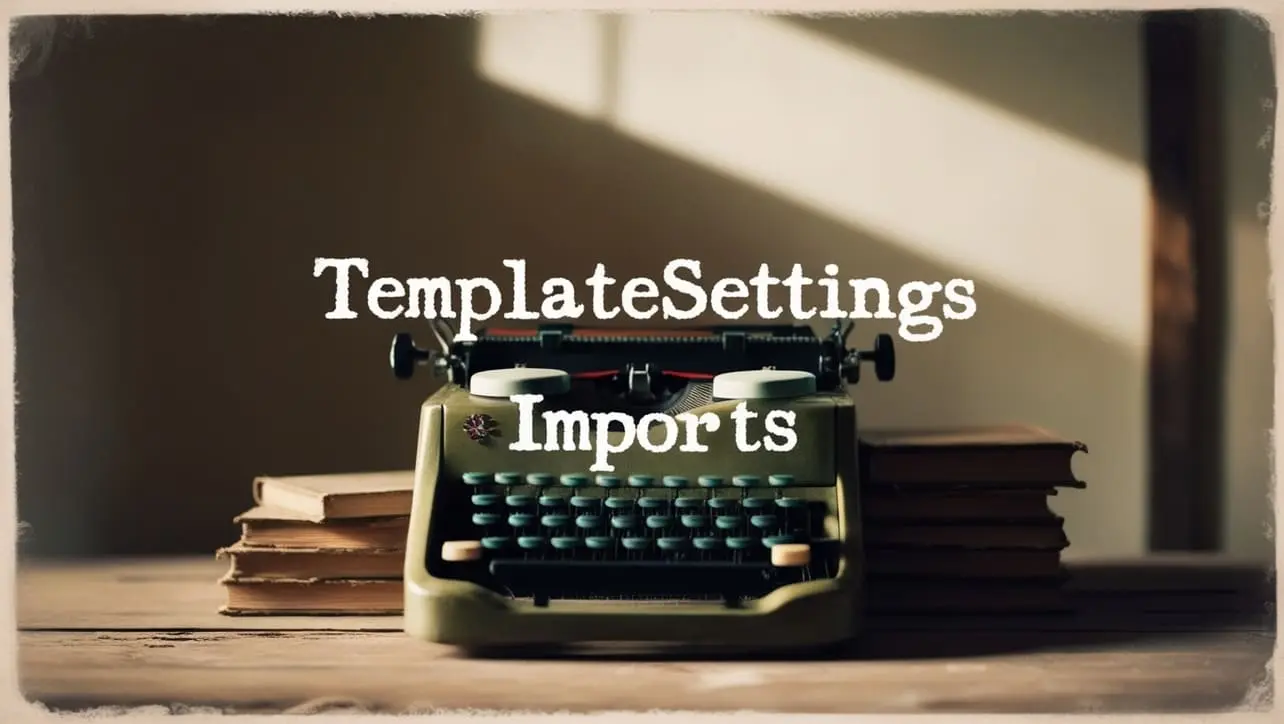
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.lt() Lang Method
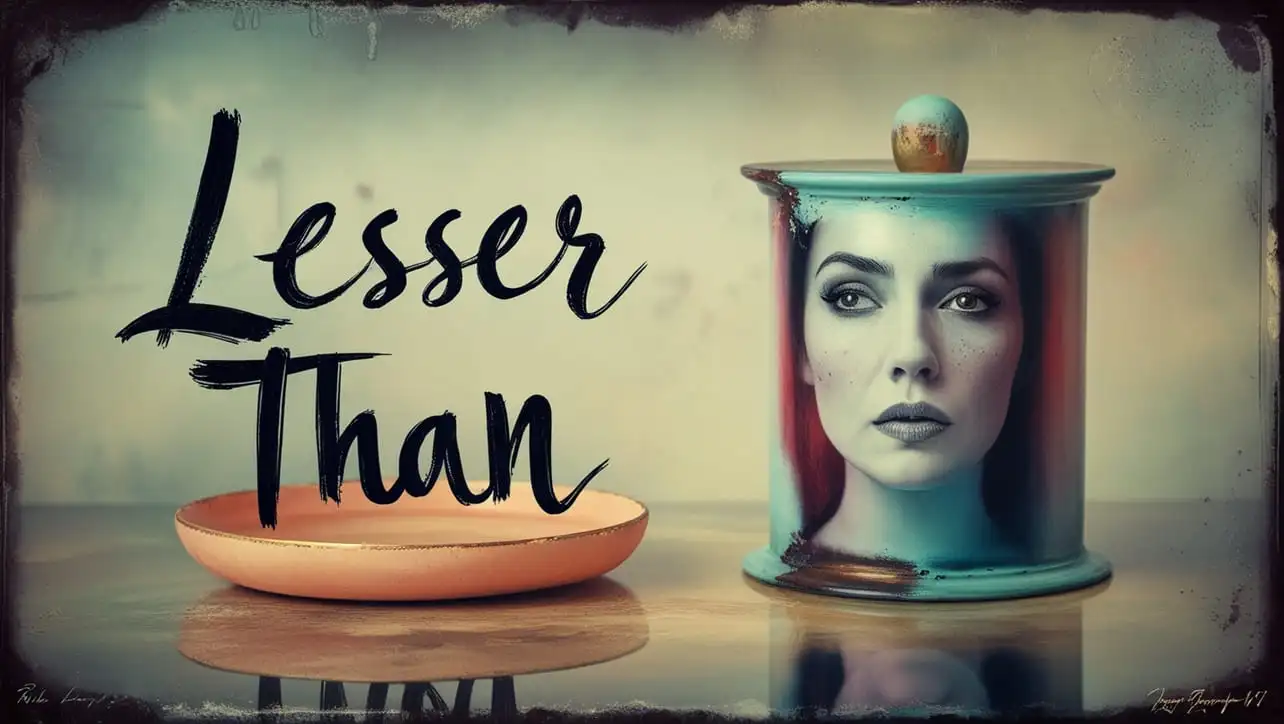
Photo Credit to CodeToFun
🙋 Introduction
In the diverse landscape of JavaScript development, handling comparisons is a fundamental task. Lodash, a feature-rich utility library, offers a variety of functions to simplify such operations. One notable method is _.lt()
, a member of the Lang category, providing an elegant solution for less-than comparisons.
This method proves invaluable when dealing with numerical or string comparisons, offering a concise and expressive syntax.
🧠 Understanding _.lt() Method
The _.lt()
method in Lodash facilitates the comparison of two values, determining whether the first value is less than the second. This straightforward functionality becomes particularly useful when implementing conditional logic or sorting operations in your JavaScript code.
💡 Syntax
The syntax for the _.lt()
method is straightforward:
_.lt(value, other)
- value: The first value to compare.
- other: The second value for comparison.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.lt()
method:
const _ = require('lodash');
const num1 = 5;
const num2 = 10;
const isLessThan = _.lt(num1, num2);
console.log(isLessThan);
// Output: true
In this example, _.lt()
compares num1 and num2, returning true since 5 is indeed less than 10.
