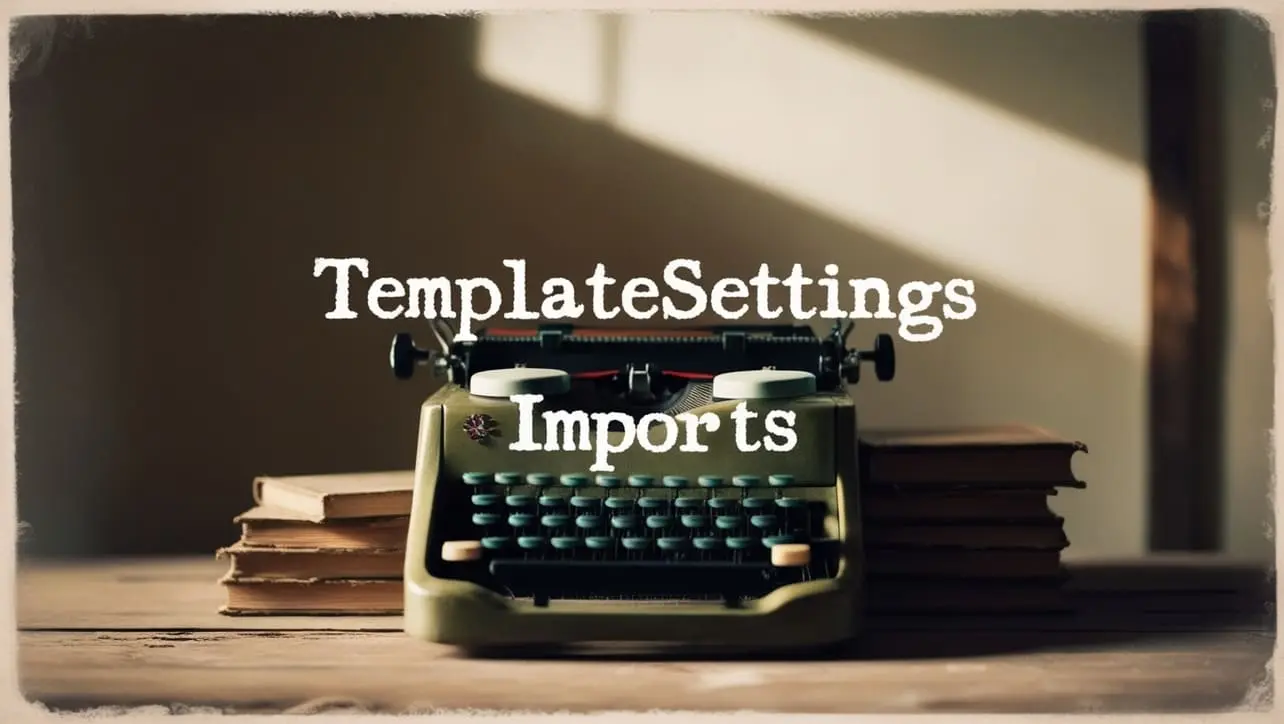
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toFinite() Lang Method
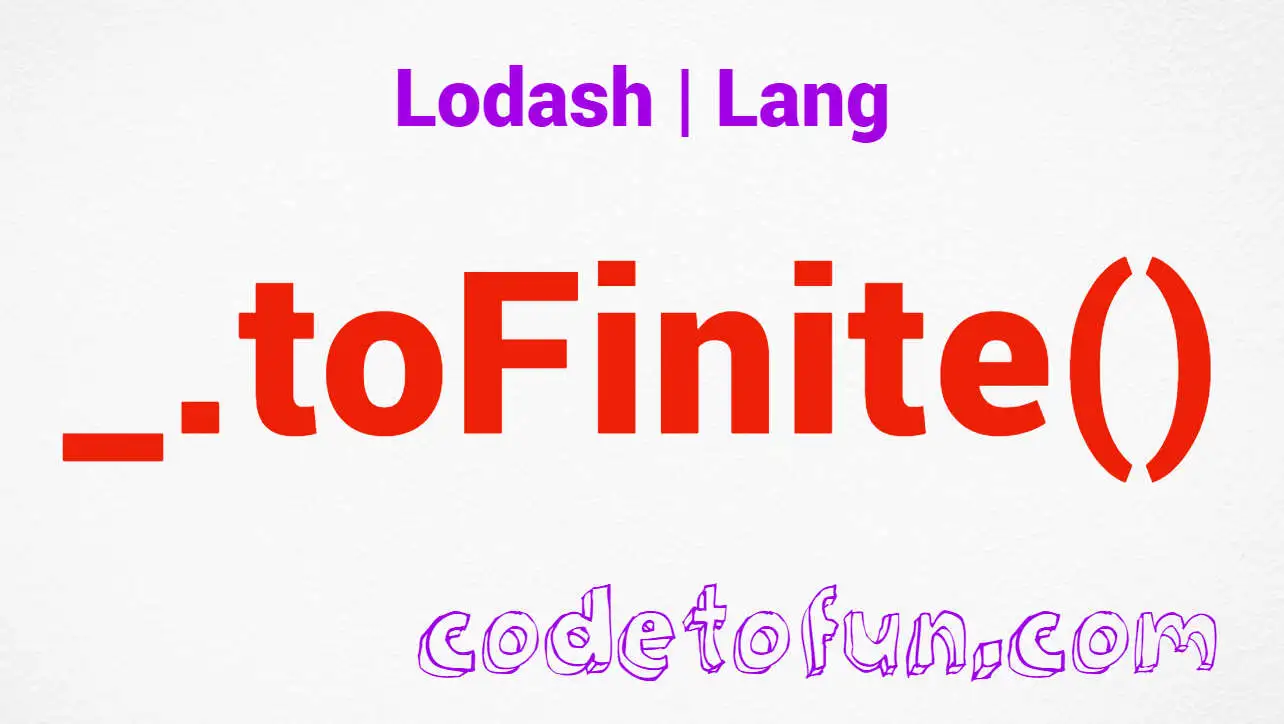
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript programming, dealing with numeric values is a common task. Lodash, a powerful utility library, provides a range of functions to streamline these operations. Among them, the _.toFinite()
method stands out as a versatile tool for converting values to finite numbers.
This method ensures that the output is a valid JavaScript number, preventing unexpected behaviors in your code.
🧠 Understanding _.toFinite() Method
The _.toFinite()
method in Lodash is designed to convert a given value to a finite number. This is particularly useful when working with user input, configuration settings, or any scenario where ensuring a valid numeric result is crucial.
💡 Syntax
The syntax for the _.toFinite()
method is straightforward:
_.toFinite(value)
- value: The value to convert.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toFinite()
method:
const _ = require('lodash');
const stringValue = '42';
const finiteNumber = _.toFinite(stringValue);
console.log(finiteNumber);
// Output: 42
In this example, the stringValue is converted to a finite number using _.toFinite()
, ensuring that the output is a valid numeric representation.
🏆 Best Practices
When working with the _.toFinite()
method, consider the following best practices:
Handling Non-Numeric Input:
Ensure that the input value is indeed a numeric type or a string representation of a number.
