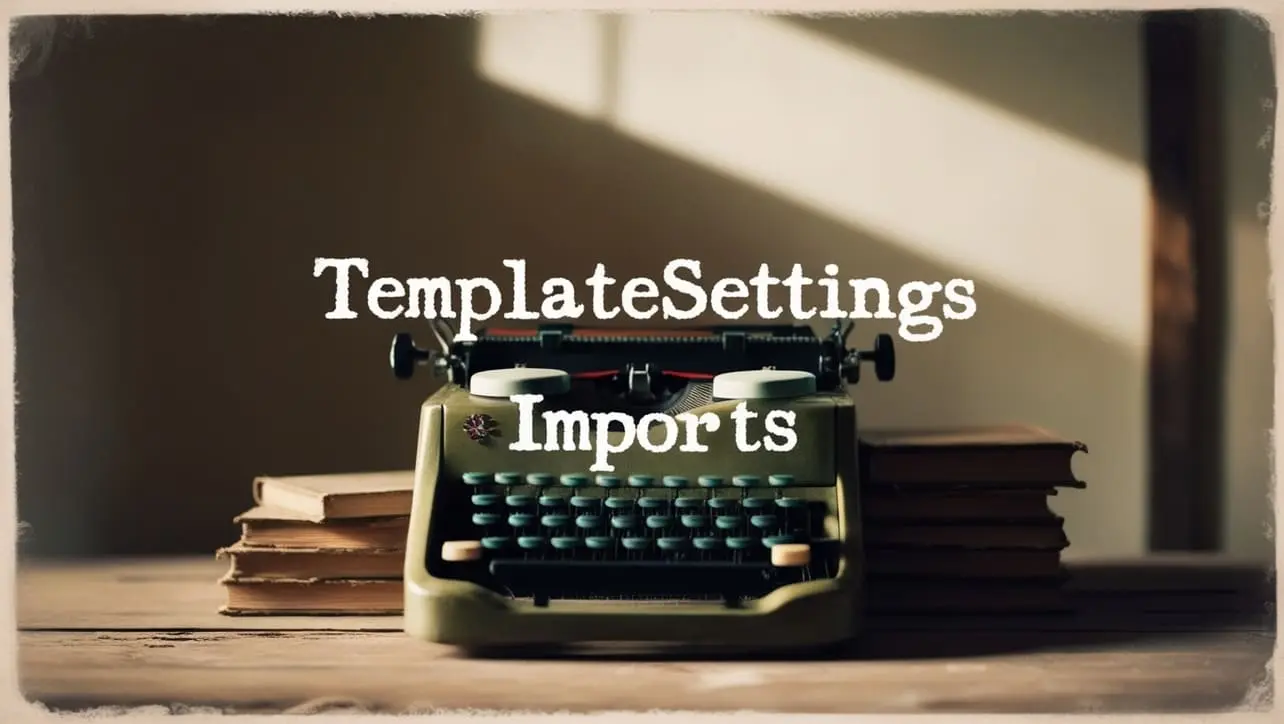
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isLength() Lang Method
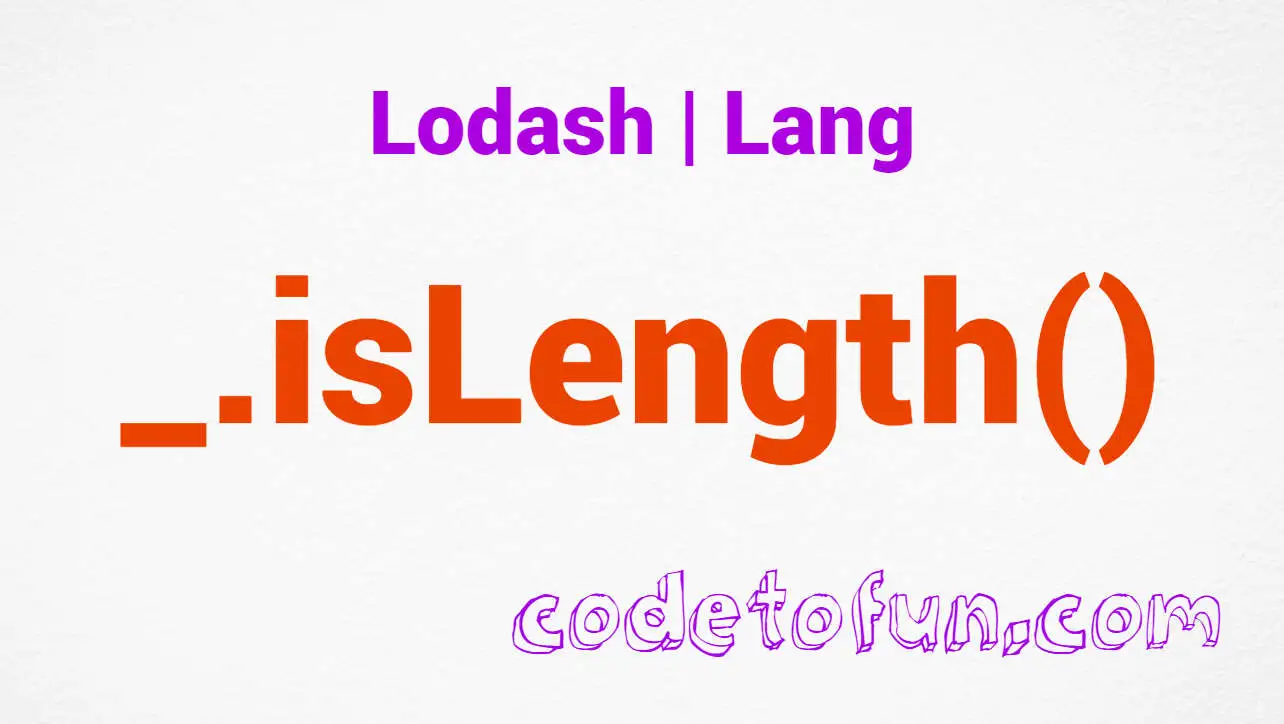
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript programming, validating the length of data structures is a common task. Lodash, the utility library, provides a helpful method for precisely this purpose: _.isLength()
.
This method allows developers to check whether a given value is a valid array-like length, ensuring robust data validation in their applications.
🧠 Understanding _.isLength() Method
The _.isLength()
method in Lodash is designed to determine whether a given value is a valid array-like length. It helps developers avoid common pitfalls related to length validation, offering a reliable solution for checking if a value can be safely used as the length property of an array or array-like object.
💡 Syntax
The syntax for the _.isLength()
method is straightforward:
_.isLength(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isLength()
method:
const _ = require('lodash');
const validLength = 42;
const invalidLength = 'not a length';
console.log(_.isLength(validLength)); // Output: true
console.log(_.isLength(invalidLength)); // Output: false
In this example, _.isLength()
confirms that validLength is a valid array-like length, while invalidLength is not.
