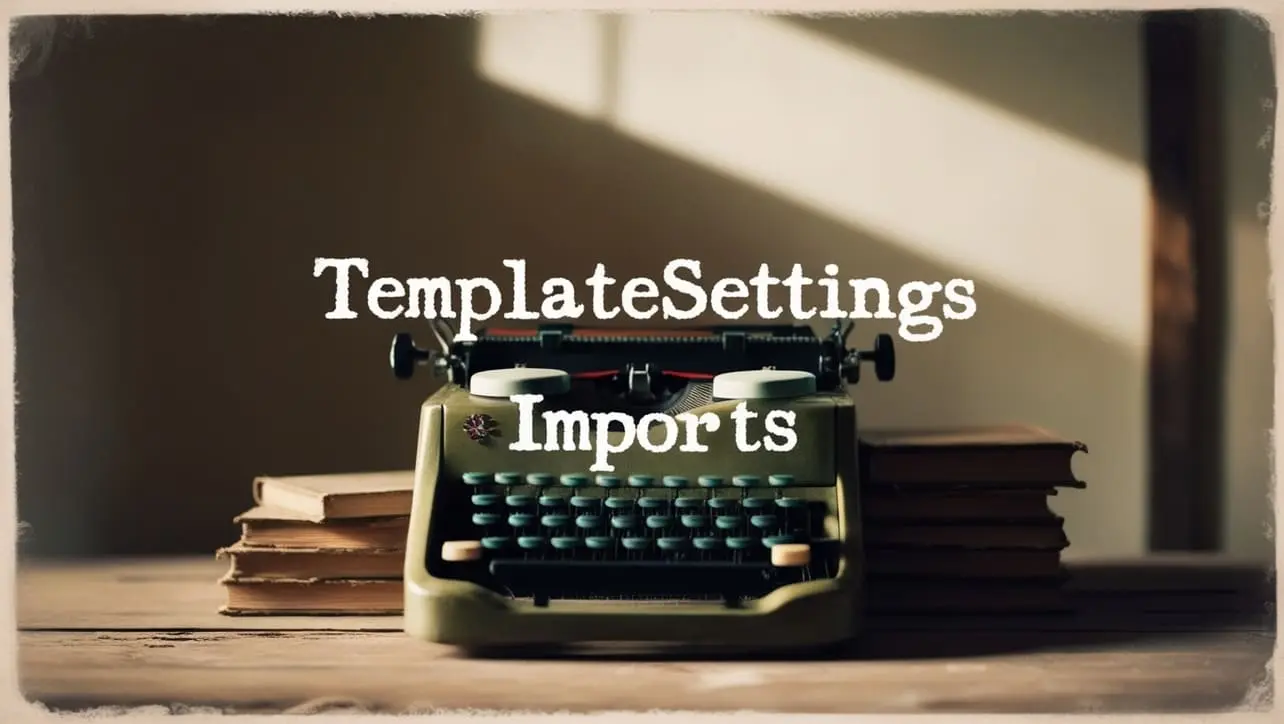
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isArrayLike() Lang Method
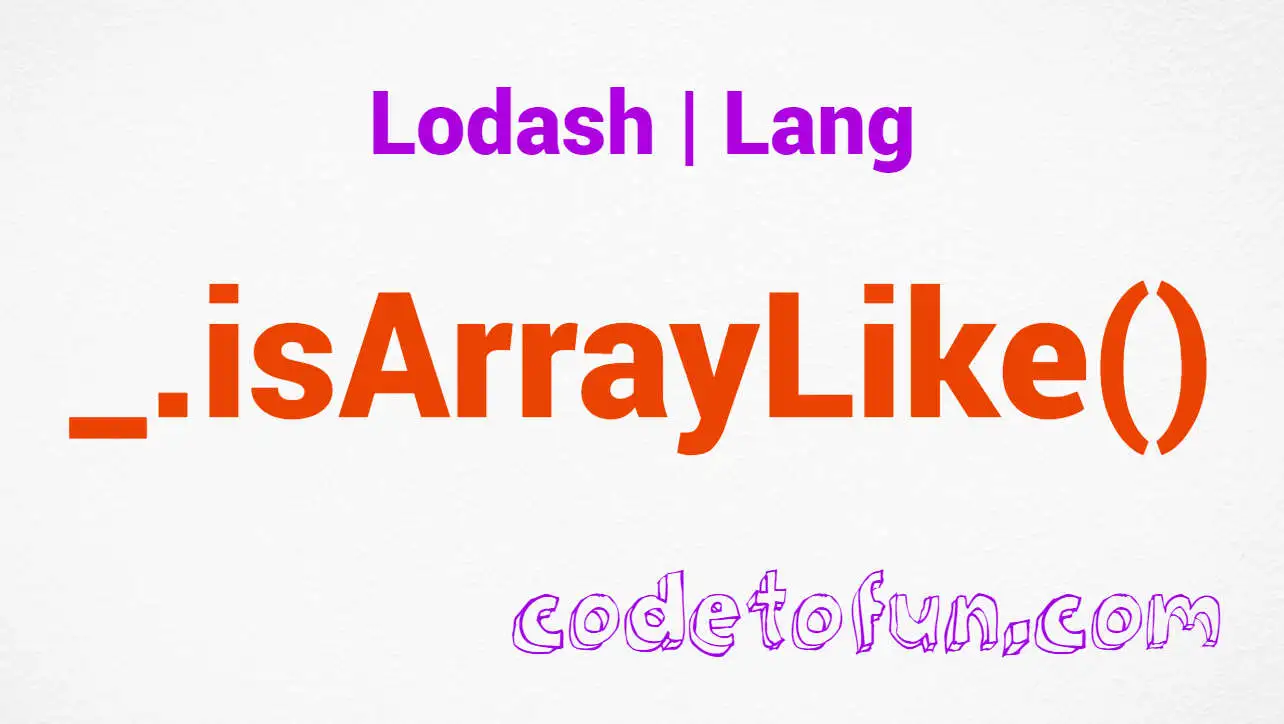
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, effective handling of data structures is paramount. Lodash, a comprehensive utility library, provides an arsenal of functions to simplify common programming tasks. Among its repertoire is the _.isArrayLike()
method, a versatile tool for determining whether an object resembles an array-like structure.
This function proves invaluable when dealing with a variety of objects, enhancing the flexibility and robustness of your code.
🧠 Understanding _.isArrayLike() Method
The _.isArrayLike()
method in Lodash is designed to assess whether an object is array-like. An array-like object, while not a true array, shares similarities such as having a length property and numeric indices. This function facilitates more inclusive and dynamic code, allowing developers to handle a broader range of data structures.
💡 Syntax
The syntax for the _.isArrayLike()
method is straightforward:
_.isArrayLike(value)
- value: The value to inspect.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isArrayLike()
method:
const _ = require('lodash');
const arrayLikeObject = { 0: 'apple', 1: 'banana', length: 2 };
const isArrayLike = _.isArrayLike(arrayLikeObject);
console.log(isArrayLike);
// Output: true
In this example, arrayLikeObject is assessed using _.isArrayLike()
, and the result indicates whether the object is array-like.
🏆 Best Practices
When working with the _.isArrayLike()
