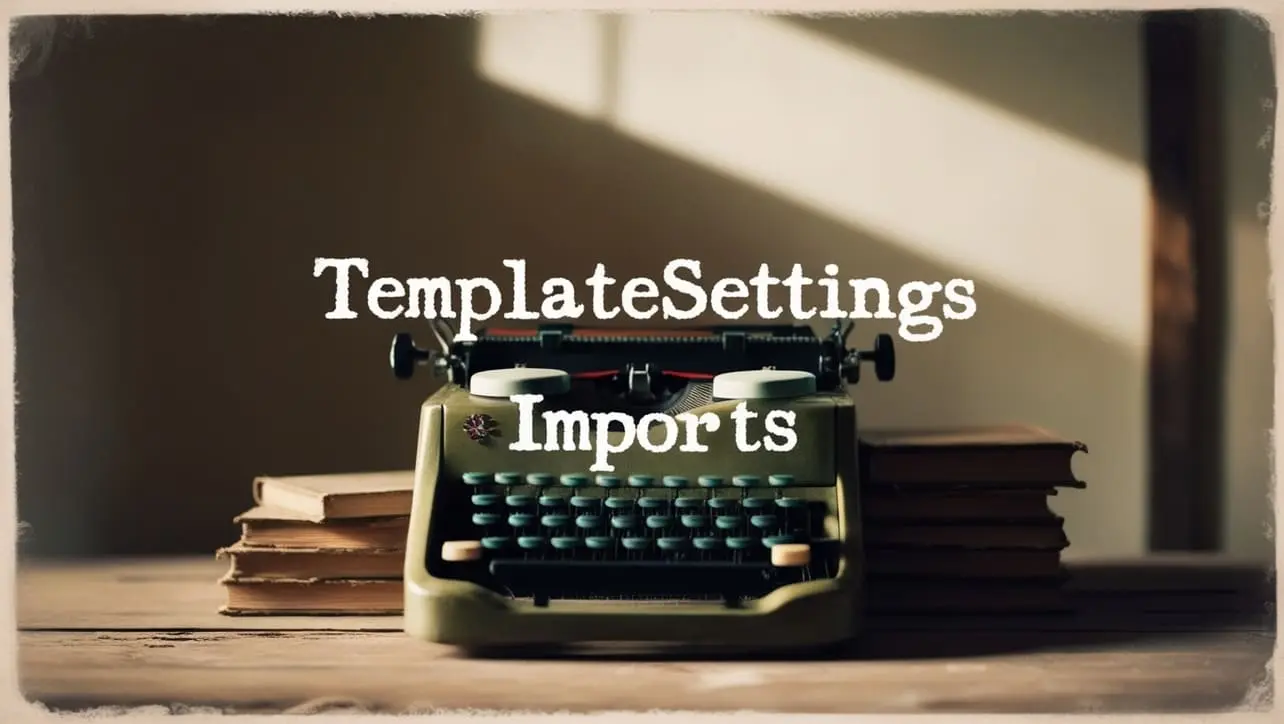
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isNaN() Lang Method
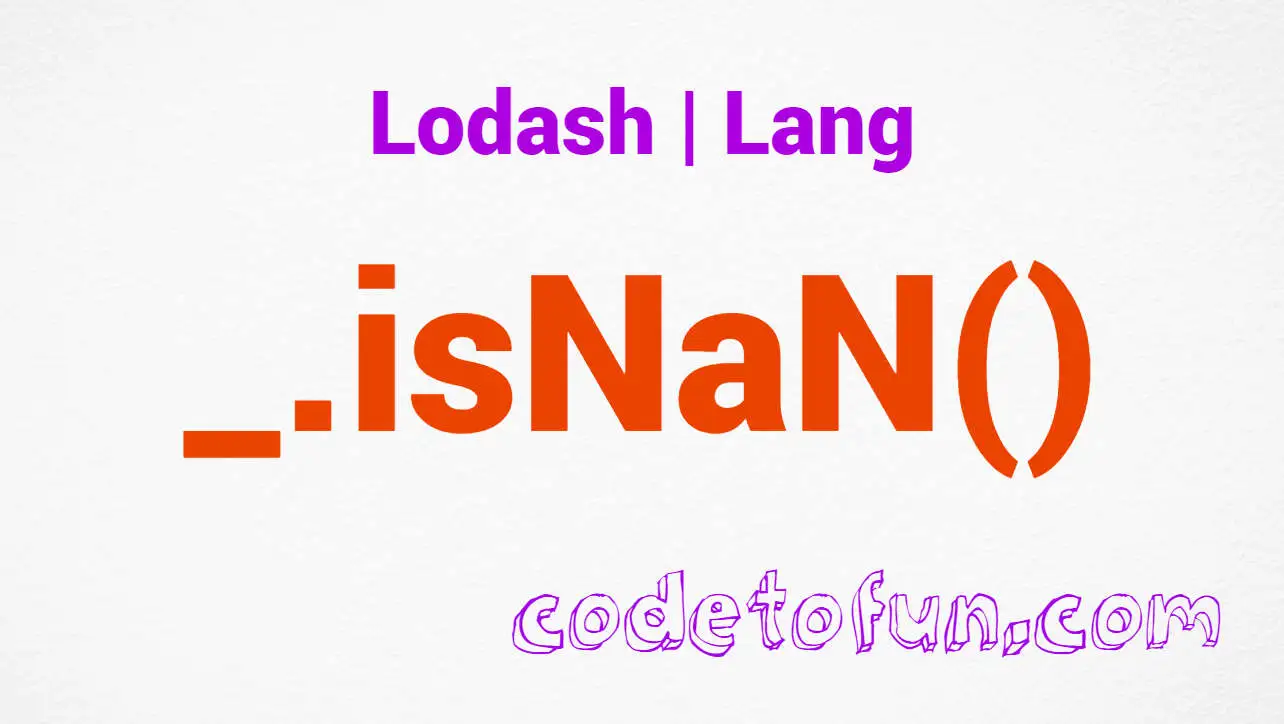
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, handling numeric values and checking for "Not a Number" (NaN) scenarios is a common requirement. Lodash, a powerful utility library, offers the _.isNaN()
method as a reliable tool for precisely determining whether a given value is NaN.
This method simplifies numeric validation, providing developers with a robust solution for dealing with the complexities of NaN in their code.
🧠 Understanding _.isNaN() Method
The _.isNaN()
method in Lodash is designed to identify whether a value is NaN, providing a consistent and effective way to handle numeric checks.
💡 Syntax
The syntax for the _.isNaN()
method is straightforward:
_.isNaN(value)
- value: The value to check for NaN.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isNaN()
method:
const _ = require('lodash');
console.log(_.isNaN(NaN));
// Output: true
console.log(_.isNaN(42));
// Output: false
console.log(_.isNaN('Not a Number'));
// Output: false
In this example, _.isNaN()
accurately determines whether the provided values are NaN.
