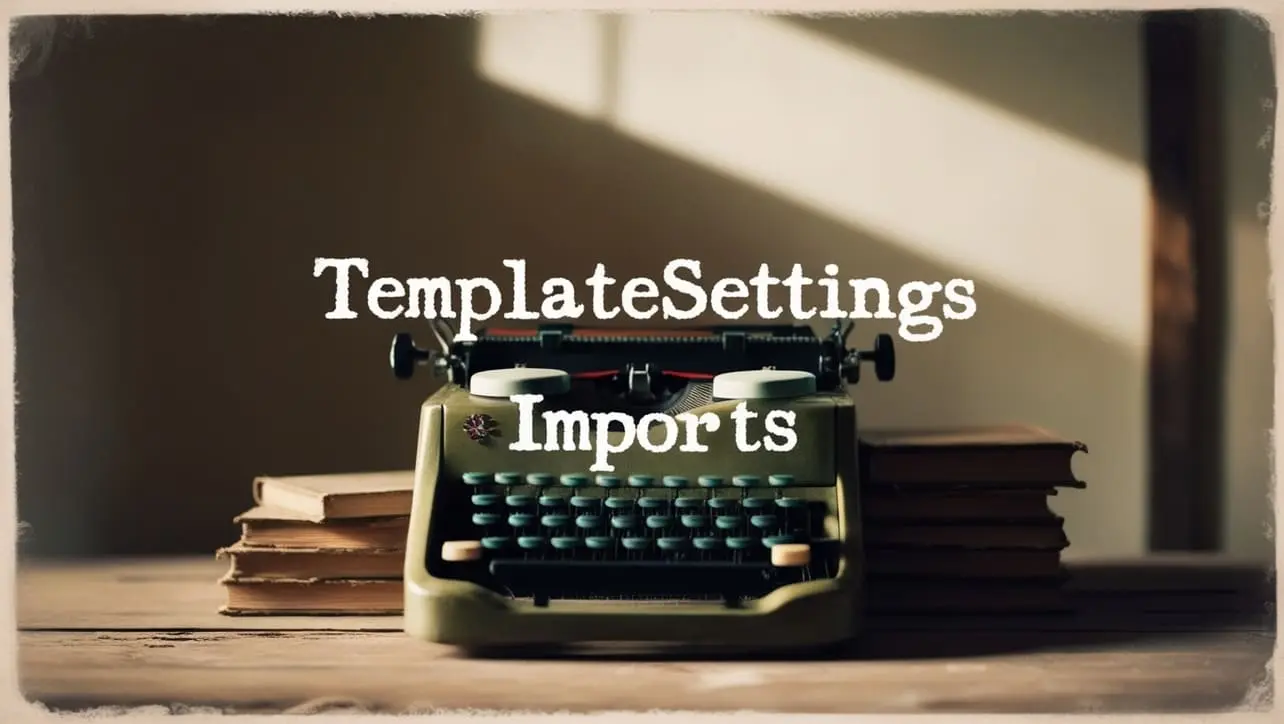
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isWeakSet() Lang Method
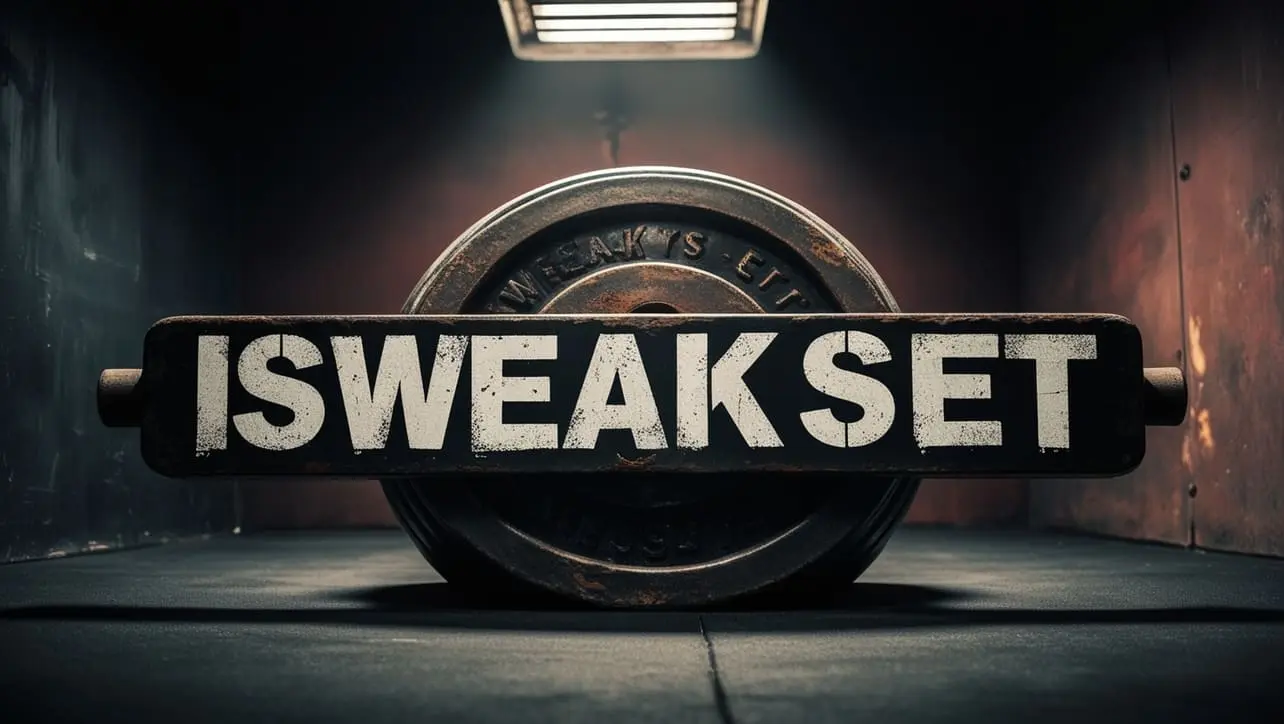
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, understanding and validating data types are essential for building robust and error-resistant applications. Lodash, a comprehensive utility library, provides a range of methods for handling various data types. One such method is _.isWeakSet()
, designed to check whether a value is a JavaScript WeakSet.
This method proves invaluable when working with complex data structures and ensuring the integrity of your code.
🧠 Understanding _.isWeakSet() Method
The _.isWeakSet()
method in Lodash is employed to determine if a given value is a JavaScript WeakSet or not. WeakSets are collections of objects where object references are held weakly, allowing for more efficient memory management. This method simplifies the process of validating whether a value adheres to the WeakSet data structure.
💡 Syntax
The syntax for the _.isWeakSet()
method is straightforward:
_.isWeakSet(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isWeakSet()
method:
const _ = require('lodash');
const weakSetExample = new WeakSet();
const isWeakSet = _.isWeakSet(weakSetExample);
console.log(isWeakSet);
// Output: true
In this example, a new WeakSet is created and then validated using _.isWeakSet()
.
