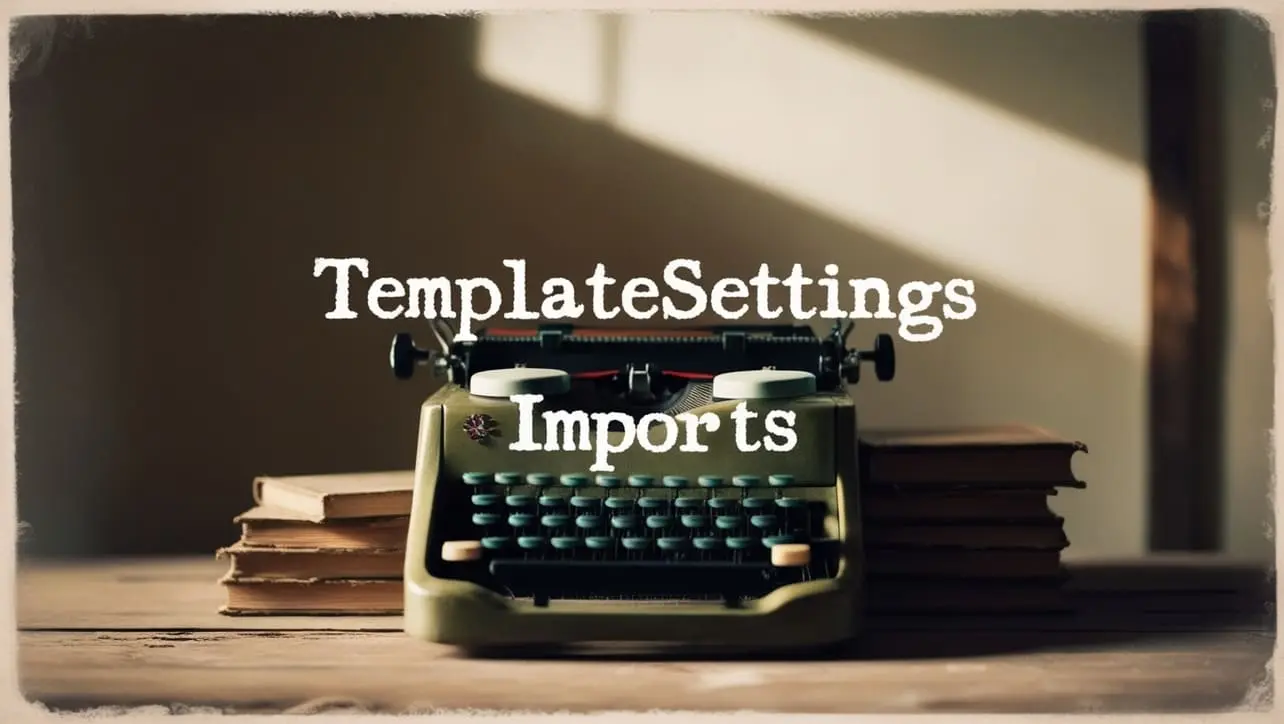
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.lte() Lang Method
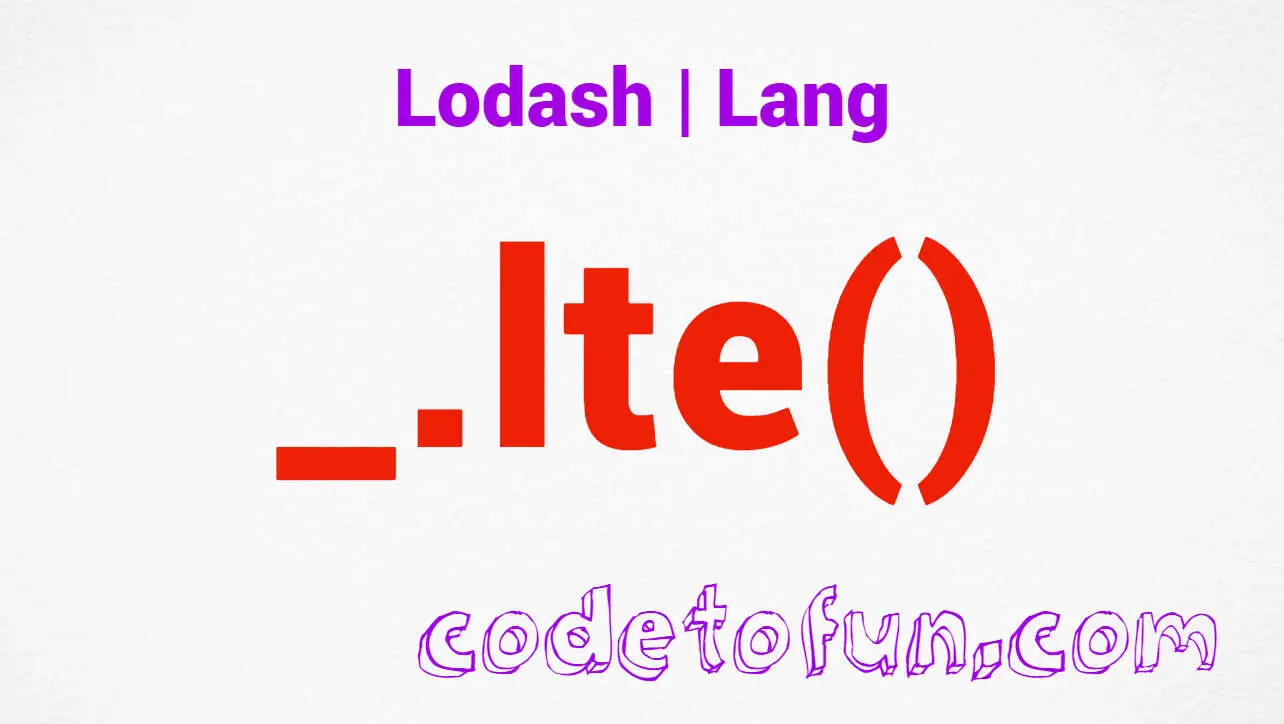
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, Lodash stands as a reliable utility library, offering a wealth of functions to simplify common programming tasks. Among these functions is the _.lte()
method, a member of the Lang category in Lodash. The _.lte()
method is designed to compare two values and determine if the first value is less than or equal to the second.
This functionality provides developers with a convenient tool for straightforward numeric comparisons, enhancing the clarity and conciseness of code.
🧠 Understanding _.lte() Method
The _.lte()
method in Lodash performs a less than or equal to comparison between two values. It returns true if the first value is less than or equal to the second; otherwise, it returns false. This method is particularly useful for numeric comparisons but can also be applied to other data types.
💡 Syntax
The syntax for the _.lte()
method is straightforward:
_.lte(value, other)
- value: The first value to compare.
- other: The second value for comparison.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.lte()
method:
const _ = require('lodash');
const num1 = 5;
const num2 = 10;
const result = _.lte(num1, num2);
console.log(result);
// Output: true
In this example, _.lte()
is used to compare num1 and num2, returning true since 5 is less than 10.
