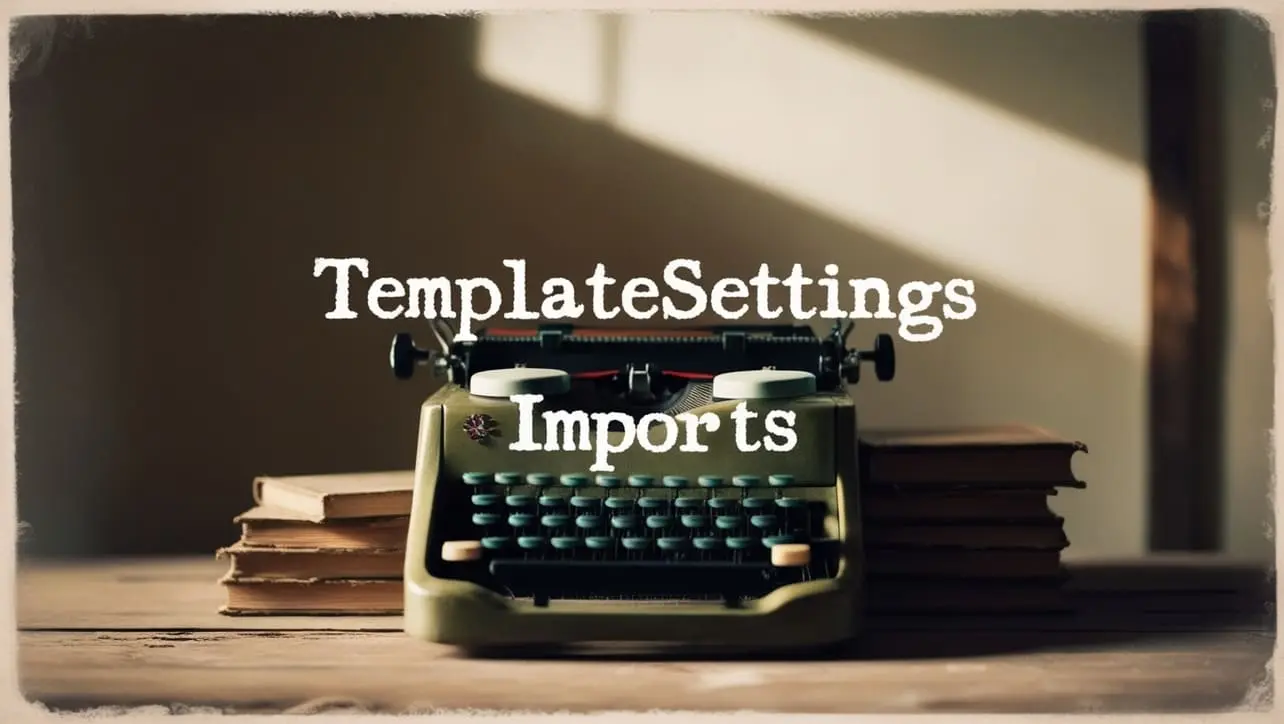
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isInteger() Lang Method
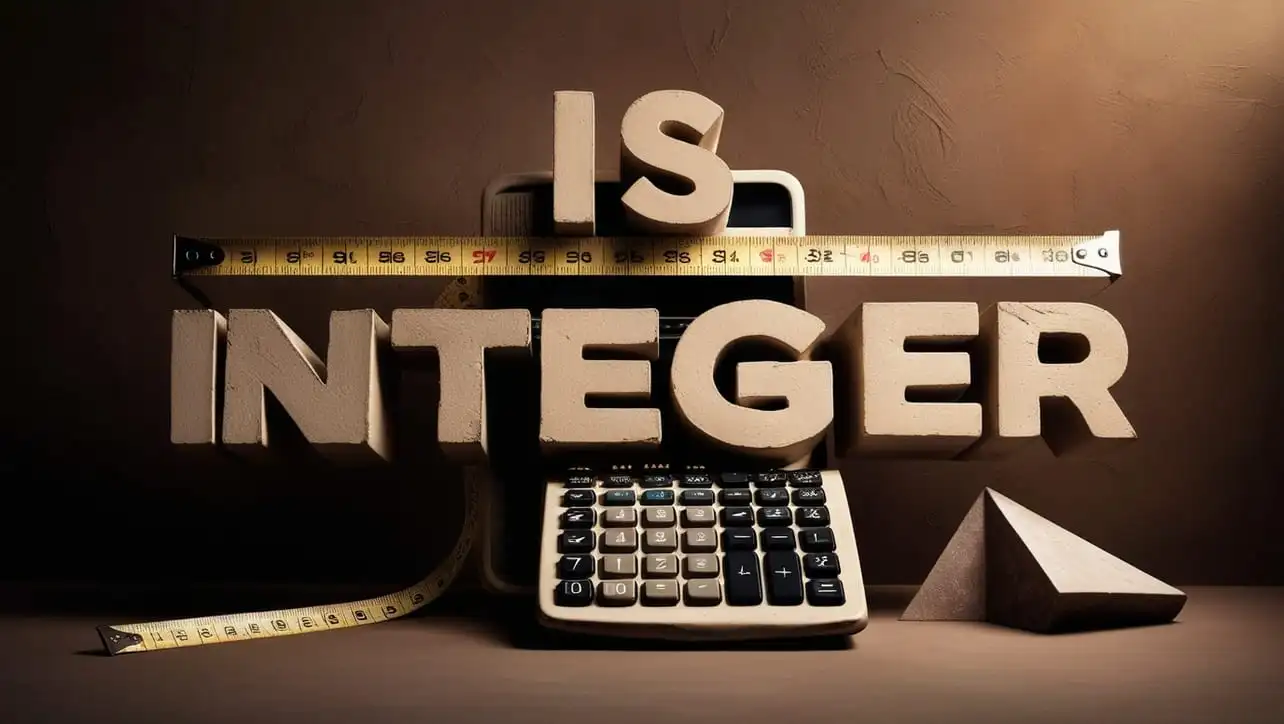
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript, data validation plays a crucial role in ensuring the integrity of your code. Lodash, a feature-rich utility library, offers a multitude of functions to simplify common programming tasks. Among these functions is the _.isInteger()
method, part of the Lang category in Lodash.
This method proves invaluable when you need to verify whether a given value is an integer, providing a reliable tool for data validation.
🧠 Understanding _.isInteger() Method
The _.isInteger()
method in Lodash is designed to determine whether a given value is an integer. It considers not only numbers but also ensures that the type of the value is explicitly "Number" and that it is not a decimal or has additional non-numeric characters.
💡 Syntax
The syntax for the _.isInteger()
method is straightforward:
_.isInteger(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isInteger()
method:
const _ = require('lodash');
console.log(_.isInteger(42));
// Output: true
console.log(_.isInteger(3.14));
// Output: false
console.log(_.isInteger('123'));
// Output: false
console.log(_.isInteger(null));
// Output: false
In this example, _.isInteger()
is used to validate different types of values, showcasing its ability to accurately determine whether a value is an integer.
🏆 Best Practices
When working with the _.isInteger()
method, consider the following best practices:
Type and Value Validation:
When using
_.isInteger()
, be aware that it not only checks if the value is numeric but also validates that it is of type "Number" and does not contain decimal points or non-numeric characters.example.jsCopiedconst validInteger = 42; const invalidInteger = '42'; console.log(_.isInteger(validInteger)); // Output: true console.log(_.isInteger(invalidInteger)); // Output: false
Handling NaN and Infinity:
Consider special cases such as NaN (Not a Number) and Infinity.
