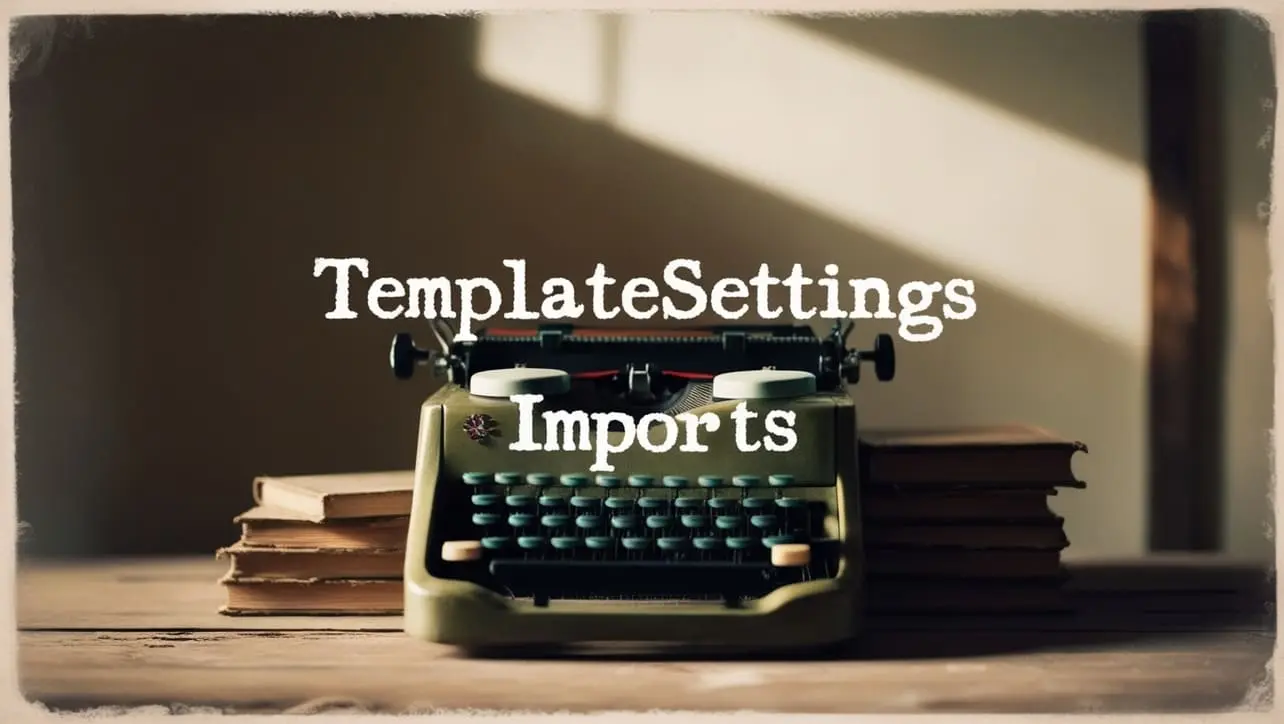
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isElement() Lang Method
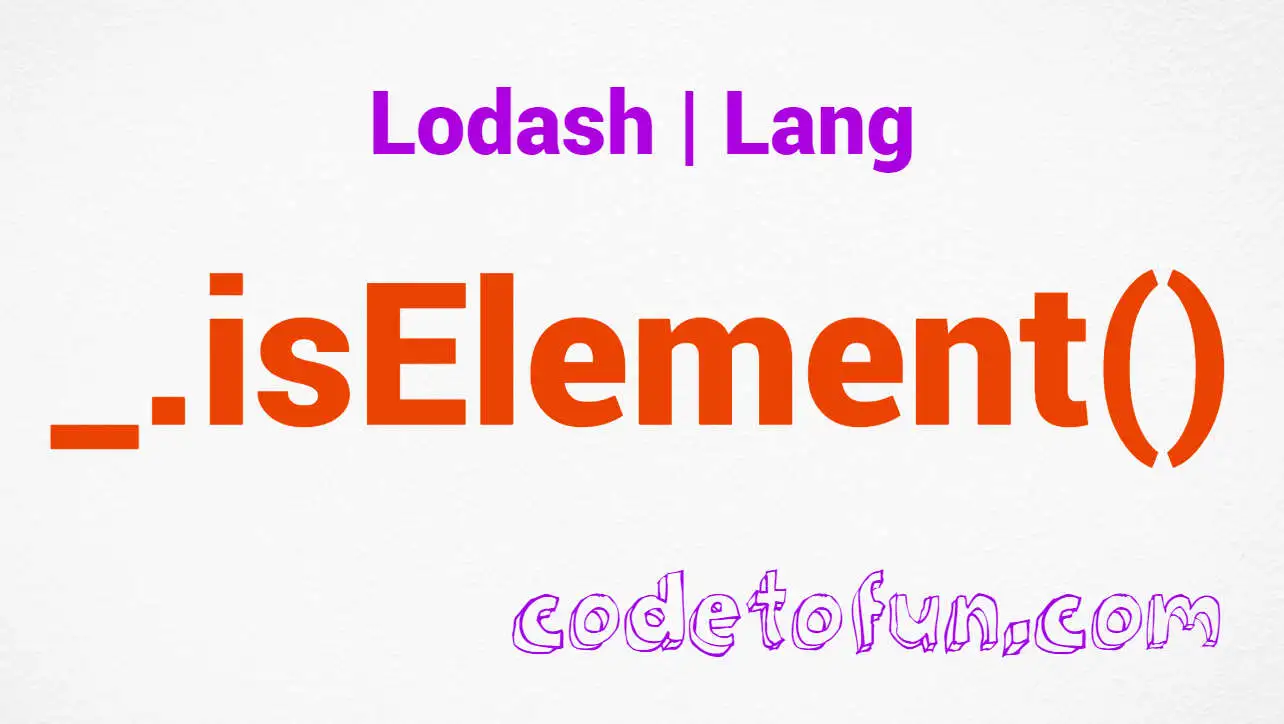
Photo Credit to CodeToFun
🙋 Introduction
n the diverse landscape of JavaScript programming, robust type-checking is essential for ensuring the integrity of data and improving code reliability. Enter Lodash, a comprehensive utility library that provides a range of functions to simplify common programming tasks. Among these functions is the _.isElement()
method, a valuable tool for checking whether a given value is a DOM element.
This method enhances the precision of type-checking, making it an indispensable asset for developers dealing with the complexities of the Document Object Model (DOM).
🧠 Understanding _.isElement() Method
The _.isElement()
method in Lodash is designed to determine whether a given value is a DOM element. This is particularly useful when working with dynamically generated content, handling user inputs, or validating data received from external sources.
💡 Syntax
The syntax for the _.isElement()
method is straightforward:
_.isElement(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isElement()
method:
const _ = require('lodash');
const validElement = document.createElement('div');
const isValid = _.isElement(validElement);
console.log(isValid);
// Output: true
In this example, validElement is a dynamically created DOM element, and _.isElement()
confirms its status as a valid DOM element.