🏆 Best Practices
When working with the _.lt()
method, consider the following best practices:
Type Coercion Awareness:
Be aware of the potential for type coercion when using
_.lt()
. JavaScript may perform type conversion, especially when comparing values of different types.example.jsCopiedconst stringNumber = '5'; const actualNumber = 5; const coercedResult = _.lt(stringNumber, actualNumber); console.log(coercedResult); // Output: true (due to type coercion)
Use Cases Beyond Numbers:
While commonly used for numerical comparisons,
_.lt()
is versatile and applicable to other data types, such as strings.example.jsCopiedconst string1 = 'apple'; const string2 = 'banana'; const isLessThanStrings = _.lt(string1, string2); console.log(isLessThanStrings); // Output: true
Combine with Logical Operators:
Enhance the power of
_.lt()
by combining it with logical operators for complex conditions.example.jsCopiedconst age = 25; const legalDrinkingAge = 21; const isEligibleToDrink = _.lt(age, legalDrinkingAge); console.log(isEligibleToDrink ? 'Cheers!' : 'Sorry, too young.');
📚 Use Cases
Conditional Rendering:
Utilize
_.lt()
in scenarios where conditional rendering is required, dynamically displaying content based on the comparison results.example.jsCopiedconst thresholdValue = 100; const currentValue = /* ...fetch current value... */ ; if (_.lt(currentValue, thresholdValue)) { console.log('Value is below the threshold. Take action!'); } else { console.log('Value is equal to or above the threshold. No action needed.'); }