🏆 Best Practices
When working with the _.isNaN()
method, consider the following best practices:
Consistent Type Checking:
Use
_.isNaN()
for consistent and accurate NaN checks, especially when dealing with user inputs or data from external sources.example.jsCopiedconst userInput = /* ...get user input... */ ; if (_.isNaN(userInput)) { console.error('Invalid numeric input'); } else { // Continue processing valid numeric input console.log('Processing input:', userInput); }
Numeric Validation in Functions:
In functions that expect numeric parameters, utilize
_.isNaN()
for robust numeric validation.example.jsCopiedfunction processNumber(value) { if (_.isNaN(value)) { console.error('Invalid numeric input'); return; } // Continue processing valid numeric input console.log('Processing input:', value); } // Example usage processNumber(25); processNumber('Invalid Input');
Handling Calculations:
When performing calculations, use
_.isNaN()
to check for potential NaN results and handle them appropriately.example.jsCopiedfunction divideNumbers(a, b) { const result = a / b; if (_.isNaN(result)) { console.error('Division resulted in NaN'); return; } // Continue processing valid result console.log('Result:', result); } // Example usage divideNumbers(10, 2); divideNumbers(5, 0);
📚 Use Cases
Input Validation:
_.isNaN()
is invaluable for input validation, ensuring that numeric inputs meet the expected criteria.example.jsCopiedconst userInput = /* ...get user input... */ ; if (_.isNaN(userInput)) { console.error('Invalid numeric input'); } else { // Continue processing valid numeric input console.log('Processing input:', userInput); }
Conditional Execution:
In scenarios where certain actions should only occur for valid numeric values,
_.isNaN()
aids in creating conditional logic.example.jsCopiedconst numericValue = /* ...get numeric value... */ ; if (!