🏆 Best Practices
When working with the _.isElement()
method, consider the following best practices:
Validate Against False Positives:
Be mindful of potential false positives when using
_.isElement()
. Ensure that the value being checked is genuinely intended to be a DOM element and not a different object or data type.example.jsCopiedconst nonElementValue = 'I am not an element'; const isValidElement = _.isElement(nonElementValue); console.log(isValidElement); // Output: false
Handle Dynamic DOM Elements:
When working with dynamically generated content, use
_.isElement()
to validate elements before manipulating them. This helps prevent errors and enhances the robustness of your code.example.jsCopiedconst dynamicallyGeneratedElement = /* ...generate or fetch a DOM element dynamically... */; if (_.isElement(dynamicallyGeneratedElement)) { // Perform operations on the valid DOM element console.log('Valid DOM element:', dynamicallyGeneratedElement); } else { console.error('Invalid DOM element:', dynamicallyGeneratedElement); }
Combine with Other Type-Checking:
For comprehensive type-checking, consider combining
_.isElement()
with other Lodash type-checking methods. This approach ensures a more thorough validation process.example.jsCopiedconst elementOrArray = document.createElement('div'); const isValidElementOrArray = _.isElement(elementOrArray) || _.isArray(elementOrArray); console.log(isValidElementOrArray); // Output: true
📚 Use Cases
Event Handling:
When dealing with event listeners or custom event handling, use
_.isElement()
to verify that the target of the event is indeed a DOM element before performing any operations.example.jsCopieddocument.addEventListener('click', event => { if (_.isElement(event.target)) { console.log('Clicked on a valid DOM element:', event.target); } });
User Input Validation:
In scenarios involving user inputs, especially in frameworks with reactive data binding, use
_.isElement()
