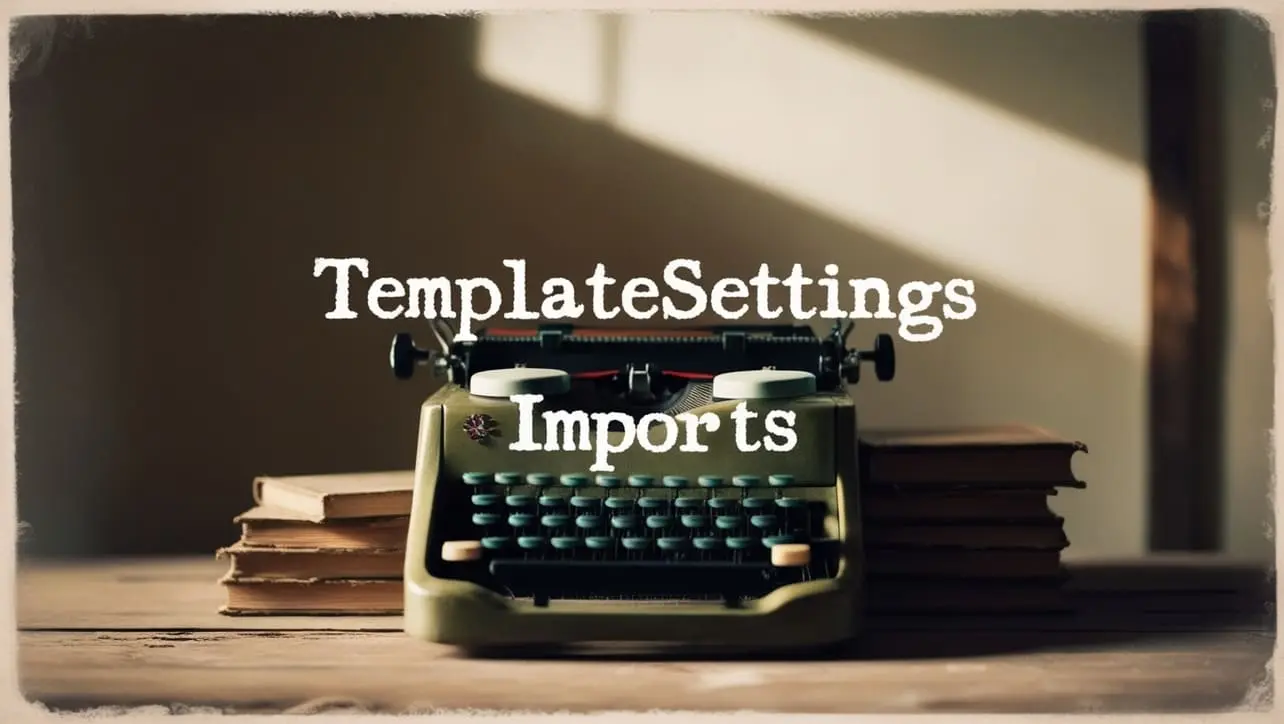
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isArray() Lang Method
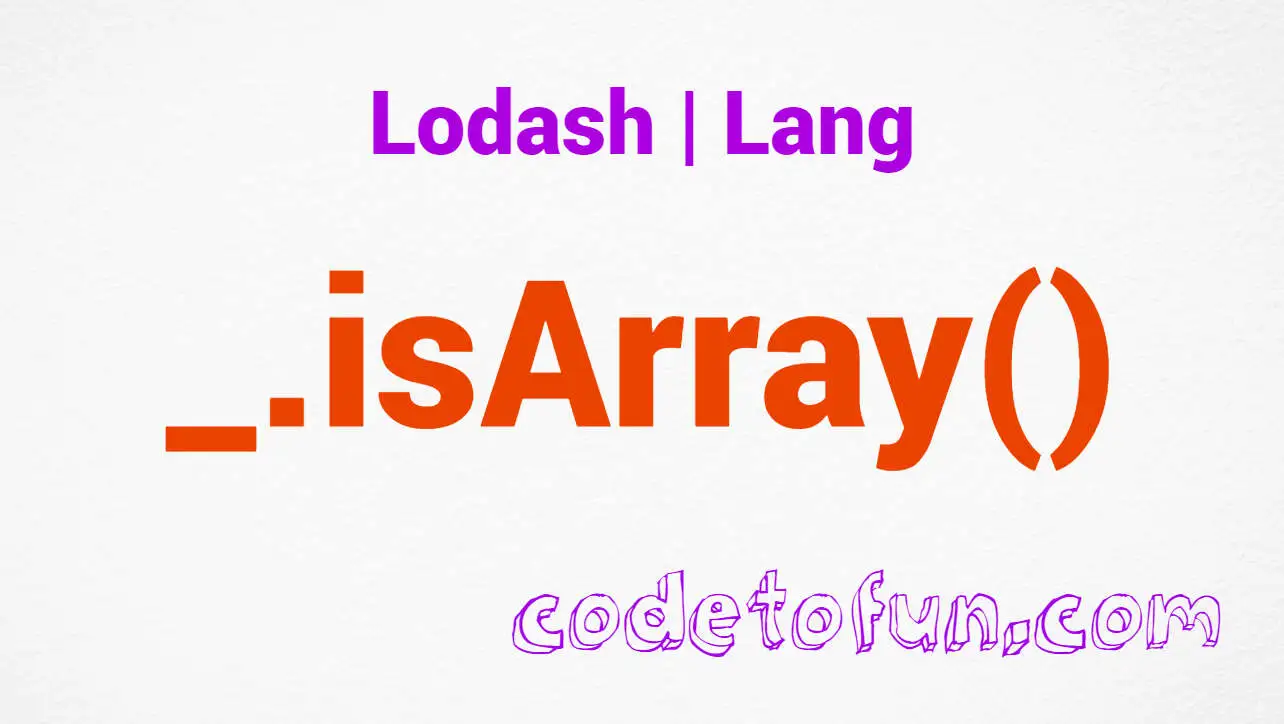
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript, determining the type of a variable is a common task. Lodash, a comprehensive utility library, offers the _.isArray()
method, a simple yet essential function for checking whether a given value is an array.
This method provides a reliable way to handle different data types in your JavaScript applications.
🧠 Understanding _.isArray() Method
The _.isArray()
method in Lodash is designed to ascertain whether a value is an array. It returns true if the value is an array and false otherwise, simplifying the process of type checking in JavaScript.
💡 Syntax
The syntax for the _.isArray()
method is straightforward:
_.isArray(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isArray()
method:
const _ = require('lodash');
const arrayExample = [1, 2, 3];
const nonArrayExample = 'Not an array';
console.log(_.isArray(arrayExample)); // Output: true
console.log(_.isArray(nonArrayExample)); // Output: false
In this example, _.isArray()
is used to check whether the variables arrayExample and nonArrayExample are arrays.
