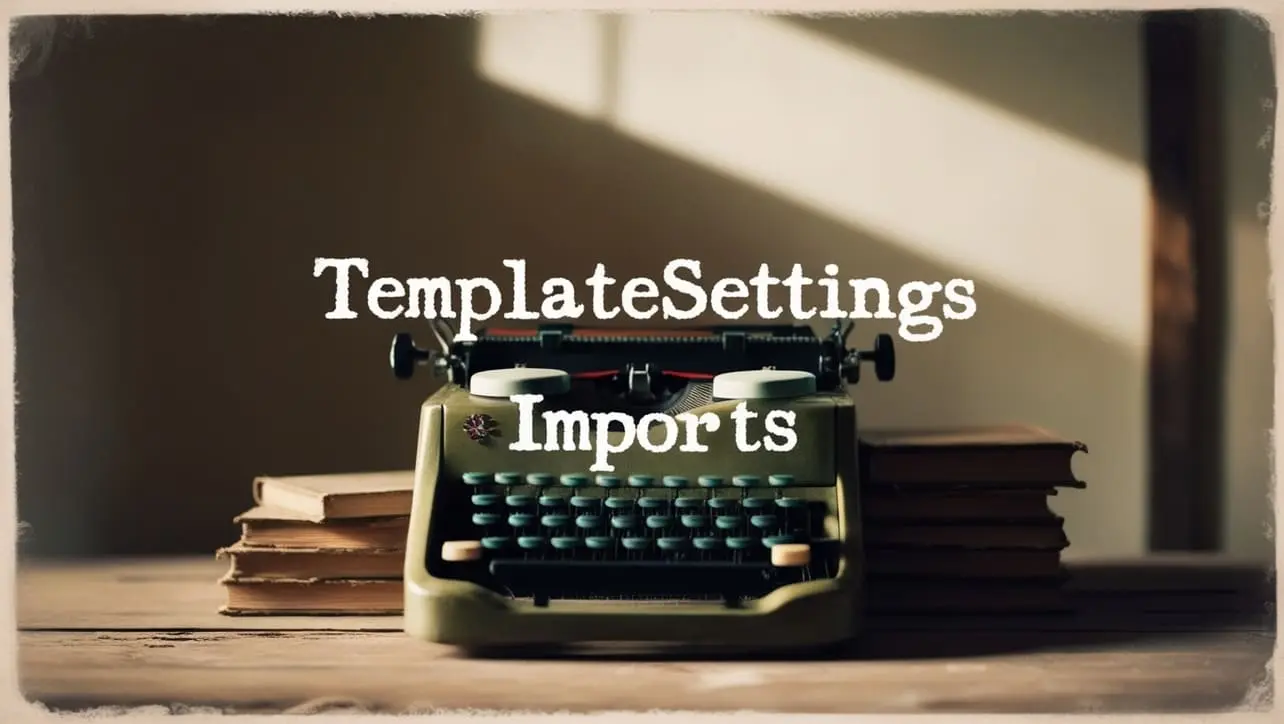
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isEmpty() Lang Method
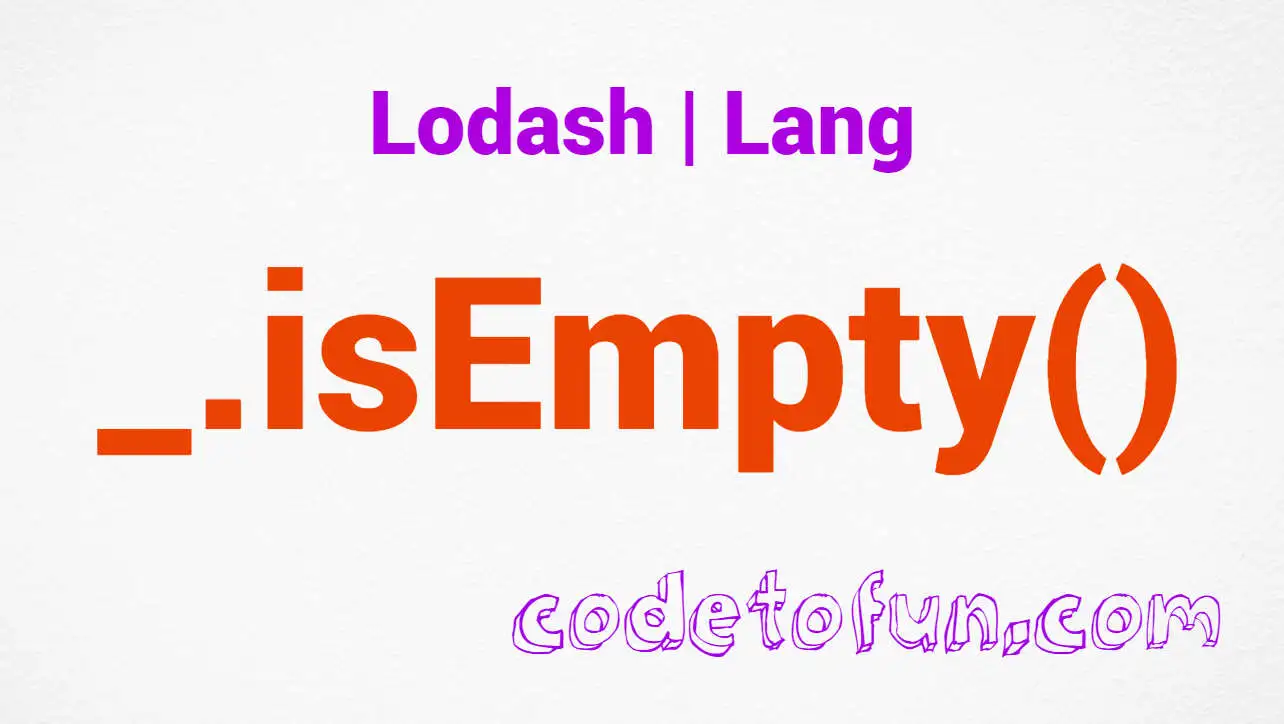
Photo Credit to CodeToFun
🙋 Introduction
When working with JavaScript objects and data structures, checking for emptiness is a common task. Lodash, a powerful utility library, provides the _.isEmpty()
method, a simple yet effective tool for determining whether a given value is considered empty.
This method is particularly handy when dealing with objects, arrays, and strings, offering a concise solution for developers aiming to enhance code clarity and maintainability.
🧠 Understanding _.isEmpty() Method
The _.isEmpty()
method in Lodash is designed to check if a value is empty. It returns true if the value is empty, false otherwise. The method works seamlessly with a variety of data types, including objects, arrays, strings, and more.
💡 Syntax
The syntax for the _.isEmpty()
method is straightforward:
_.isEmpty(value)
- value: The value to check for emptiness.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isEmpty()
method:
const _ = require('lodash');
const emptyArray = [];
const nonEmptyArray = [1, 2, 3];
console.log(_.isEmpty(emptyArray)); // Output: true
console.log(_.isEmpty(nonEmptyArray)); // Output: false
In this example, _.isEmpty()
correctly identifies the empty array as true and the non-empty array as false.
