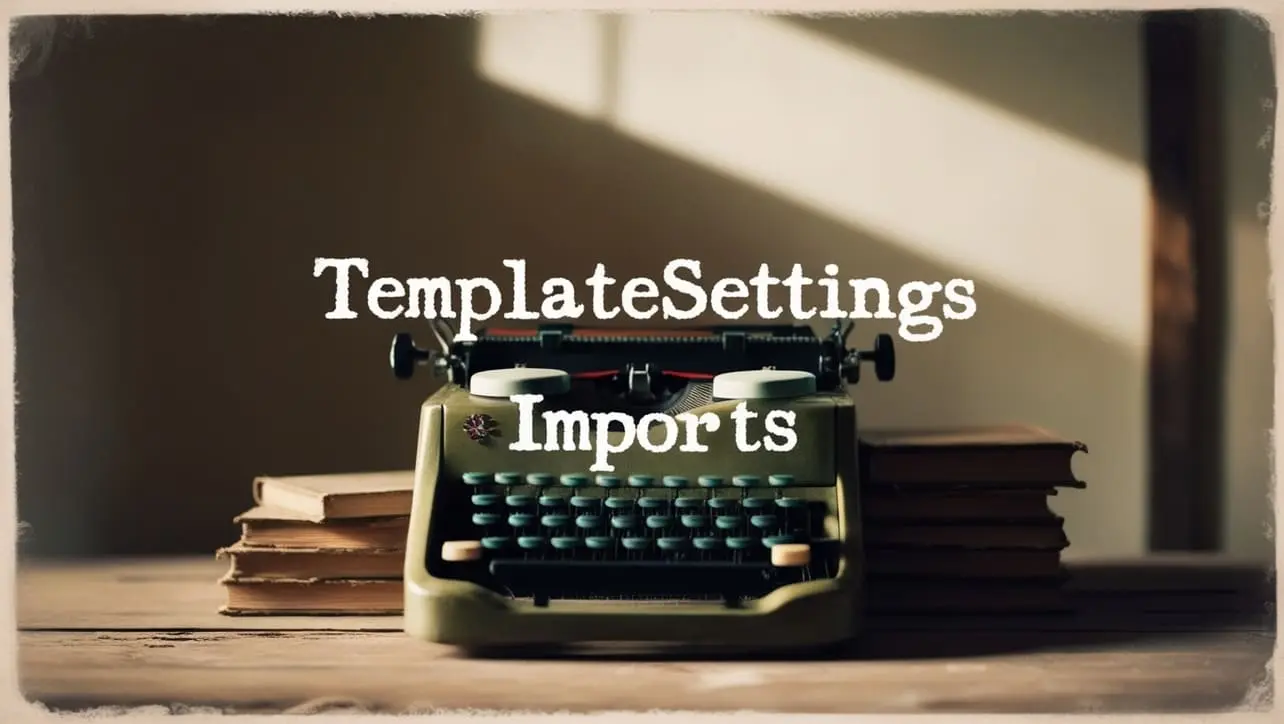
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isObjectLike() Lang Method
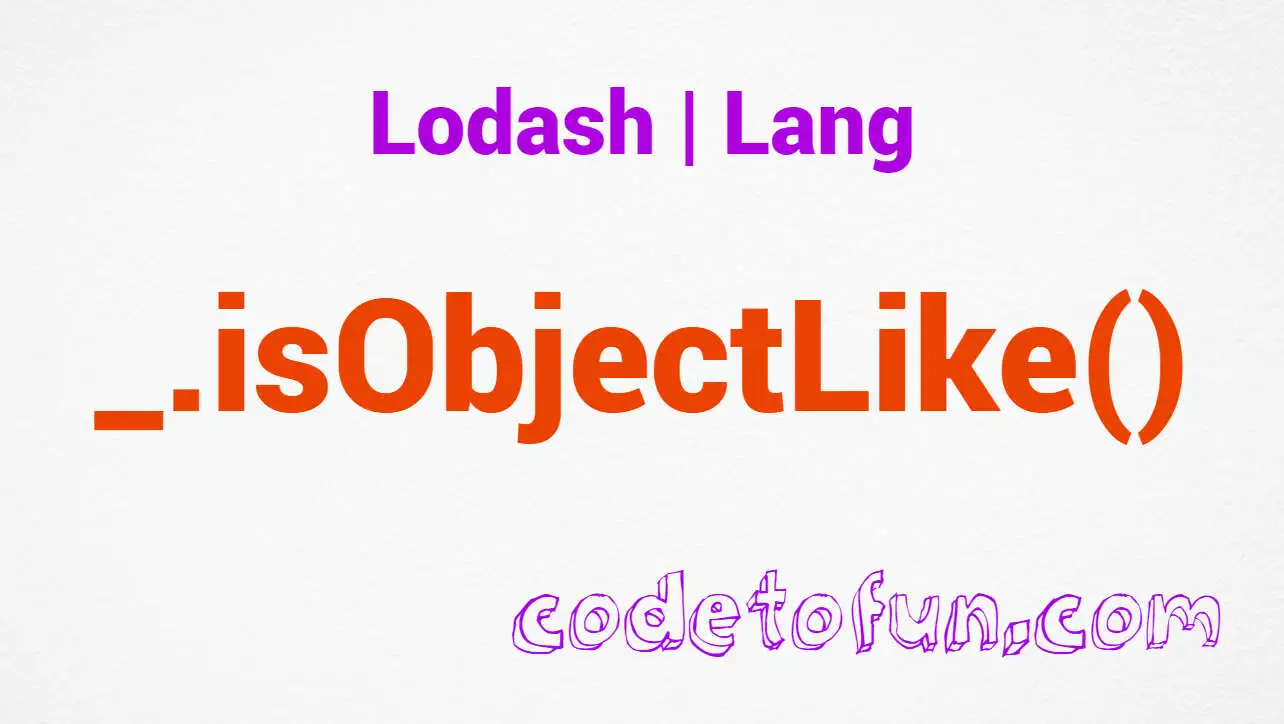
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript programming, effective type checking is crucial for robust and error-free code. Lodash, a powerful utility library, provides developers with a multitude of functions to simplify common programming tasks. One such function is _.isObjectLike()
, a valuable tool for checking if a value is object-like.
This method facilitates more precise and reliable type checking in JavaScript applications.
🧠 Understanding _.isObjectLike() Method
The _.isObjectLike()
method in Lodash is designed to determine if a given value is object-like. It considers values that are not null and have a typeof result of "object" but excludes functions.
💡 Syntax
The syntax for the _.isObjectLike()
method is straightforward:
_.isObjectLike(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isObjectLike()
method:
const _ = require('lodash');
const objectValue = { key: 'value' };
const arrayValue = [1, 2, 3];
const nonObjectValue = 'not an object';
console.log(_.isObjectLike(objectValue)); // Output: true
console.log(_.isObjectLike(arrayValue)); // Output: true
console.log(_.isObjectLike(nonObjectValue)); // Output: false
In this example, _.isObjectLike()
is used to check the object-like nature of different values, returning true for objects and arrays, and false for a non-object value.