🏆 Best Practices
When working with the _.isArray()
method, consider the following best practices:
Checking for Array Type:
Use
_.isArray()
when specifically checking for an array type. Avoid using other type-checking methods that may not be as reliable for arrays.example.jsCopiedconst someValue = /* ...some dynamic value... */; if (_.isArray(someValue)) { console.log('It is an array!'); } else { console.log('It is not an array.'); }
Combining with Conditional Statements:
Incorporate
_.isArray()
in conditional statements to execute different code paths based on whether a variable is an array or not.example.jsCopiedconst dynamicValue = /* ...some dynamic value... */; if (_.isArray(dynamicValue)) { console.log('Processing an array:', dynamicValue); } else { console.log('Handling non-array case:', dynamicValue); }
Array Type Validation in Functions:
When designing functions that expect an array as an argument, use
_.isArray()
for type validation to ensure the correct input.example.jsCopiedfunction processArray(inputArray) { if (_.isArray(inputArray)) { // Process the array console.log('Array processed:', inputArray); } else { console.error('Invalid input. Expected an array.'); } } const exampleArray = [10, 20, 30]; processArray(exampleArray);
📚 Use Cases
Input Validation in Functions:
In functions that anticipate an array as input,
_.isArray()
can be employed for validation to avoid unexpected behavior.example.jsCopiedfunction processData(data) { if (_.isArray(data)) { // Process the array data console.log('Data processed:', data); } else { console.error('Invalid data format. Expected an array.'); } } const validData = [1, 2, 3]; const invalidData = 'Not an array'; processData(validData); processData(invalidData);