🏆 Best Practices
When working with the _.isWeakSet()
method, consider the following best practices:
Type Validation:
Always perform type validation before using a WeakSet. Utilize
_.isWeakSet()
to ensure that the provided value is indeed a WeakSet.example.jsCopiedconst potentialWeakSet = /* ...get a value from somewhere... */ ; if (_.isWeakSet(potentialWeakSet)) { // Proceed with operations on the WeakSet console.log('Valid WeakSet!'); } else { console.error('Invalid WeakSet!'); }
Guard Clauses:
In functions or methods where WeakSets are expected, employ guard clauses with
_.isWeakSet()
to handle invalid inputs gracefully.example.jsCopiedfunction processWeakSet(inputWeakSet) { if (!_.isWeakSet(inputWeakSet)) { console.error('Invalid input. Expected a WeakSet.'); return; } // Continue with processing the WeakSet console.log('Processing WeakSet:', inputWeakSet); }
📚 Use Cases
Memory-Efficient Storage:
Utilize WeakSets when memory-efficient storage of object references is required. Use
_.isWeakSet()
to verify that the provided value is indeed a WeakSet.example.jsCopiedconst userSet = new WeakSet(); const adminSet = new WeakSet(); function grantAdminPrivileges(user) { if (_.isWeakSet(userSet) && _.isWeakSet(adminSet)) { adminSet.add(user); } else { console.error('Invalid WeakSets. Unable to grant admin privileges.'); } }
Garbage Collection Checks:
When working with WeakSets and implementing custom garbage collection mechanisms, employ
_.isWeakSet()
to ensure that you're dealing with WeakSets appropriately.example.jsCopiedfunction cleanUpWeakSet(dataWeakSet) { if (_.isWeakSet(dataWeakSet)) { // Implement custom garbage collection logic console.log('Cleaning up WeakSet:', dataWeakSet); dataWeakSet.clear(); } else { console.error('Invalid WeakSet. Unable to perform cleanup.'); } }
🎉 Conclusion
The _.isWeakSet()
method in Lodash serves as a reliable tool for validating whether a given value conforms to the JavaScript WeakSet data structure. By incorporating this method into your code, you can enhance the robustness of your applications, especially when dealing with memory-efficient storage and custom garbage collection strategies.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isWeakSet()
method in your Lodash projects.
👨💻 Join our Community:
Author
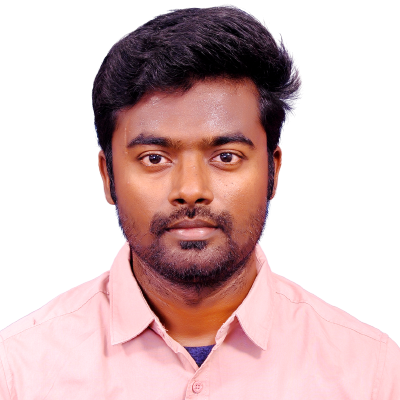