_.toFinite()
is designed for numeric conversion, and passing non-numeric values may result in unexpected outcomes.example.jsCopiedconst nonNumericValue = 'abc'; const convertedValue = _.toFinite(nonNumericValue); console.log(convertedValue); // Output: 0 (default value for non-numeric input)
Preventing NaN Results:
Consider using _.defaultTo() in conjunction with
_.toFinite()
to provide a default value in case the conversion results in NaN (Not-a-Number).example.jsCopiedconst inputValue = 'xyz'; const defaultNumericValue = 10; const convertedValue = _.defaultTo(_.toFinite(inputValue), defaultNumericValue); console.log(convertedValue); // Output: 10 (default value for non-numeric input)
Handling Infinity:
Be aware that
_.toFinite()
converts Infinity and -Infinity to finite numbers, potentially leading to unexpected results. Consider additional checks or validations if handling infinite values is critical.example.jsCopiedconst infinityValue = Infinity; const convertedValue = _.toFinite(infinityValue); console.log(convertedValue); // Output: 1.7976931348623157e+308 (a large finite number)
📚 Use Cases
User Input Processing:
When dealing with user input, especially from forms or external sources, use
_.toFinite()
to ensure that numeric input is correctly handled and prevent potential security vulnerabilities.example.jsCopiedconst userInput = /* ...retrieve user input... */; const numericValue = _.toFinite(userInput); console.log(numericValue);
Configuration Settings:
In scenarios where configuration values may be provided as strings, use
_.toFinite()
to convert these values to numbers for accurate processing.example.jsCopiedconst configValue = /* ...retrieve