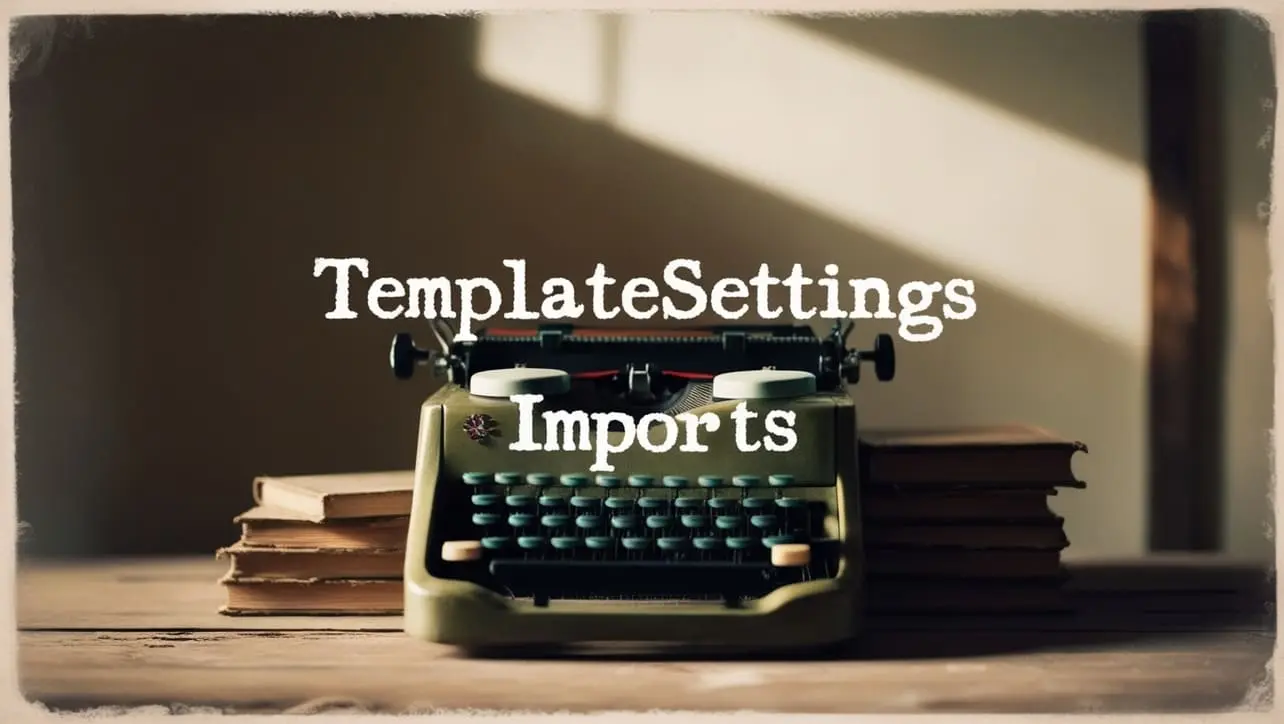
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isString() Lang Method
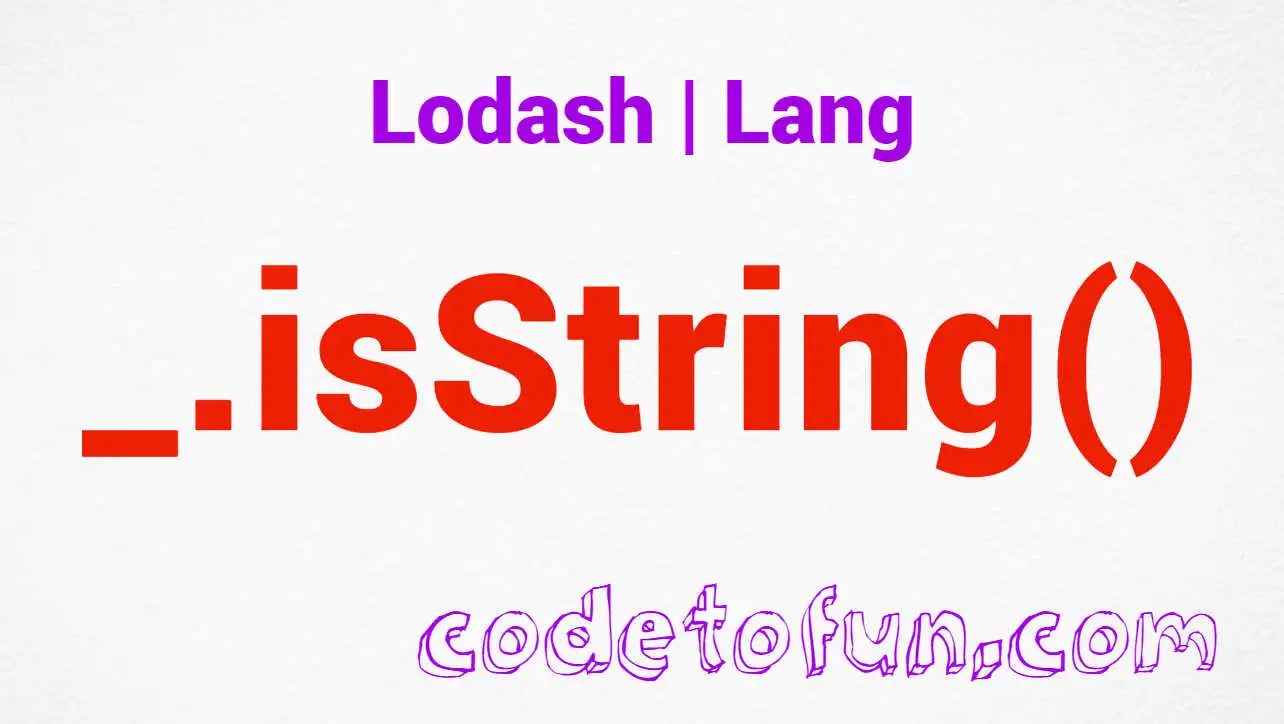
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic world of JavaScript, determining the type of a variable is a common task. Lodash, a powerful utility library, provides a variety of functions to simplify such operations. One of these functions is _.isString()
, a method designed to check whether a given value is of the string data type.
This straightforward yet essential method is a valuable tool for developers ensuring the integrity of their data and enhancing code reliability.
🧠 Understanding _.isString() Method
The _.isString()
method in Lodash is a straightforward utility to identify if a value is of the string type. This can be particularly useful in scenarios where you need to validate user inputs, handle different data types, or conditionally execute code based on the type of a variable.
💡 Syntax
The syntax for the _.isString()
method is straightforward:
_.isString(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isString()
method:
const _ = require('lodash');
const stringValue = 'Hello, Lodash!';
const numberValue = 42;
console.log(_.isString(stringValue)); // Output: true
console.log(_.isString(numberValue)); // Output: false
In this example, _.isString()
correctly identifies that stringValue is a string, while numberValue is not.
🏆 Best Practices
When working with the _.isString()
method, consider the following best practices:
Use in Input Validation:
Leverage
_.isString()
in input validation to ensure that user-provided values are of the expected string type.example.jsCopiedfunction validateUsername(username) { if (_.isString(username)) { console.log('Valid username:', username); } else { console.error('Invalid username. Please provide a string.'); } } validateUsername('JohnDoe'); // Output: Valid username: JohnDoe validateUsername(123); // Output: Invalid username. Please provide a string.
Conditionally Execute Code:
Use
_.isString()
to conditionally execute code based on the type of a variable, preventing unexpected behaviors.example.jsCopiedfunction processInput(input) { if (_.isString(input)) { console.log('Processing string input:', input.toUpperCase()); } else { console.error('Invalid input. Please provide a string.'); } } processInput('Hello'); // Output: Processing string input: HELLO processInput(42); // Output: Invalid input. Please provide a string.
