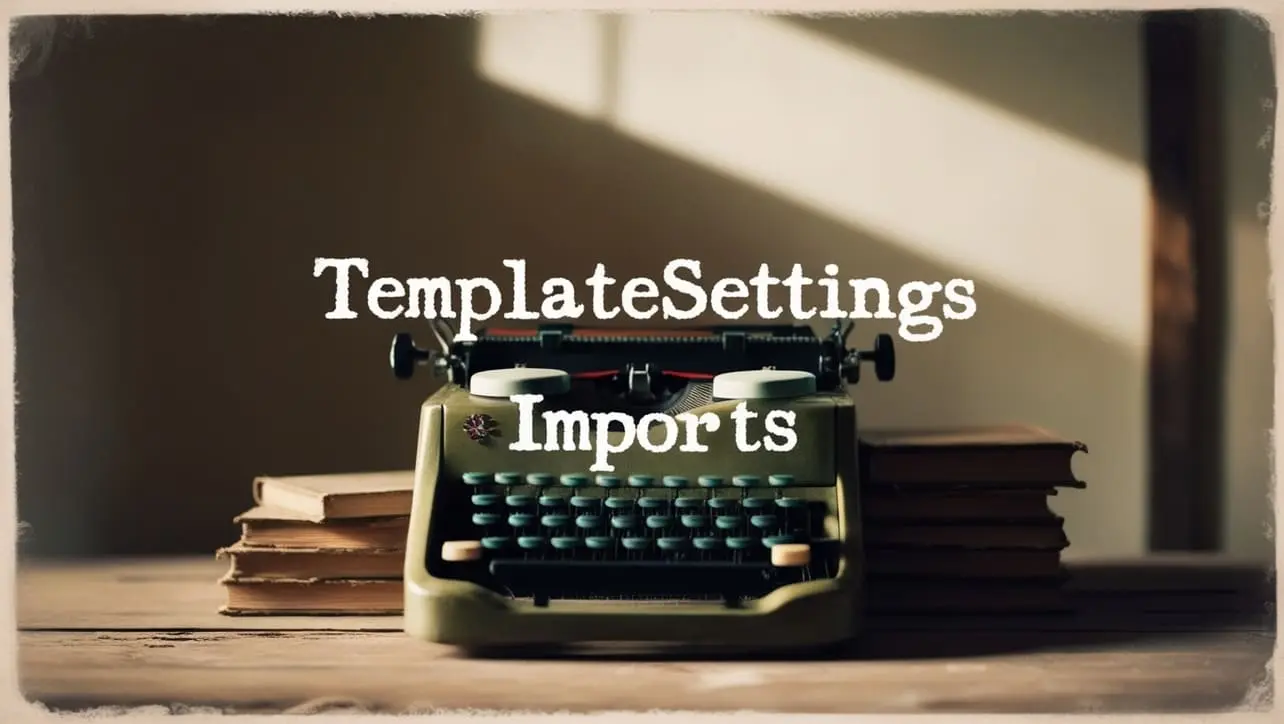
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isEqualWith() Lang Method

Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, comparing objects and values is a common task. Lodash, a comprehensive utility library, provides the _.isEqualWith()
method, a versatile tool for deep equality comparison with custom logic.
This method offers flexibility in defining how values should be compared, making it invaluable for developers dealing with complex data structures.
🧠 Understanding _.isEqualWith() Method
The _.isEqualWith()
method in Lodash performs a deep comparison between two values, allowing customization through a customizer function. This enables developers to tailor the comparison logic to accommodate specific use cases, providing a more nuanced approach to equality checking.
💡 Syntax
The syntax for the _.isEqualWith()
method is straightforward:
_.isEqualWith(value, other, customizer)
- value: The value to compare.
- other: The other value to compare.
- customizer: The function to customize comparisons.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isEqualWith()
method:
const _ = require('lodash');
const objectA = {
x: 1,
y: 2,
z: 3
};
const objectB = {
x: 1,
y: 2,
z: 4
};
const customComparison = (objA, objB, key) => {
if (key === 'z') {
return objA + 1 === objB;
}
};
const result = _.isEqualWith(objectA, objectB, customComparison);
console.log(result);
// Output: true
In this example, the custom comparison function checks if the keys are 'z' and, if so, compares objA + 1 with objB. This results in a true value, indicating equality.
