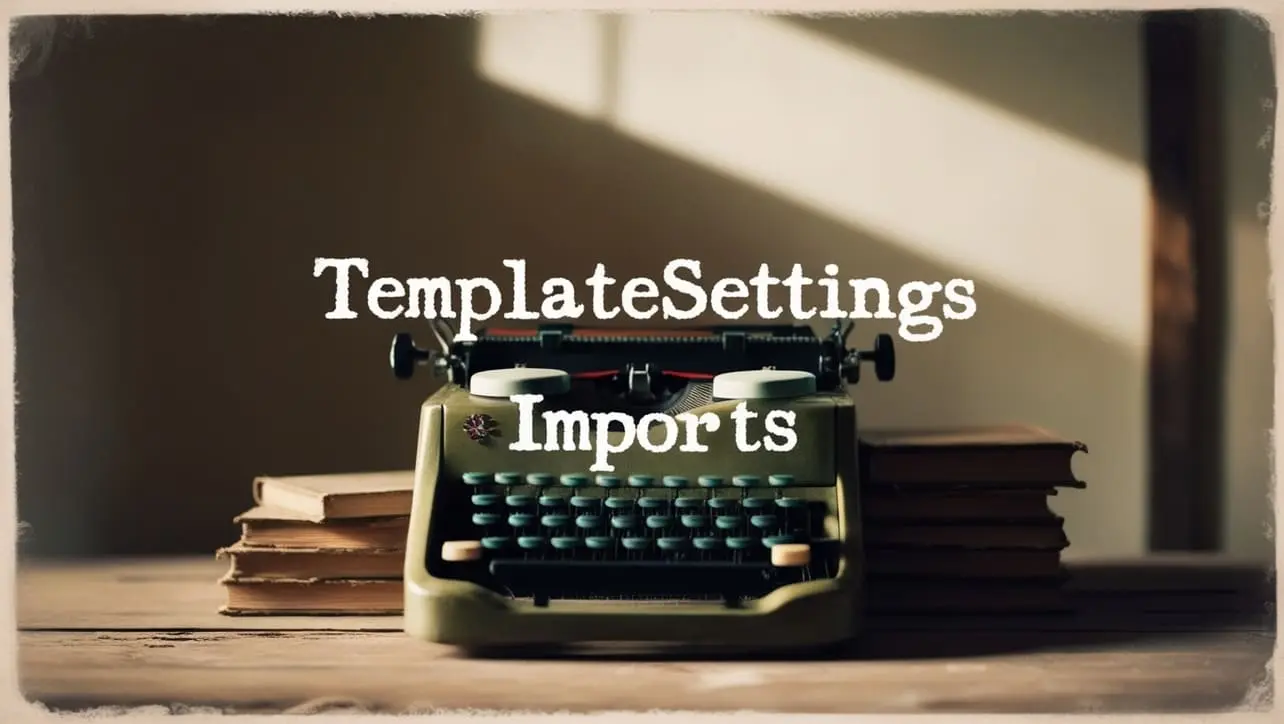
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isDate() Lang Method
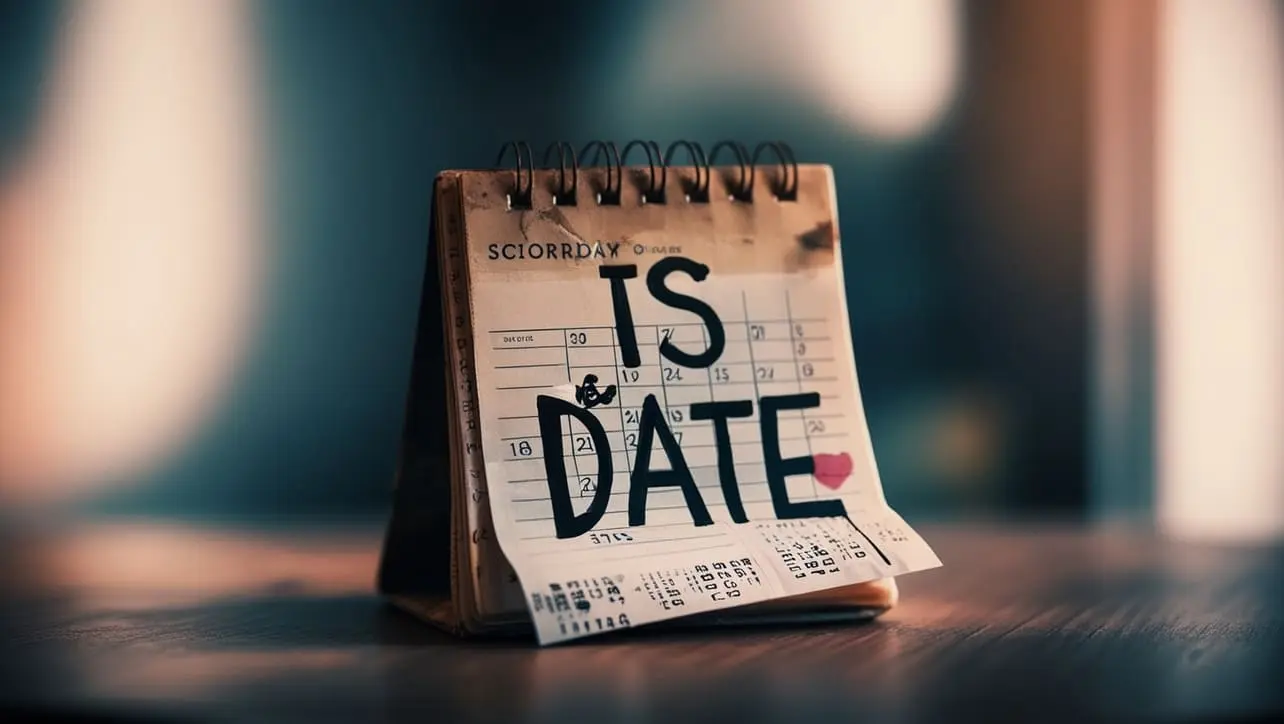
Photo Credit to CodeToFun
🙋 Introduction
When working with date and time data in JavaScript, it's essential to have reliable methods for type checking. Lodash, a versatile utility library, provides the _.isDate()
method as part of its Lang (language) module.
This method allows developers to easily determine whether a given value is a JavaScript Date object, ensuring accurate handling of date-related operations.
🧠 Understanding _.isDate() Method
The _.isDate()
method in Lodash checks if a value is of type Date. This is particularly useful when dealing with user input, API responses, or other sources where data types may not be guaranteed.
💡 Syntax
The syntax for the _.isDate()
method is straightforward:
_.isDate(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isDate()
method:
const _ = require('lodash');
const dateObject = new Date();
const isDate = _.isDate(dateObject);
console.log(isDate);
// Output: true
In this example, the dateObject is checked using _.isDate()
, and the result confirms that it is indeed a Date object.
🏆 Best Practices
When working with the _.isDate()
method, consider the following best practices:
Type Checking:
Use
_.isDate()
for robust type checking, especially when dealing with user inputs or external data sources. This helps prevent unexpected behavior in date-related operations.example.jsCopiedconst userInput = /* ...some user input... */; const isValidDate = _.isDate(new Date(userInput)); if (isValidDate) { // Proceed with date-related operations console.log('Valid date input'); } else { console.error('Invalid date input'); }
Guard Clauses:
In functions where date objects are expected, implement guard clauses with
_.isDate()
to handle unexpected or incorrect input gracefully.example.jsCopiedfunction processDateInput(inputDate) { if (!_.isDate(inputDate)) { console.error('Invalid date input'); return; } // Proceed with date-related operations console.log('Processing date:', inputDate); } const userInputDate = new Date(/* ...some user input... */); processDateInput(userInputDate);
📚 Use Cases
Form Validation:
In scenarios where user input involves dates,
_.isDate()
can be employed for form validation to ensure that the entered value is a valid date.example.jsCopiedconst submittedDate = new Date(/* ...user-submitted date... */); if (_.isDate(submittedDate)) { // Proceed with form submission console.log('Date is valid. Submitting form...'); } else { console.error('Invalid date. Please enter a valid date.'); }
API Response Handling:
When interacting with external APIs that return date-related data, use
_.isDate()
to validate the received data types before further processing.example.jsCopiedconst apiResponse = /* ...data received from an API... */; const apiDate = new Date(apiResponse.date); if (_.isDate(apiDate)) { // Proceed with handling the API response console.log('API date:', apiDate); } else { console.error('Invalid date format in API response'); }
🎉 Conclusion
The _.isDate()
method in Lodash is a valuable tool for JavaScript developers, providing a straightforward way to perform type checks on date objects. Whether you're validating user input, handling API responses, or implementing guard clauses, this method enhances the reliability of your date-related operations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isDate()
method in your Lodash projects.