🏆 Best Practices
When working with the _.isObjectLike()
method, consider the following best practices:
Robust Type Checking:
Use
_.isObjectLike()
for robust type checking, especially when dealing with values that could be objects or other types. It provides a more nuanced check compared to the generic typeof operator.example.jsCopiedfunction processData(data) { if (_.isObjectLike(data)) { // Process the data as an object console.log('Processing object-like data:', data); } else { console.error('Invalid data type. Expected an object-like value.'); } }
Complementary Checks:
Combine
_.isObjectLike()
with other type-checking methods to create comprehensive checks for different scenarios. This ensures that your code can handle a wide range of input types.example.jsCopiedfunction processInput(input) { if (_.isArray(input)) { console.log('Processing an array:', input); } else if (_.isObjectLike(input)) { console.log('Processing object-like data:', input); } else { console.error('Invalid input type. Expected an array or object-like value.'); } }
📚 Use Cases
Function Parameter Validation:
When creating functions that expect object-like parameters, use
_.isObjectLike()
to validate the input. This helps catch potential issues early and ensures that the function operates on the expected data structure.example.jsCopiedfunction processUserData(user) { if (_.isObjectLike(user)) { // Process user data console.log('Processing user data:', user); } else { console.error('Invalid user data. Expected an object-like value.'); } }
Configurations and Options:
In scenarios where configurations or options are passed as parameters, use
_.isObjectLike()
to ensure that the provided values are object-like before proceeding with further processing.example.jsCopiedfunction configureApp(options) { if (_.isObjectLike(options)) { // Configure the application with the provided options console.log('Configuring app with options:', options); } else { console.error('Invalid options. Expected an object-like value.'); } }
🎉 Conclusion
The _.isObjectLike()
method in Lodash is a valuable asset for JavaScript developers seeking reliable type-checking capabilities. Whether you're validating function parameters, handling configurations, or performing other checks, this method provides a nuanced approach to identifying object-like values.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isObjectLike()
method in your Lodash projects.
👨💻 Join our Community:
Author
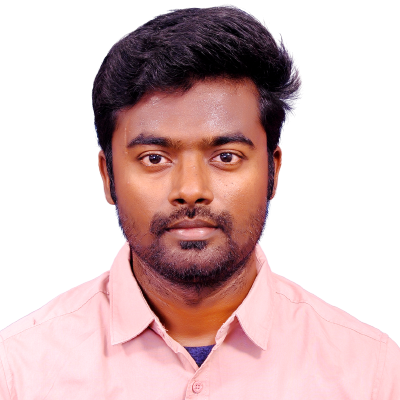
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
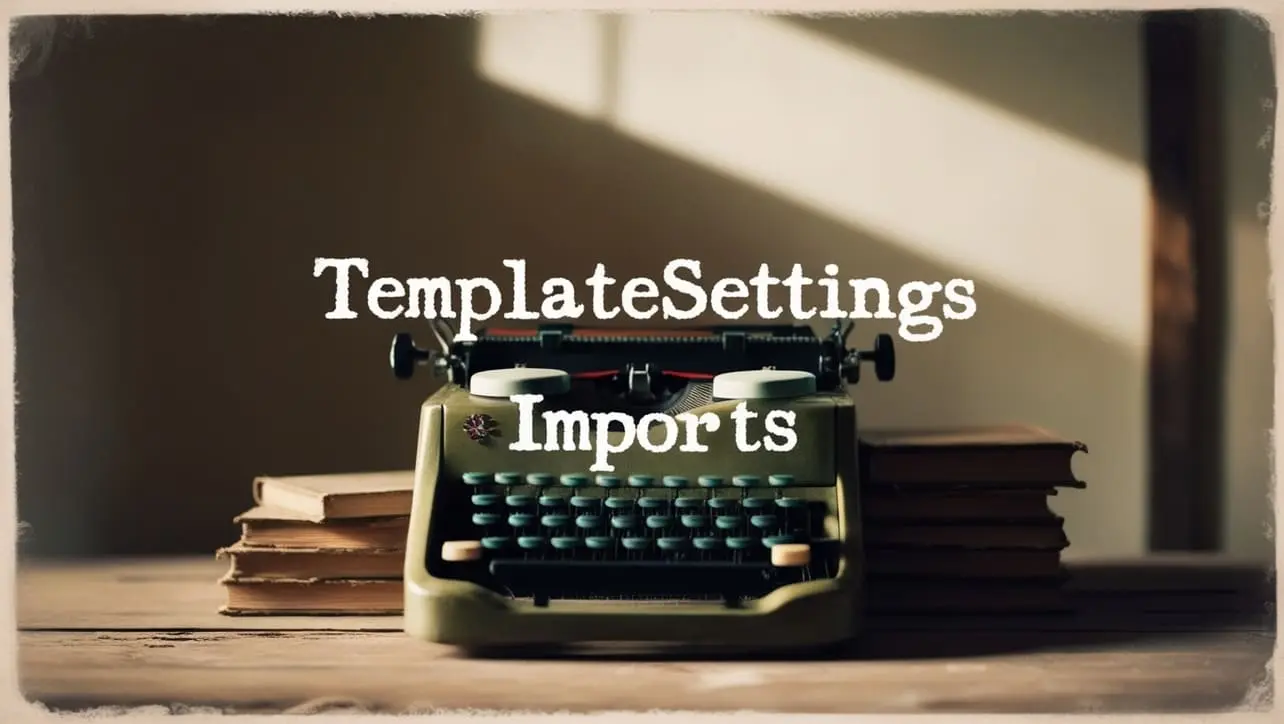
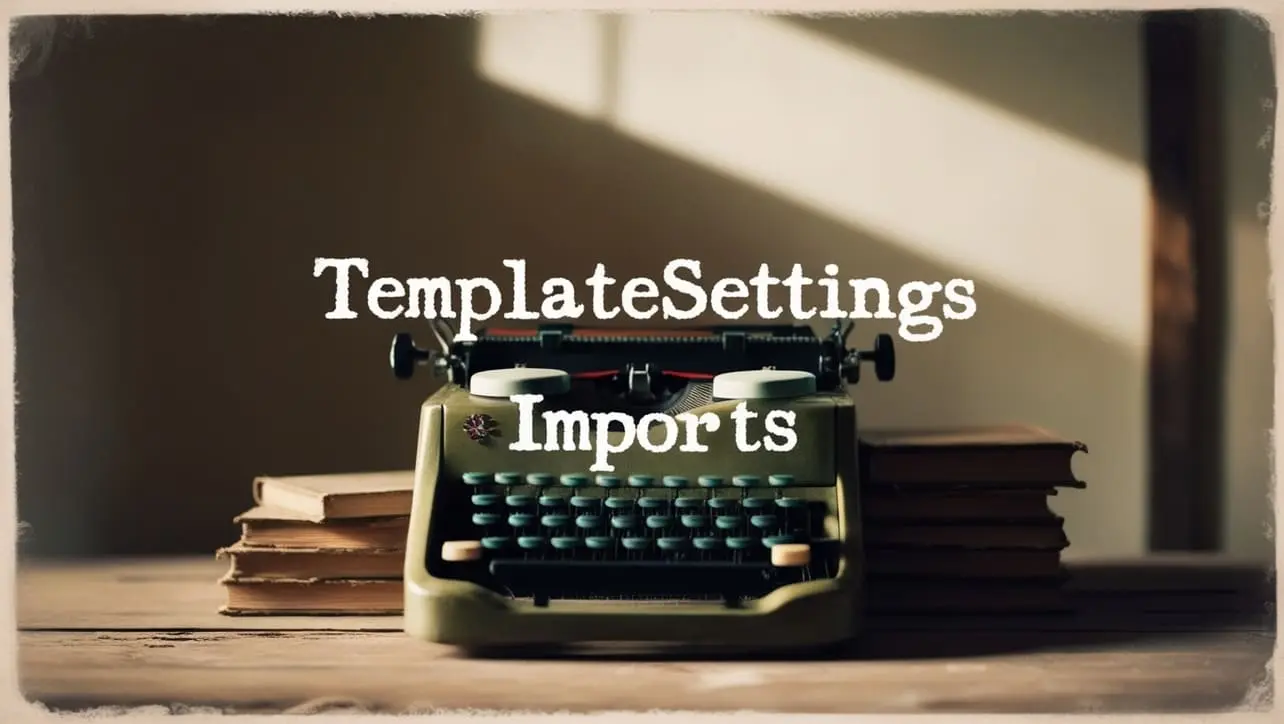
