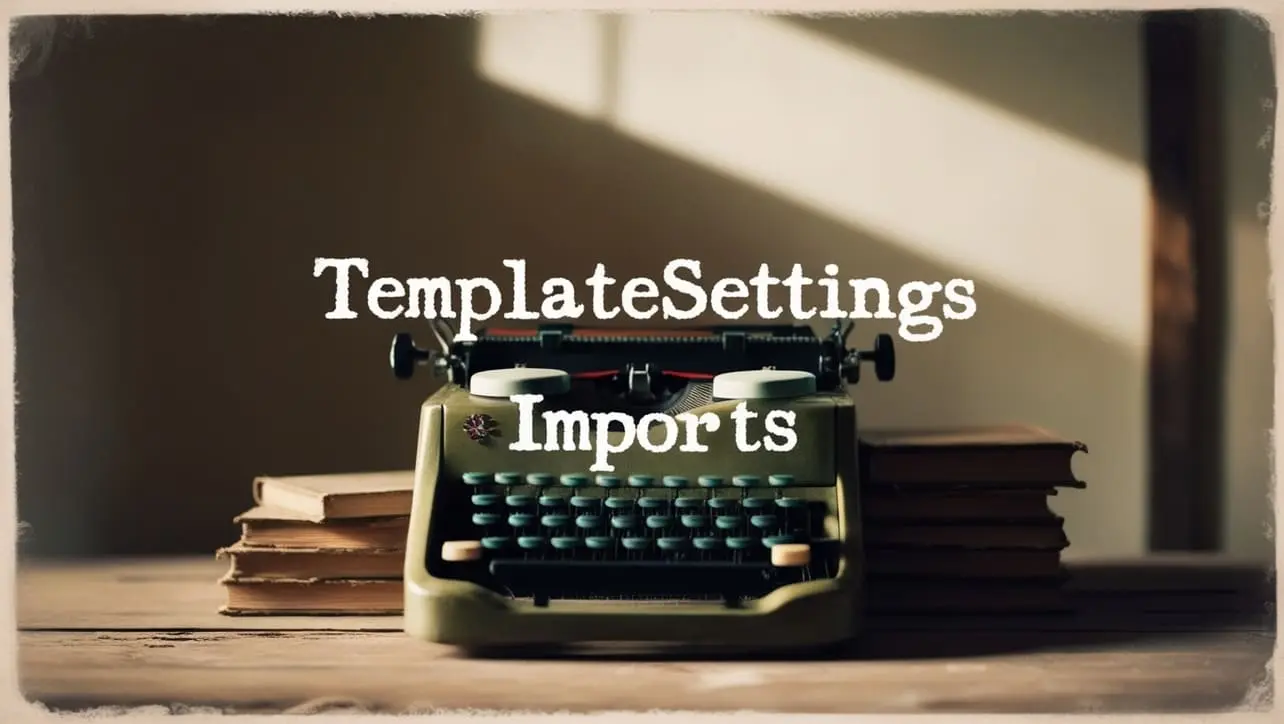
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isNumber() Lang Method
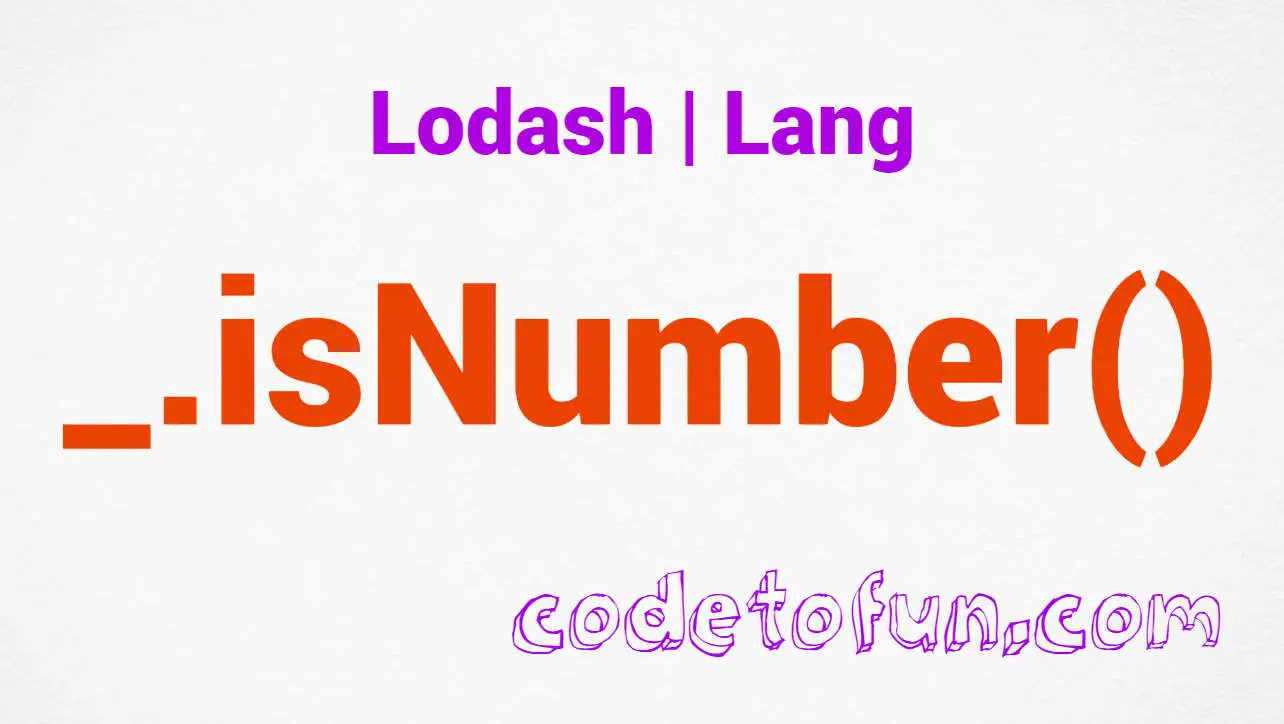
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, robust data validation is essential to ensure the integrity and reliability of code. Lodash, a versatile utility library, offers a suite of functions to simplify common programming tasks. Among these functions is _.isNumber()
, a Lang method designed to determine whether a given value is a JavaScript number.
This method aids developers in building more resilient and error-resistant code by providing a straightforward means of checking numerical data.
🧠 Understanding _.isNumber() Method
The _.isNumber()
method in Lodash is a simple yet powerful tool for validating whether a value is a number. This can be particularly useful when handling user input, API responses, or any scenario where data types need to be accurately assessed.
💡 Syntax
The syntax for the _.isNumber()
method is straightforward:
_.isNumber(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isNumber()
method:
const _ = require('lodash');
const numericValue = 42;
const nonNumericValue = 'Hello, World!';
console.log(_.isNumber(numericValue)); // Output: true
console.log(_.isNumber(nonNumericValue)); // Output: false
In this example, _.isNumber()
correctly identifies the numeric value as a number and the non-numeric value as not a number.
🏆 Best Practices
When working with the _.isNumber()
method, consider the following best practices:
Strict Type Checking:
When using
_.isNumber()
