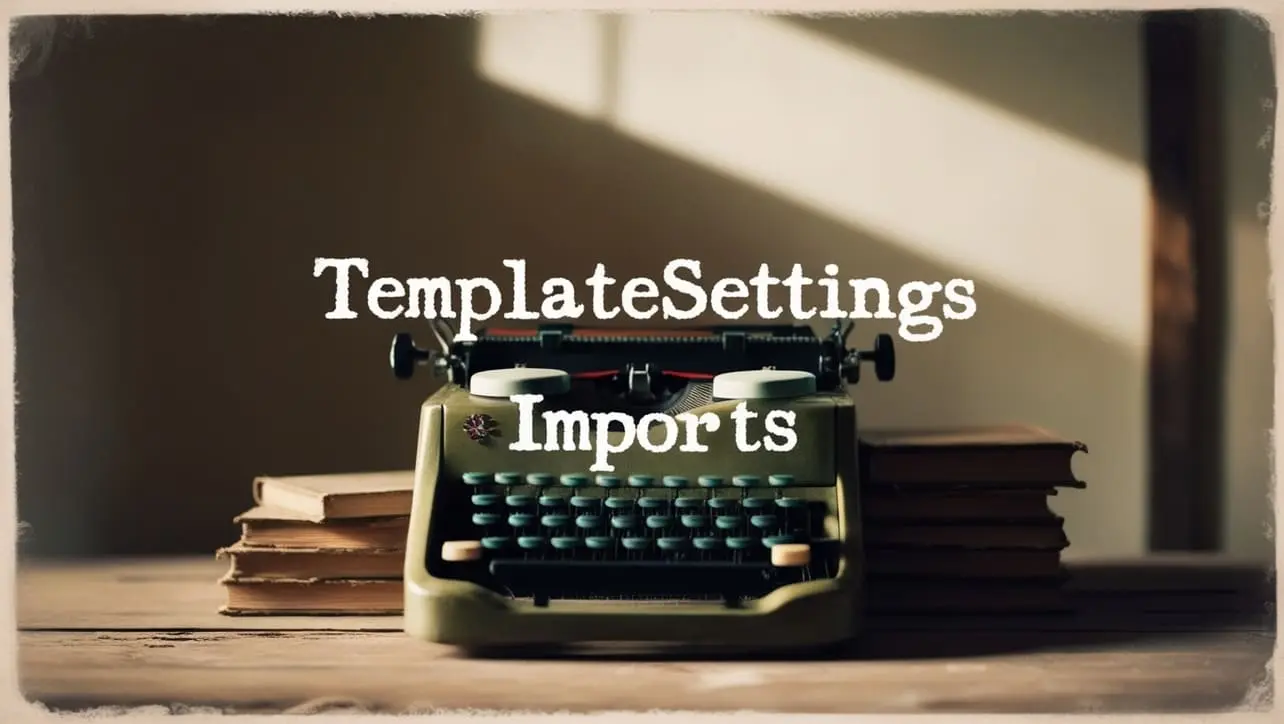
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.cloneWith() Lang Method
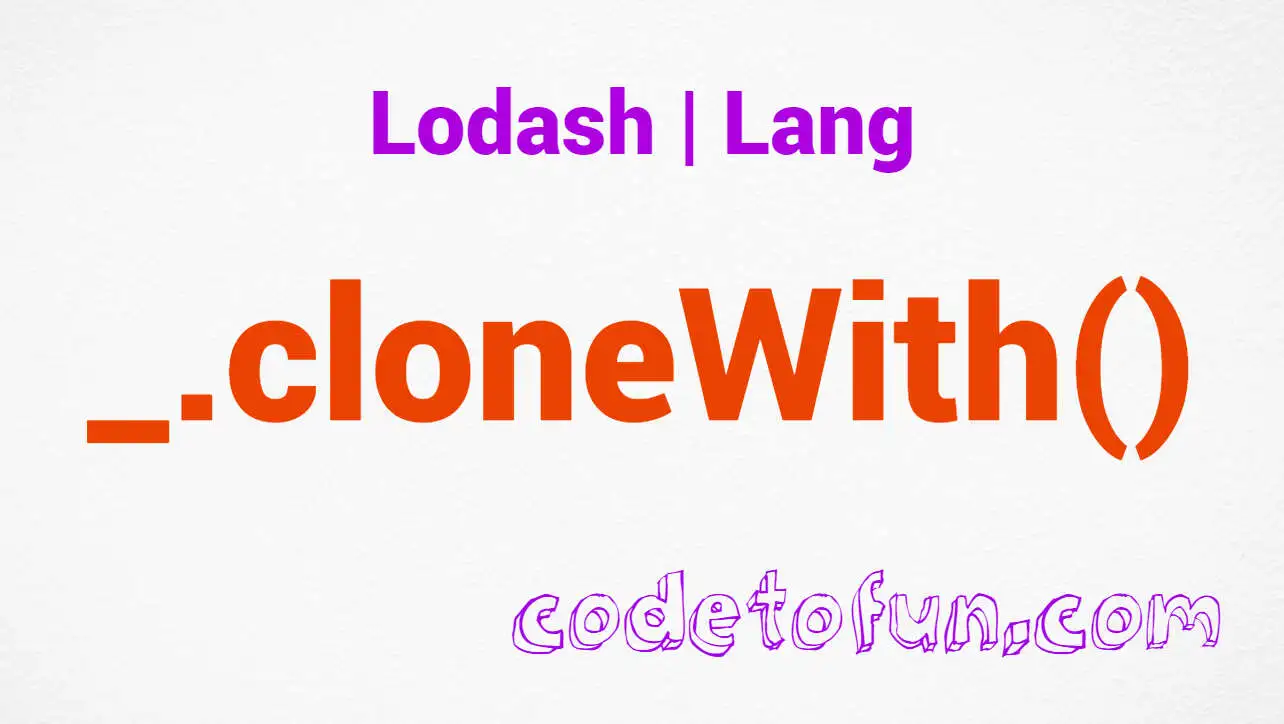
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript programming, effective handling of objects and data structures is fundamental. Lodash, a comprehensive utility library, provides an arsenal of functions to simplify complex tasks. Among these functions is the _.cloneWith()
method, a versatile tool that allows developers to create custom deep clones of objects, providing control over the cloning process.
This method is especially useful when dealing with complex data structures or when customization is needed during the cloning operation.
🧠 Understanding _.cloneWith() Method
The _.cloneWith()
method in Lodash enables developers to create deep clones of objects while allowing customization through a provided customizer function. This customization can include handling specific data transformations or cloning behaviors, offering fine-grained control over the cloning process.
💡 Syntax
The syntax for the _.cloneWith()
method is straightforward:
_.cloneWith(value, [customizer])
- value: The value to clone.
- customizer (Optional): The function to customize cloning.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.cloneWith()
method:
const _ = require('lodash');
const originalObject = {
name: 'John',
age: 30,
address: {
city: 'Example City',
country: 'Example Country',
},
};
const customClone = _.cloneWith(originalObject, (value) => {
if (_.isPlainObject(value)) {
// Customize cloning for objects
return {
...value,
source: 'Customized Clone'
};
}
// Default behavior for non-objects
return undefined;
});
console.log(customClone);
In this example, the originalObject is cloned with a customizer function that adds a source property to nested objects during the cloning process.
🏆 Best Practices
When working with the _.cloneWith()
method, consider the following best practices:
Understand Deep Cloning:
Be aware that
_.cloneWith()
performs deep cloning, meaning nested objects and arrays are cloned recursively. Understand the implications of deep cloning, especially when dealing with large or complex data structures.example.jsCopiedconst originalNestedObject = { key1: 'value1', nested: { key2: 'value2', }, }; const deepClone = _.cloneWith(originalNestedObject); console.log(deepClone);
Leverage Customization:
Harness the power of customization by providing a customizer function. This allows you to control the cloning process and make specific adjustments based on the values being cloned.
