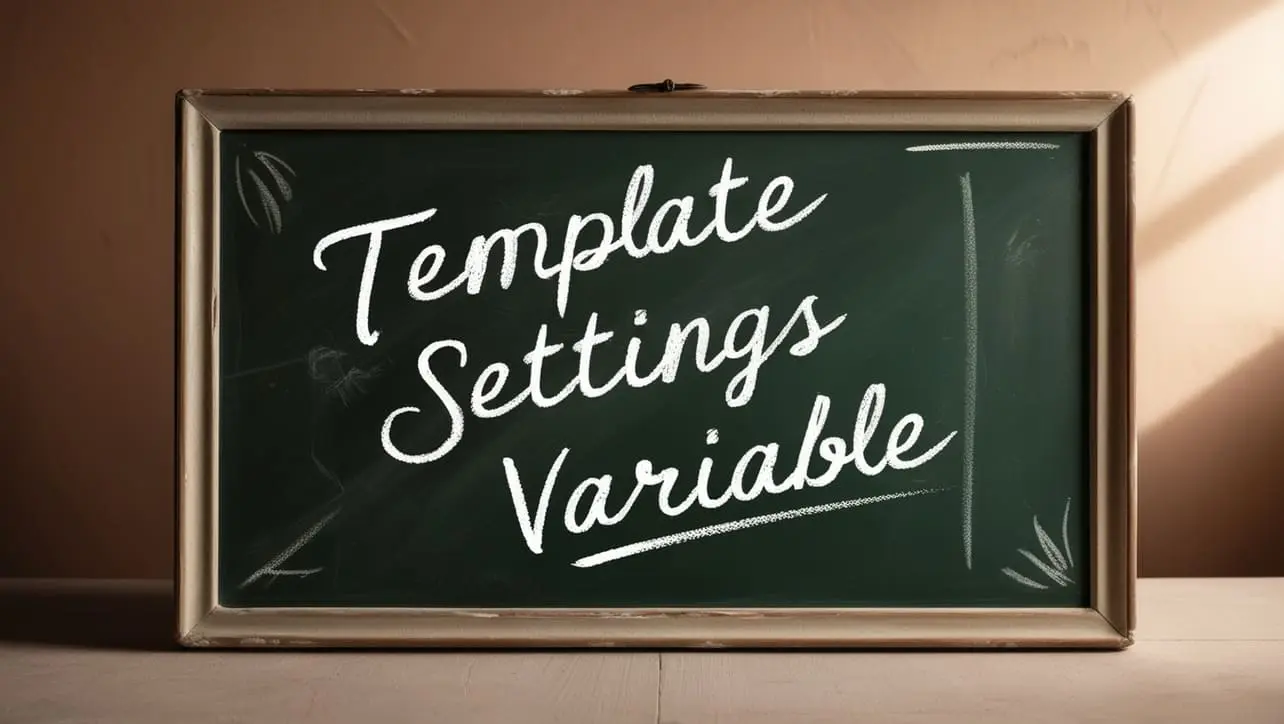
If you have any doubts regarding this article (Lodash _.toPlainObject() Lang Method), please comment here. I will help you immediately.