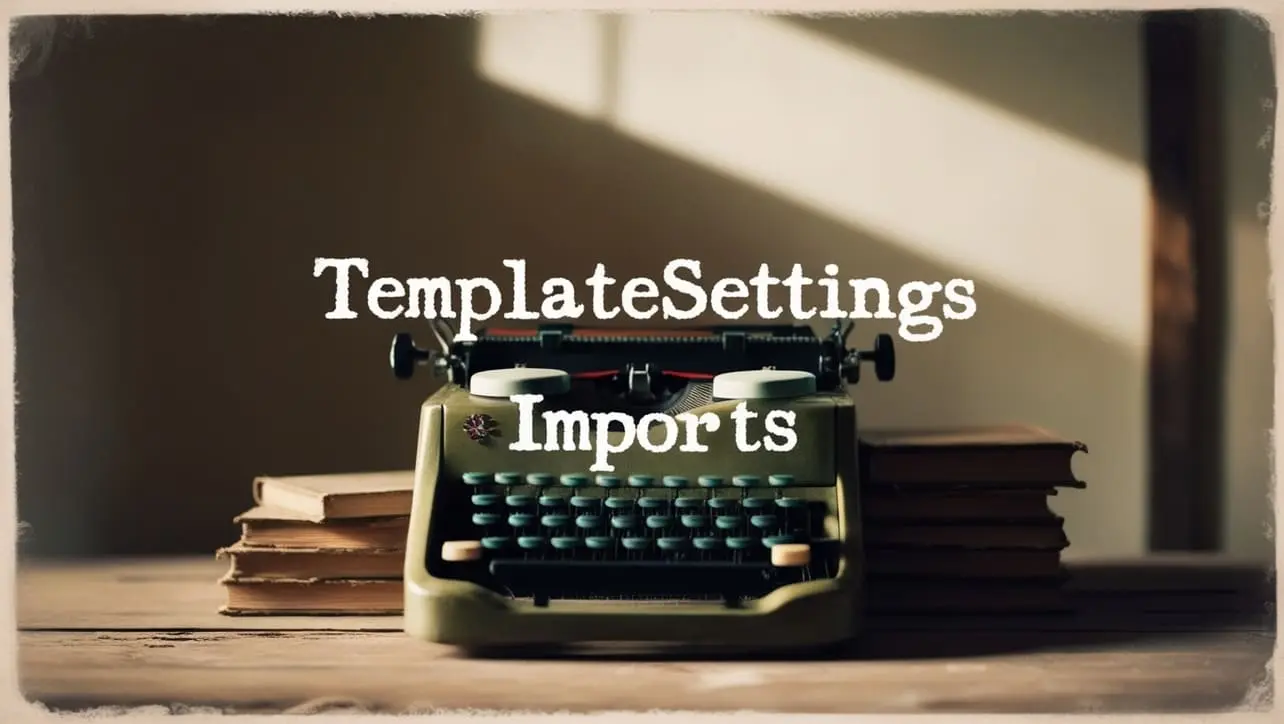
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isRegExp() Lang Method
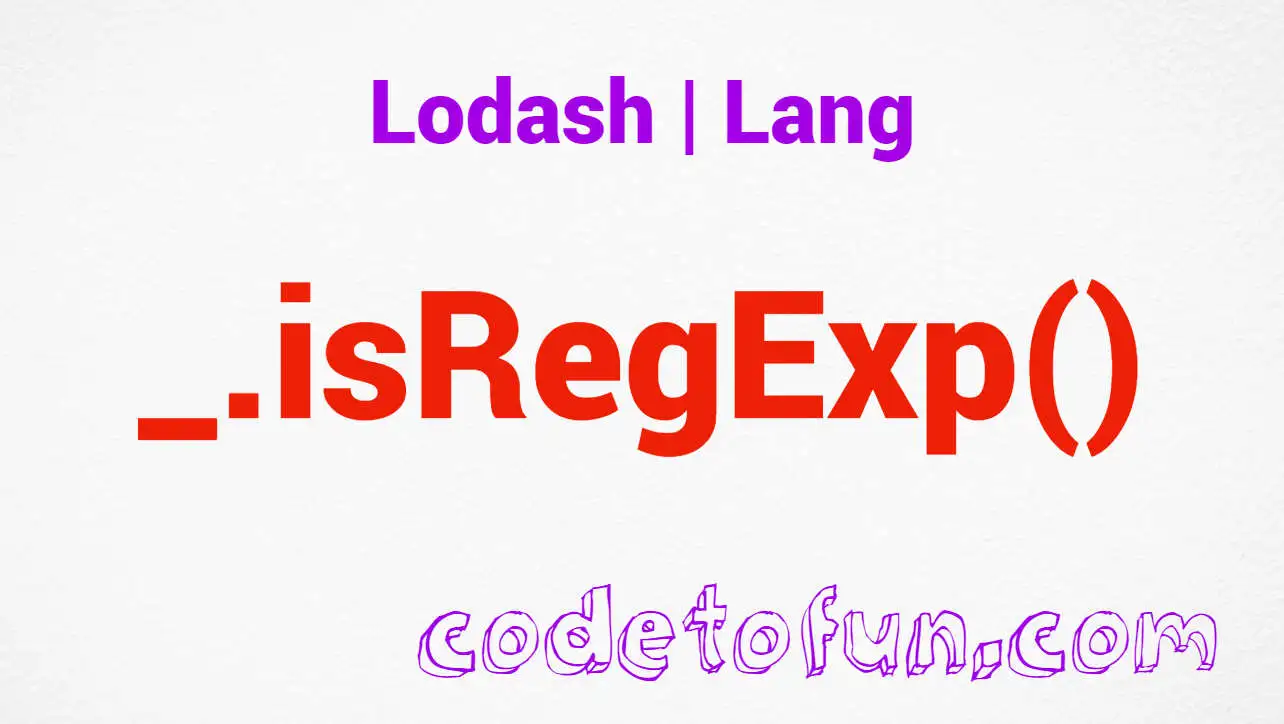
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript, data types play a crucial role in ensuring the accuracy and reliability of code. Lodash, a powerful utility library, provides developers with a variety of functions to handle common programming challenges.
One such function is _.isRegExp()
, a Lang method designed to check whether a value is a regular expression object. Understanding and utilizing this method can enhance the robustness and clarity of your JavaScript code.
🧠 Understanding _.isRegExp() Method
The _.isRegExp()
method in Lodash is a straightforward yet invaluable tool for type checking. It determines whether a given value is a regular expression object or not, helping developers avoid potential issues related to incorrect data types.
💡 Syntax
The syntax for the _.isRegExp()
method is straightforward:
_.isRegExp(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isRegExp()
method:
const _ = require('lodash');
const regExpObject = /pattern/;
const isRegExp = _.isRegExp(regExpObject);
console.log(isRegExp);
// Output: true
In this example, the regExpObject is a regular expression, and _.isRegExp()
confirms its type by returning true.
