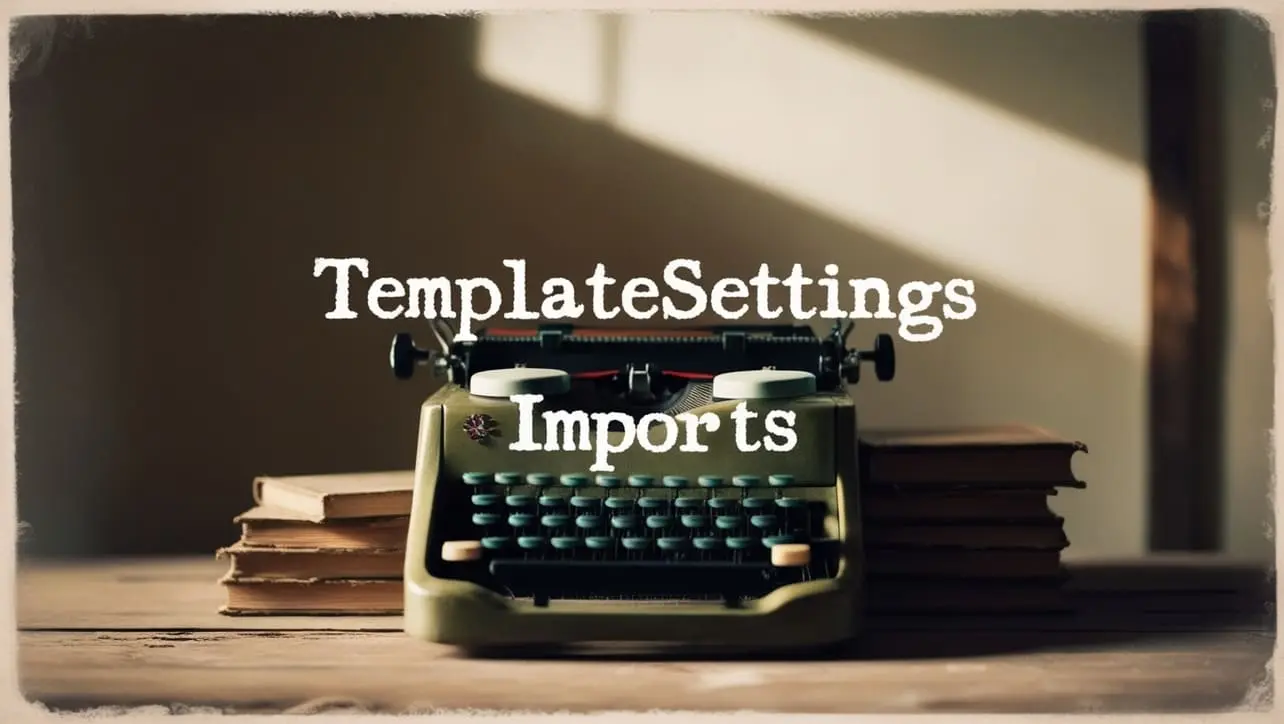
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isMatchWith() Lang Method
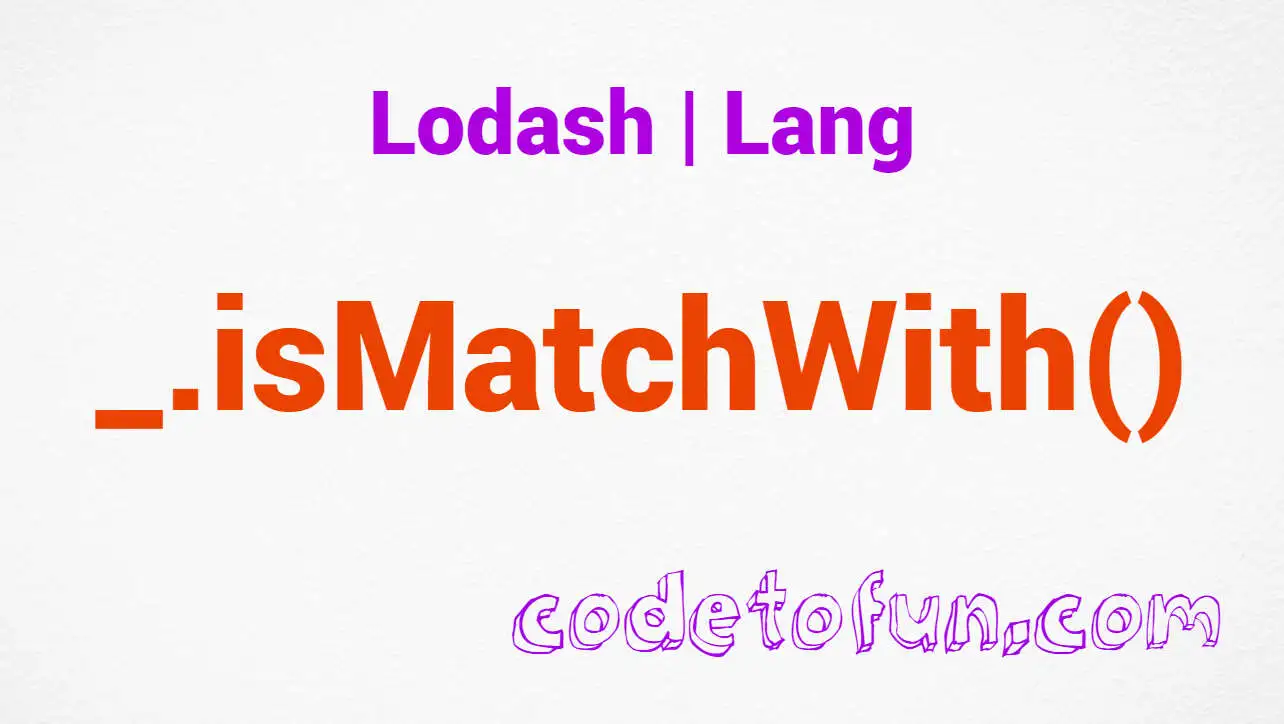
Photo Credit to CodeToFun
🙋 Introduction
In the diverse landscape of JavaScript development, effective comparison of objects is a common challenge. Lodash, a comprehensive utility library, offers the _.isMatchWith()
method, a powerful tool for custom object comparisons.
This method allows developers to define their own criteria for matching objects, providing flexibility and precision in object comparison scenarios.
🧠 Understanding _.isMatchWith() Method
The _.isMatchWith()
method in Lodash is designed to perform deep object comparison with a customizer function. This customizer function enables developers to specify how object values should be compared, allowing for intricate and tailored matching logic.
💡 Syntax
The syntax for the _.isMatchWith()
method is straightforward:
_.isMatchWith(object, source, [customizer])
- object: The object to inspect.
- source: The object of property values to match.
- customizer (Optional): The function to customize value comparisons.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isMatchWith()
method:
const _ = require('lodash');
const user = {
id: 1,
name: 'John',
age: 30
};
const searchCriteria = {
id: 1,
name: 'John'
};
const isMatch = _.isMatchWith(user, searchCriteria, (objValue, srcValue) => {
// Custom comparison logic
if (_.isNumber(objValue) && _.isNumber(srcValue)) {
return objValue === srcValue;
}
});
console.log(isMatch); // Output: true
In this example, the user object is compared to the searchCriteria object using a customizer function. The customizer function defines a specific comparison logic for numeric values.
🏆 Best Practices
When working with the _.isMatchWith()
method, consider the following best practices:
Custom Comparison Logic:
Leverage the customizer function to implement specific comparison logic tailored to your use case. This allows you to finely control how object properties are evaluated for equality.
example.jsCopiedconst objectA = { name: 'Alice', age: 25 }; const objectB = { name: 'Alice', age: '25' }; const isMatchWithCustomLogic = _.isMatchWith(objectA, objectB, (objValue, srcValue, key) => { // Custom comparison logic for 'age' property if (key === 'age') { return _.isEqual(Number(objValue), Number(srcValue)); } }); console.log(isMatchWithCustomLogic); // Output: true
Handling Special Cases:
Consider edge cases and handle them within the customizer function. This ensures that the comparison logic accommodates unique scenarios, preventing unexpected results.