configuration setting... */; const numericConfig = _.toFinite(configValue); console.log(numericConfig);
Mathematical Calculations:
When performing mathematical calculations where the input may come in various formats, applying
_.toFinite()
can ensure consistent and reliable results.example.jsCopiedconst calculationInput = /* ...retrieve input for a mathematical operation... */; const numericInput = _.toFinite(calculationInput); console.log(numericInput);
🎉 Conclusion
The _.toFinite()
method in Lodash provides a straightforward solution for converting values to finite numbers in JavaScript. Whether you're processing user input, handling configuration settings, or performing mathematical operations, this method ensures that your numeric values are valid and eliminates potential pitfalls associated with non-numeric input.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toFinite()
method in your Lodash projects.
👨💻 Join our Community:
Author
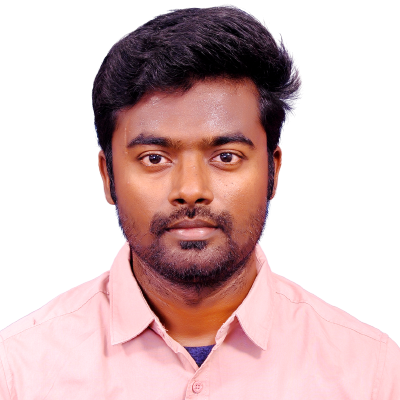
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
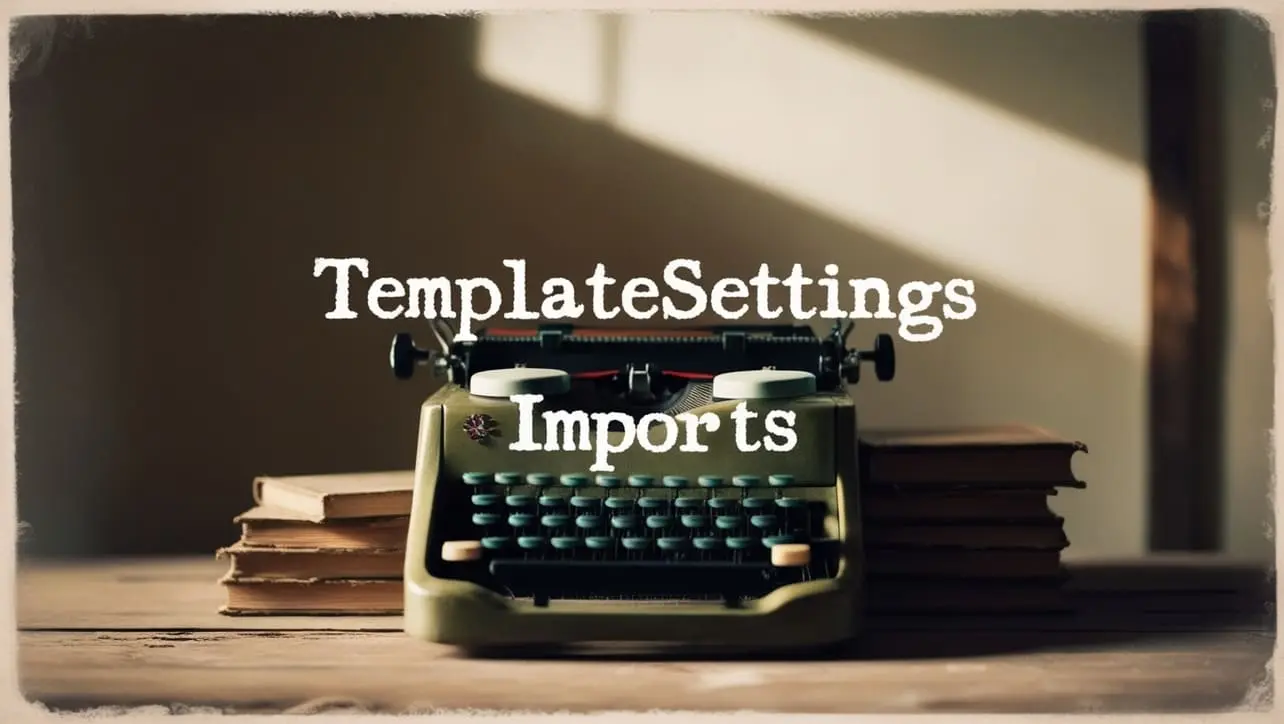
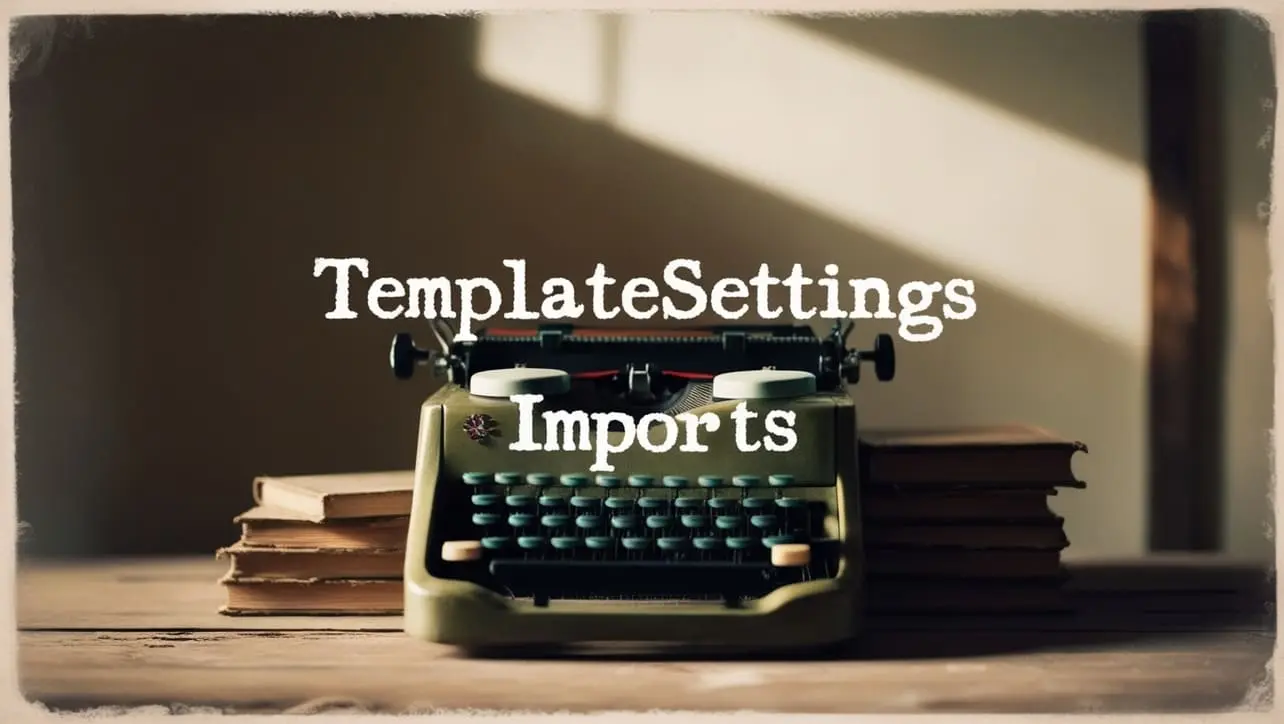