🏆 Best Practices
When working with the _.isLength()
method, consider the following best practices:
Validate Length Before Array Operations:
Use
_.isLength()
to validate length before performing array operations. This helps prevent errors and ensures that the length property adheres to the expected format.example.jsCopiedconst potentialLength = /* ...get length from user input or elsewhere... */ ; if (_.isLength(potentialLength)) { // Perform array operations using potentialLength const newArray = new Array(potentialLength); console.log(newArray); } else { console.error('Invalid length. Please provide a valid array-like length.'); }
Prevent Type Errors:
Avoid type errors by using
_.isLength()
to verify that a value is a valid array-like length before using it in operations that expect a length property.example.jsCopiedfunction createArrayWithLength(length) { if (_.isLength(length)) { return new Array(length); } else { throw new Error('Invalid length. Please provide a valid array-like length.'); } } const newArray = createArrayWithLength(10); console.log(newArray);
Validate Length in Iterative Processes:
When iterating over data structures, ensure that the length is valid using
_.isLength()
to prevent unexpected behavior during iteration.example.jsCopiedconst lengths = [5, 10, 15, 'not a length', 20]; lengths.forEach(length => { if (_.isLength(length)) { // Process data with valid length console.log(`Processing data with length: ${length}`); } else { console.error(`Invalid length: ${length}. Skipping.`); } });
