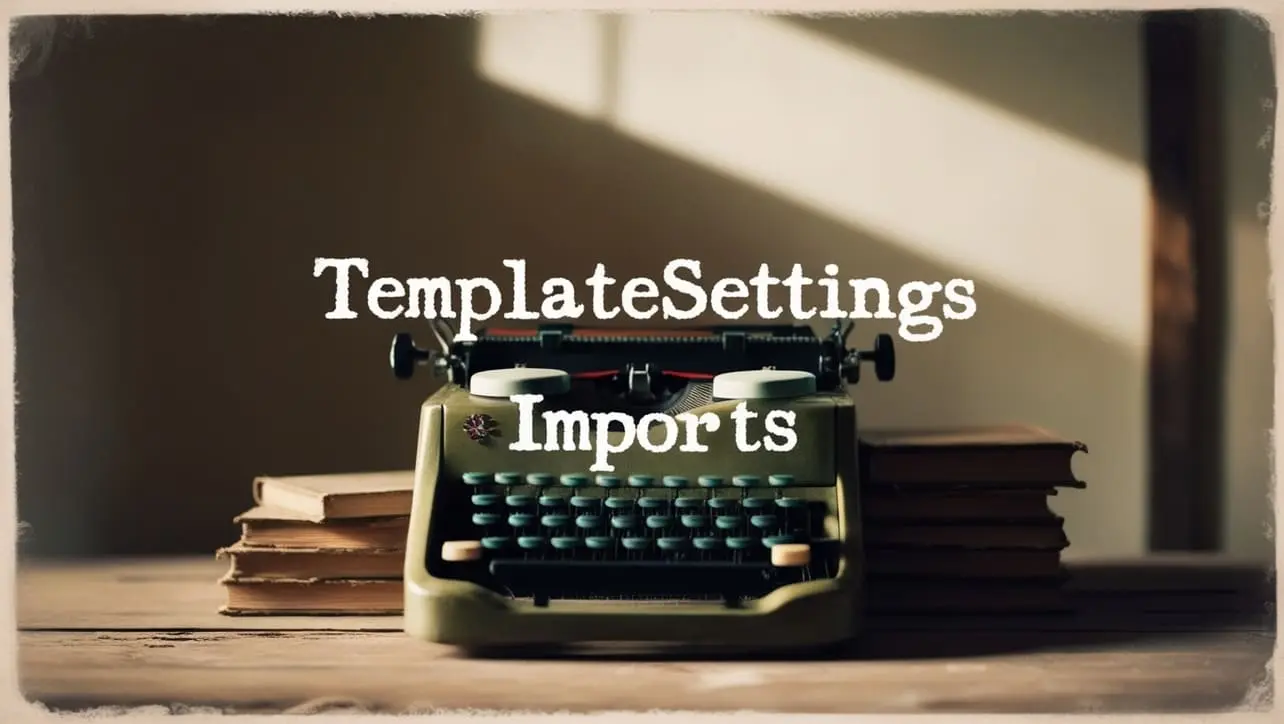
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isNative() Lang Method
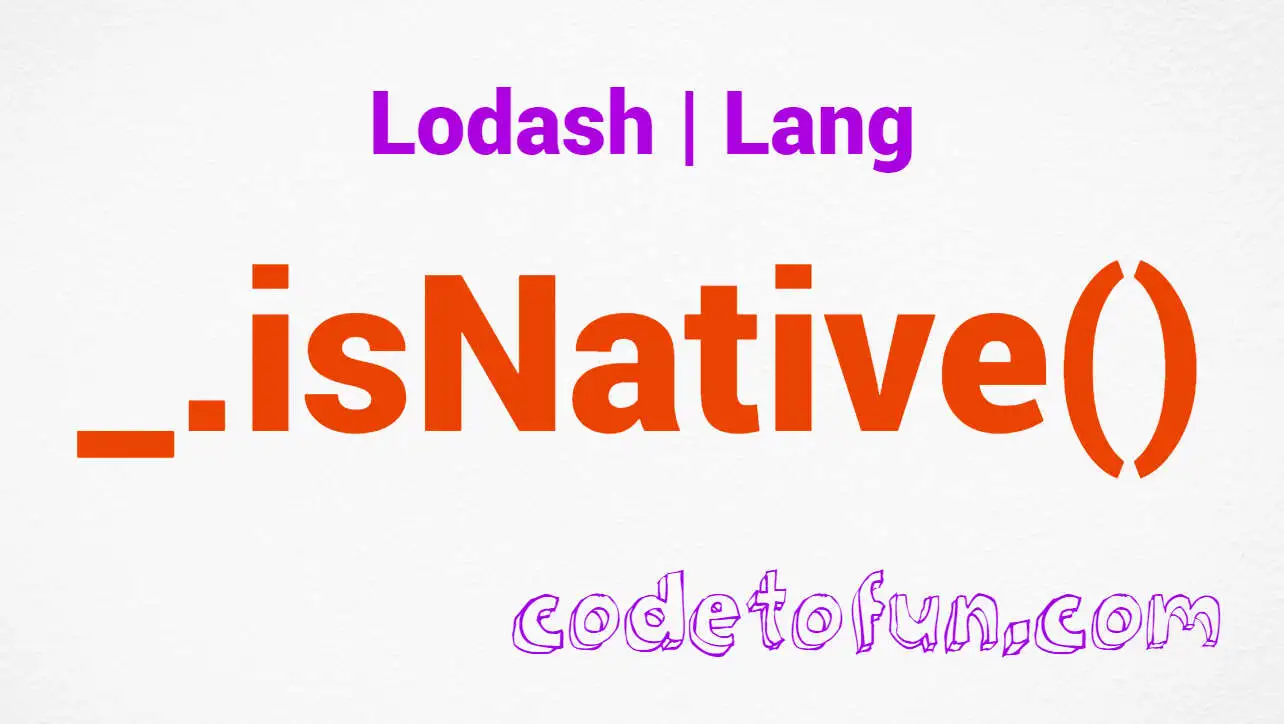
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript, understanding the nature of functions and methods is essential for effective programming. Lodash, a versatile utility library, provides the _.isNative()
method as a means of identifying native functions within the language.
This method is a valuable tool for developers seeking to distinguish between functions implemented natively in JavaScript and those created through user-defined means or libraries.
🧠 Understanding _.isNative() Method
The _.isNative()
method in Lodash is designed to determine if a given value is a native function. It aids developers in distinguishing between functions that are part of the JavaScript language specification and those that are crafted through user-defined logic or external libraries.
💡 Syntax
The syntax for the _.isNative()
method is straightforward:
_.isNative(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isNative()
method:
const _ = require('lodash');
const nativeFunction = Array.isArray;
const userDefinedFunction = function customFunction() {};
console.log(_.isNative(nativeFunction)); // Output: true
console.log(_.isNative(userDefinedFunction)); // Output: false
In this example, _.isNative()
is used to identify whether a function is native or user-defined.
🏆 Best Practices
When working with the _.isNative()
method, consider the following best practices:
Use in Feature Detection:
Employ
_.isNative()
