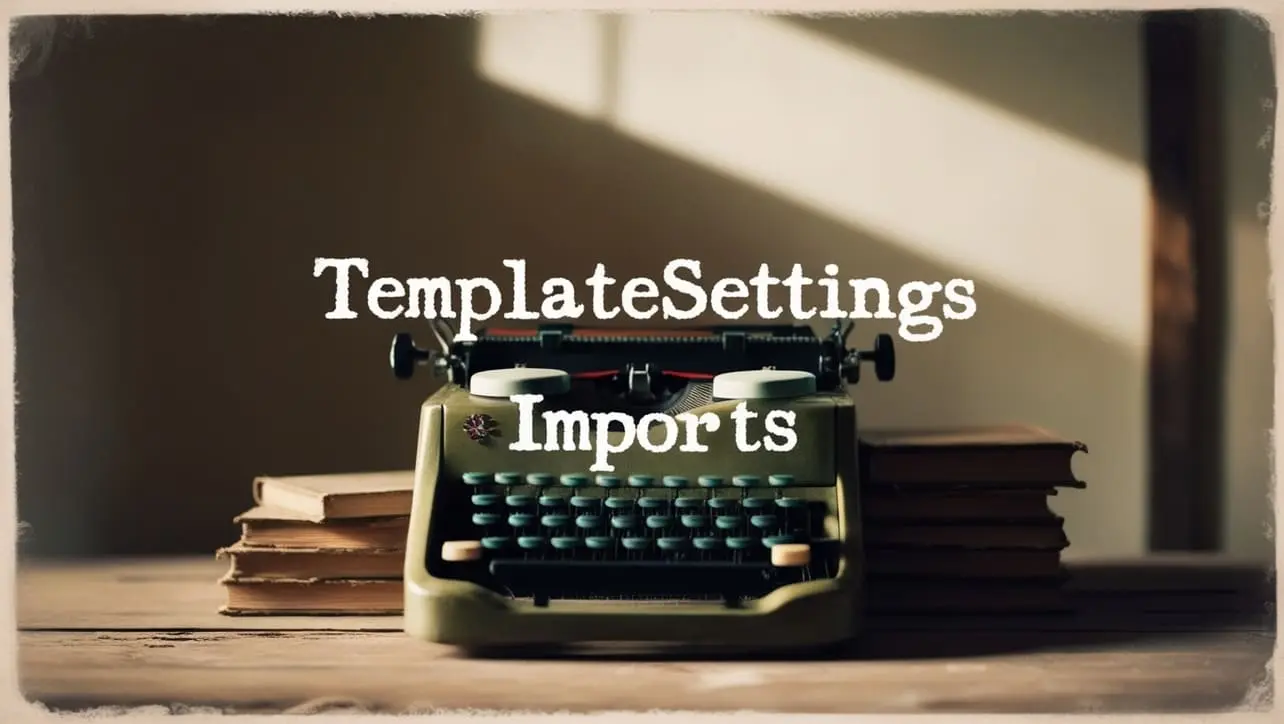
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isArrayLikeObject() Lang Method
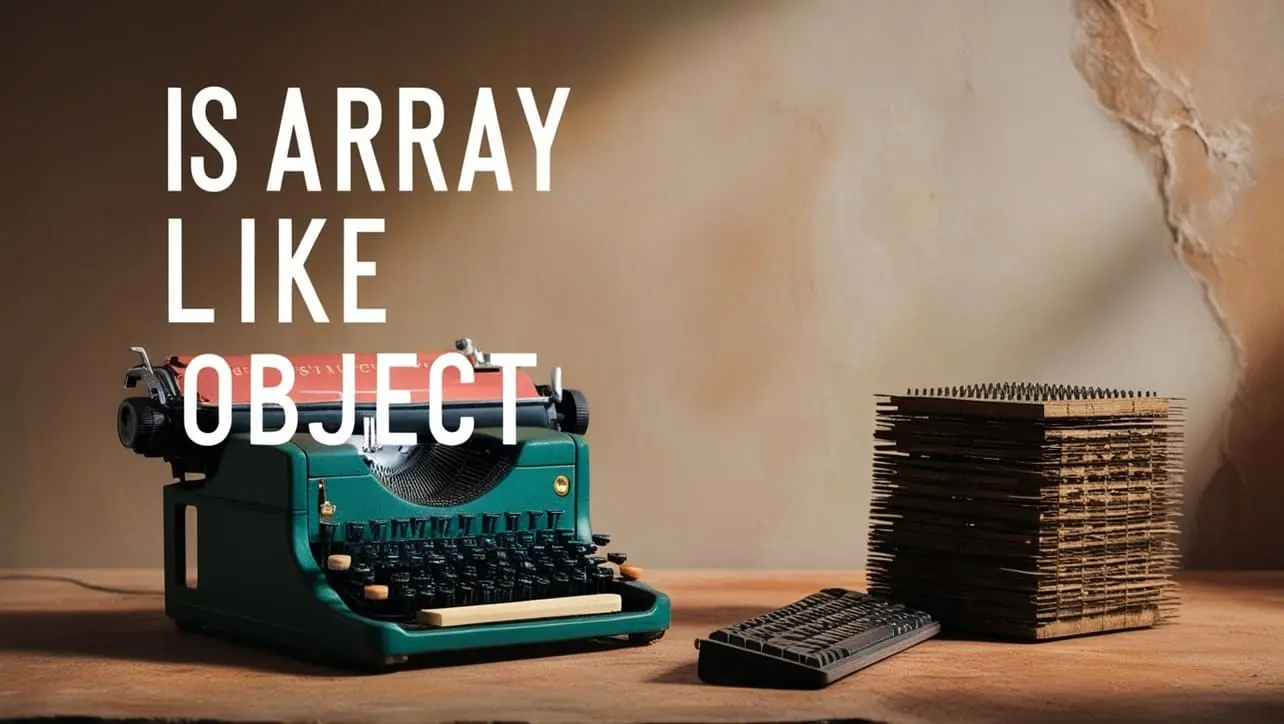
Photo Credit to CodeToFun
🙋 Introduction
Effective JavaScript programming often involves working with diverse data structures, including arrays and array-like objects. Lodash, a versatile utility library, offers the _.isArrayLikeObject()
method, a valuable tool for checking whether an object is array-like.
This method is especially handy when dealing with collections that exhibit array-like characteristics but aren't true arrays.
🧠 Understanding _.isArrayLikeObject() Method
The _.isArrayLikeObject()
method in Lodash checks if an object is array-like, meaning it has a numeric length property and property values accessible using numeric indices. This allows developers to determine whether an object is suitable for array-style operations.
💡 Syntax
The syntax for the _.isArrayLikeObject()
method is straightforward:
_.isArrayLikeObject(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isArrayLikeObject()
method:
const _ = require('lodash');
const arrayLikeObject = { 0: 'apple', 1: 'banana', 2: 'orange', length: 3 };
const isArrayLike = _.isArrayLikeObject(arrayLikeObject);
console.log(isArrayLike);
// Output: true
In this example, arrayLikeObject resembles an array with numeric indices and a length property. The _.isArrayLikeObject()
method confirms its array-like nature.
🏆 Best Practices
When working with the _.isArrayLikeObject()
method, consider the following best practices:
Understanding Array-Like Objects:
Before using
_.isArrayLikeObject()
, understand the characteristics of array-like objects. They should have a numeric length property and properties accessible using numeric indices.example.jsCopiedconst invalidArrayLike = { length: '3' }; const isValidArrayLike = _.isArrayLikeObject(invalidArrayLike); console.log(isValidArrayLike); // Output: false
Treating Strings Differently:
Be aware that strings are array-like but may not behave as expected in all scenarios. Consider handling strings separately if necessary.