method, consider the following best practices:
Robust Type Checking:
When using
_.isArrayLike()
, consider combining it with other type-checking methods to ensure comprehensive validation. This practice enhances the reliability of your code when dealing with diverse data structures.example.jsCopiedconst validateArrayLike = (value) => { if (_.isArrayLike(value) && _.isObject(value)) { console.log('Valid array-like object'); } else { console.error('Invalid array-like object'); } }; const arrayLikeObject = { 0: 'apple', 1: 'banana', length: 2 }; validateArrayLike(arrayLikeObject);
Handling Arguments Objects:
In functions that receive variable arguments, arguments is an array-like object. Use
_.isArrayLike()
to ensure proper handling of these objects.example.jsCopiedconst processArguments = function () { if (_.isArrayLike(arguments)) { console.log('Processing arguments:', arguments); } else { console.error('Invalid arguments'); } }; processArguments(1, 'apple', true);
Iterating Over Array-Like Objects:
For situations where iteration is required, use
_.isArrayLike()
to determine if the object can be safely traversed using numeric indices.example.jsCopiedconst iterateArrayLike = function (arrayLike) { if (_.isArrayLike(arrayLike)) { for (let i = 0; i < arrayLike.length; i++) { console.log(arrayLike[i]); } } else { console.error('Invalid array-like object'); } }; const sampleObject = { 0: 'apple', 1: 'banana', length: 2 }; iterateArrayLike(sampleObject);
📚 Use Cases
DOM Manipulation:
When working with the Document Object Model (DOM), elements in a NodeList are array-like.