in feature detection to check if a particular function or method is natively supported by the JavaScript environment. This is especially useful when dealing with browser compatibility or different JavaScript runtime environments.example.jsCopiedconst supportMap = { Map: _.isNative(Map), Set: _.isNative(Set), Promise: _.isNative(Promise), // Add other features as needed }; console.log(supportMap);
Enhance Code Robustness:
Leverage
_.isNative()
to enhance the robustness of your code by differentiating between native and non-native functions. This can be particularly helpful when implementing fallbacks or alternative strategies based on the availability of certain features.example.jsCopiedconst implementation = _.isNative(Promise) ? Promise : customPromiseLibrary; implementation.resolve('Success!') .then(result => console.log(result)) .catch(error => console.error(error));
Avoid Overwriting Native Functions:
Use
_.isNative()
to check if a function is native before attempting to override or modify it. This precautionary measure can prevent unintended consequences and maintain the integrity of the JavaScript runtime.example.jsCopiedif (_.isNative(Array.prototype.map)) { // Safely extend the native map method Array.prototype.customMap = function(callback) { // Your custom implementation }; }
📚 Use Cases
Feature-Based Polyfills:
Implement feature-based polyfills by utilizing
_.isNative()
to determine whether a native function is supported. This approach allows developers to selectively apply polyfills only when needed.example.jsCopiedif (!_.isNative(Array.from)) { // Polyfill for Array.from Array.from = /* custom implementation */ ; } // Use Array.from safely
Conditional Function Wrapping:
Conditionally wrap functions based on whether they are native or user-defined. This can be beneficial for implementing middleware or aspect-oriented programming in a flexible manner.