🏆 Best Practices
When working with the _.lte()
method, consider the following best practices:
Use with Numeric Values:
While
_.lte()
can be applied to various data types, it is most commonly used with numeric values for straightforward less than or equal to comparisons.example.jsCopiedconst numericValue1 = 15; const numericValue2 = 20; const isLessThanOrEqualTo = _.lte(numericValue1, numericValue2); console.log(isLessThanOrEqualTo); // Output: true
Leverage in Conditional Statements:
Integrate
_.lte()
into conditional statements to make your code more expressive and readable.example.jsCopiedconst quantityInStock = 100; const orderQuantity = 120; if (_.lte(quantityInStock, orderQuantity)) { console.log('Order can be fulfilled.'); } else { console.log('Insufficient stock for the order.'); }
Combine with Other Comparison Methods:
Combine
_.lte()
with other comparison methods from Lodash for more complex logic in your applications.example.jsCopiedconst value1 = 'abc'; const value2 = 'def'; const isAlphabeticallyBeforeOrEqual = _.lte(value1.localeCompare(value2), 0); console.log(isAlphabeticallyBeforeOrEqual); // Output: true
📚 Use Cases
Inventory Management:
Use
_.lte()
in scenarios such as inventory management to determine if the available quantity is less than or equal to the requested quantity.example.jsCopiedconst availableQuantity = 50; const requestedQuantity = 60; const canFulfillOrder = _.lte(availableQuantity, requestedQuantity); console.log(canFulfillOrder); // Output: false
Date Comparisons:
Apply
_.lte()
in date comparisons to check if one date is less than or equal to another.example.jsCopiedconst currentDate = new