_.isArrayLike()
can be employed to ensure that operations are safely applied to these structures.example.jsCopiedconst nodeList = document.querySelectorAll('.items'); if (_.isArrayLike(nodeList)) { // Perform operations on the NodeList console.log('Number of elements:', nodeList.length); }
Custom Collection Objects:
In scenarios where custom collection objects emulate array behavior,
_.isArrayLike()
aids in identifying and handling these structures appropriately.example.jsCopiedclass CustomCollection { constructor() { this.data = []; } push(value) { this.data.push(value); } get length() { return this.data.length; } } const customObject = new CustomCollection(); customObject.push('apple'); customObject.push('banana'); if (_.isArrayLike(customObject)) { console.log('Custom collection length:', customObject.length); }
Argument Validation:
When creating functions that accept variable arguments,
_.isArrayLike()
ensures proper validation, allowing for consistent and error-free processing.example.jsCopiedconst processDynamicArgs = function (...args) { if (_.isArrayLike(args)) { args.forEach(arg => console.log(arg)); } else { console.error('Invalid arguments'); } }; processDynamicArgs('apple', 'banana', 'orange');
🎉 Conclusion
The _.isArrayLike()
method in Lodash serves as a valuable asset in JavaScript development, offering a reliable means to identify array-like structures. Whether you're working with DOM elements, custom collection objects, or handling variable arguments, this method provides a versatile solution for robust and inclusive code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isArrayLike()
