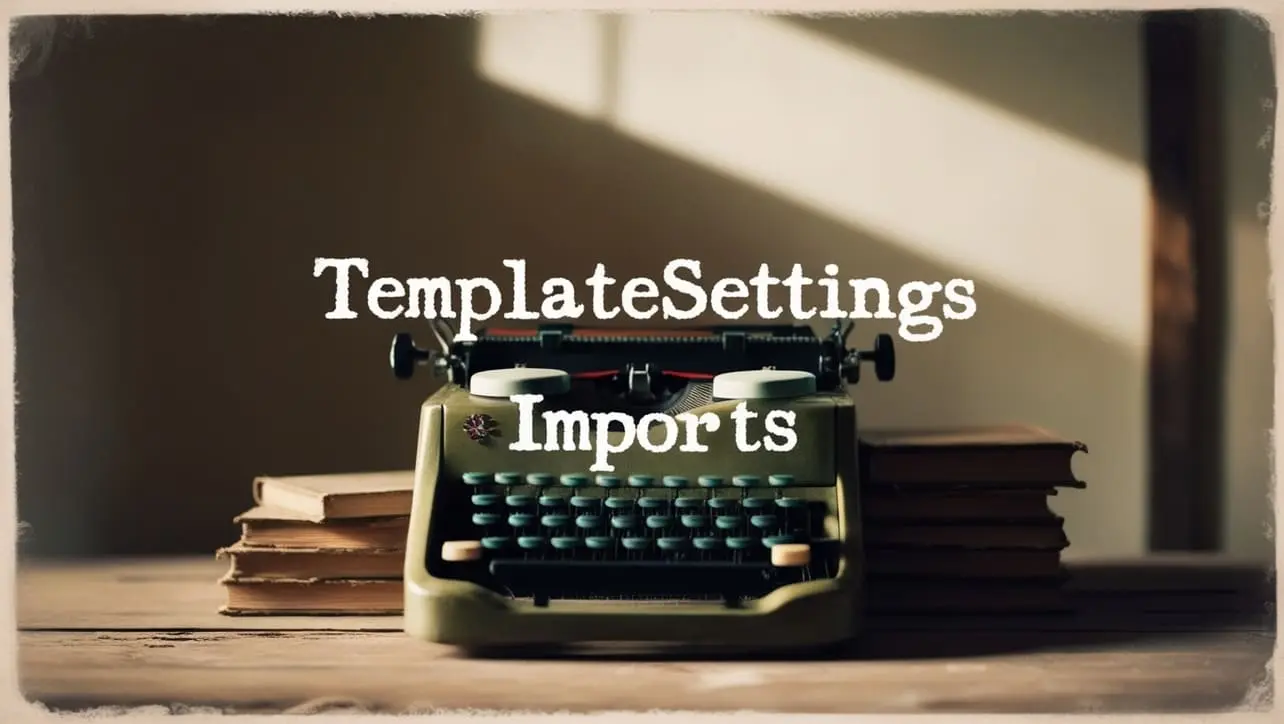
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isMatch() Lang Method
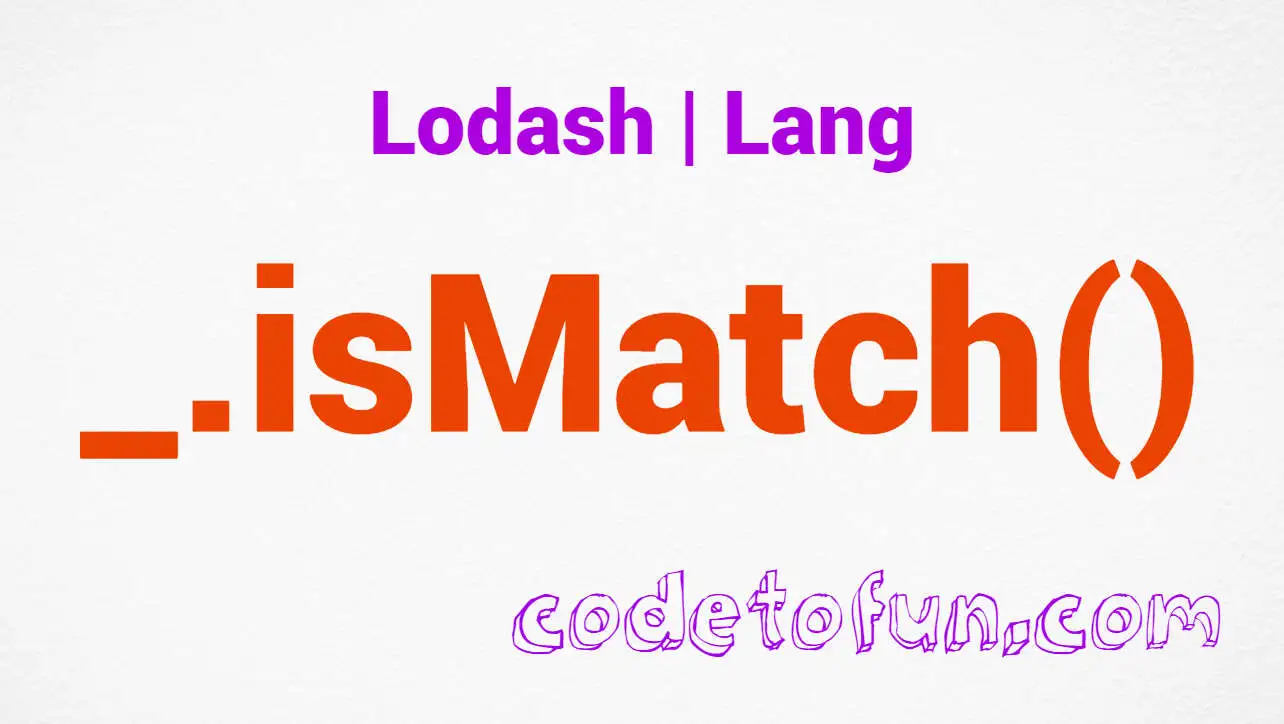
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript programming, comparing objects for equality is a common task. Lodash, a feature-rich utility library, provides the _.isMatch()
method as a reliable tool for determining whether an object has the same key-value pairs as another.
This method simplifies the process of object comparison, offering flexibility and accuracy for developers navigating complex data structures.
🧠 Understanding _.isMatch() Method
The _.isMatch()
method in Lodash checks if the provided object has the same key-value pairs as the specified source object. It returns true if the object matches, and false otherwise. This method is particularly useful when verifying whether an object contains specific properties and values.
💡 Syntax
The syntax for the _.isMatch()
method is straightforward:
_.isMatch(object, source)
- object: The object to inspect.
- source: The object of property values to match.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isMatch()
method:
const _ = require('lodash');
const user = { name: 'John', age: 25, city: 'New York' };
const criteria = { age: 25, city: 'New York' };
const isMatch = _.isMatch(user, criteria);
console.log(isMatch);
// Output: true
In this example, _.isMatch()
compares the user object with the specified criteria, confirming that it contains matching key-value pairs.
🏆 Best Practices
When working with the _.isMatch()
method, consider the following best practices:
Use Case-Specific Criteria:
Tailor the source object to match the specific criteria you are interested in. This ensures that the comparison aligns with your application's requirements.
example.jsCopiedconst book = { title: 'The Hobbit', author: 'J.R.R. Tolkien', genre: 'Fantasy' }; const criteriaForFantasy = { genre: 'Fantasy' }; const isFantasyBook = _.isMatch(book, criteriaForFantasy); console.log(isFantasyBook); // Output: true
Nested Object Comparison:
_.isMatch()
supports nested object comparison. If your criteria involve nested structures, the method can handle these scenarios.example.jsCopiedconst nestedObject = { details: { color: 'blue', size: 'medium' } }; const criteriaForSize = { details: { size: 'medium' } }; const hasMatchingSize = _.isMatch(nestedObject, criteriaForSize); console.log(hasMatchingSize); // Output: true
Array of Criteria:
You can use an array of source objects to check if the object matches any of the specified criteria. This is useful for scenarios where multiple sets of criteria are possible.
