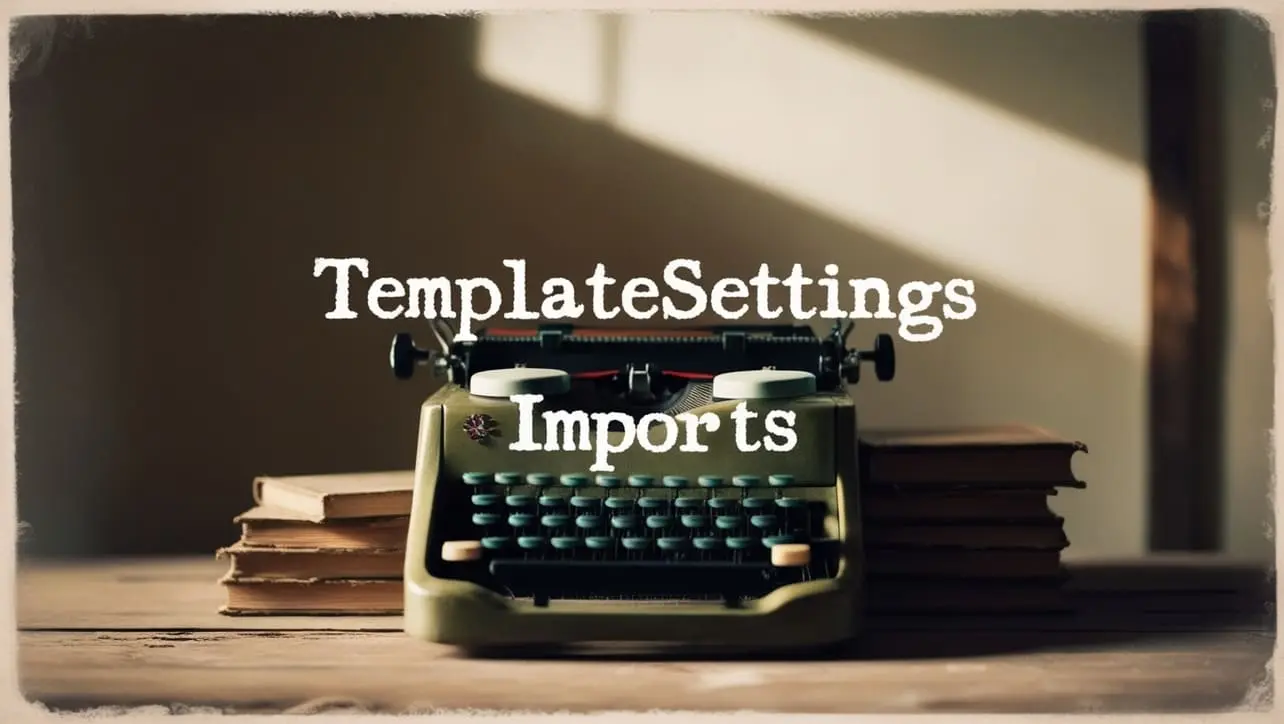
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toInteger() Lang Method
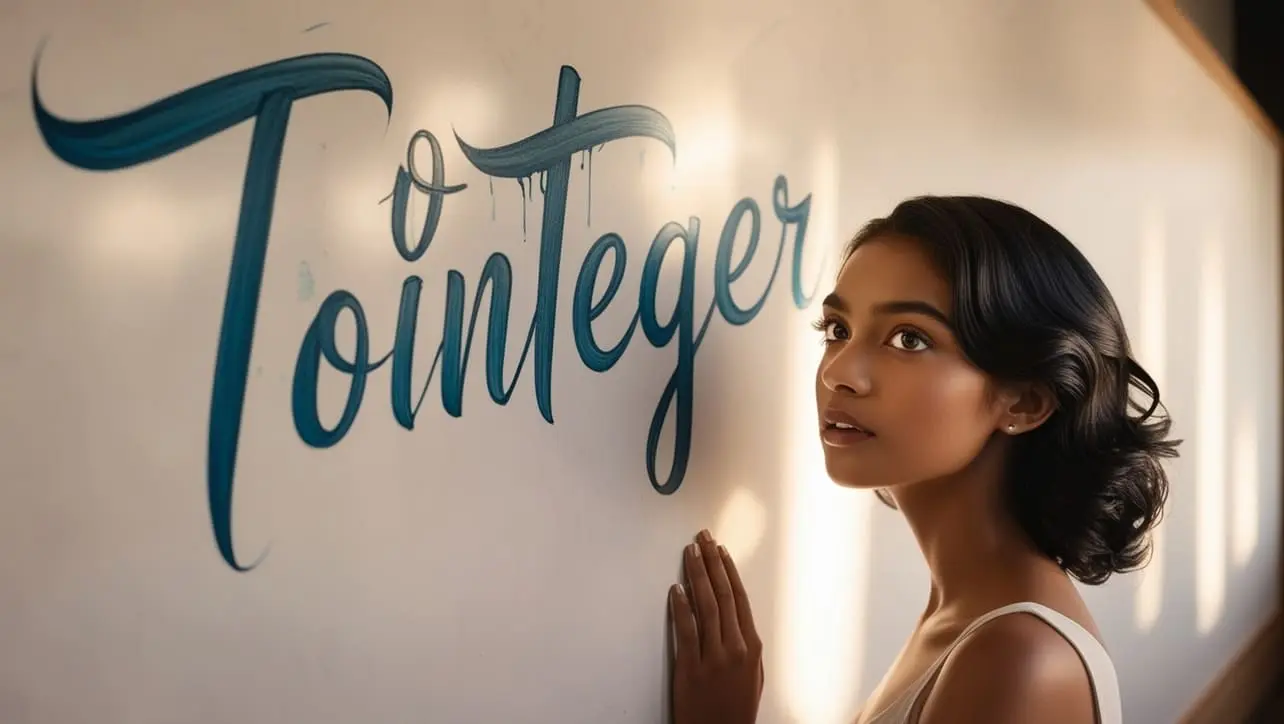
Photo Credit to CodeToFun
🙋 Introduction
In the diverse landscape of JavaScript programming, managing data types is an essential aspect of ensuring accurate and reliable code. Lodash, a powerful utility library, provides a variety of functions to simplify common programming tasks.
Among these functions, the _.toInteger()
method stands out as a valuable tool for converting values to integers, addressing common challenges related to data types in JavaScript.
🧠 Understanding _.toInteger() Method
The _.toInteger()
method in Lodash is designed to convert a given value to an integer. It gracefully handles a wide range of input types, making it a versatile solution for scenarios where ensuring an integer result is crucial.
💡 Syntax
The syntax for the _.toInteger()
method is straightforward:
_.toInteger(value)
- value: The value to convert to an integer.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toInteger()
method:
const _ = require('lodash');
const floatValue = 42.7;
const integerValue = _.toInteger(floatValue);
console.log(integerValue);
// Output: 42
In this example, the floatValue is converted to an integer using _.toInteger()
, resulting in the removal of the decimal part.
🏆 Best Practices
When working with the _.toInteger()
method, consider the following best practices:
Handling Invalid Values:
Account for cases where the input value might be invalid for conversion to an integer. Use appropriate validation to ensure the method is applied to valid data.