🏆 Best Practices
When working with the _.isEqualWith()
method, consider the following best practices:
Custom Comparison Logic:
Leverage the power of
_.isEqualWith()
by providing a custom comparison function. This allows you to define specific rules for certain key-value pairs during the equality check.example.jsCopiedconst customComparison = (objA, objB, key) => { if (key === 'date') { // Custom comparison for date values return new Date(objA).getTime() === new Date(objB).getTime(); } }; const result = _.isEqualWith({ date: '2022-01-01' }, { date: '2022-01-01' }, customComparison); console.log(result); // Output: true
Handling Special Cases:
Use
_.isEqualWith()
to handle special cases or edge scenarios where the default equality comparison might not suffice. This ensures that your comparisons align with the specific requirements of your application.example.jsCopiedconst customComparison = (value, other) => { if (Array.isArray(value) && Array.isArray(other)) { // Custom comparison for arrays return value.length === other.length; } }; const result = _.isEqualWith([1, 2, 3], [4, 5, 6], customComparison); console.log(result); // Output: true
Deep Comparison Control:
Take advantage of the customizer function to control the depth of the comparison. You can choose to perform a shallow comparison or extend it to deeper levels based on your specific requirements.
example.jsCopiedconst customComparison = (value, other, key, stack) => { // Perform a deep comparison only for certain keys if (key === 'nestedObject') { return _.isEqualWith(value, other, customComparison); } }; const result = _.isEqualWith( { nestedObject: { a: 1, b: 2 } }, { nestedObject: { a: 1, b: 2 } }, customComparison ); console.log(result); // Output: true
📚 Use Cases
Deep Object Comparison:
_.isEqualWith()
is ideal for deep object comparison, allowing you to customize how specific properties are compared. This is especially useful when dealing with nested objects with varying structures.example.jsCopiedconst objectA = { name: 'John', address: { city: 'New York', zip: '10001' } }; const objectB = { name: 'John', address: { city: 'New York', zip: '10002' } }; const customComparison = (objA, objB, key) => { if (key === 'zip') { return objA.startsWith(objB.substring(0, 3)); // Custom zip code comparison } }; const result = _.isEqualWith(objectA, objectB, customComparison); console.log(result); // Output: true
Handling Complex Data Structures:
When dealing with complex data structures like arrays of objects,
_.isEqualWith()
provides a way to define custom rules for equality, ensuring accurate comparisons.example.jsCopiedconst arrayA = [{ id: 1, value: 'apple' }, { id: 2, value: 'banana' }]; const arrayB = [{ id: 1, value: 'apple' }, { id: 2, value: 'orange' }]; const customComparison = (objA, objB, key) => { if (key === 'value') { return objA.charAt(0) === objB.charAt(0); // Custom comparison for the first character } }; const result = _.isEqualWith(arrayA, arrayB, customComparison); console.log(result); // Output: true
Flexible Value Comparison:
Customize the comparison logic to handle diverse value types, ensuring that the equality check aligns with the unique characteristics of your data.