example.jsCopiedconst product = { name: 'Laptop', brand: 'ABC', price: 1200 }; const possibleCriteria = [ { brand: 'ABC', price: 1200 }, { name: 'Laptop', price: 1000 } ]; const matchesAnyCriteria = _.some(possibleCriteria, criteria => _.isMatch(product, criteria)); console.log(matchesAnyCriteria); // Output: true
📚 Use Cases
Validating User Input:
When handling user input,
_.isMatch()
can be employed to validate whether the provided object matches the expected structure.example.jsCopiedconst userInput = { username: 'john_doe', email: 'john@example.com' }; const expectedStructure = { username: String, email: String }; const isValidInput = _.isMatch(userInput, expectedStructure); console.log(isValidInput); // Output: true
Filtering Objects:
In scenarios where you need to filter an array of objects based on certain criteria,
_.isMatch()
can help identify matching objects.example.jsCopiedconst products = [ { name: 'Phone', brand: 'X', price: 800 }, { name: 'Laptop', brand: 'Y', price: 1200 }, { name: 'Tablet', brand: 'X', price: 500 } ]; const criteriaForXBrand = { brand: 'X' }; const filteredProducts = _.filter(products, product => _.isMatch(product, criteriaForXBrand)); console.log(filteredProducts); // Output: [{ name: 'Phone', brand: 'X', price: 800 }, { name: 'Tablet', brand: 'X', price: 500 }]
Configuration Matching:
When working with configurations or settings,
_.isMatch()