🏆 Best Practices
When working with the _.isEmpty()
method, consider the following best practices:
Consistent Handling of Edge Cases:
Use
_.isEmpty()
to consistently handle edge cases where checking for emptiness is essential. This method provides a uniform approach across different data types.example.jsCopiedconst checkEmptiness = (data) => { if (_.isEmpty(data)) { console.log('Data is empty'); } else { console.log('Data is not empty'); } }; checkEmptiness([]); // Output: Data is empty checkEmptiness({}); // Output: Data is empty checkEmptiness(''); // Output: Data is empty
Avoid Length Checks for Strings:
When dealing with strings, prefer
_.isEmpty()
over length checks (str.length === 0). It handles empty strings more intuitively and is generally more readable.example.jsCopiedconst emptyString = ''; const nonEmptyString = 'Hello, Lodash!'; console.log(_.isEmpty(emptyString)); // Output: true console.log(_.isEmpty(nonEmptyString)); // Output: false
Check for Empty Objects:
Use
_.isEmpty()
to easily check if an object is empty. This is especially useful when dealing with dynamic data structures.example.jsCopiedconst emptyObject = {}; const nonEmptyObject = { key: 'value' }; console.log(_.isEmpty(emptyObject)); // Output: true console.log(_.isEmpty(nonEmptyObject)); // Output: false
