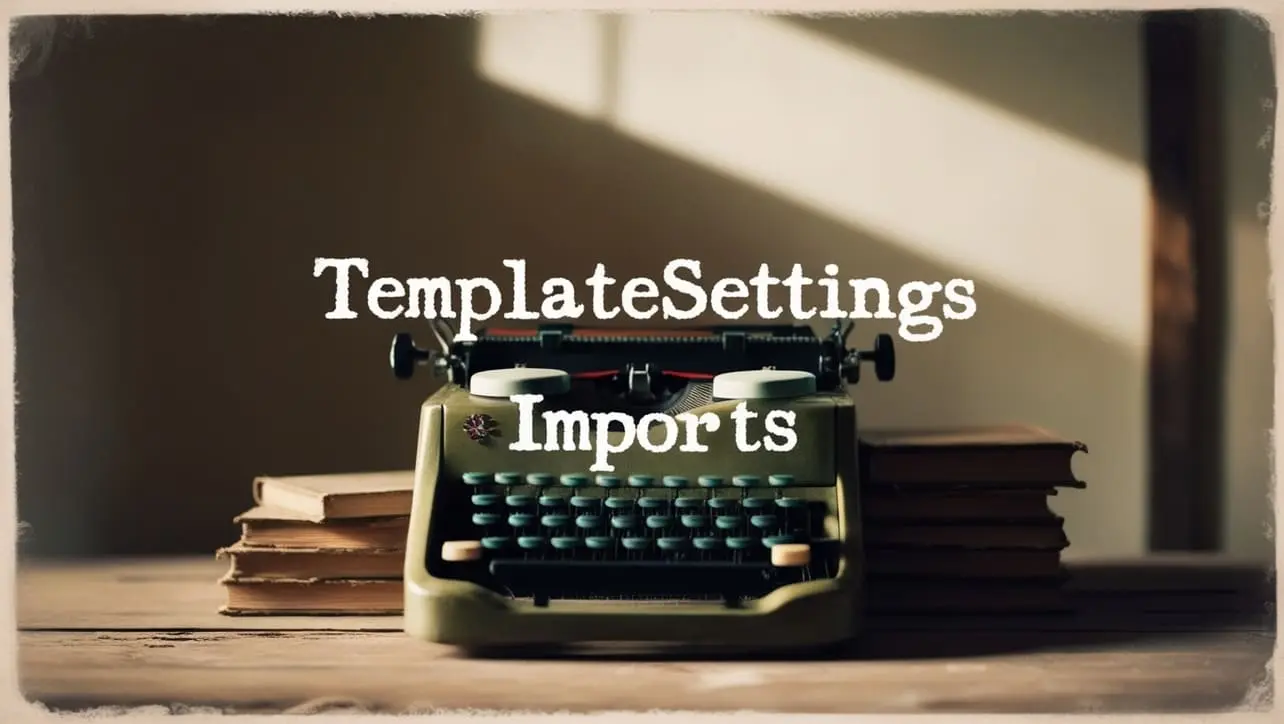
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.gt() Lang Method
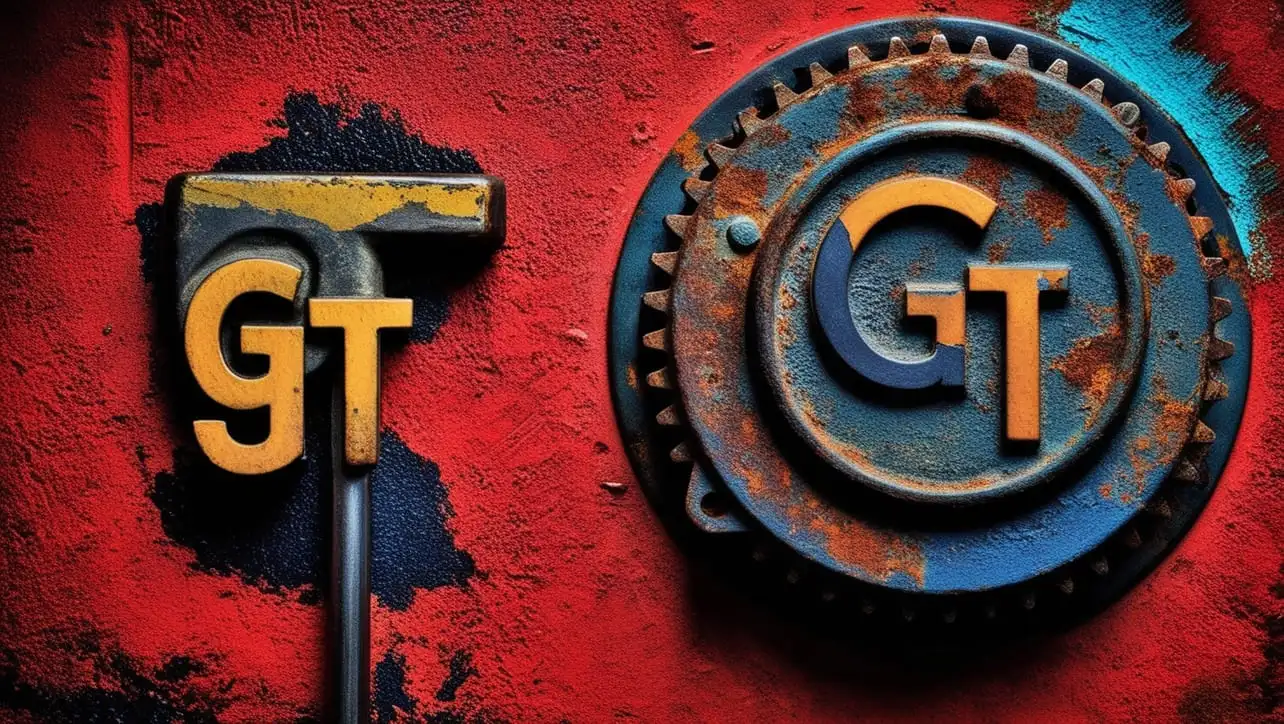
Photo Credit to CodeToFun
🙋 Introduction
In the diverse landscape of JavaScript programming, Lodash stands out as a comprehensive utility library, offering an array of functions to simplify and enhance development. Among these functions is the _.gt()
Lang method, providing a straightforward means to compare values using the greater-than (>) operator.
This method empowers developers with a convenient tool for numeric and string comparisons, streamlining conditional logic in their code.
🧠 Understanding _.gt() Method
The _.gt()
method in Lodash falls under the Lang category, specifically designed for language-related operations. This particular method focuses on the greater-than comparison, allowing developers to easily check if one value is greater than another.
💡 Syntax
The syntax for the _.gt()
method is straightforward:
_.gt(value, other)
- value: The first value to compare.
- other: The second value to compare.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.gt()
method:
const _ = require('lodash');
const result = _.gt(5, 3);
console.log(result);
// Output: true
In this example, the _.gt()
method compares the values 5 and 3 using the greater-than operator, resulting in true.