can be utilized to check if a given object matches a predefined configuration.example.jsCopiedconst defaultConfig = { theme: 'light', fontSize: 16 }; const userConfig = { theme: 'dark', fontSize: 16 }; const matchesDefaultConfig = _.isMatch(userConfig, defaultConfig); console.log(matchesDefaultConfig); // Output: false
🎉 Conclusion
The _.isMatch()
method in Lodash provides a versatile and efficient solution for comparing objects based on key-value pairs. Whether you are validating user input, filtering arrays, or checking configurations, this method offers a reliable tool for simplifying object comparison in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isMatch()
method in your Lodash projects.
👨💻 Join our Community:
Author
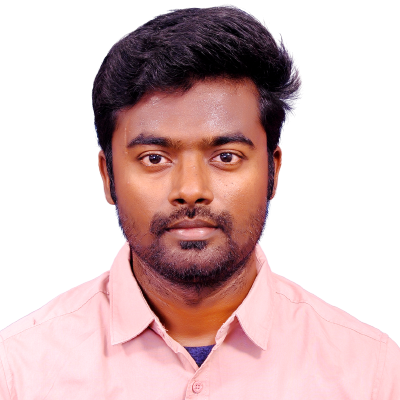
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
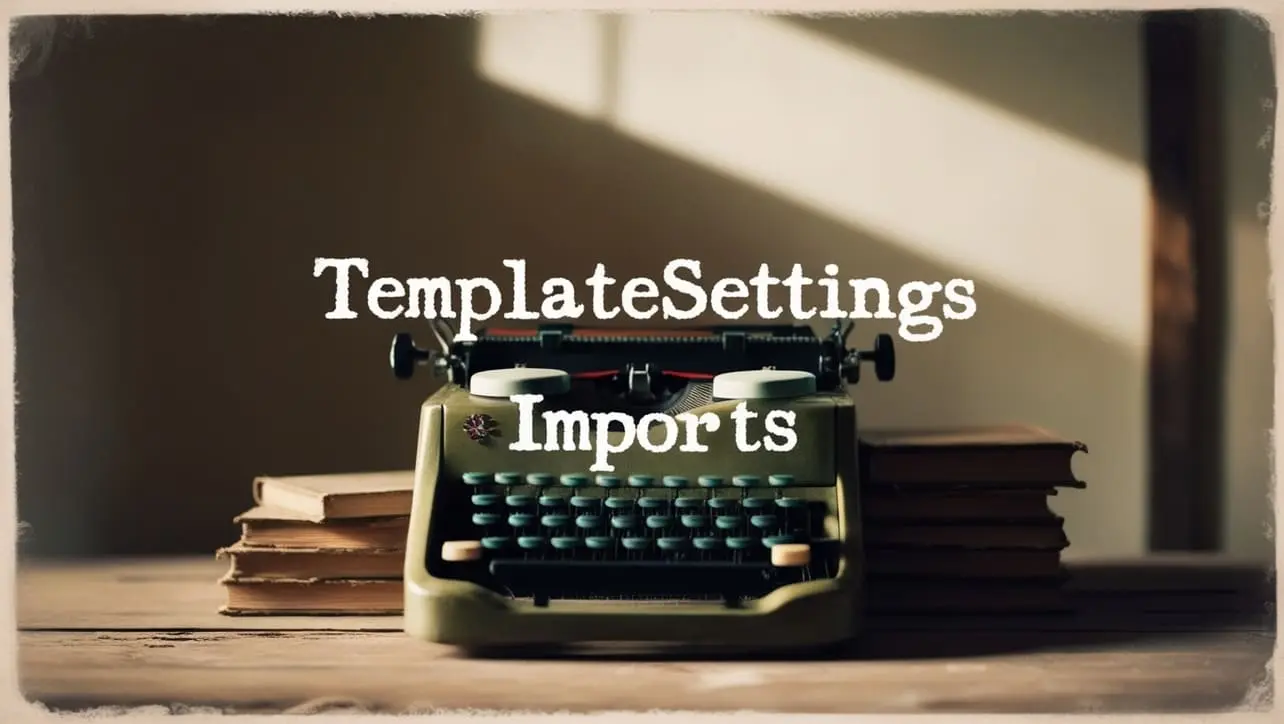
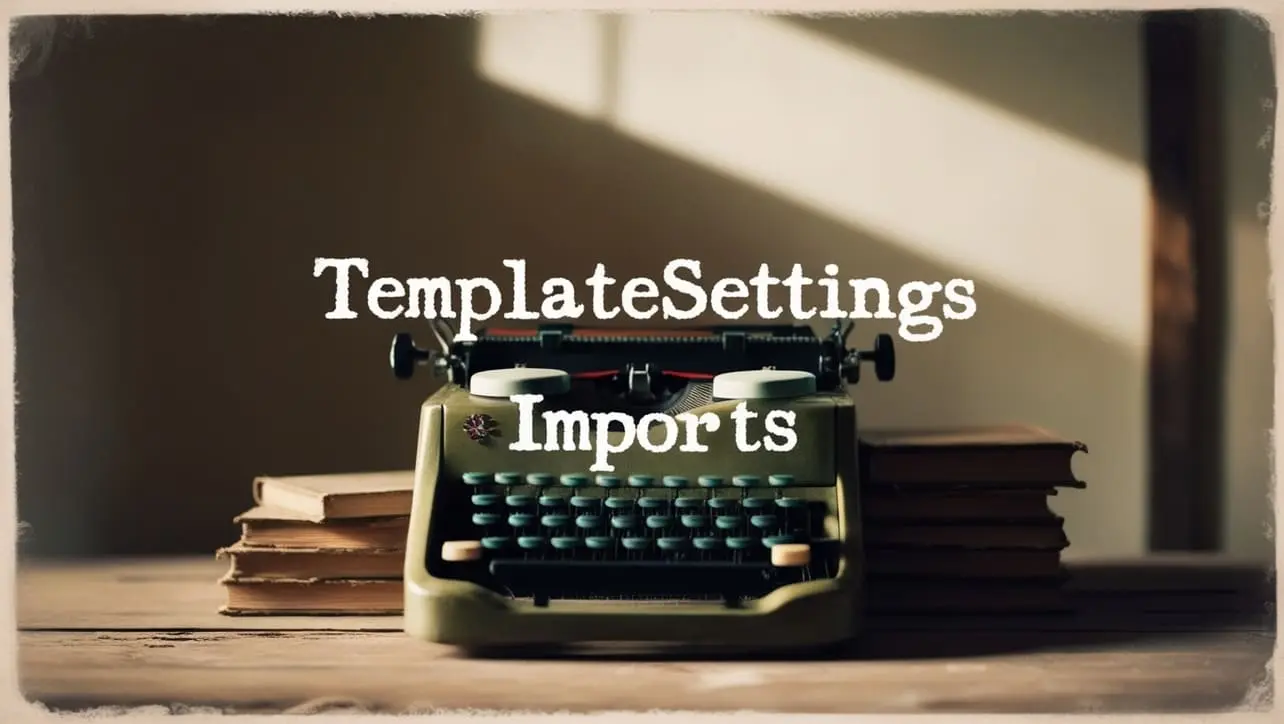
Lodash _.templateSettings.imports Property
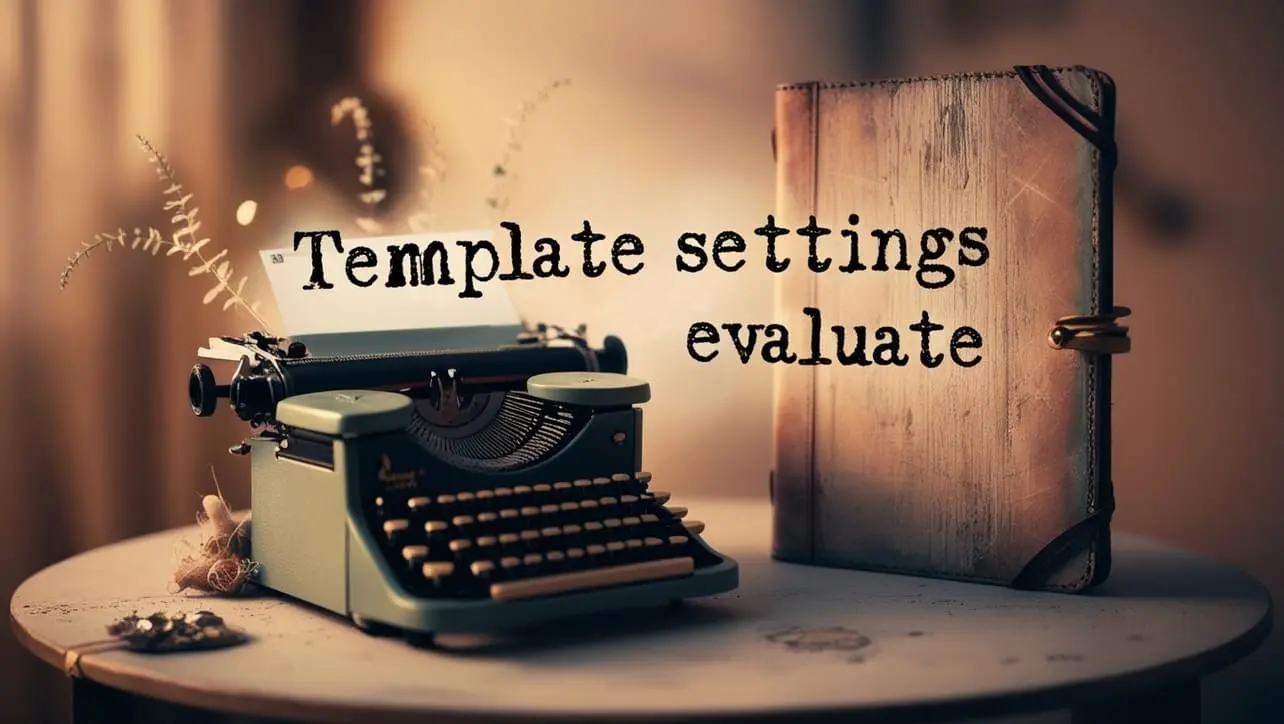