Date(); const deadlineDate = new Date('2022-12-31'); const isBeforeOrEqualDeadline = _.lte(currentDate, deadlineDate); console.log(isBeforeOrEqualDeadline); // Output: true
Scoreboard Rankings:
Utilize
_.lte()
to compare scores in a scoreboard and determine if a player's score is less than or equal to another player's score.example.jsCopiedconst player1Score = 1500; const player2Score = 1800; const isPlayer1BehindOrEqual = _.lte(player1Score, player2Score); console.log(isPlayer1BehindOrEqual); // Output: true
🎉 Conclusion
The _.lte()
method in Lodash provides a simple yet powerful tool for less than or equal to comparisons in JavaScript. Whether you're dealing with numeric values, dates, or other data types, _.lte()
offers a concise and expressive way to enhance your code's readability and logic.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.lte()
method in your Lodash projects.
👨💻 Join our Community:
Author
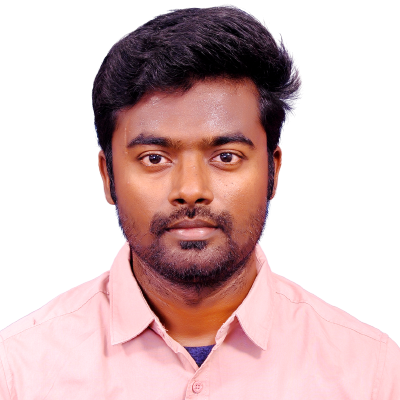
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
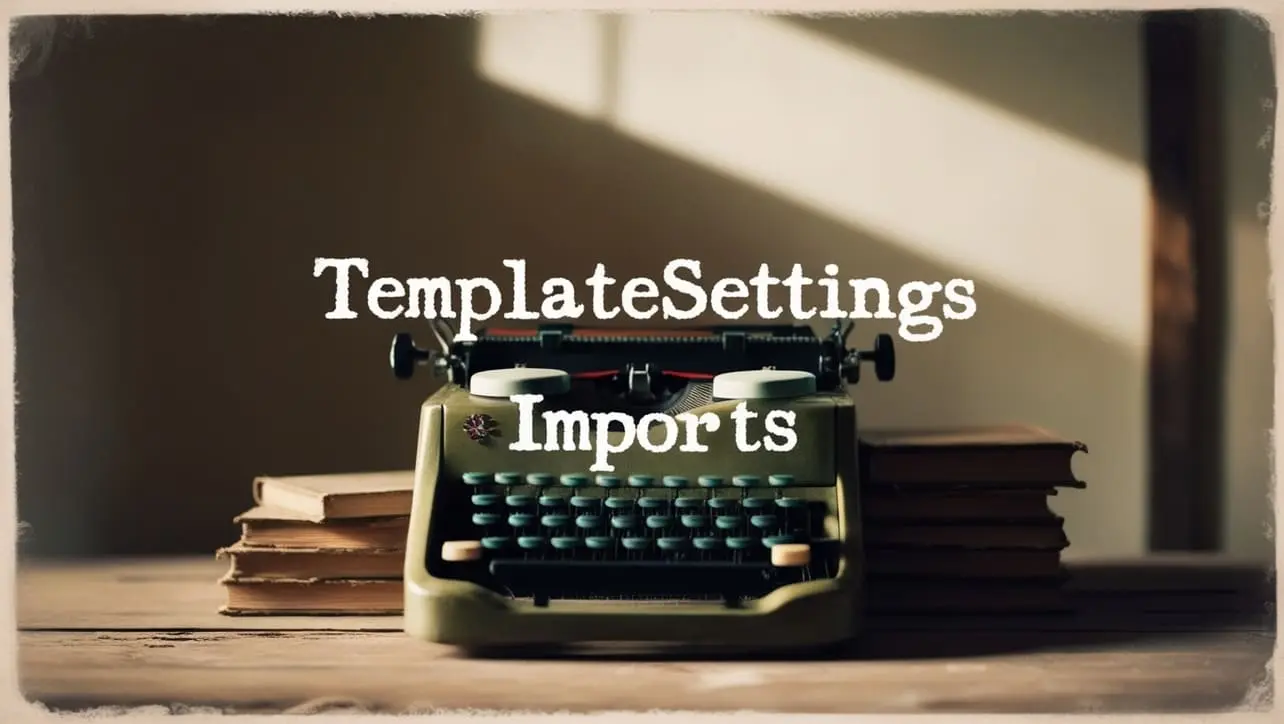
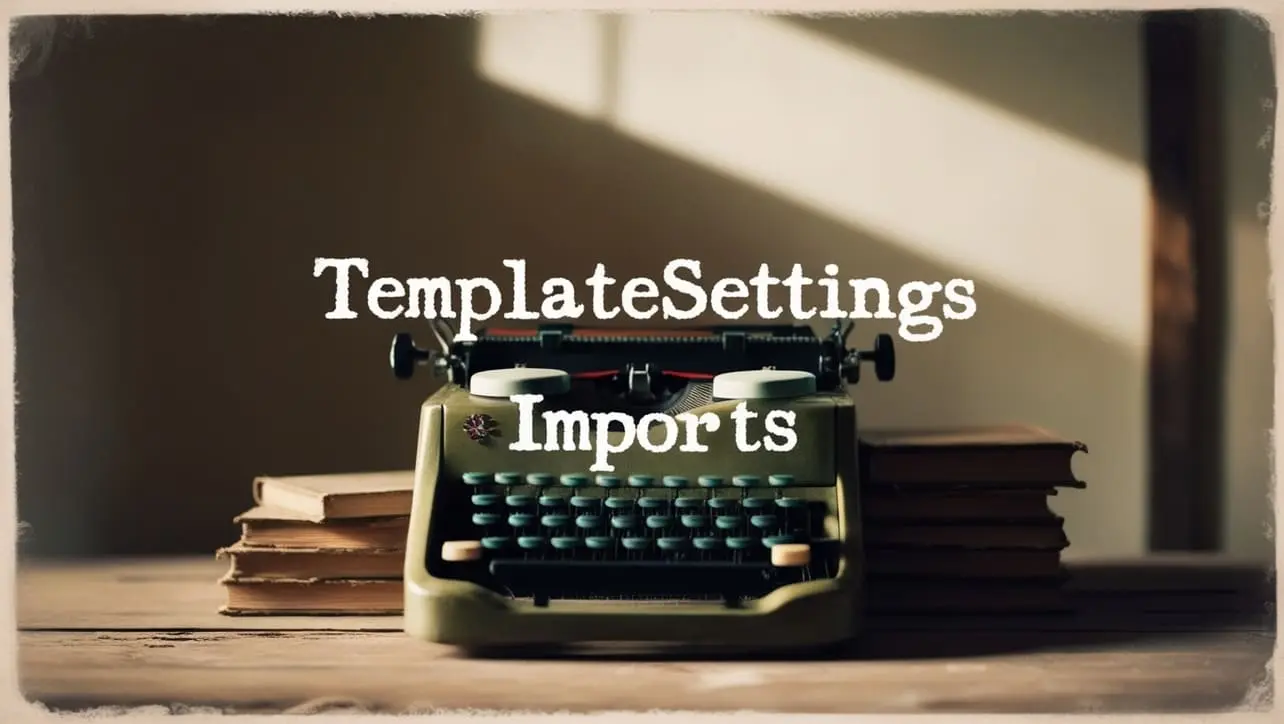