example.jsCopiedconst complexObjectA = { data: { id: 1, value: 'A' } }; const complexObjectB = { data: { id: '1', value: 'A' } }; const isMatchWithSpecialHandling = _.isMatchWith(complexObjectA, complexObjectB, (objValue, srcValue, key) => { // Special handling for 'id' property if (key === 'id') { return _.isEqual(Number(objValue), Number(srcValue)); } }); console.log(isMatchWithSpecialHandling); // Output: true
Performance Considerations:
Be mindful of the performance implications when implementing complex customizer functions. Strive for a balance between customization and efficiency, especially when dealing with large datasets.
example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */ ; const searchCriteria = /* ...define search criteria... */ ; const isMatchInLargeDataset = _.isMatchWith(largeDataset, searchCriteria, (objValue, srcValue) => { // Custom comparison logic // ... // Ensure the custom logic is efficient }); console.log(isMatchInLargeDataset);
📚 Use Cases
Custom Object Matching:
_.isMatchWith()
is particularly useful when you need to perform custom deep object comparisons, allowing you to define specific rules for equality.example.jsCopiedconst employeeA = { id: 1, name: 'Bob', department: 'HR' }; const employeeB = { id: 1, name: 'Bob', department: 'Human Resources' }; const customObjectMatching = _.isMatchWith(employeeA, employeeB, (objValue, srcValue, key) => { // Custom comparison logic for 'department' property if (key === 'department') { return objValue.toLowerCase() === srcValue.toLowerCase(); } }); console.log(customObjectMatching); // Output: true
Filtering Objects Based on Criteria:
When filtering objects based on specific criteria,
_.isMatchWith()
empowers you to implement intricate filtering rules, ensuring accurate results.example.jsCopiedconst employees = /* ...an array of employee objects... */ ; const searchCriteria = /* ...define filter