🏆 Best Practices
When working with the _.gt()
method, consider the following best practices:
Type-Agnostic Comparison:
The
_.gt()
method performs a type-agnostic comparison, meaning it can handle comparisons between different types, such as numbers and strings. Be mindful of the types you are comparing to avoid unexpected results.example.jsCopiedconst numericResult = _.gt(10, '5'); console.log(numericResult); // Output: true (numeric comparison) const stringResult = _.gt('apple', 'banana'); console.log(stringResult); // Output: false (lexicographical comparison)
NaN Handling:
When comparing values that may result in NaN (Not-a-Number), be aware that
_.gt()
considers NaN to be greater than any other value, including itself.example.jsCopiedconst nanResult = _.gt(NaN, 42); console.log(nanResult); // Output: true
Use in Conditional Statements:
Integrate
_.gt()
into conditional statements to enhance the readability of your code, especially when dealing with complex comparisons.example.jsCopiedconst temperature = 25; if (_.gt(temperature, 20)) { console.log('It\'s a warm day!'); } else { console.log('The weather is cool.'); }
📚 Use Cases
Numeric Value Comparison:
_.gt()
is particularly useful when comparing numeric values, simplifying conditional checks based on magnitude.example.jsCopiedconst userScore = 120; const passingScore = 100; if (_.gt(userScore, passingScore)) { console.log('Congratulations! You passed the exam.'); } else { console.log('Unfortunately, you did not meet the passing score.'); }
String Lexicographical Comparison:
Beyond numeric values,
_.gt()