example.jsCopiedfunction middlewareWrapper(func) { if (_.isNative(func)) { return function() { console.log('Executing native function'); return func.apply(this, arguments); }; } else { return func; // No wrapping for user-defined functions } } const wrappedMap = middlewareWrapper(Array.prototype.map); // Use wrappedMap as a safer alternative to Array.prototype.map
Library Compatibility Checks:
When developing or using libraries, employ
_.isNative()
to check the compatibility of functions or methods. This ensures that the library seamlessly integrates with the native features of the JavaScript environment.example.jsCopiedif (_.isNative(Object.entries)) { // Use Object.entries directly } else { // Implement a custom version or use a polyfill }
🎉 Conclusion
The _.isNative()
method in Lodash serves as a valuable tool for developers navigating the intricacies of JavaScript function identification. Whether you're conducting feature detection, ensuring code robustness, or implementing conditional logic, _.isNative()
empowers you to make informed decisions in your JavaScript development journey.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isNative()
method in your Lodash projects.
👨💻 Join our Community:
Author
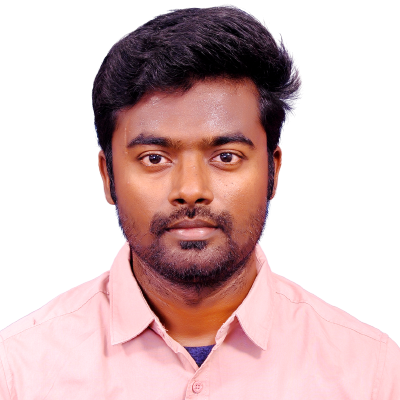
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
