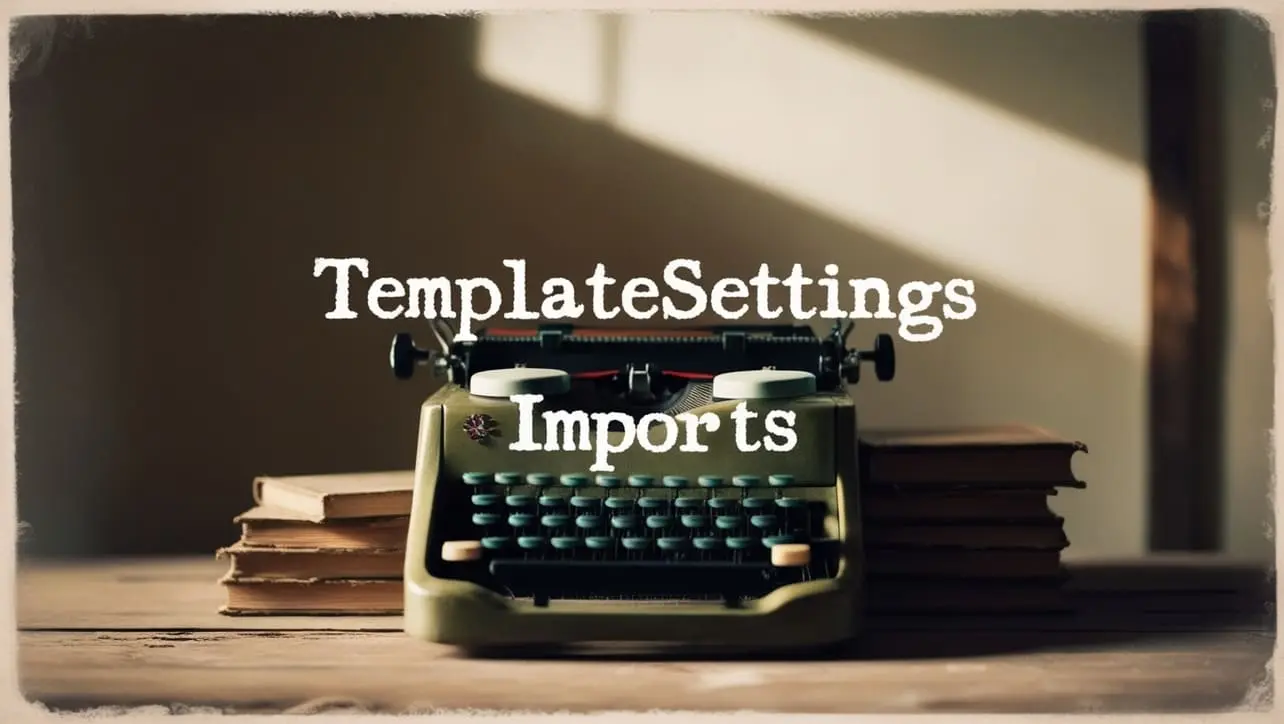
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isUndefined() Lang Method
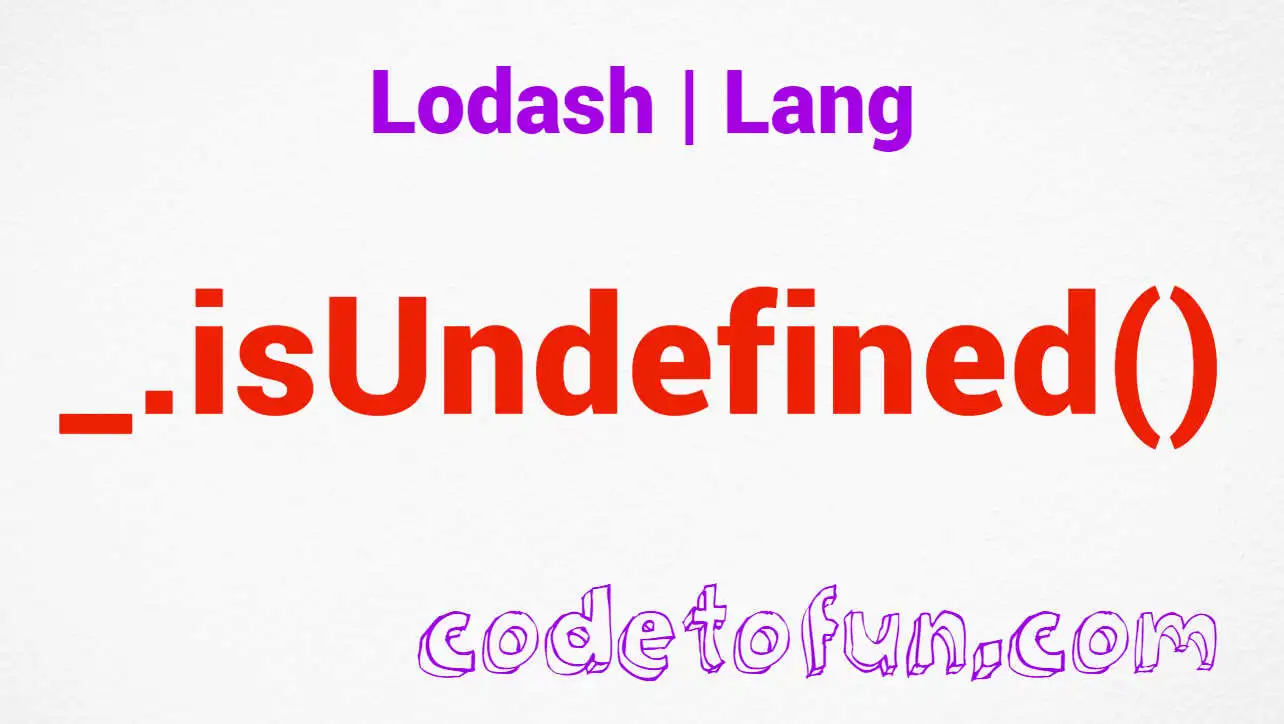
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, effective handling of variable states is essential. Lodash, a powerful utility library, provides a collection of functions to streamline common programming tasks. Among these functions is the _.isUndefined()
method, designed to simplify the process of checking whether a value is undefined.
This method is invaluable for developers seeking a robust and consistent way to manage undefined values in their applications.
🧠 Understanding _.isUndefined() Method
The _.isUndefined()
method in Lodash is a straightforward utility for determining whether a given value is undefined. It offers a concise and reliable approach to handling undefined variables, promoting code clarity and reducing the likelihood of bugs related to undefined values.
💡 Syntax
The syntax for the _.isUndefined()
method is straightforward:
_.isUndefined(value)
- value: The value to check for undefined.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isUndefined()
method:
const _ = require('lodash');
const undefinedValue = undefined;
const definedValue = 'Hello, Lodash!';
console.log(_.isUndefined(undefinedValue));
// Output: true
console.log(_.isUndefined(definedValue));
// Output: false
In this example, the _.isUndefined()
method accurately identifies whether a variable contains the value undefined.