can be applied to strings for lexicographical comparisons, determining which string comes later in dictionary order.example.jsCopiedconst firstWord = 'apple'; const secondWord = 'banana'; if (_.gt(secondWord, firstWord)) { console.log(`${secondWord} comes after ${firstWord} in dictionary order.`); } else { console.log(`${firstWord} comes after ${secondWord} in dictionary order.`); }
Conditional Rendering in UI:
In user interface development, you can leverage
_.gt()
for conditional rendering based on values such as screen width or user input.example.jsCopiedconst screenWidth = /* ...fetch screen width... */ ; if (_.gt(screenWidth, 768)) { // Render desktop layout } else { // Render mobile layout }
🎉 Conclusion
The _.gt()
Lang method in Lodash provides a concise and versatile solution for performing greater-than comparisons in JavaScript. Whether you're dealing with numeric values, strings, or implementing conditional logic in your applications, this method offers a straightforward way to handle comparisons.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.gt()
method in your Lodash projects.
👨💻 Join our Community:
Author
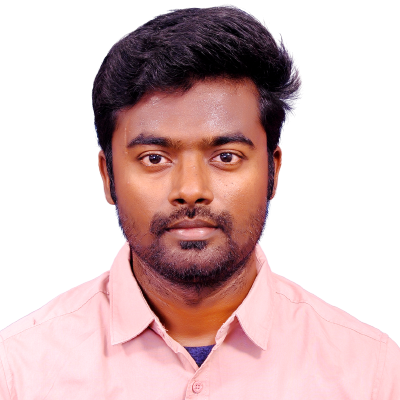
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
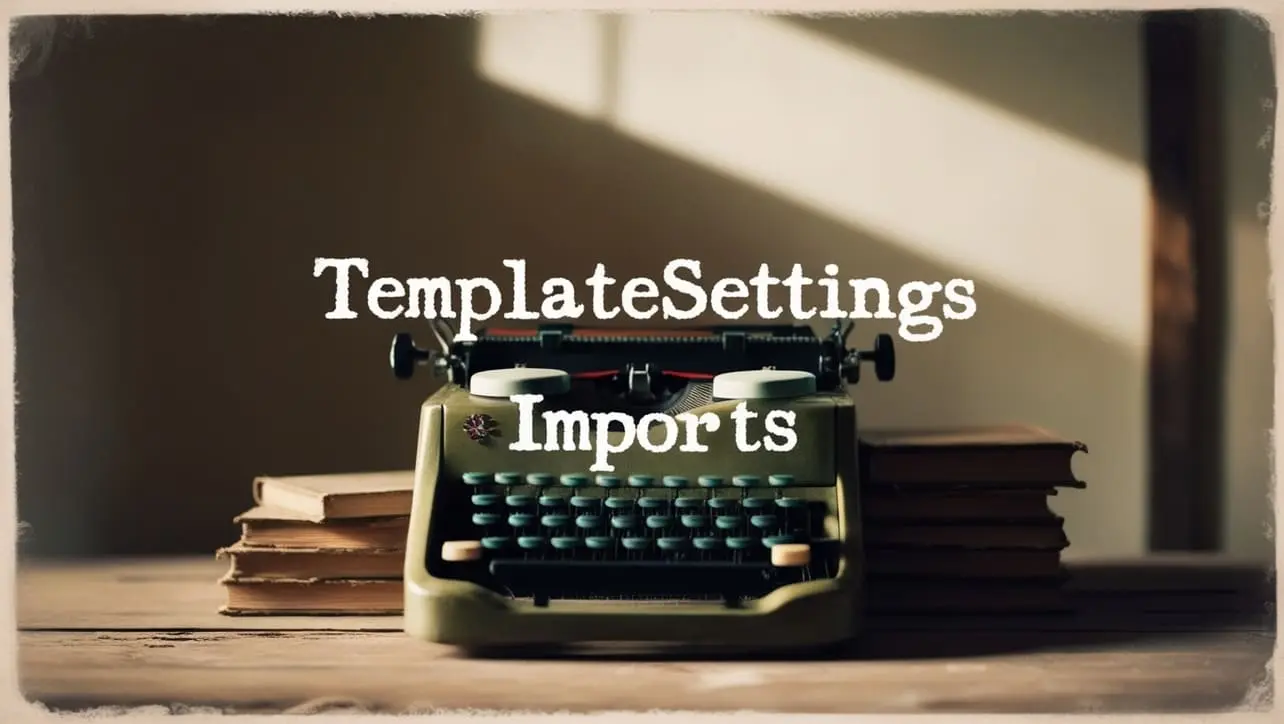
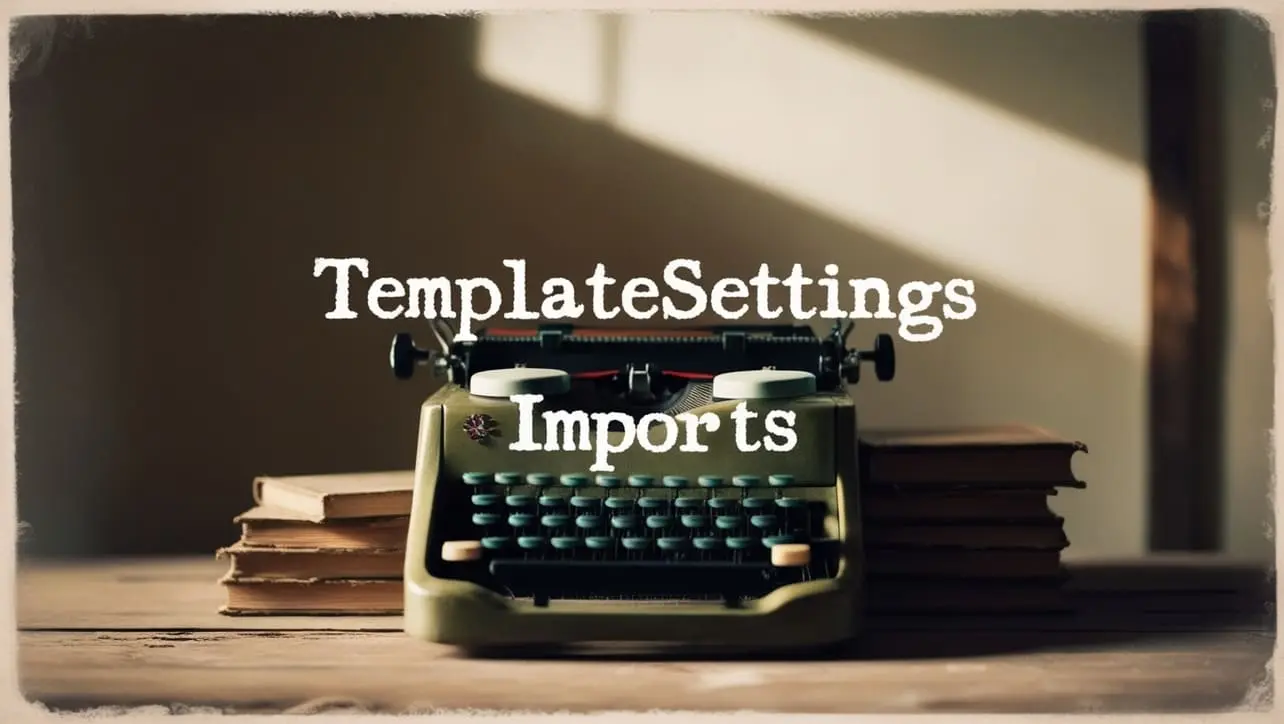
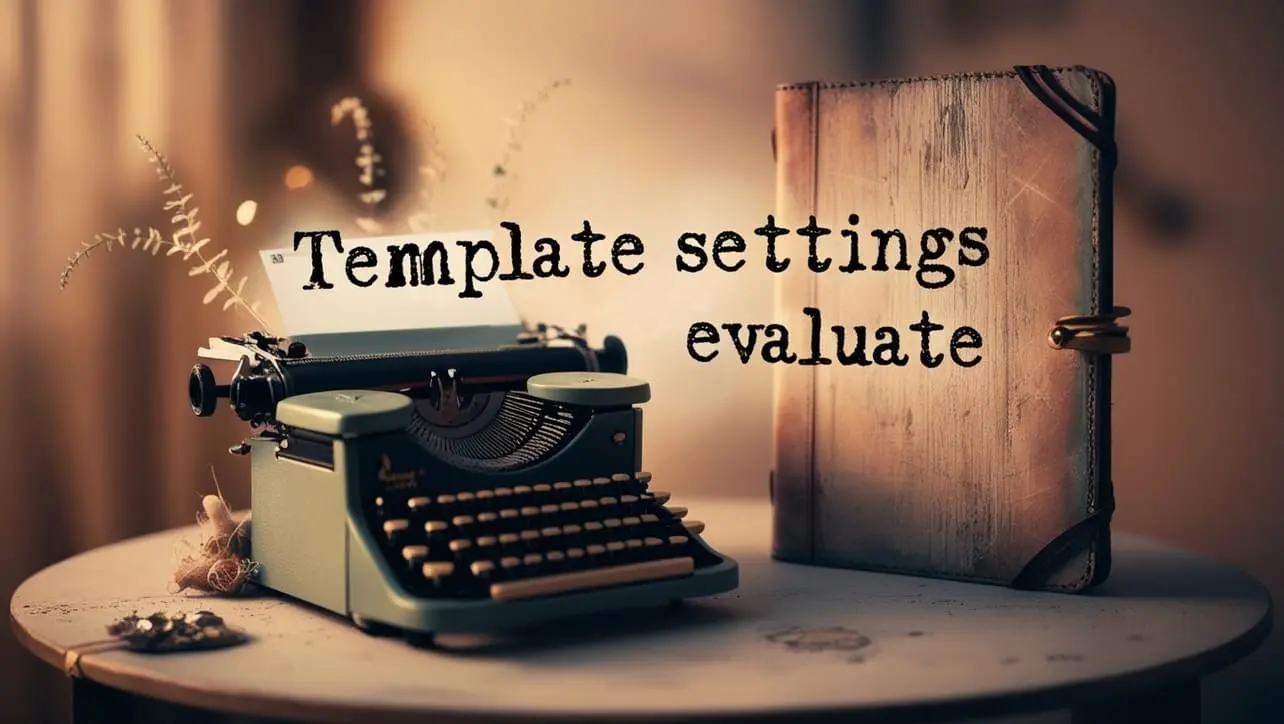
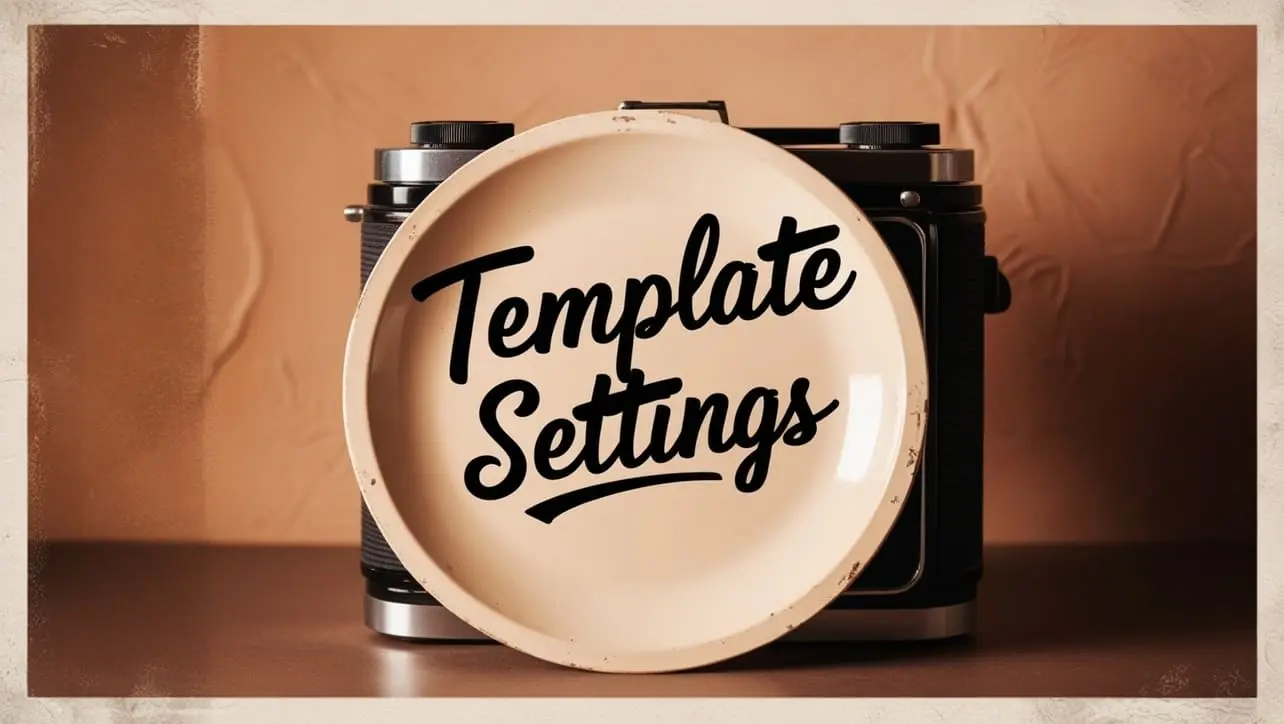
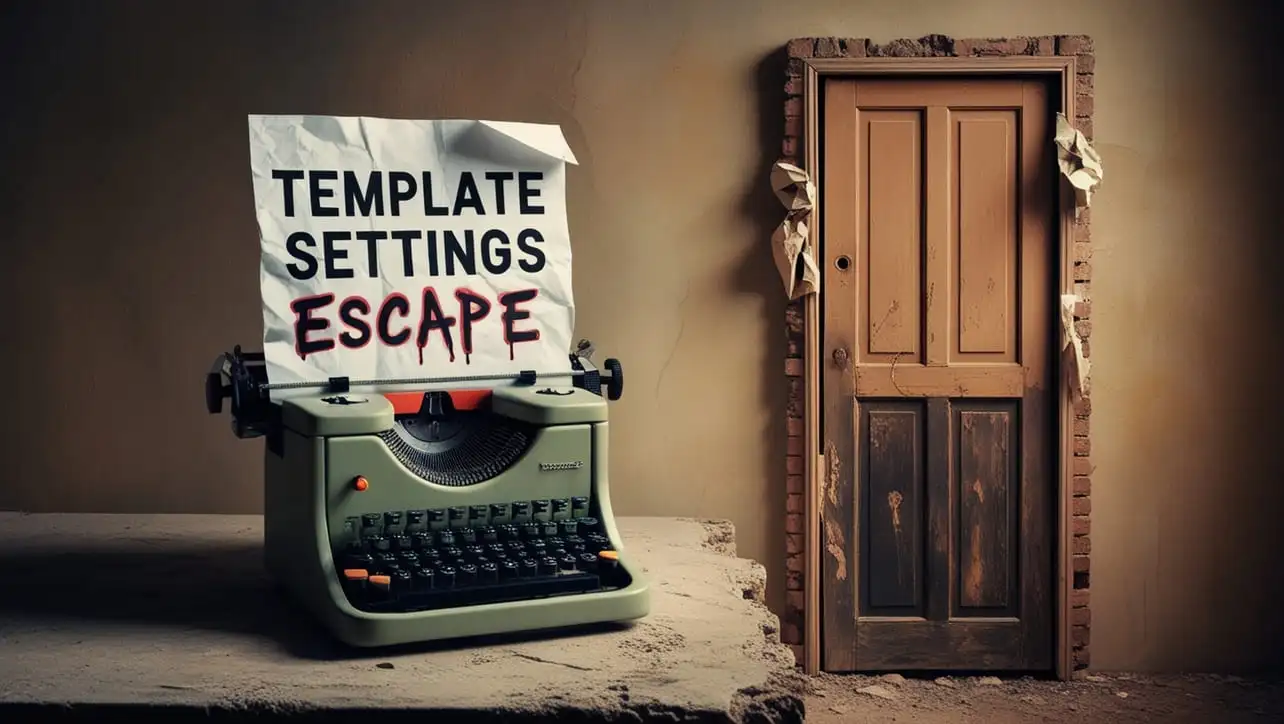
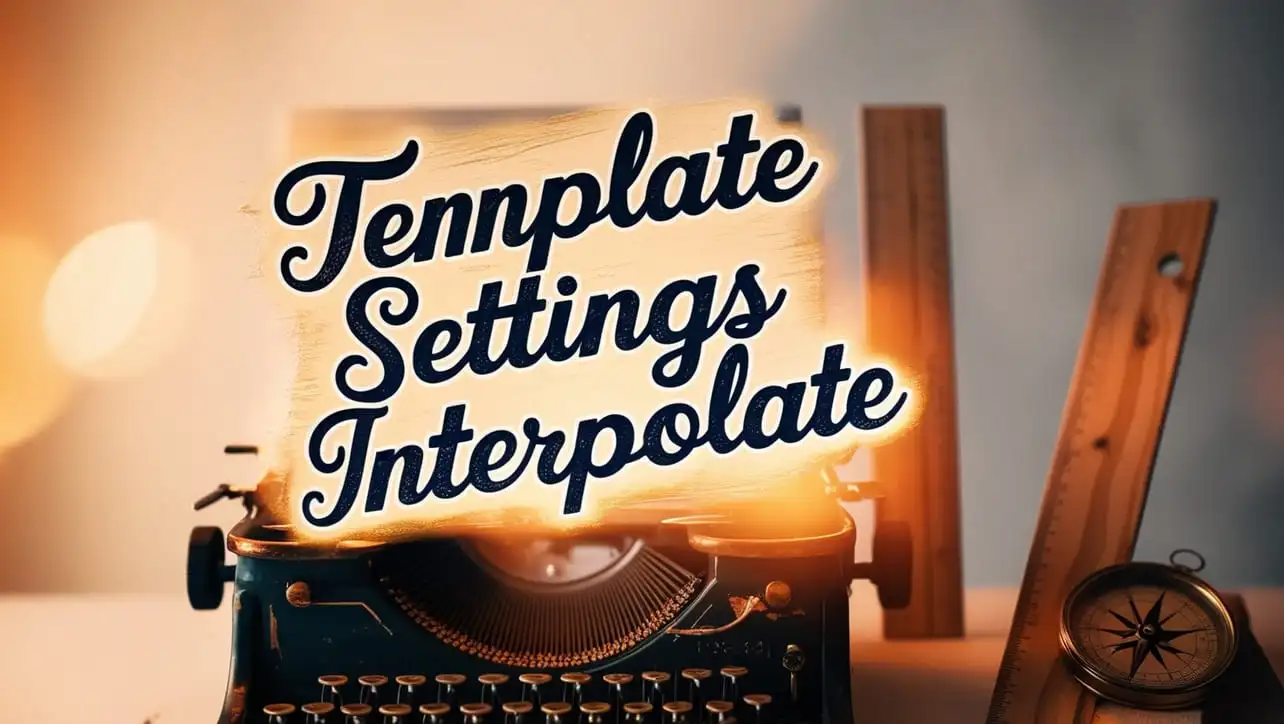
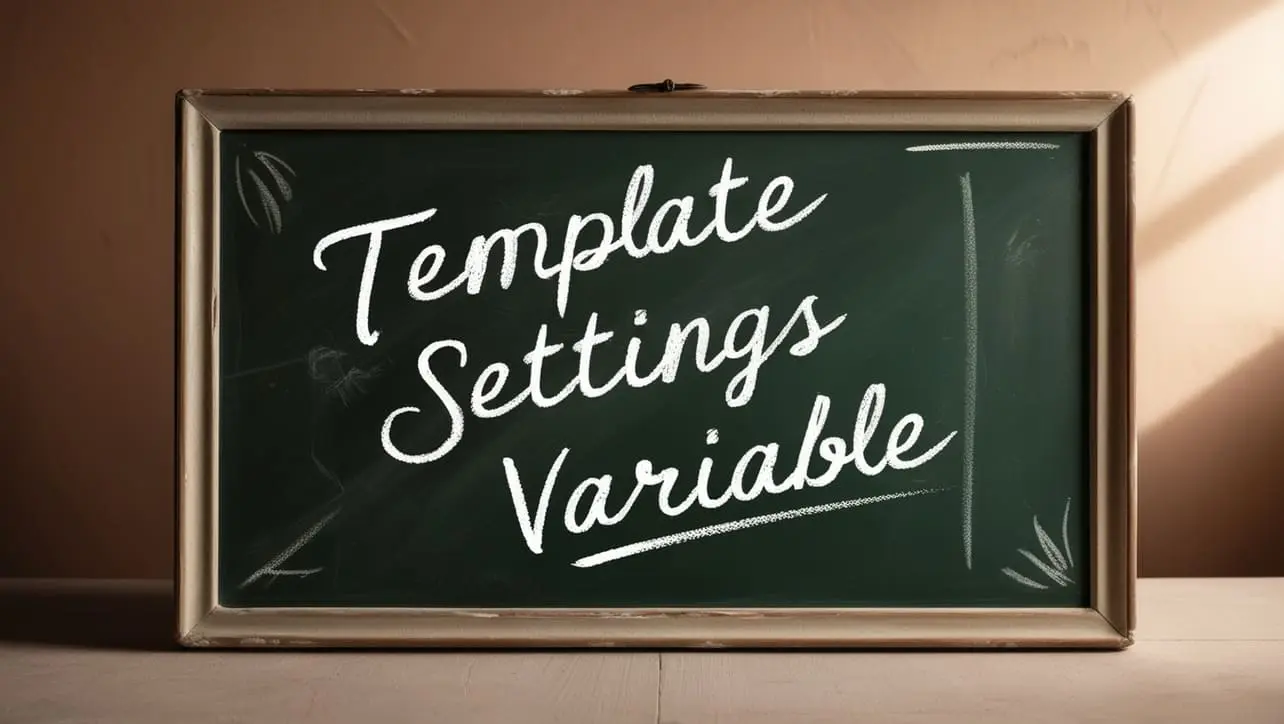
If you have any doubts regarding this article (Lodash _.gt() Lang Method), please comment here. I will help you immediately.