example.jsCopiedconst originalData = { value: 'Hello, World!', metadata: { source: 'Original Data', }, }; const customClone = _.cloneWith(originalData, (value) => { if (_.isString(value)) { // Customize cloning for strings return value.toUpperCase(); } // Default behavior for non-strings return undefined; }); console.log(customClone);
Be Mindful of Performance:
While
_.cloneWith()
provides flexibility, be mindful of performance implications, especially when dealing with large datasets. Customization functions can introduce overhead, so ensure your customizations are necessary and optimized.example.jsCopiedconst largeData = /* ...generate or fetch a large dataset... */ ; console.time('customClone'); const customClone = _.cloneWith(largeData, (value) => { // Customization logic return value; }); console.timeEnd('customClone'); console.log(customClone);
📚 Use Cases
Data Transformation:
Use
_.cloneWith()
for data transformation during cloning. This is particularly useful when you need to modify or enhance the cloned data based on specific criteria.example.jsCopiedconst originalData = { amount: 100, currency: 'USD', }; const transformedClone = _.cloneWith(originalData, (value, key) => { if (key === 'amount') { // Transform the 'amount' value during cloning return value * 2; } // Default behavior for other keys return undefined; }); console.log(transformedClone);
Customized Cloning for Objects:
Tailor the cloning process for specific object properties.
_.cloneWith()
allows you to provide custom logic for cloning nested objects or handling specific properties.example.jsCopiedconst originalObject = { name: 'Alice', details: { age: 25, location: 'City', }, }; const customizedClone = _.cloneWith(originalObject, (value, key) => { if (key === 'details') { // Customize cloning for the 'details' object