🏆 Best Practices
When working with the _.isUndefined()
method, consider the following best practices:
Consistent Type Checking:
Maintain consistency in type checking across your codebase. Use
_.isUndefined()
when specifically checking for undefined values, and consider other Lodash methods for different types of checks.example.jsCopiedconst variable = /* ...some variable... */ ; if (_.isUndefined(variable)) { // Handle undefined case } else if (_.isString(variable)) { // Handle string case } else if (_.isArray(variable)) { // Handle array case } else { // Handle other cases }
Default Values:
When dealing with potentially undefined variables, use
_.isUndefined()
to set default values and avoid unexpected behavior.example.jsCopiedfunction processValue(value) { const safeValue = _.isUndefined(value) ? 'Default Value' : value; // Continue processing with safeValue }
Avoid Direct Comparison:
Prefer using
_.isUndefined()
over direct comparisons (=== undefined) for improved readability and consistency in your code.example.jsCopiedconst variable = /* ...some variable... */ ; if (_.isUndefined(variable)) { // Handle undefined case } else { // Handle defined case }
📚 Use Cases
Input Validation:
In scenarios where you expect certain parameters to be provided, use
_.isUndefined()
to validate and handle potential missing values.example.jsCopiedfunction processData(options) { if (_.isUndefined(options.data)) { console.error('Missing data parameter'); return; } // Continue processing with options.data }
Object Property Existence:
When working with objects and checking for the existence of a specific property,
_.isUndefined()