example.jsCopiedconst customComparison = (value, other) => { if (typeof value === 'string' && typeof other === 'string') { // Custom comparison for string values return value.toLowerCase() === other.toLowerCase(); } }; const result = _.isEqualWith('Hello', 'hello', customComparison); console.log(result); // Output: true
🎉 Conclusion
The _.isEqualWith()
method in Lodash empowers developers to perform deep equality comparisons with a high degree of customization. By providing a custom comparison function, you can tailor the equality check to handle specific cases and nuances in your data. Whether dealing with nested objects, complex data structures, or unique value comparisons, _.isEqualWith()
offers a flexible and powerful solution.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isEqualWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
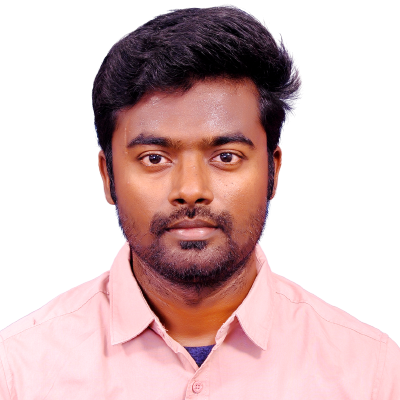
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
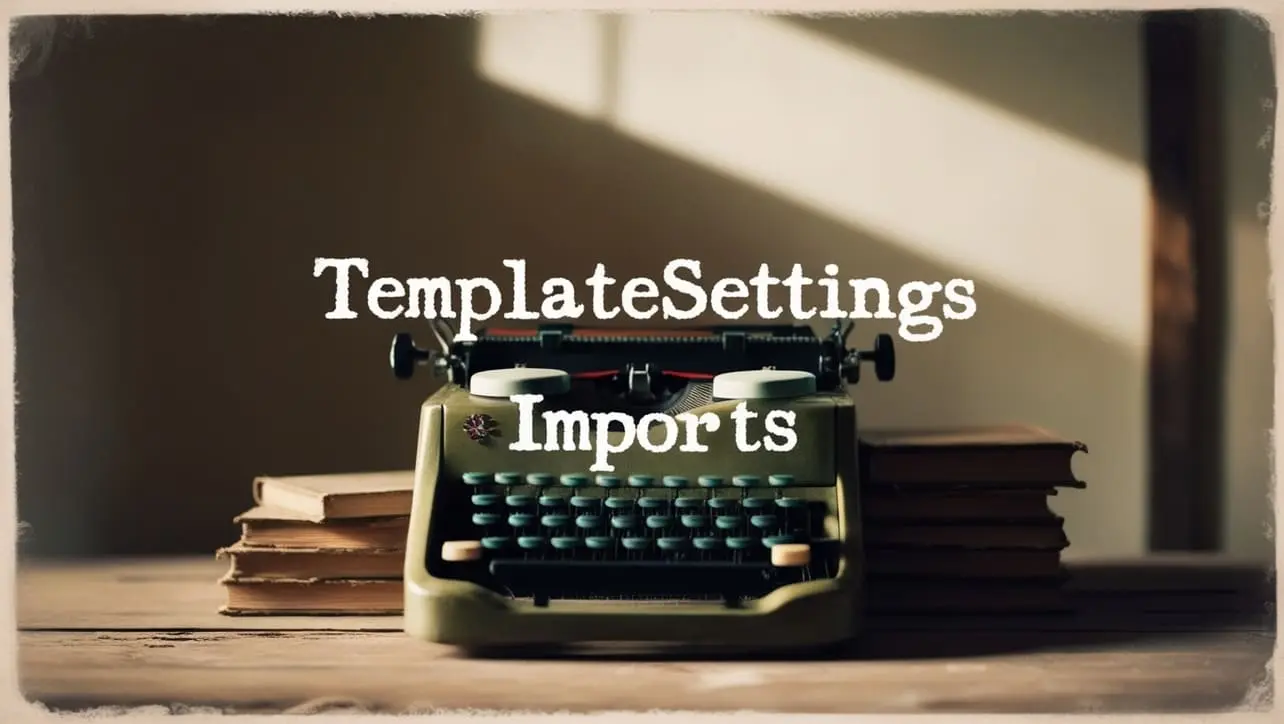
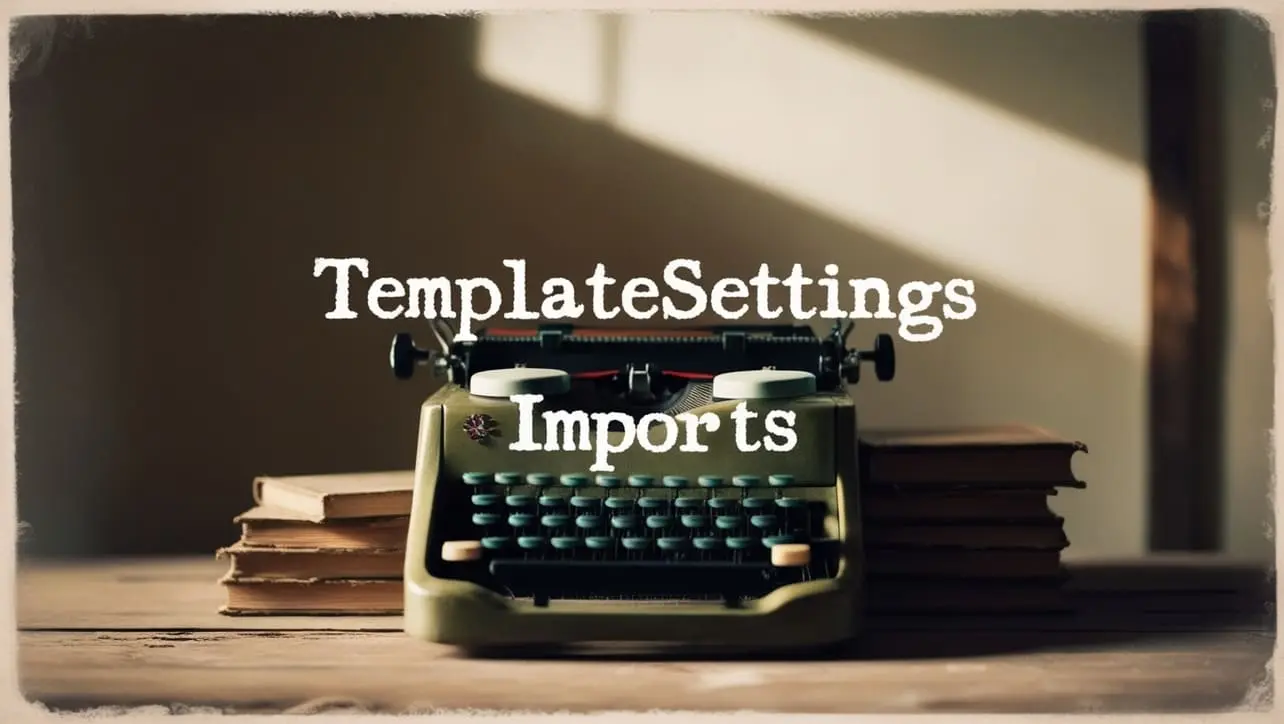
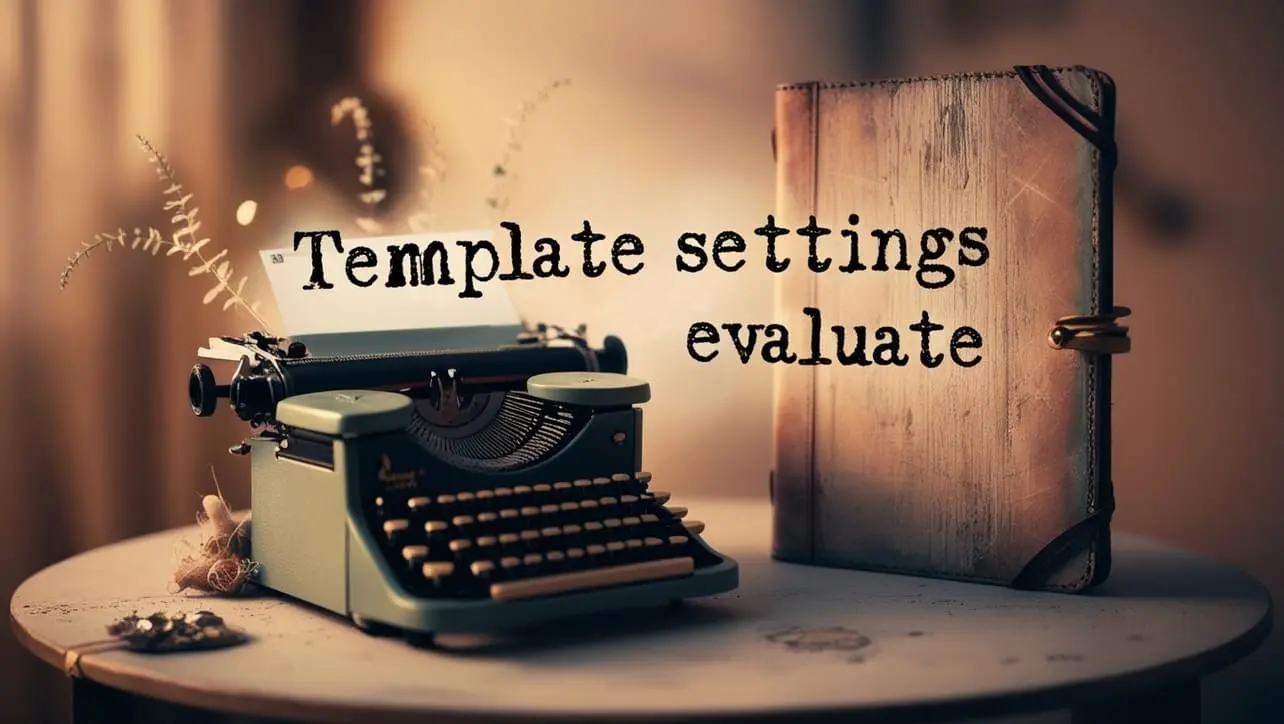
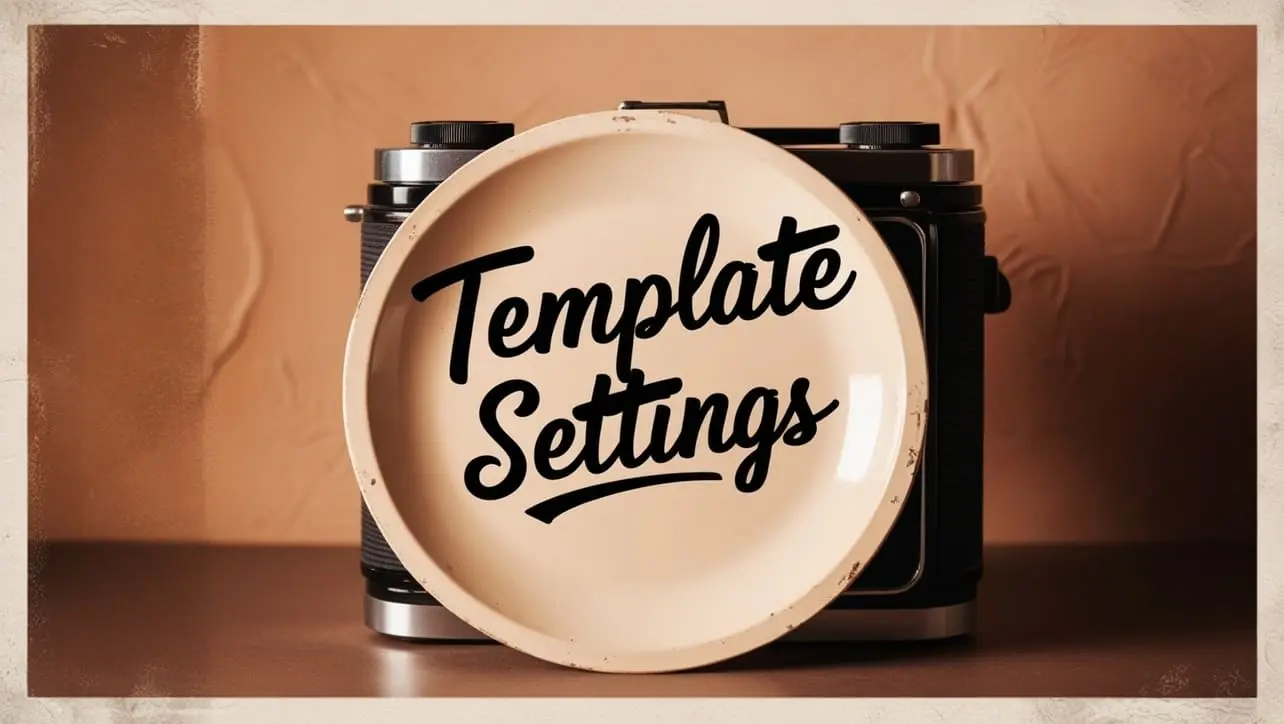