👨💻 Join our Community:
Author
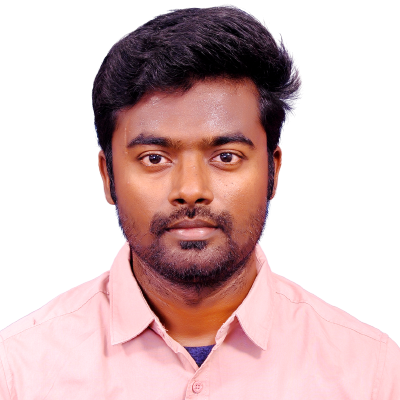
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
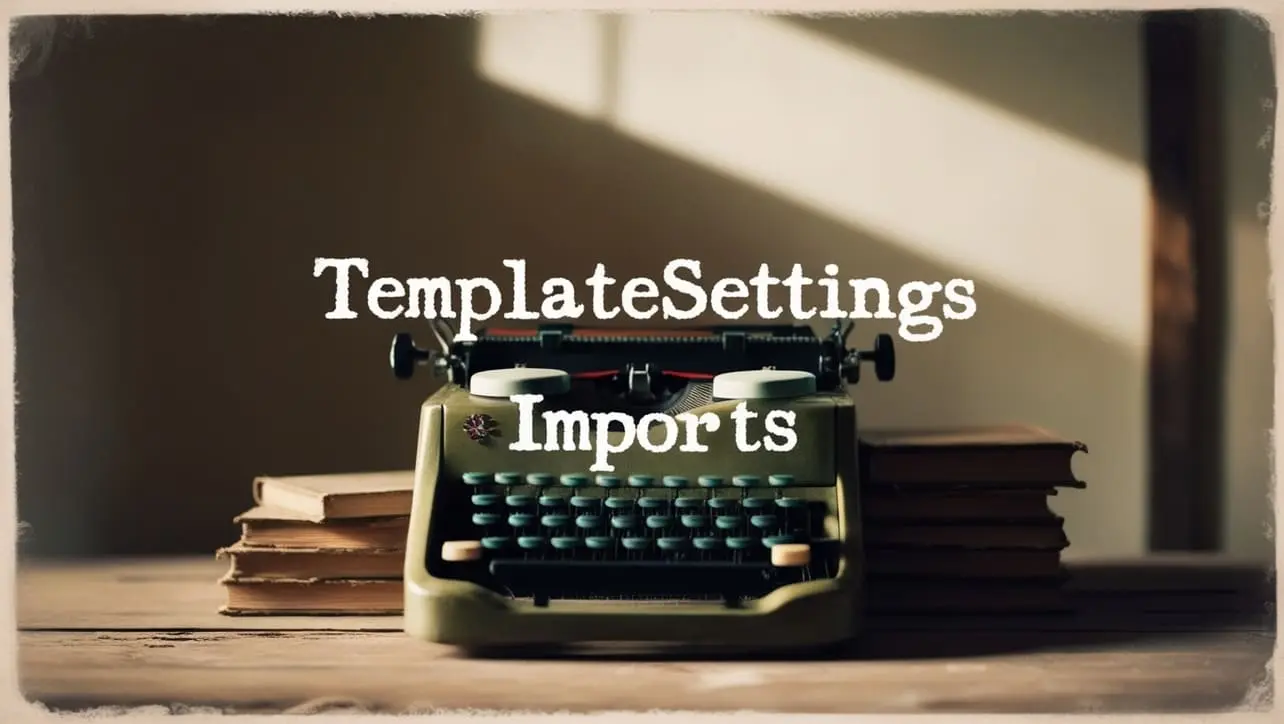
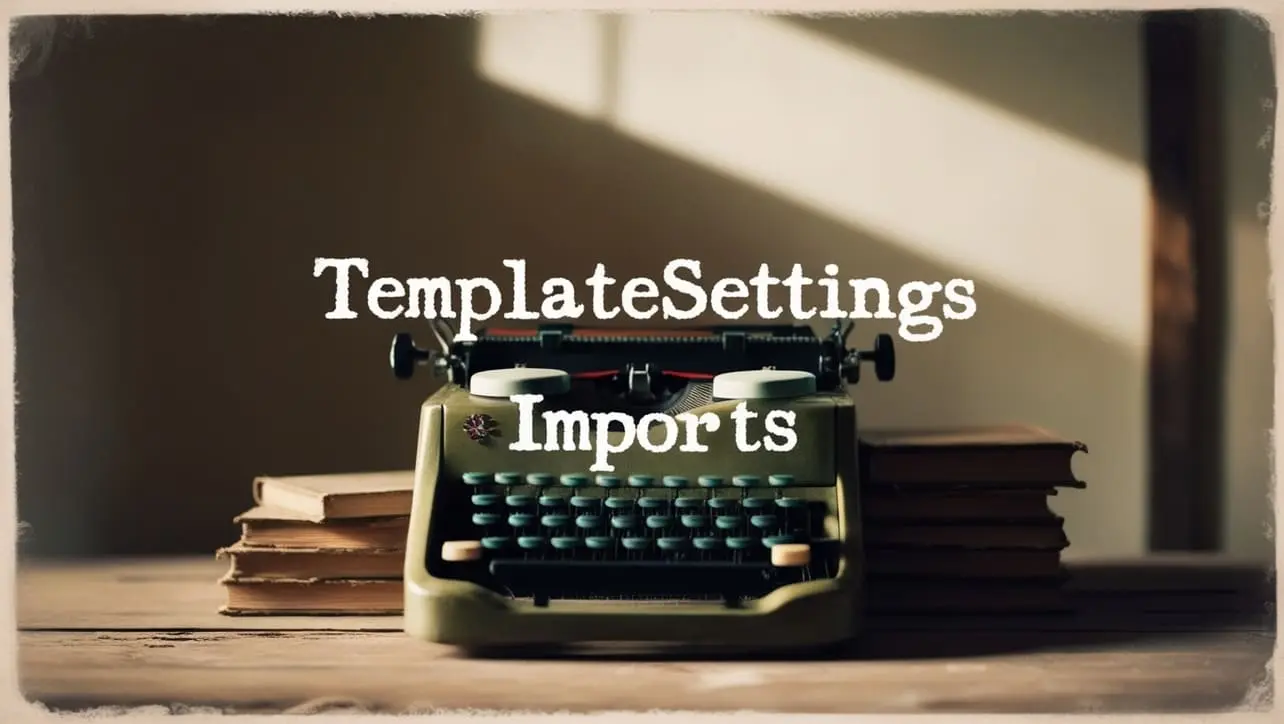