Conditional Rendering in UI:
In web development, when dynamically rendering content based on data types,
_.isArray()
can be used for conditional rendering.example.jsCopiedconst dynamicContent = /* ...some dynamic content... */; if (_.isArray(dynamicContent)) { renderArrayContent(dynamicContent); } else { renderNonArrayContent(dynamicContent); }
Type-Specific Functionality:
Implement type-specific functionality based on whether a variable is an array or not.
example.jsCopiedconst dataToProcess = /* ...some data... */; if (_.isArray(dataToProcess)) { processArrayData(dataToProcess); } else { handleNonArrayData(dataToProcess); }
🎉 Conclusion
The _.isArray()
method in Lodash is a valuable tool for JavaScript developers seeking a reliable and straightforward way to check if a value is an array. By incorporating this method into your code, you can enhance the robustness and clarity of your type-checking mechanisms.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isArray()
method in your Lodash projects.
👨💻 Join our Community:
Author
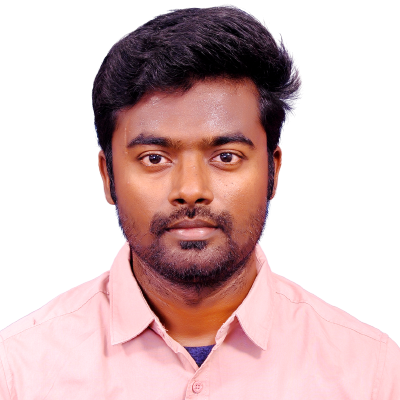
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
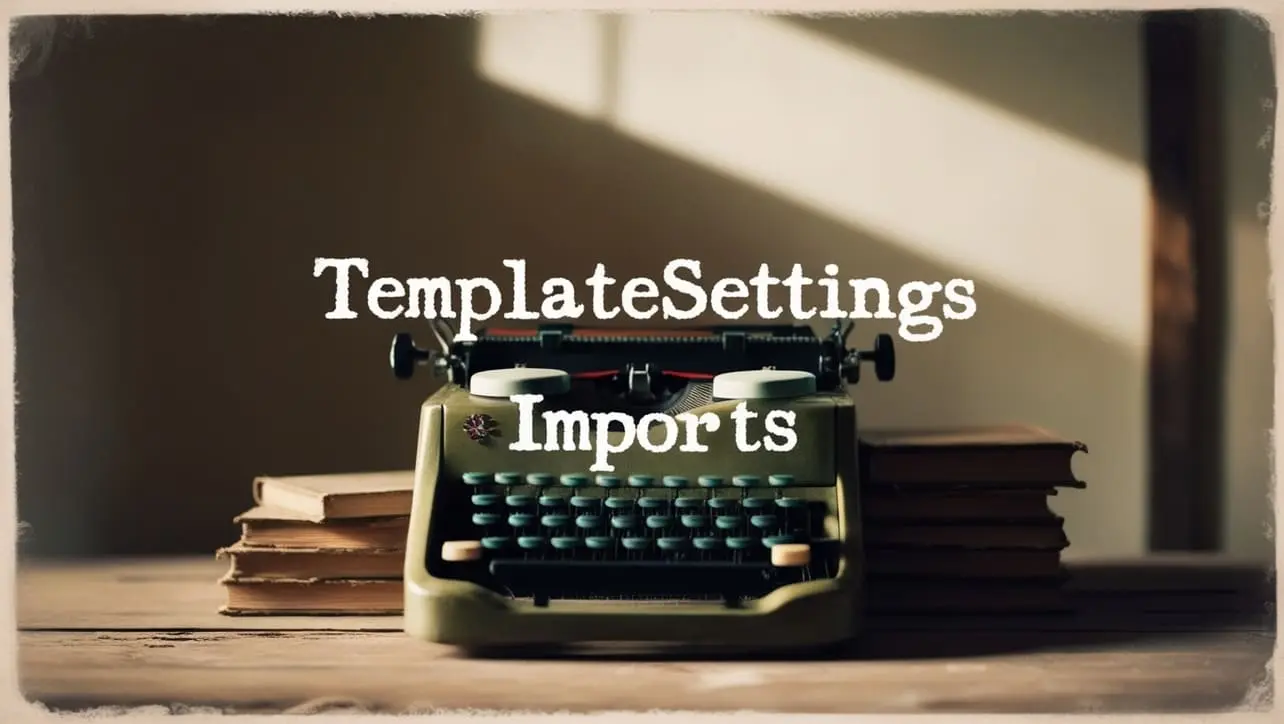