return { ...value, modified: true }; } // Default behavior for other keys return undefined; }); console.log(customizedClone);
Handling Special Cases:
Address special cases or edge scenarios by providing custom cloning logic. This can be beneficial when certain values require unique treatment during cloning.
example.jsCopiedconst originalData = { name: 'Bob', specialValue: /* ...some special value... */ , }; const specialClone = _.cloneWith(originalData, (value, key) => { if (key === 'specialValue') { // Handle special cloning logic return /* ...custom cloning for special value... */; } // Default behavior for other keys return undefined; }); console.log(specialClone);
🎉 Conclusion
The _.cloneWith()
method in Lodash empowers developers to create deep clones of objects with the added flexibility of customization. Whether you're transforming data during cloning or handling unique cloning scenarios, this method provides a robust solution for object cloning in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.cloneWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
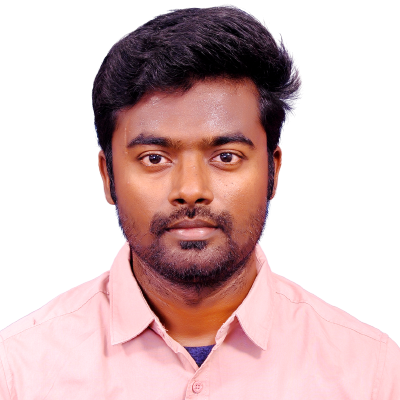
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
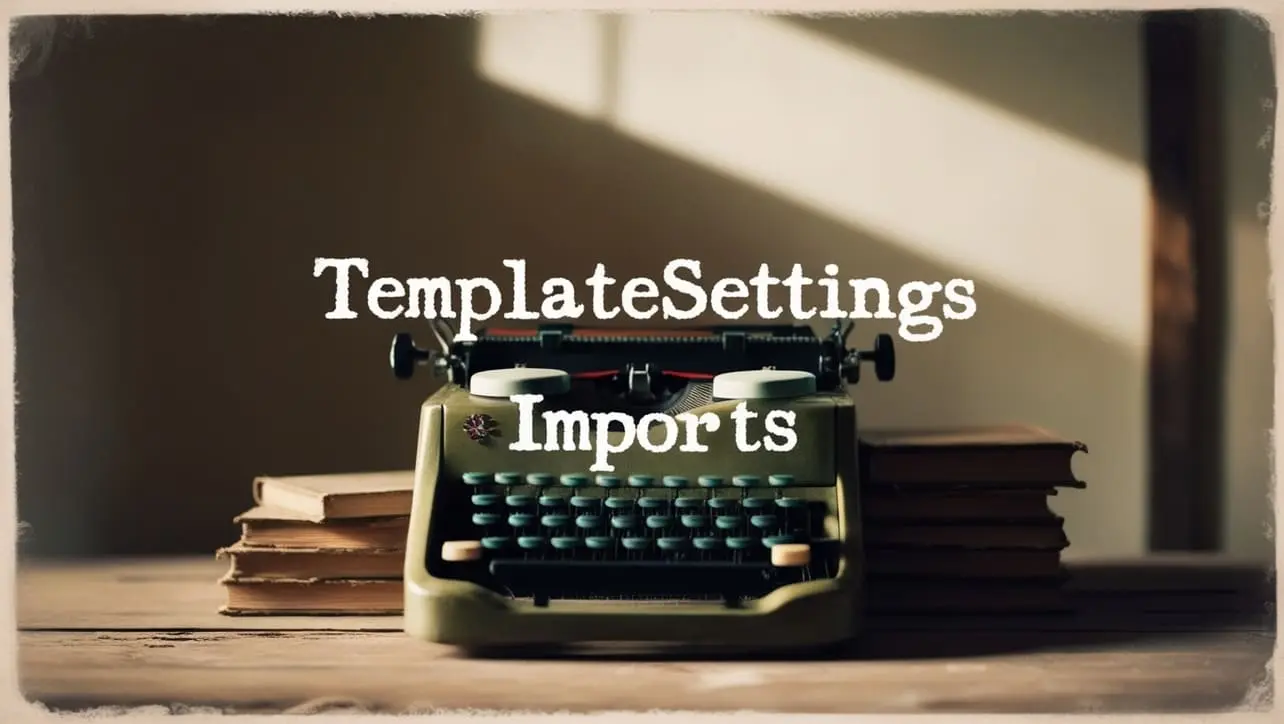