example.jsCopiedconst invalidValue = 'abc'; const integerValue = _.toInteger(invalidValue); console.log(integerValue); // Output: 0 (default value for invalid input)
Rounding Behavior:
Understand that
_.toInteger()
follows the same rounding behavior as the built-in Math.floor() function. Be mindful of this behavior when using the method in scenarios where specific rounding rules are critical.example.jsCopiedconst negativeFloatValue = -42.7; const roundedInteger = _.toInteger(negativeFloatValue); console.log(roundedInteger); // Output: -43
Parsing Numeric Strings:
_.toInteger()
is capable of parsing numeric strings, making it useful in scenarios where input values might be received as strings.example.jsCopiedconst numericString = '987'; const parsedInteger = _.toInteger(numericString); console.log(parsedInteger); // Output: 987
📚 Use Cases
User Input Handling:
When dealing with user input, especially in forms or interactive applications, using
_.toInteger()
ensures that numeric values are processed correctly, even if entered as strings.example.jsCopiedconst userInput = /* ...retrieve user input... */; const processedValue = _.toInteger(userInput); console.log(processedValue);
Configuration Settings:
In applications where configuration settings are managed,
_.toInteger()
can be applied to ensure that numeric values in configuration files or input are converted to integers.example.jsCopiedconst