to validate whether the input corresponds to a valid DOM element.example.jsCopiedconst userInput = /* ...get user input from a form or user interaction... */; if (_.isElement(userInput)) { console.log('Valid DOM element from user input:', userInput); } else { console.error('Invalid input, not a DOM element:', userInput); }
Integration with Frameworks:
When working with JavaScript frameworks or libraries that manipulate the DOM, leverage
_.isElement()
to ensure compatibility and validate the elements being manipulated.example.jsCopiedconst frameworkGeneratedElement = /* ...generate or manipulate a DOM element using a framework... */; if (_.isElement(frameworkGeneratedElement)) { console.log('Valid DOM element from the framework:', frameworkGeneratedElement); } else { console.error('Invalid DOM element from the framework:', frameworkGeneratedElement); }
🎉 Conclusion
The _.isElement()
method in Lodash provides a reliable and efficient way to check whether a value is a DOM element. By incorporating this method into your projects, you can enhance the precision of type-checking, leading to more robust and error-resistant JavaScript code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isElement()
method in your Lodash projects.
👨💻 Join our Community:
Author
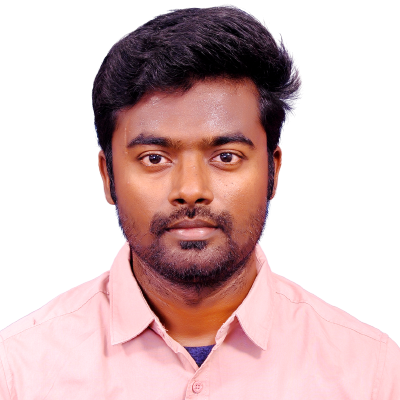