example.jsCopiedconst stringWithLengthProperty = { length: 5 }; const isStringArrayLike = _.isArrayLikeObject(stringWithLengthProperty); console.log(isStringArrayLike); // Output: true
Other Checks for Arrays:
Remember that
_.isArrayLikeObject()
specifically checks for array-like objects. If you want to check for arrays, consider using Array.isArray().example.jsCopiedconst regularArray = [1, 2, 3]; const isArray = Array.isArray(regularArray); console.log(isArray); // Output: true
📚 Use Cases
Iterating Over Array-Like Objects:
_.isArrayLikeObject()
is beneficial when you need to iterate over objects with array-like characteristics using array iteration methods.example.jsCopiedconst iterableObject = { 0: 'apple', 1: 'banana', 2: 'orange', length: 3 }; if (_.isArrayLikeObject(iterableObject)) { _.forEach(iterableObject, value => { console.log(value); }); }
Validating Function Arguments:
When designing functions that accept array-like objects as arguments, use
_.isArrayLikeObject()
to validate the input.example.jsCopiedfunction processArrayLike(input) { if (_.isArrayLikeObject(input)) { // Perform array-like operations on the input console.log('Processing array-like object'); } else { console.error('Invalid input. Expected array-like object.'); } } const validInput = { 0: 'apple', 1: 'banana', 2: 'orange', length: 3 }; const invalidInput = 'not an array-like object'; processArrayLike(validInput); // Output: Processing array-like object processArrayLike(invalidInput); // Output: Invalid input. Expected array-like object.
Polymorphic Function Behavior:
Design polymorphic functions that can handle both arrays and array-like objects by incorporating
_.isArrayLikeObject()