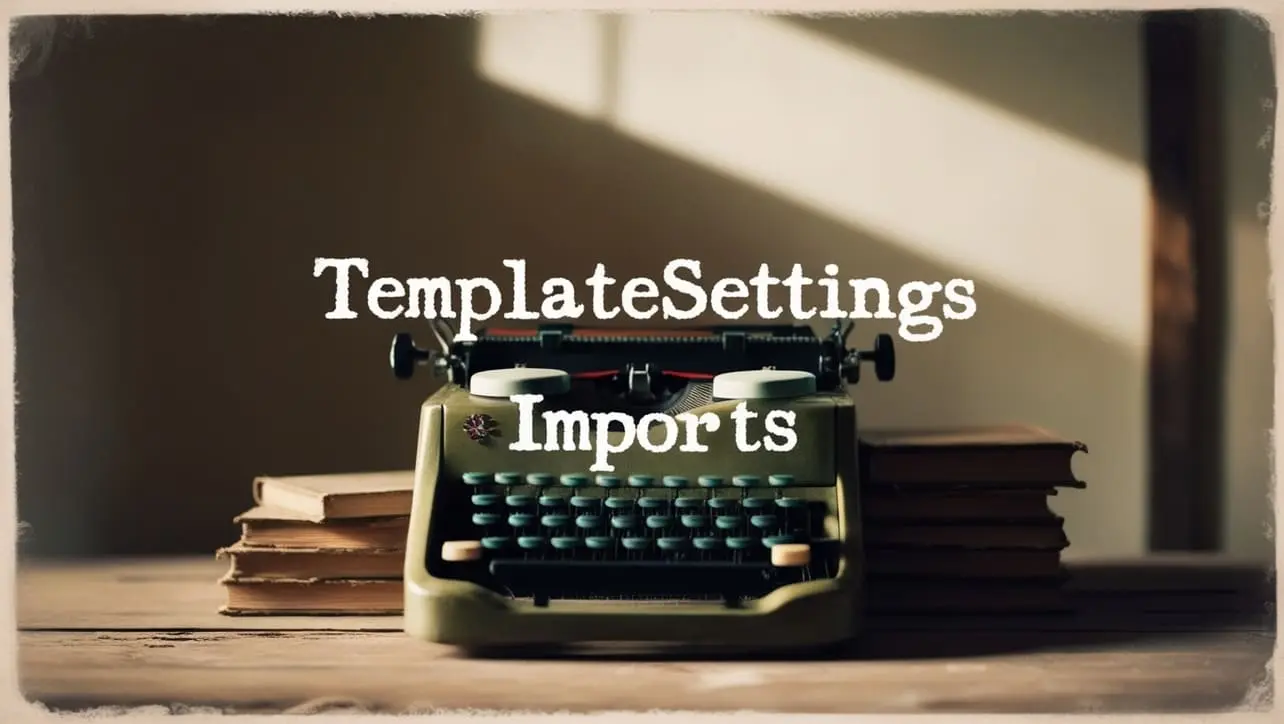
Lodash _.templateSettings.imports Property
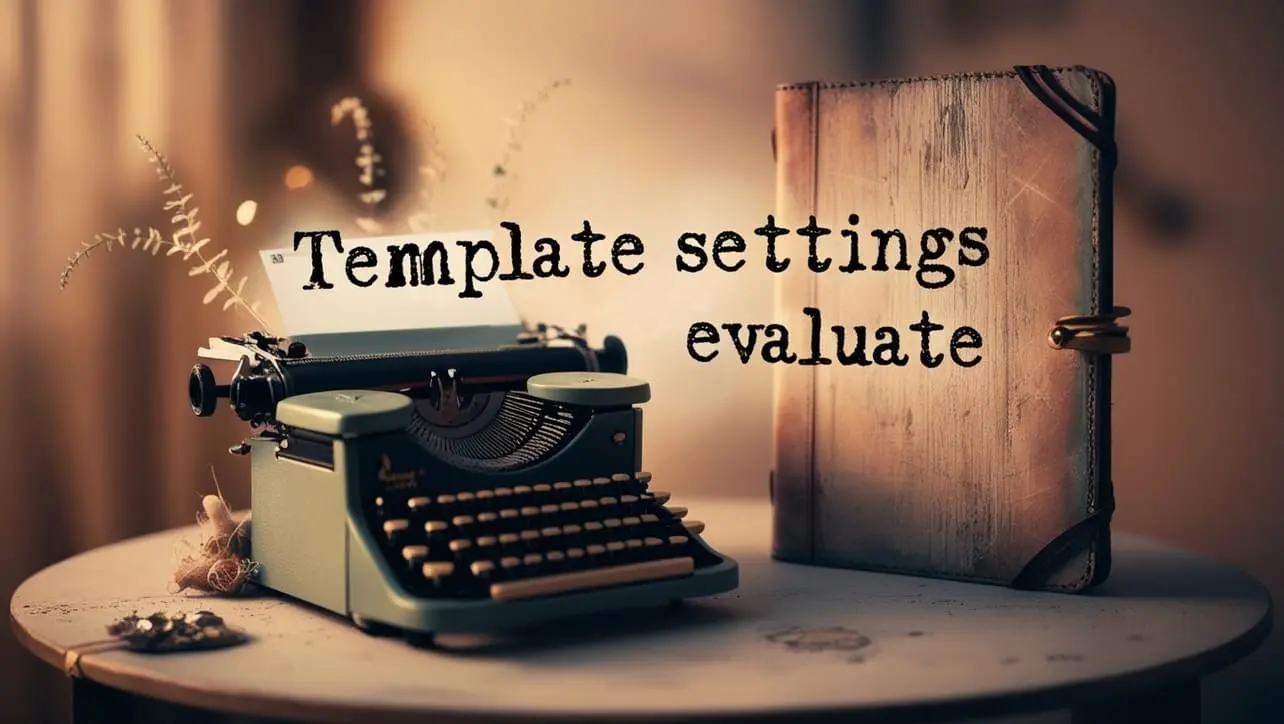
Lodash _.templateSettings.evaluate Property
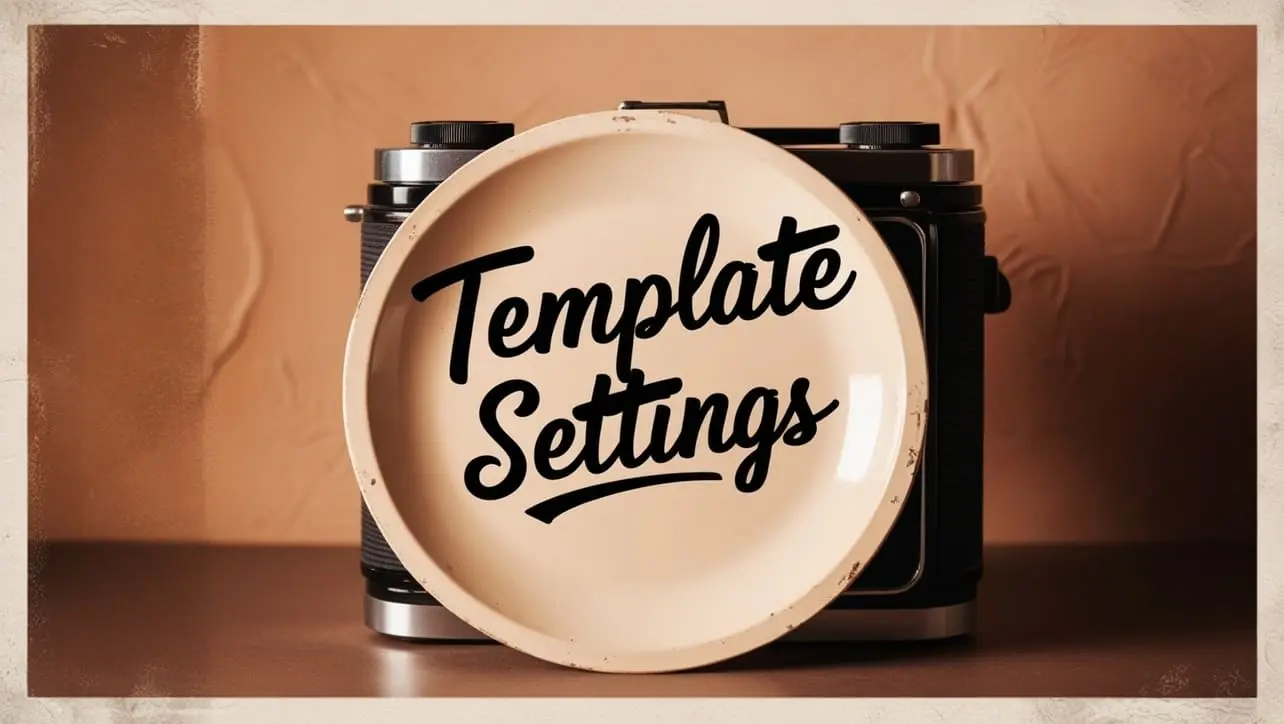
Lodash _.templateSettings Property
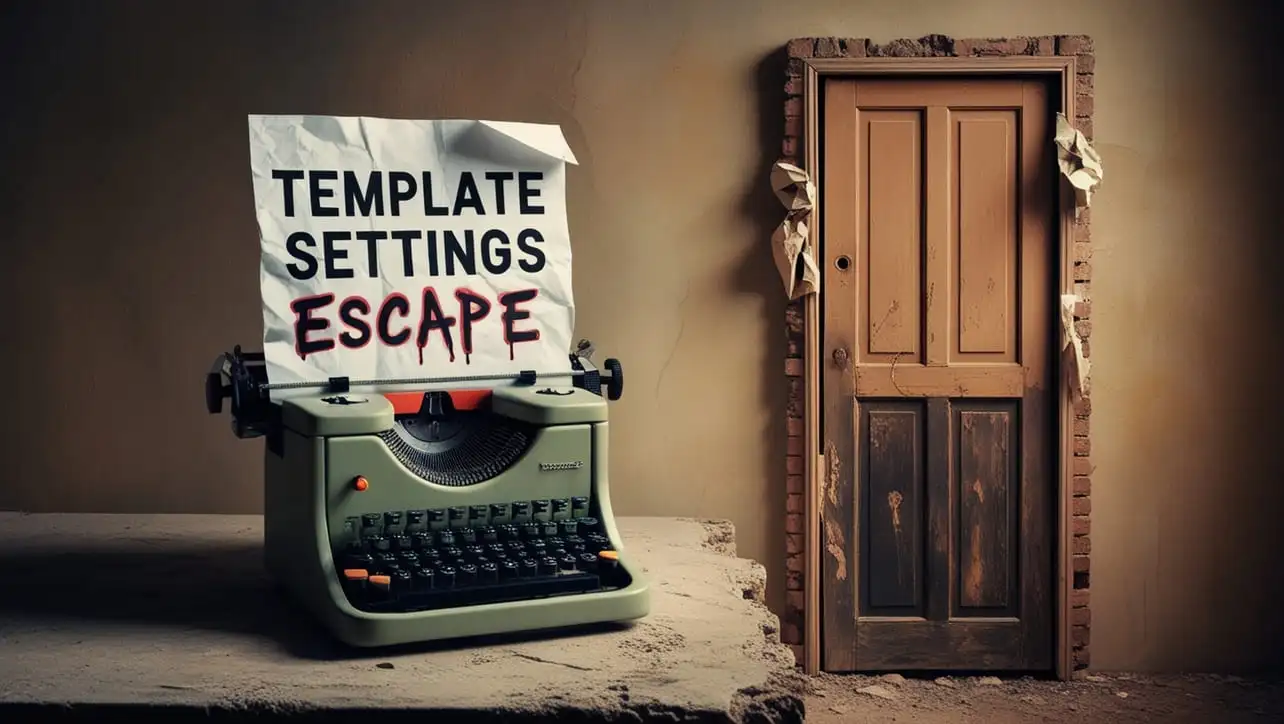
Lodash _.templateSettings.escape Property
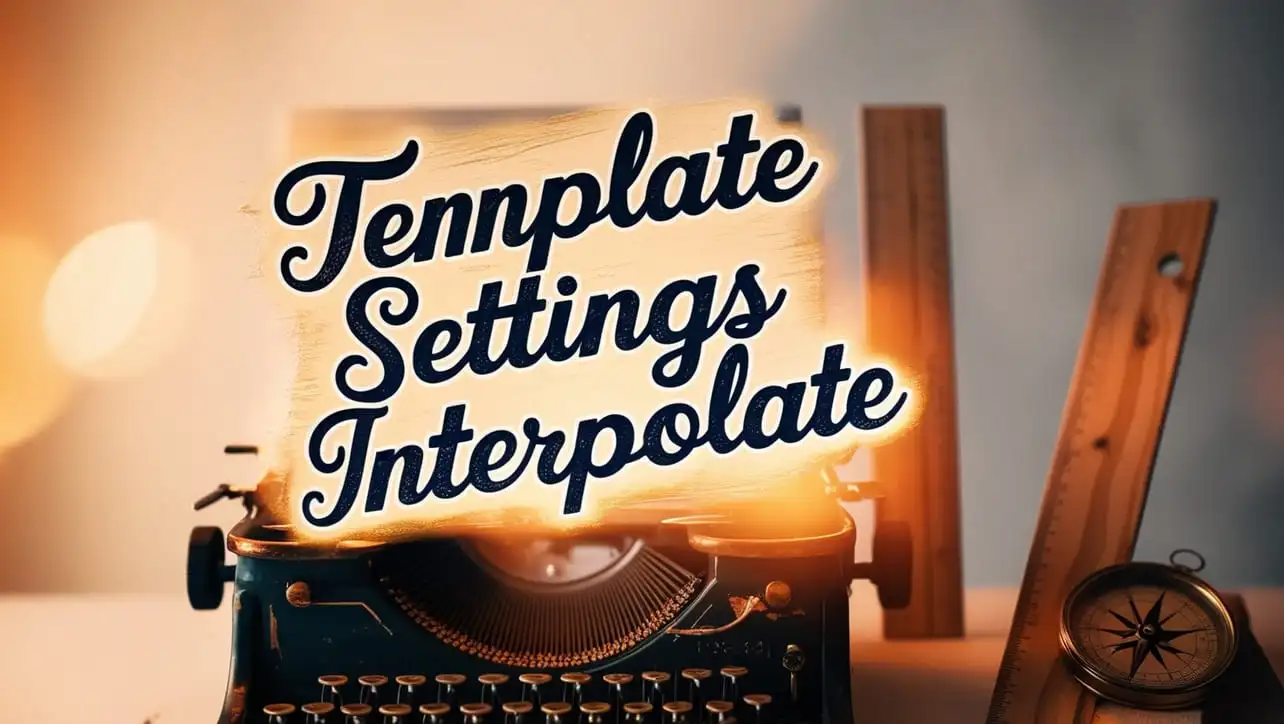
Lodash _.templateSettings.interpolate Property
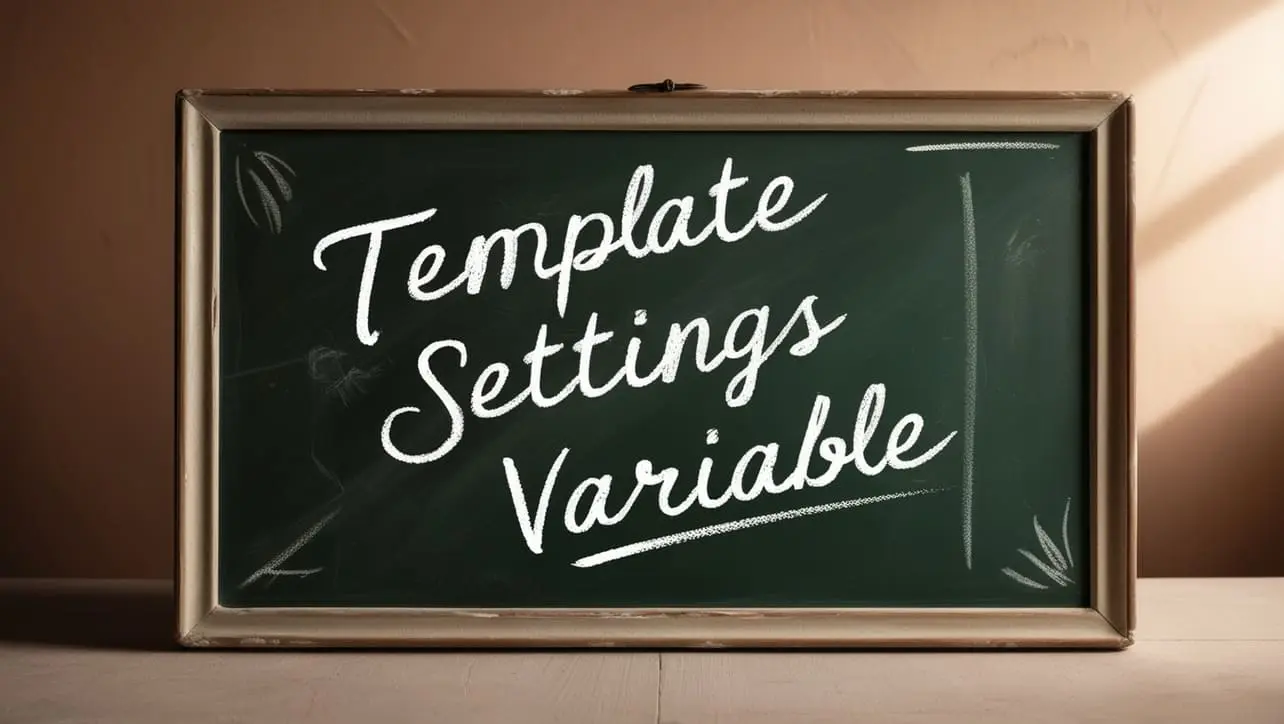
If you have any doubts regarding this article (Lodash _.cloneWith() Lang Method), please comment here. I will help you immediately.