📚 Use Cases
Array Initialization:
_.isLength()
is valuable when initializing arrays to ensure that the provided length is valid before creating the array.example.jsCopiedfunction initializeArrayWithLength(length) { if (_.isLength(length)) { return new Array(length).fill(null); } else { throw new Error('Invalid length. Please provide a valid array-like length.'); } } const initializedArray = initializeArrayWithLength(8); console.log(initializedArray);
Length Validation in Custom Functions:
Integrate
_.isLength()
in custom functions that involve length-related operations, ensuring that the provided lengths are valid.example.jsCopiedfunction performCustomOperation(data, length) { if (_.isLength(length)) { // Perform custom operation using data and length console.log(`Custom operation with data length: ${length}`); } else { throw new Error('Invalid length. Please provide a valid array-like length.'); } } const data = [1, 2, 3, 4, 5]; const customLength = 5; performCustomOperation(data, customLength);
Length Validation in Libraries or APIs:
When designing libraries or APIs that expect length parameters, use
_.isLength()
to validate the input and ensure compatibility with array-related operations.example.jsCopiedfunction processDataWithLength(data, length) { if (_.isLength(length)) { // Process data with valid length console.log(`Processing data with length: ${length}`); } else { throw new Error('Invalid length. Please provide a valid array-like length.'); } } const dataset = [ /* ...array of data... */ ]; const userProvidedLength = 'not a length'; try { processDataWithLength(dataset, userProvidedLength); } catch (error) { console.error(error.message); }
🎉 Conclusion
The _.isLength()
method in Lodash serves as a valuable tool for validating array-like lengths, helping developers build robust and error-resistant applications. By incorporating this method into your code, you can enhance the reliability of operations involving lengths and contribute to the overall stability of your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isLength()
method in your Lodash projects.
👨💻 Join our Community:
