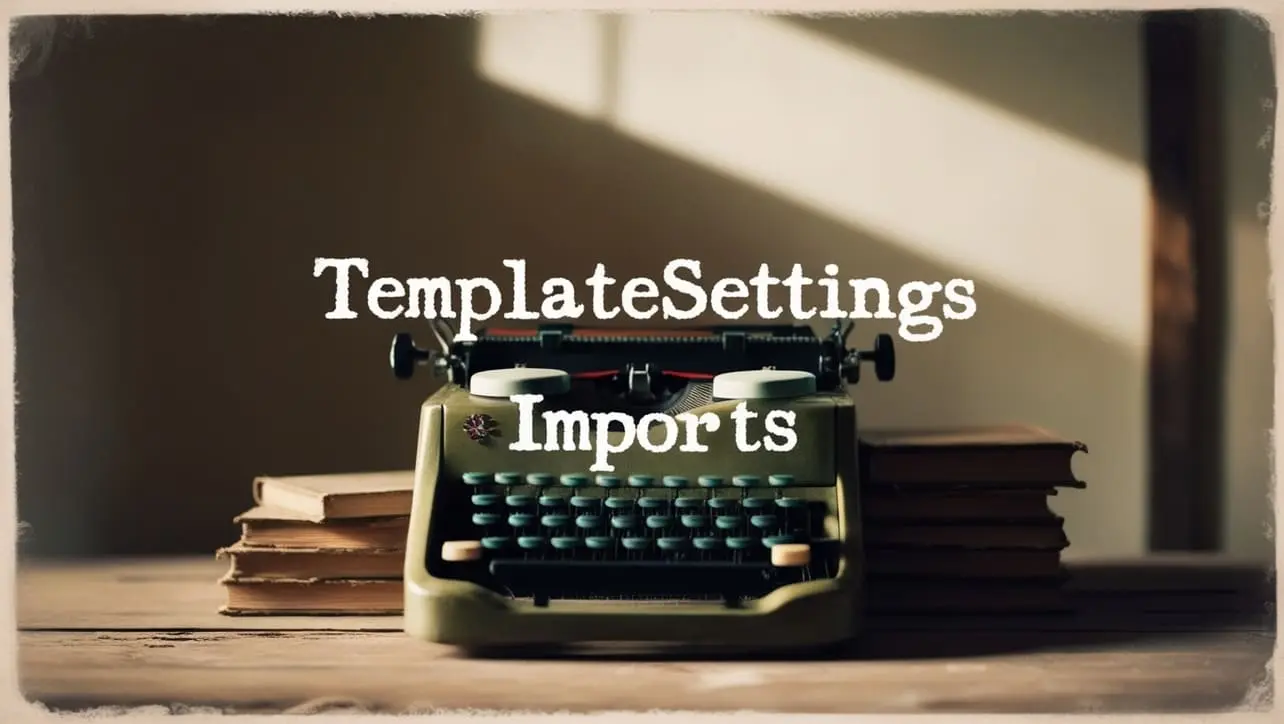
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isNil() Lang Method
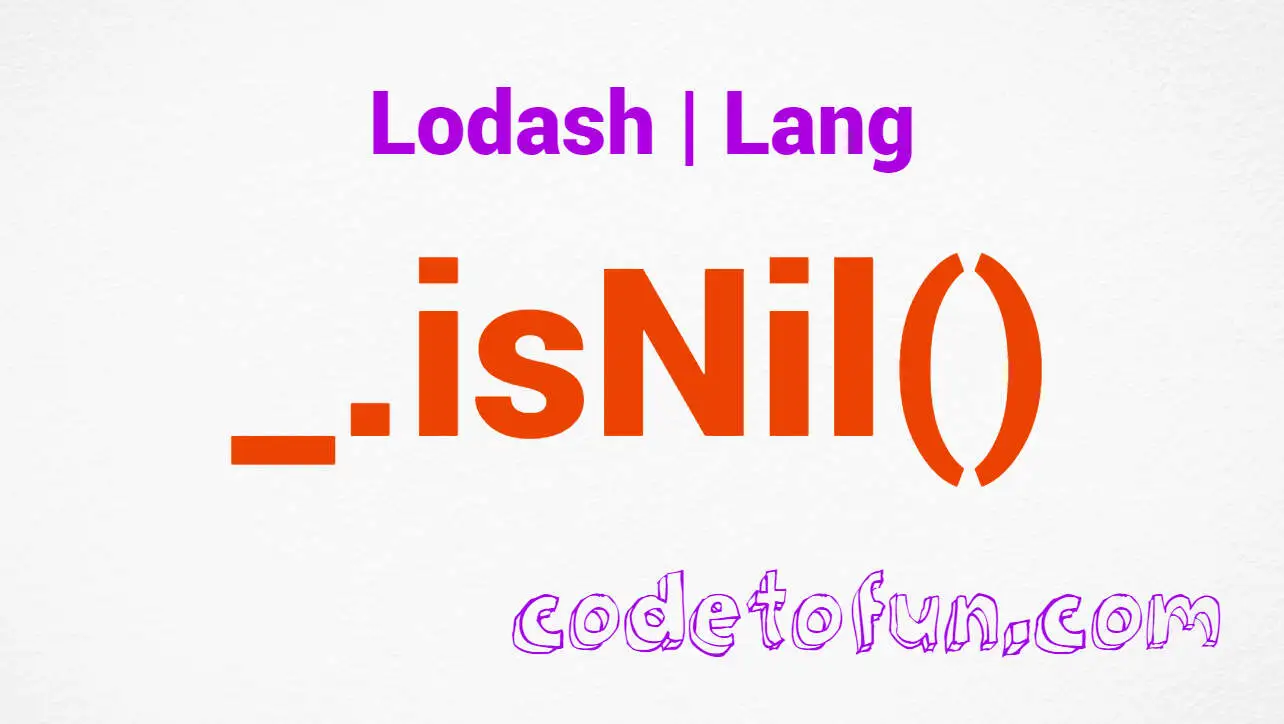
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic world of JavaScript, handling variables with care is crucial for robust and error-free code. Lodash, a powerful utility library, provides a variety of functions to simplify common programming tasks. One such handy method is _.isNil()
, belonging to the Lang category.
This method proves invaluable when you need to check whether a value is null or undefined, streamlining your code and enhancing its clarity.
🧠 Understanding _.isNil() Method
The _.isNil()
method in Lodash is designed to determine if a value is either null or undefined. This is particularly useful when you want to check for the absence of a meaningful value without explicitly comparing to both null and undefined.
💡 Syntax
The syntax for the _.isNil()
method is straightforward:
_.isNil(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isNil()
method:
const _ = require('lodash');
const exampleValue = null;
if (_.isNil(exampleValue)) {
console.log('The value is either null or undefined.');
} else {
console.log('The value is not null or undefined.');
}
In this example, the _.isNil()
method is used to determine whether exampleValue is either null or undefined.