method in your Lodash projects.
👨💻 Join our Community:
Author
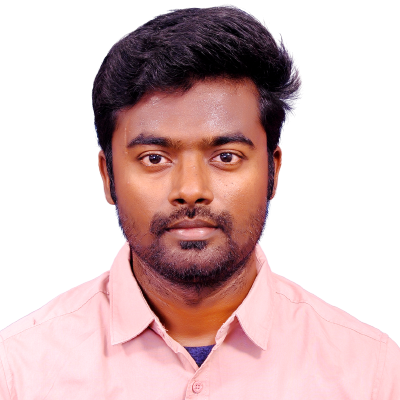
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
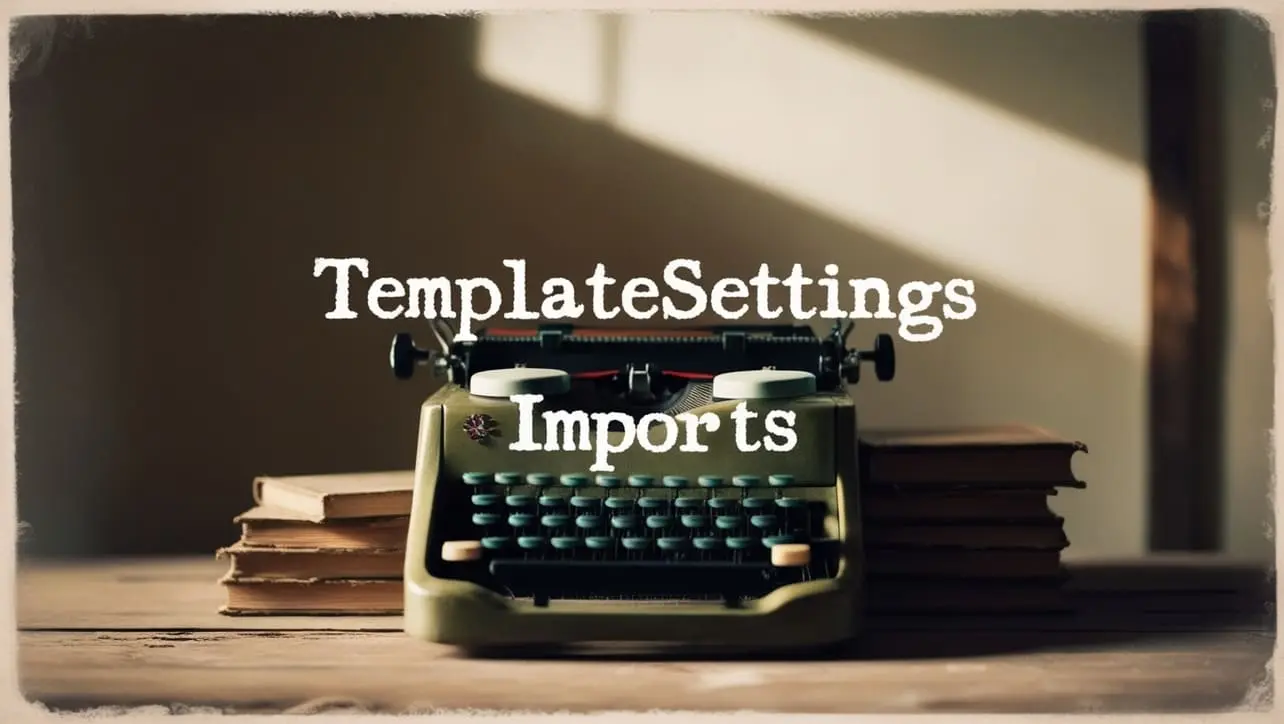
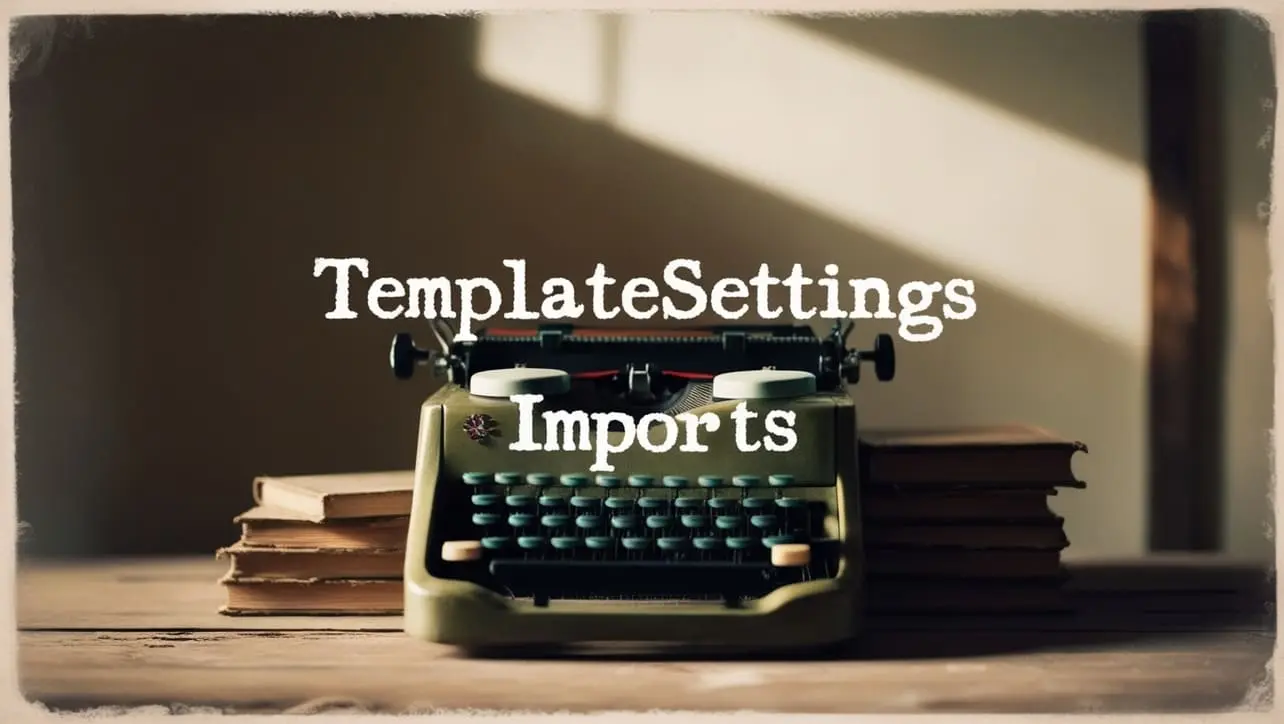
Lodash _.templateSettings.imports Property
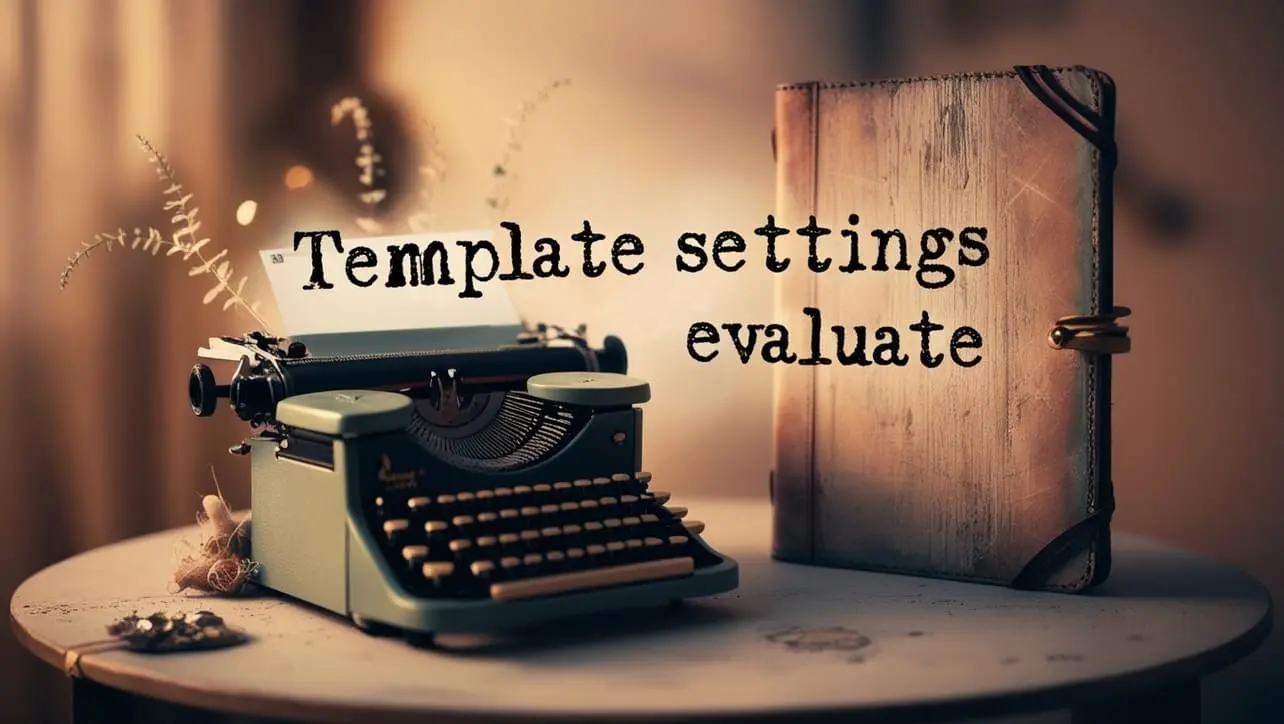
Lodash _.templateSettings.evaluate Property