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
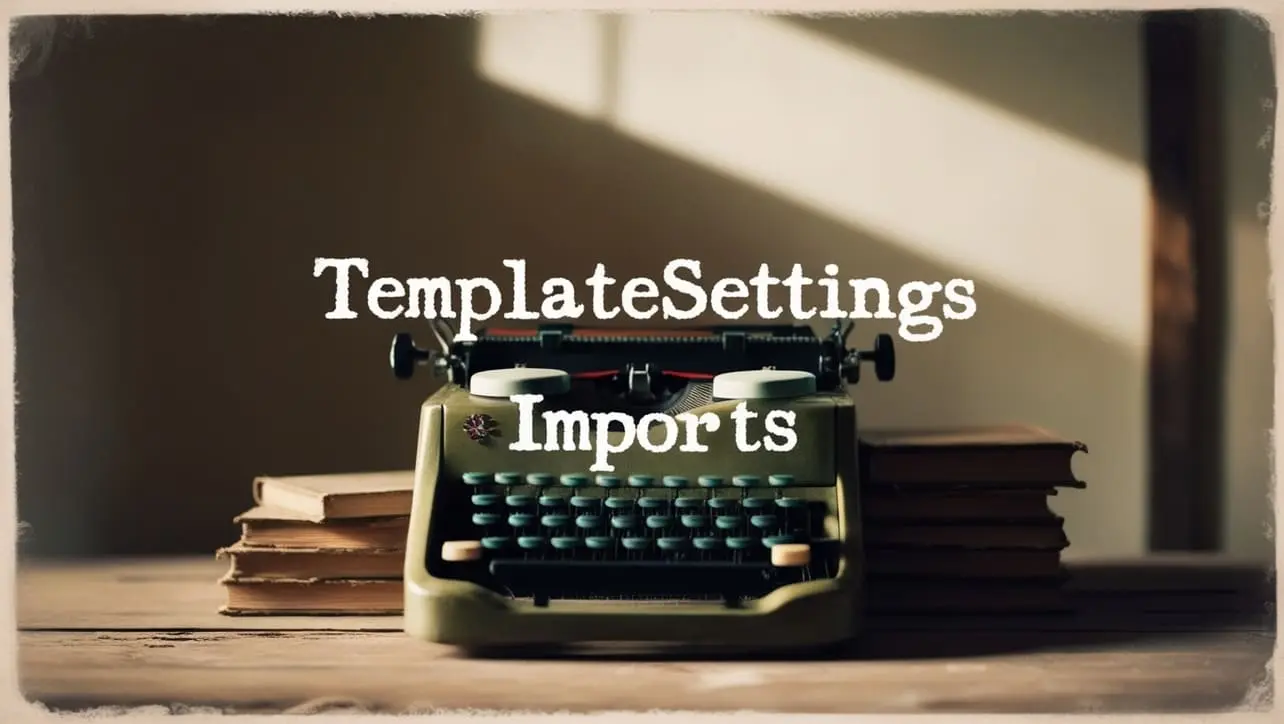
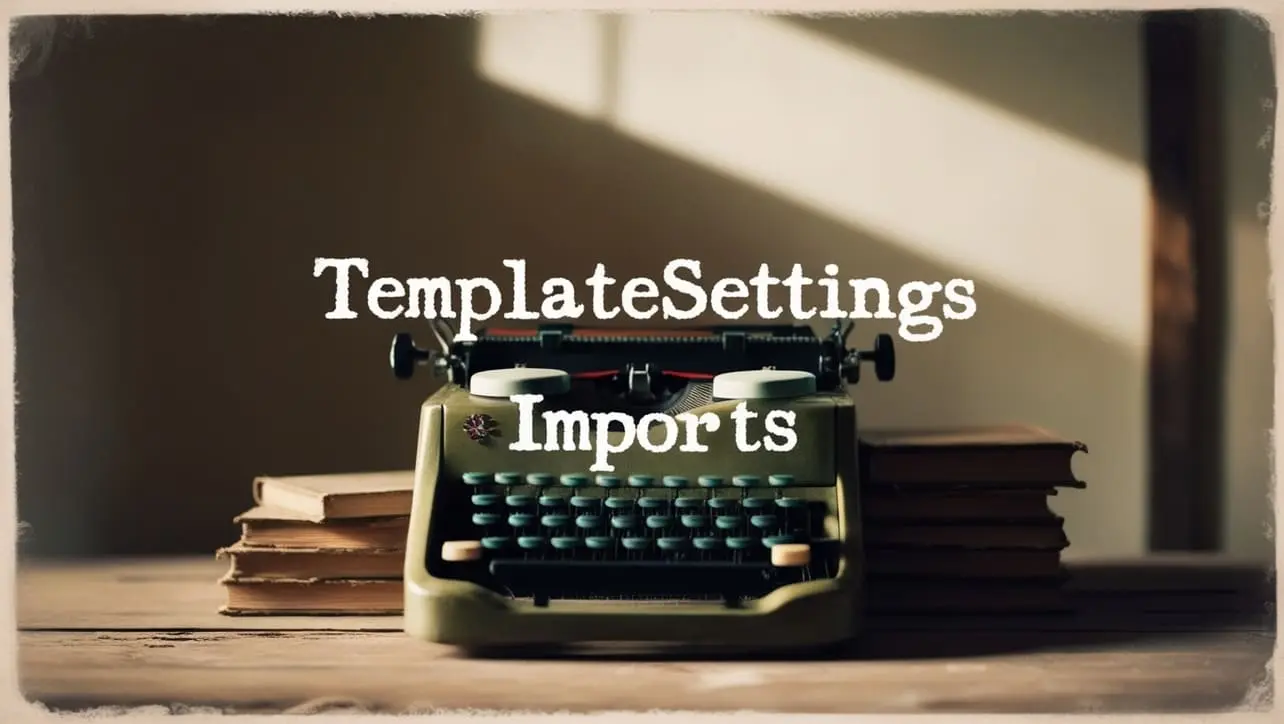
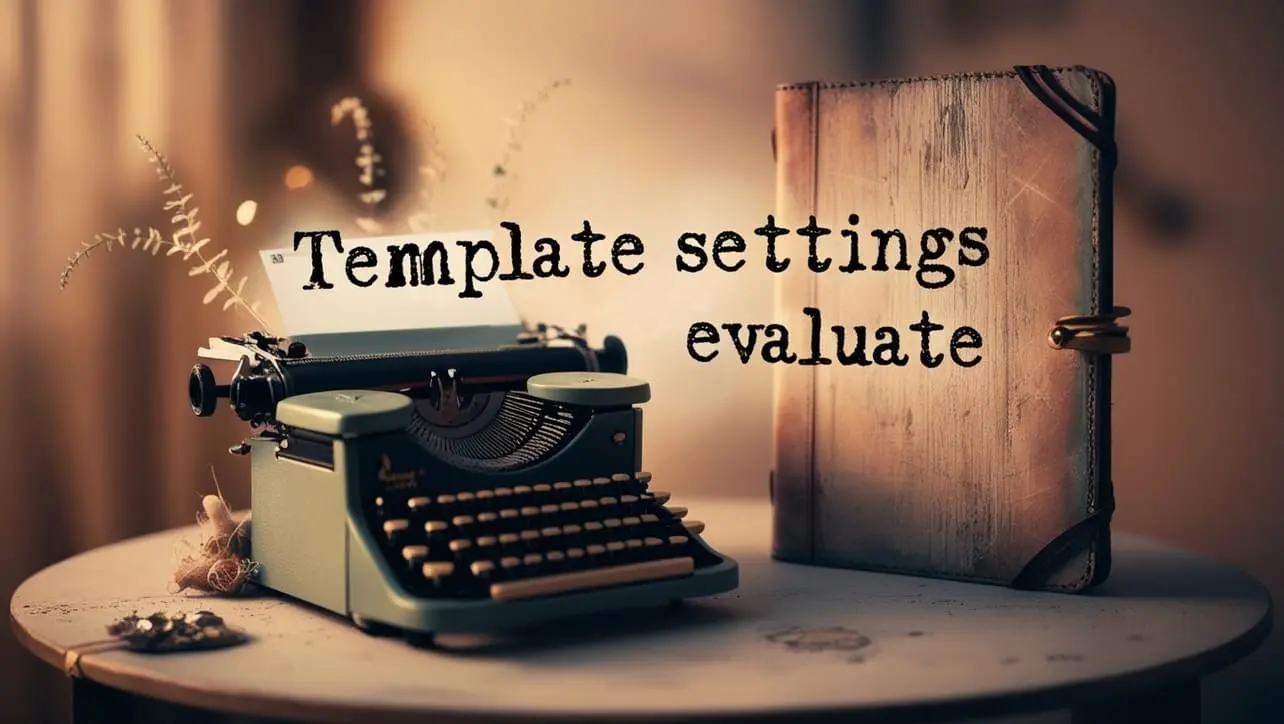
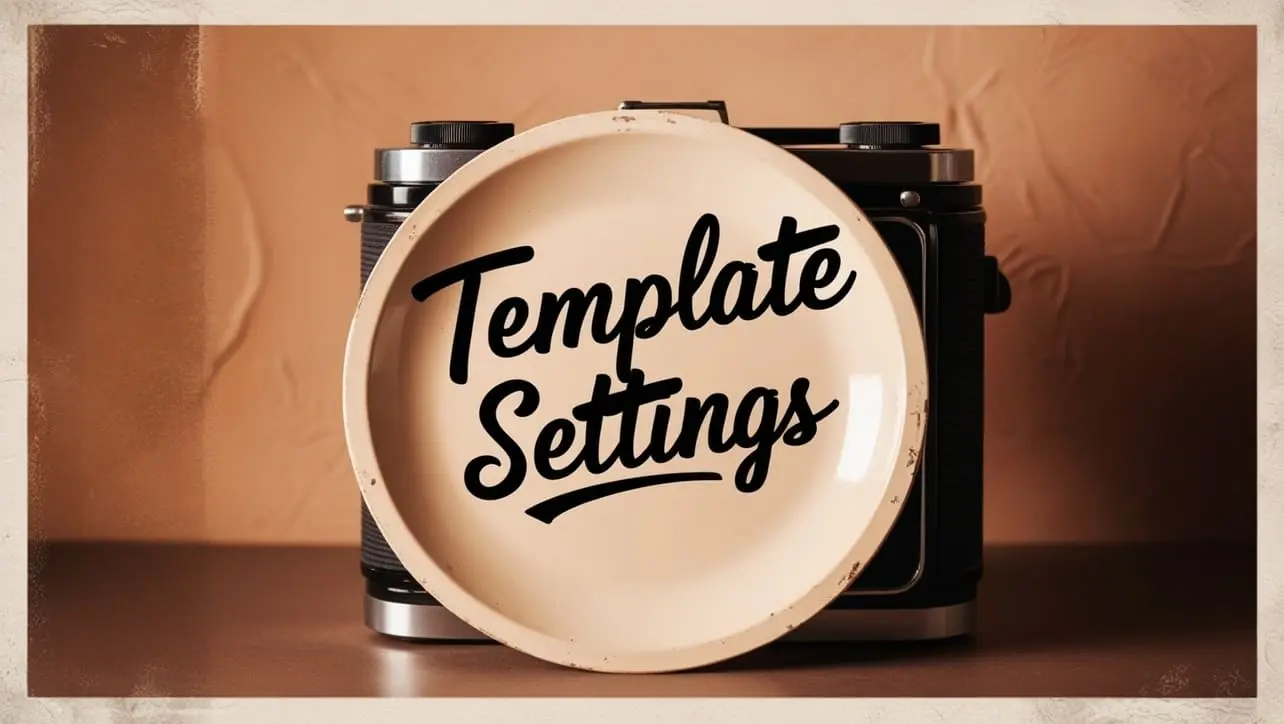
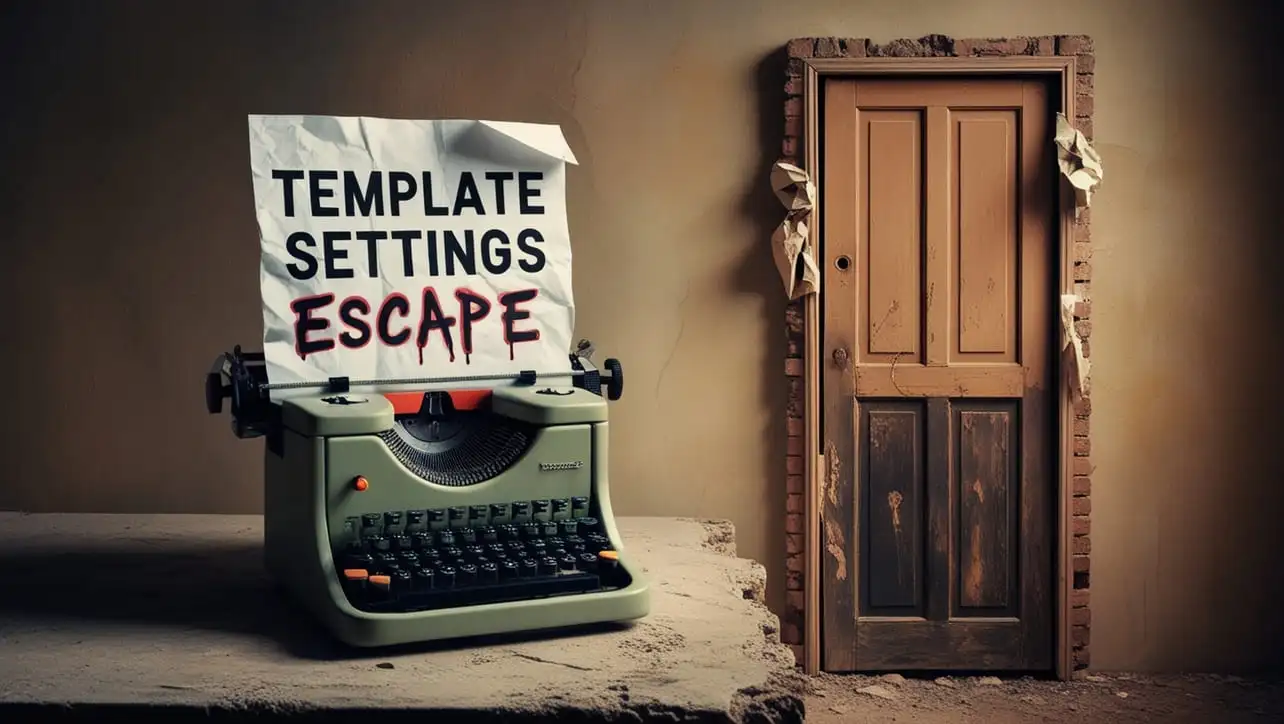
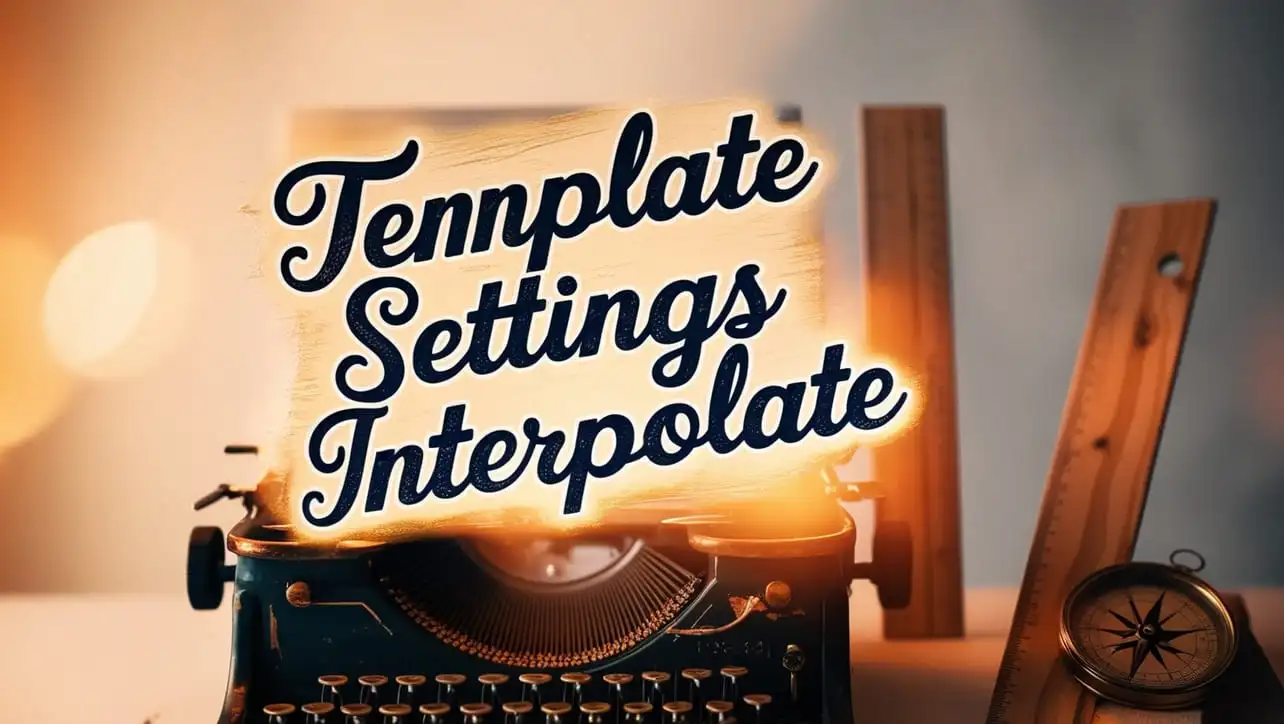
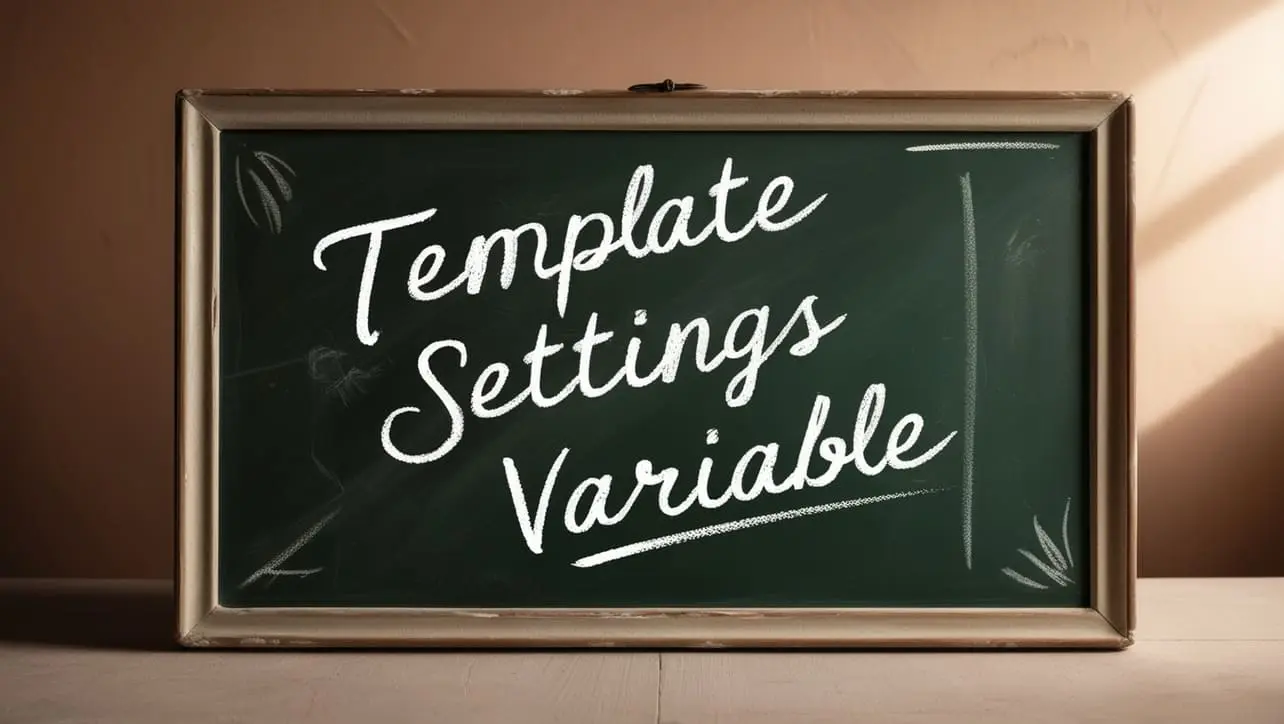
If you have any doubts regarding this article (Lodash _.isWeakSet() Lang Method), please comment here. I will help you immediately.