_.isNaN(numericValue)) { // Perform actions only for valid numeric values console.log('Valid numeric value:', numericValue); } else { console.error('Invalid numeric value'); }
Calculations and Formulas:
When dealing with complex calculations or formulas, use
_.isNaN()
to handle potential NaN results gracefully.example.jsCopiedfunction calculateComplexFormula(a, b, c) { const result = /* ...perform complex calculation... */ ; if (_.isNaN(result)) { console.error('Invalid result. Check input values.'); return; } // Continue processing valid result console.log('Result:', result); } // Example usage calculateComplexFormula(10, 2, 5); calculateComplexFormula(5, 0, 8);
🎉 Conclusion
The _.isNaN()
method in Lodash provides a reliable and consistent approach to checking for NaN in JavaScript. Whether you're validating user inputs, handling numeric parameters in functions, or performing complex calculations, _.isNaN()
is a versatile tool for managing the intricacies of NaN scenarios.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isNaN()
method in your Lodash projects.
👨💻 Join our Community:
Author
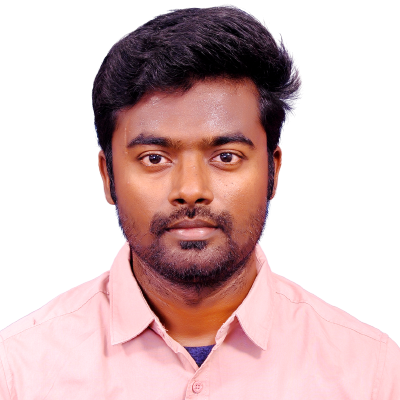
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