can provide a clear and consistent approach.example.jsCopiedconst user = { name: 'John', age: 30 }; if (_.isUndefined(user.address)) { console.log('User has no address information'); }
Error Handling:
In error-handling scenarios, use
_.isUndefined()
to gracefully handle cases where expected values may be missing.example.jsCopiedfunction handleApiResponse(response) { if (_.isUndefined(response.data)) { console.error('Unexpected API response format'); return; } // Continue processing with response.data }
🎉 Conclusion
The _.isUndefined()
method in Lodash is a valuable tool for JavaScript developers seeking a consistent and reliable way to check for undefined values. Whether you're validating input parameters, setting default values, or handling error scenarios, this method provides a clear and concise solution.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isUndefined()
method in your Lodash projects.
👨💻 Join our Community:
Author
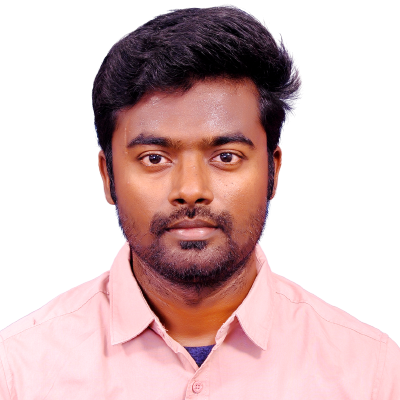
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
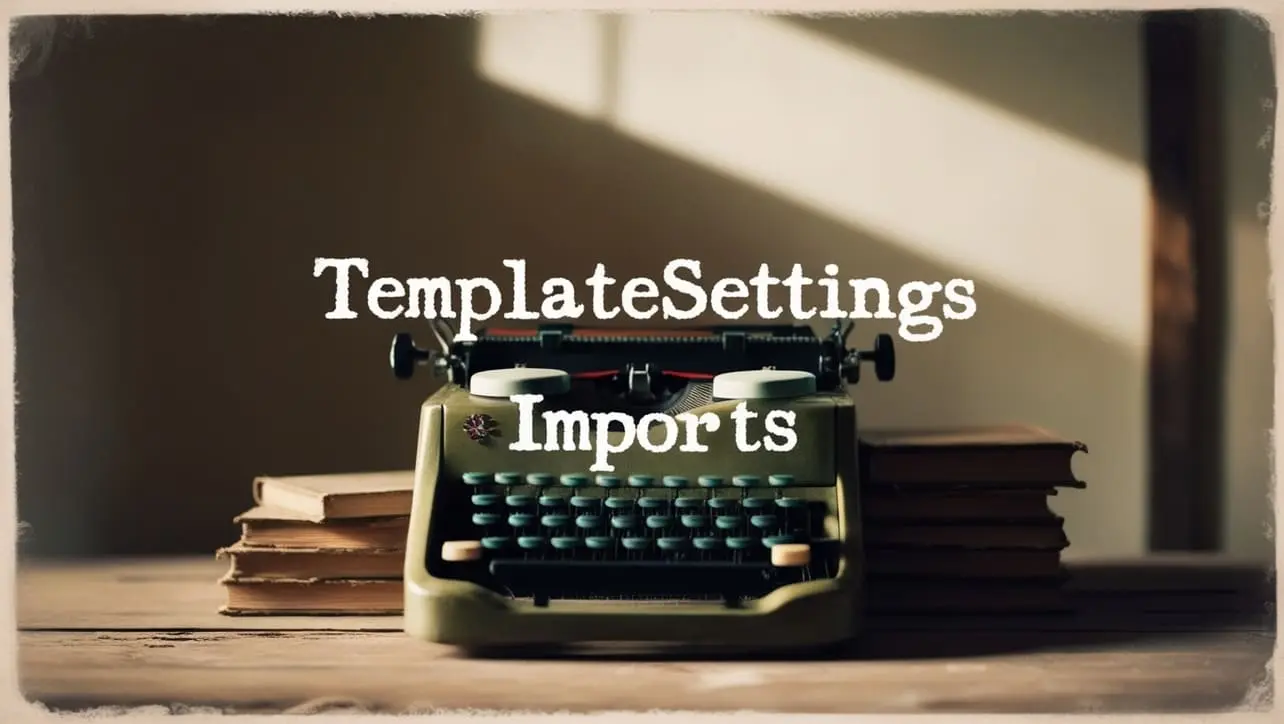
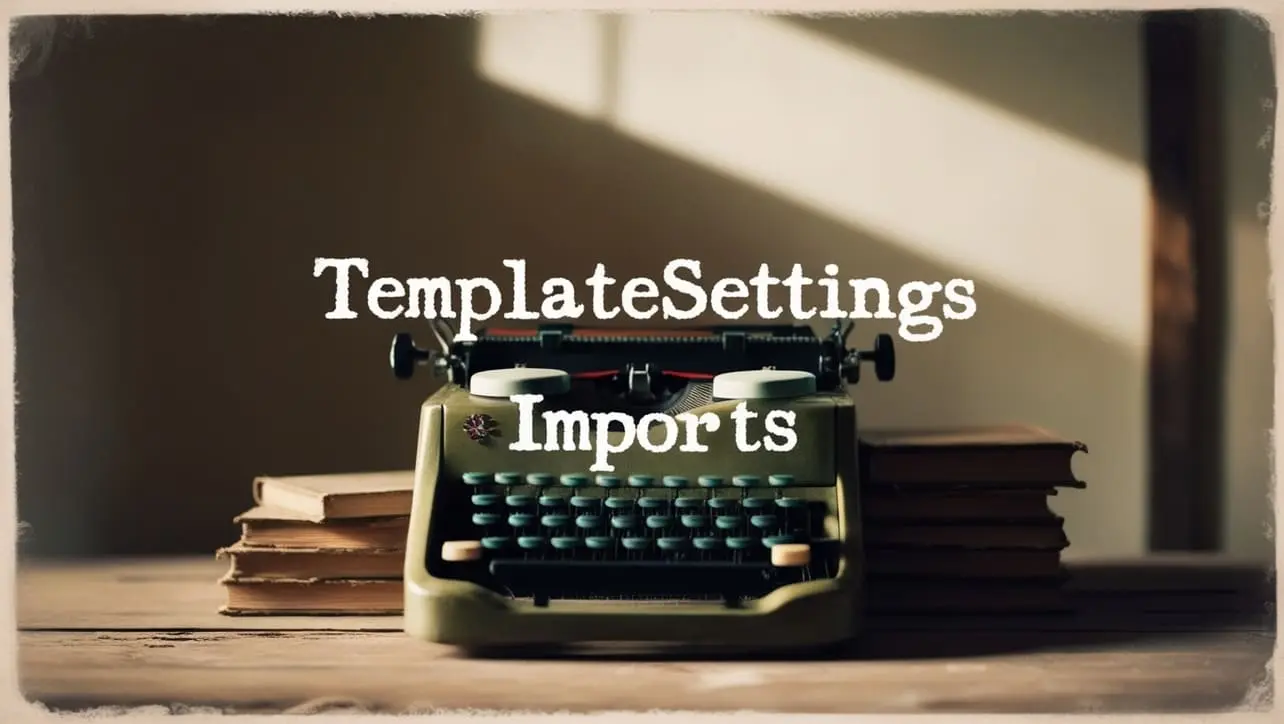