Lodash _.templateSettings.imports Property
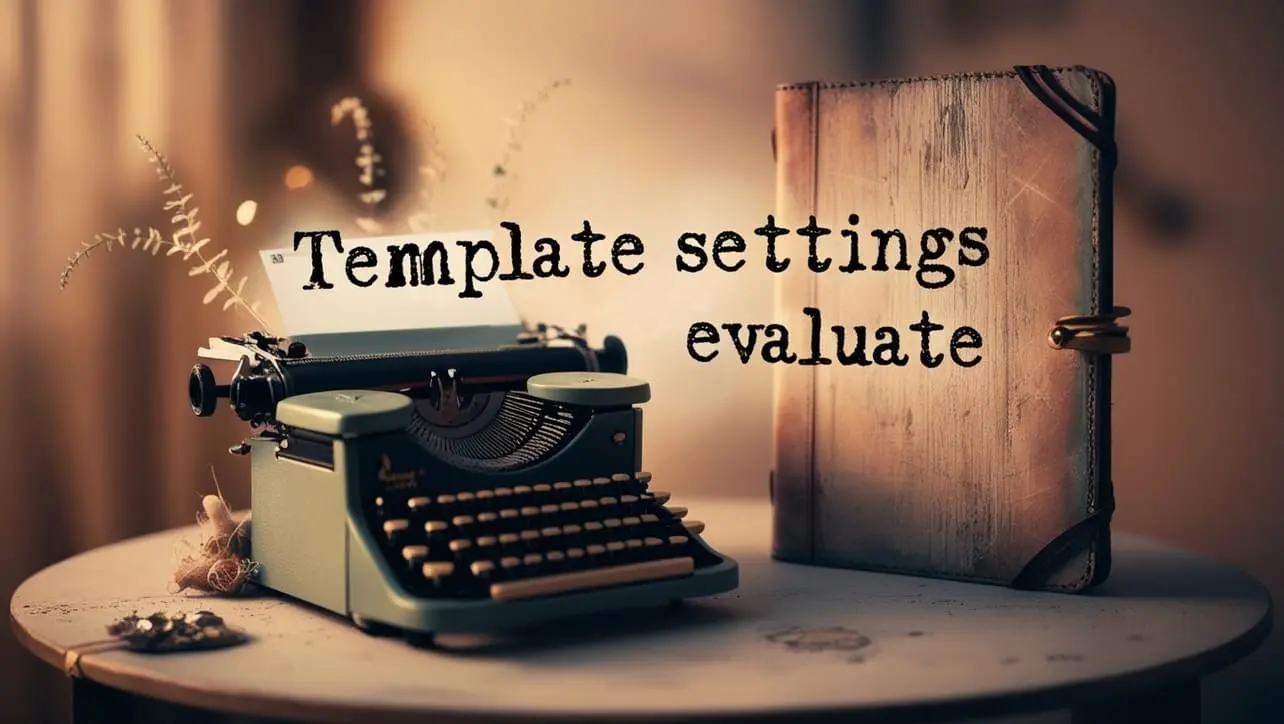
Lodash _.templateSettings.evaluate Property
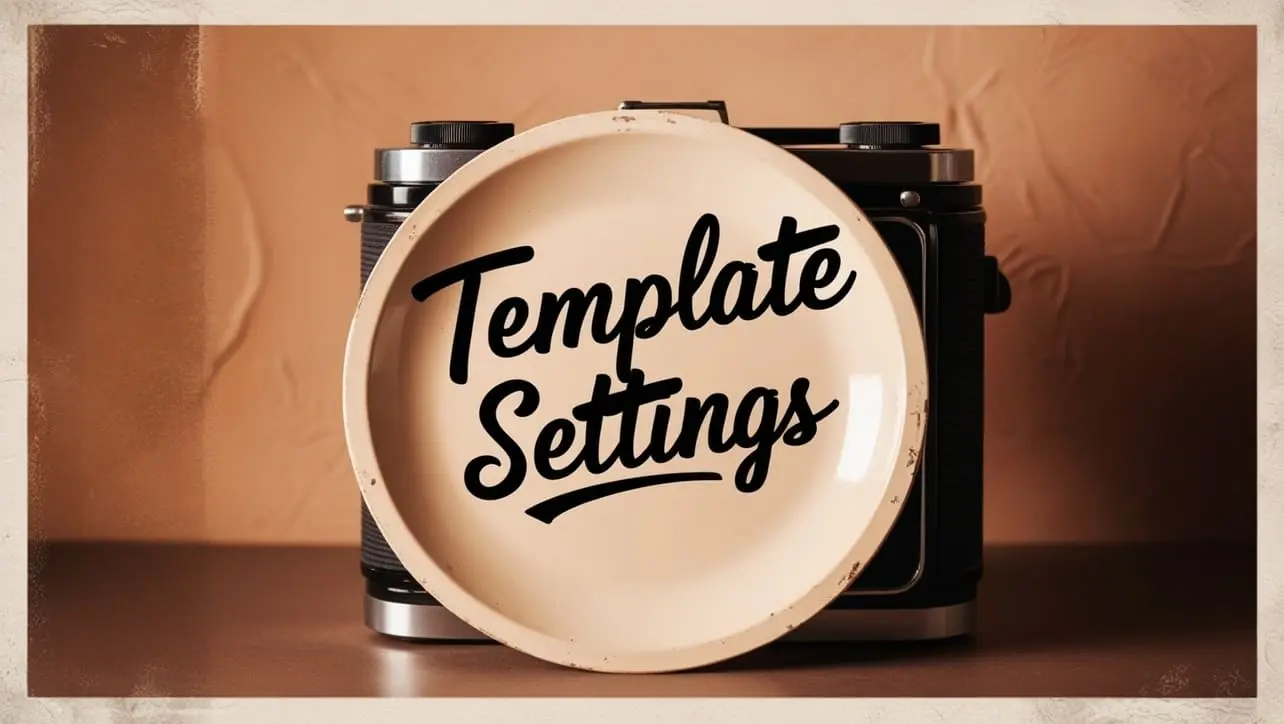
Lodash _.templateSettings Property
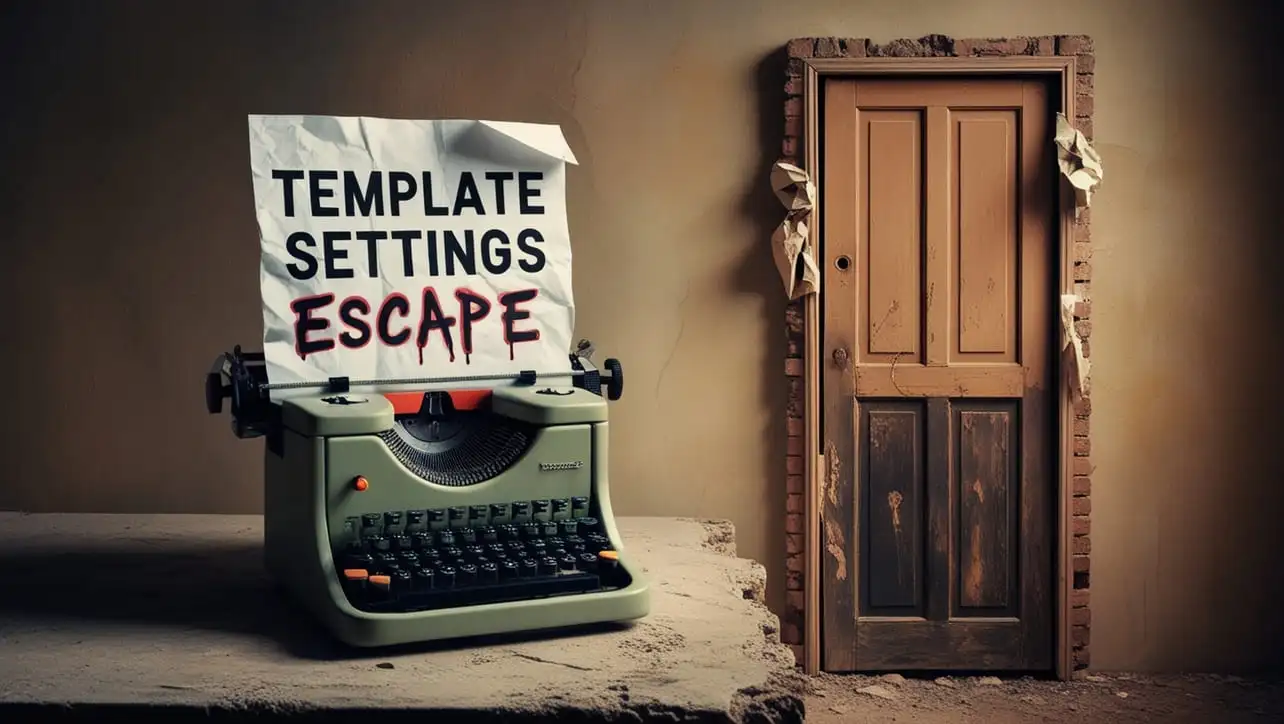
Lodash _.templateSettings.escape Property
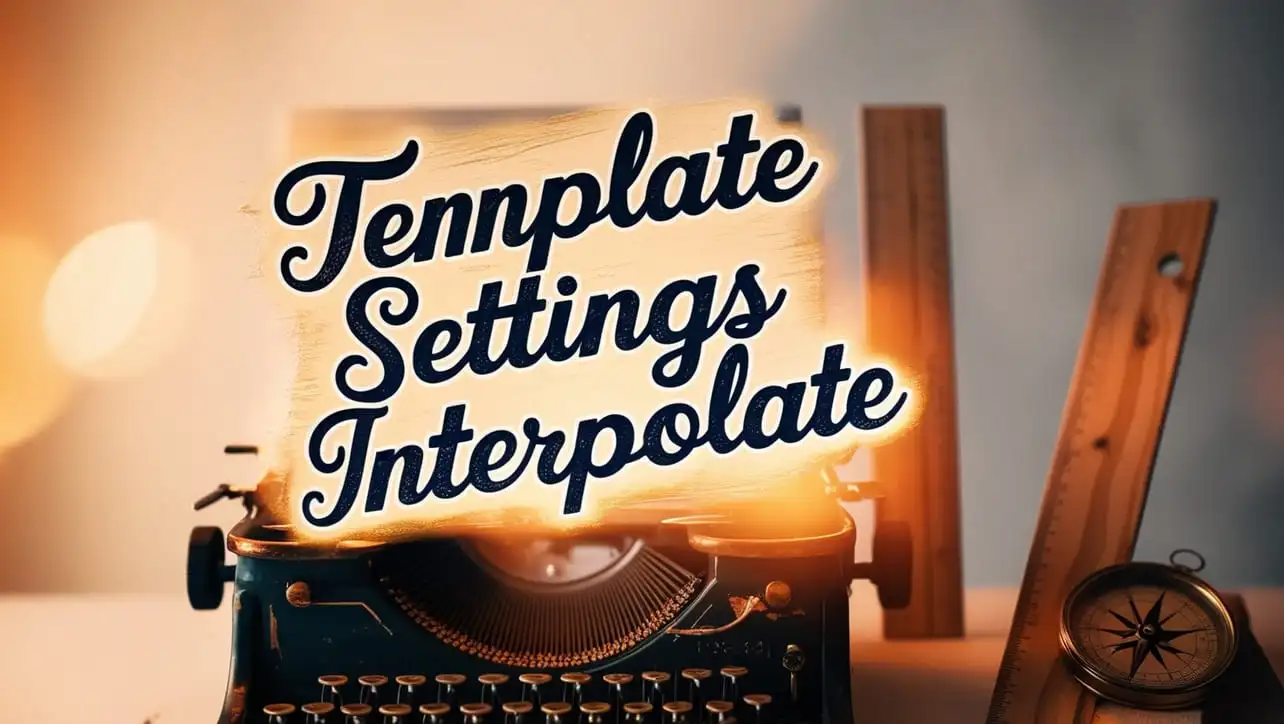
Lodash _.templateSettings.interpolate Property
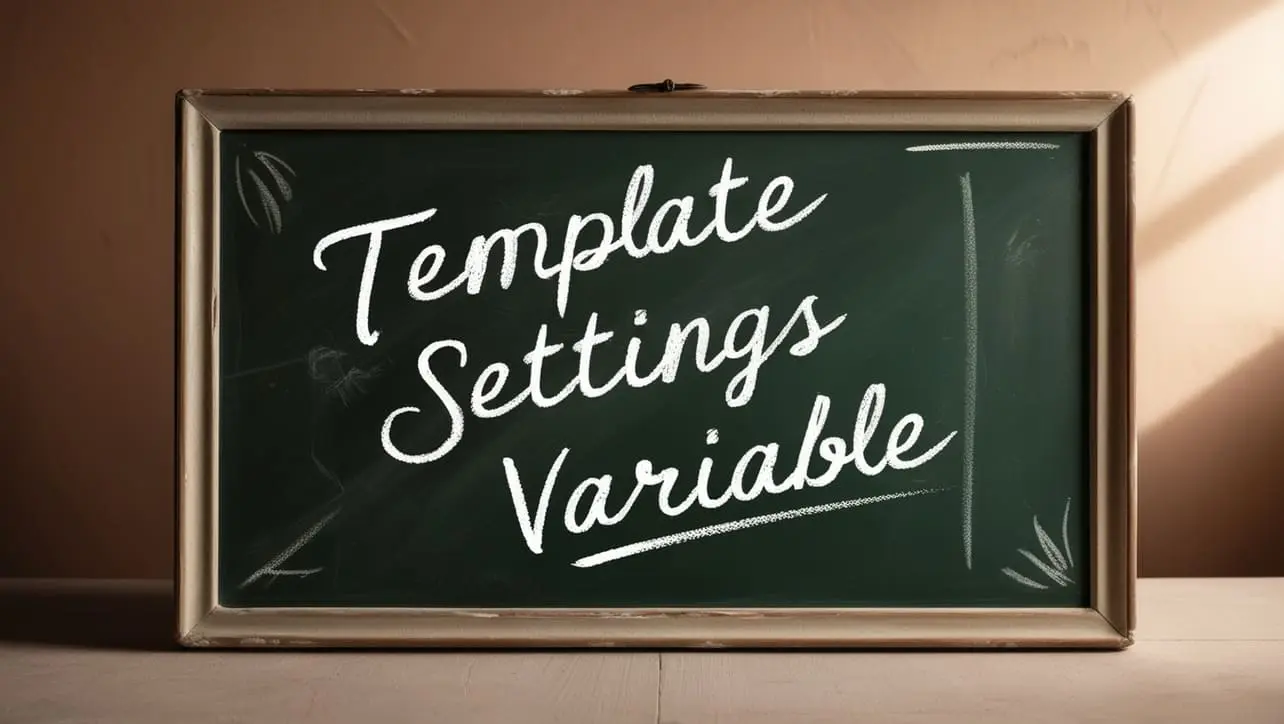
If you have any doubts regarding this article (Lodash _.lte() Lang Method), please comment here. I will help you immediately.