🏆 Best Practices
When working with the _.isNil()
method, consider the following best practices:
Simplify Null and Undefined Checks:
Use
_.isNil()
to simplify checks for both null and undefined. This makes your code more concise and easier to understand.example.jsCopiedconst myValue = /* ...some value that may be null or undefined... */ ; if (_.isNil(myValue)) { console.log('The value is either null or undefined.'); } else { console.log('The value is not null or undefined.'); }
Avoid Redundant Checks:
When using
_.isNil()
, you don't need separate checks for null and undefined. The method handles both cases, reducing redundancy in your code.example.jsCopiedconst anotherValue = /* ...some value... */ ; if (_.isNil(anotherValue)) { console.log('The value is either null or undefined.'); } else { console.log('The value is not null or undefined.'); }
Combine with Other Checks:
Combine
_.isNil()
with other checks to create more comprehensive conditionals, enhancing the overall robustness of your code.example.jsCopiedconst combinedValue = /* ...some value... */ ; if (_.isNil(combinedValue) || combinedValue === 0) { console.log('The value is either null, undefined, or zero.'); } else { console.log('The value is not null, undefined, and not zero.'); }
📚 Use Cases
Initialization Checks:
Use
_.isNil()
to check whether a variable has been initialized. This is especially helpful when dealing with optional parameters or variables that may or may not have values.example.jsCopiedlet optionalParameter; if (_.isNil(optionalParameter)) { optionalParameter = /* ...initialize with a default value... */ ; } console.log('Optional parameter:', optionalParameter);
Object Property Existence:
Check if an object property exists and is not null or undefined using
