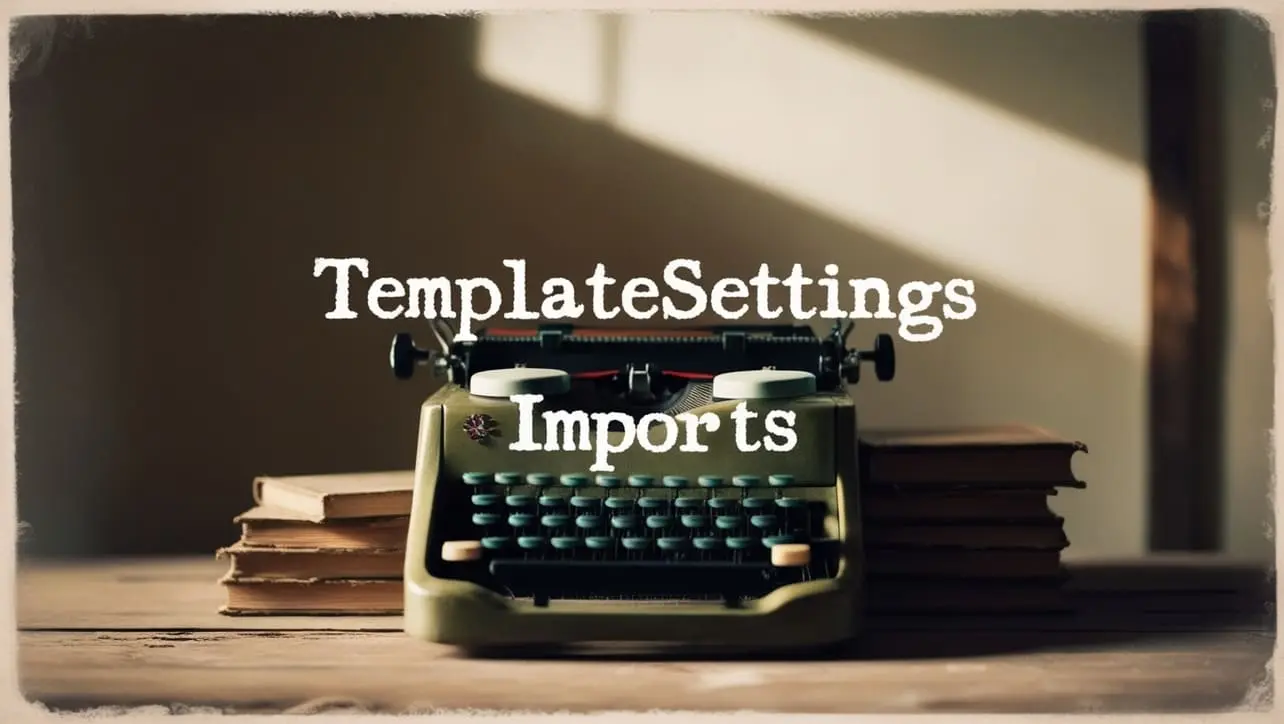
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toSafeInteger() Lang Method
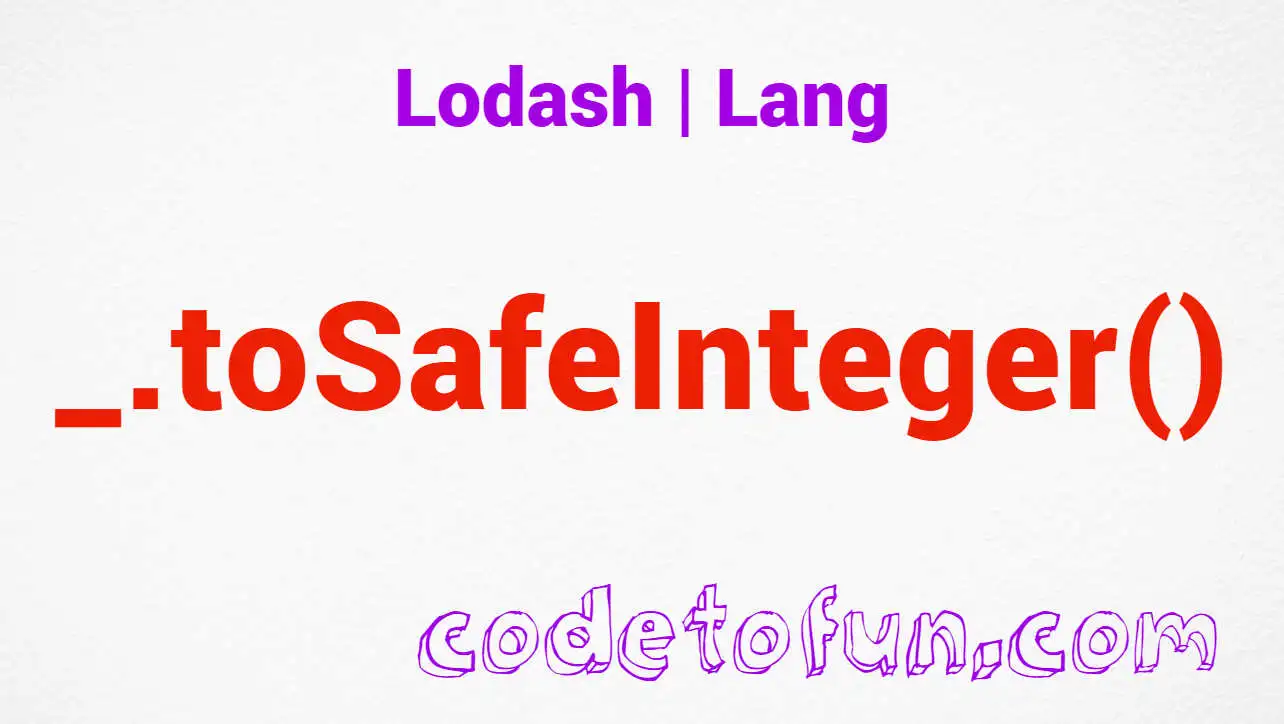
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript programming, data types and conversions play a crucial role. Lodash, a powerful utility library, offers a variety of methods to simplify these operations. Among them is the _.toSafeInteger()
method, a valuable tool for converting values to safe integers, mitigating the risk of unexpected results or overflows.
Understanding and utilizing this method can enhance the robustness and reliability of your JavaScript code.
🧠 Understanding _.toSafeInteger() Method
The _.toSafeInteger()
method in Lodash is designed to convert a given value to a safe integer. This ensures that the result is within the range of safe integer values in JavaScript, preventing potential issues associated with integer overflow or underflow.
💡 Syntax
The syntax for the _.toSafeInteger()
method is straightforward:
_.toSafeInteger(value)
- value: The value to convert.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toSafeInteger()
method:
const _ = require('lodash');
const unsafeValue = 9007199254740992; // Beyond the safe integer range
const safeInteger = _.toSafeInteger(unsafeValue);
console.log(safeInteger);
// Output: 9007199254740991 (the maximum safe integer value)
In this example, unsafeValue exceeds the safe integer range, but _.toSafeInteger()
ensures that the result is a safe integer.