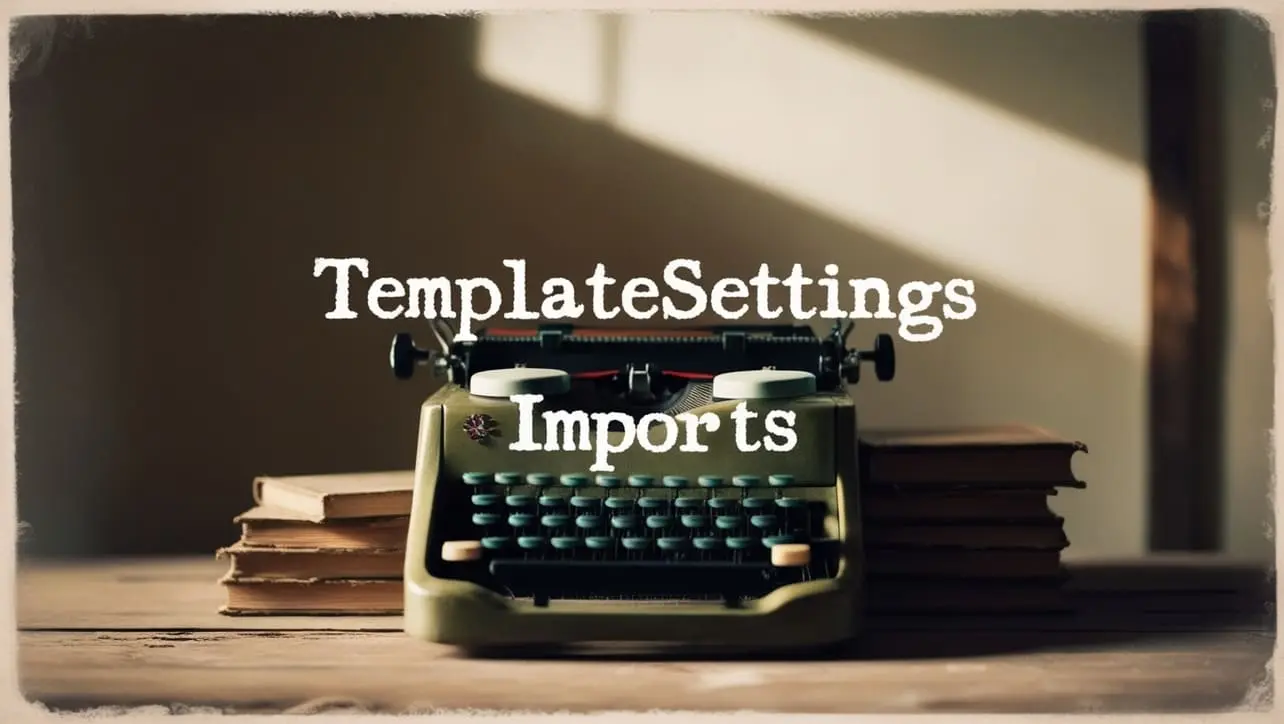
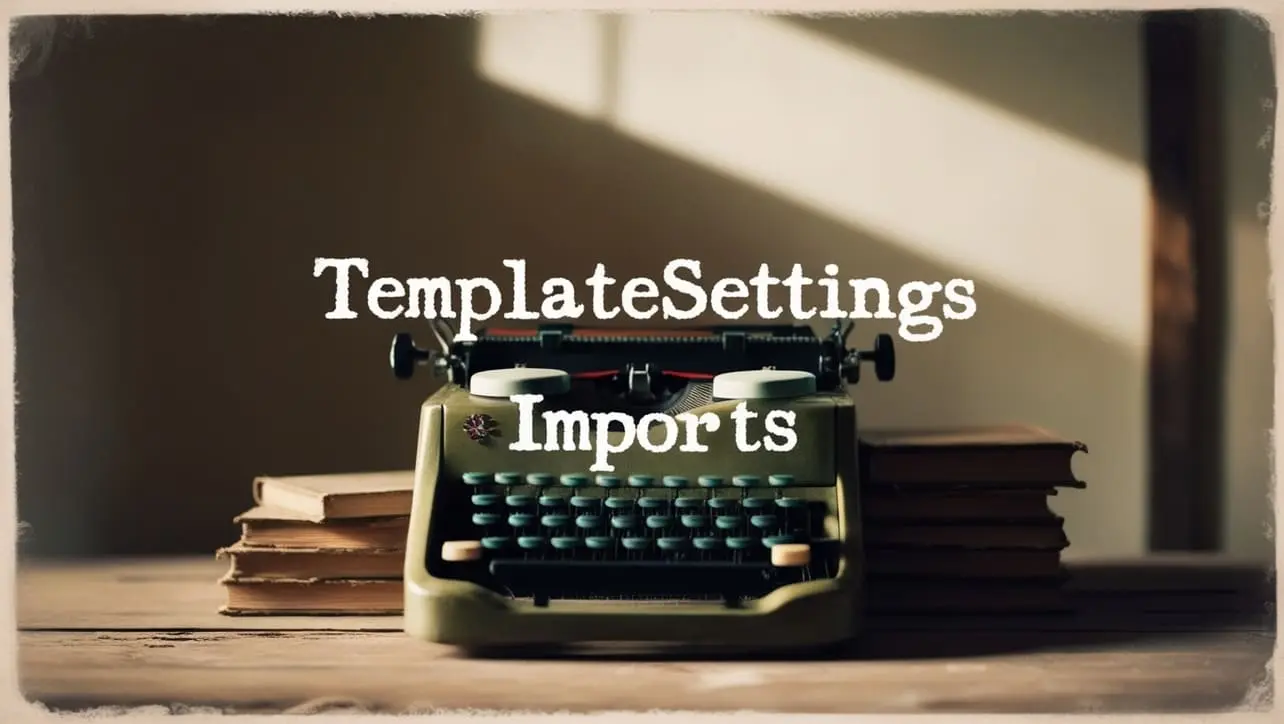
Lodash _.templateSettings.imports Property
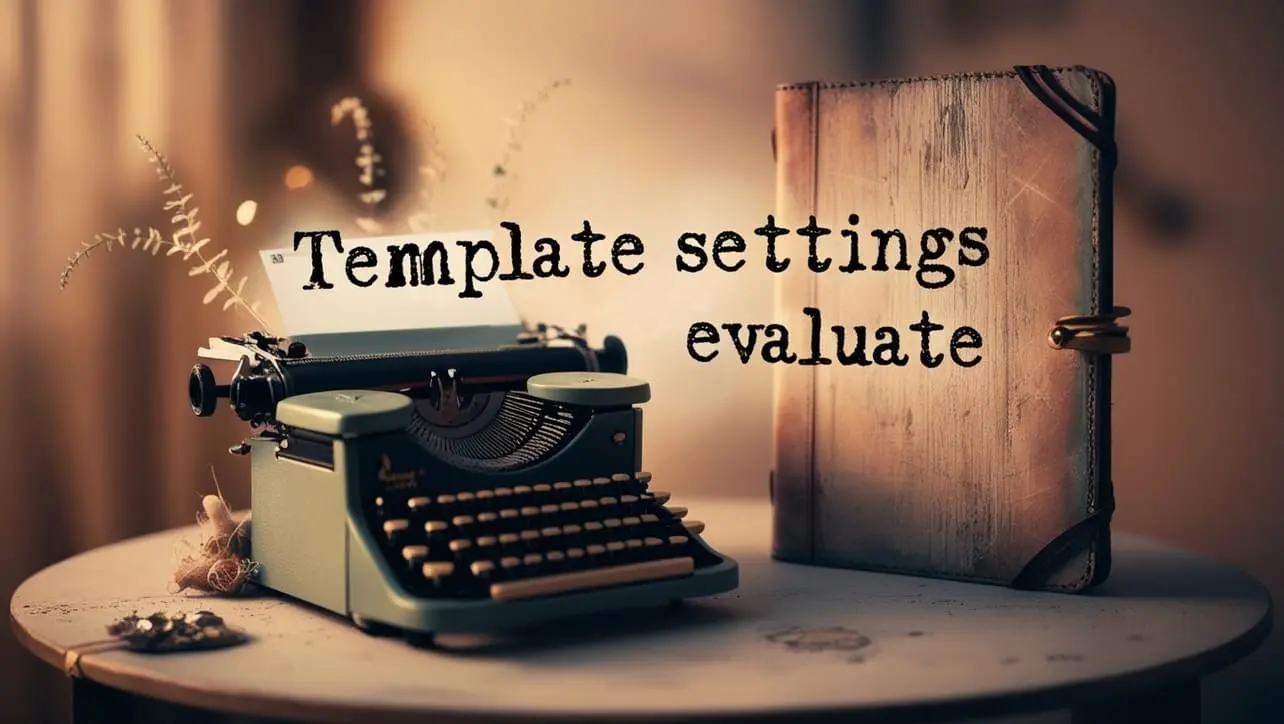
Lodash _.templateSettings.evaluate Property
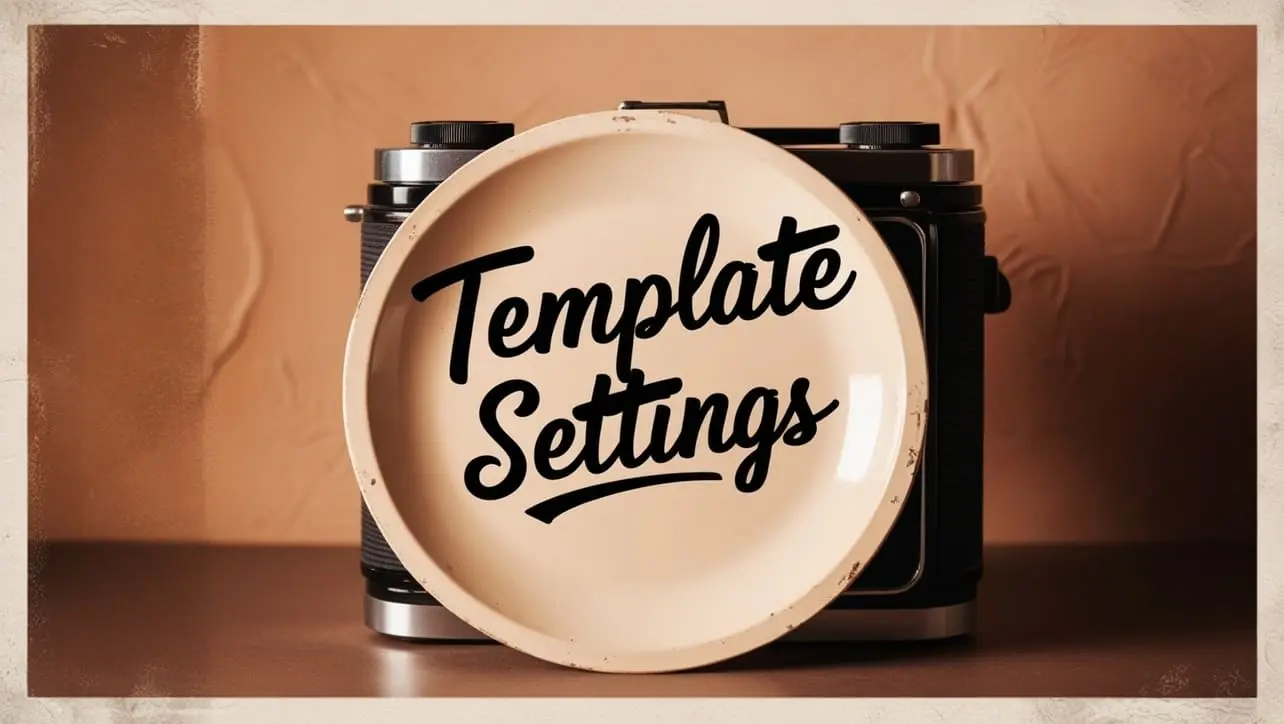
Lodash _.templateSettings Property