_.isNil()
.example.jsCopiedconst myObject = { key: /* ...some value... */ }; if (_.isNil(myObject.key)) { console.log('The property "key" is either null or undefined.'); } else { console.log('The property "key" is not null or undefined.'); }
Defensive Programming:
In scenarios where defensive programming is crucial, use
_.isNil()
to ensure that variables are in an expected state before proceeding with operations.example.jsCopiedfunction processData(data) { if (_.isNil(data)) { console.error('Invalid data. Cannot process.'); return; } // Continue with data processing logic... }
🎉 Conclusion
The _.isNil()
method in Lodash is a valuable tool for simplifying null and undefined checks in JavaScript. By incorporating this method into your code, you can enhance readability and reduce redundancy, ultimately leading to more robust and maintainable applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isNil()
method in your Lodash projects.
👨💻 Join our Community:
Author
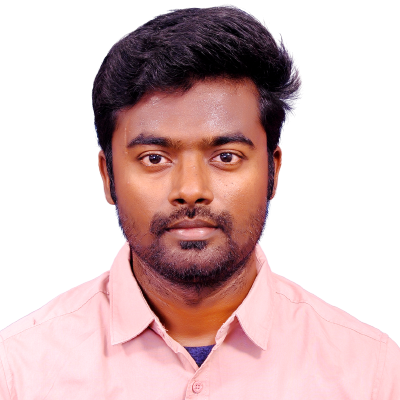
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
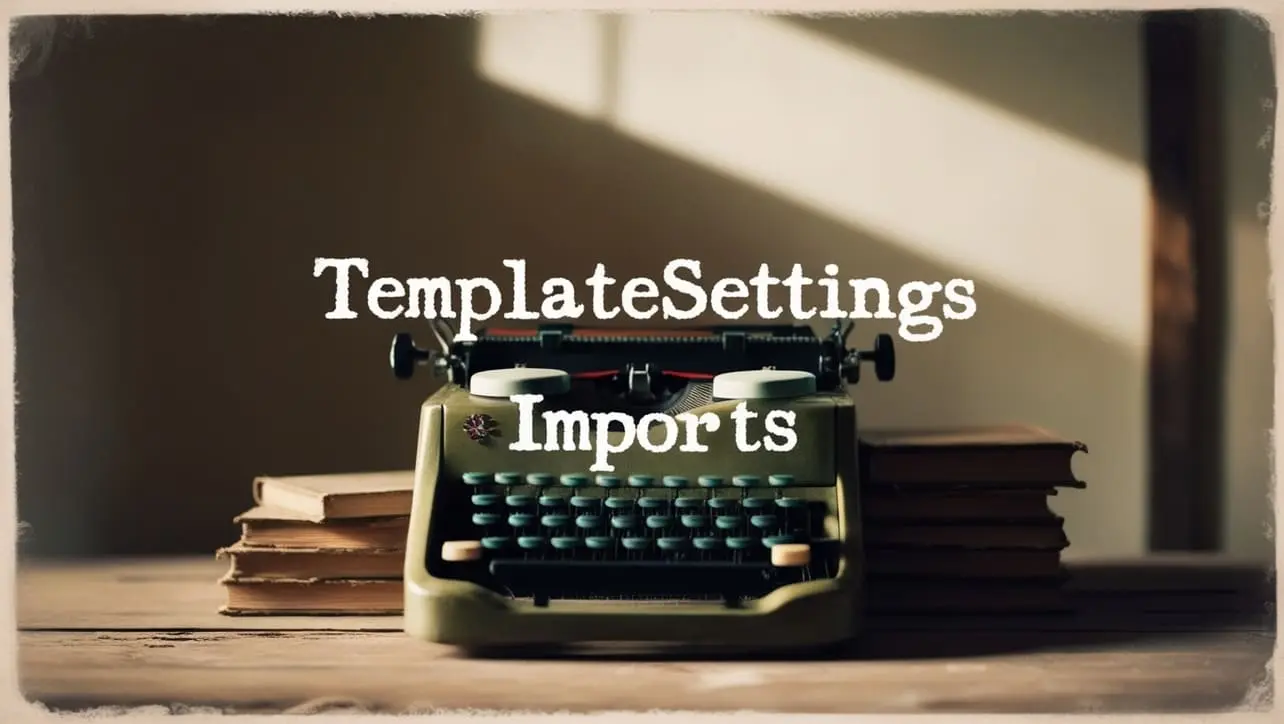