Lodash _.templateSettings.evaluate Property
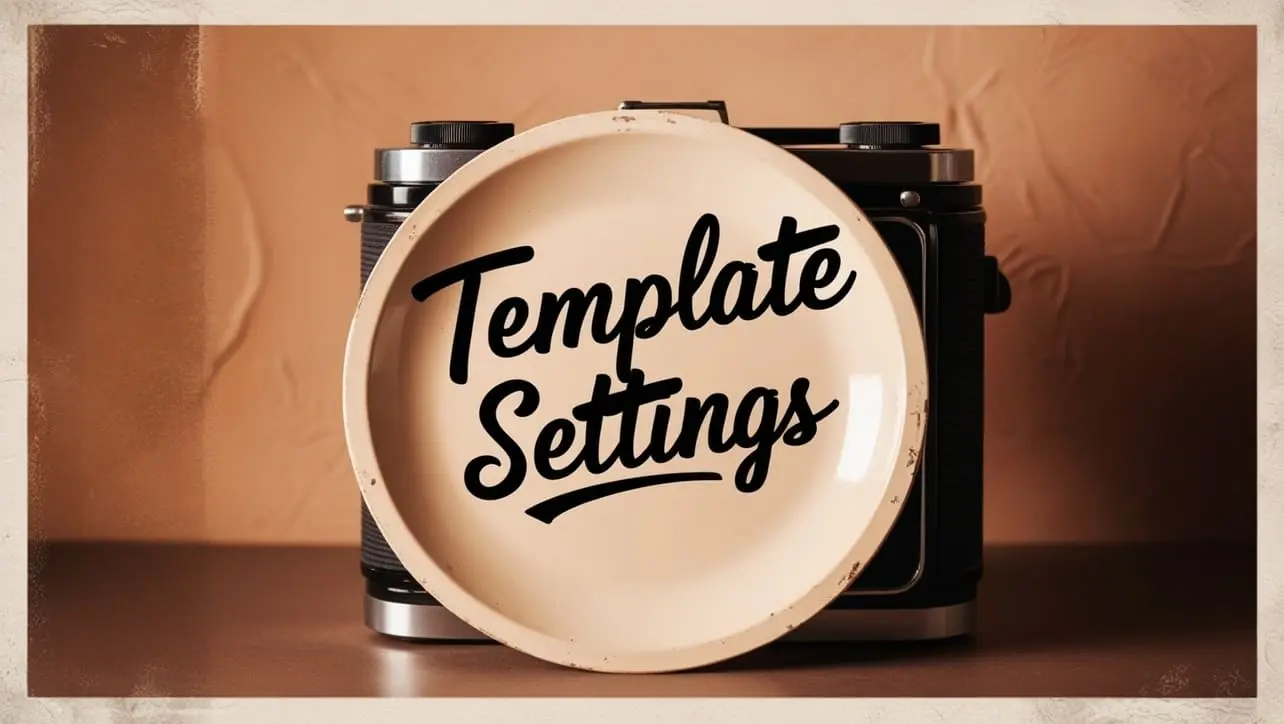
Lodash _.templateSettings Property
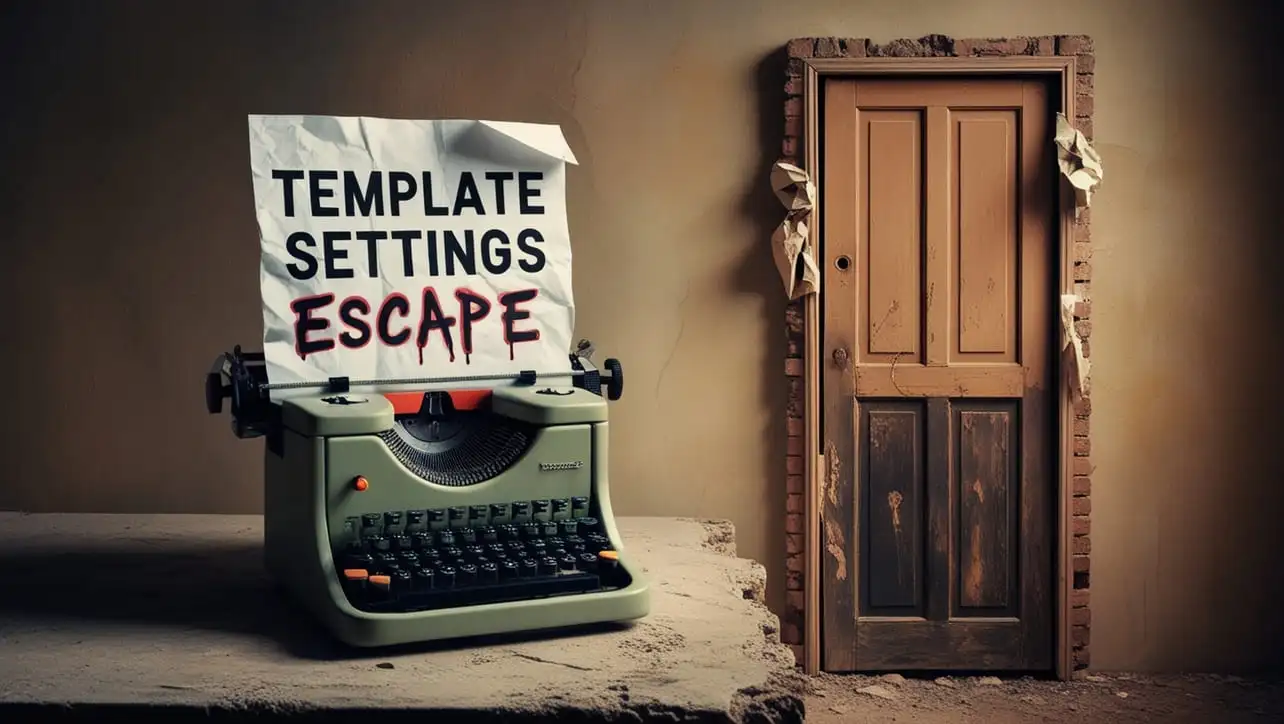
Lodash _.templateSettings.escape Property
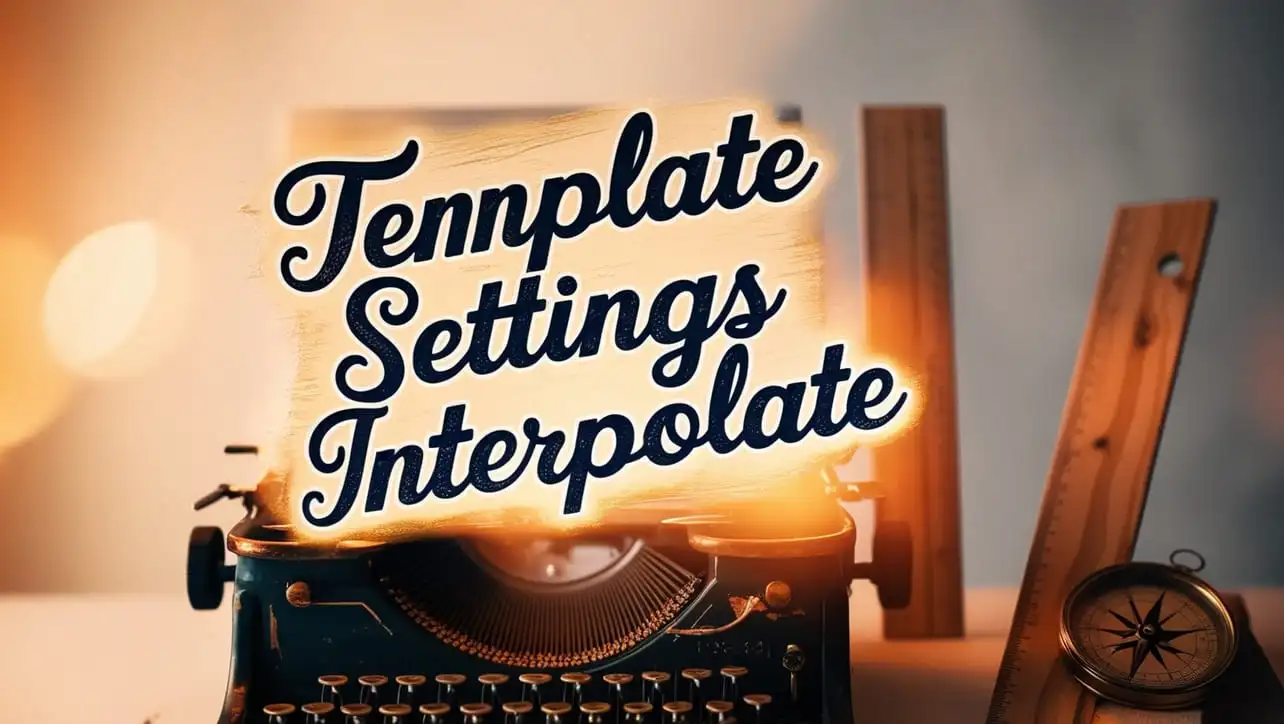
Lodash _.templateSettings.interpolate Property
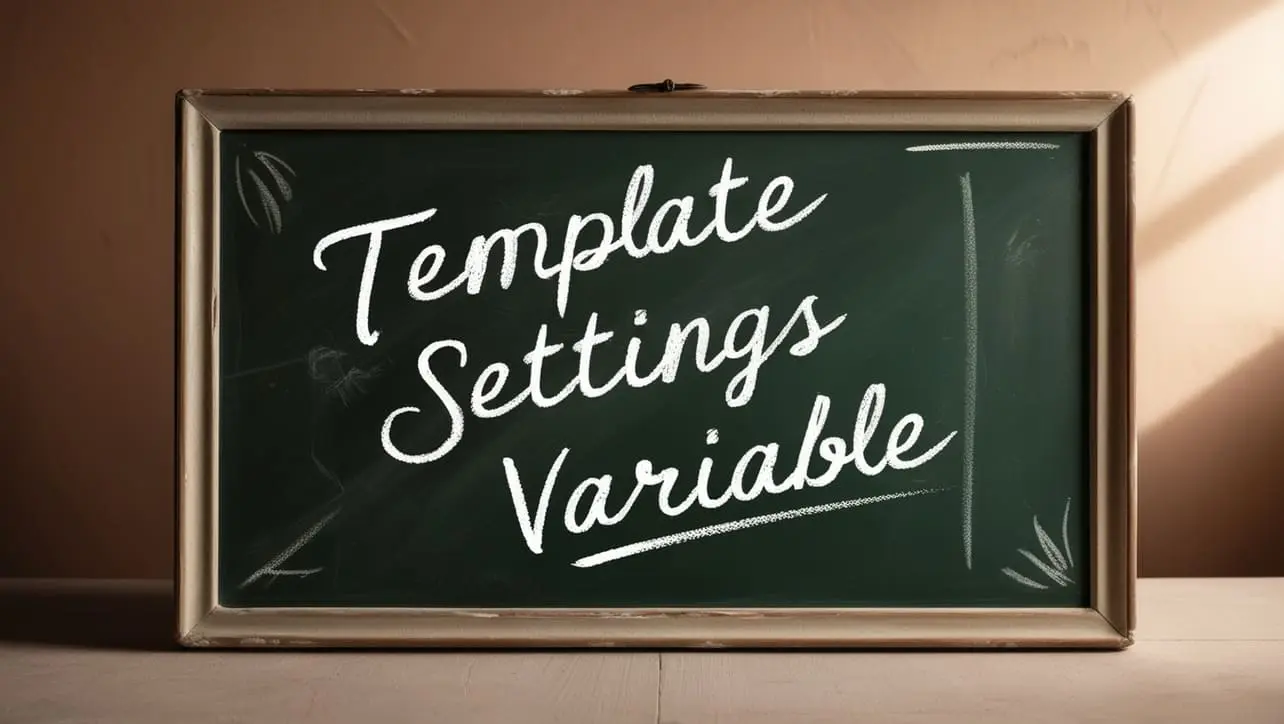
If you have any doubts regarding this article (Lodash _.isMatch() Lang Method), please comment here. I will help you immediately.