_.isInteger()
returns false for these cases, as they are not finite integers.example.jsCopiedconst notANumber = NaN; const infinityValue = Infinity; console.log(_.isInteger(notANumber)); // Output: false console.log(_.isInteger(infinityValue)); // Output: false
Use in Data Validation:
Integrate
_.isInteger()
into your data validation routines to ensure that variables or user inputs are appropriate numeric values.example.jsCopiedfunction validateUserInput(input) { if (_.isInteger(input)) { console.log('Input is a valid integer.'); } else { console.log('Input is not a valid integer.'); } } validateUserInput(25); validateUserInput('abc');
📚 Use Cases
Form Validation:
In scenarios where user input is expected to be an integer,
_.isInteger()
can be employed to validate form data and provide real-time feedback to users.example.jsCopiedconst userInput = /* ...retrieve user input from a form... */ ; if (_.isInteger(userInput)) { console.log('Valid integer entered.'); } else { console.log('Please enter a valid integer.'); }
Configuration Settings:
When working with configuration settings or environment variables that should represent integers, use
_.isInteger()
to validate and handle them appropriately.example.jsCopiedconst config = { maxItems: /* ...retrieve from configuration... */ , }; if (_.isInteger(config.maxItems)) { console.log('Valid configuration setting for maxItems.'); } else { console.error('Invalid maxItems configuration. Please provide a valid integer.'); }