Lodash _.templateSettings.imports Property
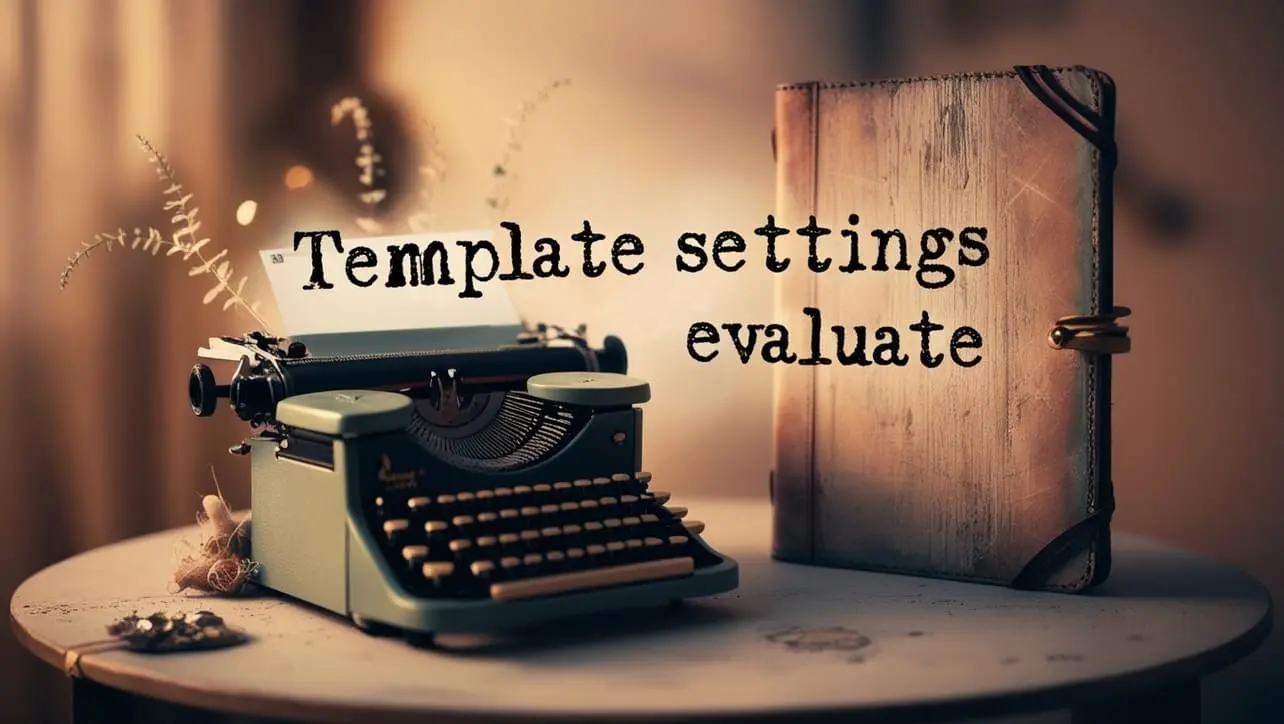
Lodash _.templateSettings.evaluate Property
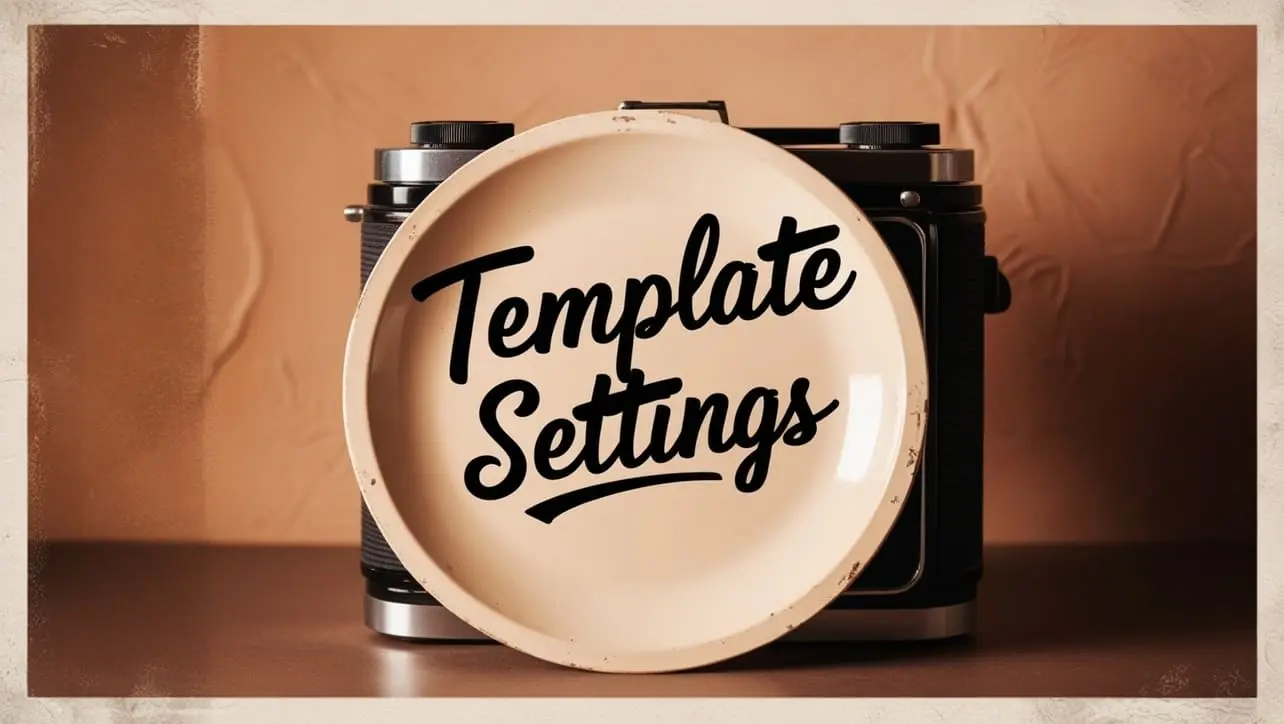
Lodash _.templateSettings Property
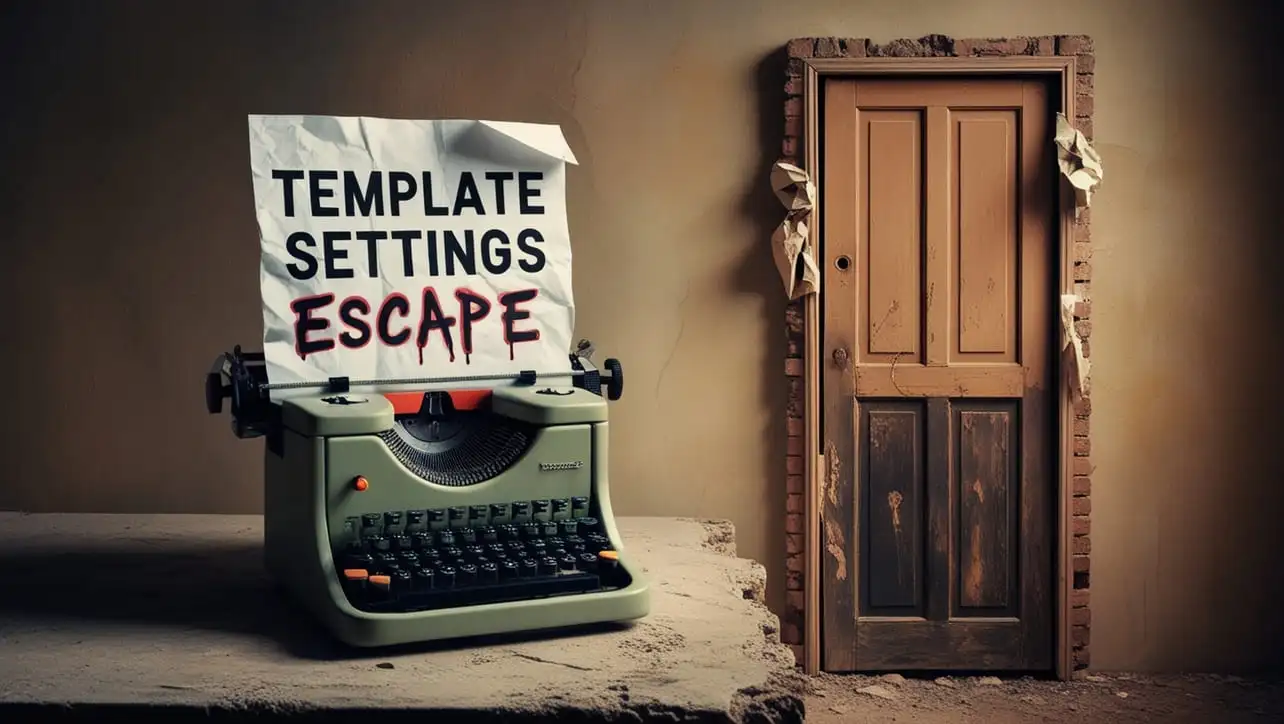
Lodash _.templateSettings.escape Property
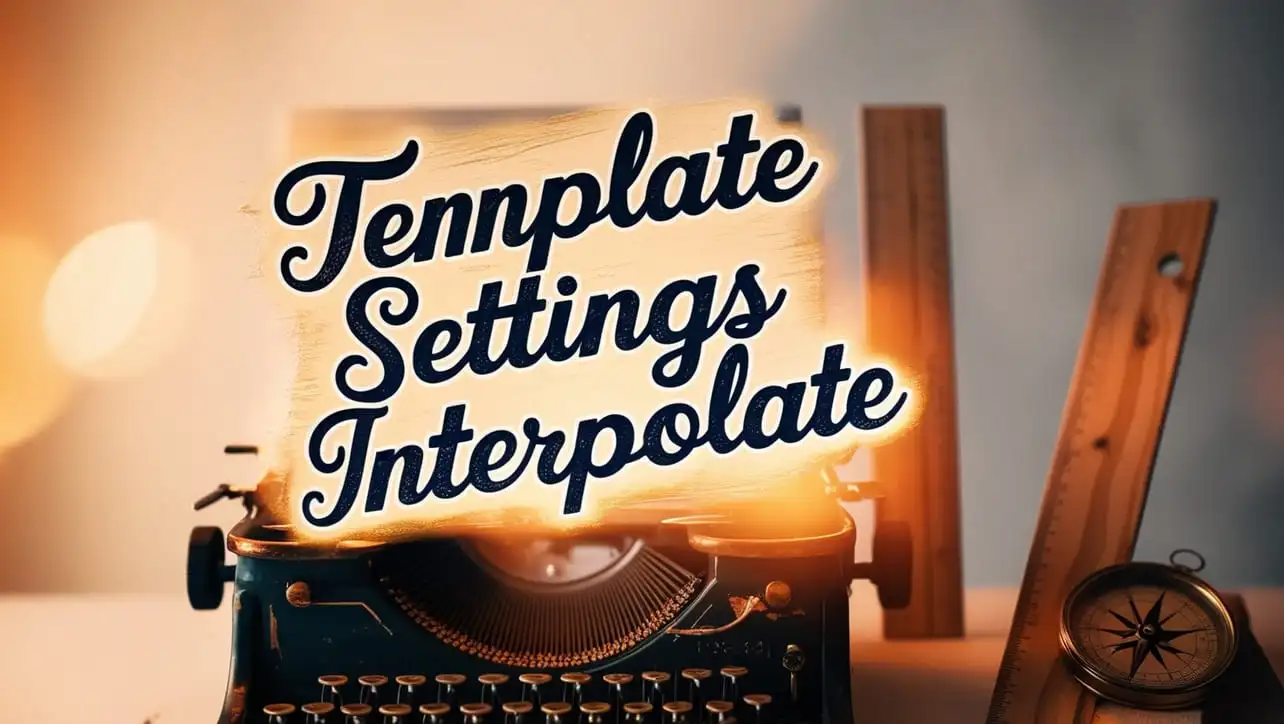
Lodash _.templateSettings.interpolate Property
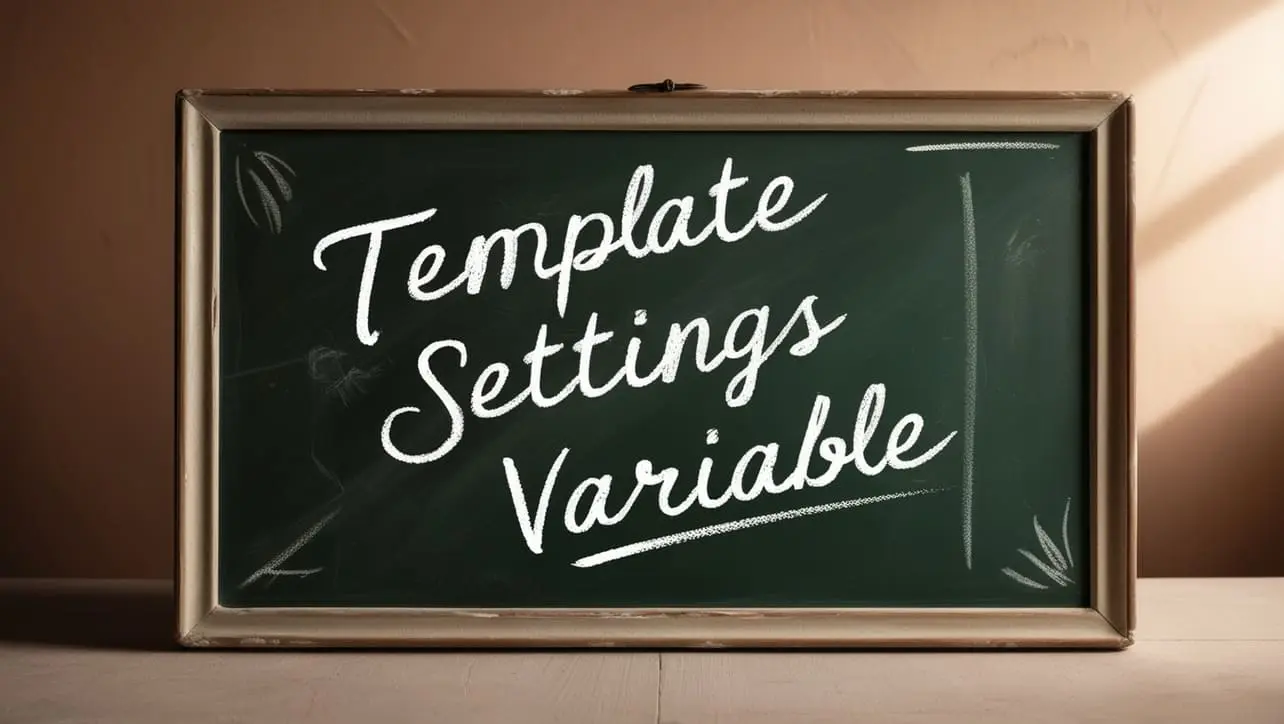
If you have any doubts regarding this article (Lodash _.toFinite() Lang Method), please comment here. I will help you immediately.