, consider whether you need strict type checking. By default, this method performs loose type checking, meaning it returns true for values that can be coerced into numbers. If strict type checking is required, additional checks may be necessary.example.jsCopiedconst numericString = '42'; console.log(_.isNumber(numericString)); // Output: true (loose type checking) console.log(Number.isNaN(numericString)); // Output: true (strict type checking)
Handling NaN (Not a Number):
Be aware that
_.isNumber()
returns false for NaN values. If your use case involves distinguishing between NaN and other non-numeric values, consider additional checks using isNaN().example.jsCopiedconst nanValue = NaN; console.log(_.isNumber(nanValue)); // Output: false console.log(Number.isNaN(nanValue)); // Output: true
Numeric Validation in Conditional Statements:
When using
_.isNumber()
in conditional statements, consider potential edge cases and whether a fallback behavior is needed for non-numeric values.example.jsCopiedconst userInput = /* ...get user input... */ ; if (_.isNumber(userInput)) { console.log('Valid numeric input:', userInput); } else { console.log('Invalid input. Please enter a number.'); }
📚 Use Cases
User Input Validation:
When dealing with user input, especially in forms or interactive applications,
_.isNumber()
can be employed to validate whether the entered data is a numeric value.example.jsCopiedconst userInput = /* ...get user input... */ ; if (_.isNumber(userInput)) { console.log('Valid numeric input:', userInput); } else { console.log('Invalid input. Please enter a number.'); }
API Response Handling:
When working with external APIs, validating numeric data in the received responses is crucial.