Mathematical Operations:
Before performing mathematical operations that require integer operands, use
_.isInteger()
to validate the input values.example.jsCopiedfunction performIntegerOperation(a, b) { if (_.isInteger(a) && _.isInteger(b)) { const result = a + b; console.log('Result of integer operation:', result); } else { console.error('Invalid input for integer operation. Both operands should be integers.'); } } performIntegerOperation(5, 10); performIntegerOperation('abc', 10);
🎉 Conclusion
The _.isInteger()
method in Lodash provides a reliable solution for checking whether a value is an integer, offering a straightforward approach to data validation in JavaScript. Whether you're validating user input, configuration settings, or performing mathematical operations, this method proves to be a valuable asset in maintaining data integrity.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isInteger()
method in your Lodash projects.
👨💻 Join our Community:
Author
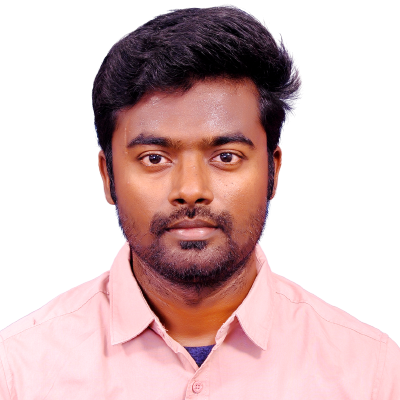
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
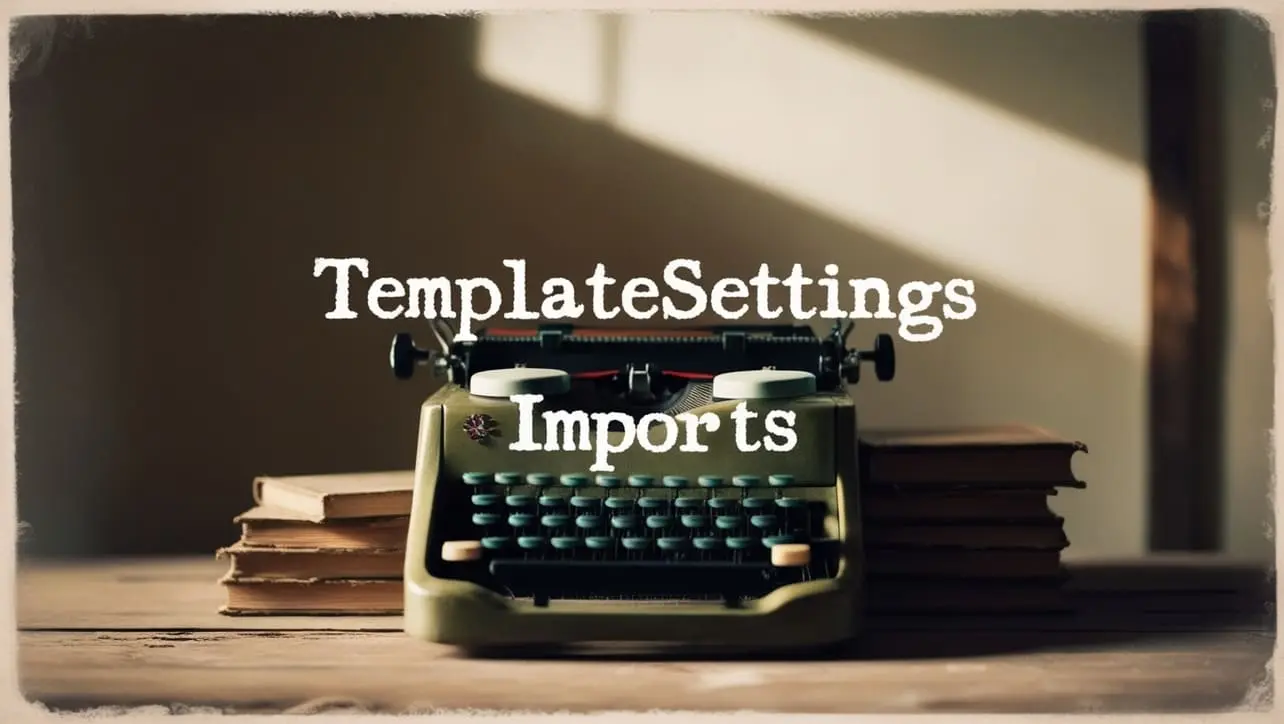
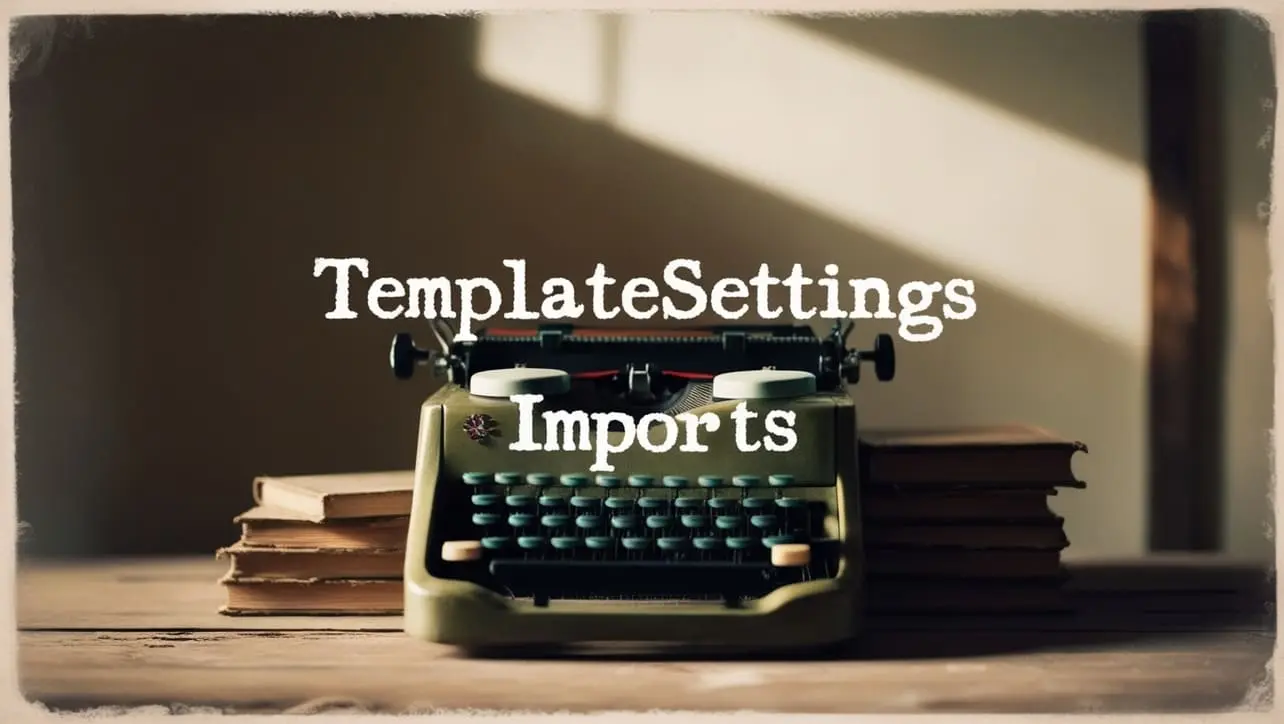
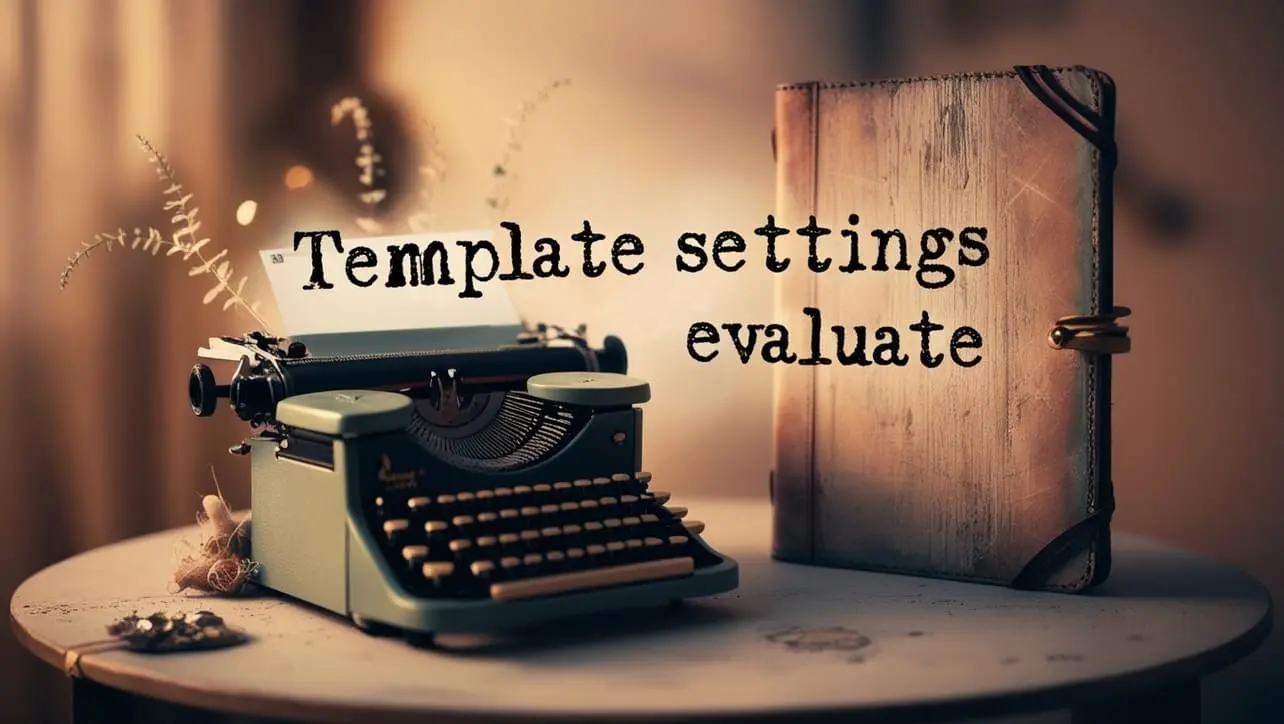
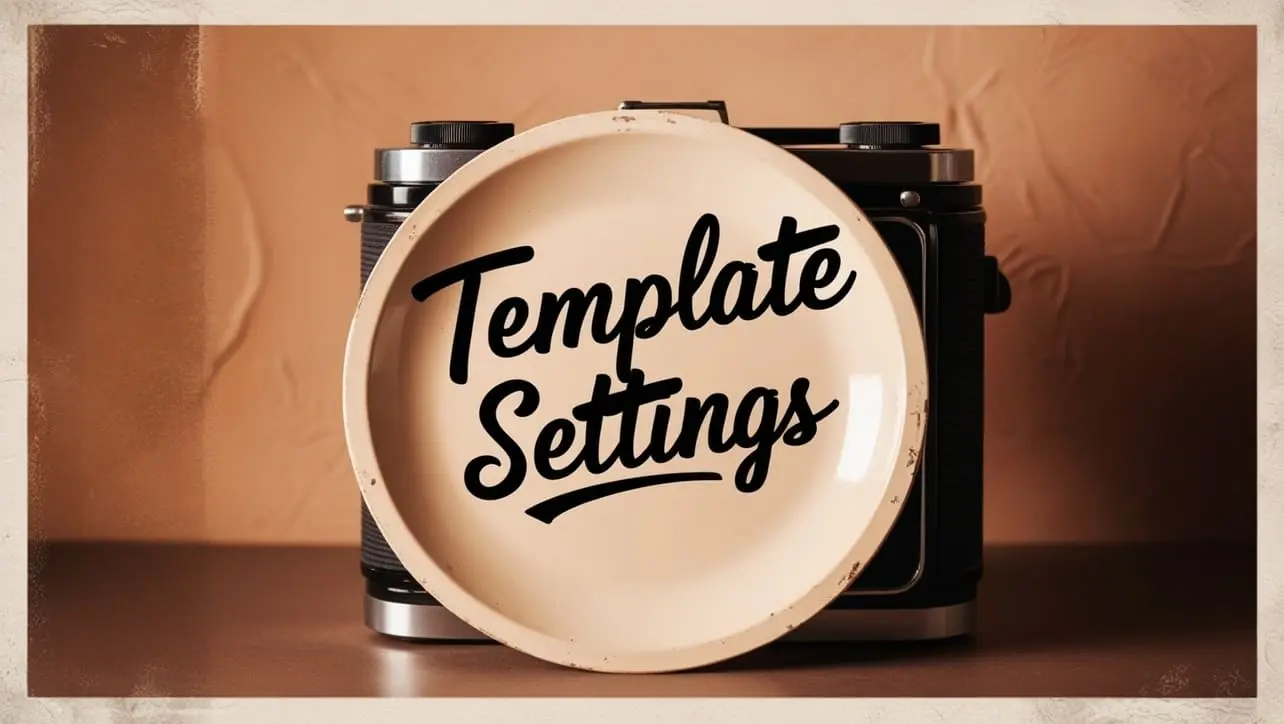
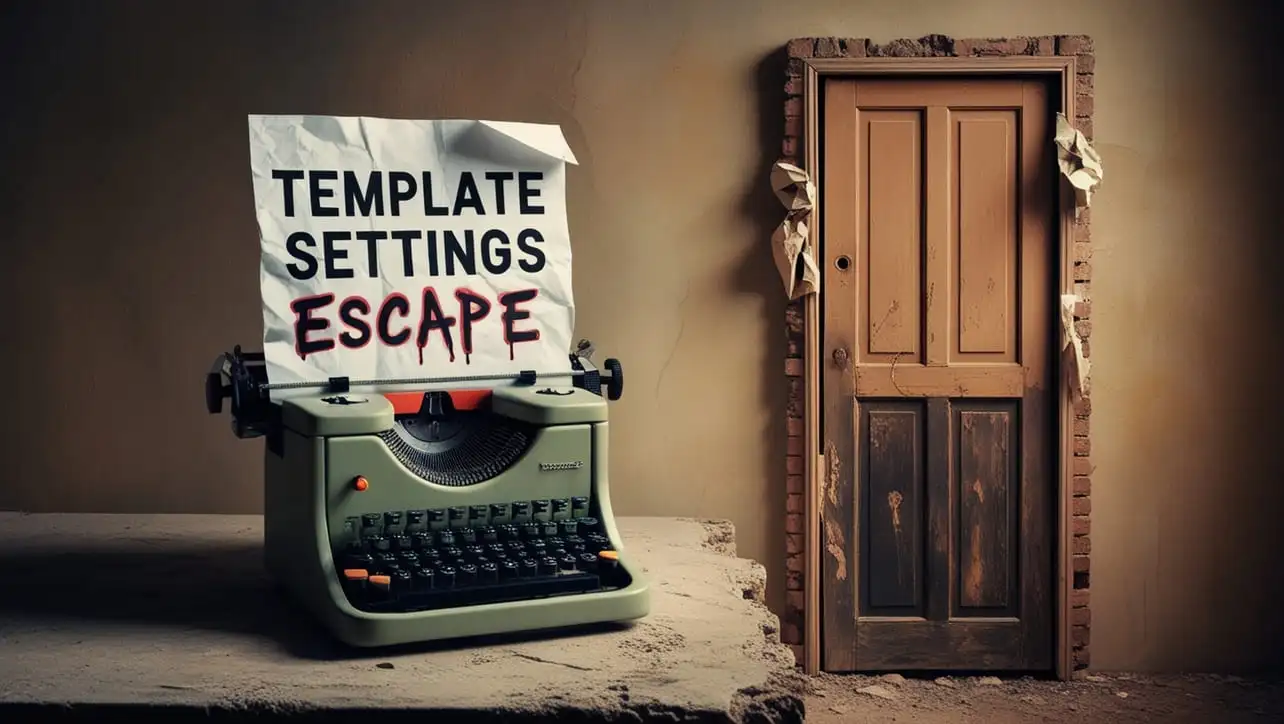
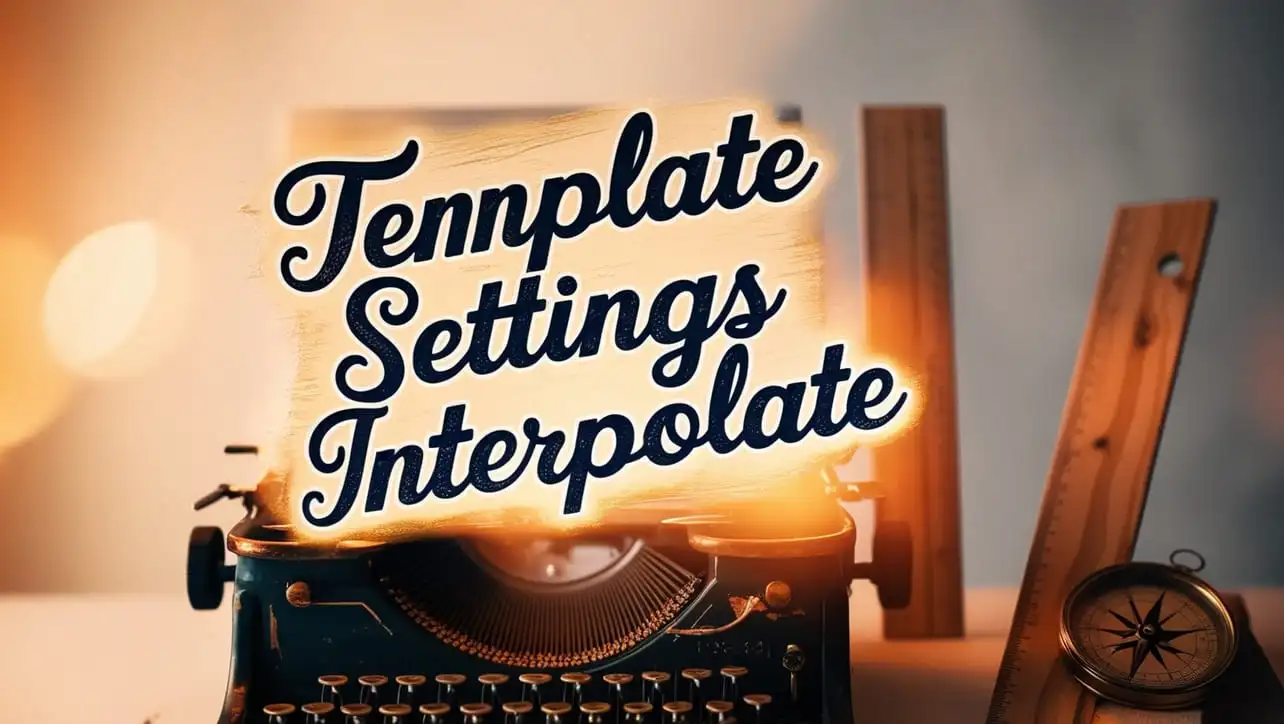
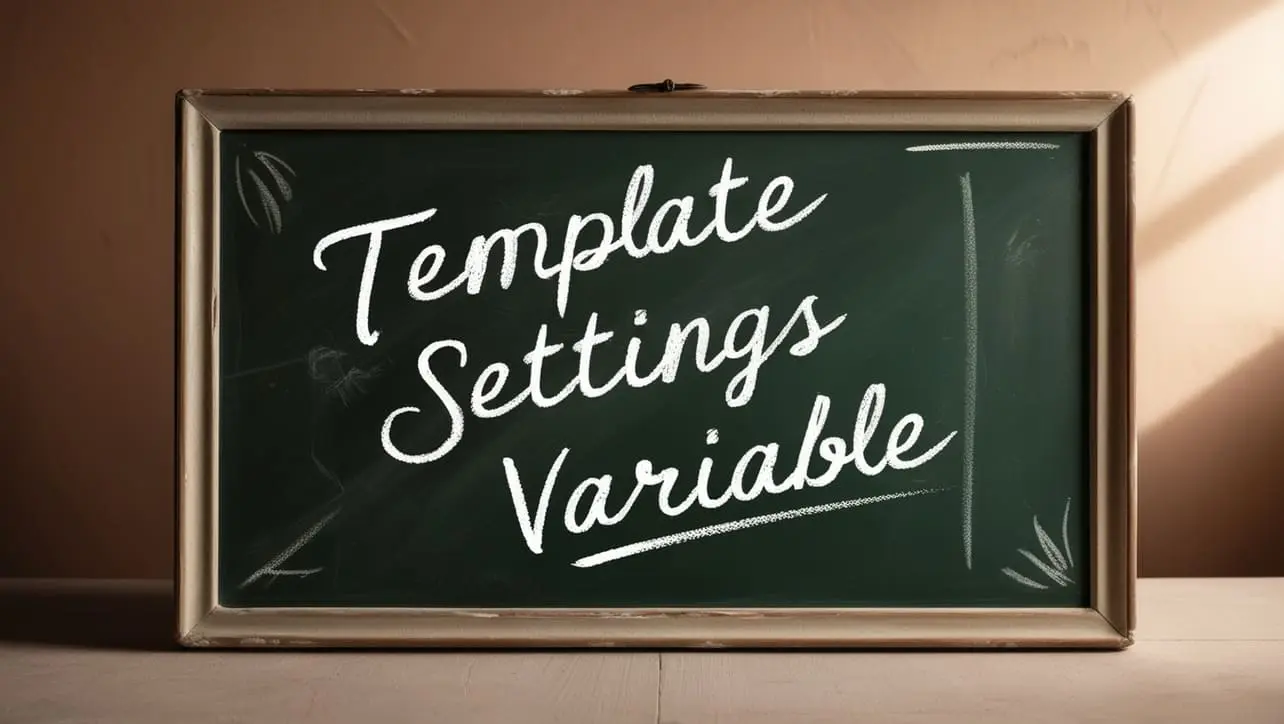
If you have any doubts regarding this article (Lodash _.isInteger() Lang Method), please comment here. I will help you immediately.