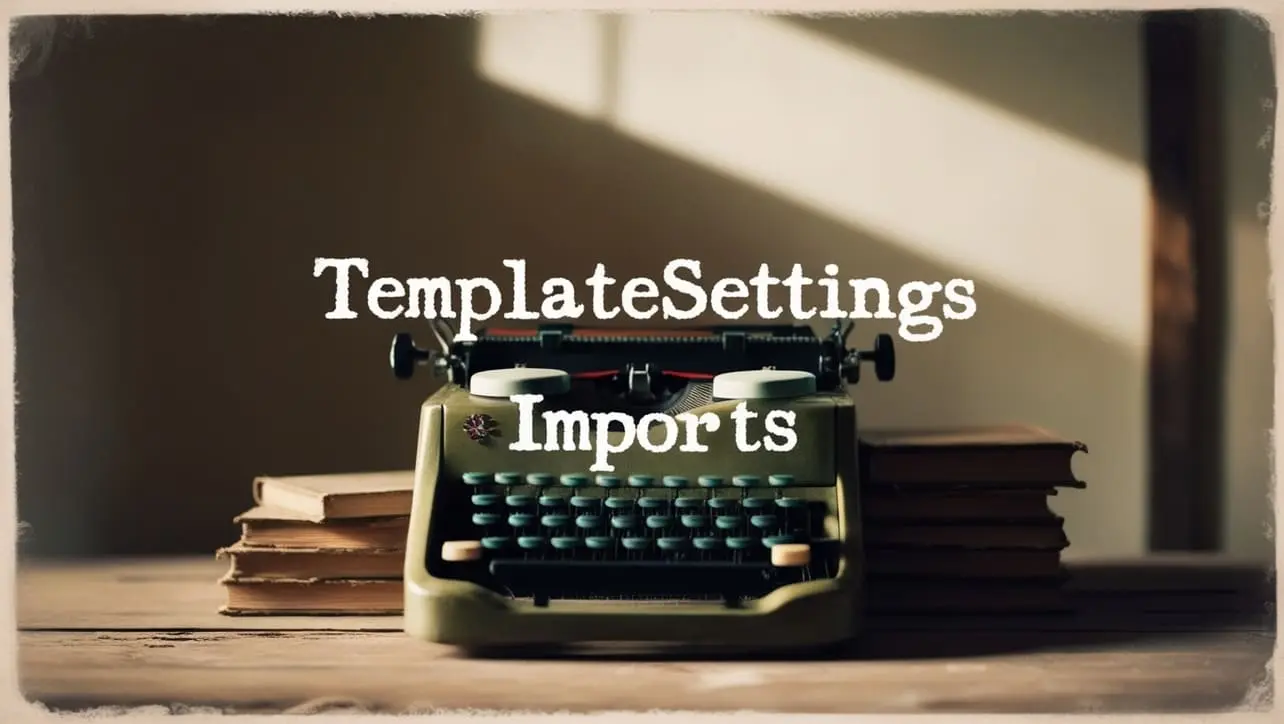
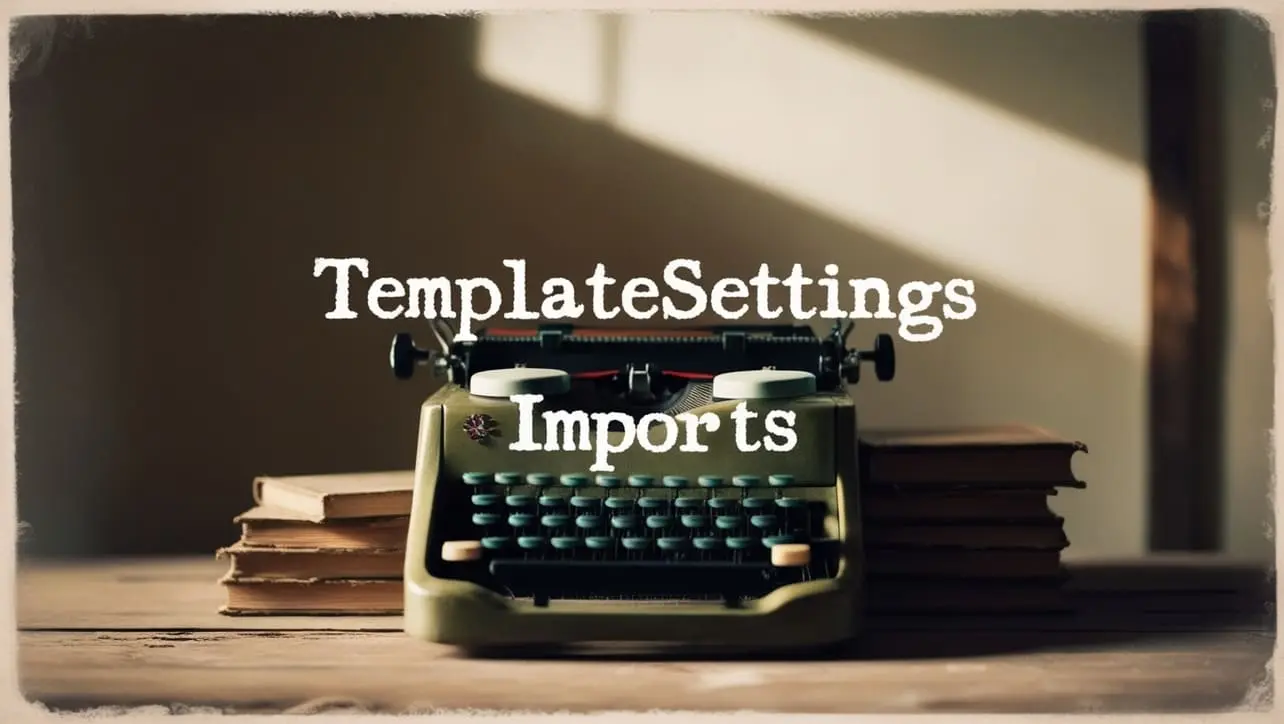
Lodash _.templateSettings.imports Property
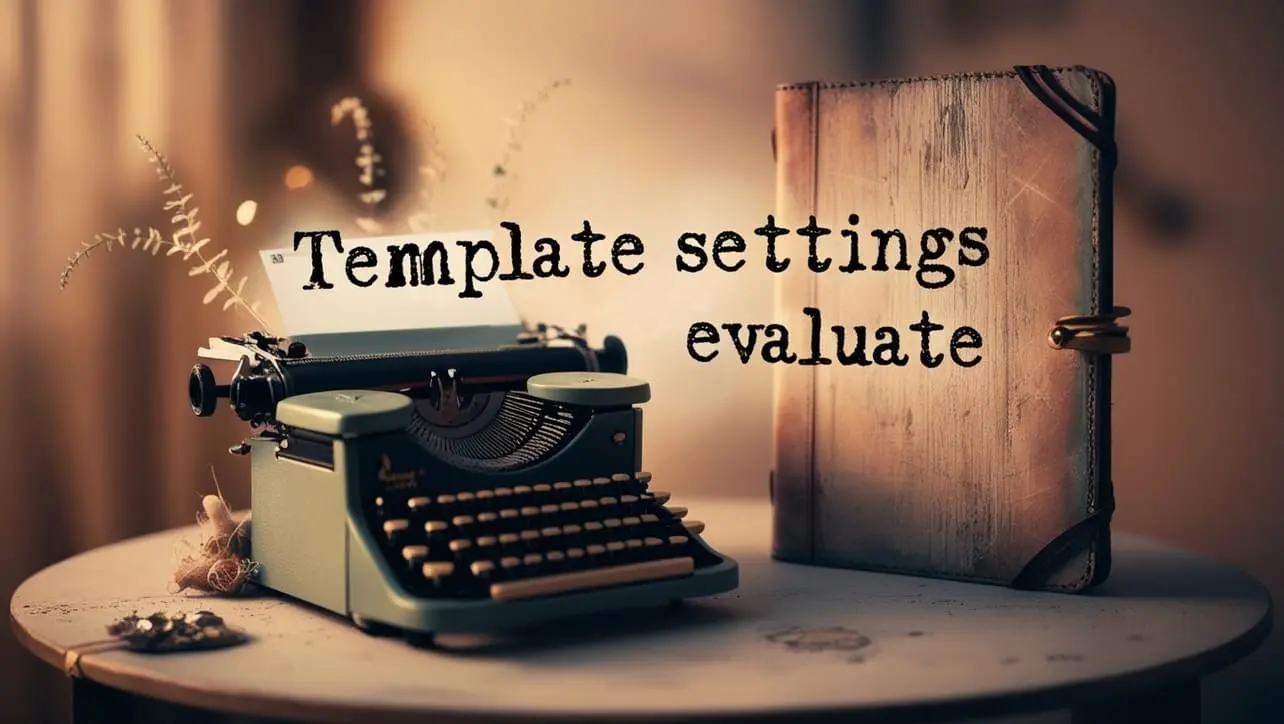
Lodash _.templateSettings.evaluate Property
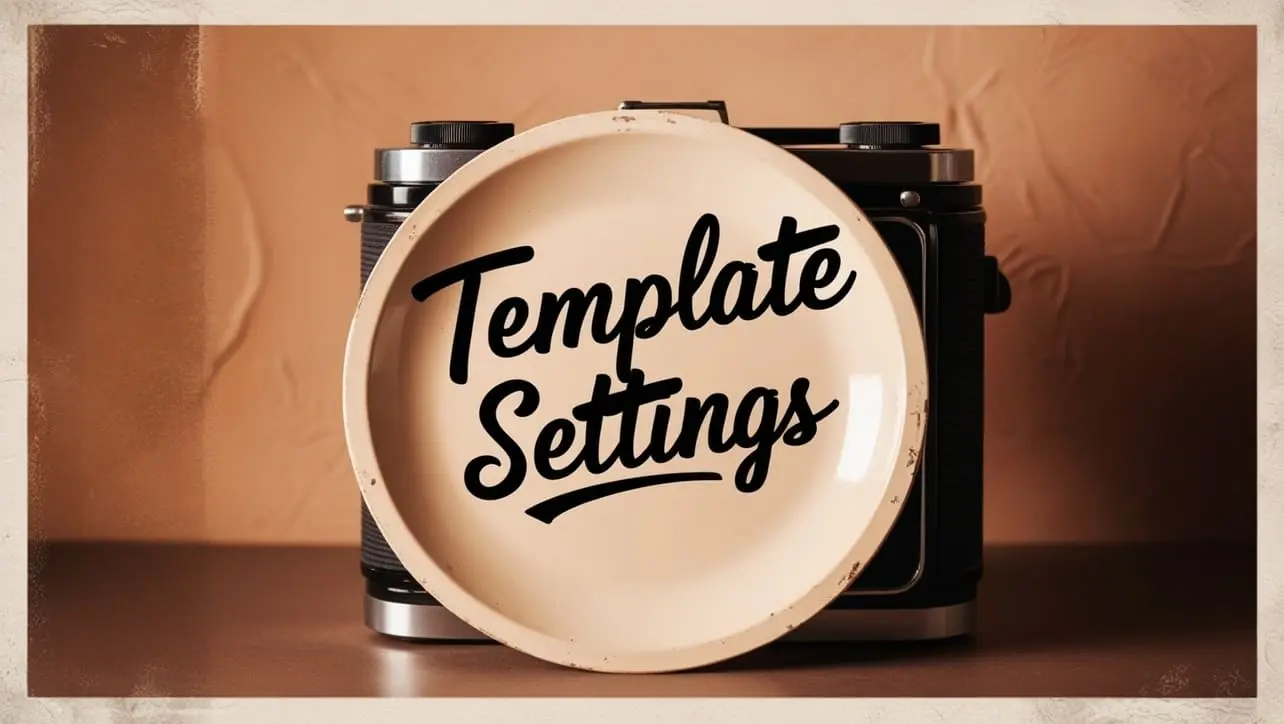
Lodash _.templateSettings Property
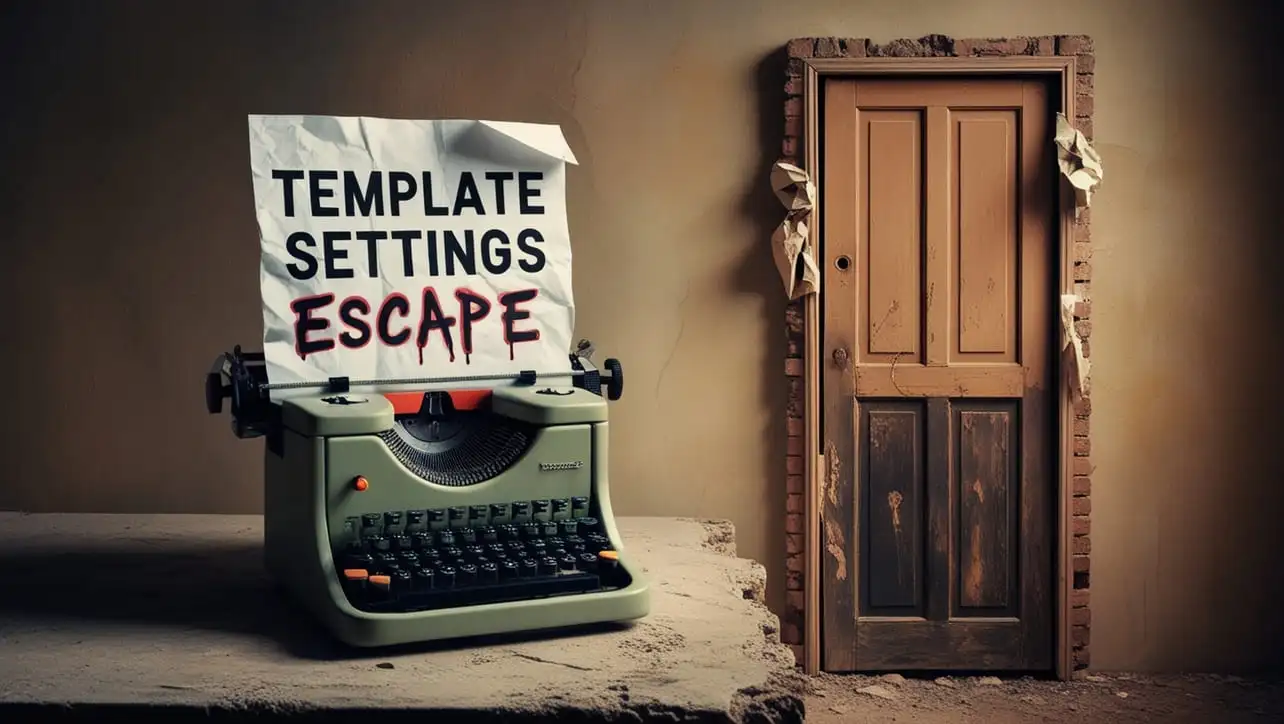
Lodash _.templateSettings.escape Property
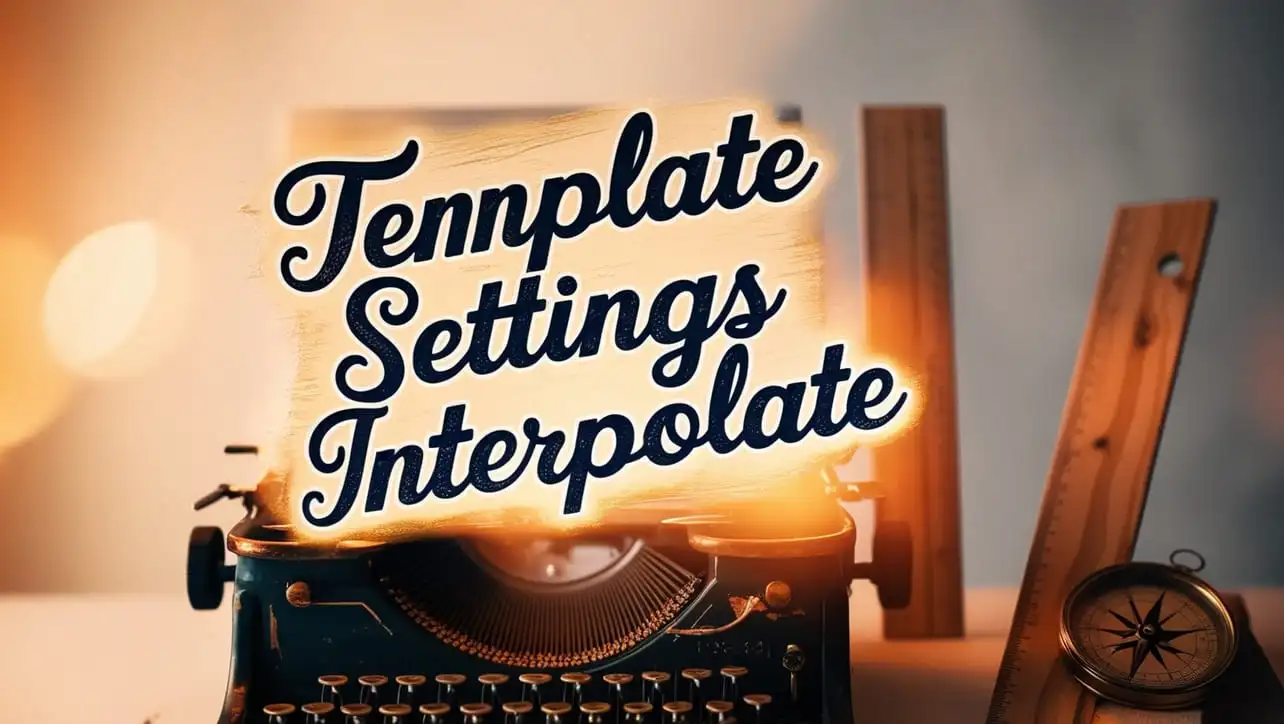
Lodash _.templateSettings.interpolate Property
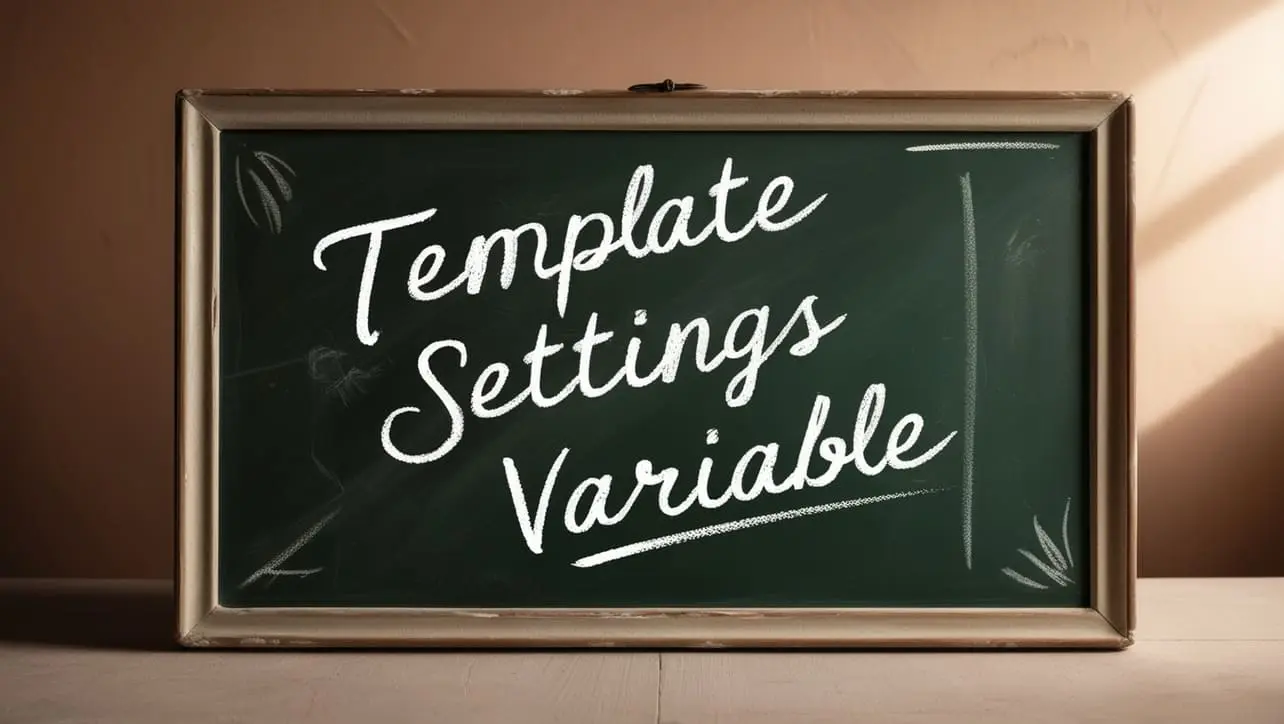
If you have any doubts regarding this article (Lodash _.isNaN() Lang Method), please comment here. I will help you immediately.