Lodash _.templateSettings.imports Property
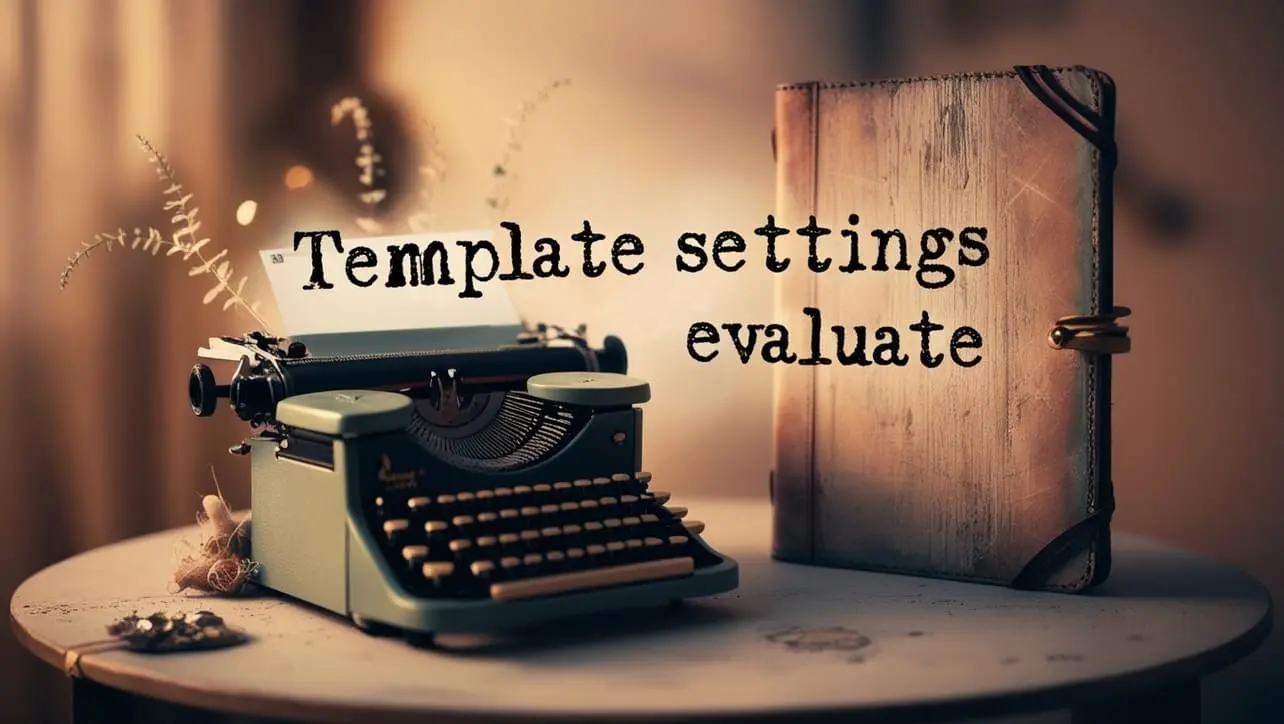
Lodash _.templateSettings.evaluate Property
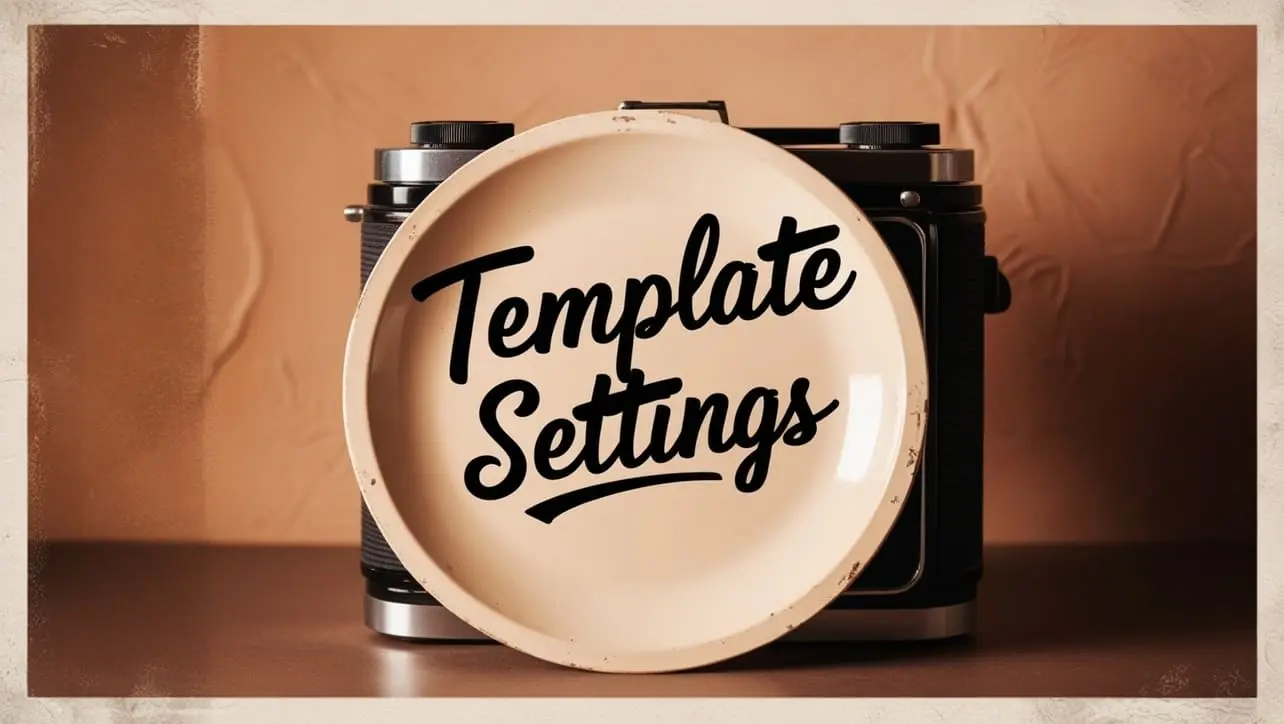
Lodash _.templateSettings Property
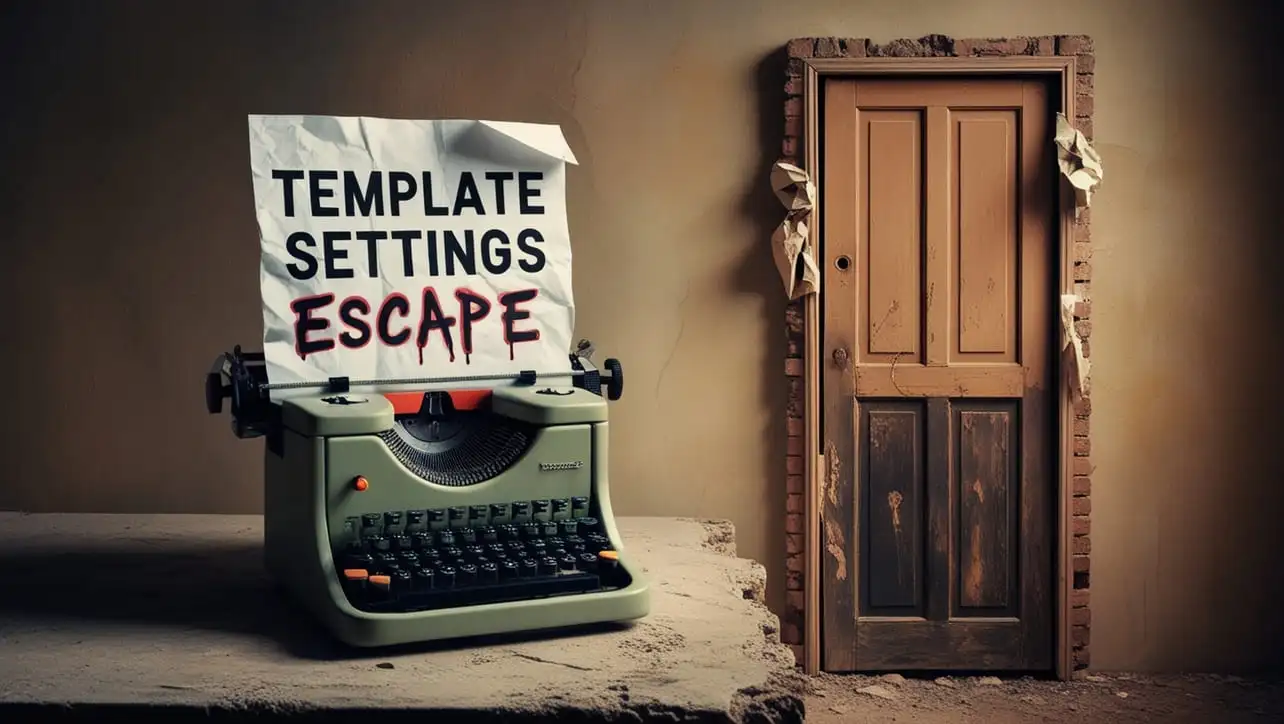
Lodash _.templateSettings.escape Property
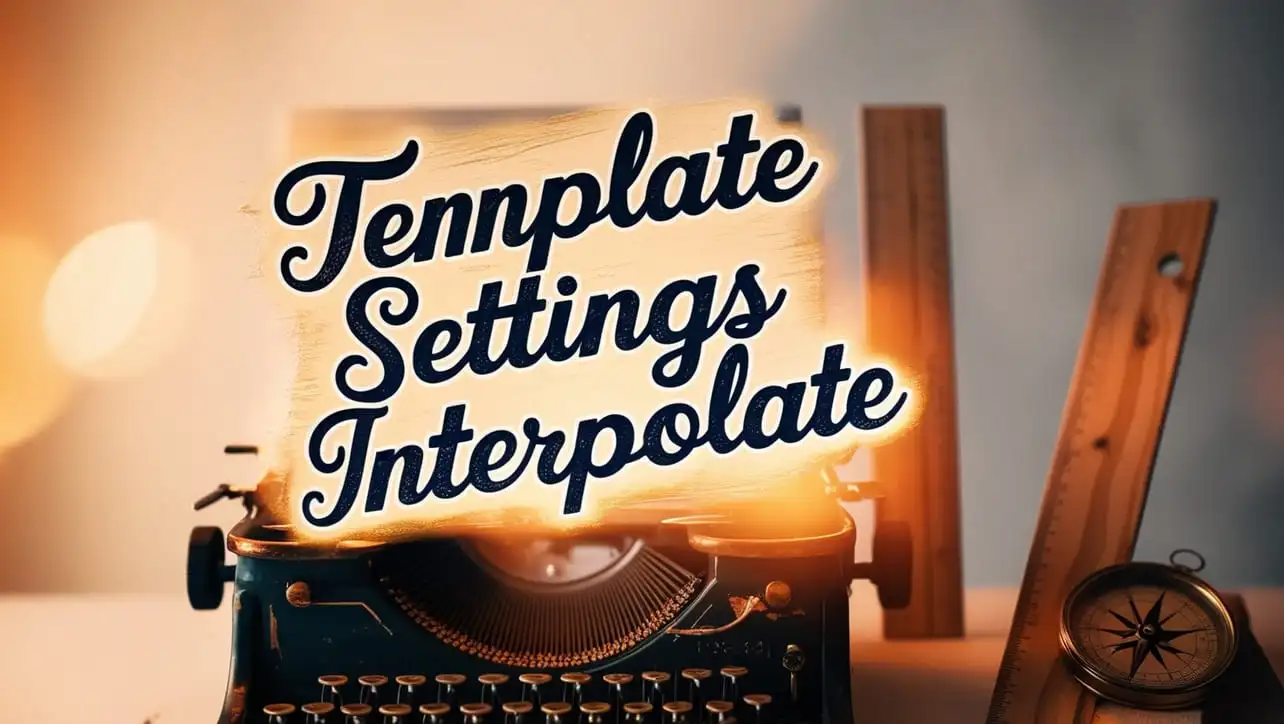
Lodash _.templateSettings.interpolate Property
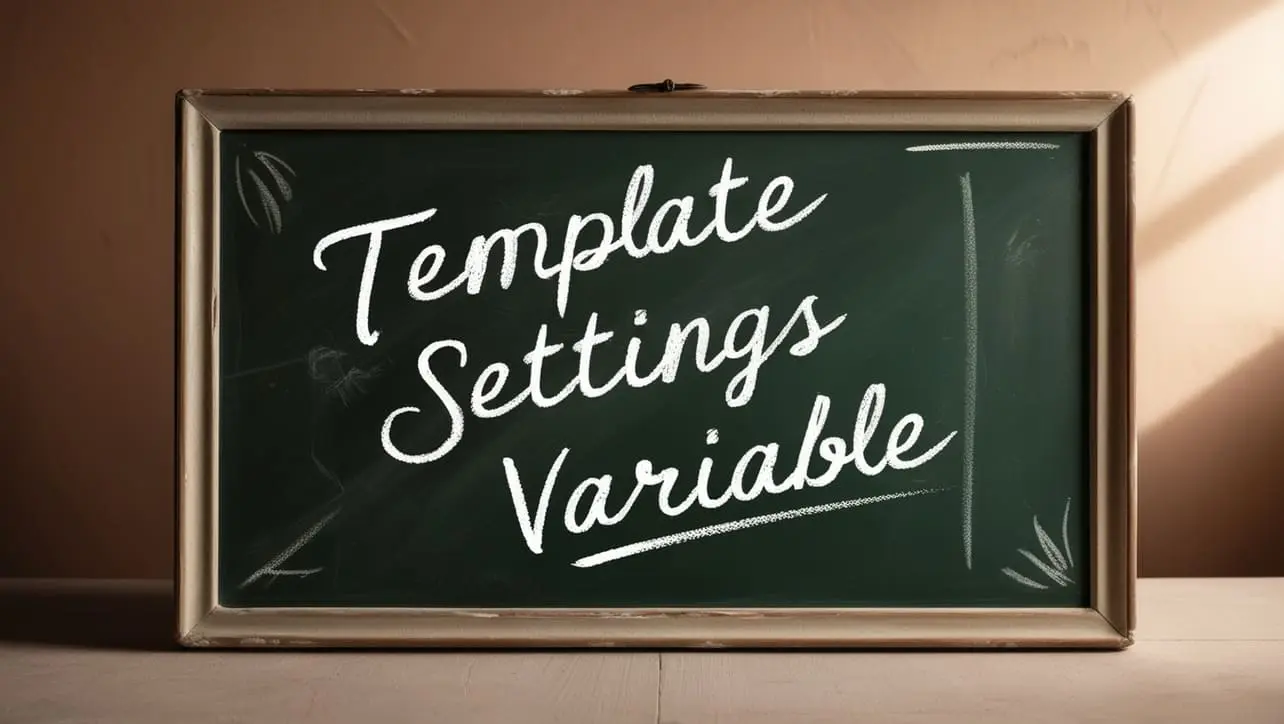
If you have any doubts regarding this article (Lodash _.isUndefined() Lang Method), please comment here. I will help you immediately.