in your logic.example.jsCopiedfunction processCollection(collection) { if (_.isArrayLikeObject(collection)) { // Handle array-like objects console.log('Processing array-like object'); } else if (Array.isArray(collection)) { // Handle arrays console.log('Processing array'); } else { console.error('Invalid input. Expected array or array-like object.'); } } const arrayInput = [1, 2, 3]; const arrayLikeInput = { 0: 'apple', 1: 'banana', 2: 'orange', length: 3 }; const invalidInput = 'not a collection'; processCollection(arrayInput); // Output: Processing array processCollection(arrayLikeInput); // Output: Processing array-like object processCollection(invalidInput); // Output: Invalid input. Expected array or array-like object.
🎉 Conclusion
The _.isArrayLikeObject()
method in Lodash provides a reliable way to determine whether an object exhibits array-like characteristics. Whether you're iterating over objects, validating function arguments, or designing polymorphic functions, this method empowers you to handle array-like scenarios with confidence in your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isArrayLikeObject()
method in your Lodash projects.
👨💻 Join our Community:
Author
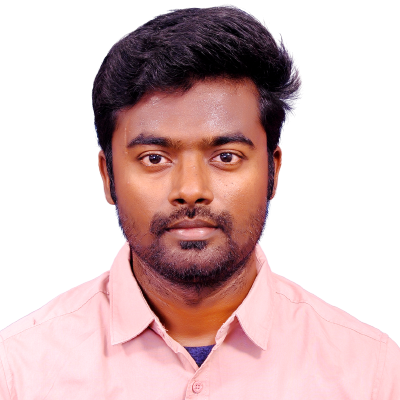
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
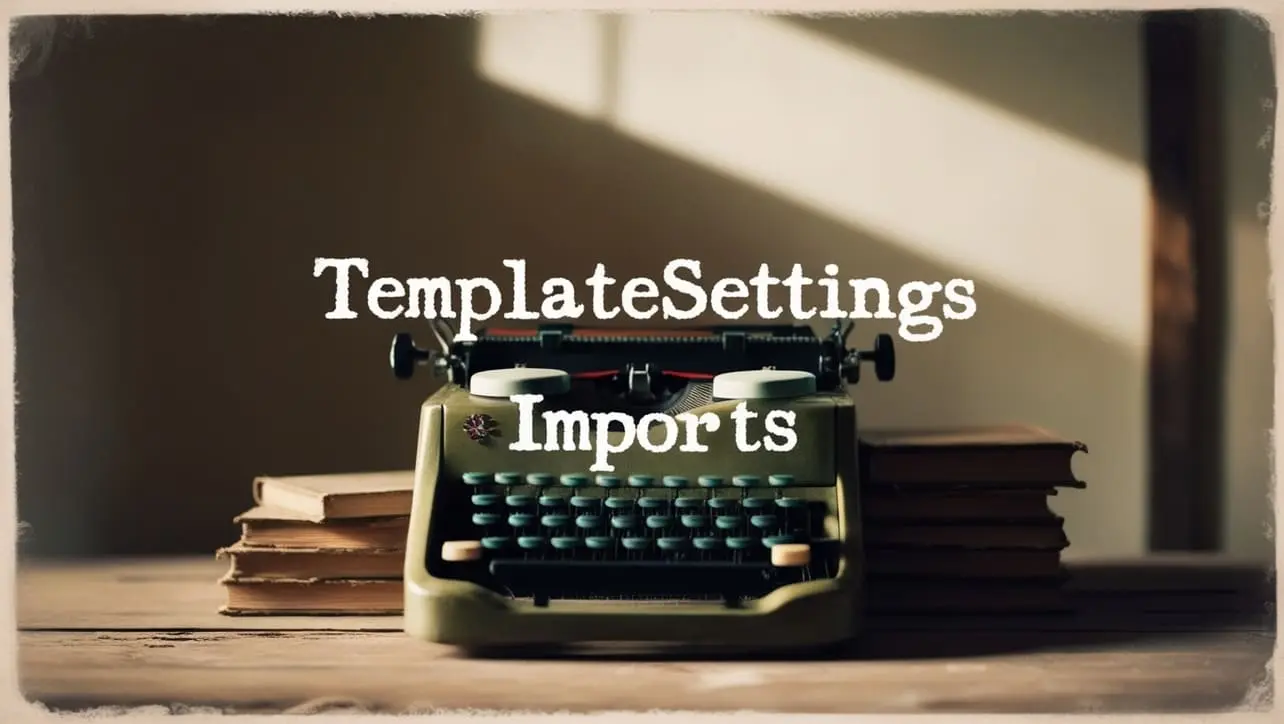
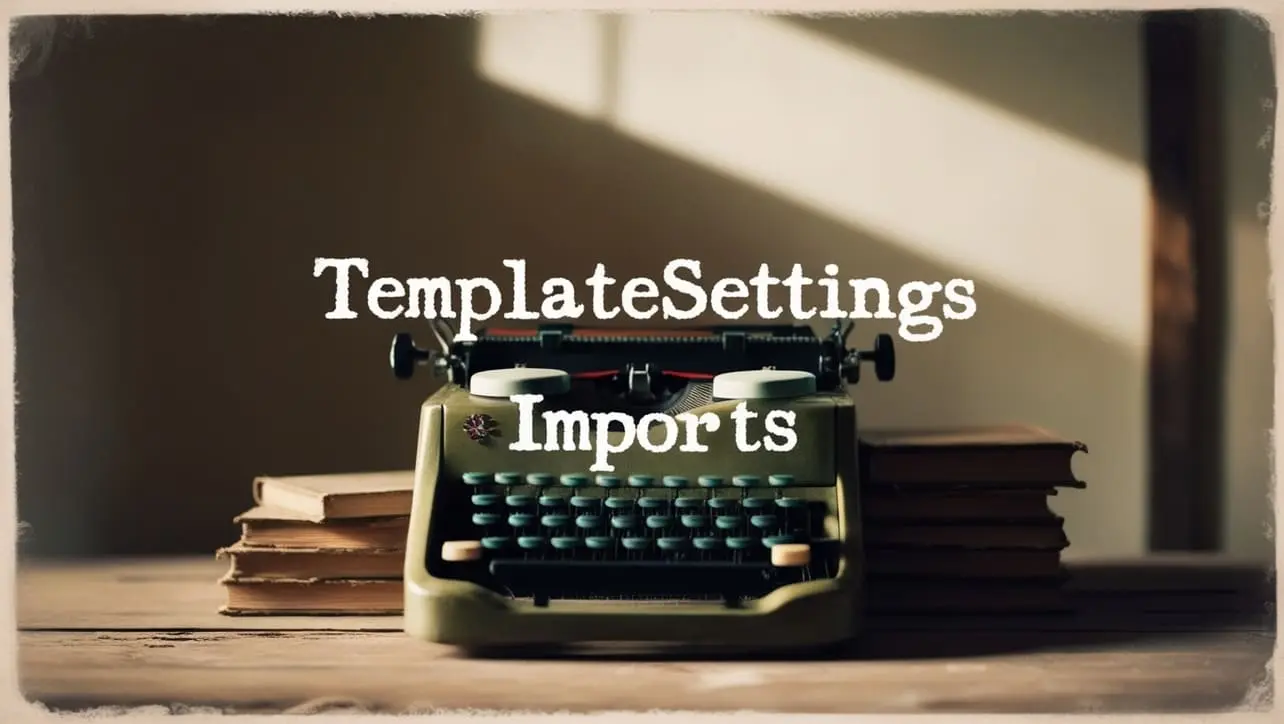
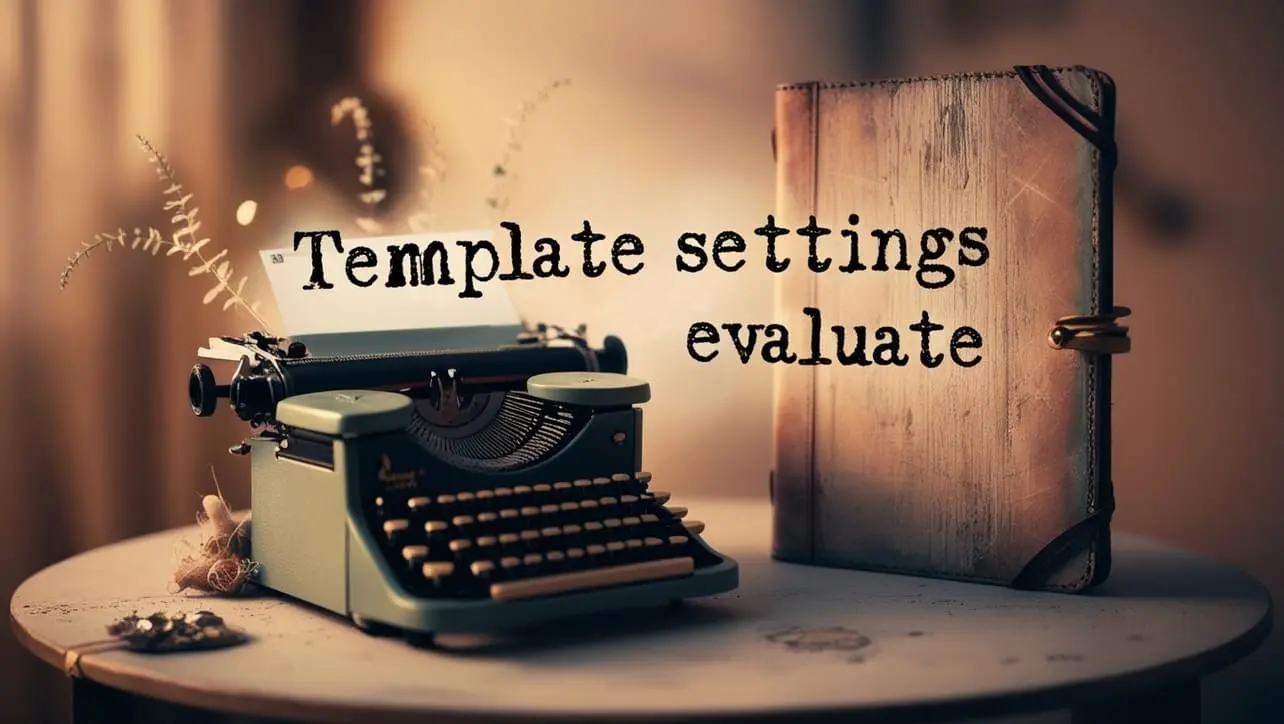
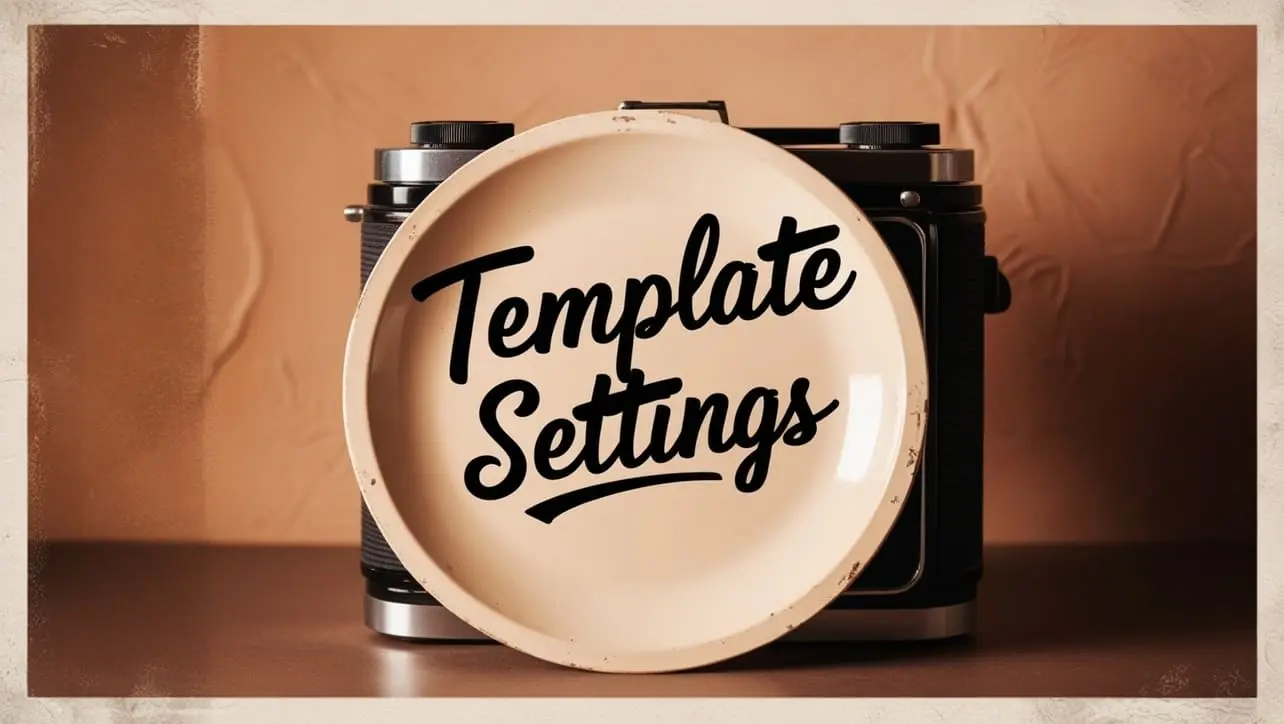
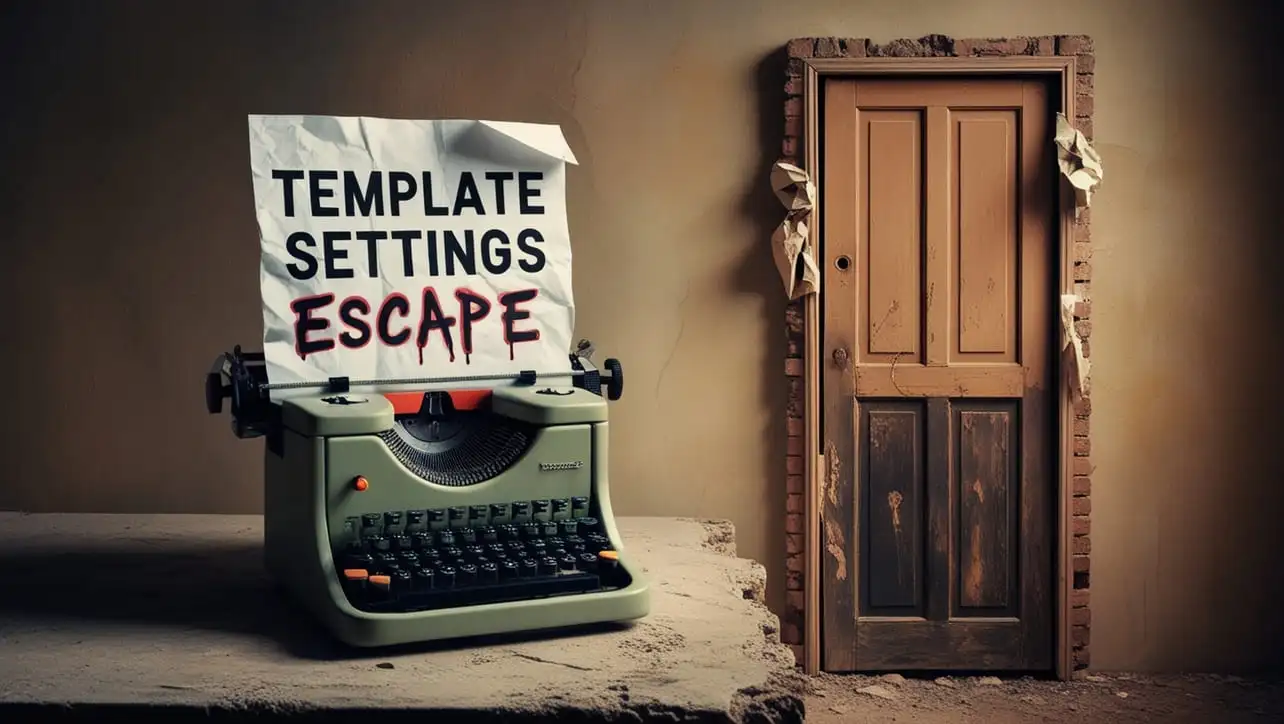
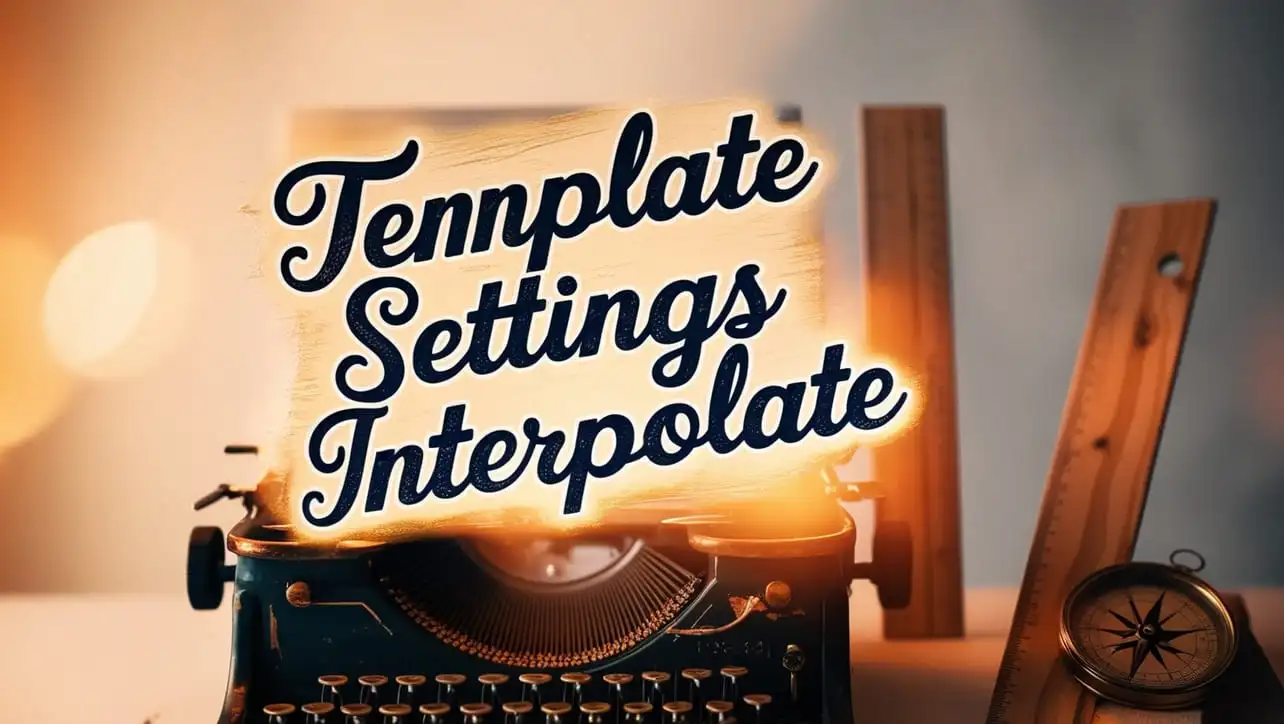
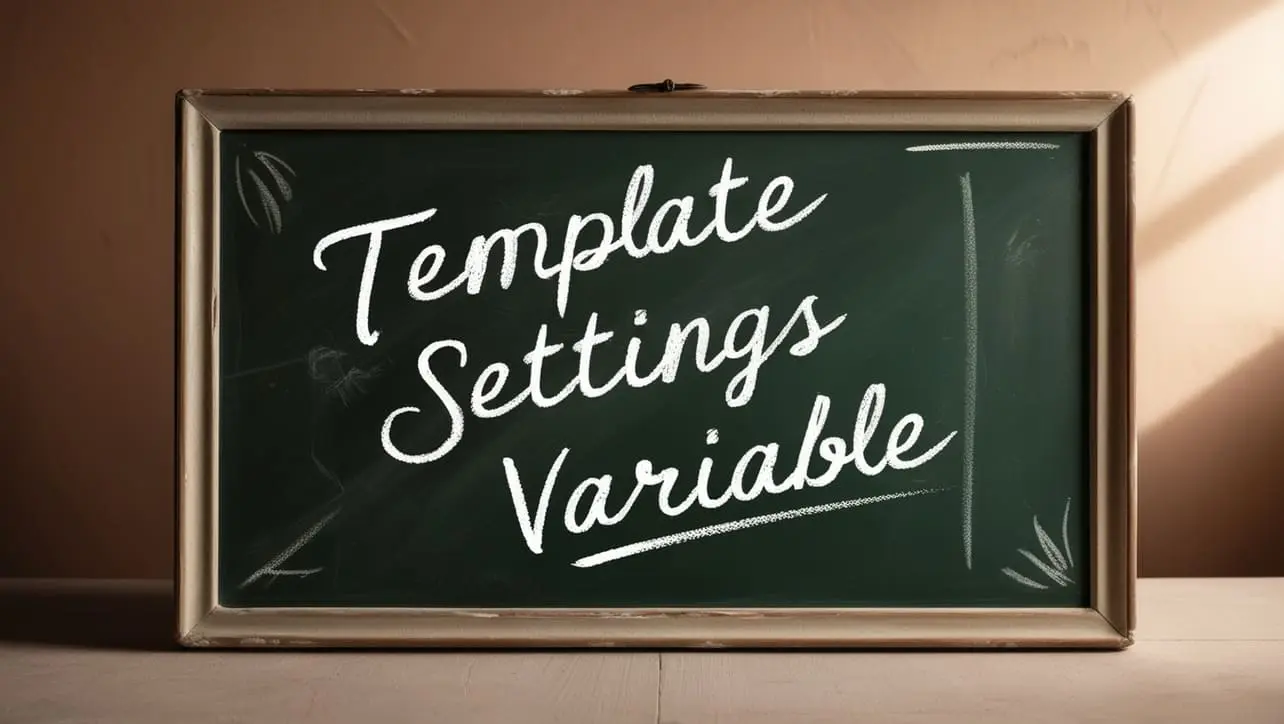
If you have any doubts regarding this article (Lodash _.isArrayLikeObject() Lang Method), please comment here. I will help you immediately.