Lodash _.templateSettings.imports Property
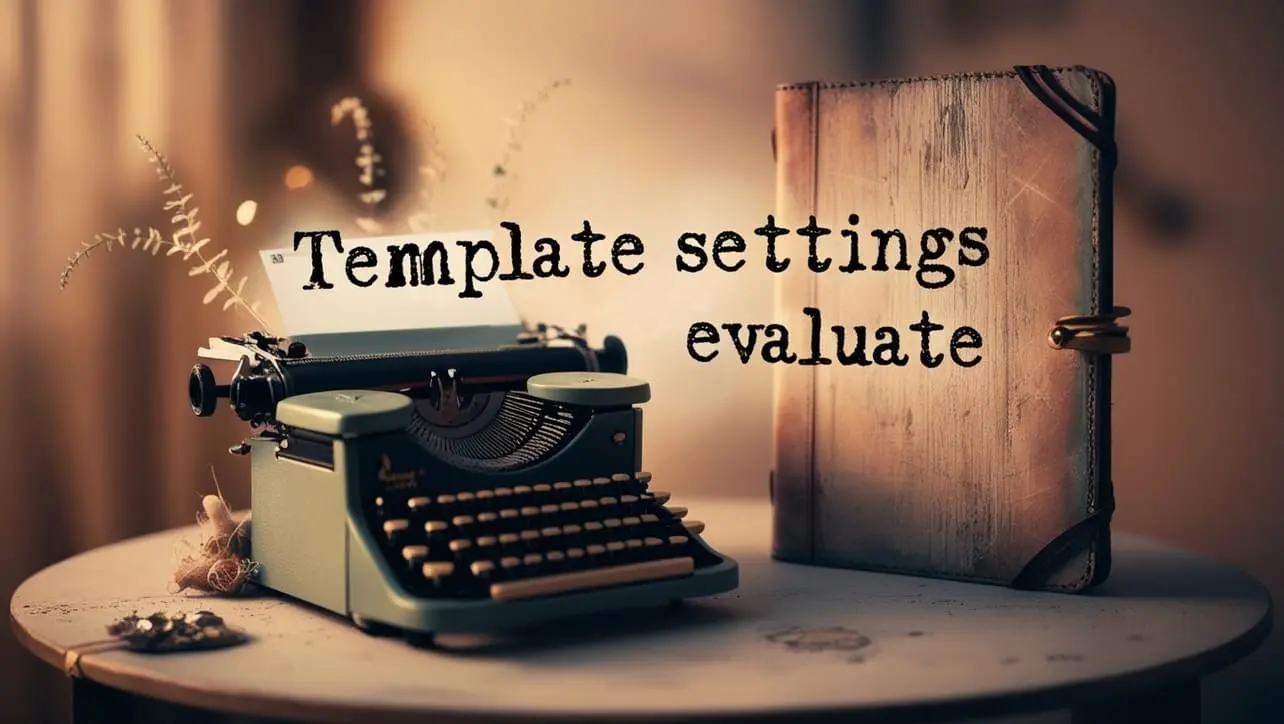
Lodash _.templateSettings.evaluate Property
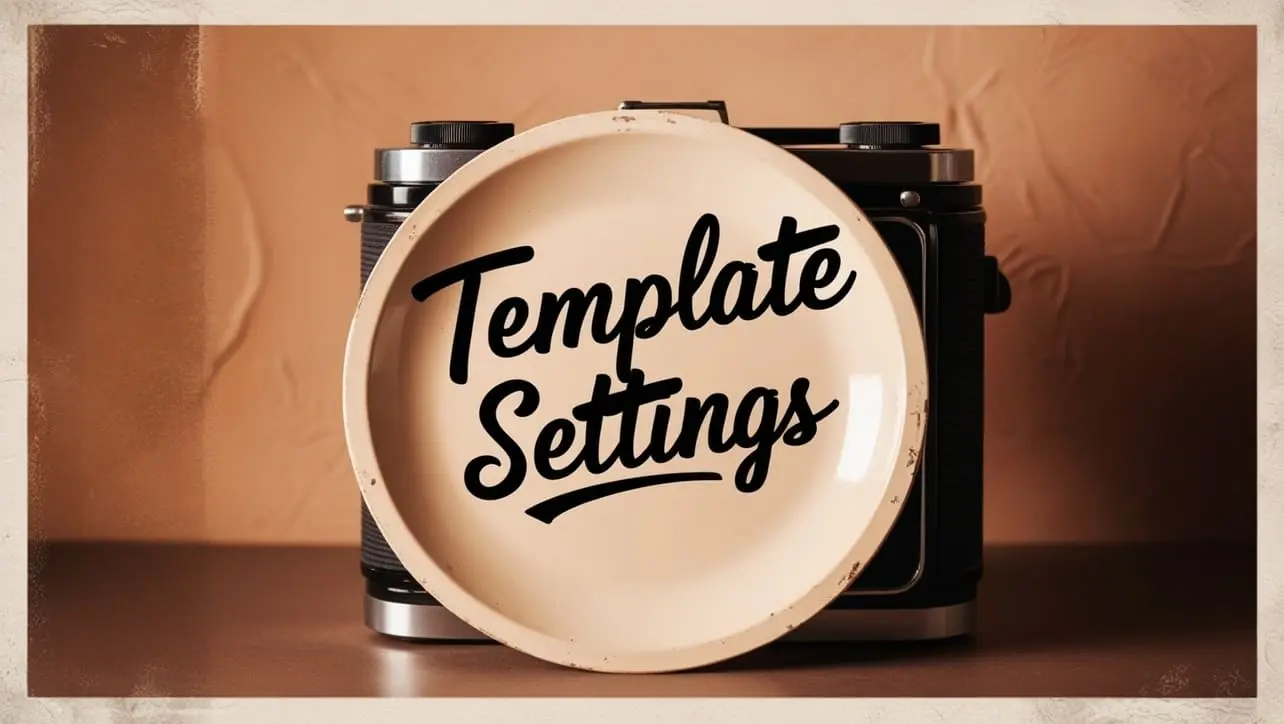
Lodash _.templateSettings Property
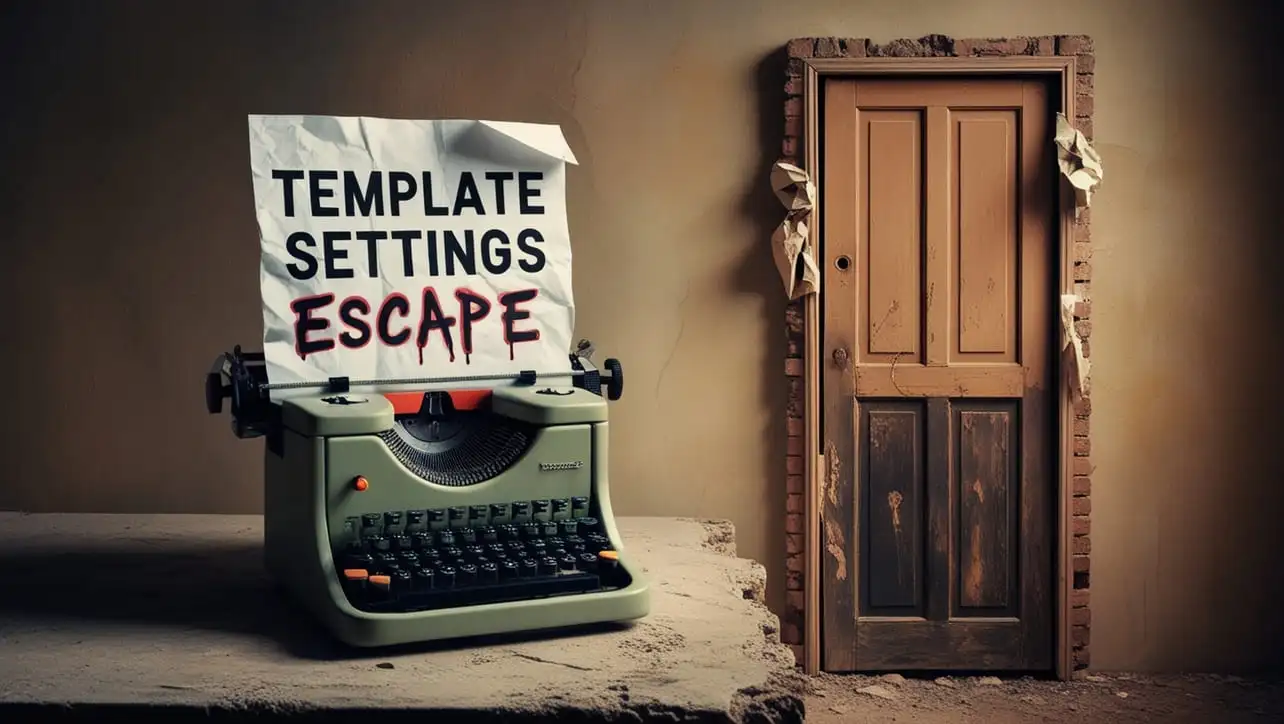
Lodash _.templateSettings.escape Property
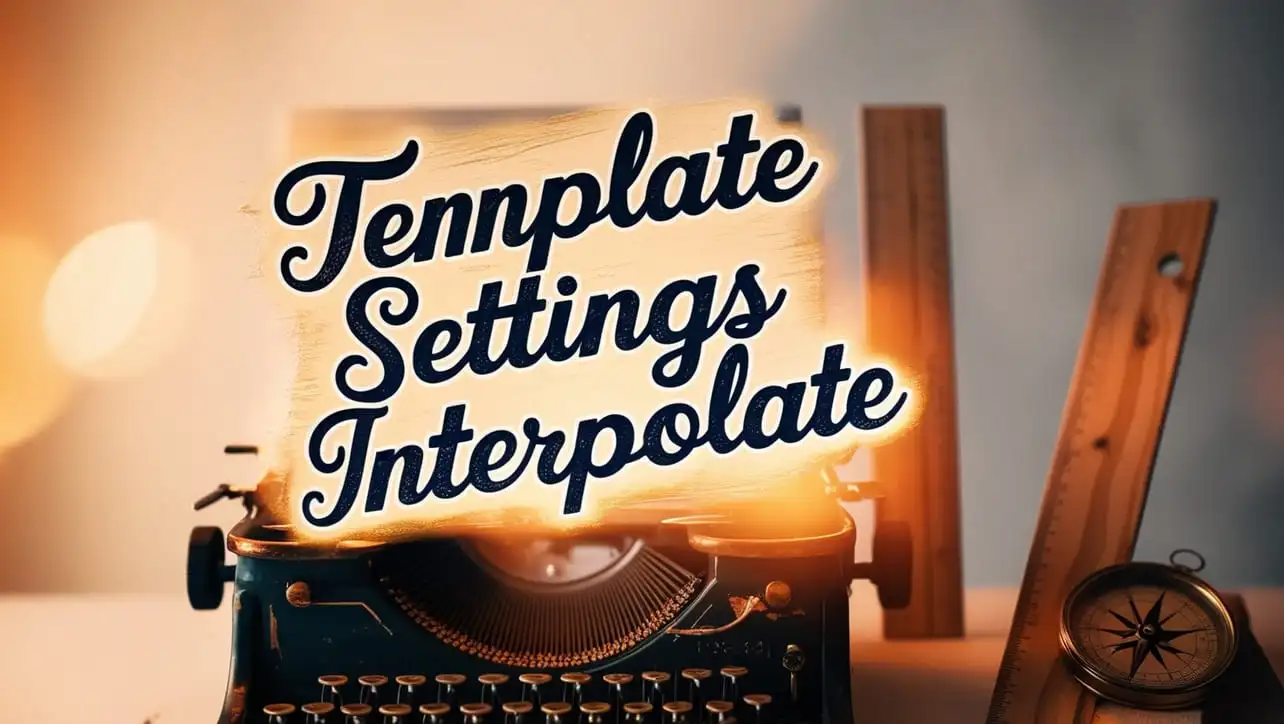
Lodash _.templateSettings.interpolate Property
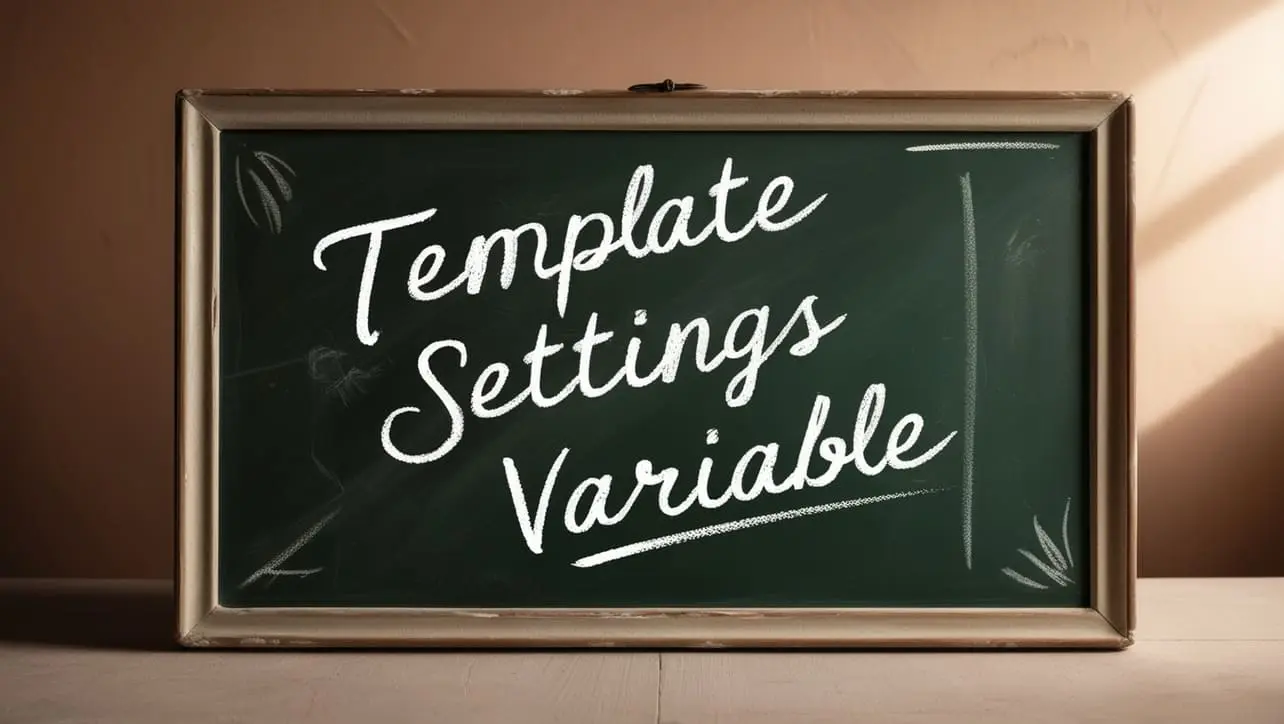
If you have any doubts regarding this article (Lodash _.isObjectLike() Lang Method), please comment here. I will help you immediately.