configSetting = /* ...retrieve configuration setting... */; const integerValue = _.toInteger(configSetting); console.log(integerValue);
Mathematical Operations:
When performing mathematical operations and precision is not a primary concern, using
_.toInteger()
ensures that the result is an integer, avoiding unexpected behaviors due to floating-point precision.example.jsCopiedconst result = _.toInteger(42.5) * 2; console.log(result); // Output: 84
🎉 Conclusion
The _.toInteger()
method in Lodash offers a reliable solution for converting values to integers in JavaScript. Whether you're dealing with user input, configuration settings, or mathematical operations, this method provides a straightforward and consistent approach to ensure data integrity.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toInteger()
method in your Lodash projects.
👨💻 Join our Community:
Author
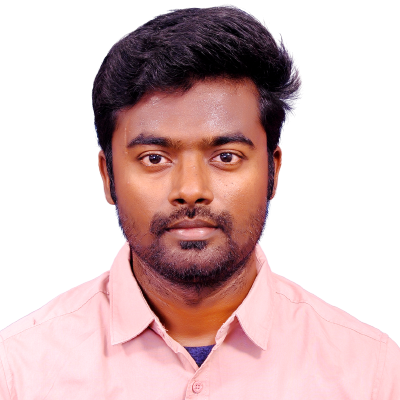