Sorting Arrays:
In array sorting algorithms,
_.lt()
can be employed to define custom sorting criteria.example.jsCopiedconst unsortedNumbers = [10, 5, 8, 2, 15]; const sortedNumbers = _.sortBy(unsortedNumbers, num => num); console.log(sortedNumbers); // Output: [2, 5, 8, 10, 15]
Custom Comparisons:
For complex objects or custom data structures,
_.lt()
allows you to define custom comparison logic.example.jsCopiedconst person1 = { age: 25 }; const person2 = { age: 30 }; const isYounger = _.lt(person1.age, person2.age); console.log(isYounger); // Output: true
🎉 Conclusion
The _.lt()
method in Lodash serves as a reliable tool for less-than comparisons, offering simplicity and flexibility in your JavaScript code. Whether you're working with numerical values, strings, or implementing custom comparisons, _.lt()
provides an expressive and concise solution.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.lt()
method in your Lodash projects.
👨💻 Join our Community:
Author
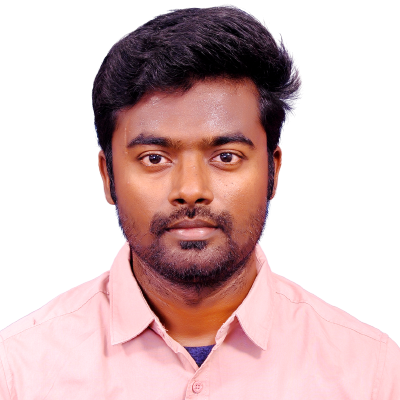
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
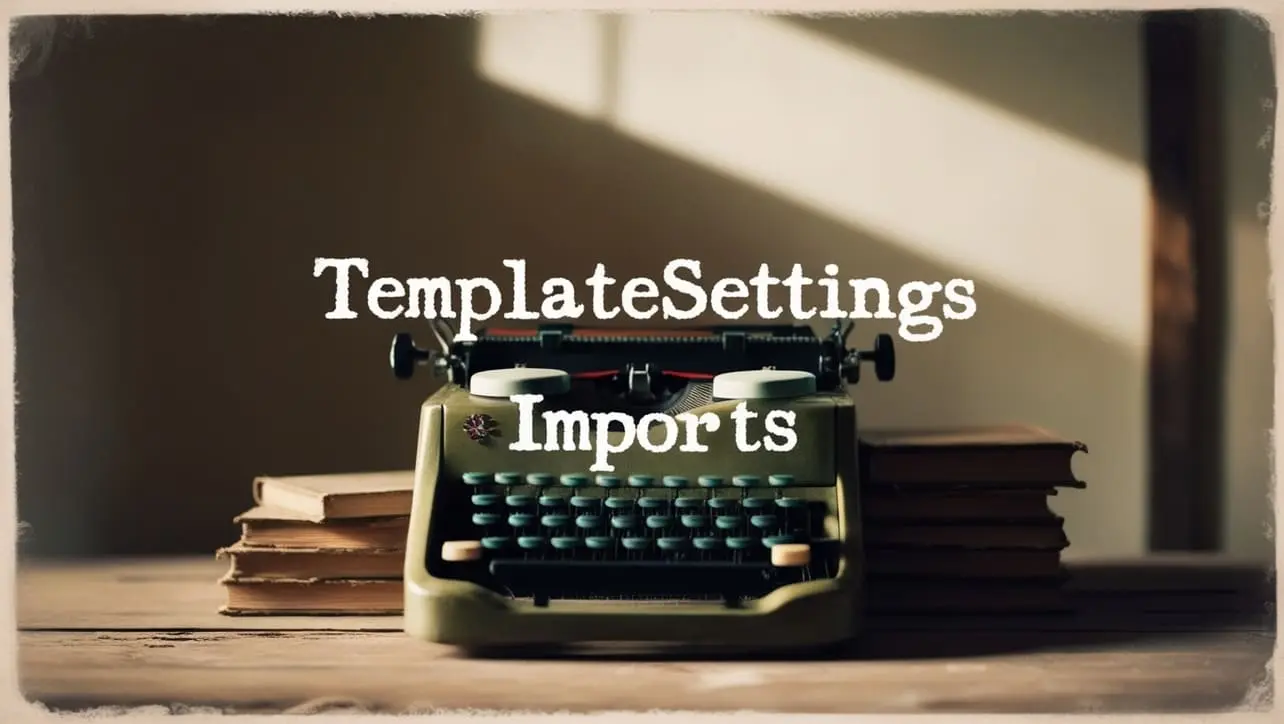
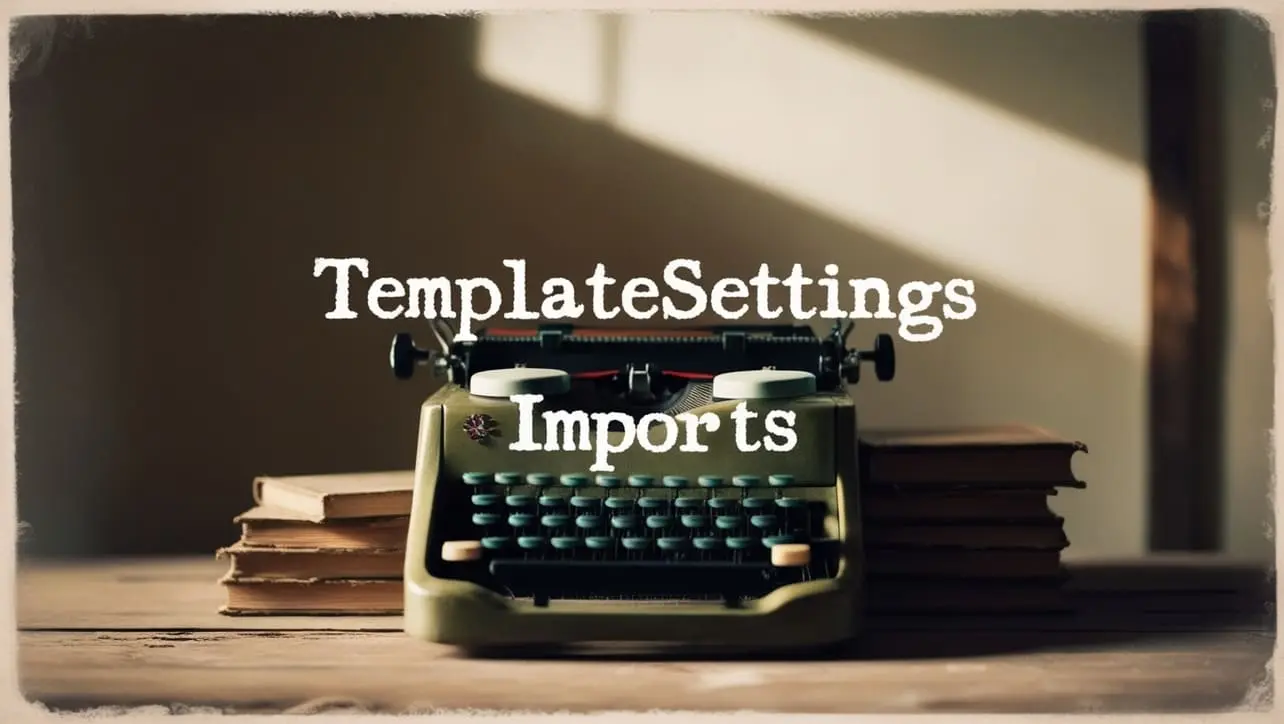
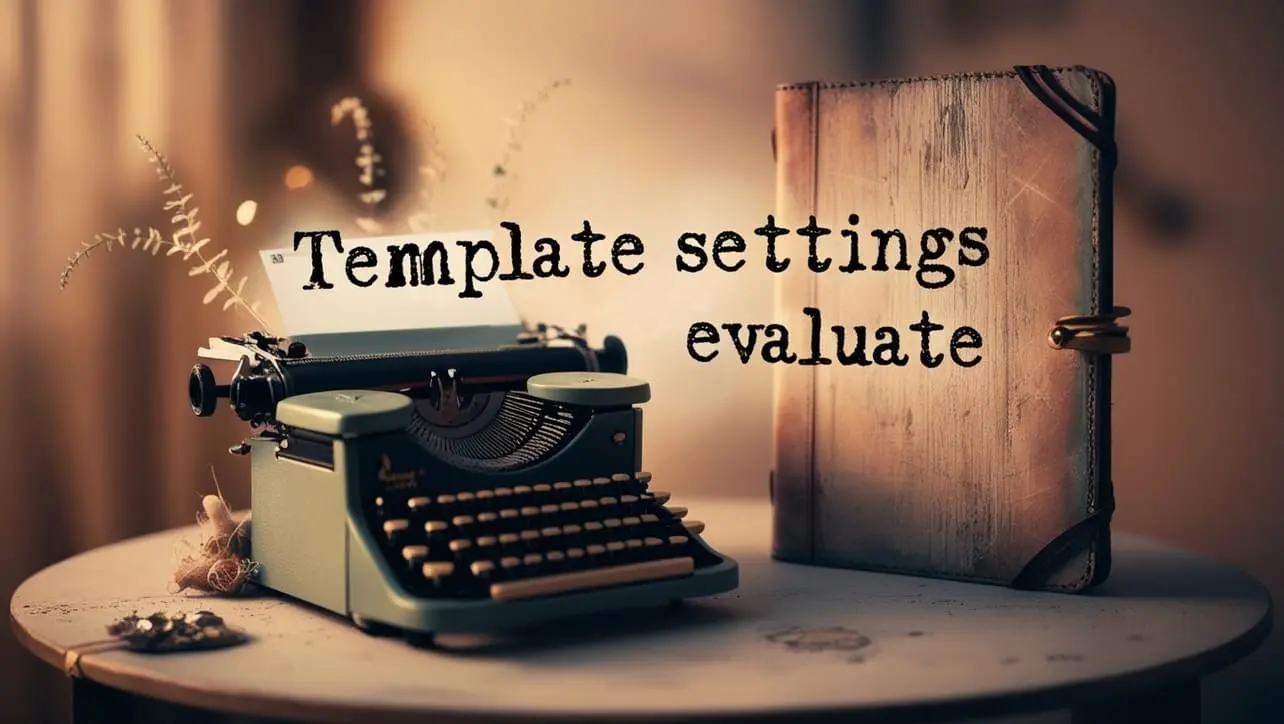
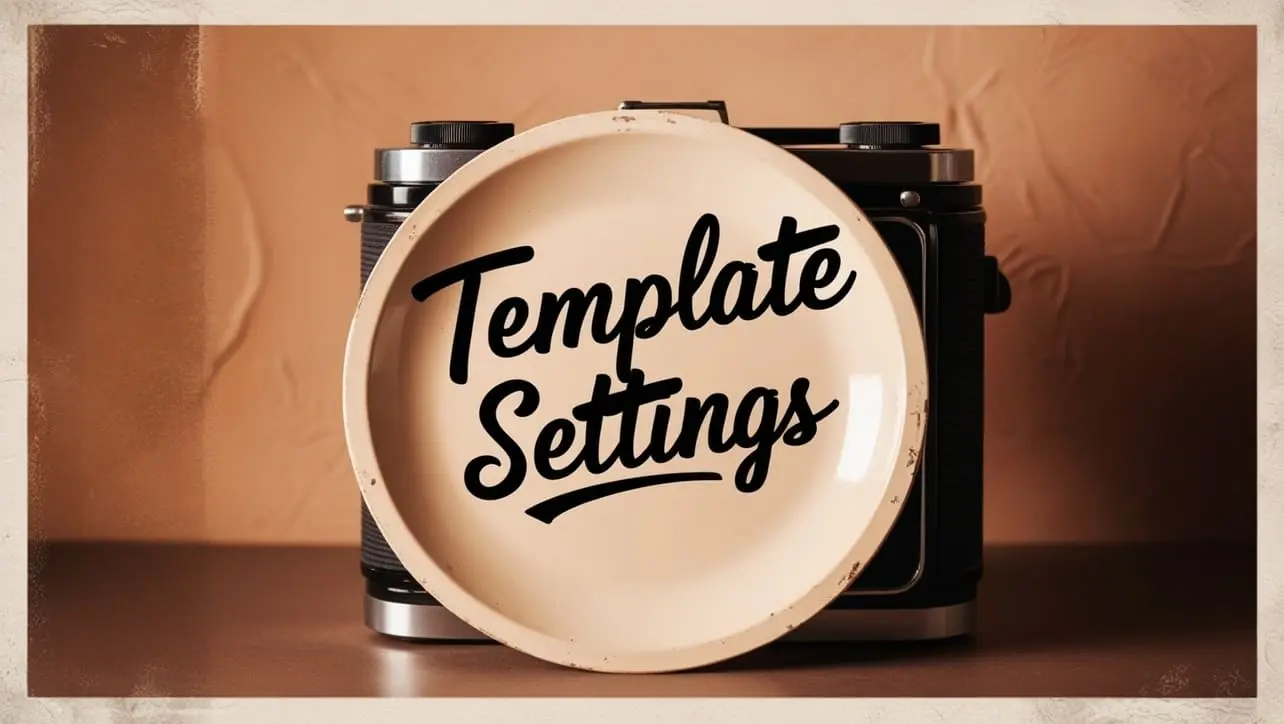
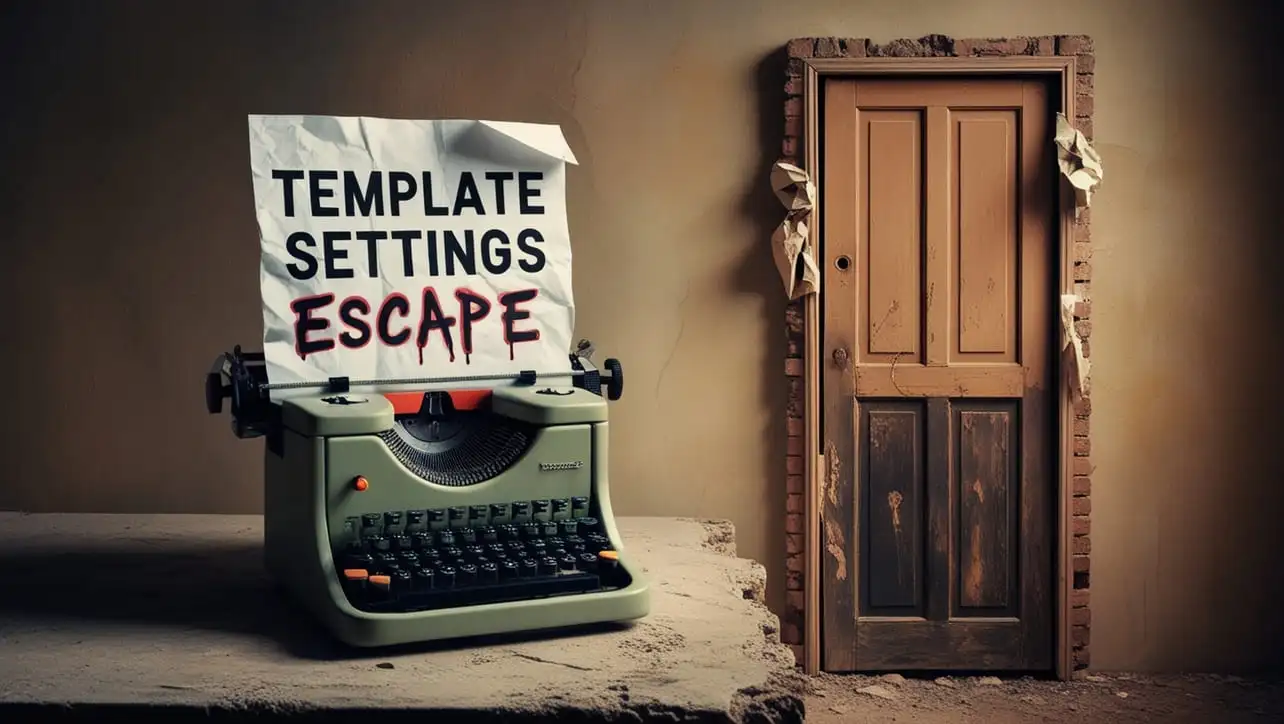
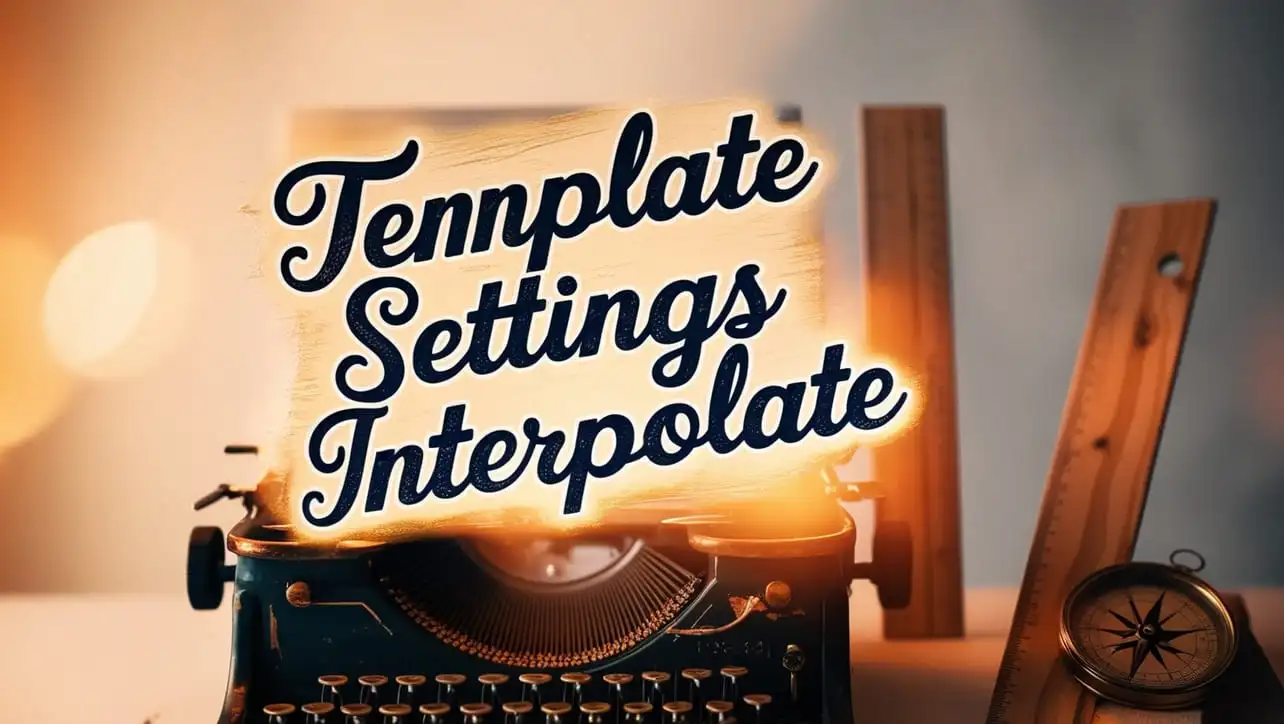
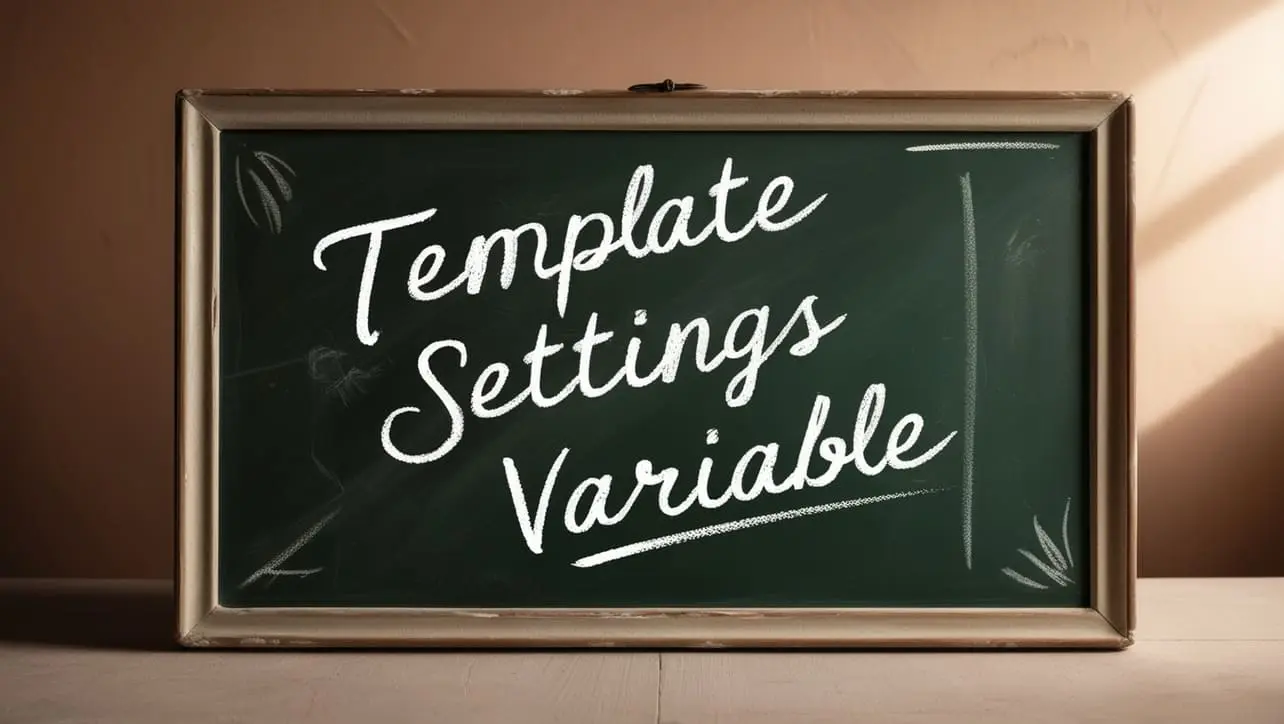
If you have any doubts regarding this article (Lodash _.lt() Lang Method), please comment here. I will help you immediately.