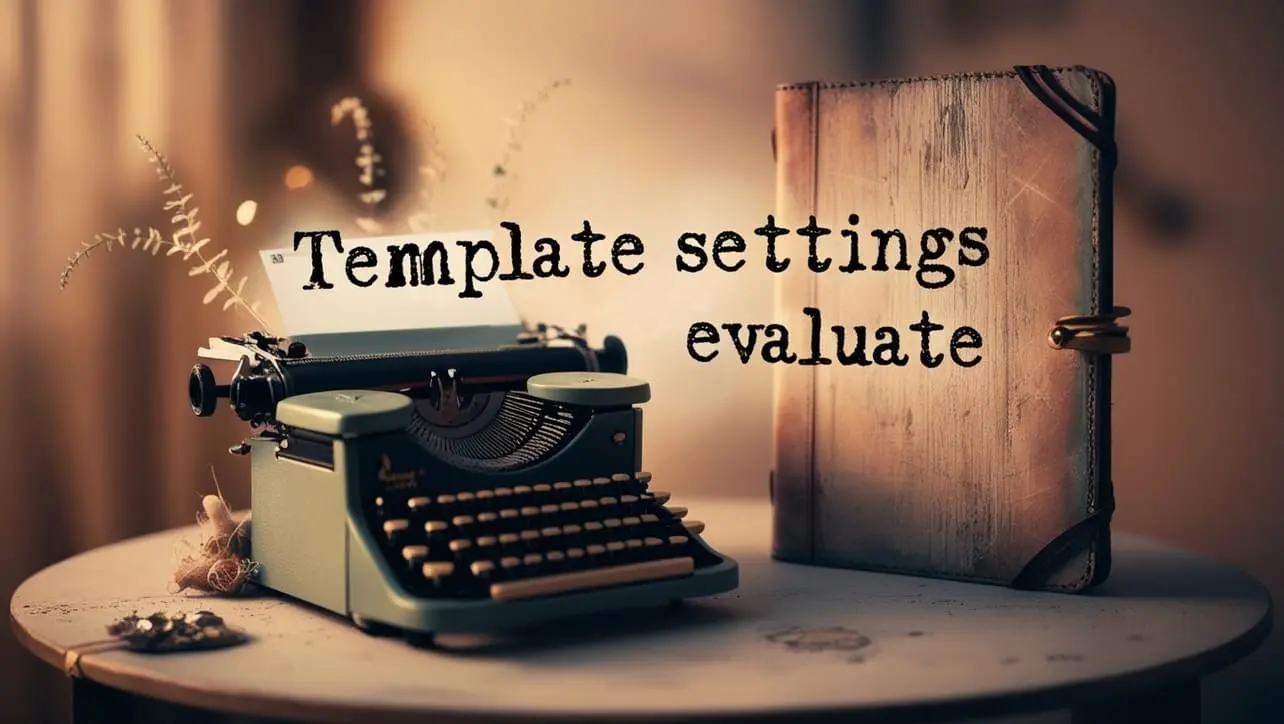
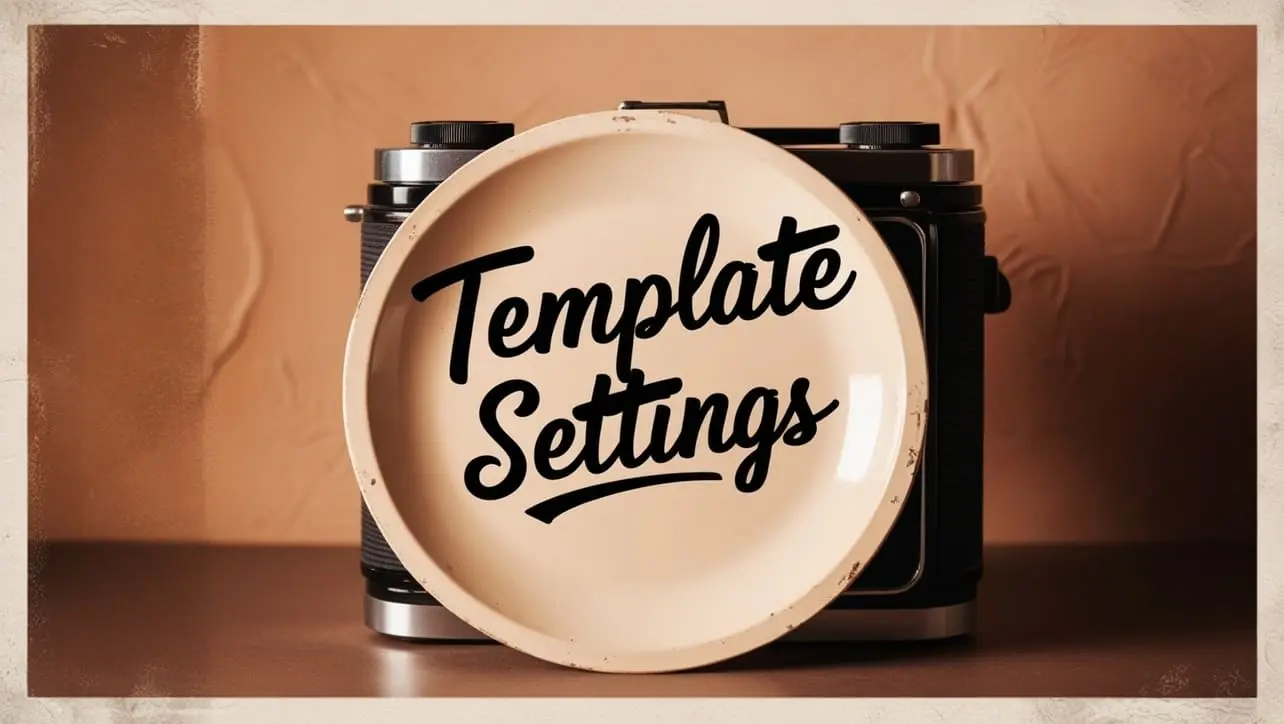
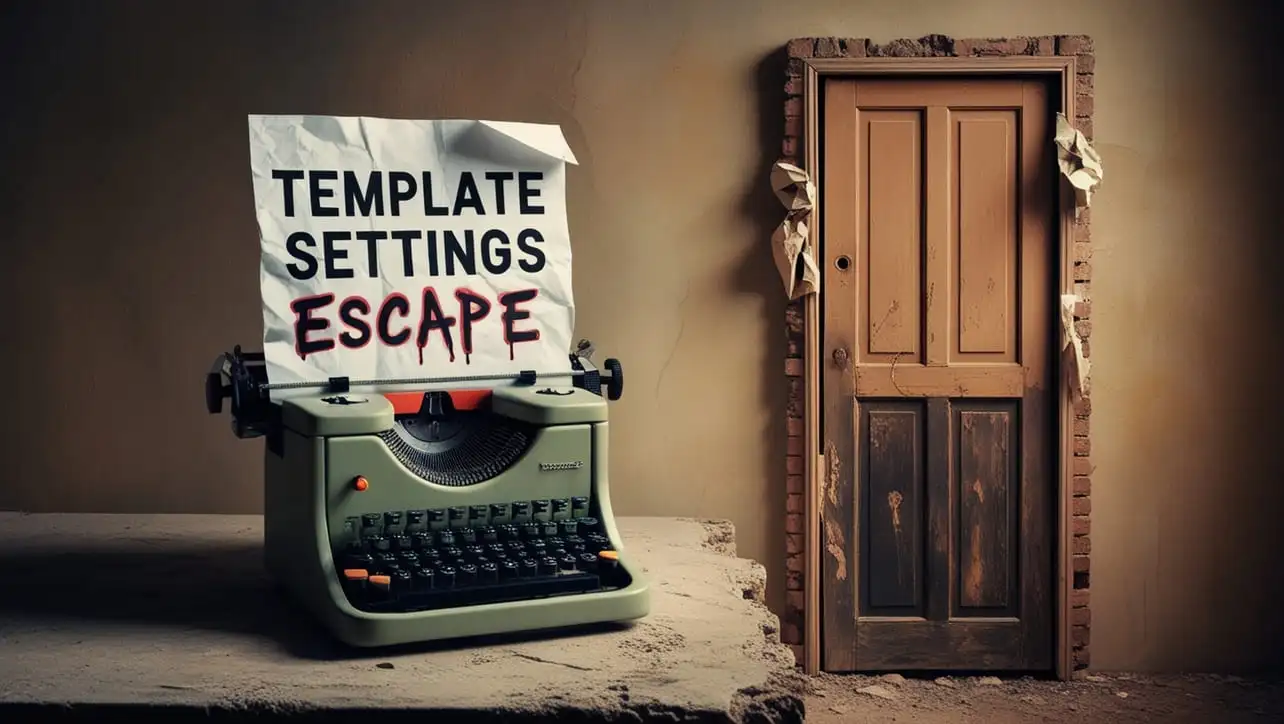