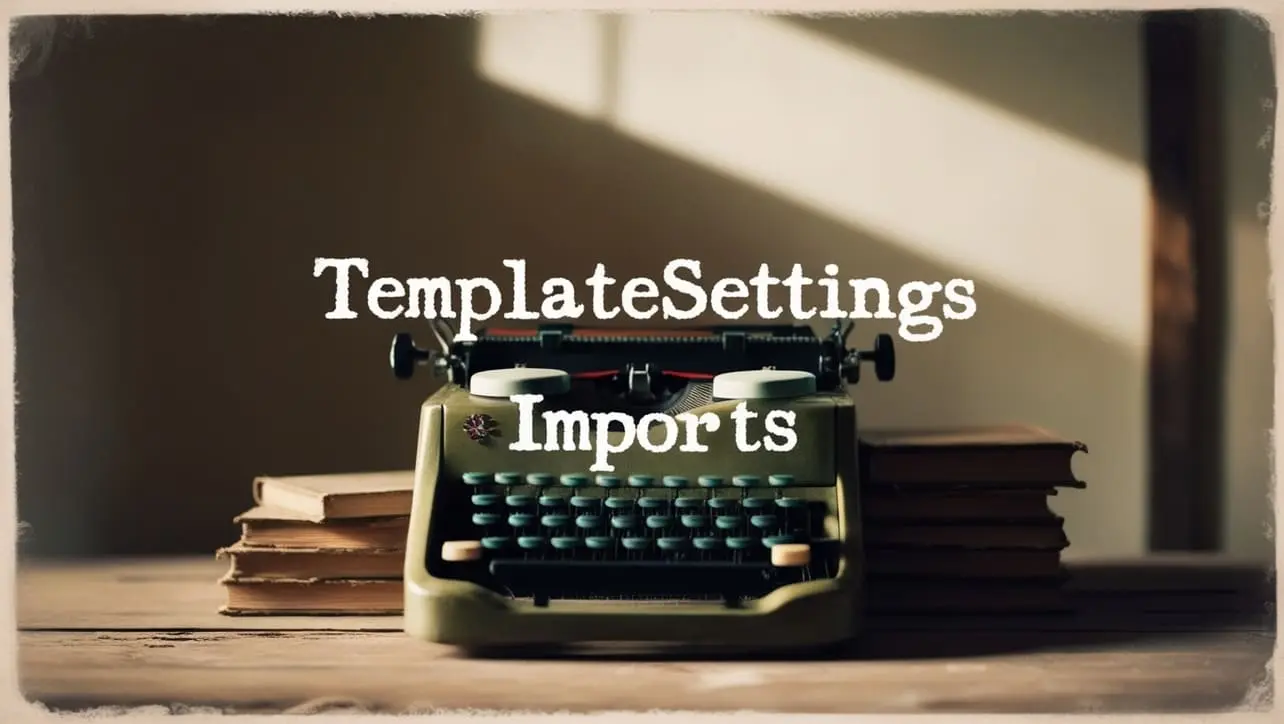
Lodash _.templateSettings.imports Property
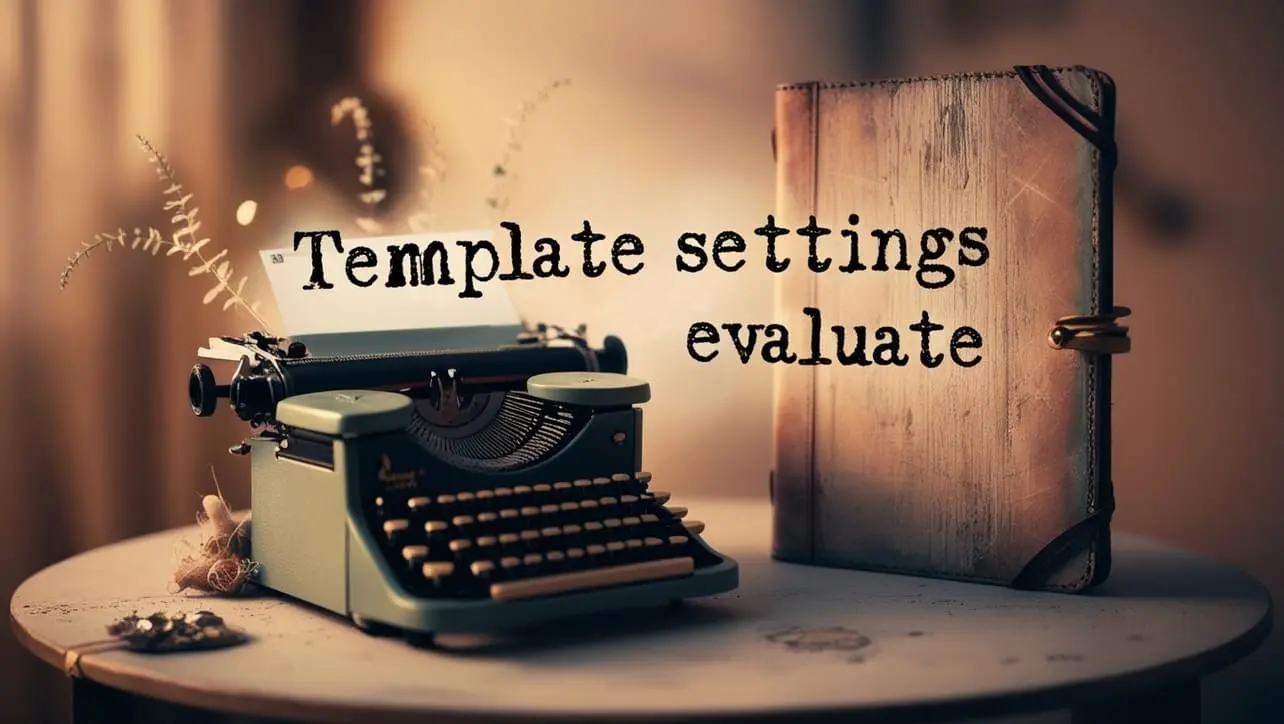
Lodash _.templateSettings.evaluate Property
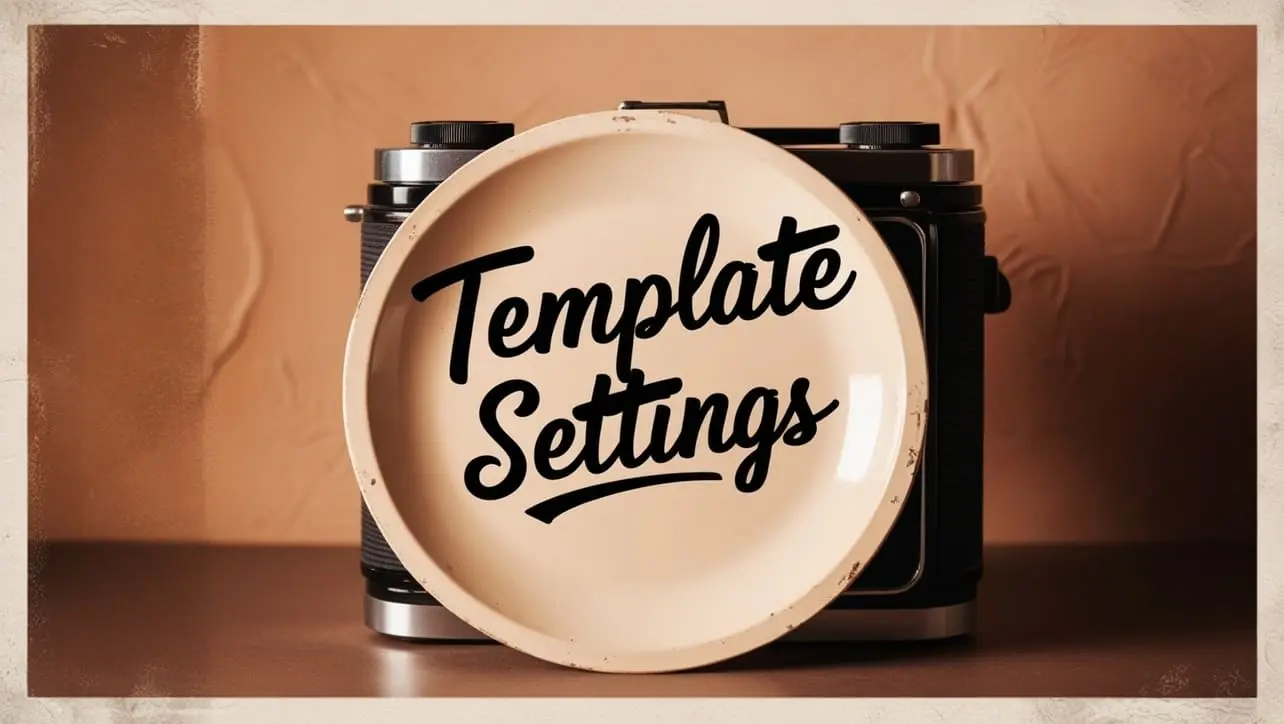
Lodash _.templateSettings Property
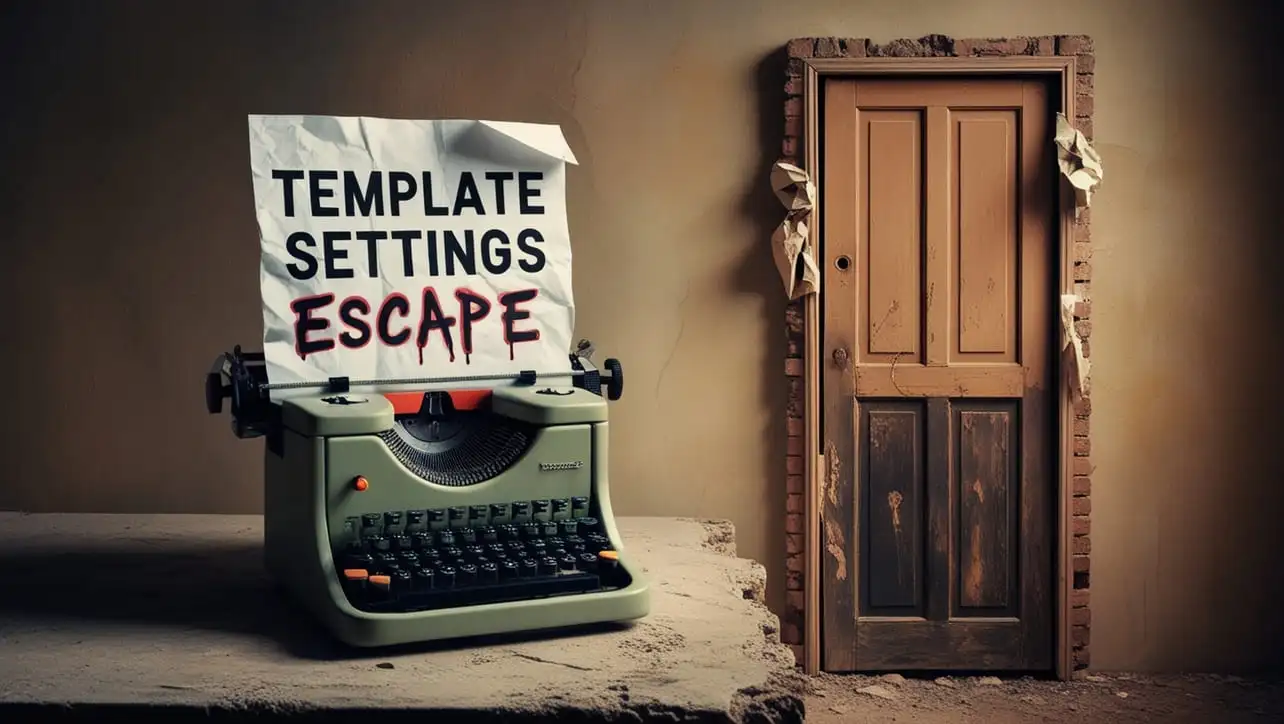
Lodash _.templateSettings.escape Property
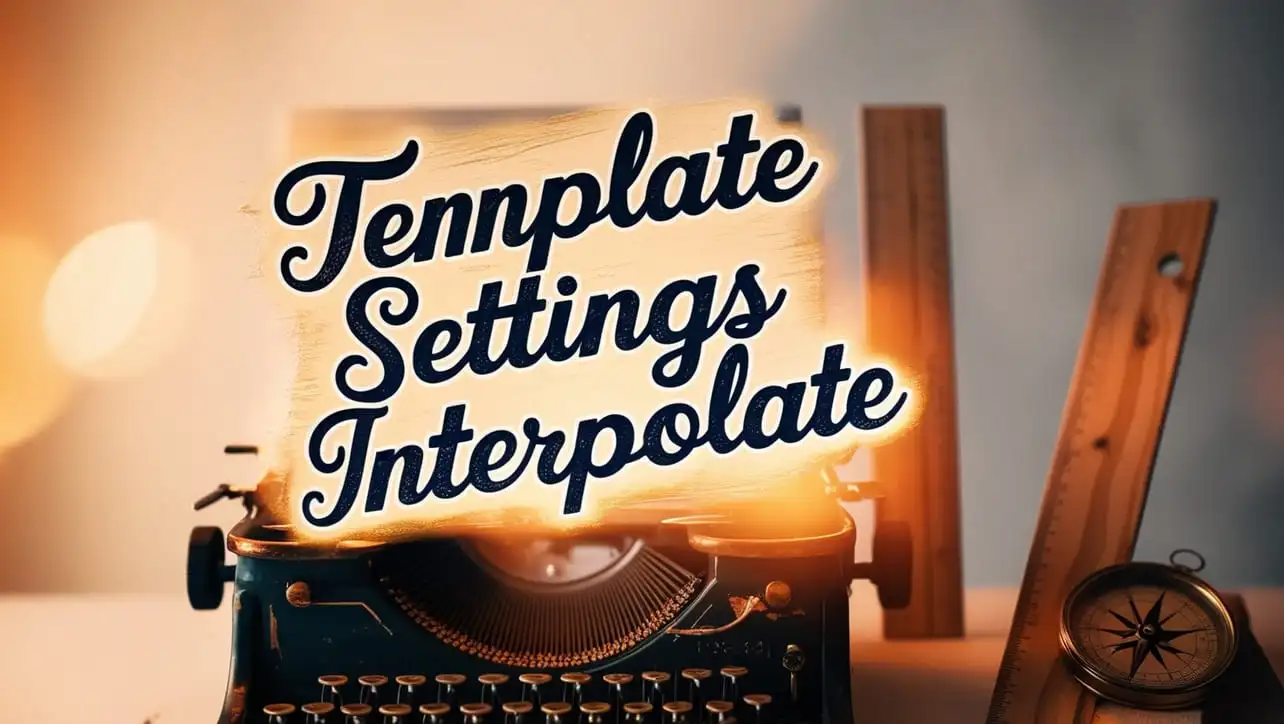
Lodash _.templateSettings.interpolate Property
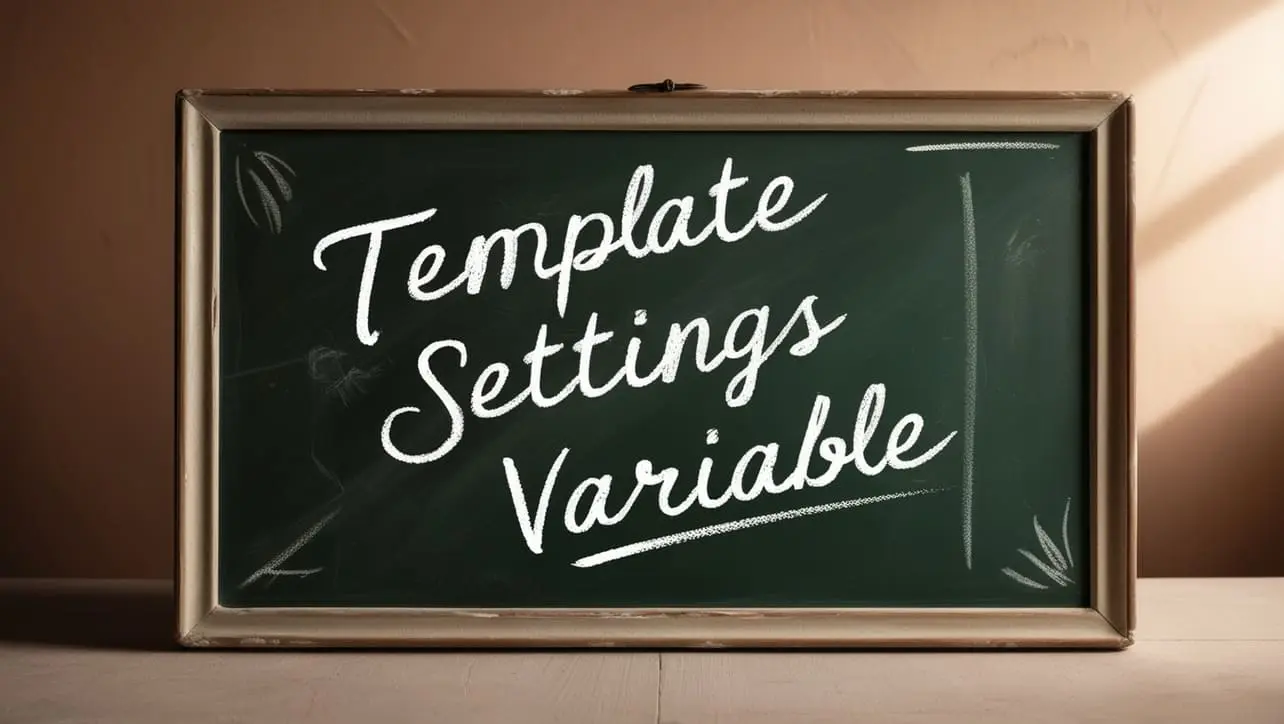
If you have any doubts regarding this article (Lodash _.isNil() Lang Method), please comment here. I will help you immediately.