Author
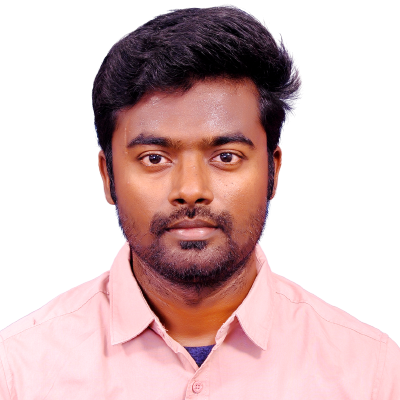
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
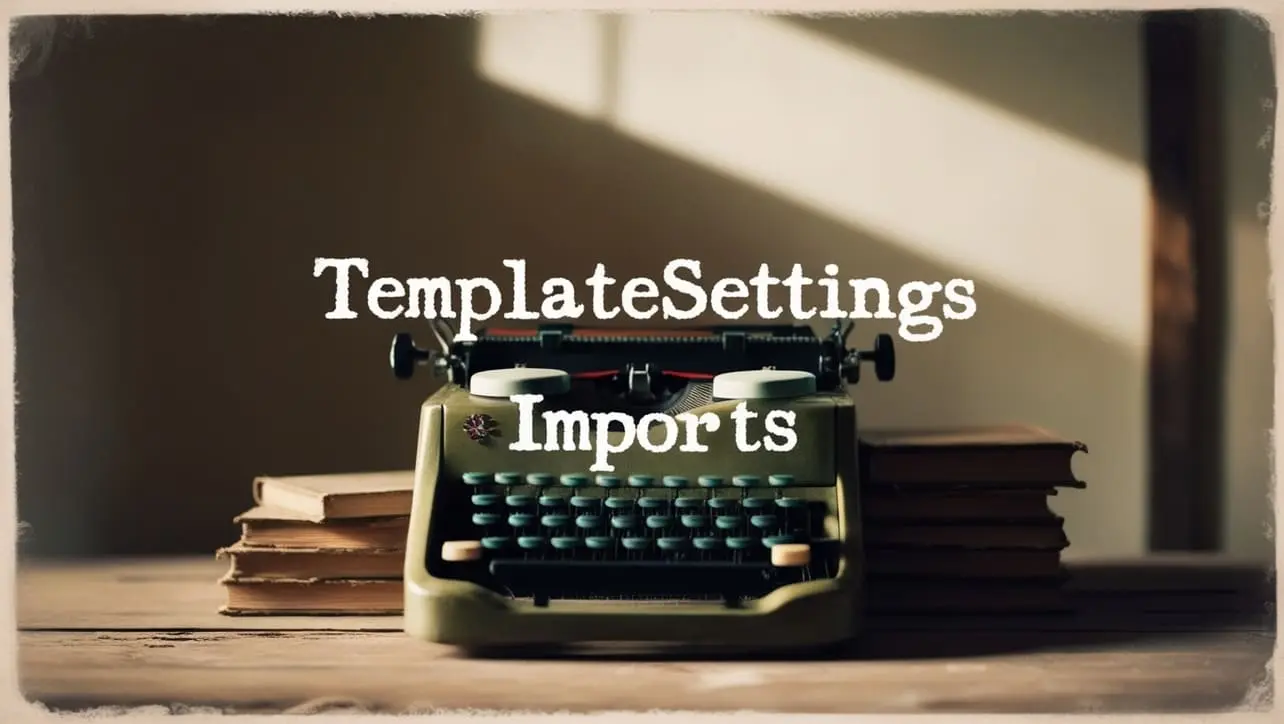
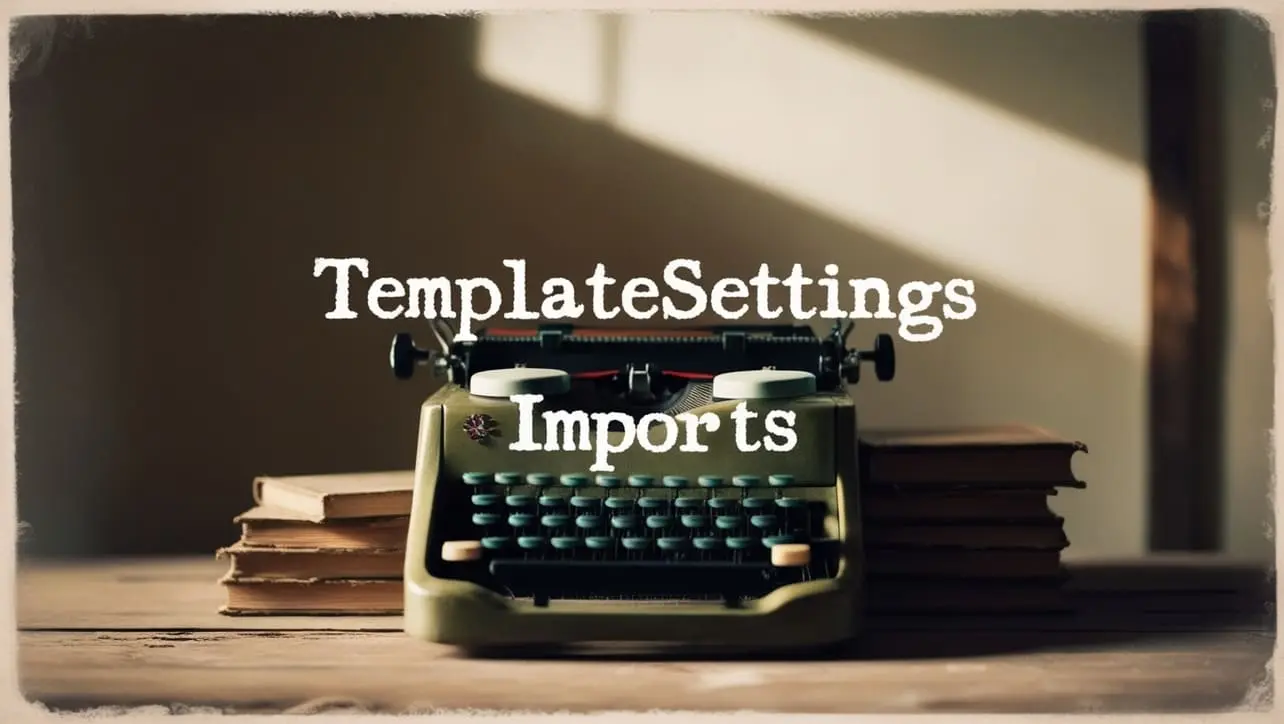
Lodash _.templateSettings.imports Property
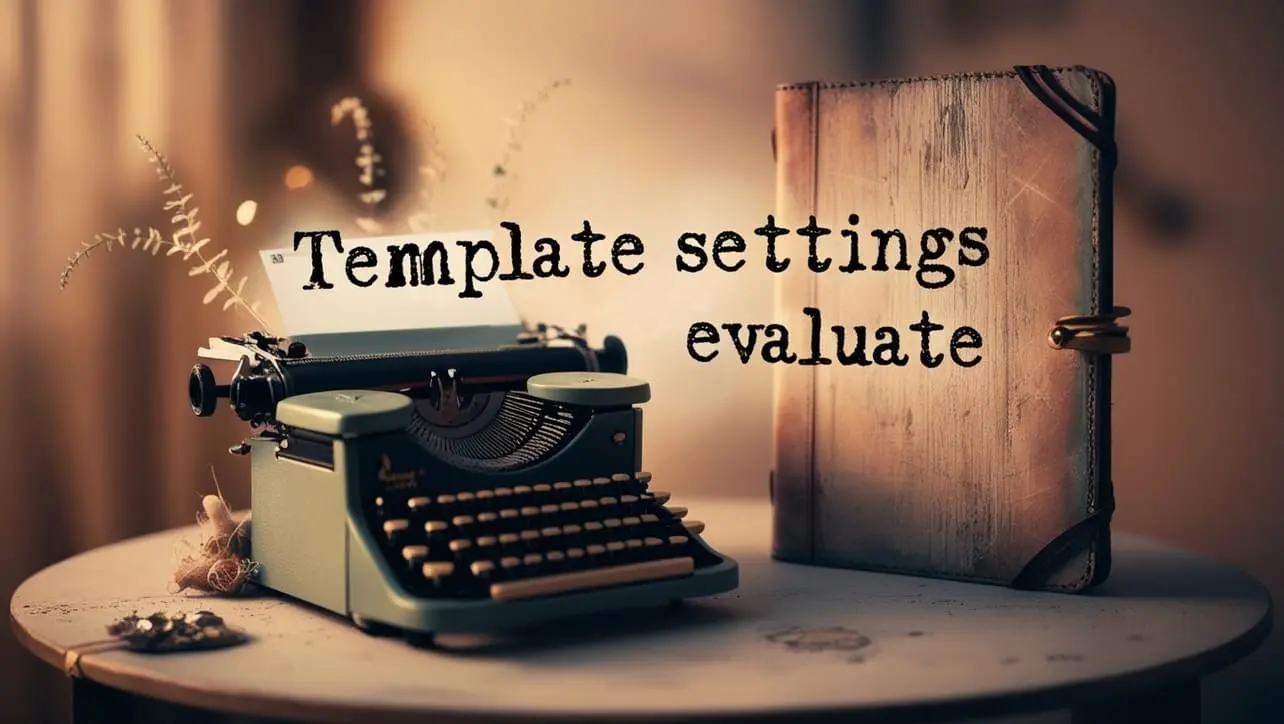
Lodash _.templateSettings.evaluate Property
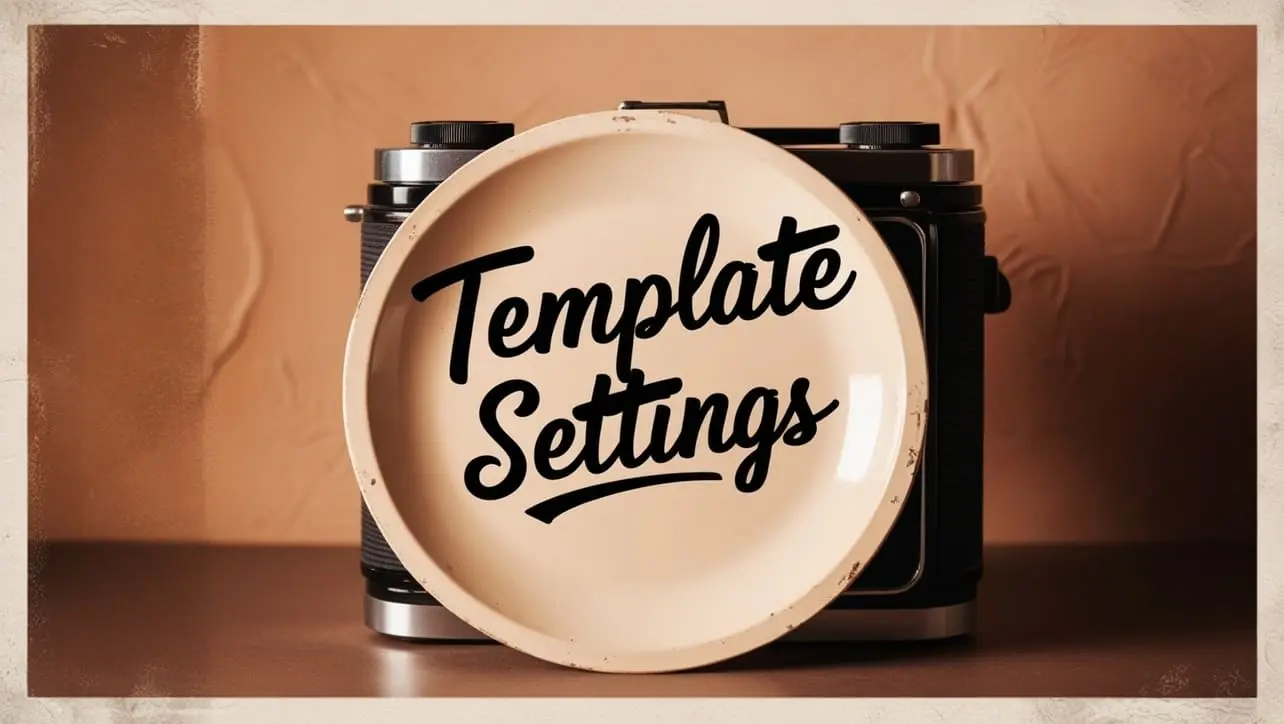
Lodash _.templateSettings Property
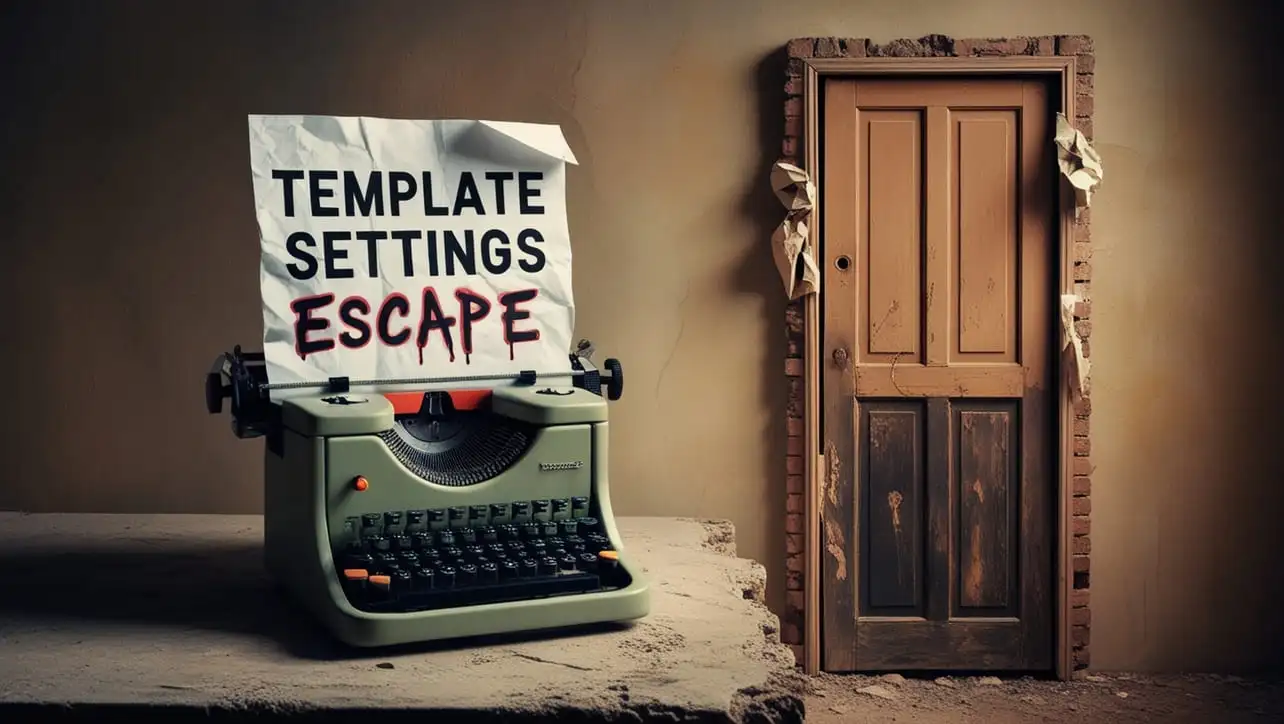
Lodash _.templateSettings.escape Property
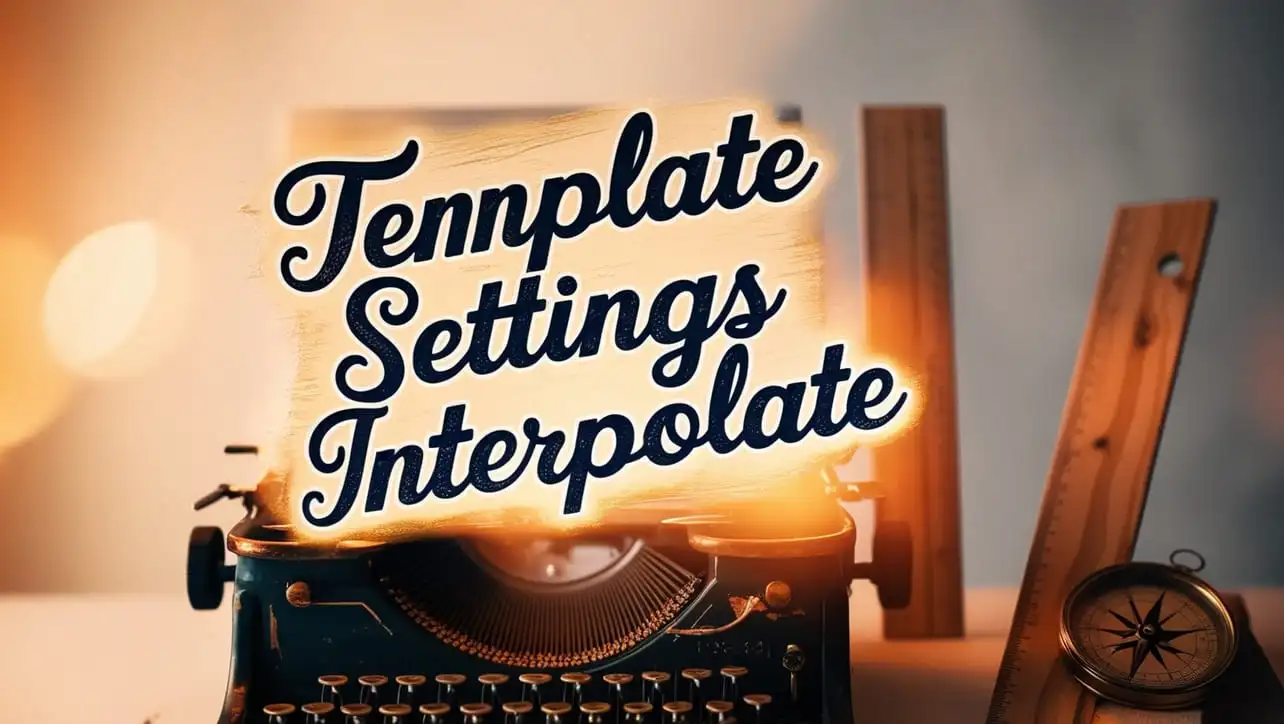
Lodash _.templateSettings.interpolate Property
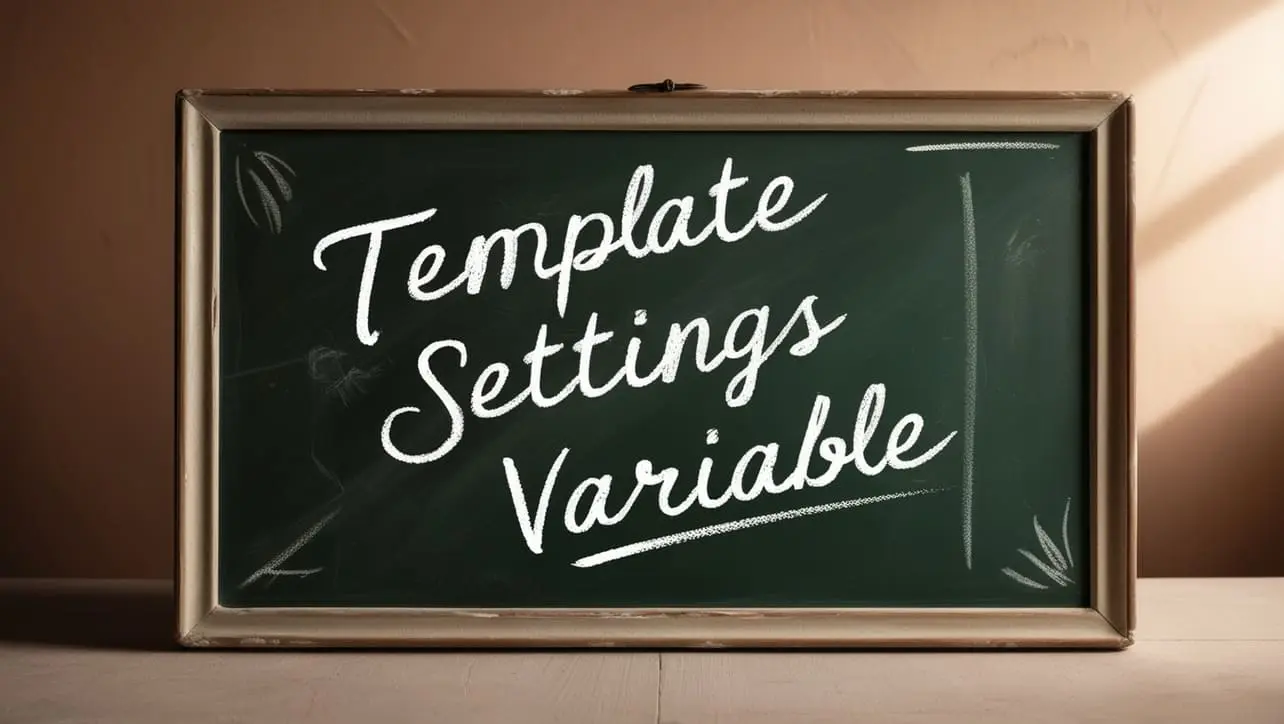
If you have any doubts regarding this article (Lodash _.isLength() Lang Method), please comment here. I will help you immediately.