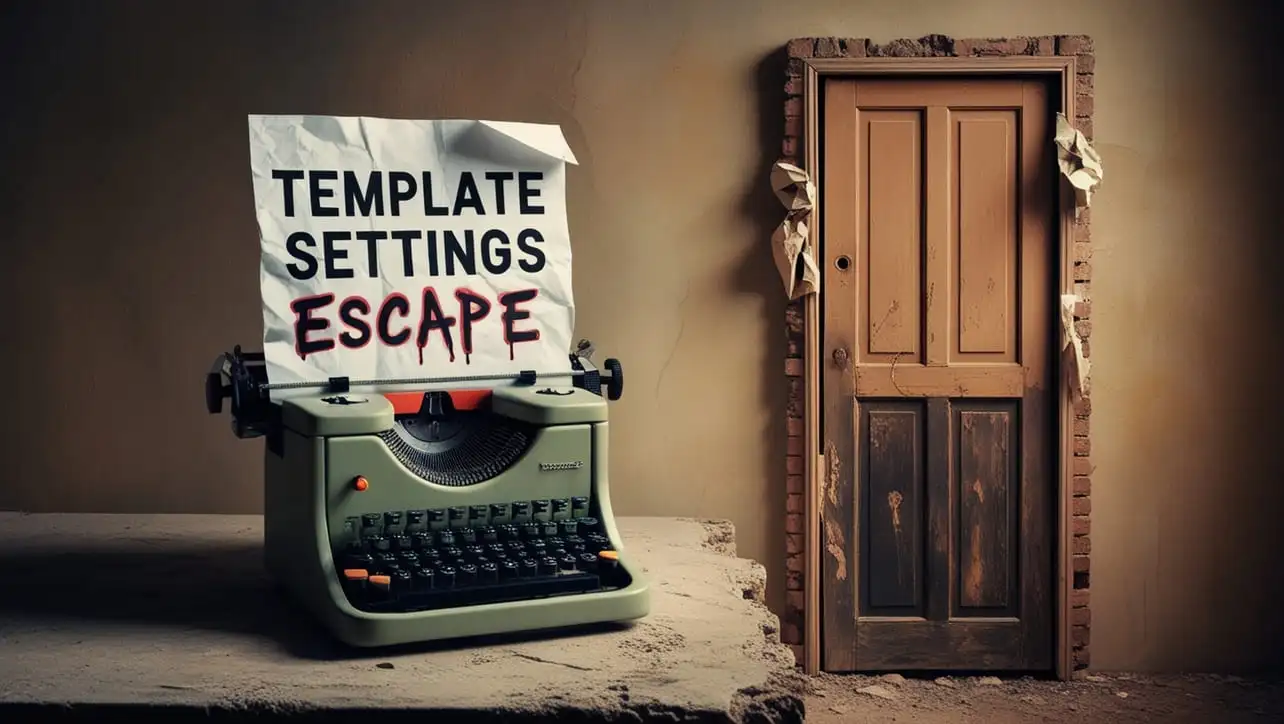
Lodash _.templateSettings.escape Property
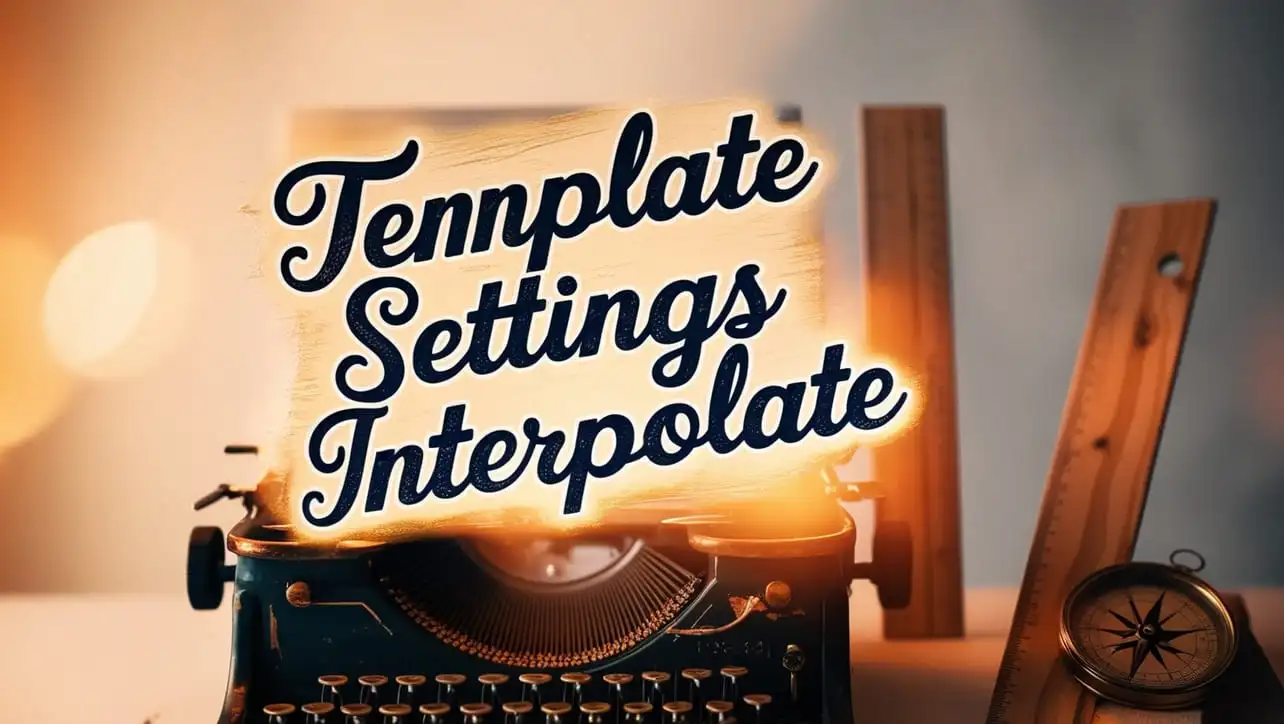
Lodash _.templateSettings.interpolate Property
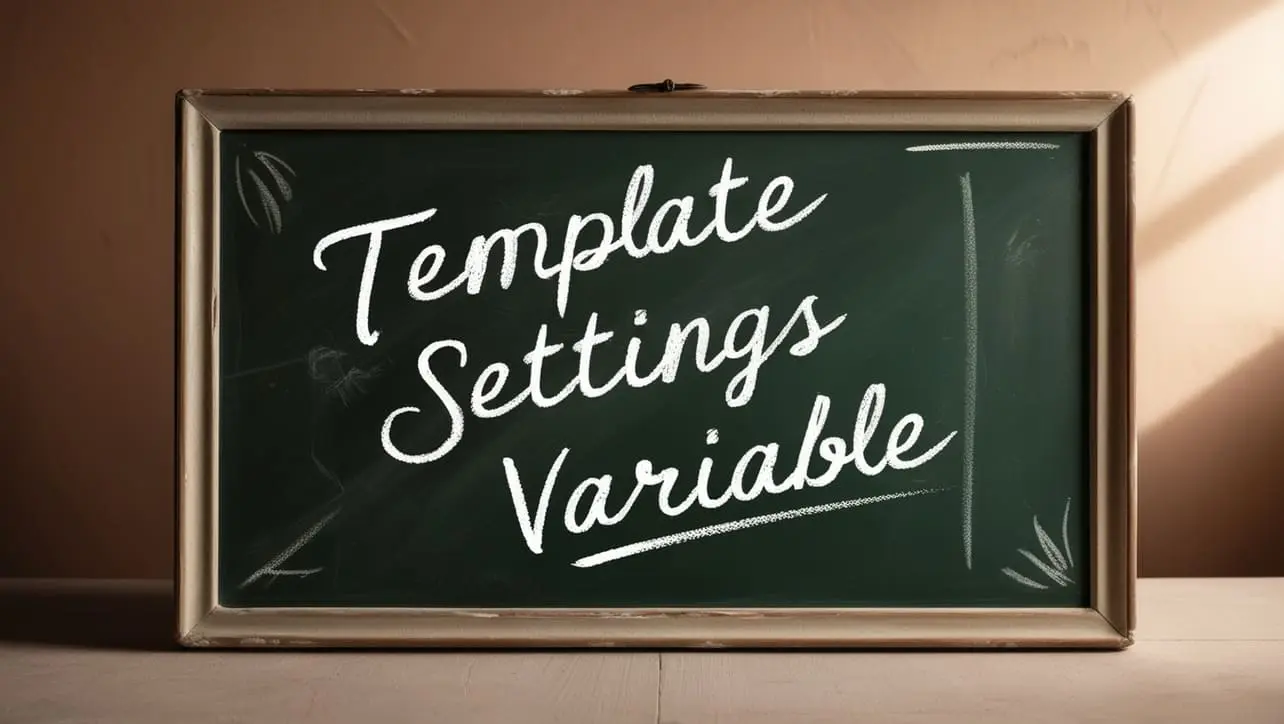
If you have any doubts regarding this article (Lodash _.isNative() Lang Method), please comment here. I will help you immediately.