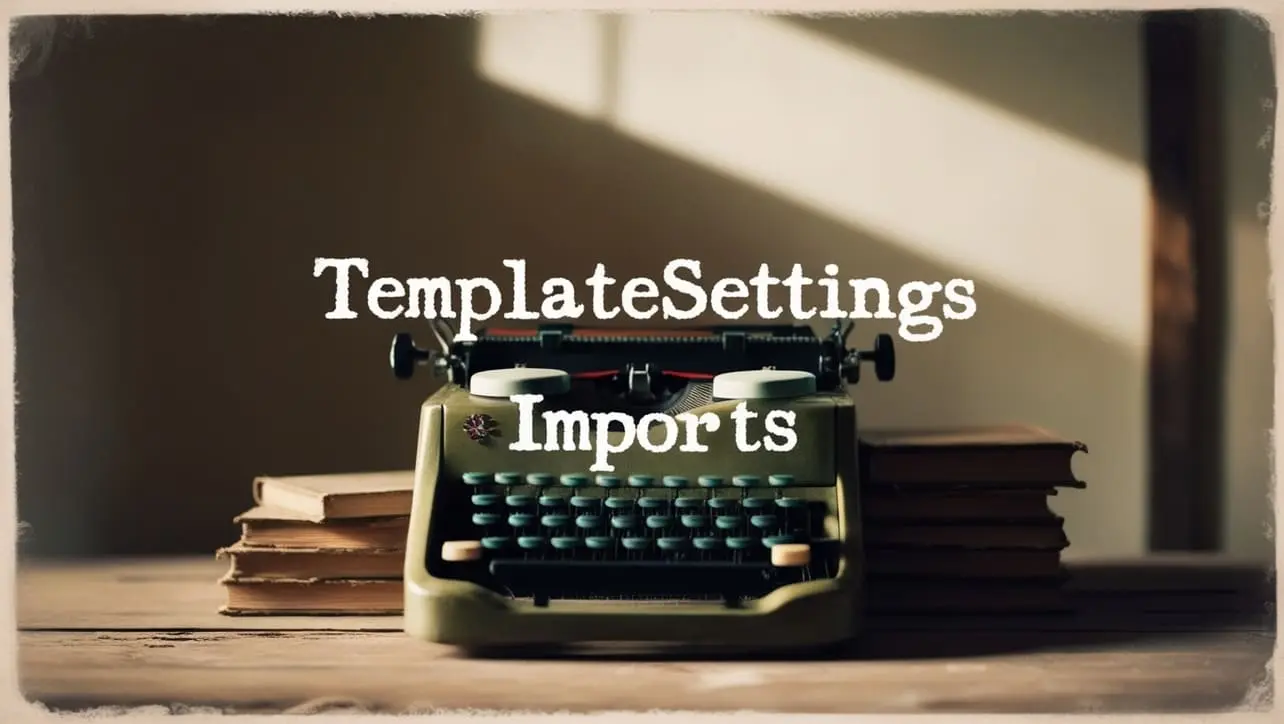
Lodash _.templateSettings.imports Property
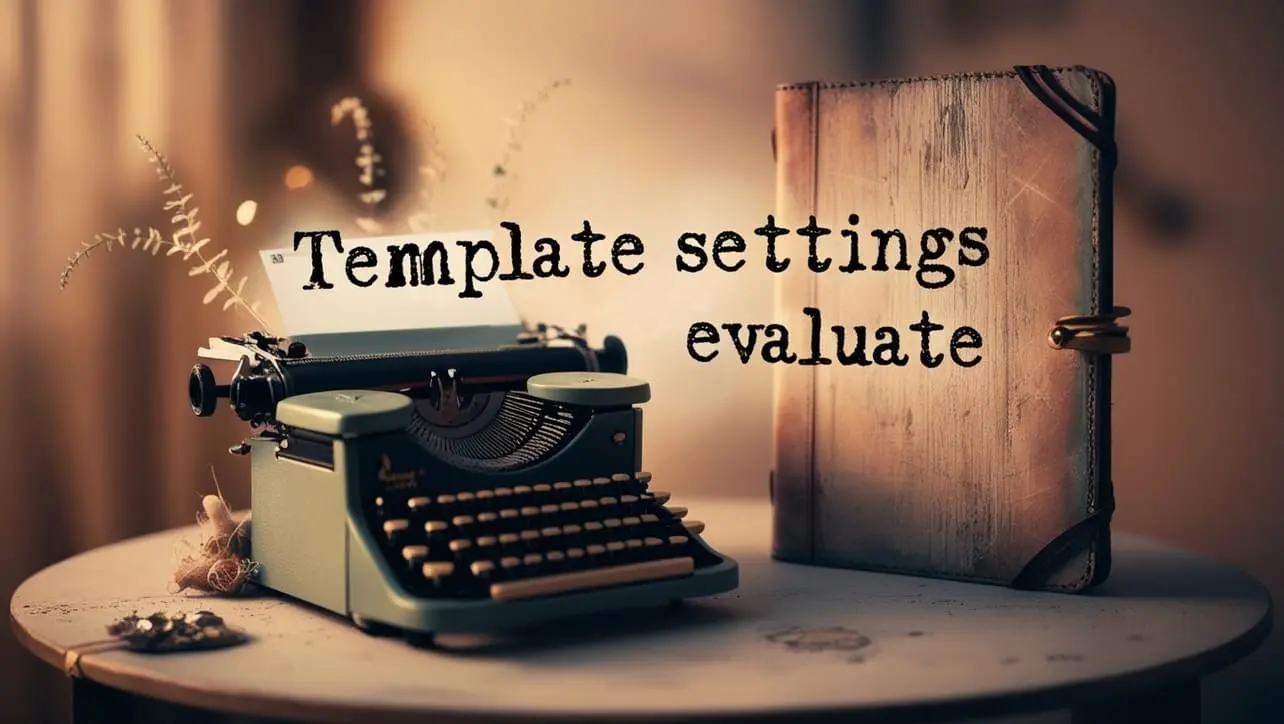
Lodash _.templateSettings.evaluate Property
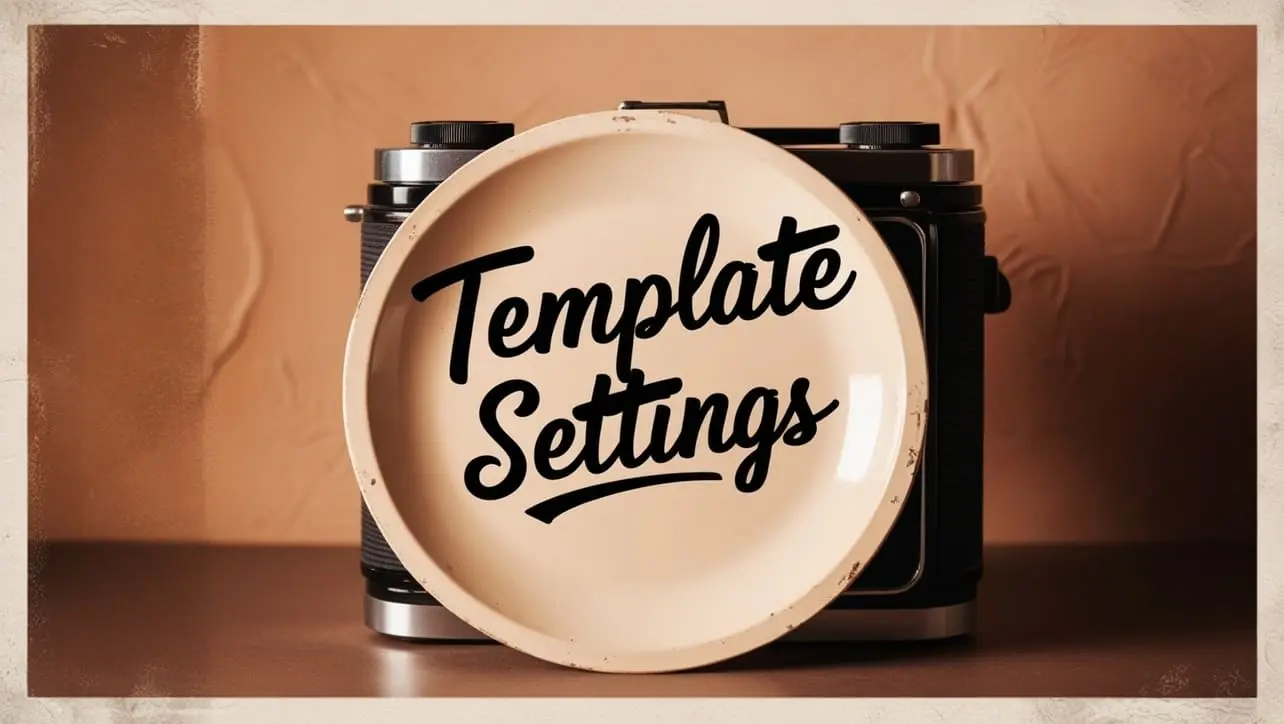
Lodash _.templateSettings Property
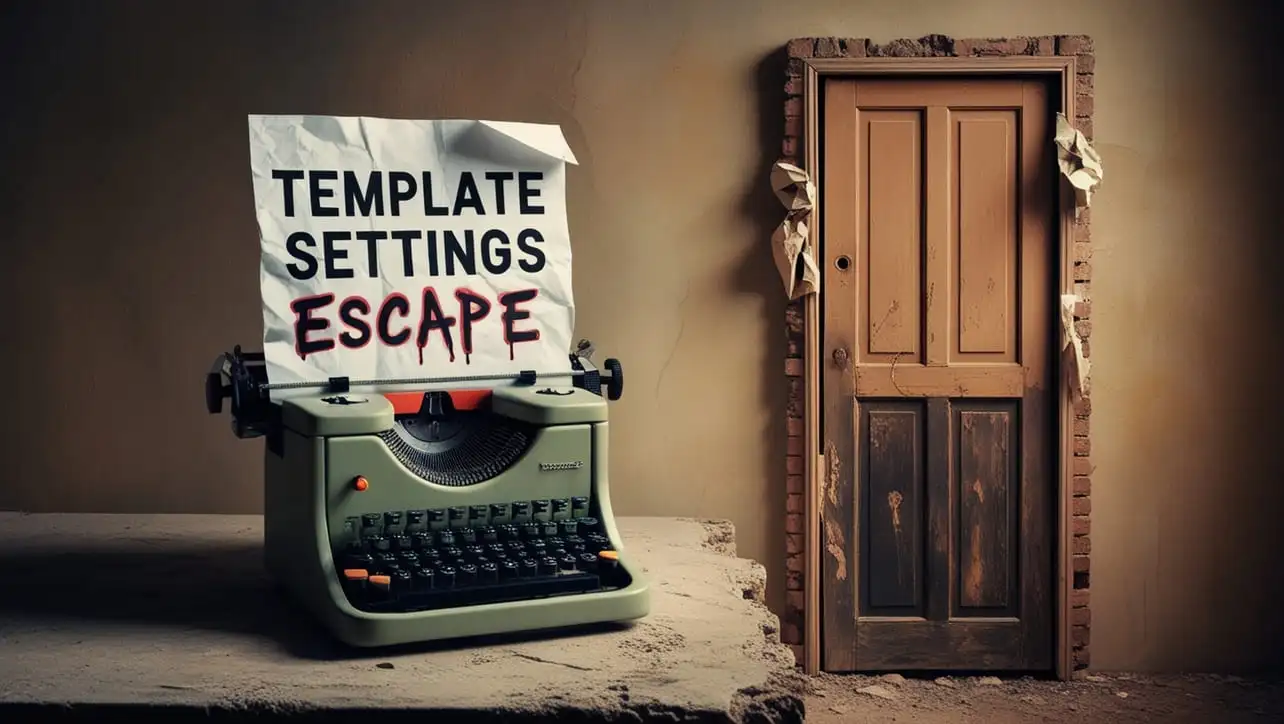
Lodash _.templateSettings.escape Property
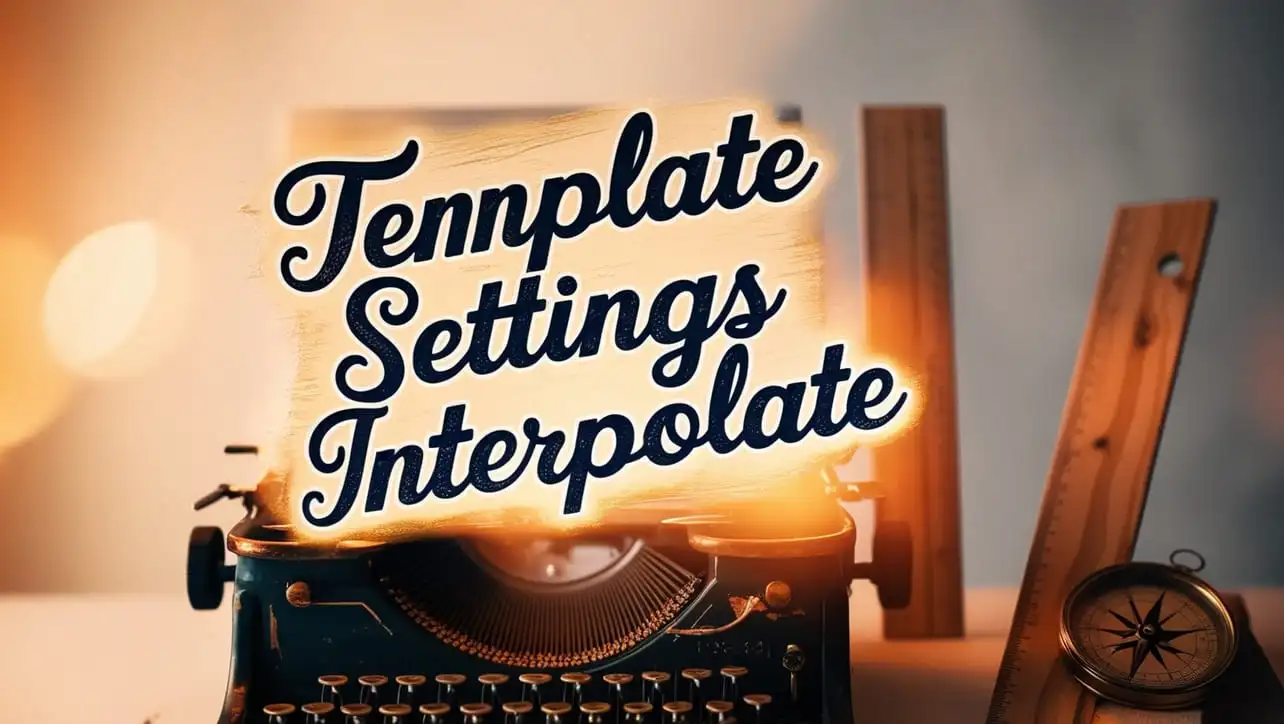
Lodash _.templateSettings.interpolate Property
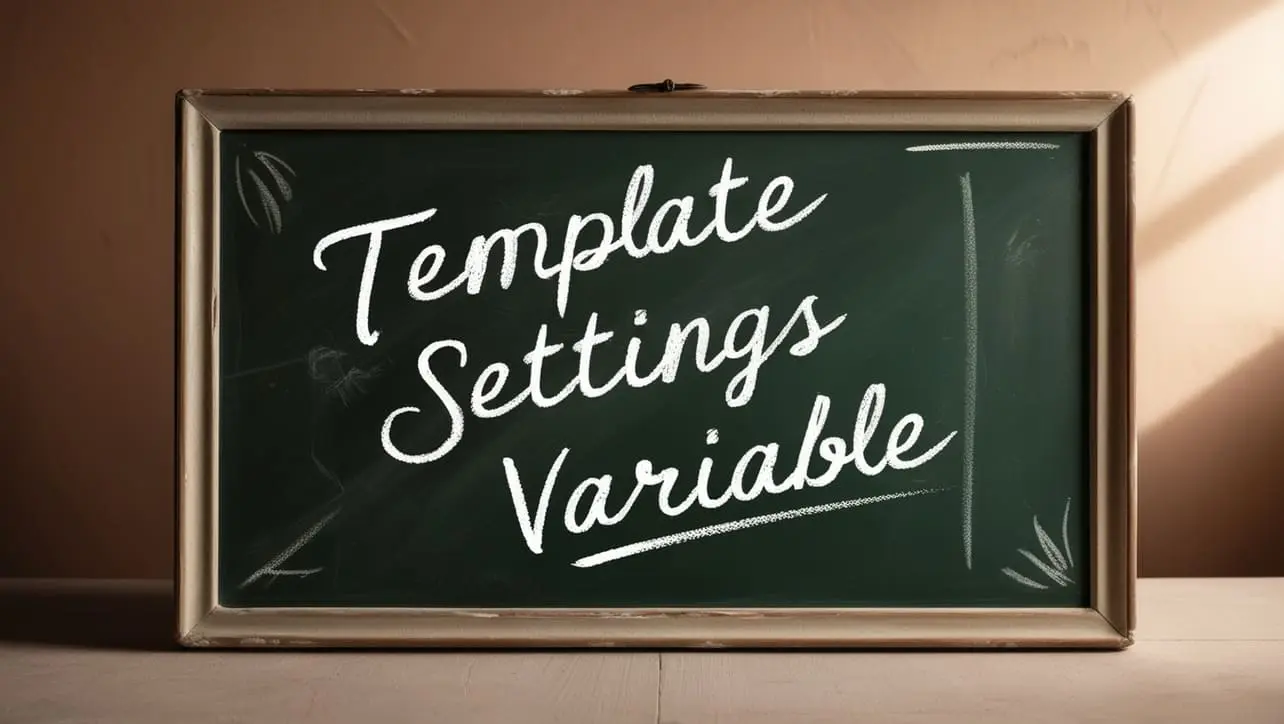
If you have any doubts regarding this article (Lodash _.isArray() Lang Method), please comment here. I will help you immediately.