criteria... */ ; const filteredEmployees = employees.filter(employee => { return _.isMatchWith(employee, searchCriteria, (objValue, srcValue, key) => { // Custom comparison logic based on search criteria // ... }); }); console.log(filteredEmployees);
Configurable Object Matching:
For scenarios where object matching needs to be configurable,
_.isMatchWith()
provides a dynamic solution by allowing developers to pass custom comparison logic.example.jsCopiedconst objectToMatch = /* ...an object to match... */; const customComparisonLogic = /* ...a dynamically generated customizer function... */; const isMatchWithConfigurableLogic = _.isMatchWith(objectToMatch, searchCriteria, customComparisonLogic); console.log(isMatchWithConfigurableLogic);
🎉 Conclusion
The _.isMatchWith()
method in Lodash offers a robust solution for performing custom deep object comparisons in JavaScript. Whether you're dealing with complex data structures or implementing specific matching criteria, this method provides the flexibility needed to achieve precise and tailored object comparisons.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isMatchWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
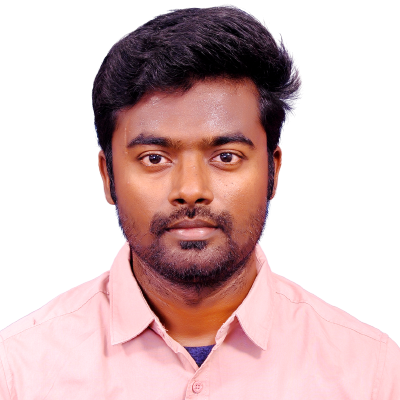
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