Combine with Other Type Checks:
Combine
_.isString()
with other type-checking methods to create comprehensive validation logic.example.jsCopiedfunction validateUserData(user) { if (_.isObject(user) && _.isString(user.name) && _.isNumber(user.age)) { console.log('Valid user data:', user); } else { console.error('Invalid user data. Please provide a valid object with name as string and age as number.'); } } const validUser = { name: 'Alice', age: 30 }; const invalidUser = { name: 42, age: 'unknown' }; validateUserData(validUser); // Output: Valid user data: { name: 'Alice', age: 30 } validateUserData(invalidUser); // Output: Invalid user data. Please provide a valid object with name as string and age as number.
📚 Use Cases
Input Validation:
Use
_.isString()
to validate and sanitize user inputs, ensuring they meet the expected string format.example.jsCopiedfunction processInput(input) { if (_.isString(input)) { console.log('Processing string input:', input.trim()); } else { console.error('Invalid input. Please provide a string.'); } } processInput(' Hello '); // Output: Processing string input: Hello processInput(42); // Output: Invalid input. Please provide a string.
Type-Safe Function Arguments:
Ensure type safety when defining function arguments by using
_.isString()
to validate input parameters.example.jsCopiedfunction greet(name) { if (_.isString(name)) { console.log('Hello, ' + name + '!'); } else { console.error('Invalid input. Please provide a string as the name.'); } } greet('Alice'); // Output: Hello, Alice! greet(42); // Output: Invalid input. Please provide a string as the name.
Conditional Rendering in UI:
In user interfaces, use
_.isString()