🏆 Best Practices
When working with the _.isRegExp()
method, consider the following best practices:
Type Checking:
Use
_.isRegExp()
for precise type checking when dealing with dynamic values that may vary in type. This ensures that your code expects and handles regular expressions appropriately.example.jsCopiedfunction processInput(value) { if (_.isRegExp(value)) { // Handle regular expression logic console.log('Processing regular expression:', value); } else { // Handle other data types console.log('Processing non-regular expression data:', value); } } const regExpInput = /example/; const stringInput = 'not a regular expression'; processInput(regExpInput); processInput(stringInput);
Guard Clauses:
In scenarios where a function or module expects a regular expression, implement guard clauses using
_.isRegExp()
to ensure the correct type is provided.example.jsCopiedfunction validateRegularExpression(input) { if (!_.isRegExp(input)) { throw new Error('Invalid regular expression provided'); } // Continue with regular expression validation logic console.log('Validating regular expression:', input); } const validRegExp = /valid/; const invalidInput = 'not a regular expression'; validateRegularExpression(validRegExp); // Throws an error for invalidInput // Output: Error: Invalid regular expression provided
Safeguarding Regular Expression Usage:
Before using a value as a regular expression, verify its type with
_.isRegExp()
to prevent runtime errors.example.jsCopiedfunction processText(text, pattern) { if (_.isRegExp(pattern)) { // Safely use the regular expression const matches = text.match(pattern); console.log('Matches found:', matches); } else { console.error('Invalid regular expression provided'); } } const inputText = 'Sample text'; const validPattern = /sample/; const invalidPattern = 'not a regular expression'; processText(inputText, validPattern); processText(inputText, invalidPattern);
📚 Use Cases
Validating User Input:
When dealing with user-provided values that are expected to be regular expressions, use
_.isRegExp()