_.isNumber()
can assist in ensuring the expected data types.example.jsCopiedconst apiResponse = /* ...fetch data from API... */ ; if (_.isNumber(apiResponse.value)) { console.log('Numeric value from API:', apiResponse.value); } else { console.log('Unexpected or non-numeric value in API response.'); }
Data Processing and Filtering:
In scenarios involving data processing or filtering,
_.isNumber()
can be utilized to selectively process or filter out numeric values.example.jsCopiedconst dataToFilter = [42, 'apple', 23, 'orange', 17]; const numericValues = _.filter(dataToFilter, _.isNumber); console.log('Numeric values:', numericValues); // Output: [42, 23, 17]
🎉 Conclusion
The _.isNumber()
Lang method in Lodash provides a simple yet effective way to check whether a given value is a JavaScript number. Whether you're validating user input, handling API responses, or filtering data, this method offers a valuable tool for maintaining data integrity in your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isNumber()
method in your Lodash projects.
👨💻 Join our Community:
Author
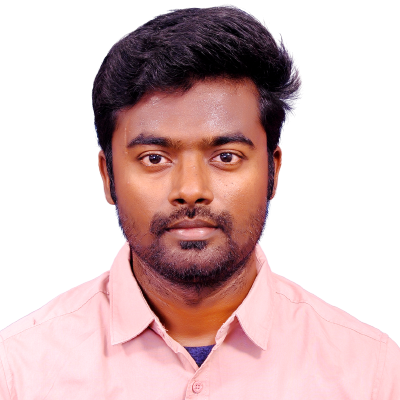
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
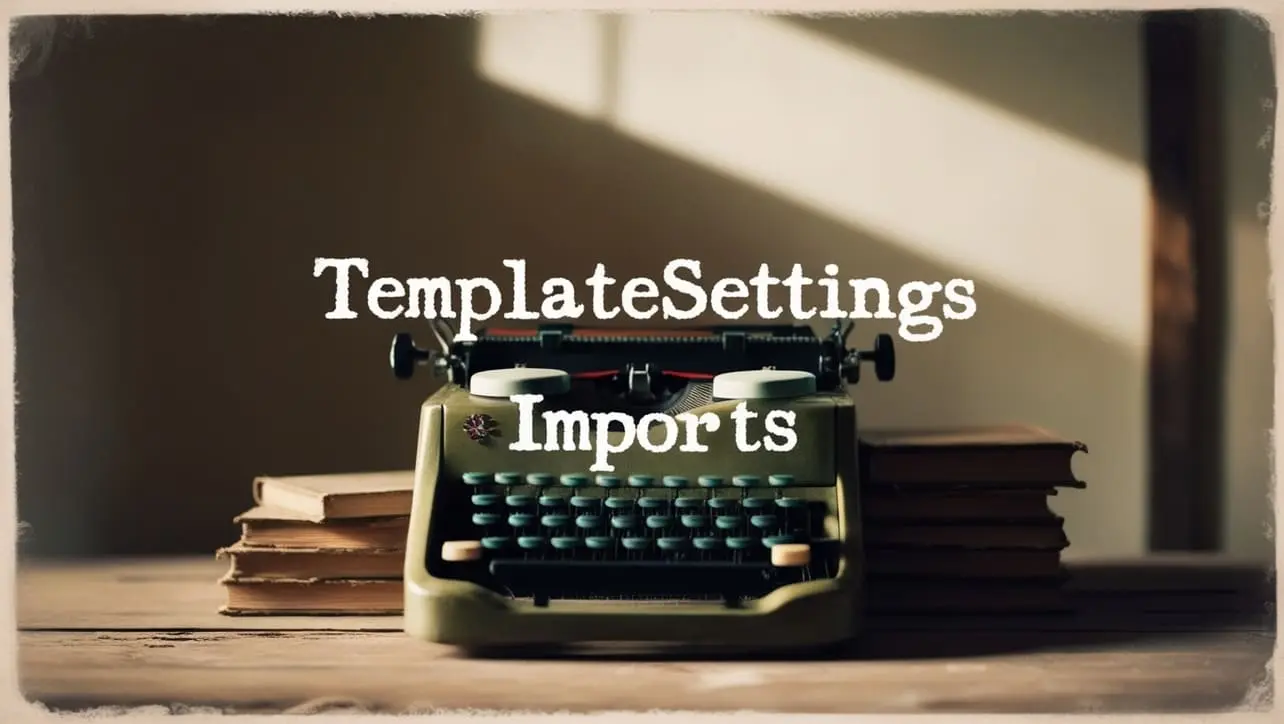