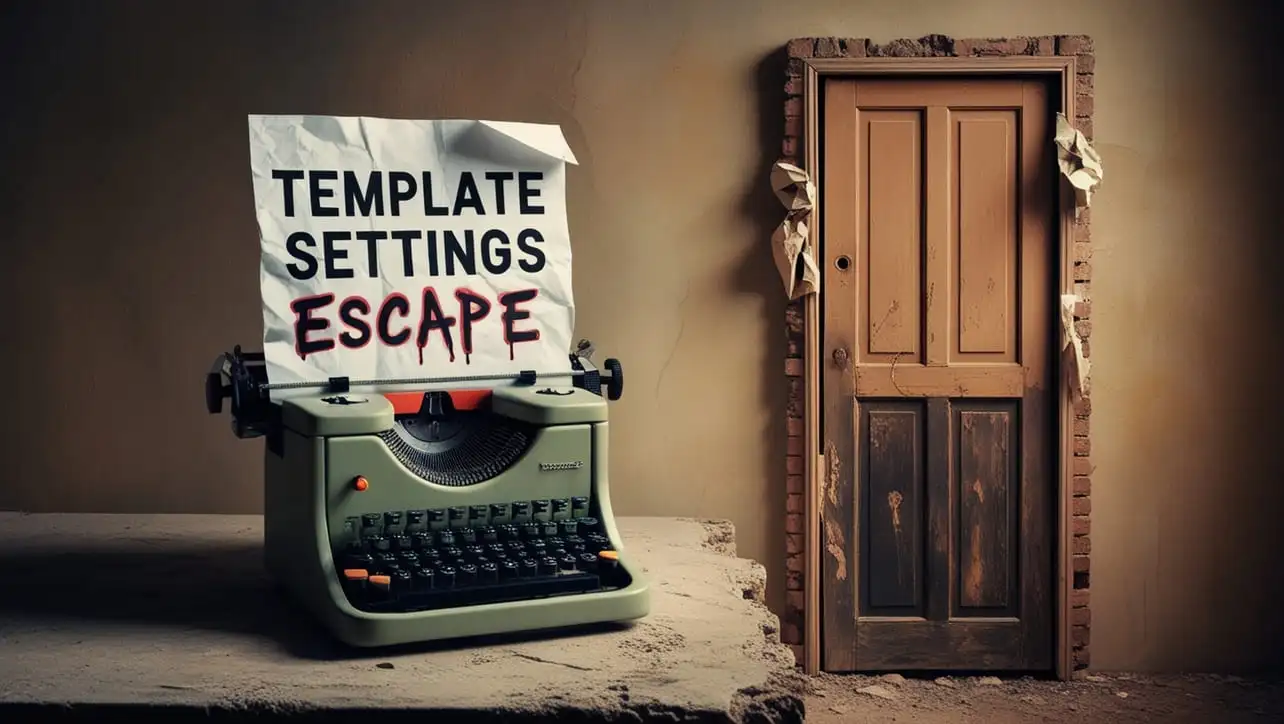
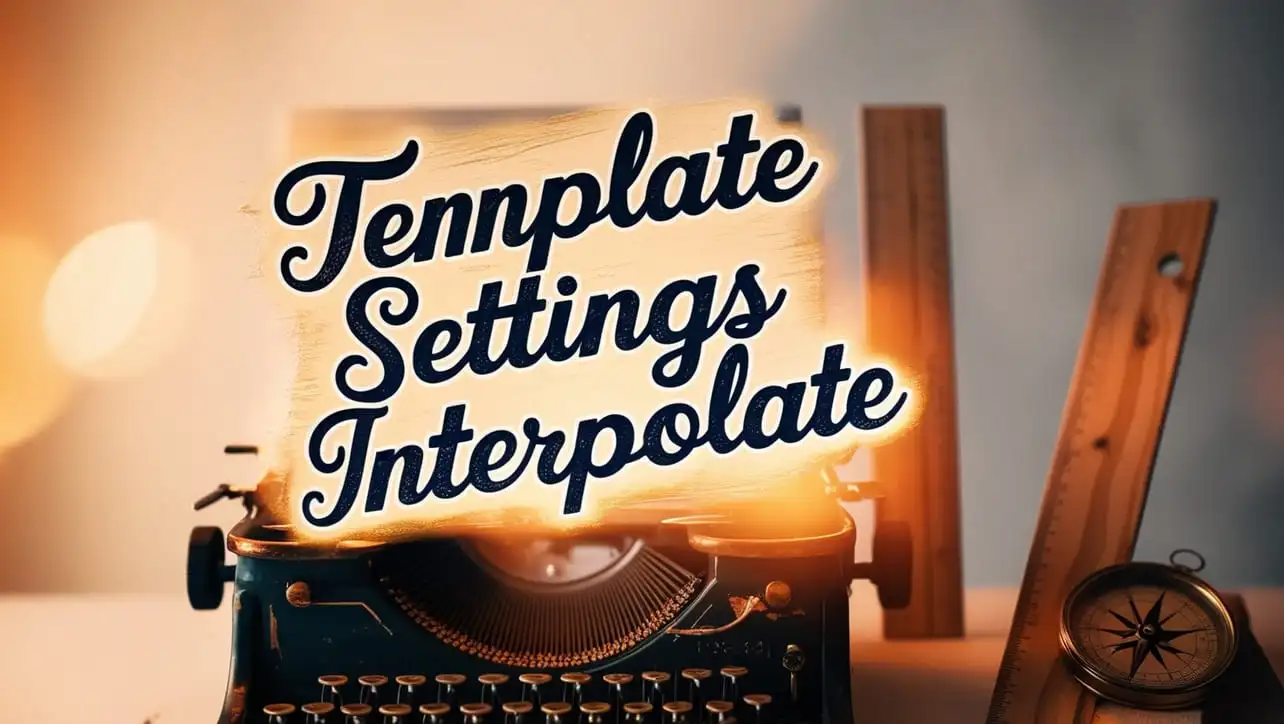
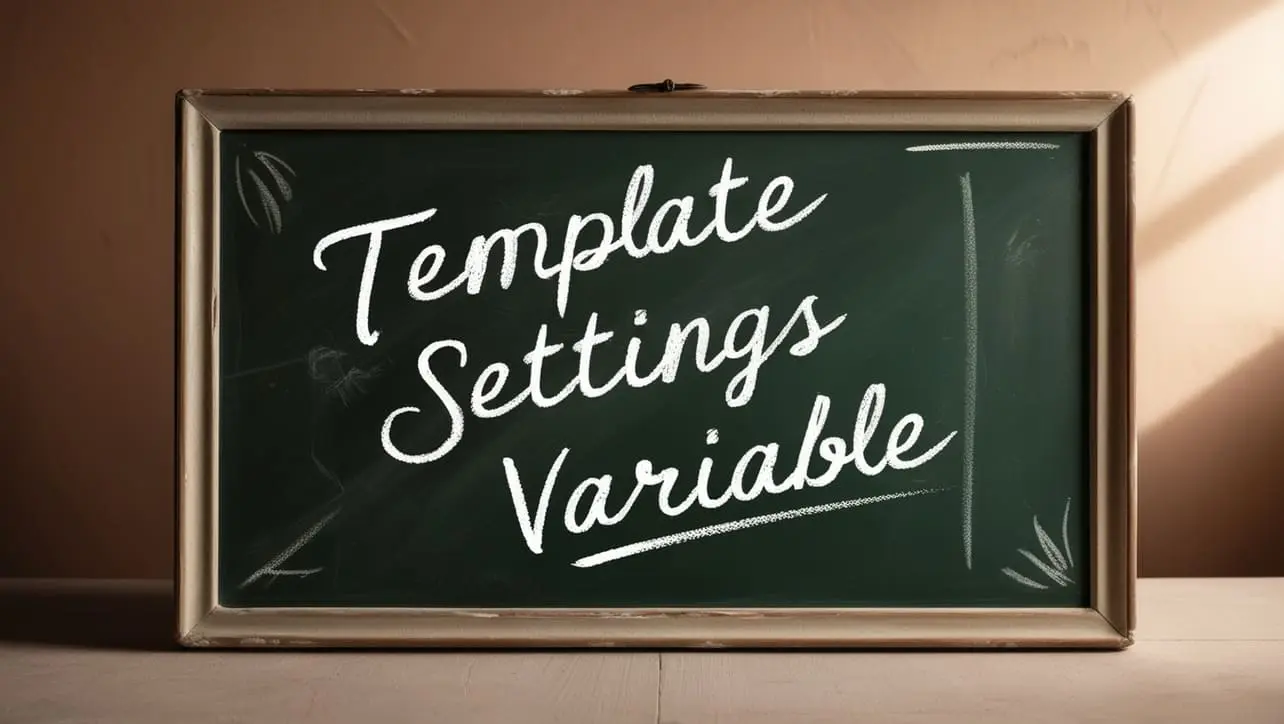
If you have any doubts regarding this article (Lodash _.isEqualWith() Lang Method), please comment here. I will help you immediately.