to validate and handle the input appropriately.example.jsCopiedfunction processUserInput(userInput) { if (_.isRegExp(userInput)) { // Process user input as a regular expression console.log('User input is a regular expression:', userInput); } else { console.error('Invalid regular expression provided by the user'); } } const userRegExpInput = new RegExp('dynamic-pattern'); const invalidUserInput = 'not a regular expression'; processUserInput(userRegExpInput); processUserInput(invalidUserInput);
Conditional Logic:
Implement conditional logic based on whether a value is a regular expression or not using
_.isRegExp()
.example.jsCopiedfunction processDynamicValue(value) { if (_.isRegExp(value)) { console.log('Processing as a regular expression'); // Additional logic for regular expressions } else { console.log('Processing as a non-regular expression'); // Additional logic for other data types } } const dynamicValue1 = /dynamic/; const dynamicValue2 = 42; processDynamicValue(dynamicValue1); processDynamicValue(dynamicValue2);
🎉 Conclusion
The _.isRegExp()
method in Lodash provides a reliable means of checking whether a value is a regular expression, offering flexibility and clarity in your JavaScript code. By incorporating this Lang method into your projects, you can enhance type safety and improve the overall robustness of your applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isRegExp()
method in your Lodash projects.
👨💻 Join our Community:
Author