to conditionally render components or handle data based on whether it's a string.example.jsCopiedfunction displayMessage(message) { if (_.isString(message)) { // Render UI component for displaying strings console.log('Displaying message:', message); } else { console.error('Invalid message. Please provide a string.'); } } displayMessage('Welcome!'); // Output: Displaying message: Welcome! displayMessage({ text: 'Hello' }); // Output: Invalid message. Please provide a string.
🎉 Conclusion
The _.isString()
method in Lodash is a handy utility for identifying string values, offering a simple yet effective way to enhance the reliability of your code. Whether you're validating user inputs, implementing type-safe functions, or handling diverse data types, _.isString()
proves to be a valuable tool in your JavaScript toolkit.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isString()
method in your Lodash projects.
👨💻 Join our Community:
Author
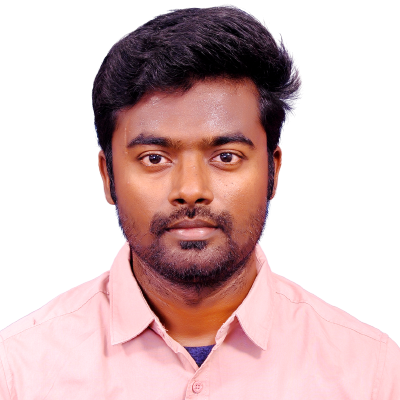
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
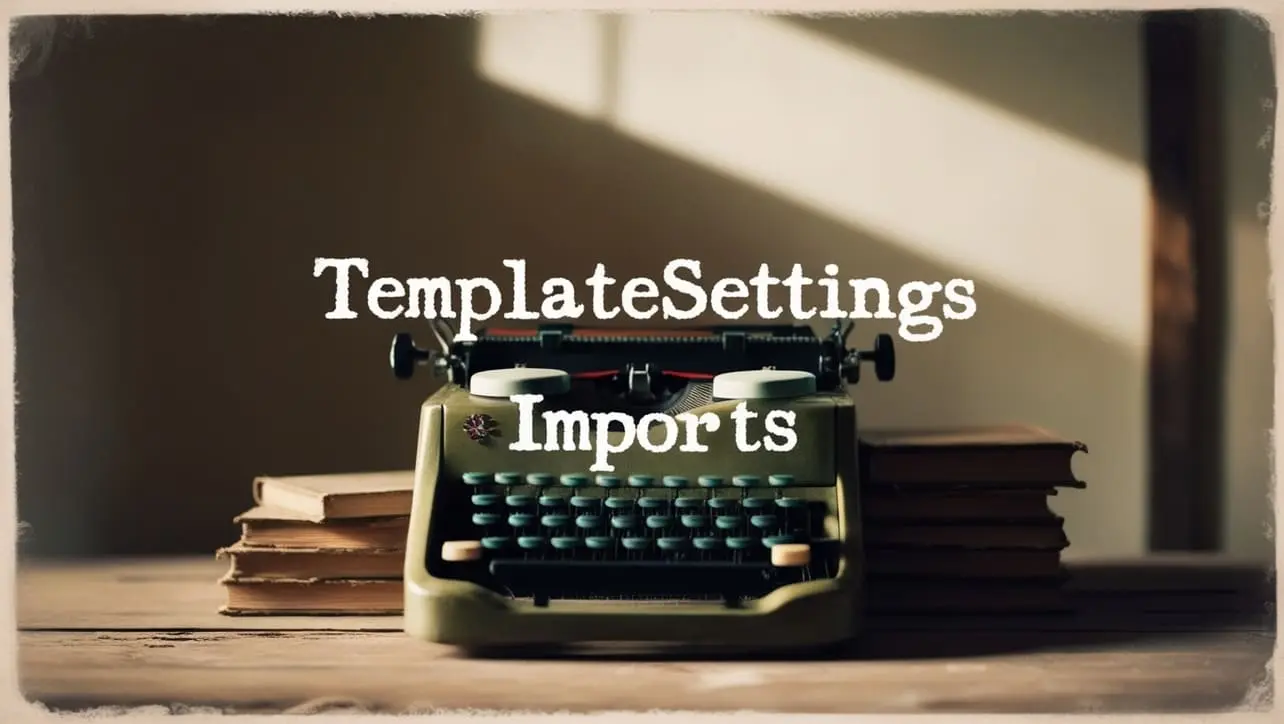
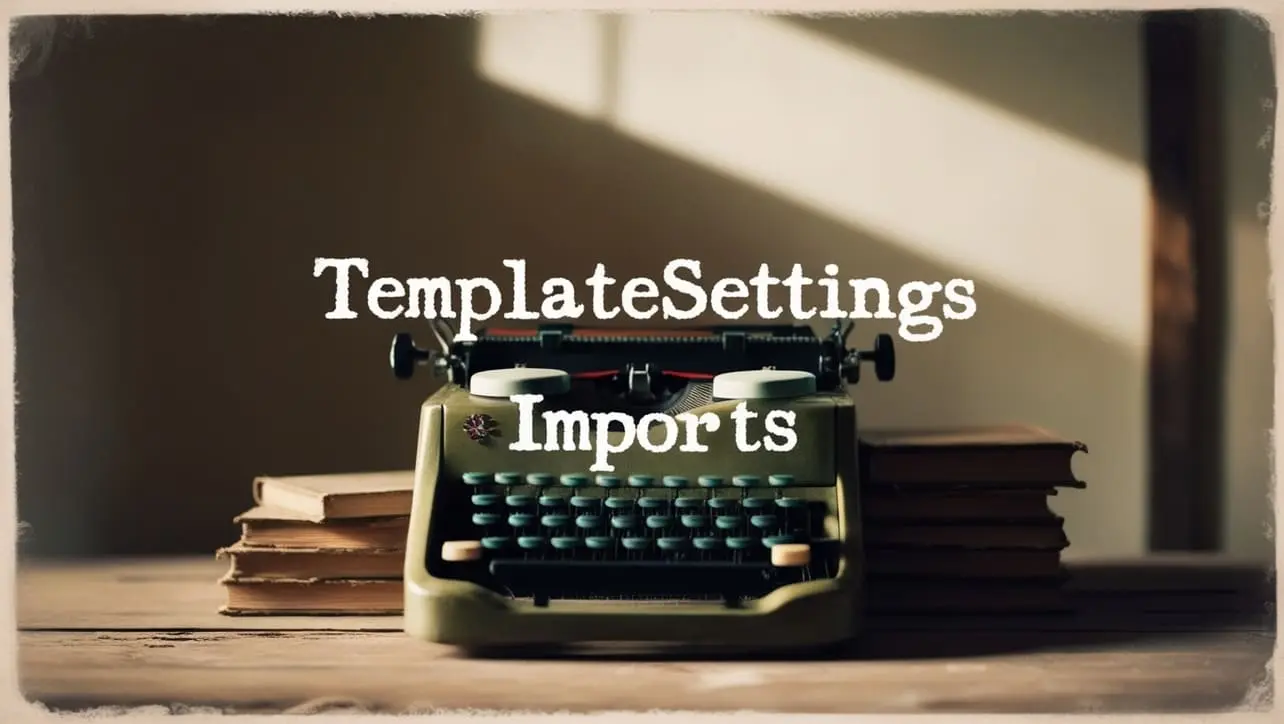