📚 Use Cases
Input Validation:
When validating user input or external data,
_.isEmpty()
can help ensure that the provided values are not empty before further processing.example.jsCopiedconst userInput = /* ...get user input... */; if (_.isEmpty(userInput)) { console.error('Please provide valid input.'); } else { // Proceed with processing the input console.log('Processing:', userInput); }
Conditional Rendering:
In UI development, you might want to conditionally render elements based on whether certain data is empty or not.
_.isEmpty()
simplifies this check.example.jsCopiedconst dataToDisplay = /* ...fetch data from API or elsewhere... */; if (_.isEmpty(dataToDisplay)) { console.log('No data available.'); } else { // Render the data console.log('Rendering data:', dataToDisplay); }
Object Cleanup:
Before performing operations on an object, use
_.isEmpty()
to check if it's empty. This can help avoid unnecessary computations or iterations.example.jsCopiedconst dataObject = /* ...get data object... */; if (_.isEmpty(dataObject)) { console.log('No data to process.'); } else { // Process the data console.log('Processing data:', dataObject); }
🎉 Conclusion
The _.isEmpty()
method in Lodash provides a versatile and consistent approach to checking for emptiness across various data types. Whether you're validating input, conditionally rendering elements, or cleaning up objects, this method simplifies the code and contributes to a more expressive and maintainable JavaScript codebase.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isEmpty()
method in your Lodash projects.
👨💻 Join our Community:
Author
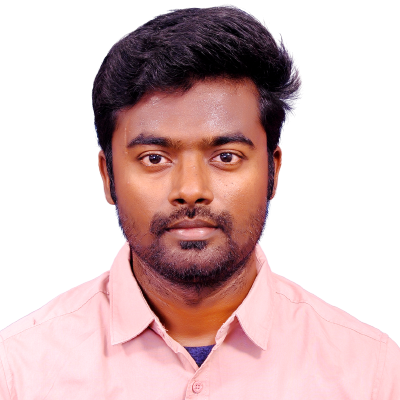
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