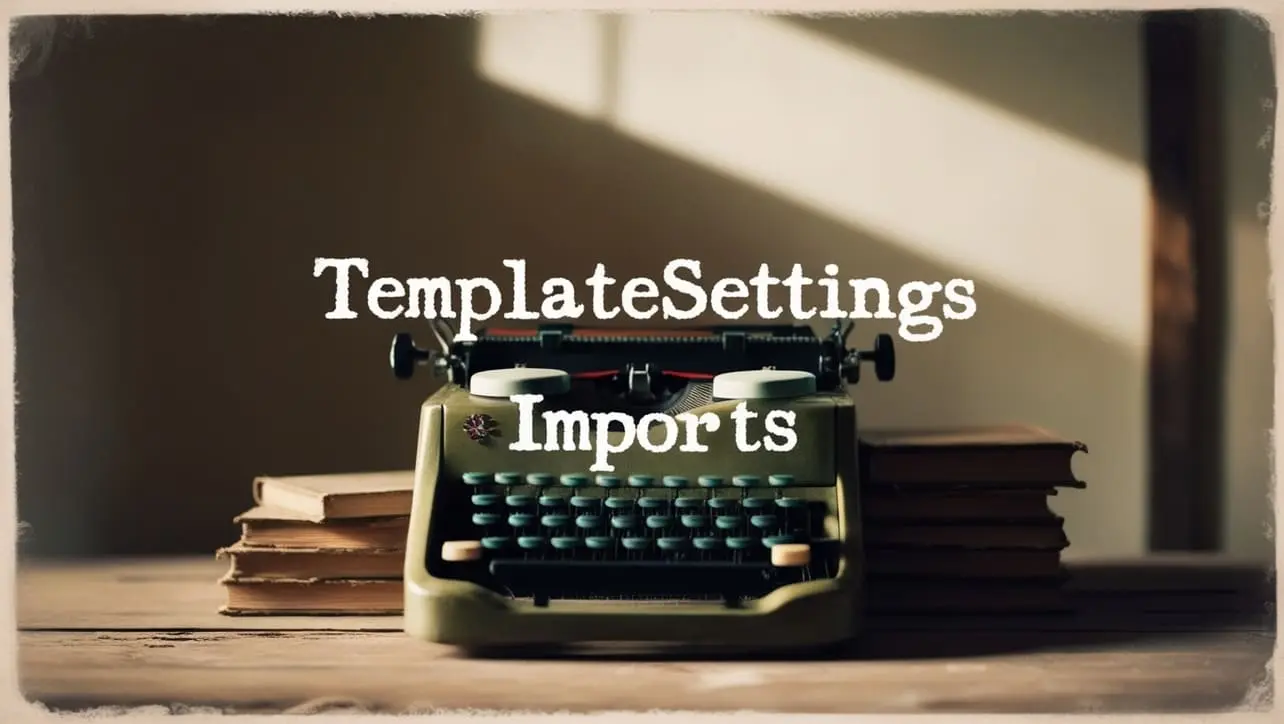
Lodash _.templateSettings.imports Property
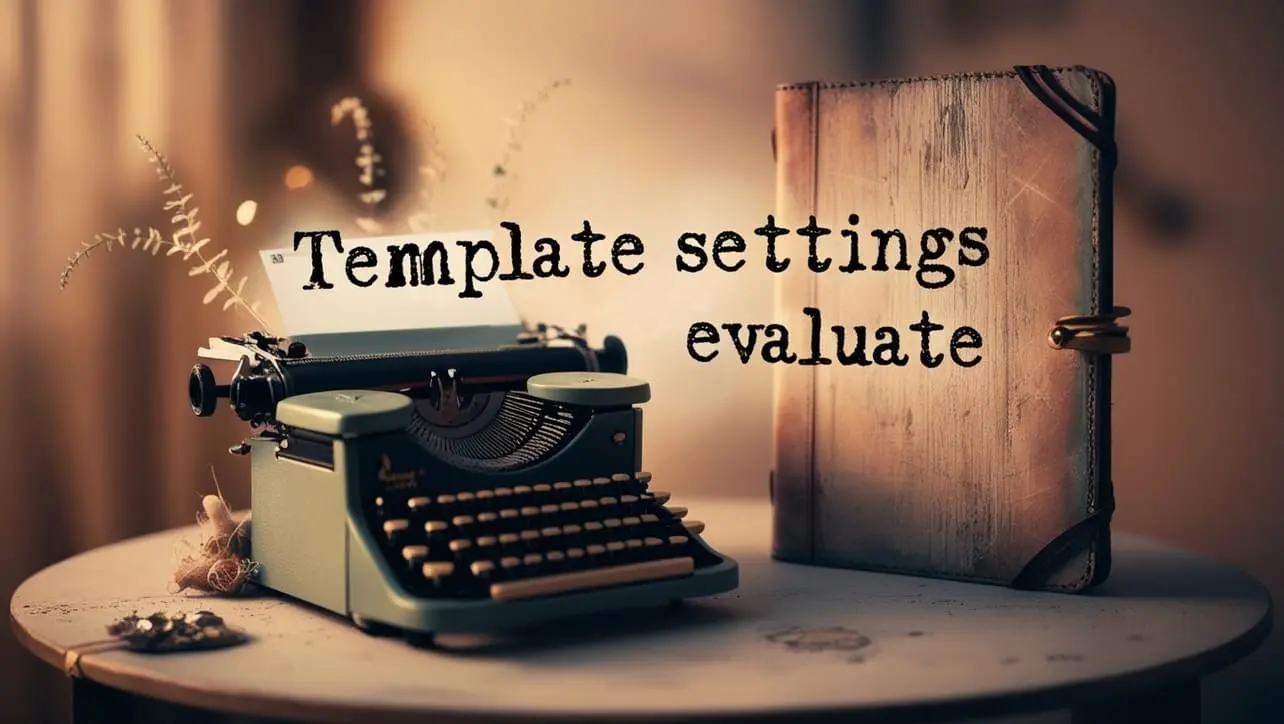
Lodash _.templateSettings.evaluate Property
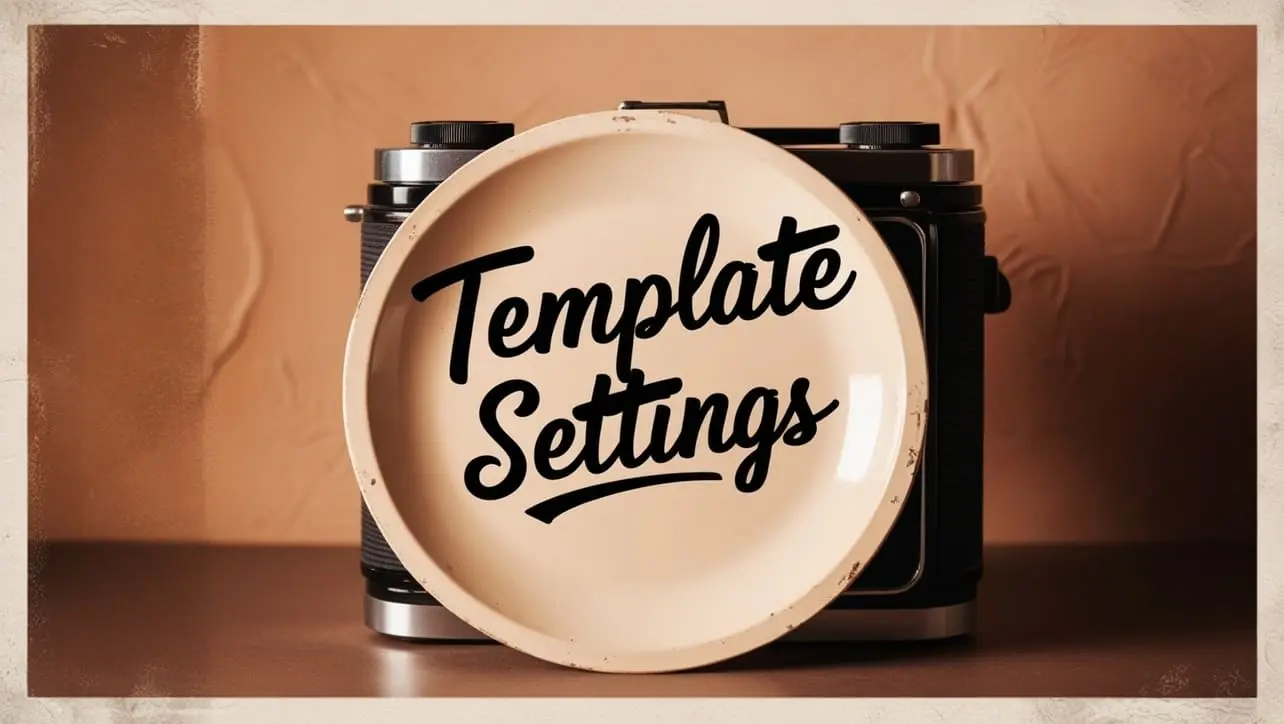
Lodash _.templateSettings Property
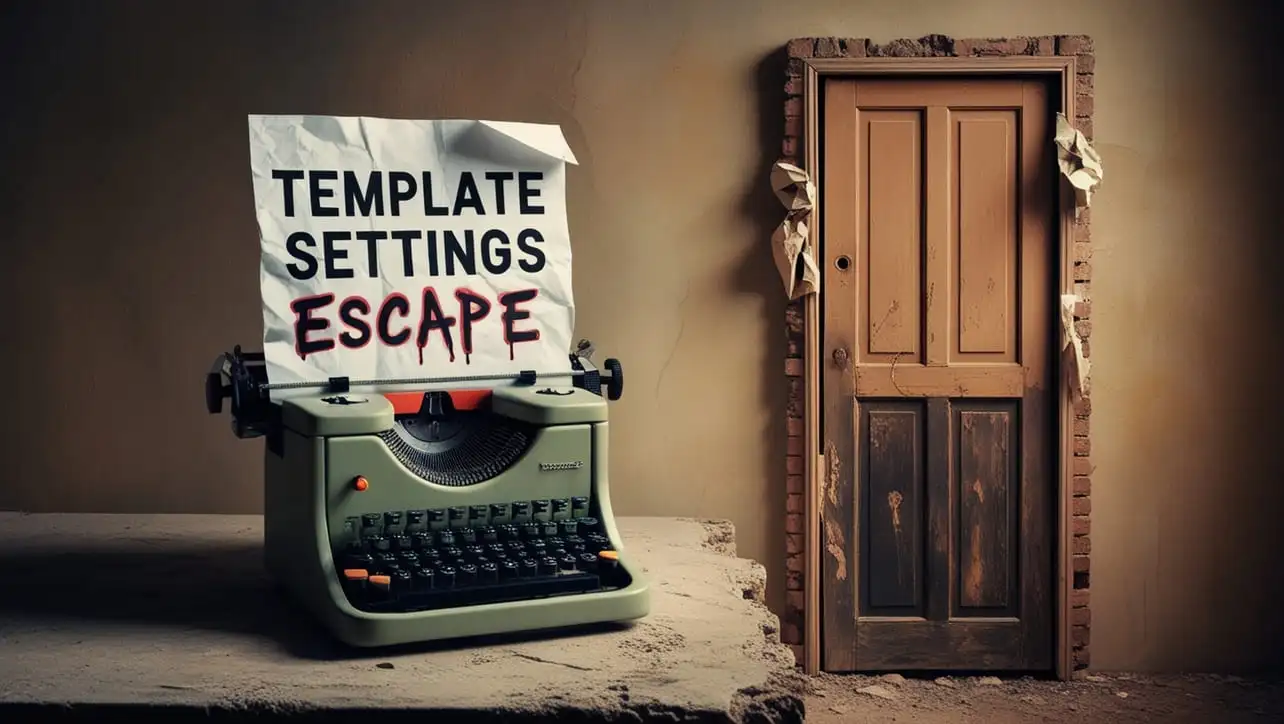