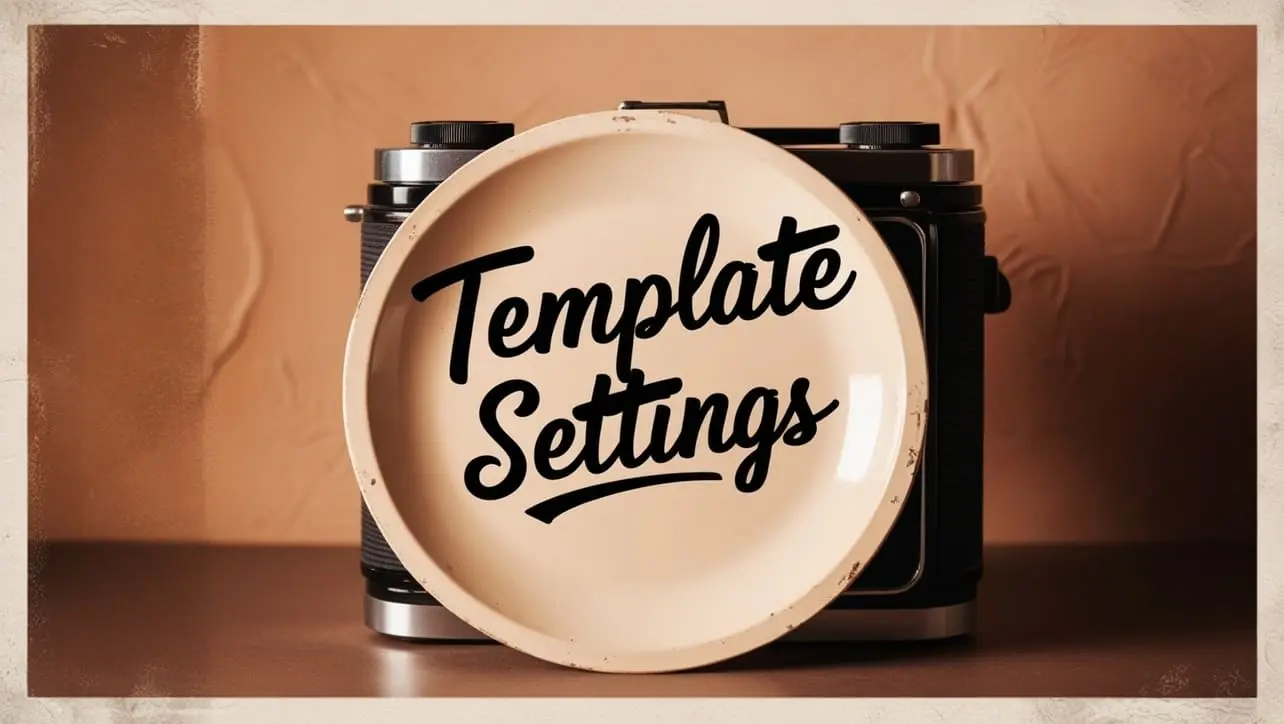
Lodash _.templateSettings Property
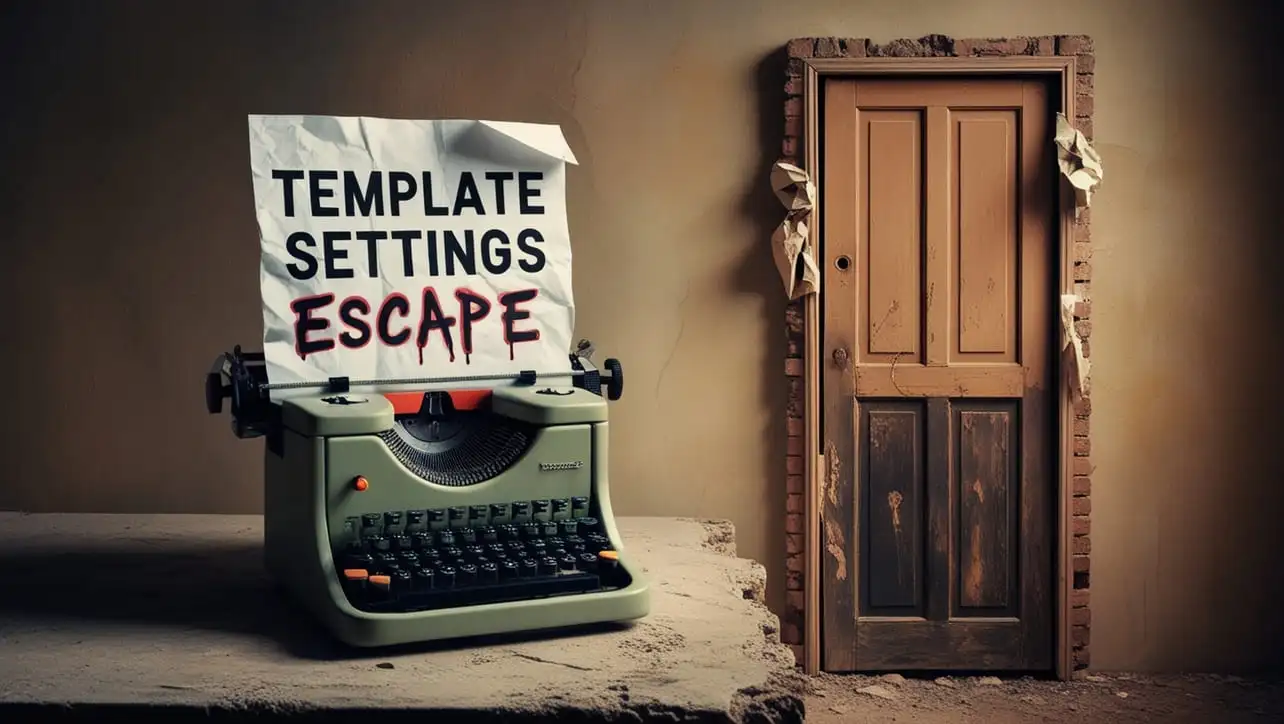
Lodash _.templateSettings.escape Property
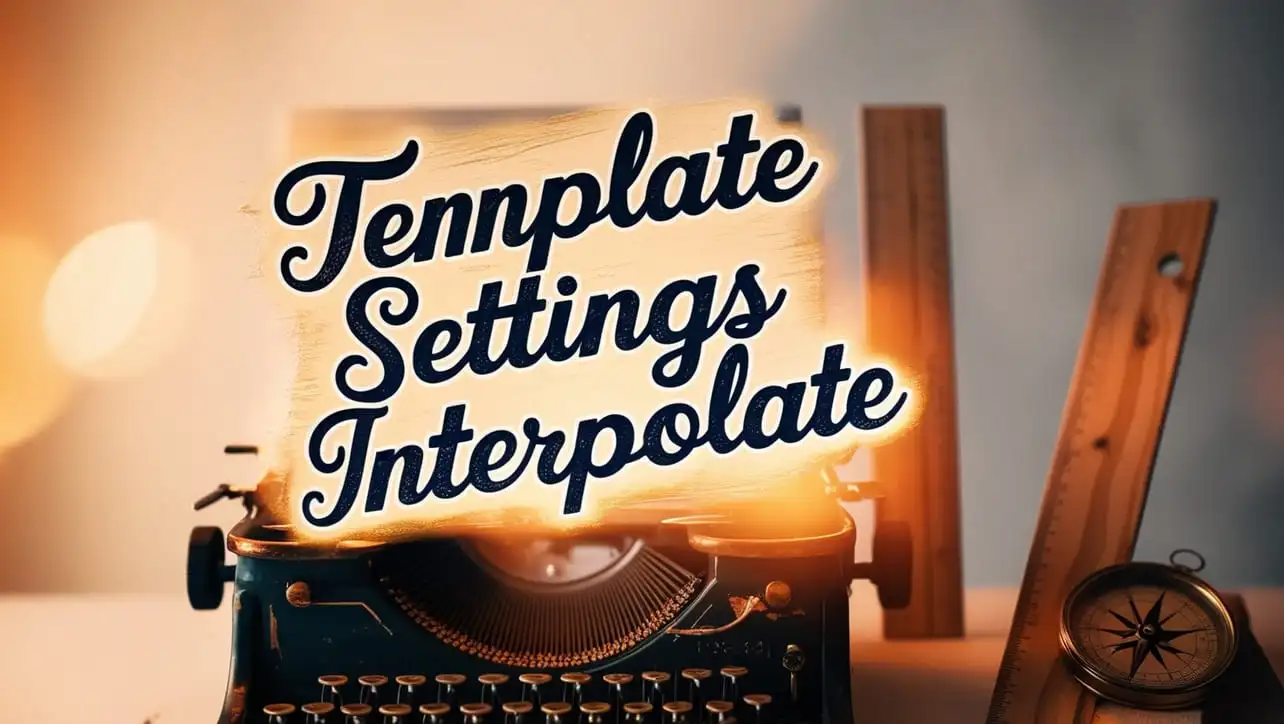
Lodash _.templateSettings.interpolate Property
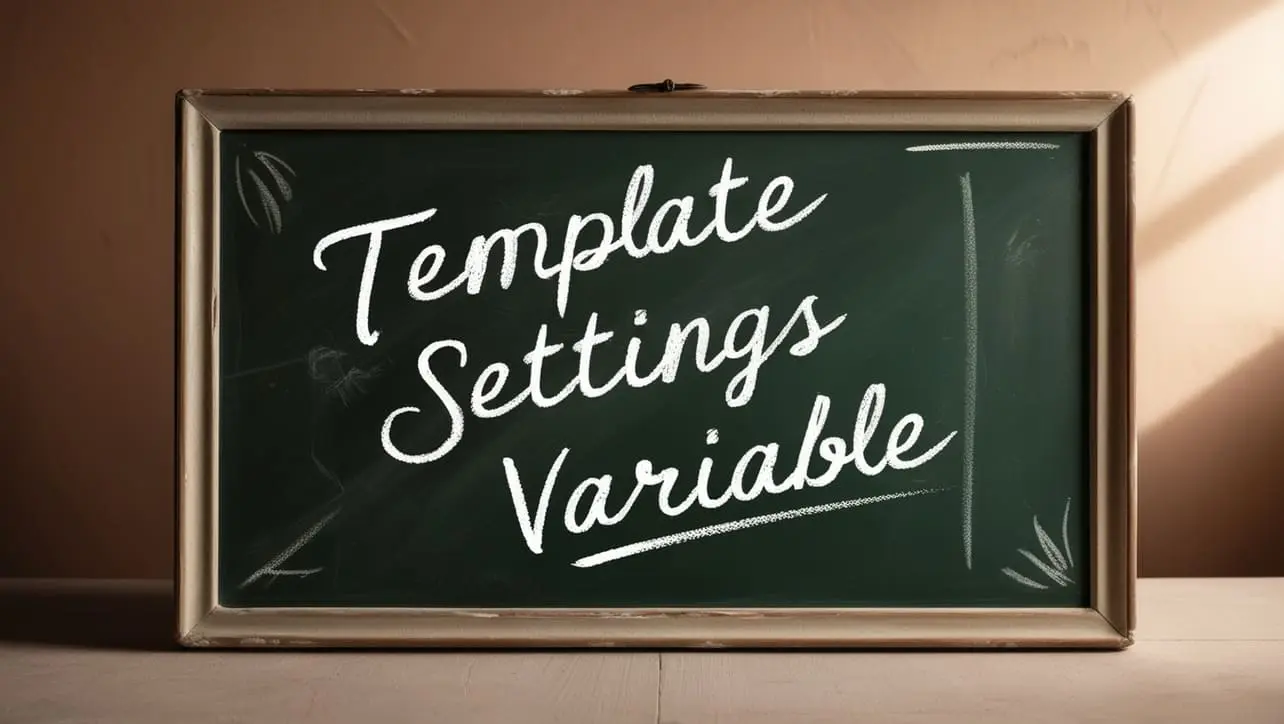
If you have any doubts regarding this article (Lodash _.isArrayLike() Lang Method), please comment here. I will help you immediately.