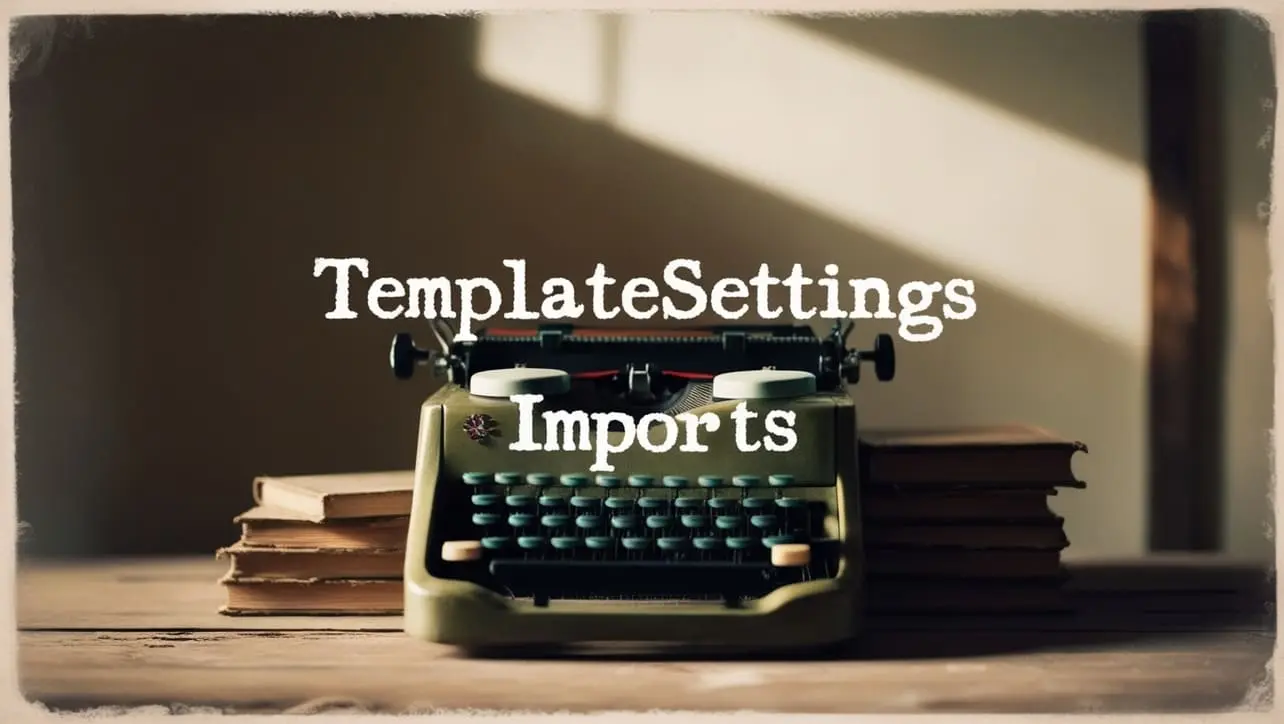
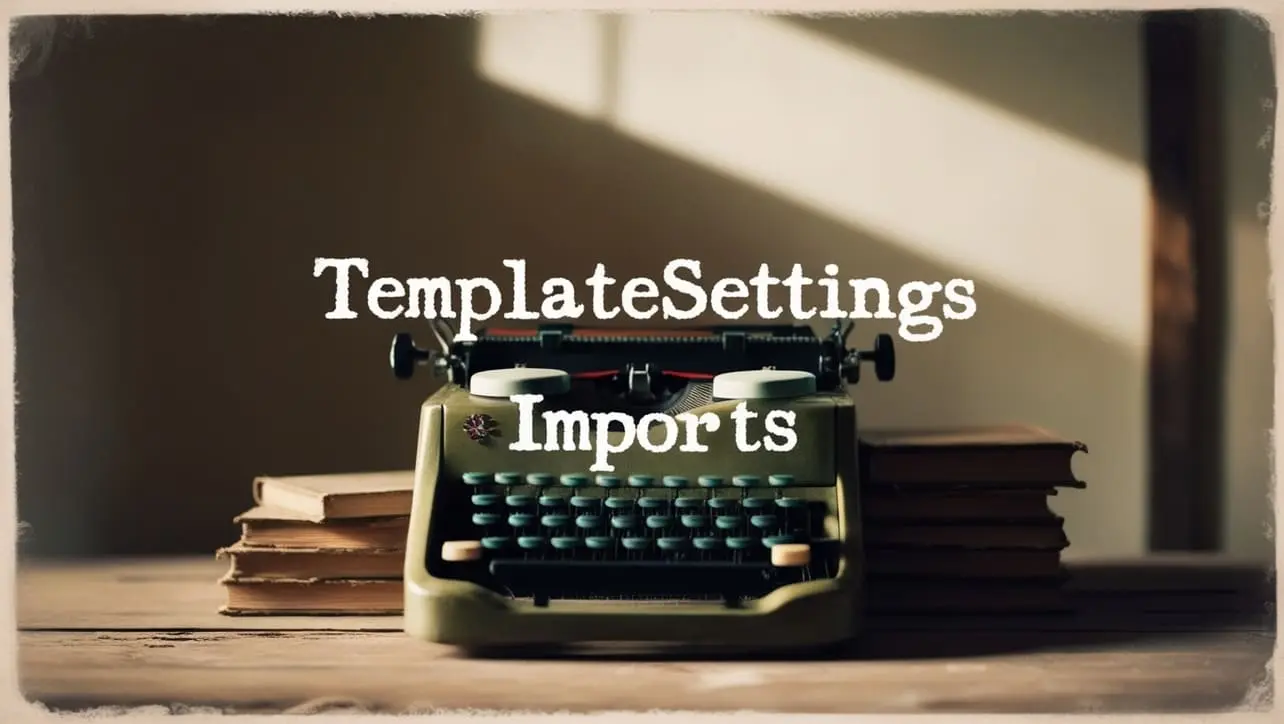
Lodash _.templateSettings.imports Property
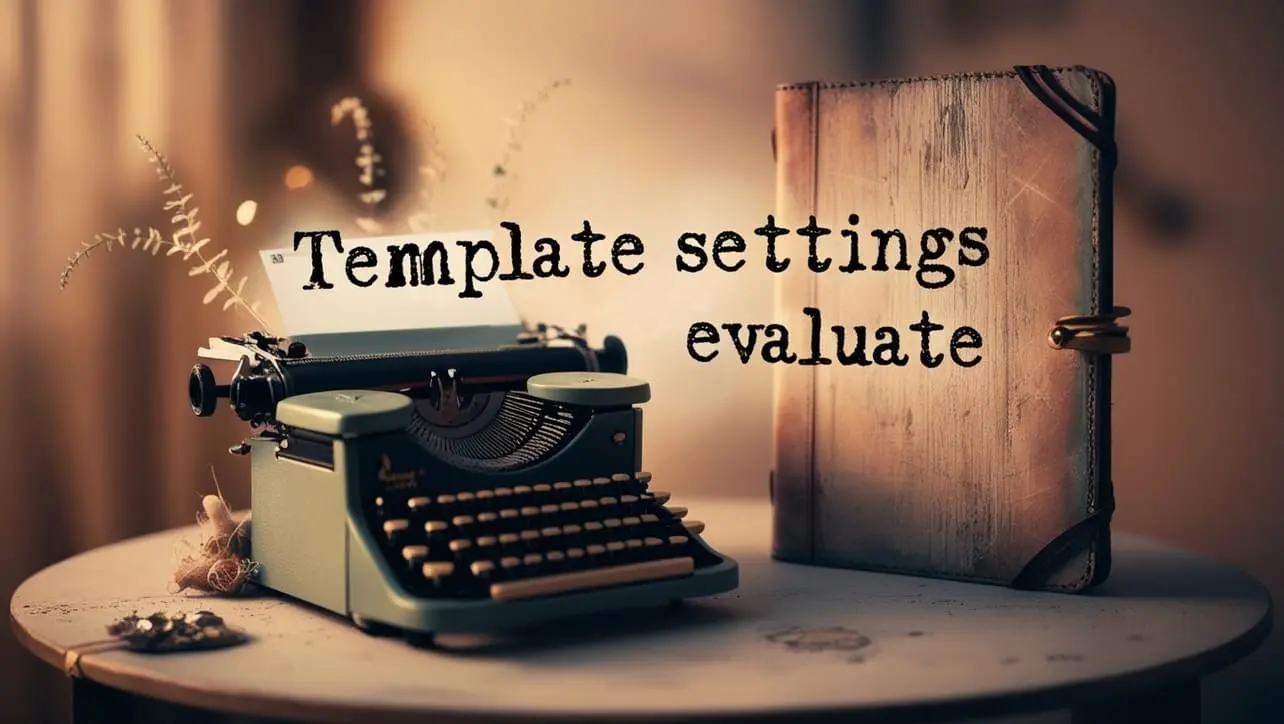
Lodash _.templateSettings.evaluate Property
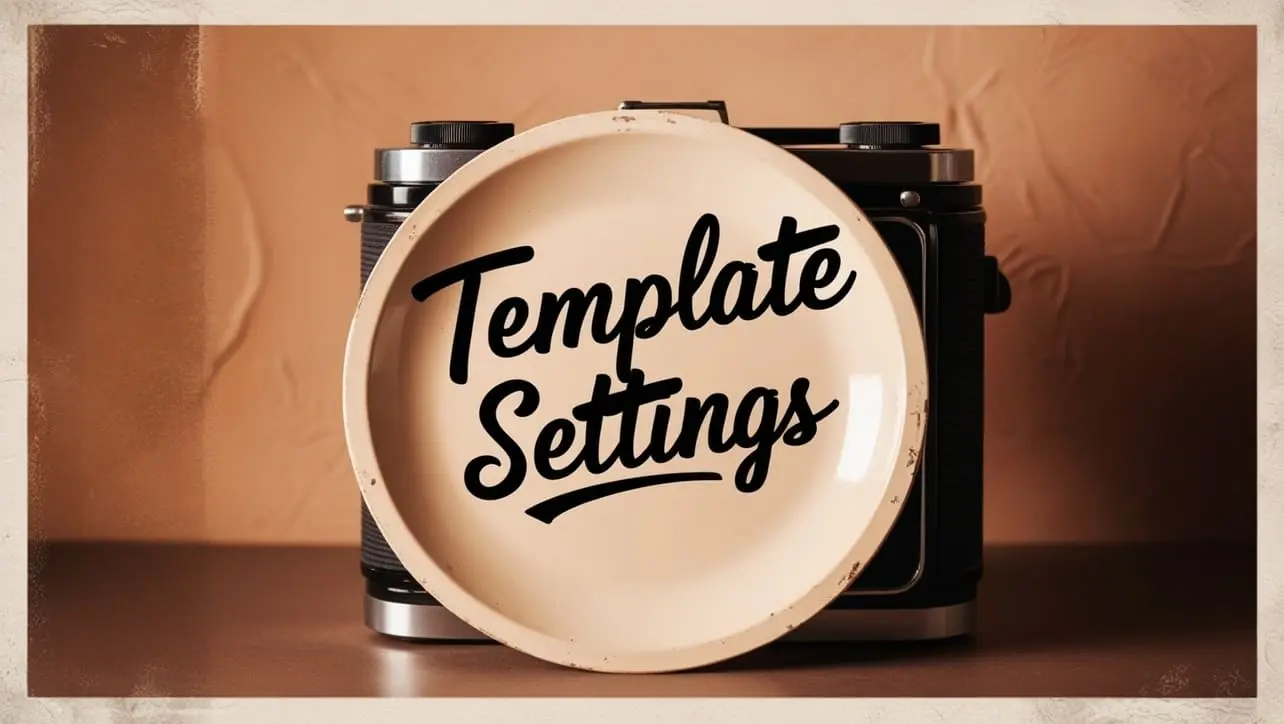
Lodash _.templateSettings Property
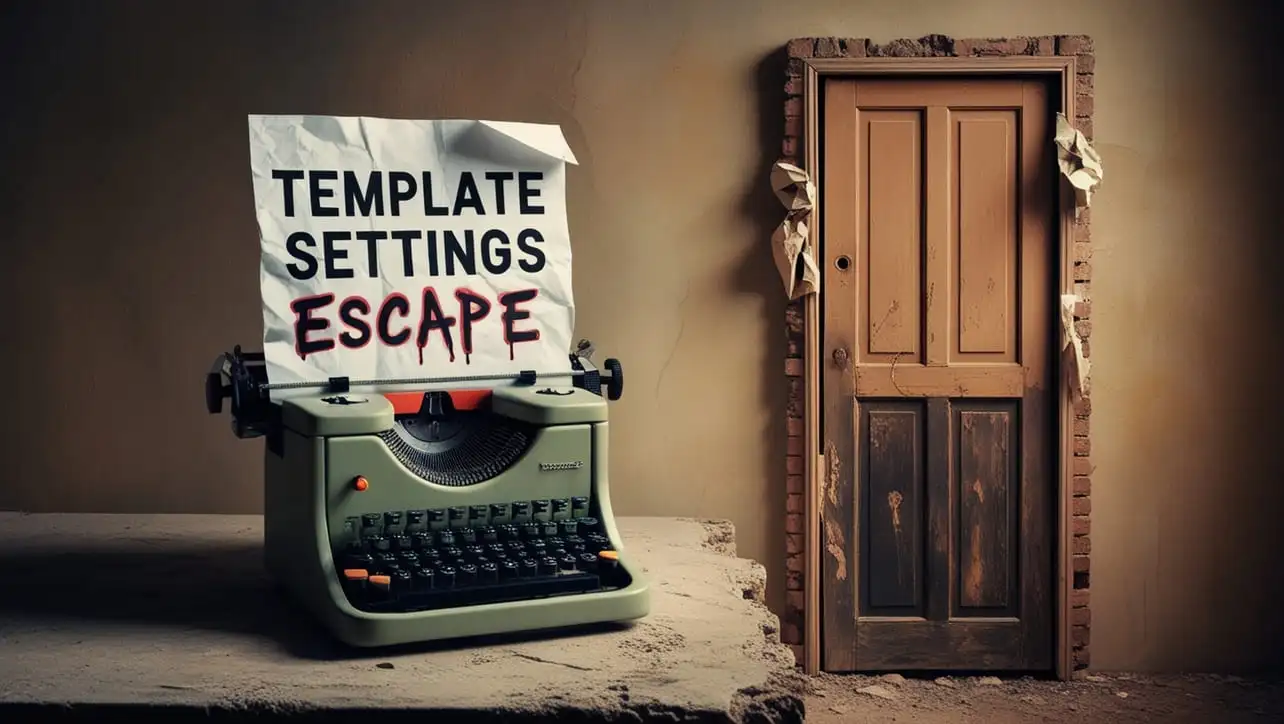
Lodash _.templateSettings.escape Property
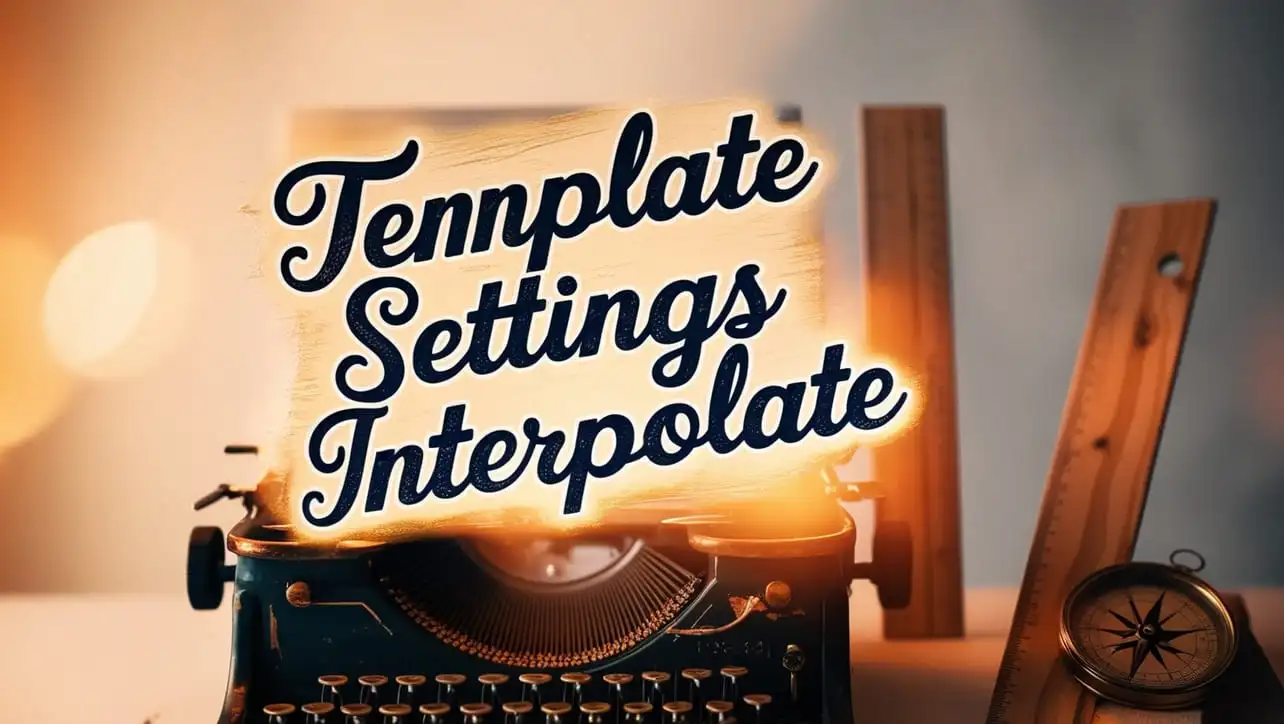
Lodash _.templateSettings.interpolate Property
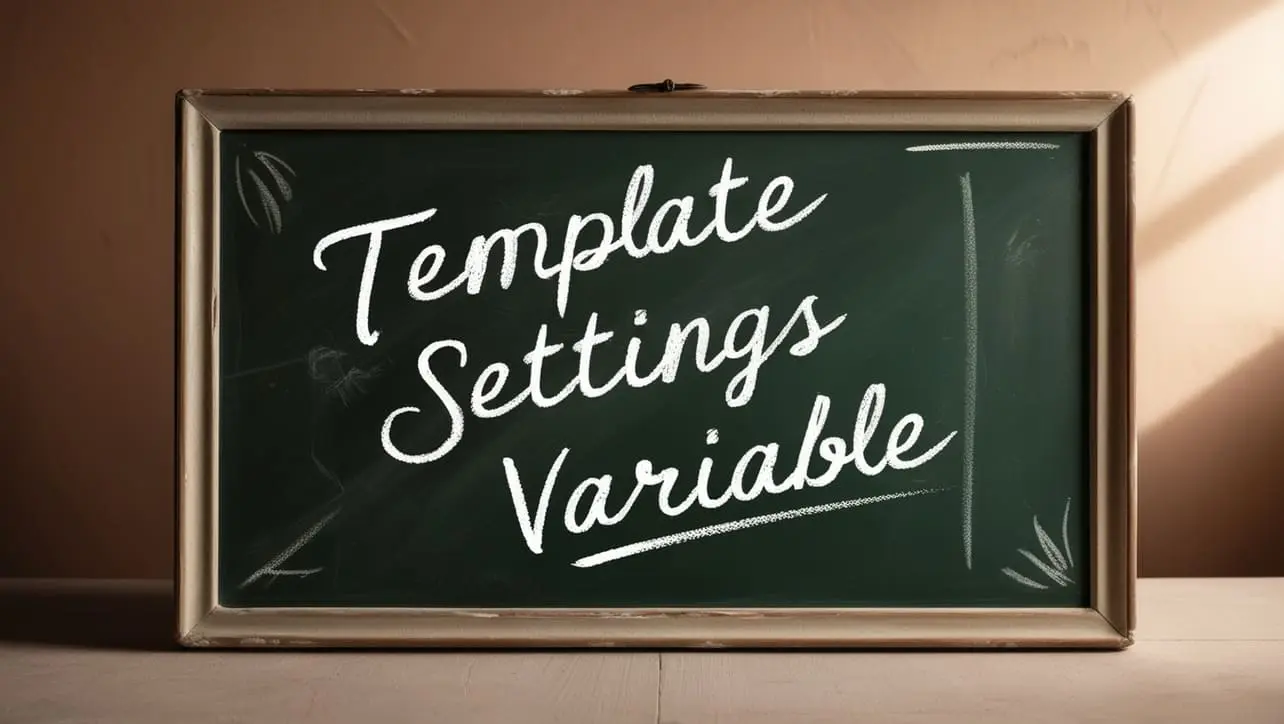
If you have any doubts regarding this article (Lodash _.isEmpty() Lang Method), please comment here. I will help you immediately.