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
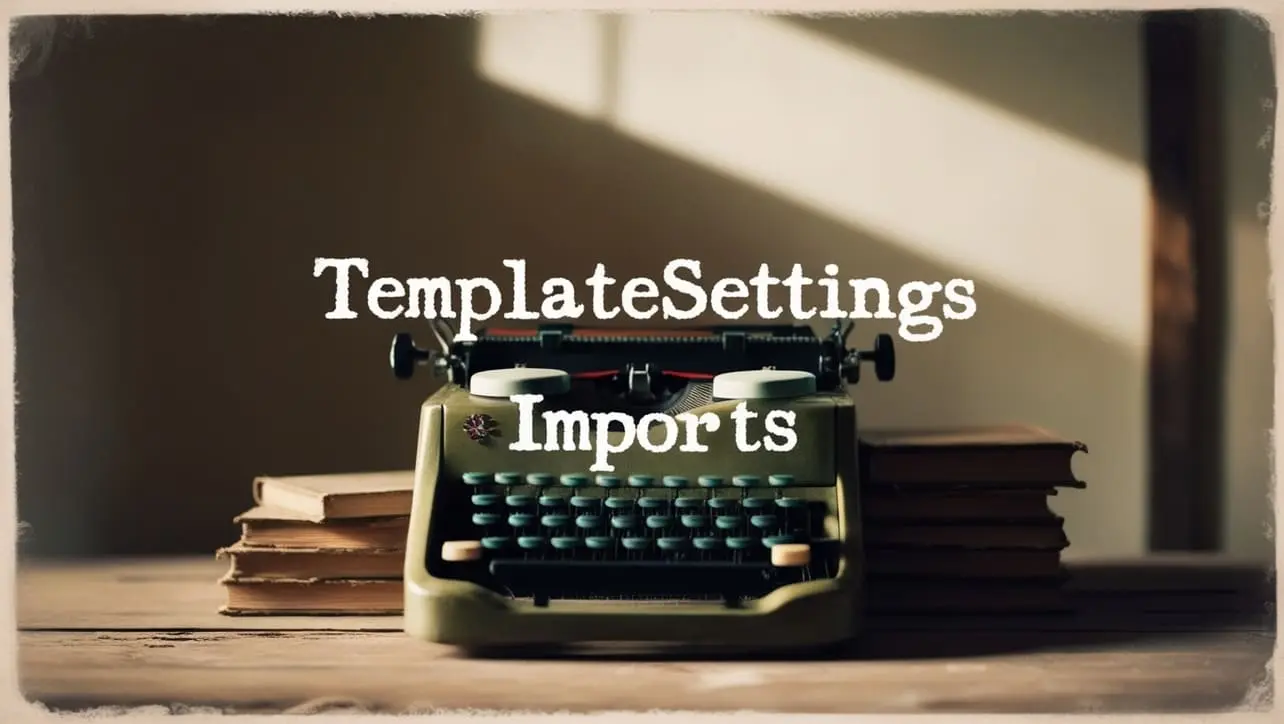
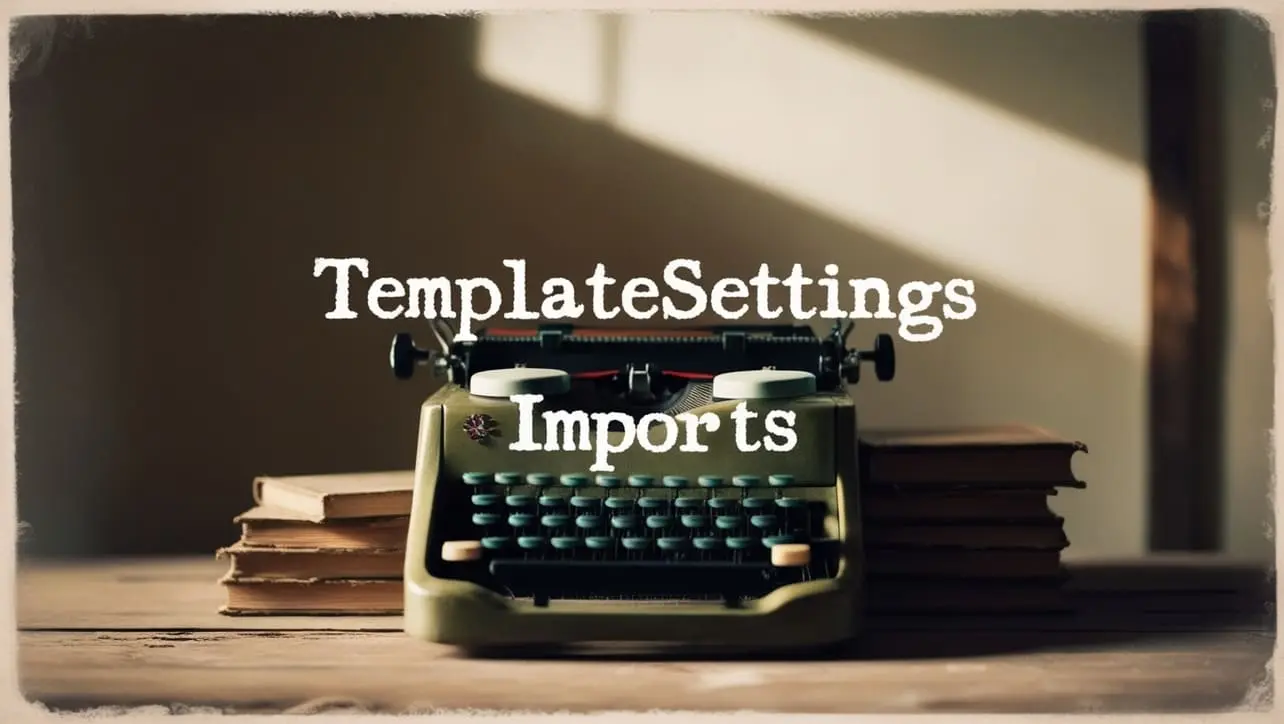
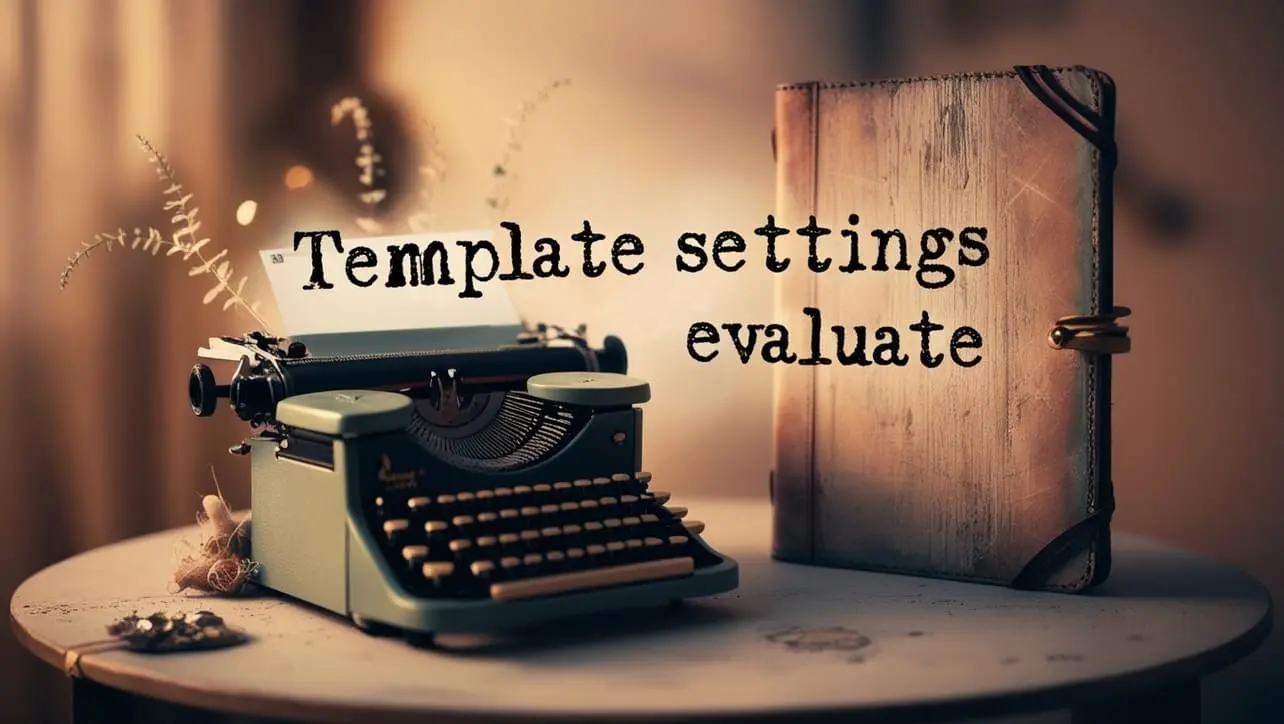
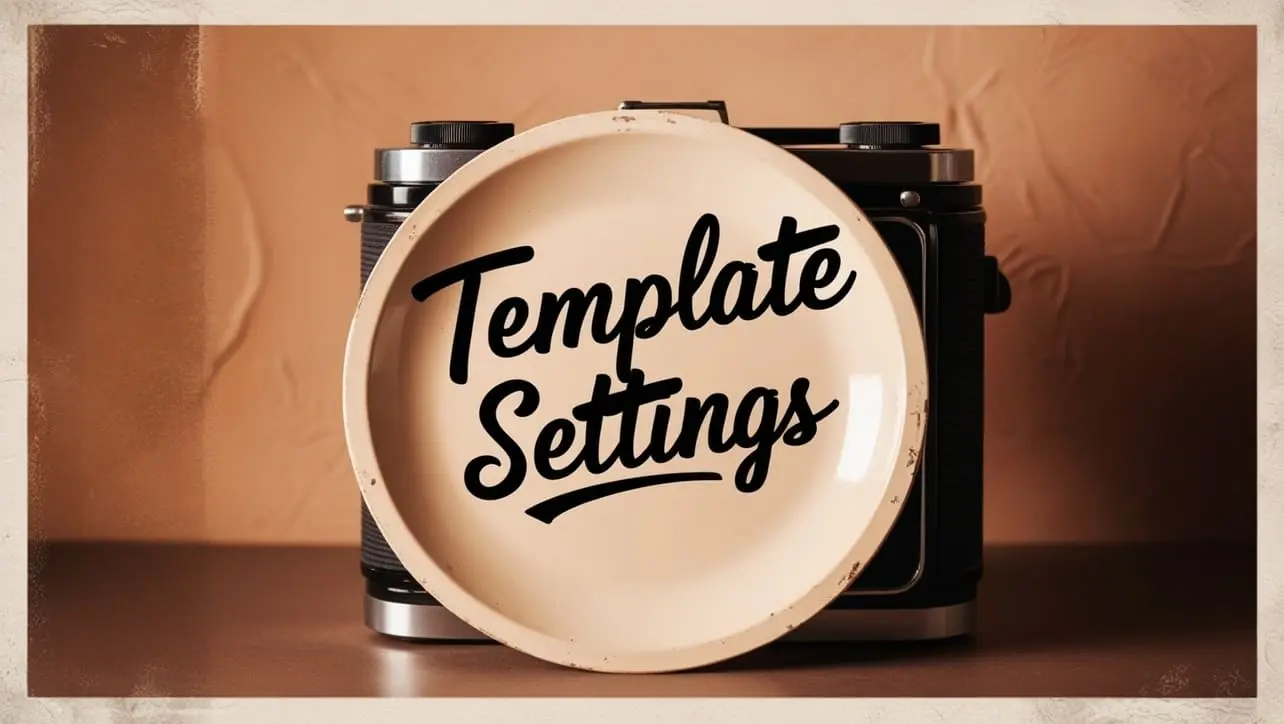
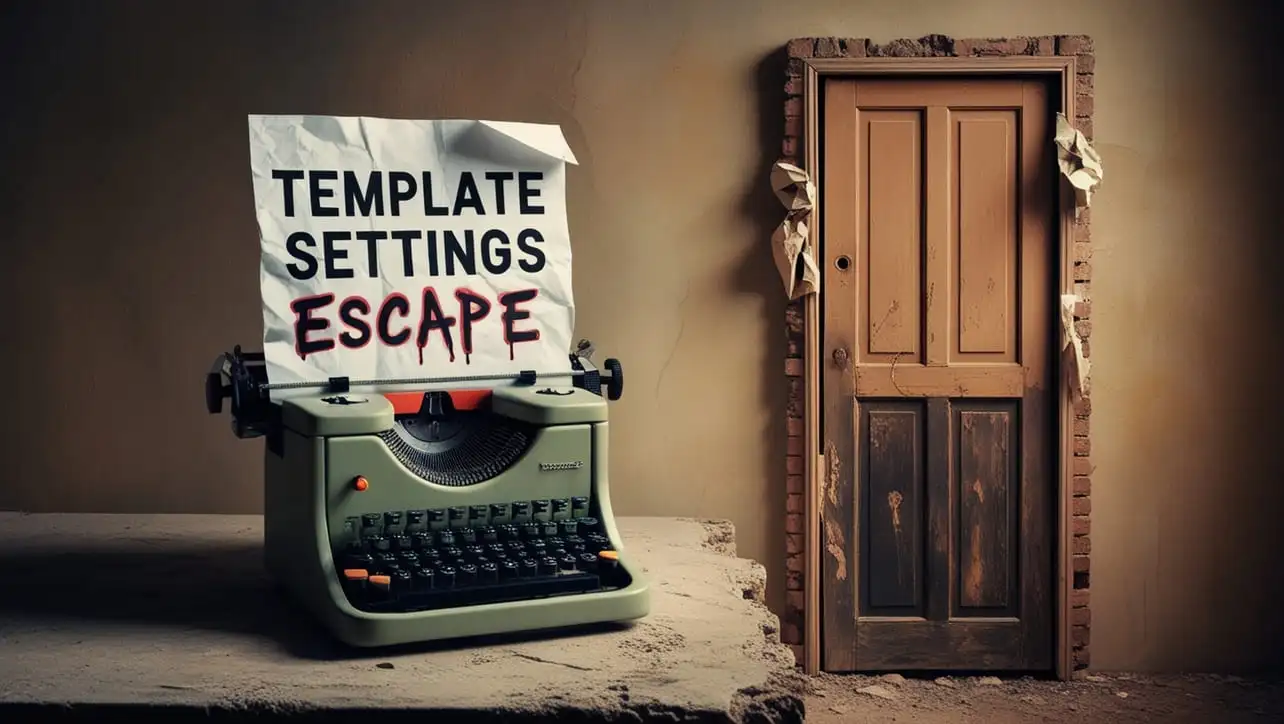
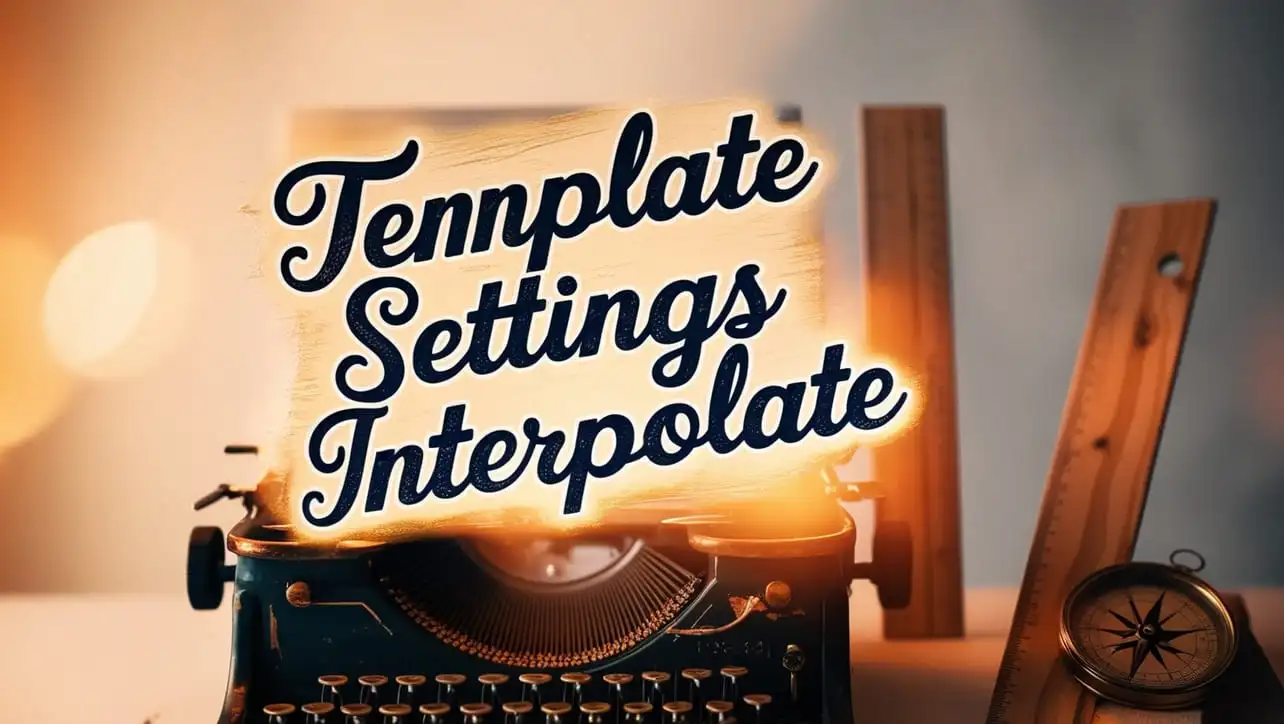
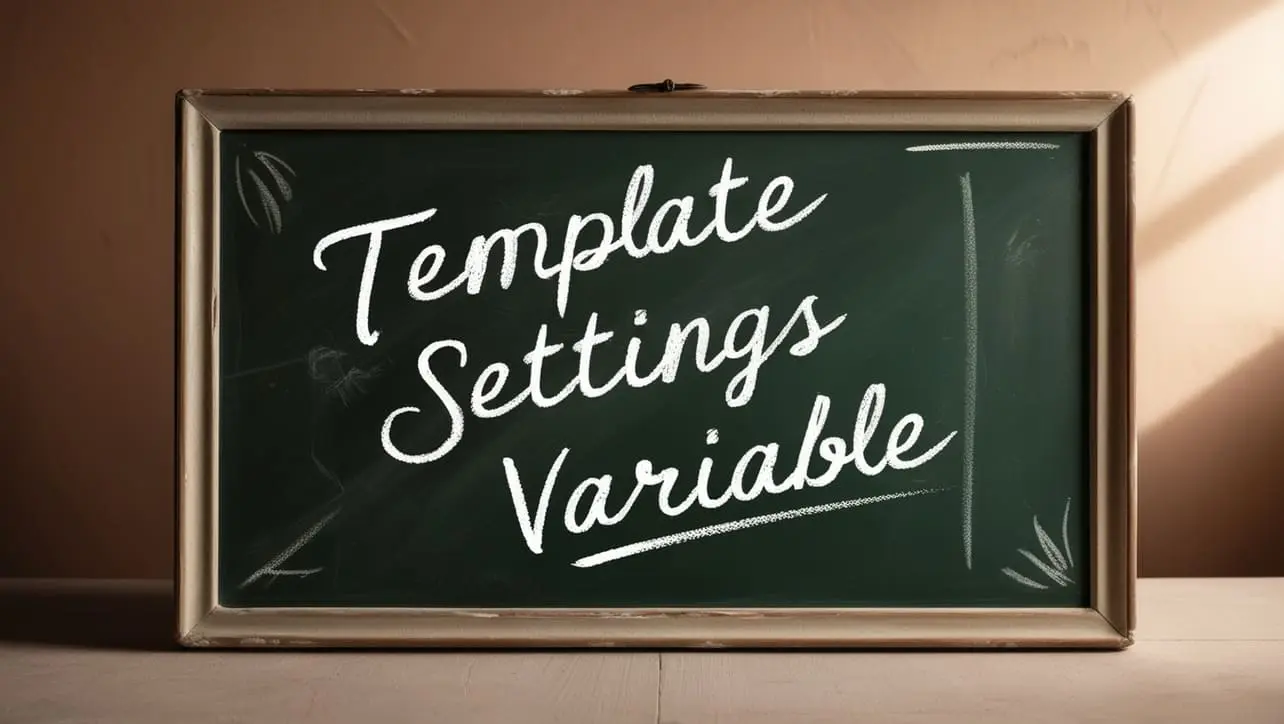
If you have any doubts regarding this article (Lodash _.toInteger() Lang Method), please comment here. I will help you immediately.