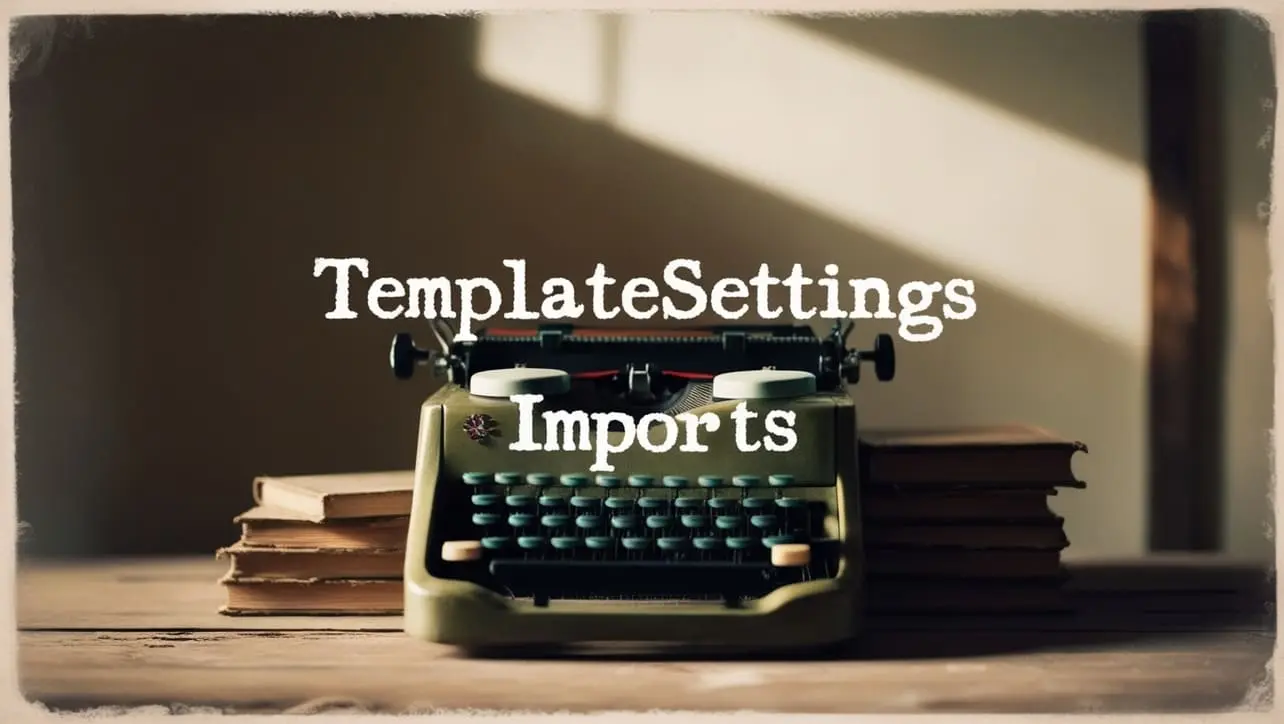
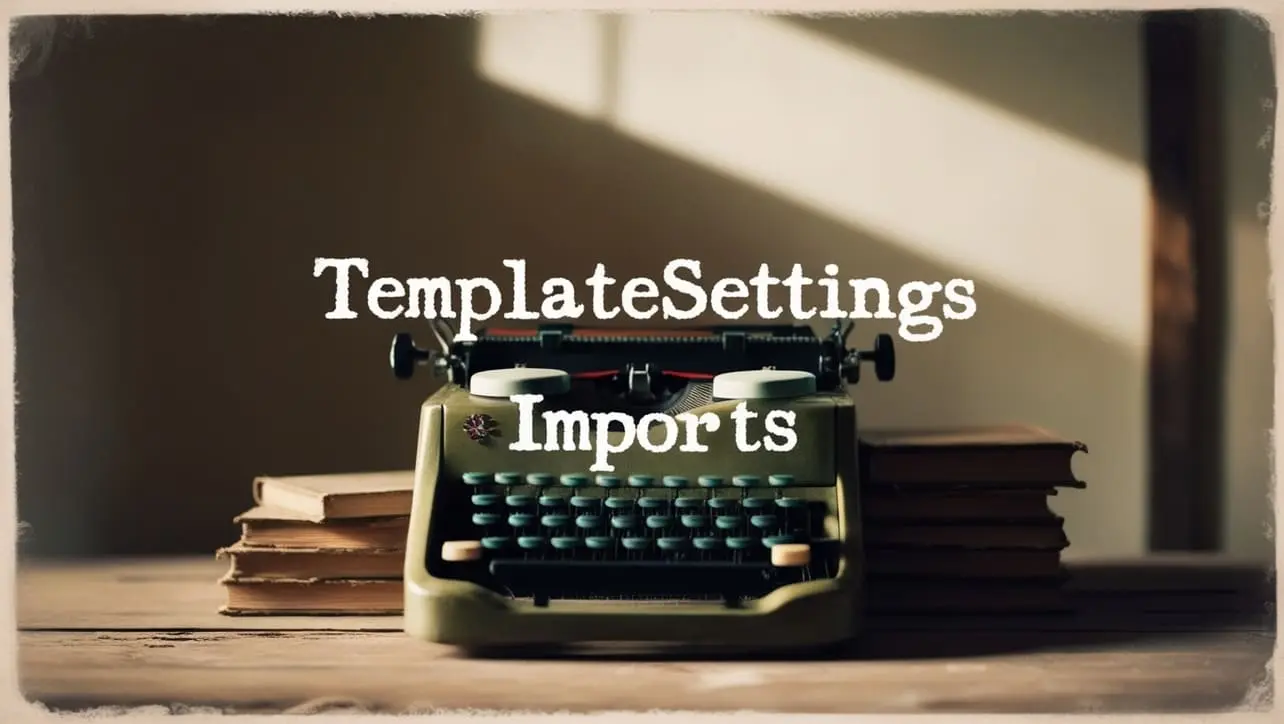
Lodash _.templateSettings.imports Property
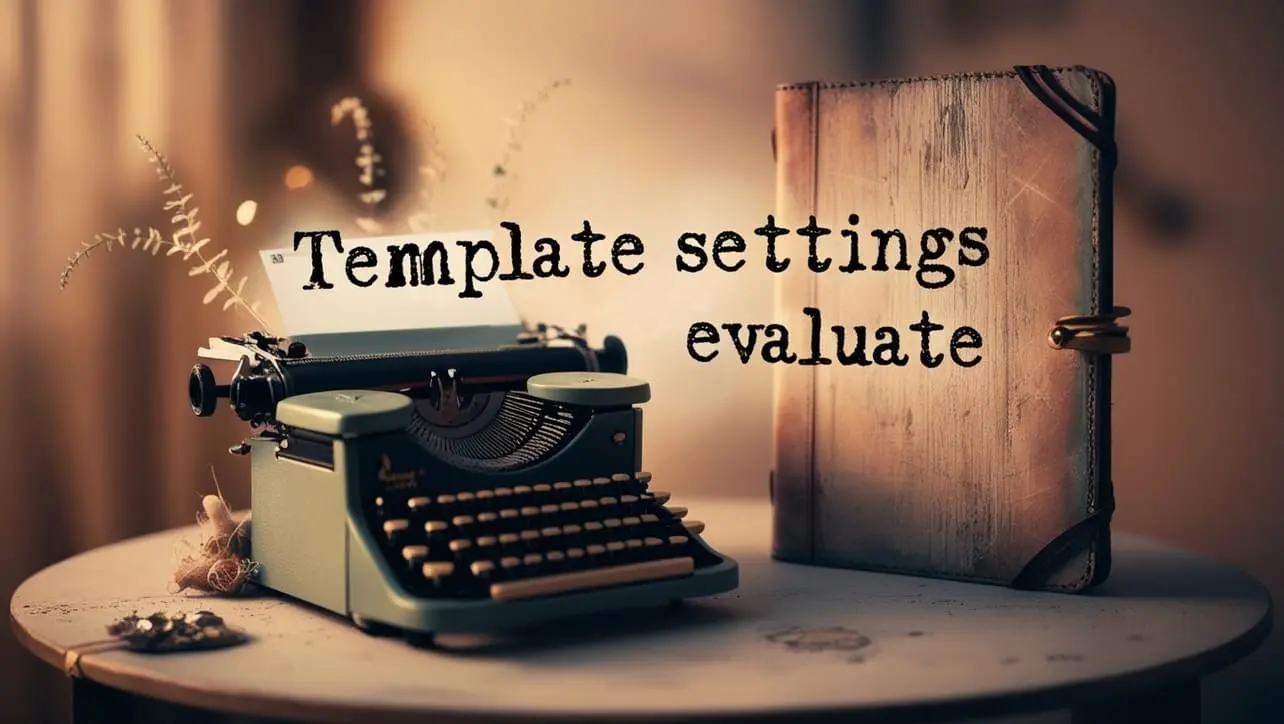
Lodash _.templateSettings.evaluate Property
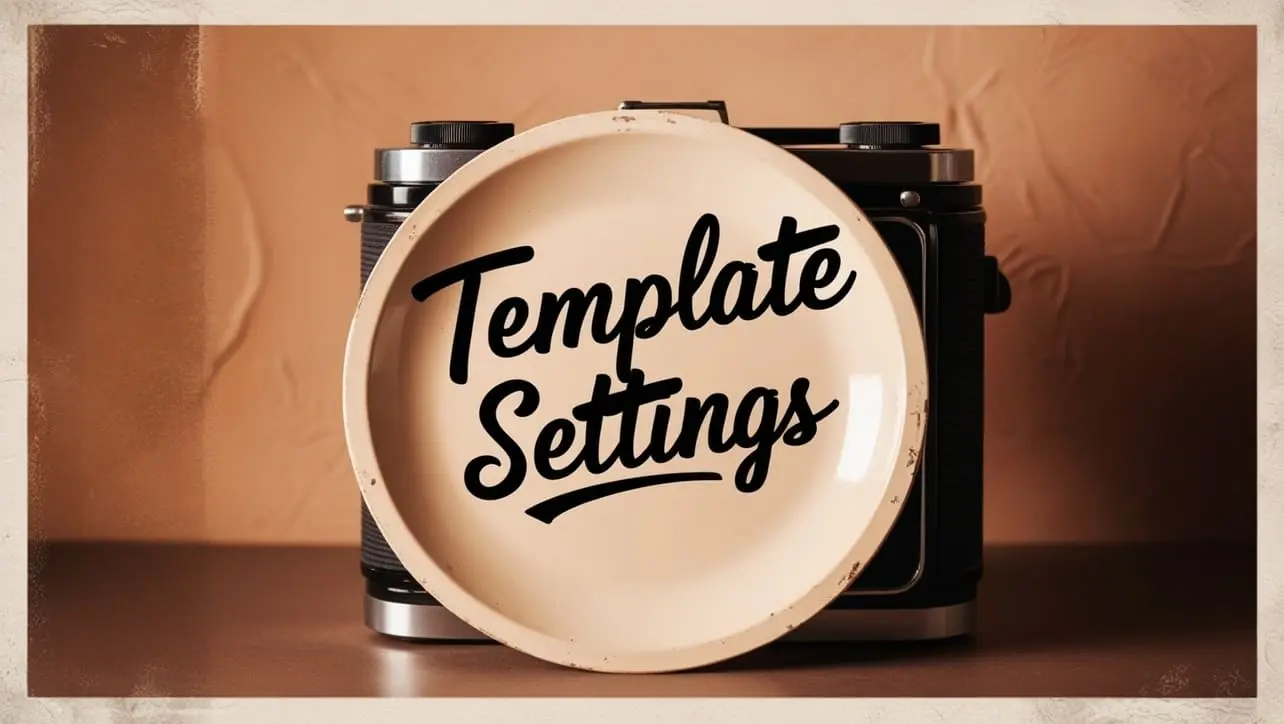
Lodash _.templateSettings Property
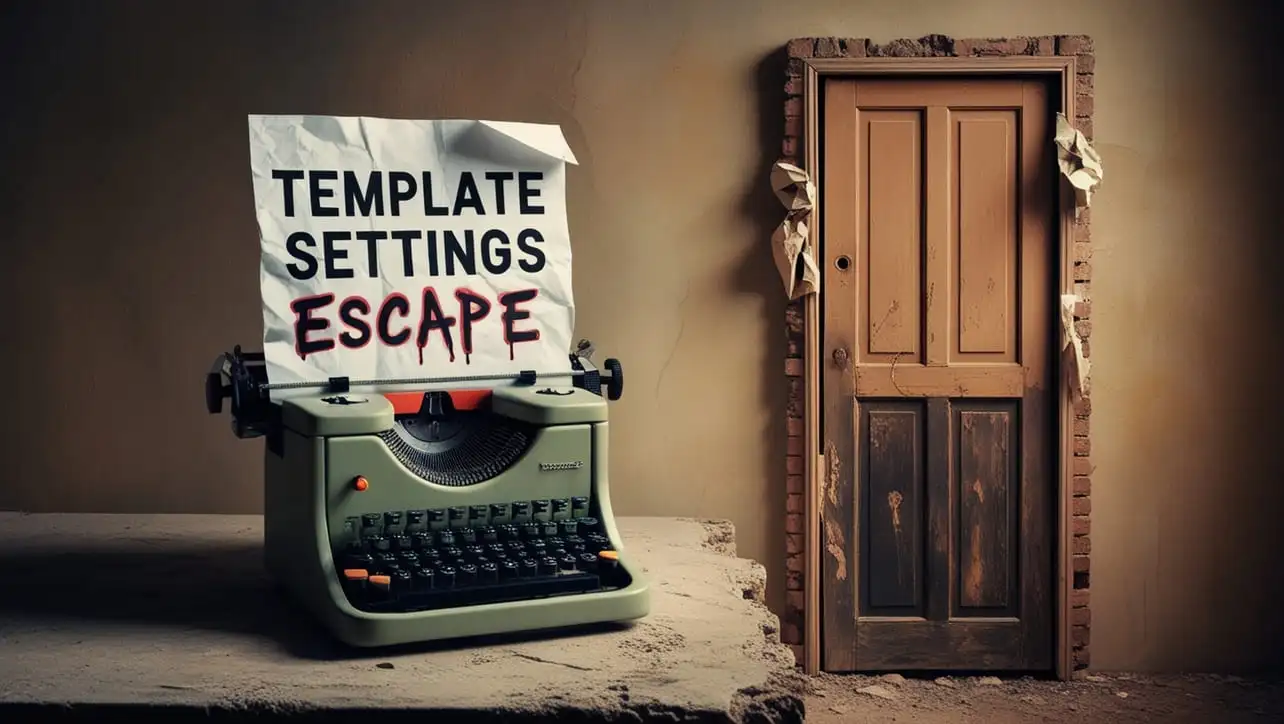
Lodash _.templateSettings.escape Property
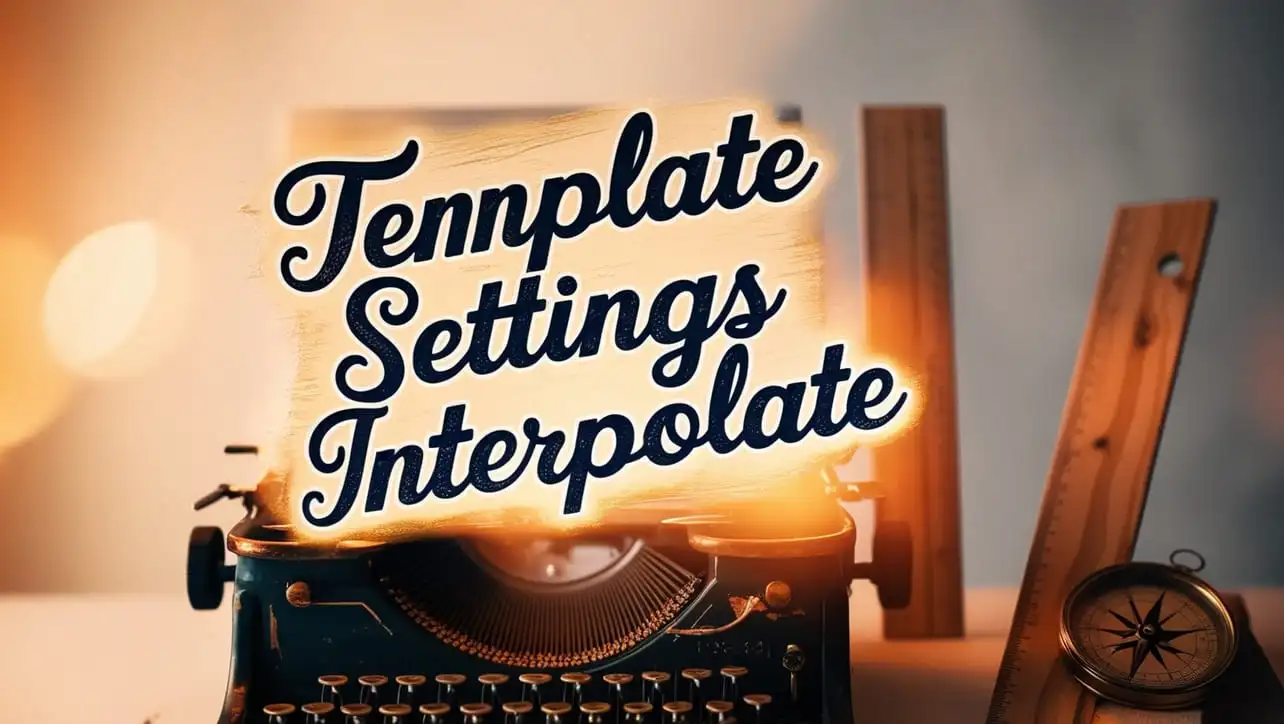
Lodash _.templateSettings.interpolate Property
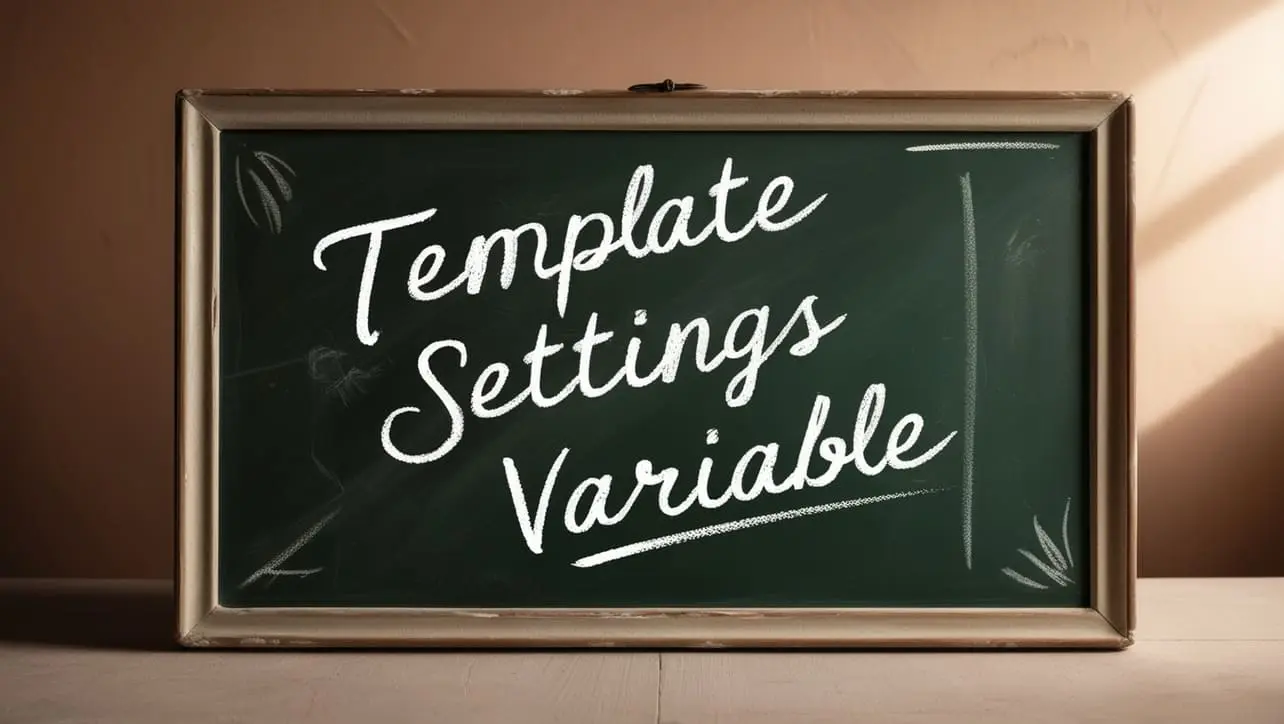
If you have any doubts regarding this article (Lodash _.isMatchWith() Lang Method), please comment here. I will help you immediately.