🏆 Best Practices
When working with the _.toSafeInteger()
method, consider the following best practices:
Handling Large Numbers:
When working with potentially large numbers, especially those derived from external sources or calculations, use
_.toSafeInteger()
to safeguard against integer overflows.example.jsCopiedconst largeNumber = /* ...some potentially large number... */; const safeResult = _.toSafeInteger(largeNumber); console.log(safeResult);
Input Validation:
Before applying
_.toSafeInteger()
, consider implementing input validation to ensure that the provided value is of the expected type and within the acceptable range.example.jsCopiedfunction convertToSafeInteger(value) { if (typeof value !== 'number') { console.error('Invalid input: Expected a number'); return; } const safeResult = _.toSafeInteger(value); console.log(safeResult); } convertToSafeInteger('abc'); // Output: Invalid input: Expected a number convertToSafeInteger(12345); // Output: 12345
Consistent Type Handling:
Ensure consistent handling of different types when using
_.toSafeInteger()
. Understanding how this method treats non-numeric values can help prevent unexpected behavior in your code.example.jsCopiedconst nonNumericValue = '12345'; const numericValue = 67890; const safeResultNonNumeric = _.toSafeInteger(nonNumericValue); const safeResultNumeric = _.toSafeInteger(numericValue); console.log(safeResultNonNumeric); // Output: 12345 console.log(safeResultNumeric); // Output: 67890
📚 Use Cases
Parsing User Input:
When dealing with user input, especially in scenarios where numeric values are expected, use
_.toSafeInteger()
to handle potential input errors gracefully.example.jsCopiedconst userInput = /* ...user-provided input... */; const parsedInteger = _.toSafeInteger(userInput); console.log(parsedInteger);
Database Operations:
In database interactions, particularly when working with numeric data retrieved from external sources, apply
_.toSafeInteger()
to ensure that the values are within the safe integer range.example.jsCopiedconst rawDatabaseValue = /* ...retrieve value from the database... */; const safeDatabaseValue = _.toSafeInteger(rawDatabaseValue); console.log(safeDatabaseValue);
Mathematical Calculations:
When performing mathematical calculations that may result in large numbers, use
_.toSafeInteger()
to prevent overflow issues.example.jsCopiedconst resultOfCalculation = /* ...some mathematical calculation... */; const safeResult = _.toSafeInteger(resultOfCalculation); console.log(safeResult);
🎉 Conclusion
The _.toSafeInteger()
method in Lodash is a valuable asset when dealing with numeric values in JavaScript. Whether you're parsing user input, performing database operations, or handling mathematical calculations, this method provides a robust solution to ensure that your integers stay within the safe range.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toSafeInteger()
method in your Lodash projects.
👨💻 Join our Community:
Author
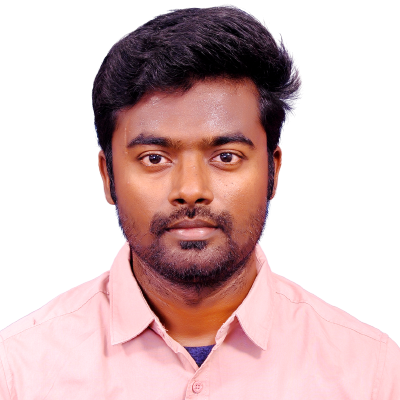
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