Lodash _.templateSettings.imports Property
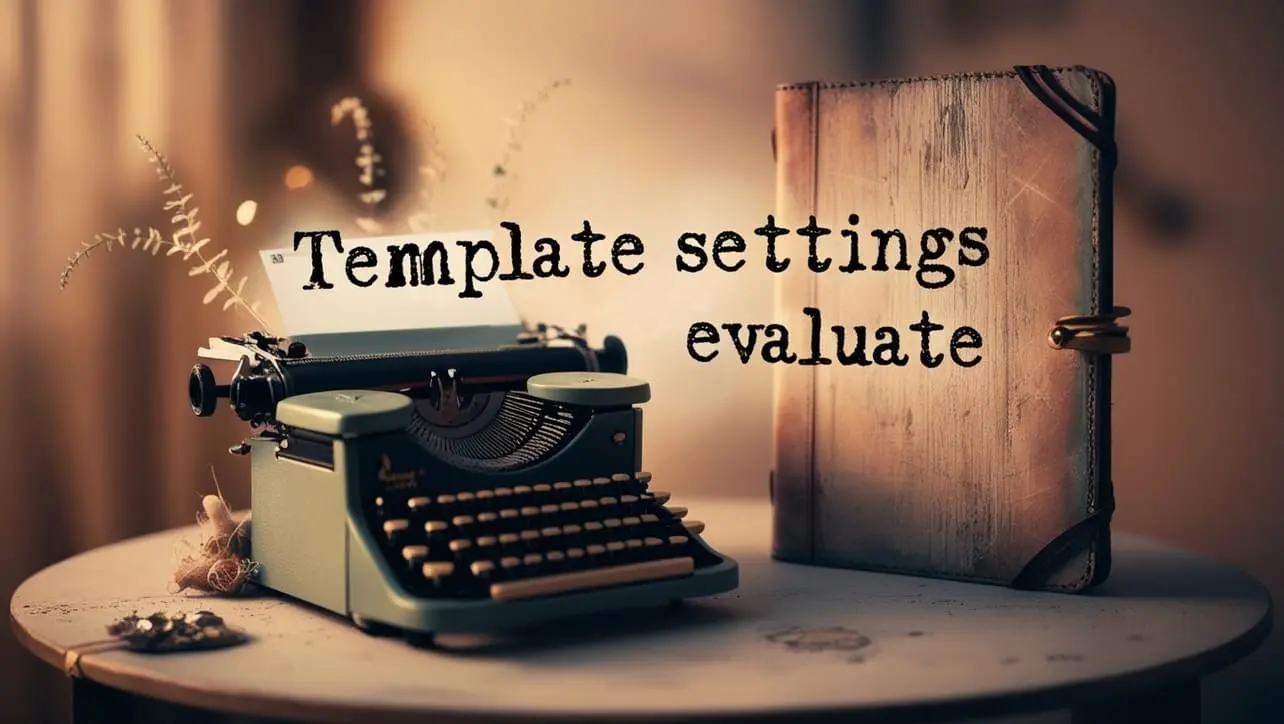
Lodash _.templateSettings.evaluate Property
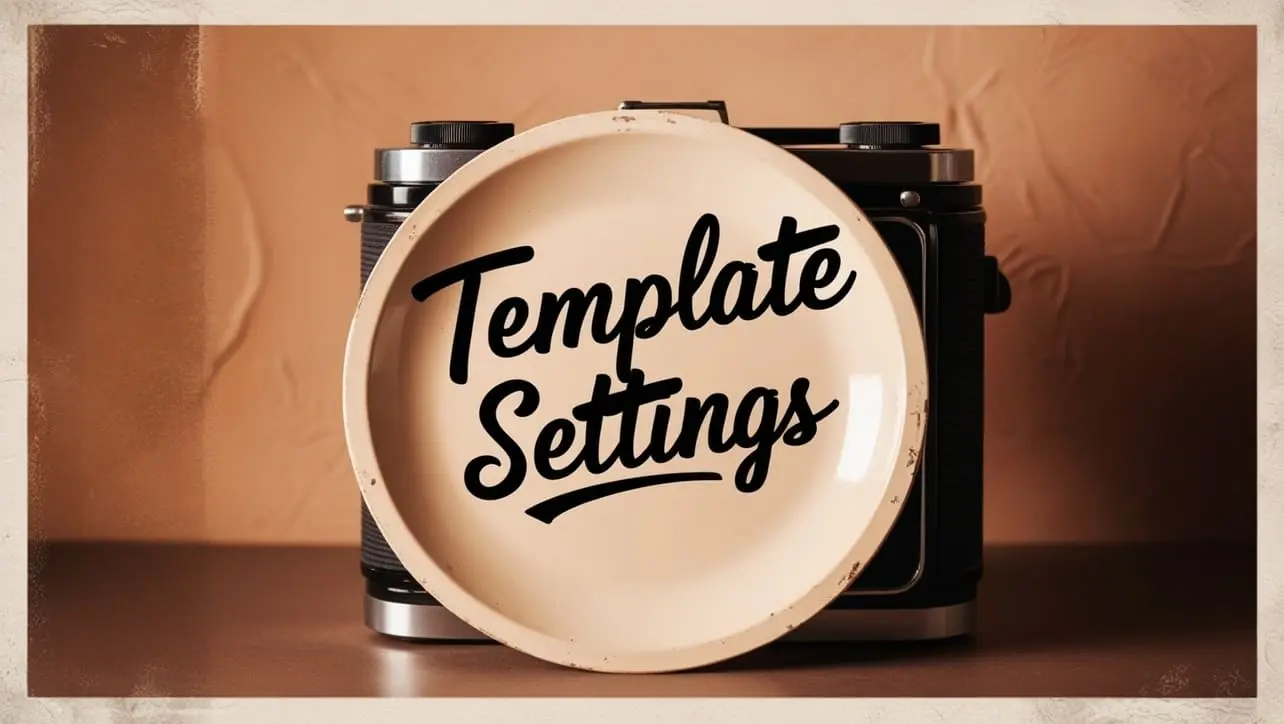
Lodash _.templateSettings Property
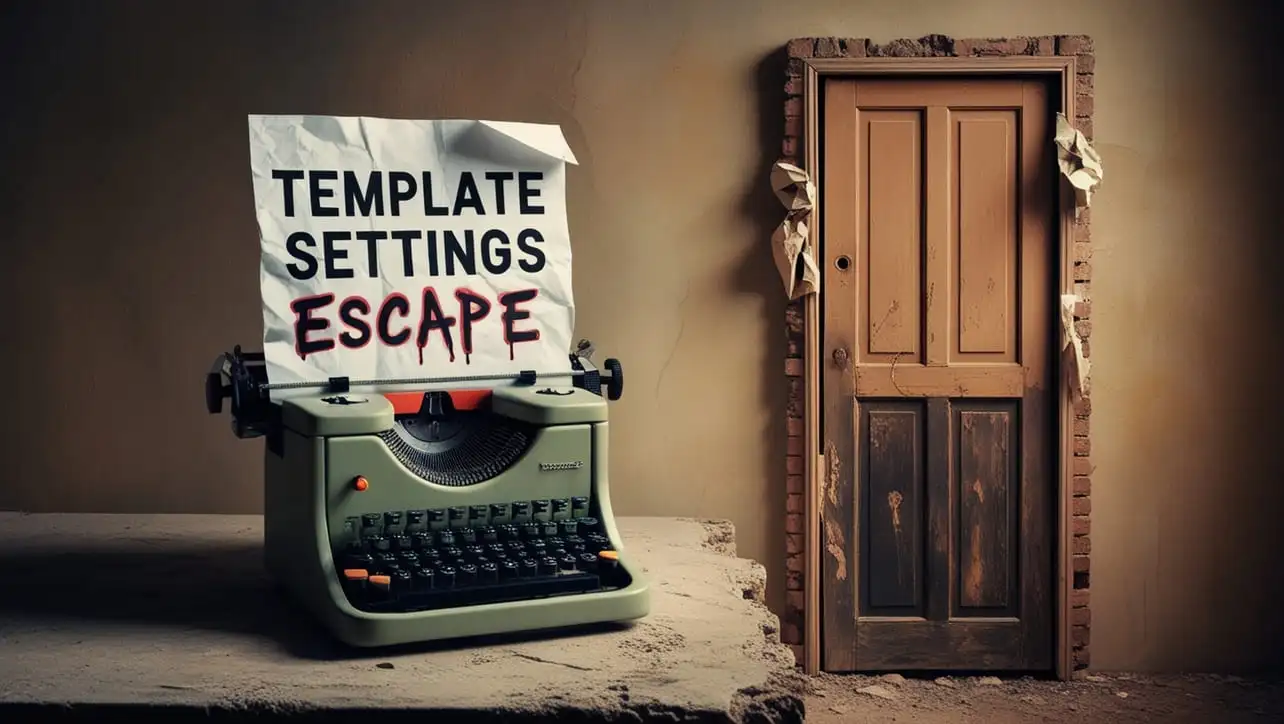
Lodash _.templateSettings.escape Property
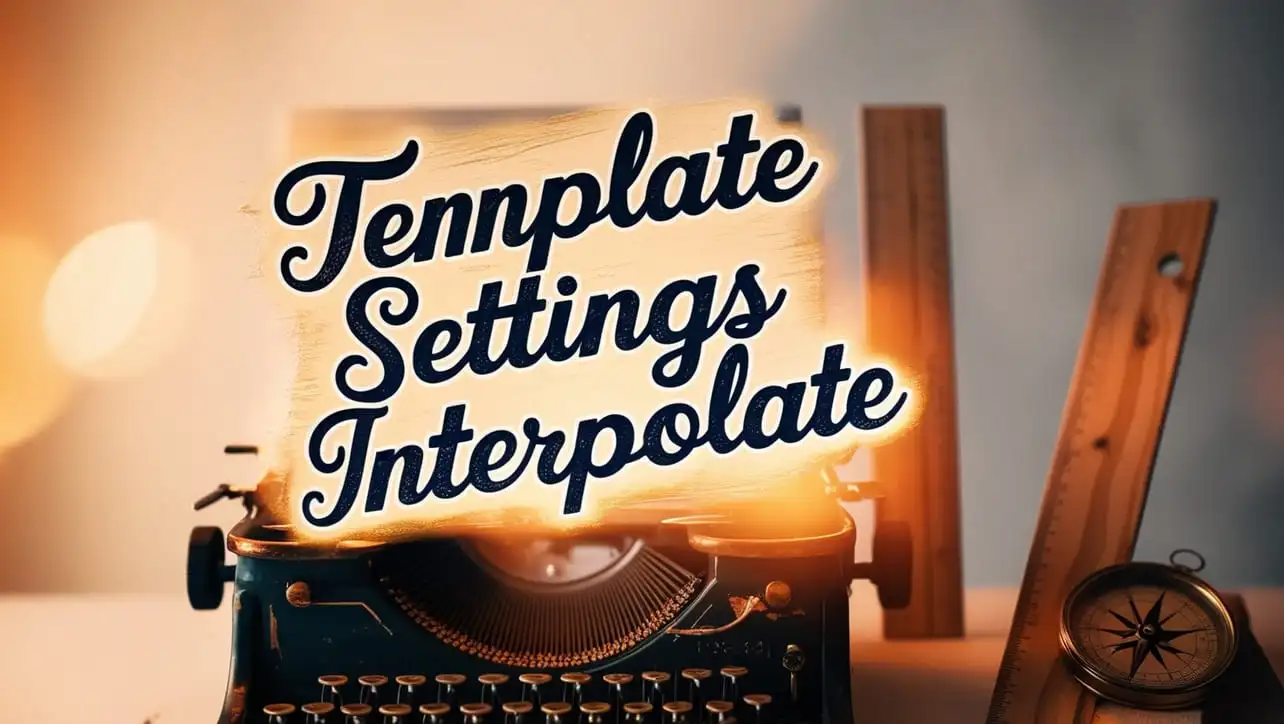
Lodash _.templateSettings.interpolate Property
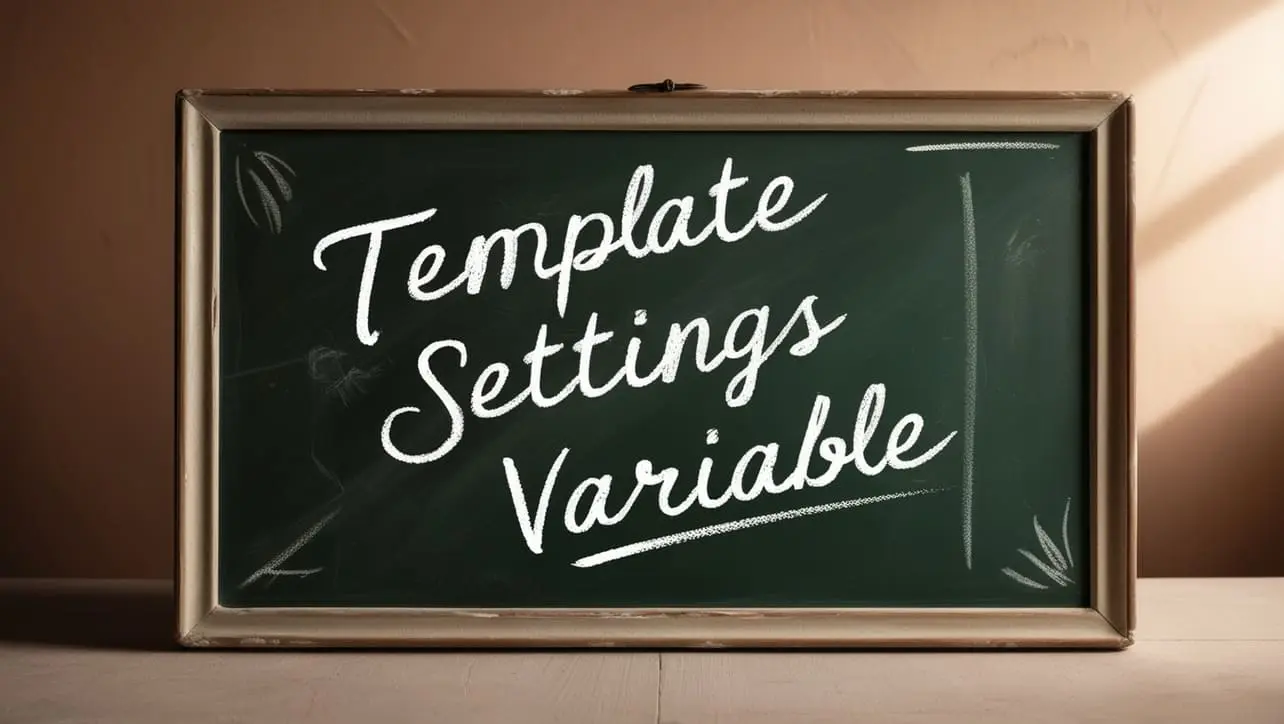
If you have any doubts regarding this article (Lodash _.isString() Lang Method), please comment here. I will help you immediately.