Lodash _.templateSettings.escape Property
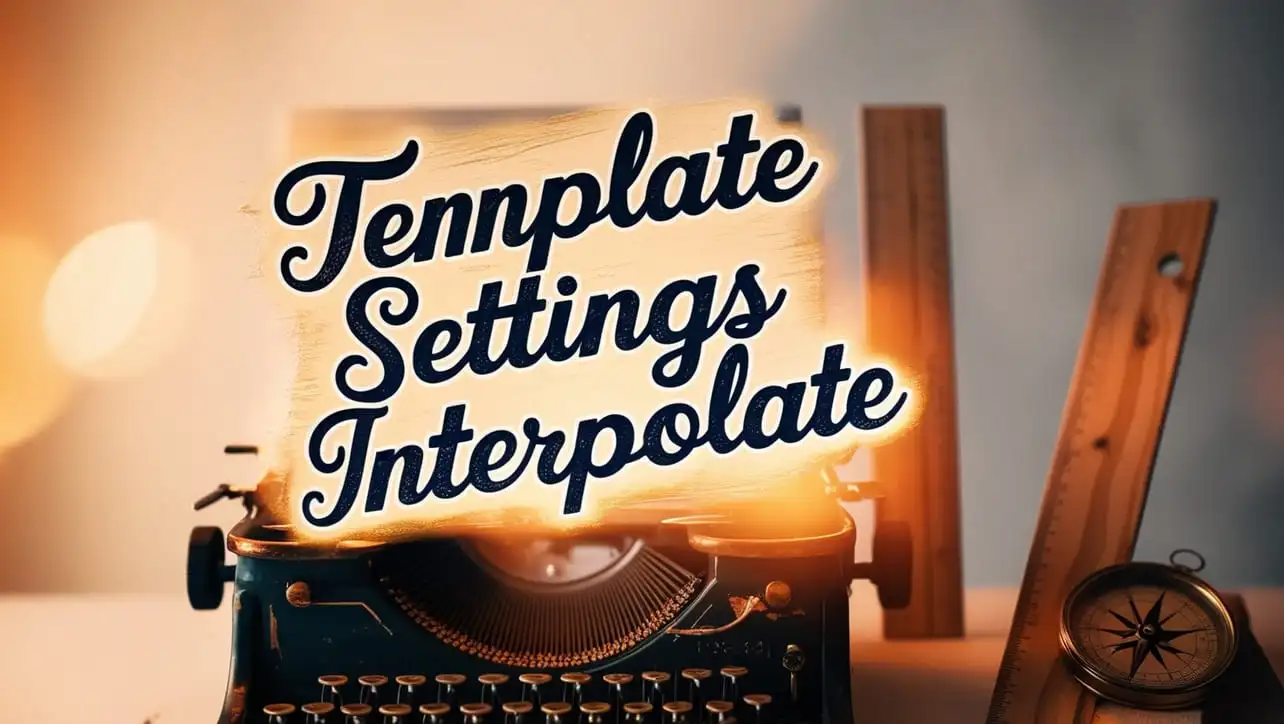
Lodash _.templateSettings.interpolate Property
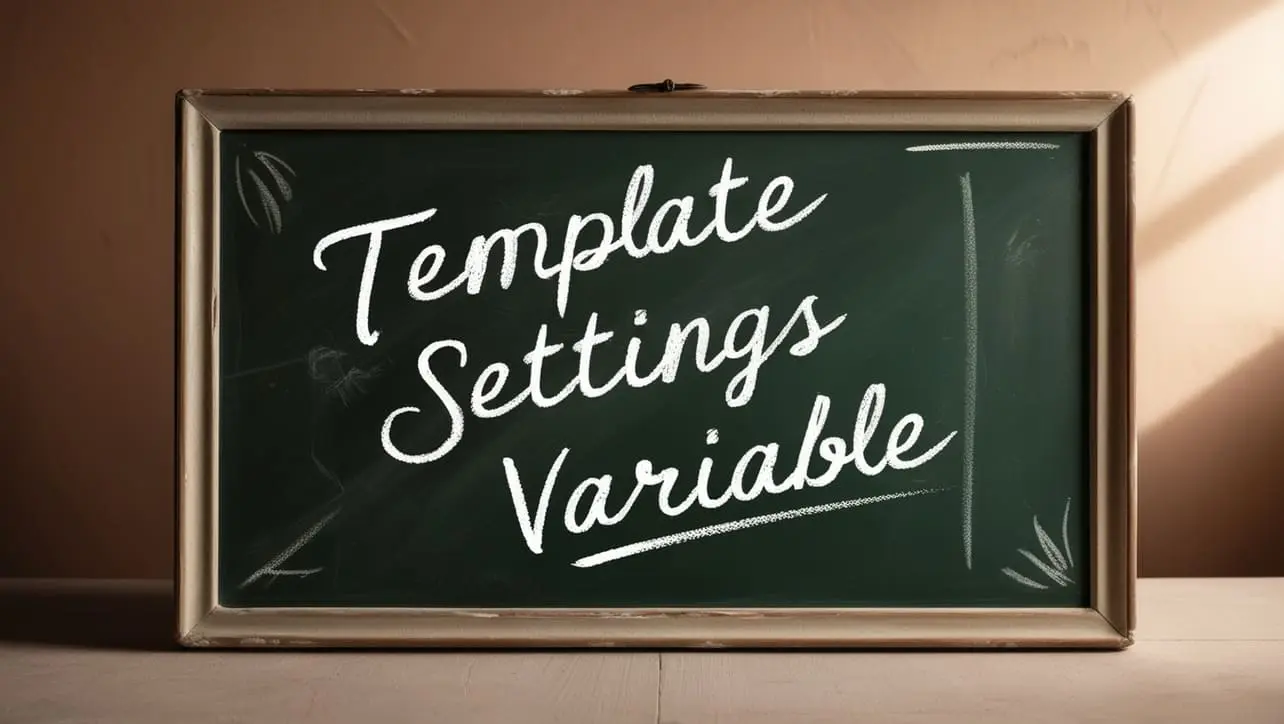
If you have any doubts regarding this article (Lodash _.isNumber() Lang Method), please comment here. I will help you immediately.