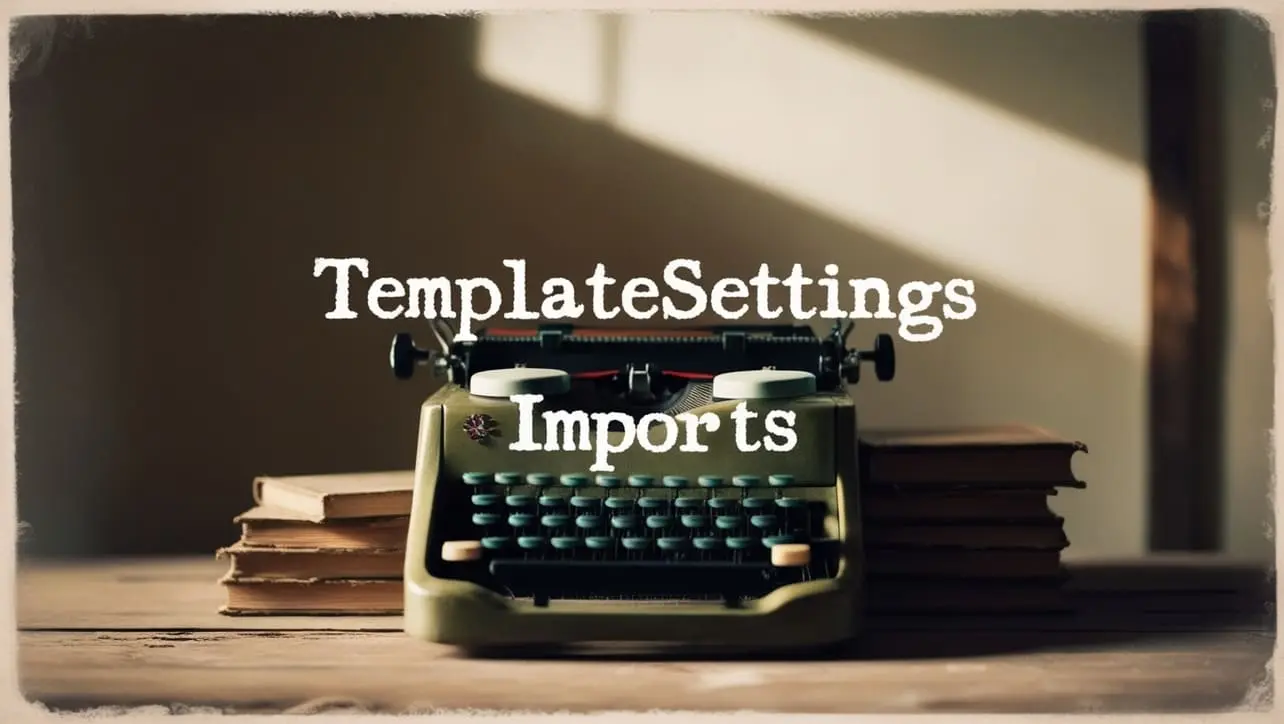
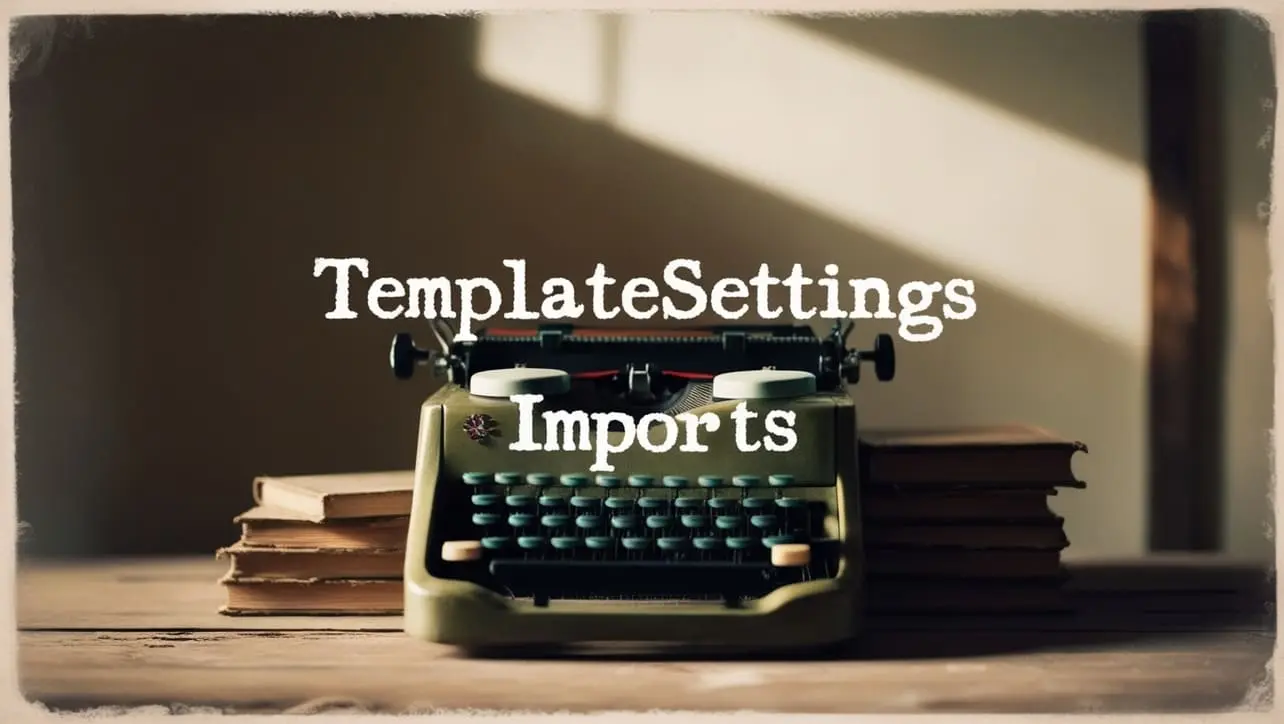
Lodash _.templateSettings.imports Property
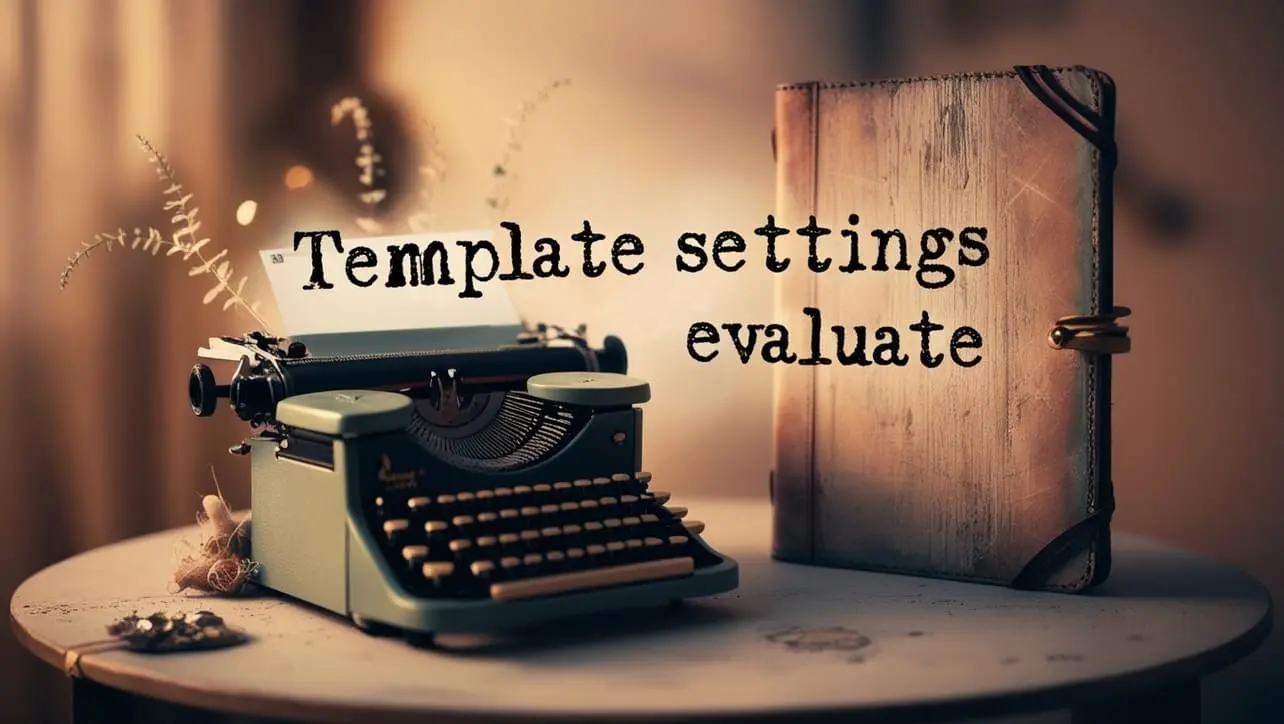
Lodash _.templateSettings.evaluate Property
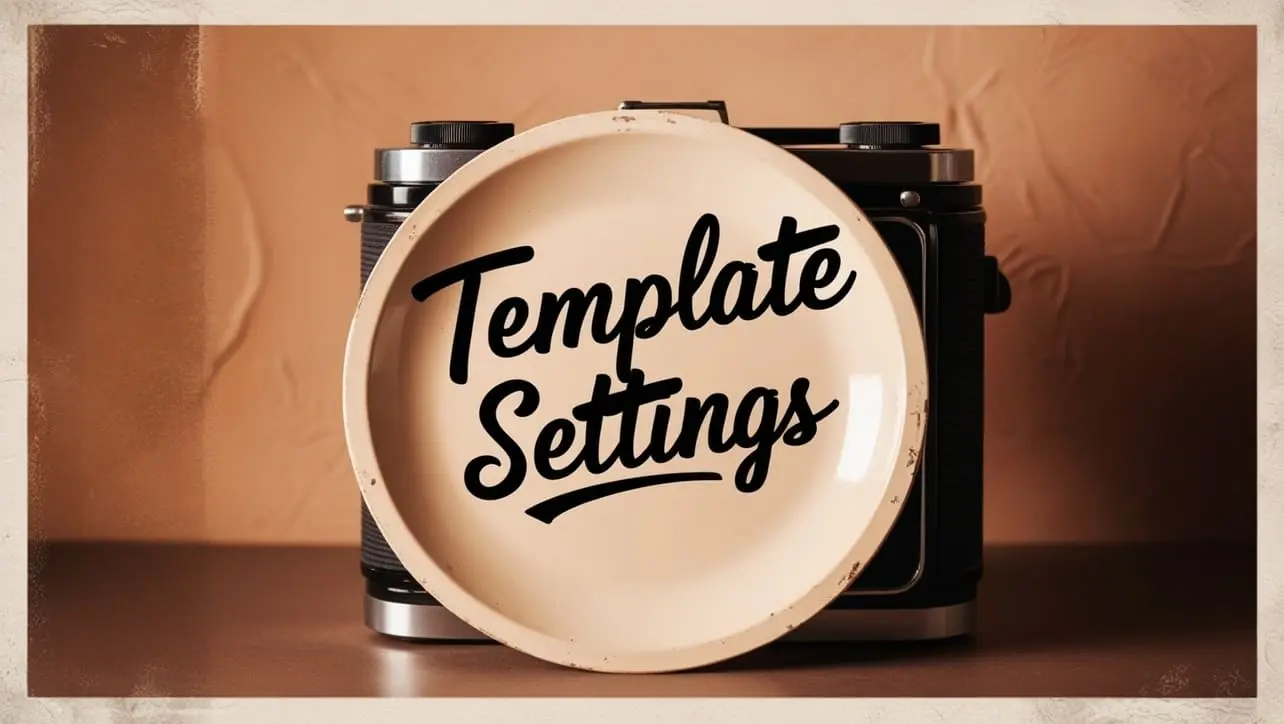
Lodash _.templateSettings Property
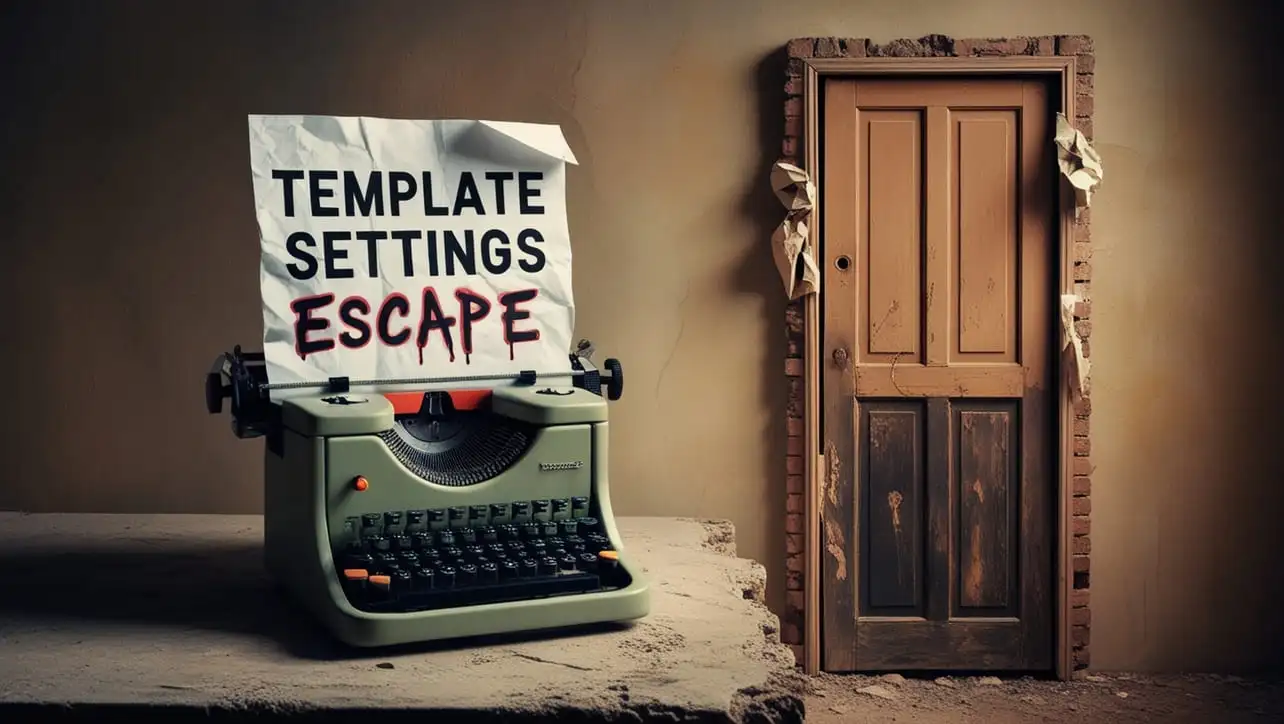
Lodash _.templateSettings.escape Property
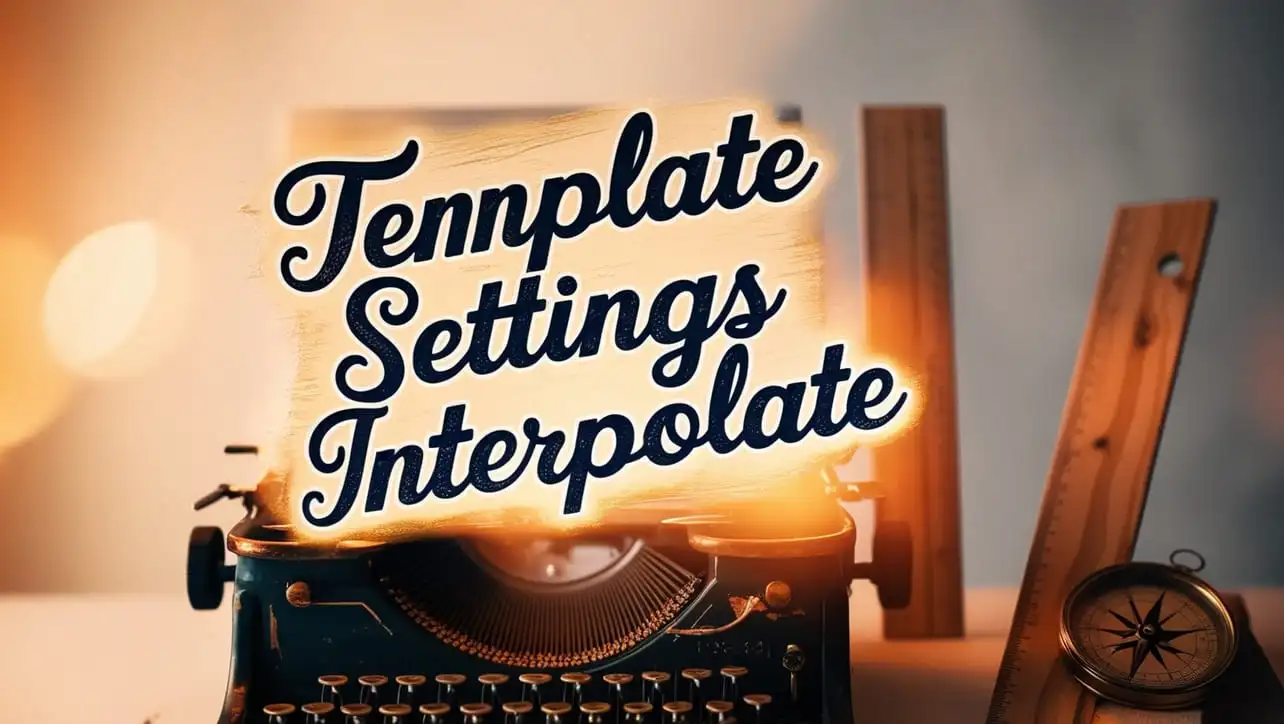
Lodash _.templateSettings.interpolate Property
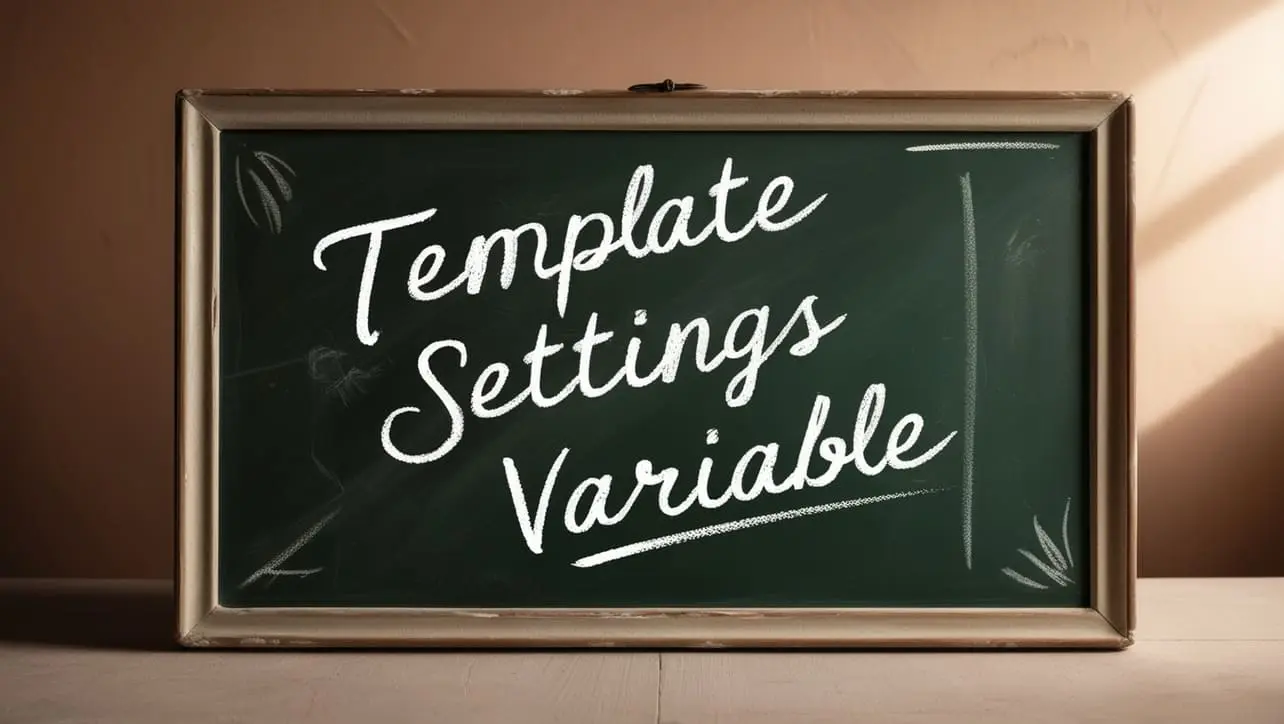
If you have any doubts regarding this article (Lodash _.toSafeInteger() Lang Method), please comment here. I will help you immediately.