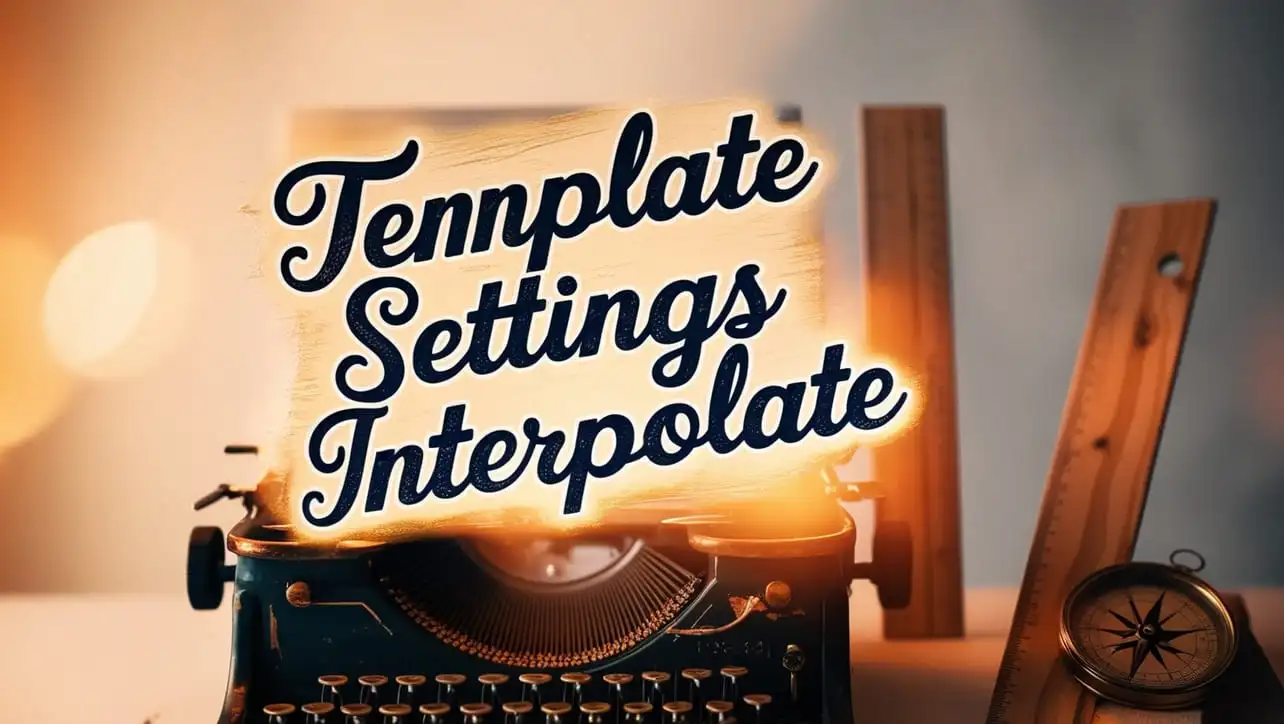
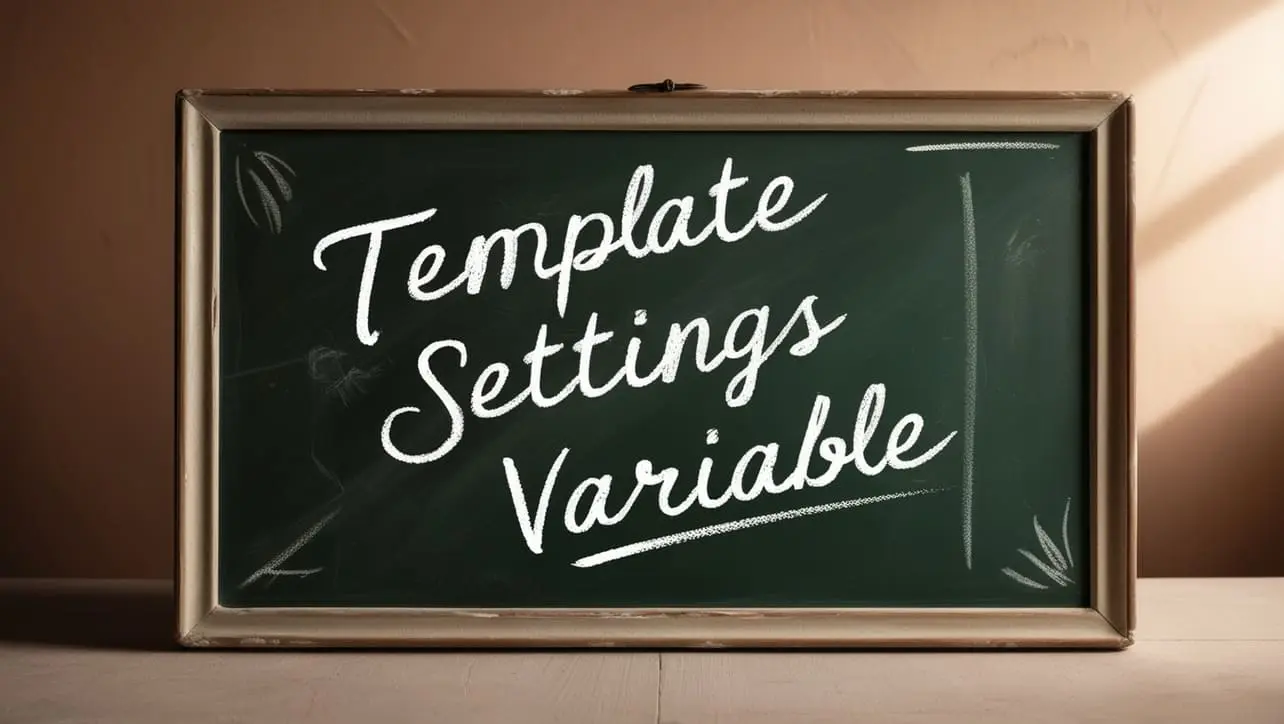
If you have any doubts regarding this article (Lodash _.isDate() Lang Method), please comment here. I will help you immediately.