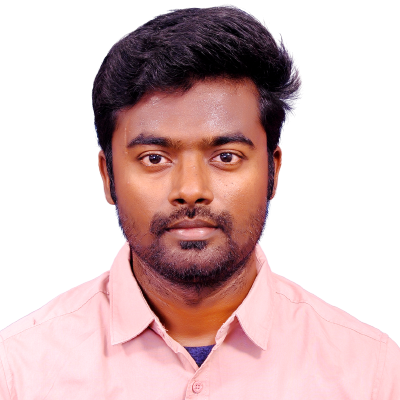
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
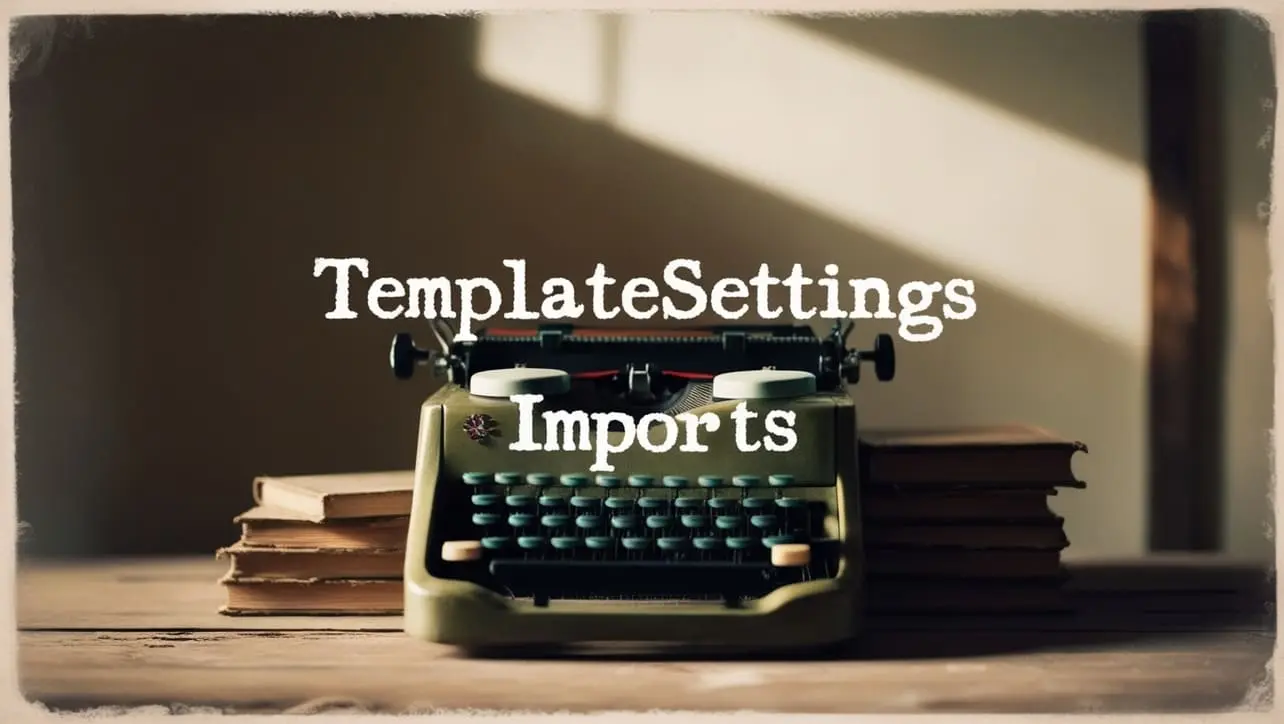
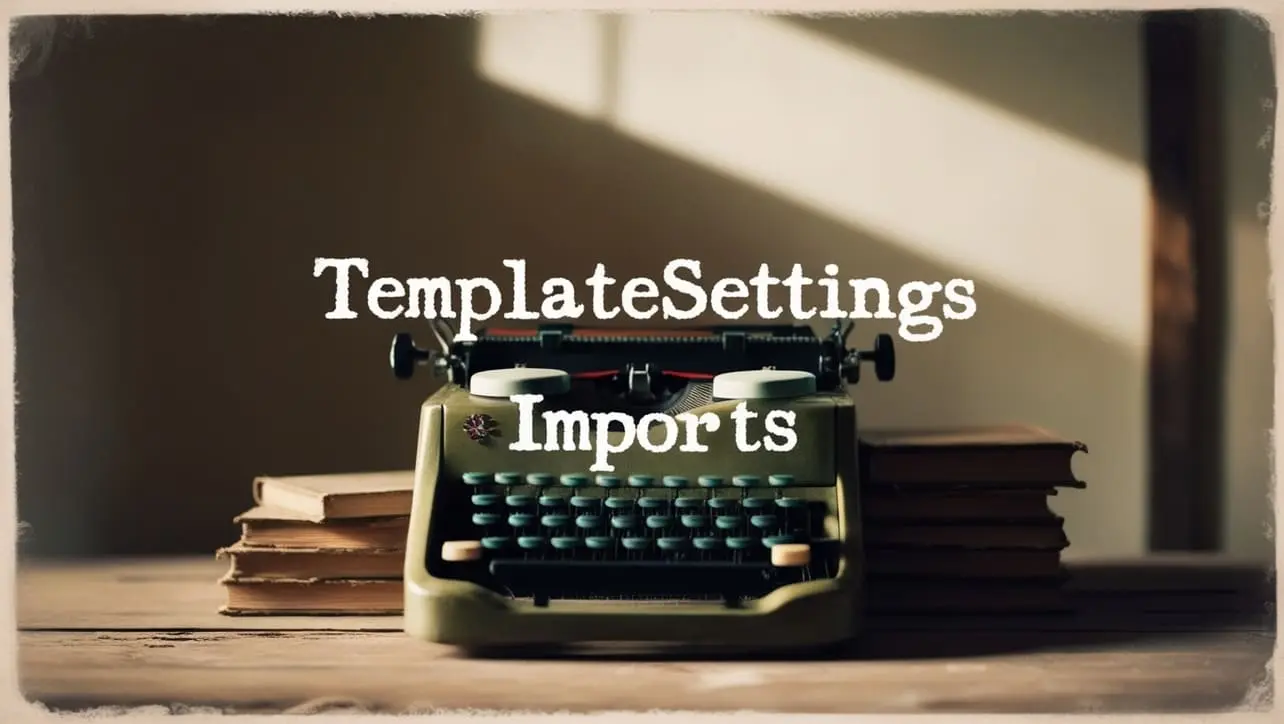
Lodash _.templateSettings.imports Property
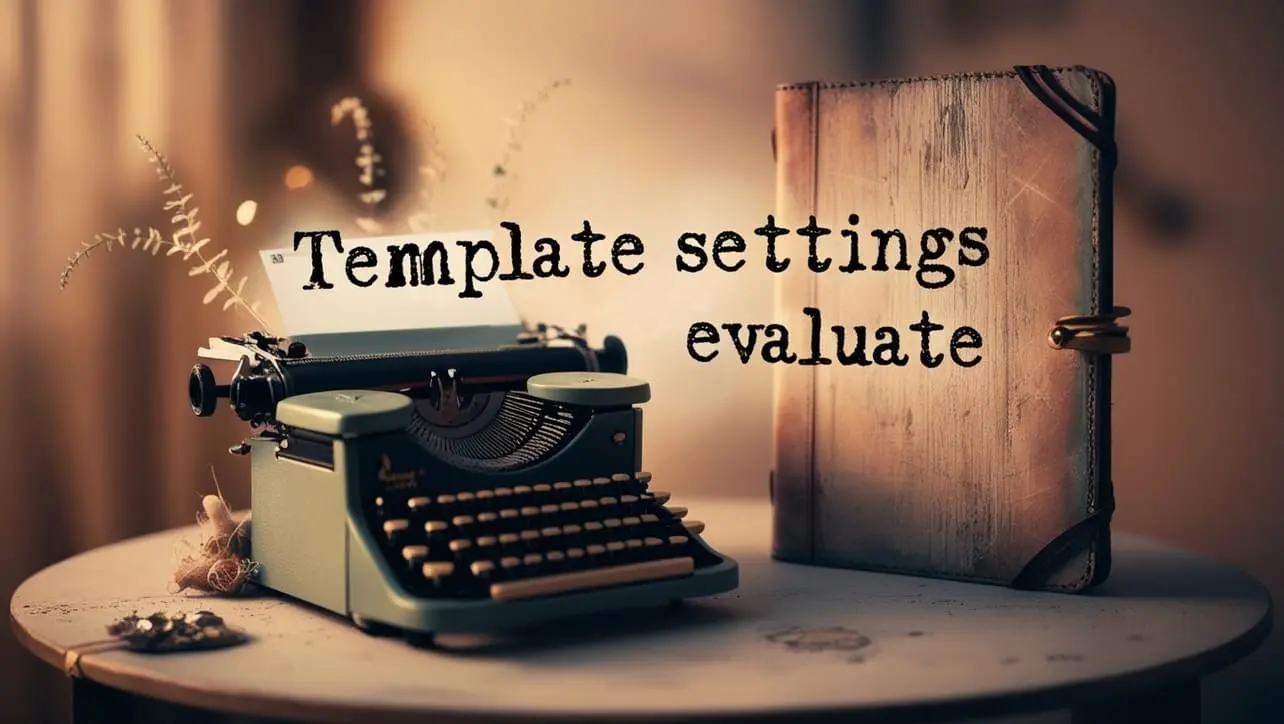
Lodash _.templateSettings.evaluate Property
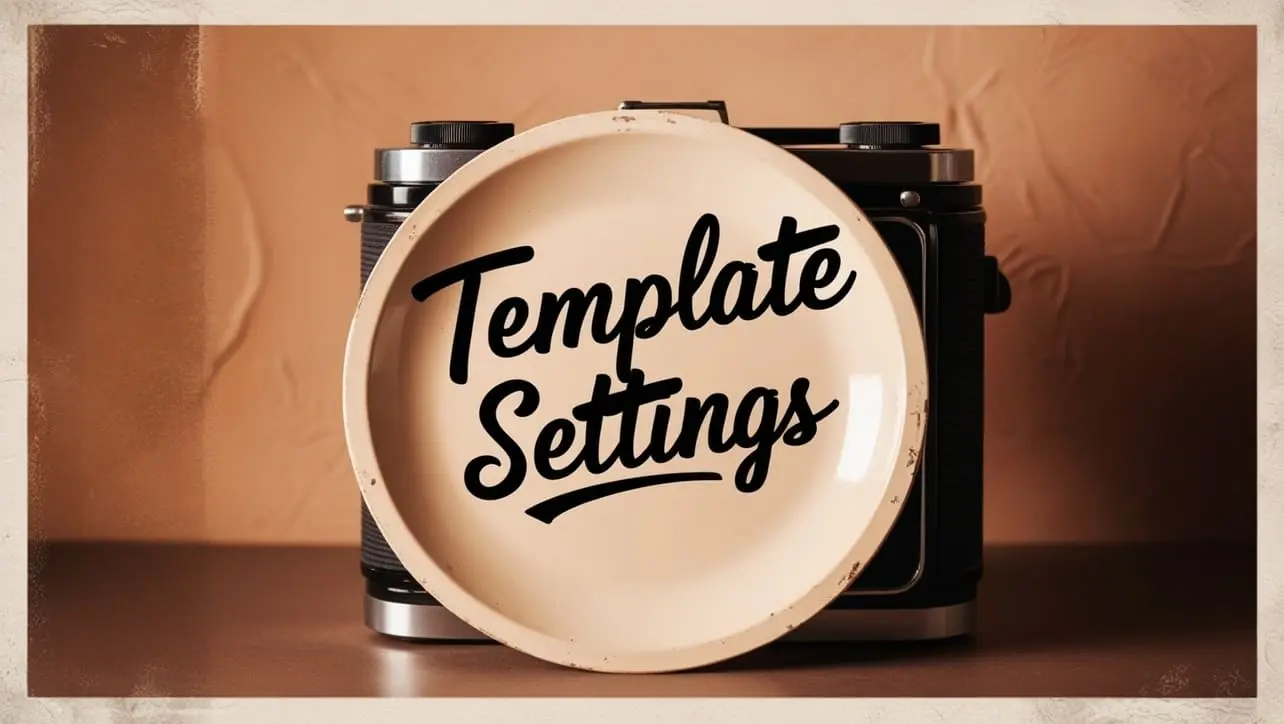
Lodash _.templateSettings Property
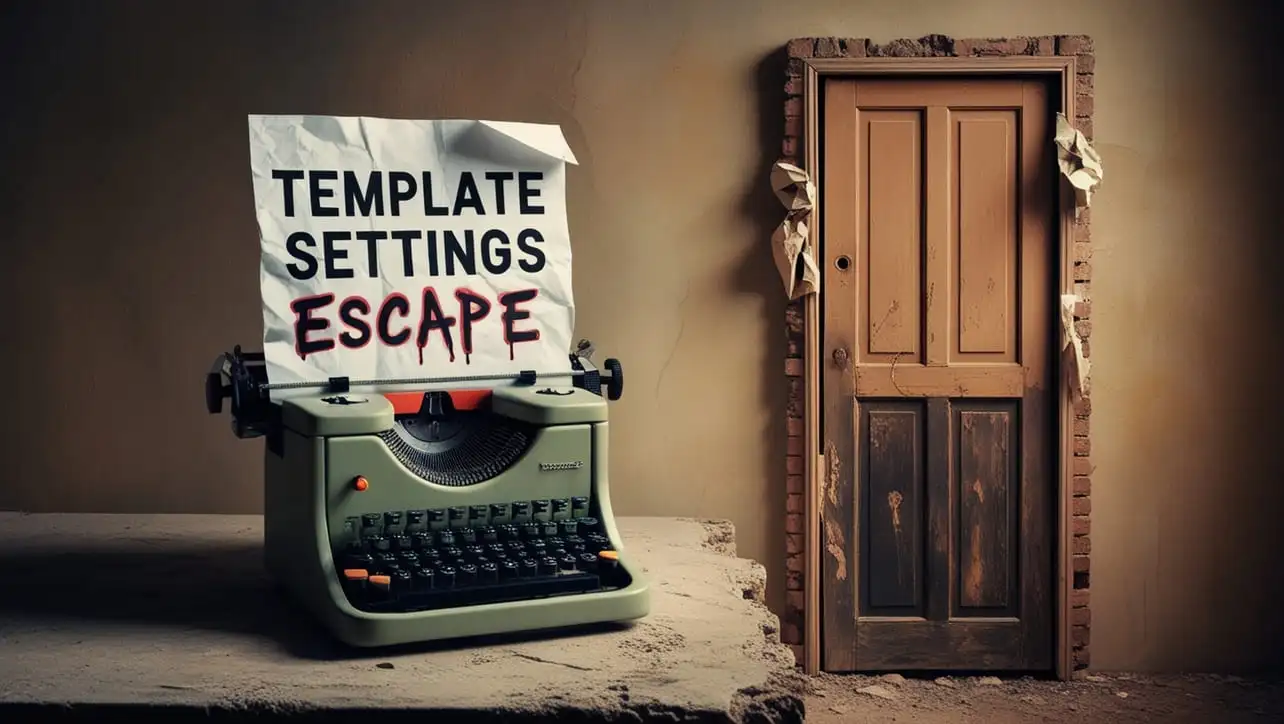
Lodash _.templateSettings.escape Property
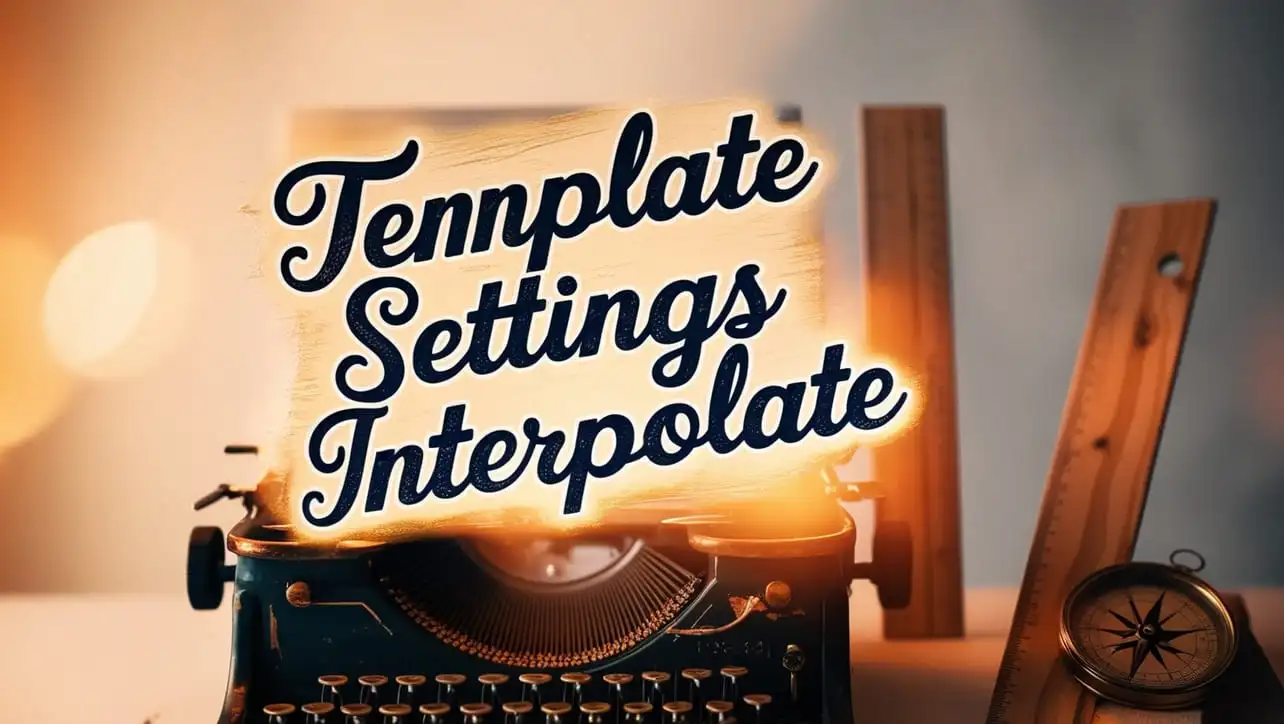
Lodash _.templateSettings.interpolate Property
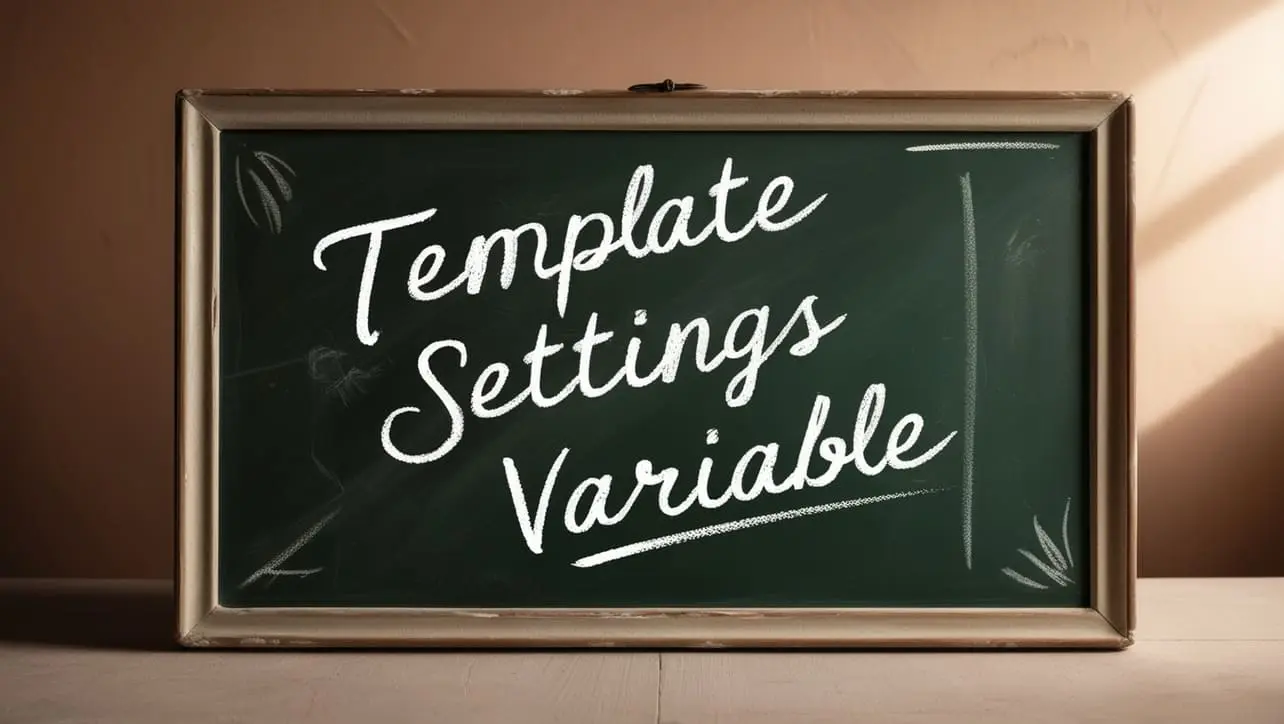
If you have any doubts regarding this article (Lodash _.isRegExp() Lang Method), please comment here. I will help you immediately.