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
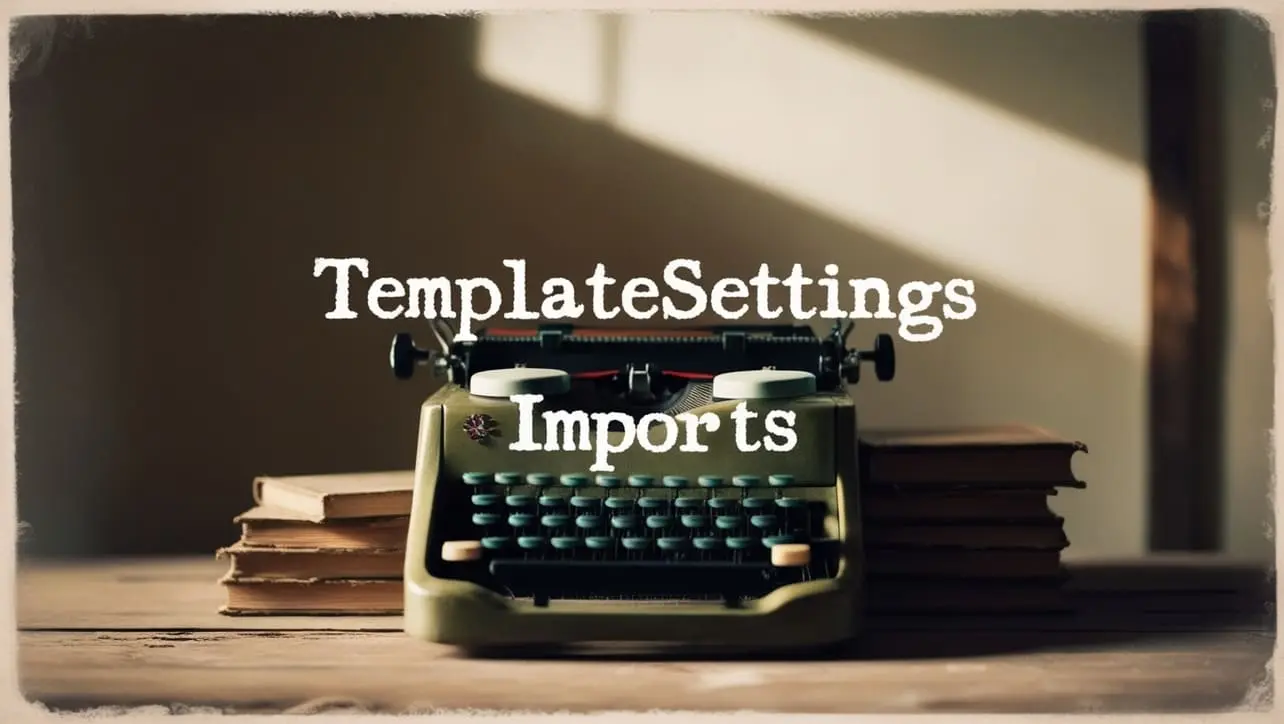
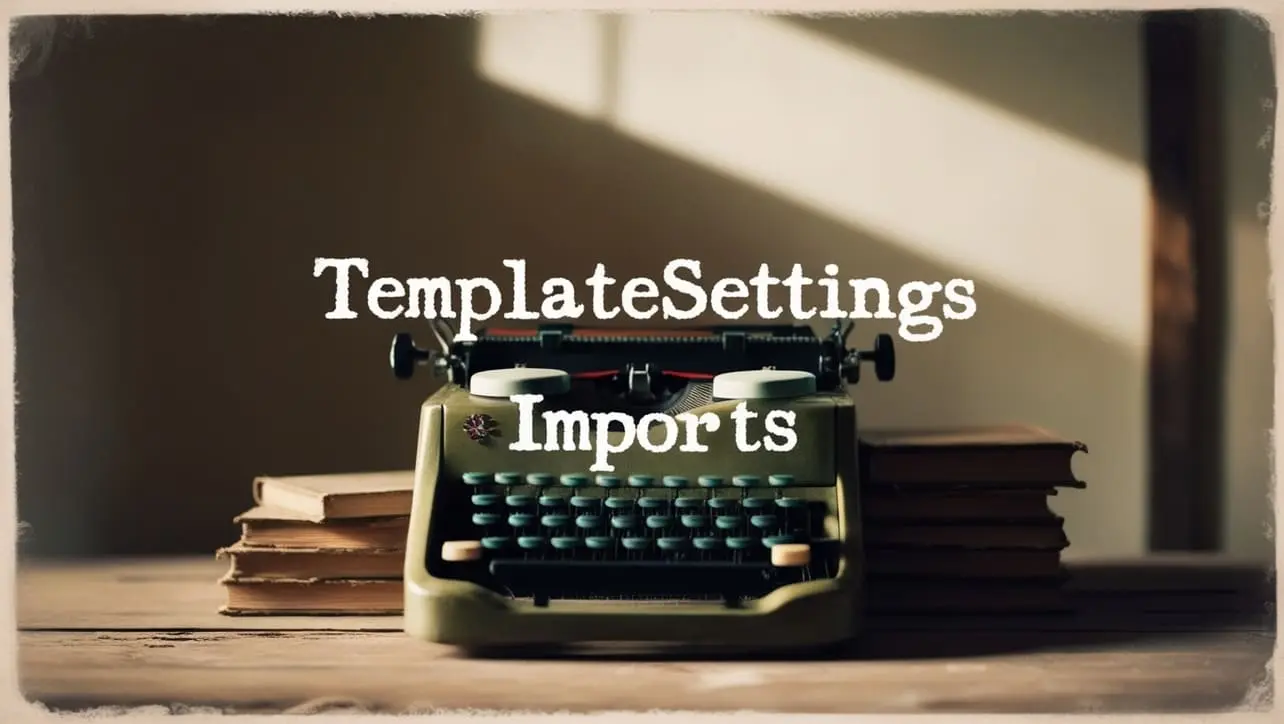
Lodash _.templateSettings.imports Property
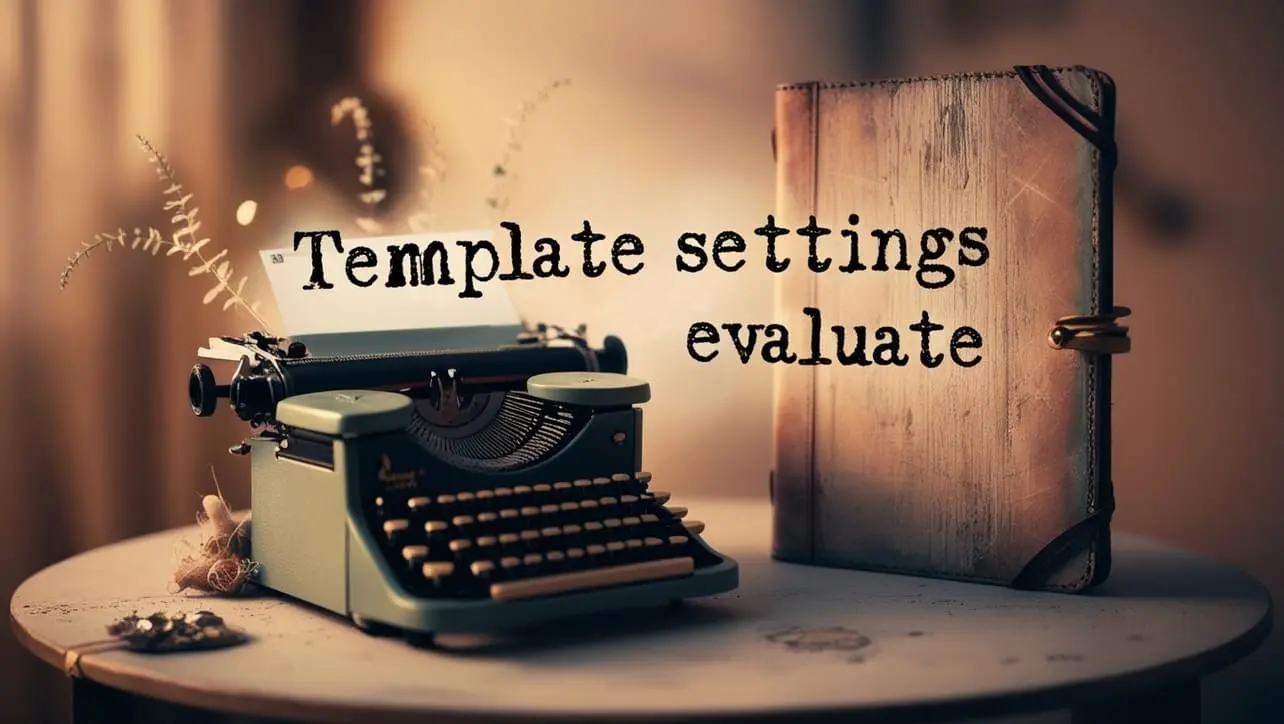
Lodash _.templateSettings.evaluate Property
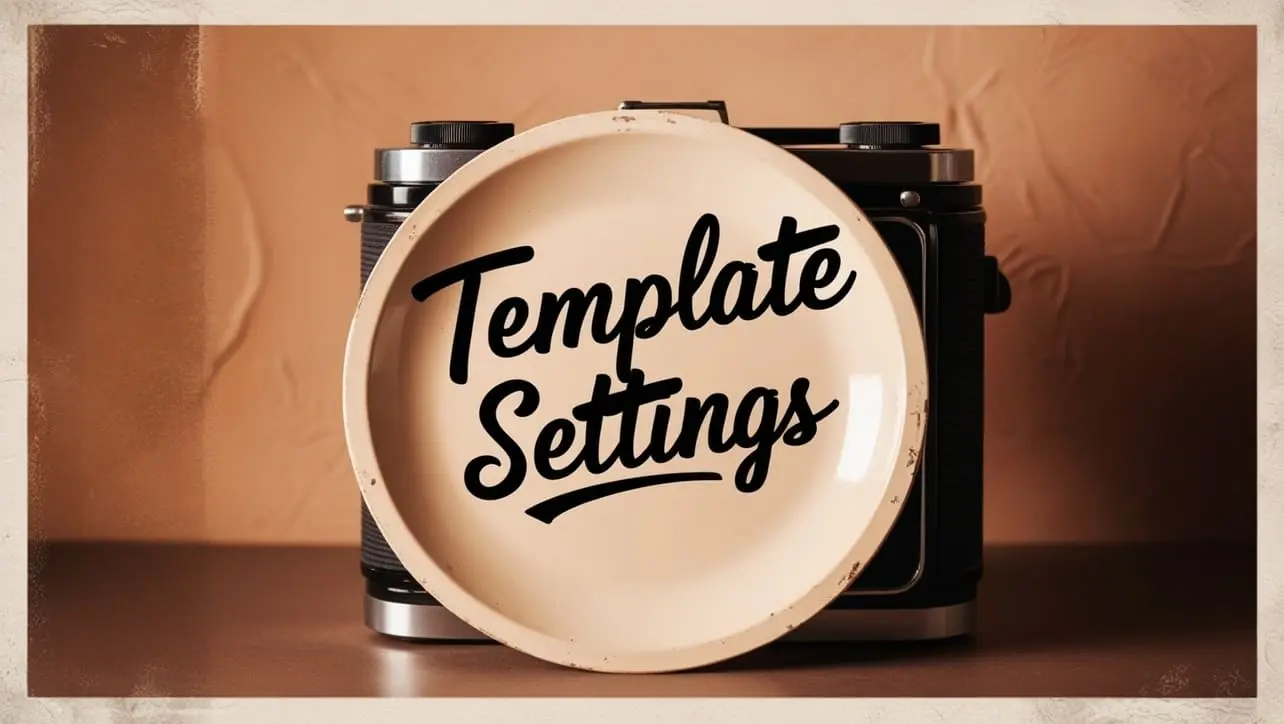
Lodash _.templateSettings Property
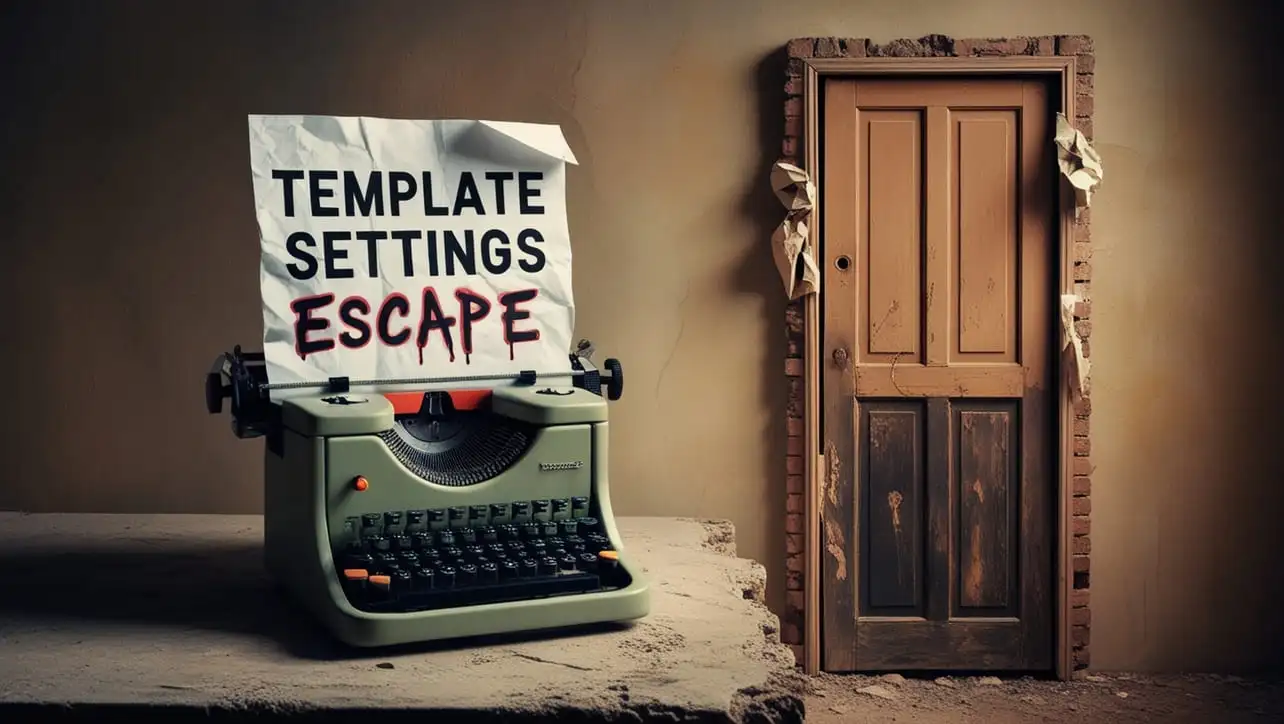
Lodash _.templateSettings.escape Property
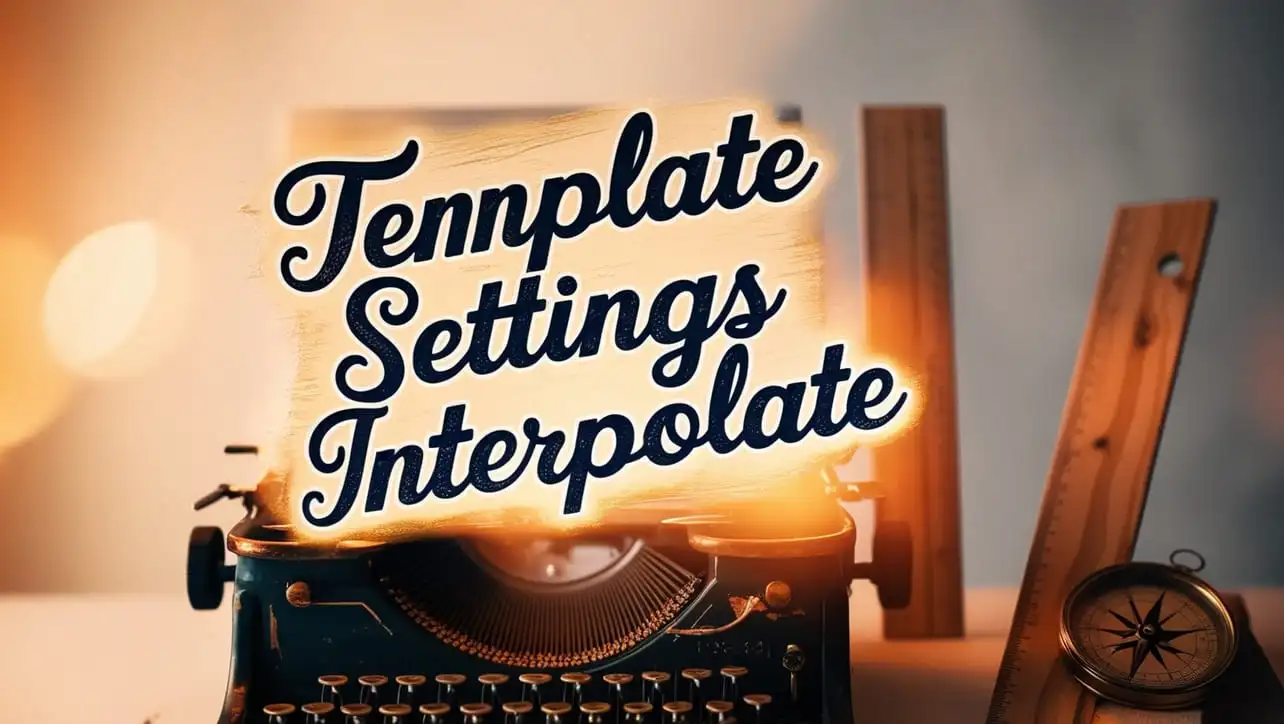
Lodash _.templateSettings.interpolate Property
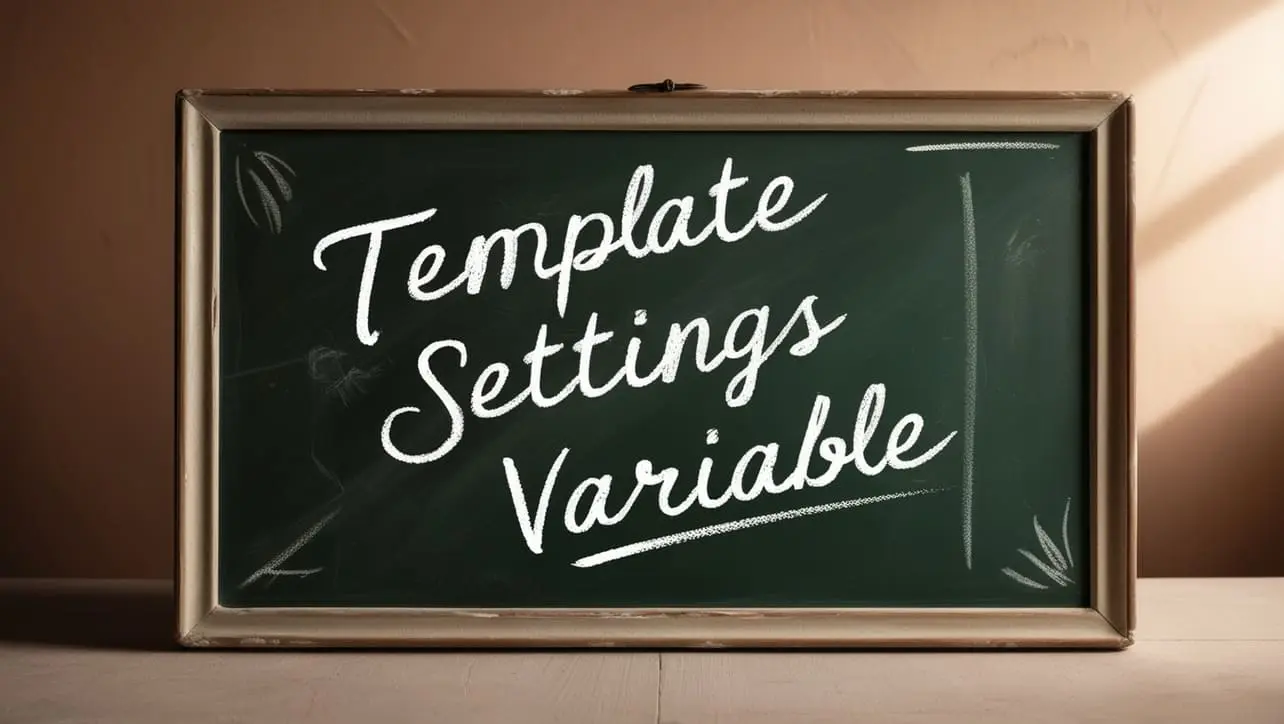
If you have any doubts regarding this article (Lodash _.isElement() Lang Method), please comment here. I will help you immediately.